Accelerated C++ is a programming book that focuses on teaching the C++ language through practical examples and a hands-on approach, enabling learners to quickly write useful programs.
Here's a simple code snippet demonstrating the use of vectors in C++:
#include <iostream>
#include <vector>
int main() {
std::vector<int> numbers = {1, 2, 3, 4, 5};
for (int num : numbers) {
std::cout << num << " ";
}
return 0;
}
What is Accelerated C++?
Accelerated C++ is a highly regarded programming book authored by Andrew Koenig and Barbara E. Moo. This book serves as an essential guide for both beginners and experienced programmers looking to enhance their C++ skills through practical application. Its unique approach focuses on teaching readers how to effectively use C++ by exposing them to real-world examples right from the start.
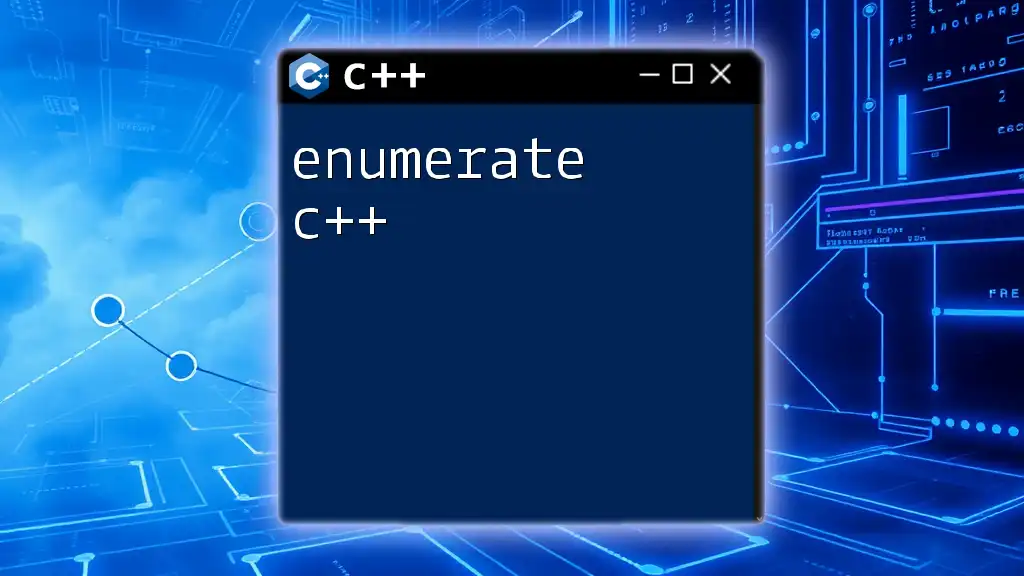
Why Choose Accelerated C++?
One of the main advantages of Accelerated C++ is its emphasis on practical learning. Unlike traditional C++ textbooks that often start with complex grammar and concepts, this book dives directly into programming tasks and projects.
By employing an example-driven approach, readers quickly gain confidence in writing C++ code and understanding its principles. This method nurtures critical thinking and problem-solving skills—essential attributes for any successful programmer.
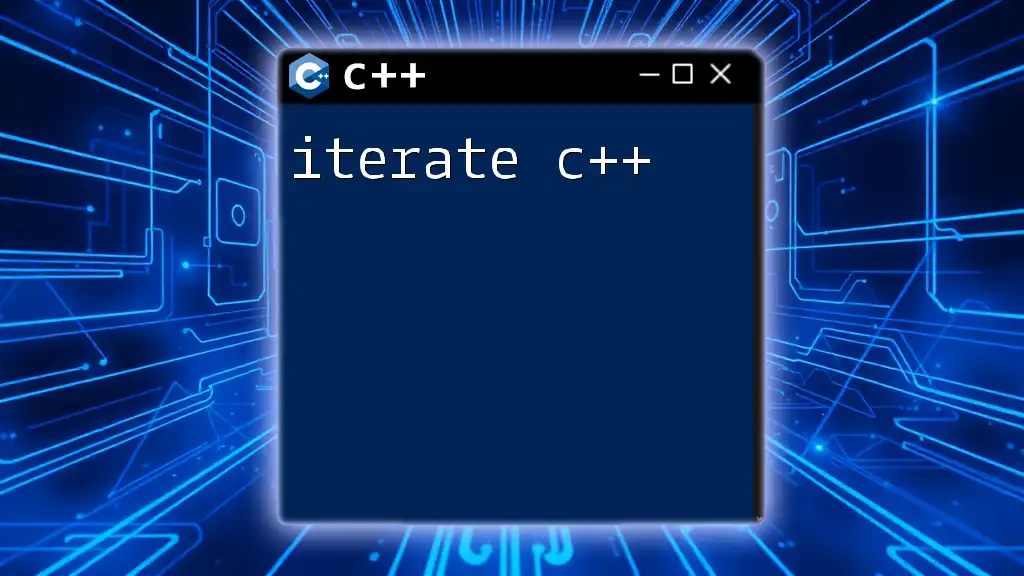
The Structure of Accelerated C++
The book is structured in a way that promotes gradual learning. Each chapter is meticulously designed to build upon the previous one, fostering a seamless learning curve.
