Instantiation in C++ refers to the creation of an object from a class, allowing you to use its attributes and methods in your program.
Here's a code snippet demonstrating how to instantiate an object of a class:
class Dog {
public:
void bark() {
std::cout << "Woof!" << std::endl;
}
};
int main() {
Dog myDog; // Instantiation of Dog class
myDog.bark(); // Calling a method on the instantiated object
return 0;
}
Understanding Instantiation in C++
What is Instantiation?
Instantiation in programming refers to the process of creating an instance or object from a class. In C++, instantiation is a fundamental concept, as it enables developers to work with classes, encapsulating both data and functionality. The ability to instantiate objects is crucial for implementing Object-Oriented Programming (OOP) principles effectively. It allows you to create reusable components that can interact with one another.
How Instantiation Works in C++
When an object is instantiated in C++, there are several critical steps involved. First, memory is allocated for the object, which involves invoking the constructor defined in the class. This constructor initializes the object's attributes, ensuring that it is ready for use. Understanding how instantiation works is essential for optimal memory management and performance in your C++ applications.
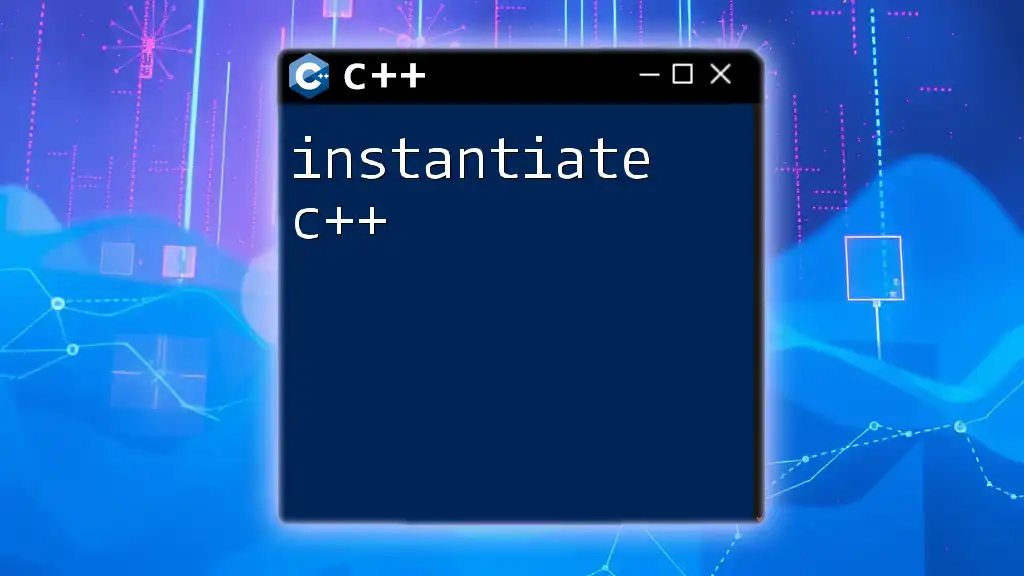
Types of Instantiation in C++
Static Instantiation
Static instantiation occurs when an object is created with automatic storage duration. This means that the object's memory is allocated on the stack and automatically deallocated when it goes out of scope. Static instantiation is straightforward and fast, making it a suitable option for many situations.
For example:
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
MyClass obj(5); // Static instantiation example
In this example, an object `obj` of class `MyClass` is instantiated with the value `5`, and its memory is managed automatically.
Dynamic Instantiation
Dynamic instantiation, on the other hand, involves allocating memory for an object on the heap using the `new` keyword. This method allows for more flexible memory management but requires careful handling to prevent memory leaks.
For example:
MyClass* obj = new MyClass(10); // Dynamic instantiation example
In this case, the object `obj` is dynamically created and initialized with the value `10`. Remember, when using dynamic instantiation, it's essential to properly manage memory by calling `delete` when the object is no longer needed to release the allocated memory.