The chapters introduce concepts through coding tasks, gradually increasing in complexity, and concluding with exercises that reinforce the learned material. This progressive layout encourages readers to practice coding and internalize concepts effectively.
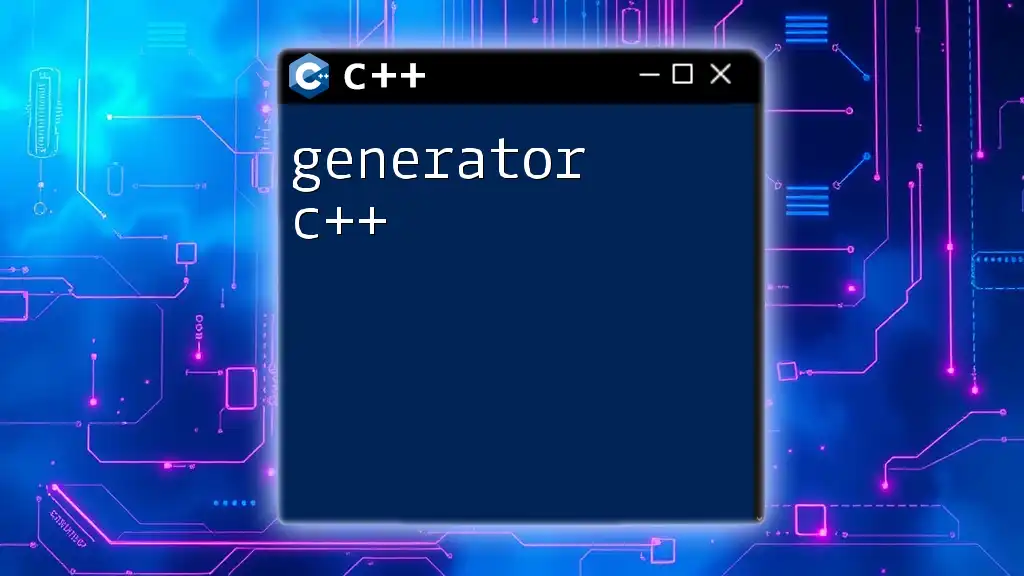
Understanding C++ Basics Quickly
Variables and Data Types
A strong foundation in C++ requires an understanding of variables and their data types. Variables act as storage locations for data, and knowing the different types helps determine how data is used and manipulated.
Key data types include:
- `int`: Represents integers.
- `float`: Represents floating-point numbers.
- `char`: Represents a single character.
Here’s a simple code snippet demonstrating variable declaration:
int main() {
int age = 30;
float height = 5.9;
char initial = 'A';
return 0;
}
Control Structures
Control structures are fundamental components that dictate the flow of a program. Common structures include conditional statements and loops.
Example of a simple loop:
for (int i = 0; i < 10; i++) {
std::cout << i << std::endl;
}
This loop effectively prints numbers from 0 to 9, demonstrating how control structures can manage the execution sequence in coding.
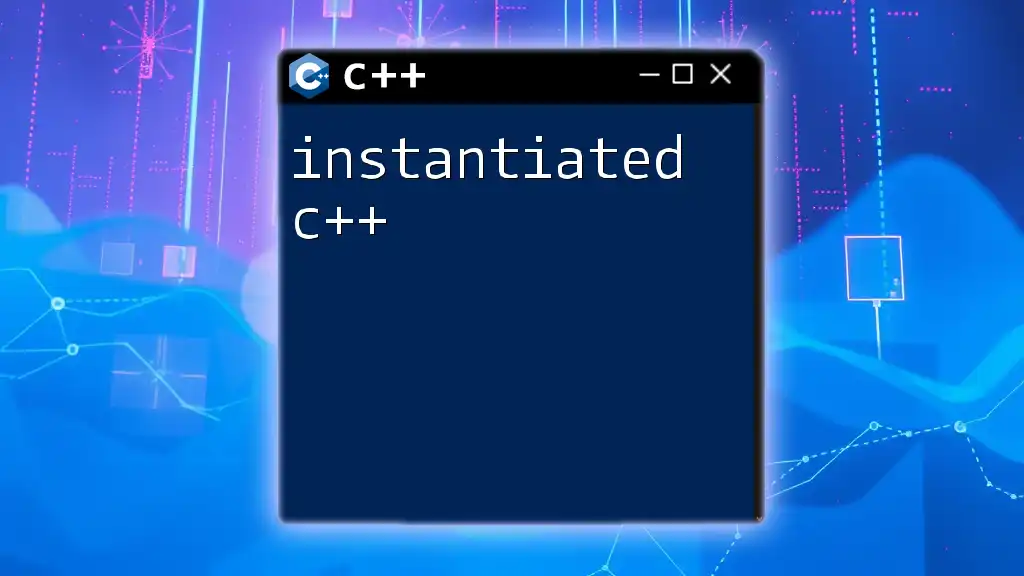
C++ Standard Library
Standard Template Library (STL)
The Standard Template Library (STL) provides a collection of template classes and functions for managing data structures and algorithms. It enables developers to write more efficient and maintainable code.
Introduction to Containers
STL includes several data structures known as containers—these include `vector`, `list`, `map`, and more.
Example of a vector declaration and initialization:
#include <vector>
std::vector<int> nums = {1, 2, 3, 4, 5};
Iterators and Algorithms
Iterators are tools that allow traversing elements in a container. Using iterators in conjunction with algorithms like sorting and searching simplifies complex operations.
Here’s a snippet demonstrating sorting a vector:
#include <algorithm>
std::sort(nums.begin(), nums.end());
The use of `std::sort` from the `<algorithm>` header efficiently arranges elements in ascending order.
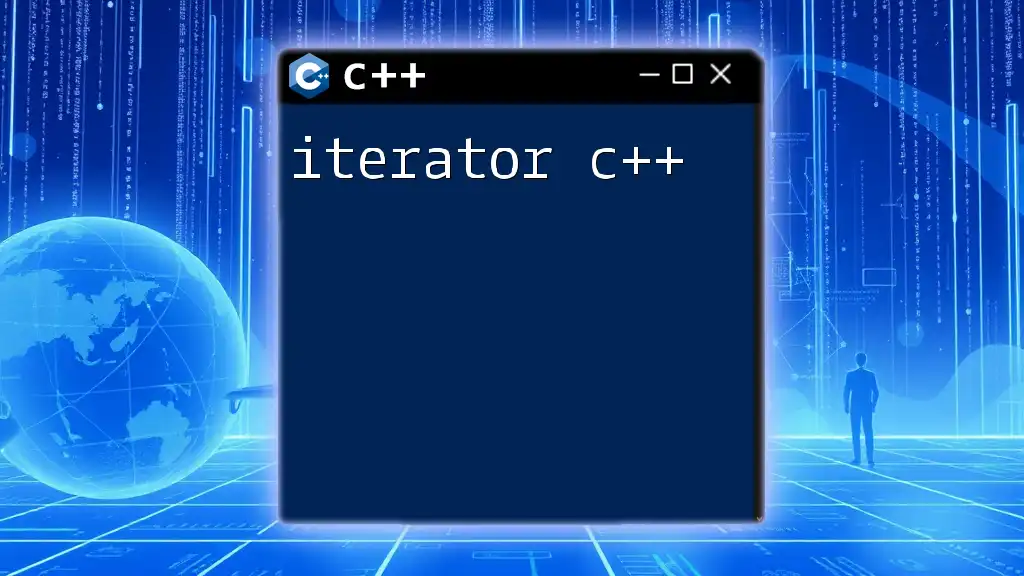
Object-Oriented Programming in C++
Classes and Objects
C++ is object-oriented, meaning it supports encapsulation, inheritance, and polymorphism through classes and objects.