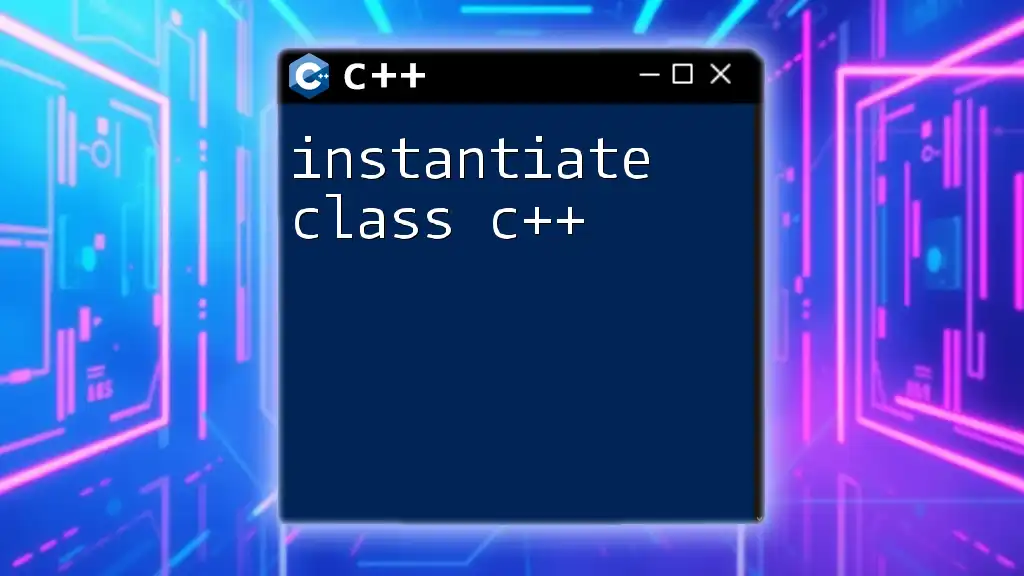
Different Ways to Instantiate Objects
Using Default Constructors
A default constructor is a constructor that takes no parameters. This type of instantiation is useful for creating objects when specific initial values are not necessary.
For instance:
class MyClass {
public:
MyClass() {
// Default constructor
}
};
MyClass obj; // Instantiation with default constructor
Here, the object `obj` is instantiated using the default constructor, and no initial values are provided.
Using Parameterized Constructors
Parameterized constructors allow you to pass values to an object upon instantiation, enabling more tailored and effective object creation.
Consider this example:
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
};
MyClass obj(20); // Parameterized constructor example
In this case, the object `obj` is instantiated with a value of `20`, allowing the object to be initialized with meaningful data right from creation.
Using Copy Constructors
Copy constructors create a new object as a copy of an existing object. They play a crucial role in managing resources effectively, especially with dynamic memory.
Here's how it works:
class MyClass {
public:
int value;
MyClass(int v) : value(v) {}
MyClass(const MyClass &obj) {
value = obj.value; // Copy constructor
}
};
MyClass obj1(30);
MyClass obj2(obj1); // Copy instantiation example
In this example, `obj2` is instantiated as a copy of `obj1`, utilizing the copy constructor, which ensures that both objects have their own separate memory even though their values are initially the same.
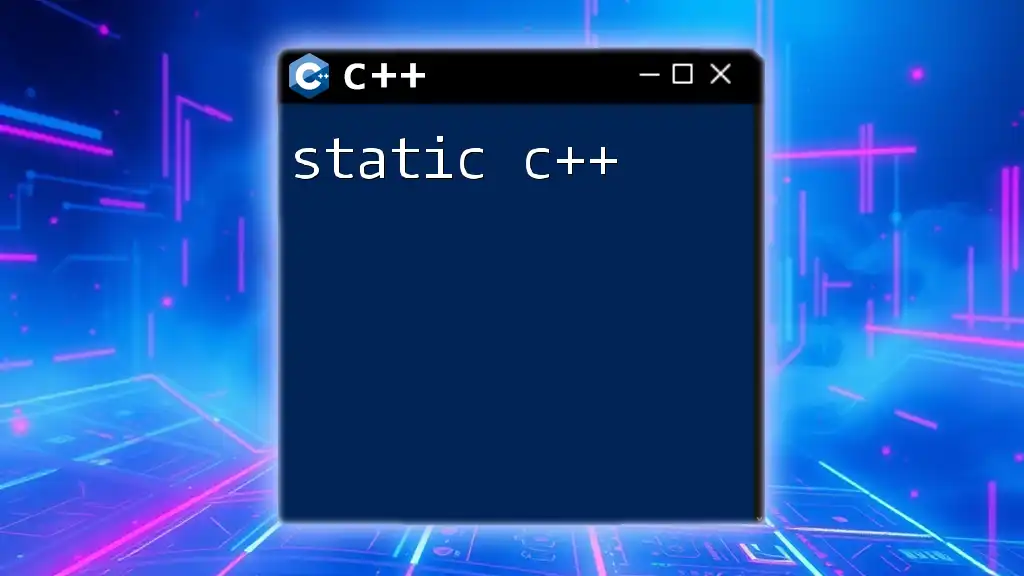
Template Instantiation
What is Template Instantiation?