Example of a simple class definition:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
In this snippet, we define a `Dog` class with a member function that allows a dog to "bark."
Principles of OOP
Encapsulation
Encapsulation refers to bundling the data (attributes) and methods (functions) operating on the data within a single unit or class. This hides the internal object state and controls access through public interfaces.
Inheritance
Inheritance allows one class to inherit characteristics (attributes and methods) from another. For instance:
class Animal {};
class Cat : public Animal {};
This snippet shows that `Cat` inherits from `Animal`, enabling code reuse and hierarchy.
Polymorphism
Polymorphism allows for methods to do different things based on the object that it is acting upon, enhancing flexibility in code design.
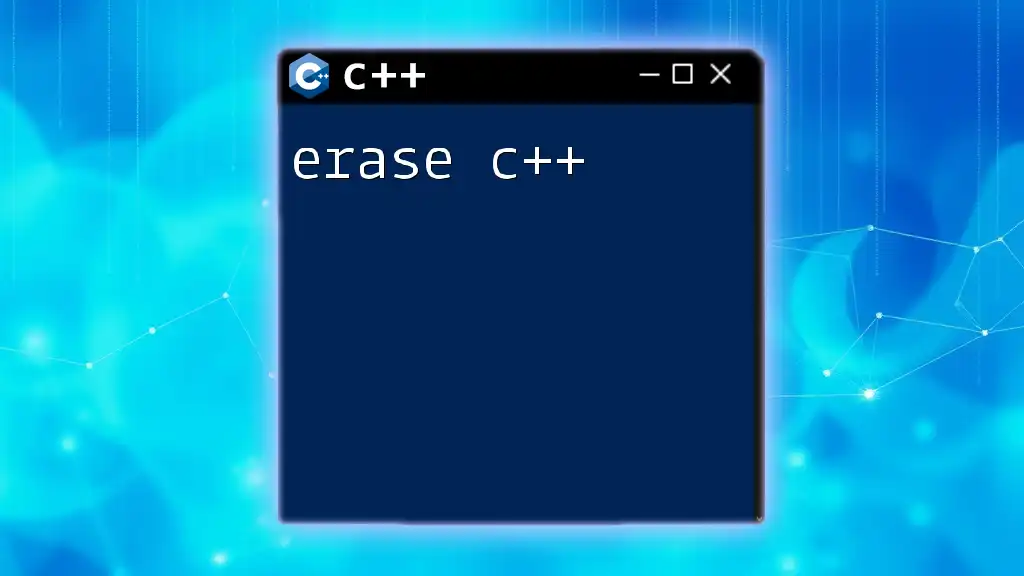
Practical Applications of C++
C++ is widely used to create various real-world applications—from software development to game programming. Understanding the basics from Accelerated C++ empowers learners to conceptualize projects effectively.
Example projects include:
- A simple calculator
- A text-based game
- A file management system
Each of these projects can help consolidate knowledge and practice the fundamentals learned throughout the book.
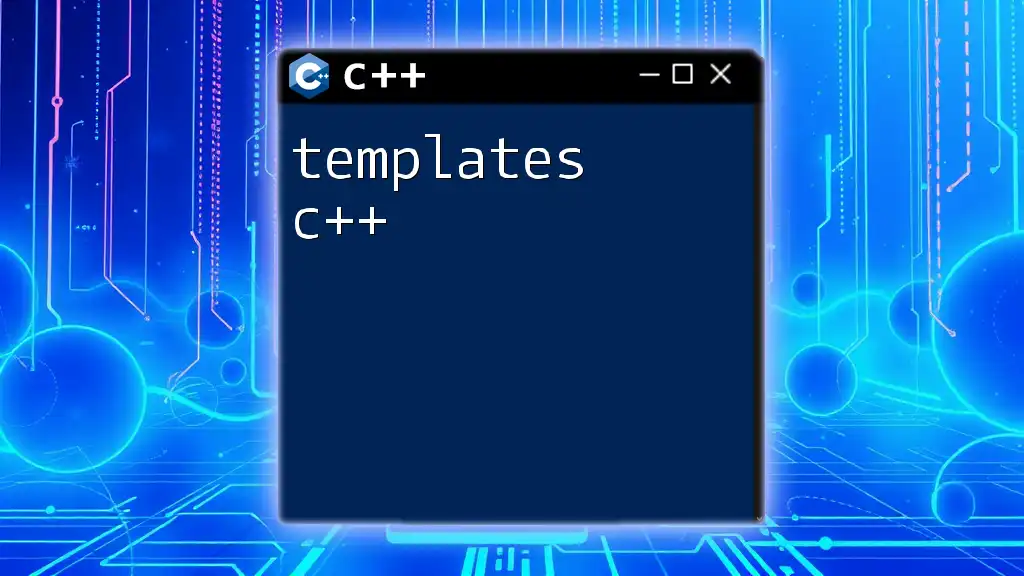
Best Practices in C++ Programming
Writing Clean and Efficient Code
Clean code is essential for readability and maintainability. Adopting meaningful variable names, commenting effectively, and organizing code will help ensure it remains understandable for others (and yourself) in the future.