Templates in C++ allow you to write generic and reusable code. Template instantiation refers to the process by which the compiler creates specific versions of a template class or function, based on the types provided during instantiation. This capability enhances code flexibility and reusability.
How to Use Templates in Instantiation
You can instantiate templates by providing specific types to general templates, allowing you to create objects that are type-safe tailored to your needs.
Here’s an example of template instantiation:
template<typename T>
class MyClass {
public:
T value;
MyClass(T v) : value(v) {}
};
MyClass<int> obj1(50); // Template instantiation example with int
MyClass<std::string> obj2("Hello"); // Template instantiation with string
In this example, `obj1` is instantiated with an integer type, while `obj2` uses a string type, demonstrating how templates can be specialized for different data types.
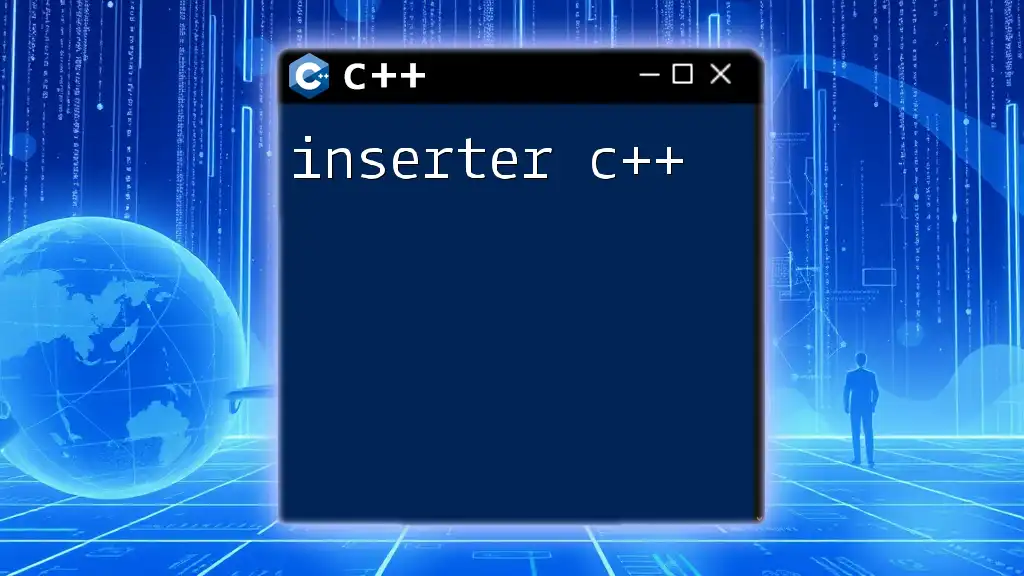
Common Issues with Instantiation
Undefined Behavior
A common pitfall in C++ programming is undefined behavior, which can occur during instantiation if you access an uninitialized object or if your code violates language rules. Always initialize your objects correctly to avoid ambiguities and unexpected crashes.
Memory Leaks
Memory leaks frequently occur in dynamic instantiation when allocated memory is not released. Use smart pointers, like `std::unique_ptr` or `std::shared_ptr`, to manage memory automatically and reduce the risk of leaks.
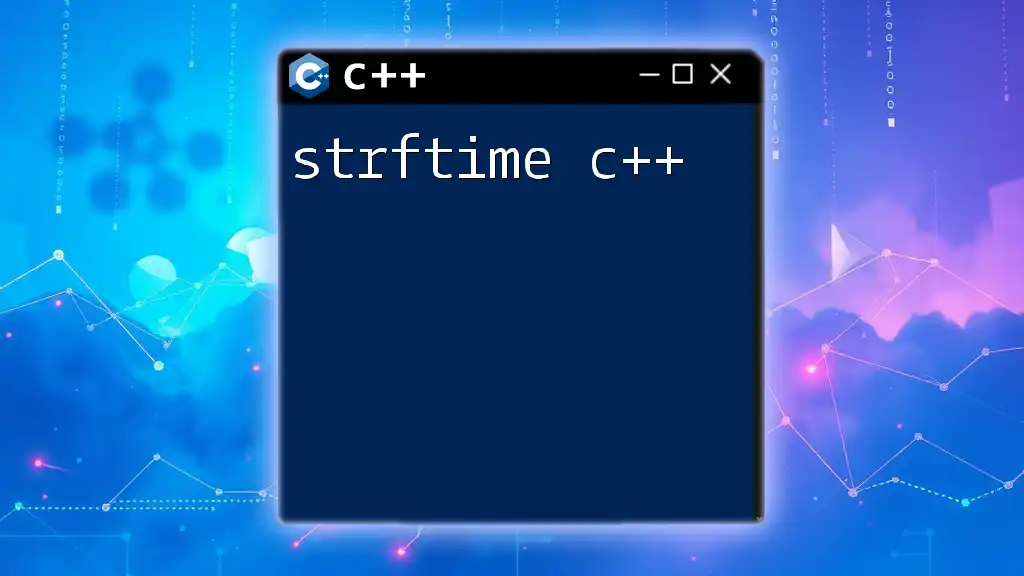
Best Practices for Instantiation
Choosing the Right Instantiation Method
Selecting between static and dynamic instantiation depends on your application's needs. Static instantiation is generally quicker and simpler, while dynamic instantiation provides necessary flexibility for managing lifetimes of objects, particularly in larger applications.
Constructor Initialization Lists
Initialization lists in constructors provide a more efficient way to initialize objects. They ensure that members are initialized before the constructor body executes, helping prevent redundant assignments.
For example:
class MyClass {
public:
int x, y;
MyClass(int a, int b) : x(a), y(b) {} // Initialization list
};
MyClass obj(5, 10);
In this example, `x` and `y` are initialized using the initialization list, making the process faster and more efficient.
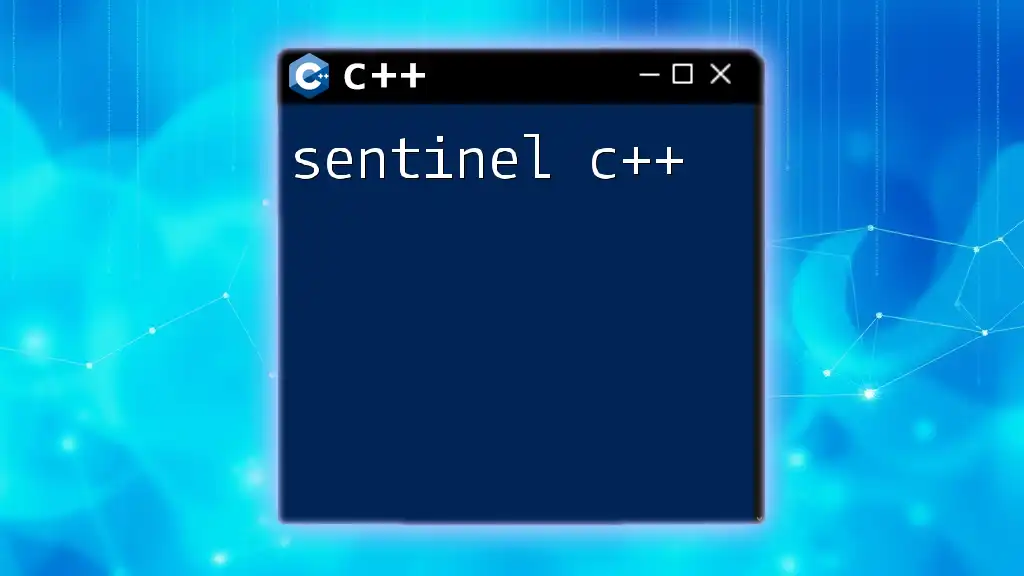
Conclusion
Recap of Key Points
In summary, instantiation in C++ is a multi-faceted concept that encompasses various techniques and practices, from static and dynamic approaches to using constructors and templates. Understanding these principles is fundamental to mastering C++ and developing robust applications.
Further Reading and Resources
To dive deeper into the concept of instantiated C++, consider exploring advanced topics such as memory management, smart pointers, and object-oriented design patterns. Continuous learning will not only enhance your skills but also enable you to write better, more efficient code.
Call to Action
Now that you have a comprehensive understanding of instantiated C++, it's time to put these principles into practice. Experiment with creating your own classes and instantiate them using different methods. Share your experiences and reach out if you have questions or insights!