Common Mistakes to Avoid
New C++ programmers often face challenges such as:
- Memory leaks
- Improper use of pointers
- Ignoring compiler warnings
Becoming familiar with these pitfalls will enhance coding efficiency and code quality.
![Effortless Memory Management: Delete[] in C++ Explained](/images/posts/d/delete-cpp.webp)
Resources for Continued Learning
To further your understanding of accelerated C++, numerous resources are available:
- Books: Titles such as "Effective C++" by Scott Meyers provide advanced insights.
- Online Courses: Platforms like Coursera and Udemy offer interactive C++ courses.
- Communities: Engaging with forums, chat rooms, and developer communities like Stack Overflow can provide valuable insights and support.
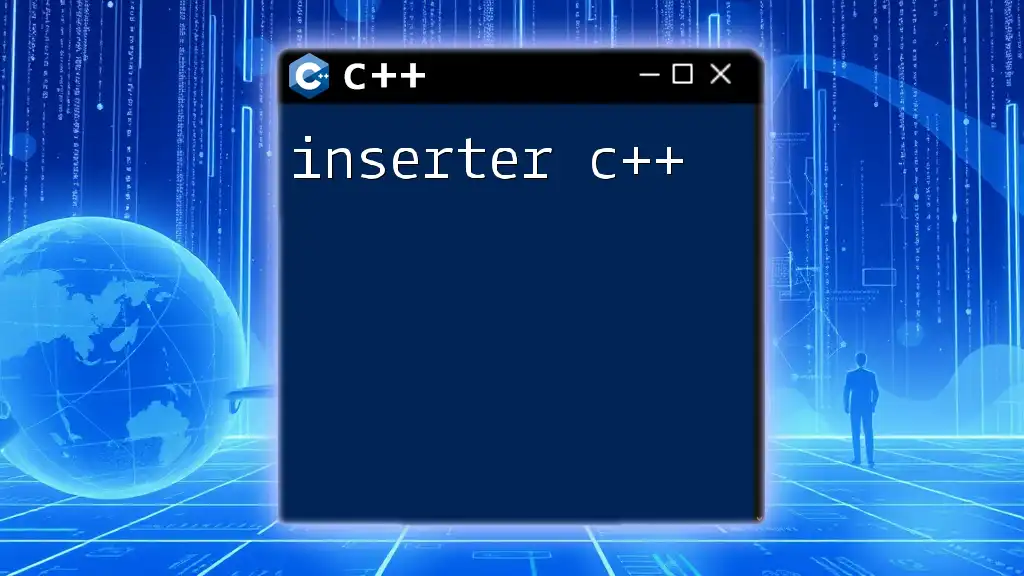
Conclusion
Accelerated C++ is a vital resource for anyone looking to speed up their learning process in C++. By employing hands-on techniques, readers transition from basic concepts to practical implementations swiftly. Engaging with real-world coding scenarios ensures a better grasp of the language and engineering practices, paving the way for a successful programming career.
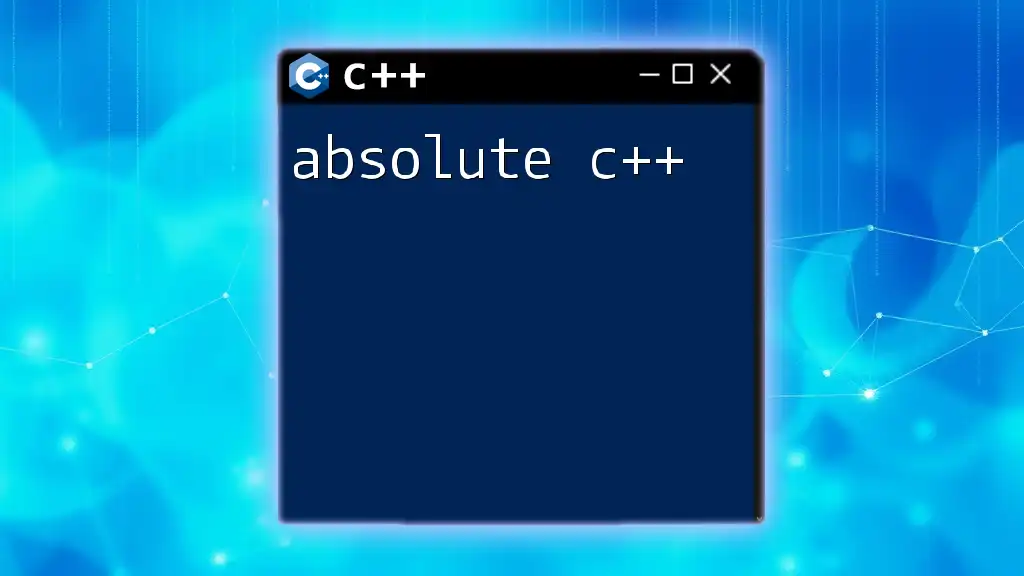
Code Snippets and Examples Repository
To bolster understanding, access a GitHub repository linked in this article, which contains all code examples discussed, along with additional projects to practice C++.
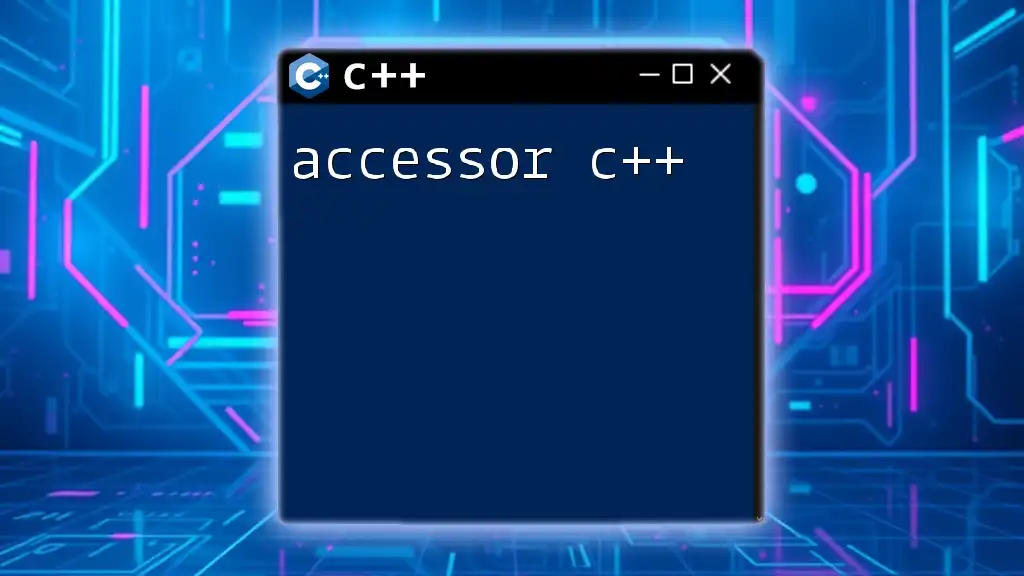
FAQs
Common Questions About Accelerated C++
What prior knowledge do I need before using Accelerated C++? Basic programming concepts can be helpful, but the book is suitable for complete beginners.
How long does it take to learn from the book? While it varies based on individual pace, many find they can grasp the fundamentals within a few weeks of dedicated study.
With this comprehensive guide on accelerated C++, readers can begin their journey toward mastering a powerful programming language and applying it effectively in various domains.