C++ templates enable the creation of generic functions and classes that can operate with any data type, promoting code reusability and type safety.
#include <iostream>
using namespace std;
// Function template
template <typename T>
T add(T a, T b) {
return a + b;
}
int main() {
cout << add<int>(5, 3) << endl; // Output: 8
cout << add<double>(5.5, 2.3) << endl; // Output: 7.8
return 0;
}
Understanding Templates in C++
Templates in C++ provide a powerful mechanism for writing generic and type-safe code. They allow you to define functions and classes with placeholders for types, enabling better code reuse and flexibility. One of the primary motivations for using templates is to reduce code duplication, leading to more maintainable and scalable codebases.
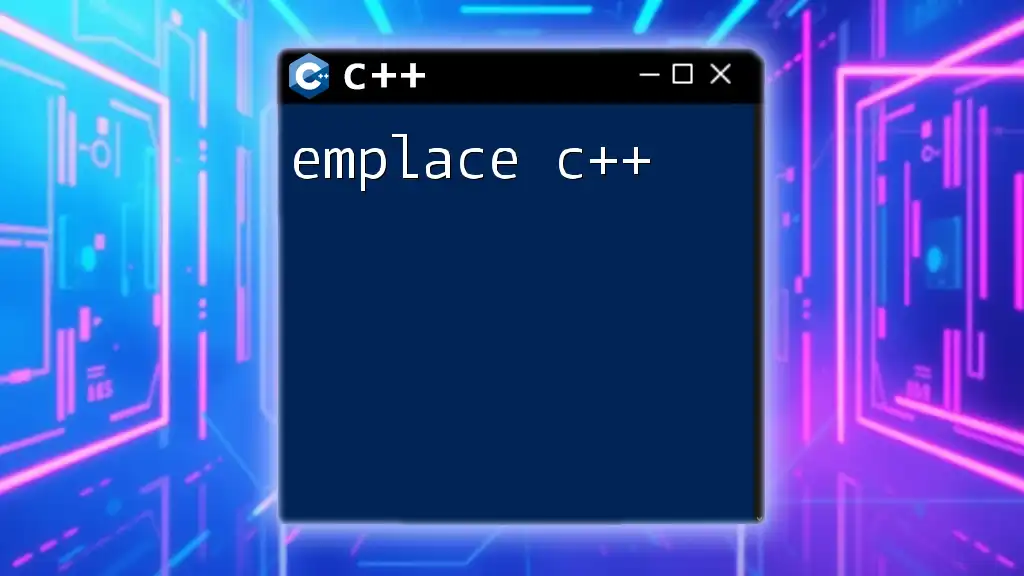
Why Use C++ Templates?
Utilizing C++ templates comes with several distinct advantages:
- Type Safety: Templates ensure that type mismatches are caught at compile time, reducing runtime errors and enhancing reliability.
- Performance: Templates can optimize performance by allowing for inline expansions, meaning that code specific to a type can be executed without the overhead associated with function calls.
- Reducing Code Duplication: A single template implementation can work with any data type, eliminating the need for multiple overloaded functions or class definitions.
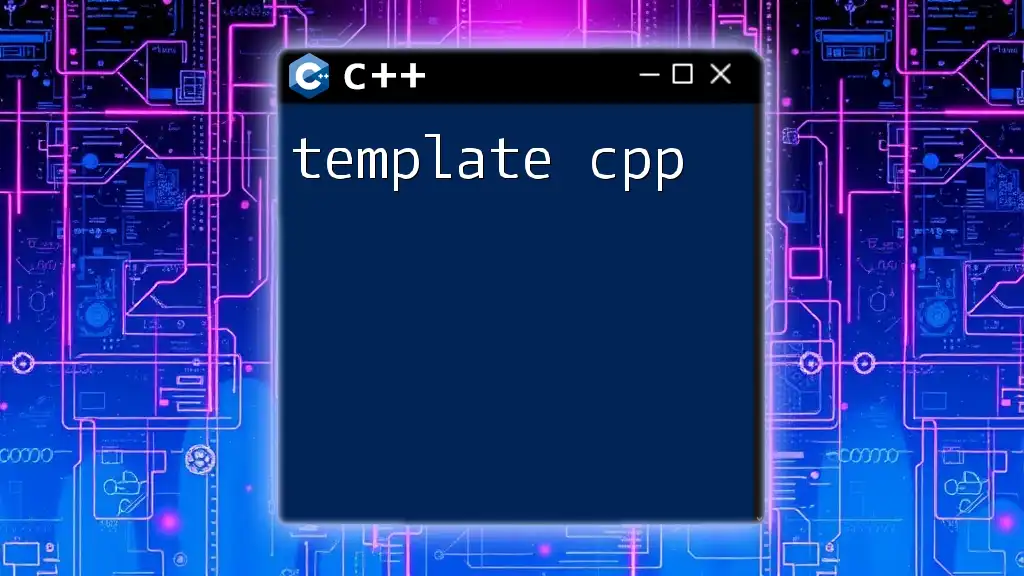
Types of C++ Templates
Function Templates
Function templates allow you to create a function that can operate on different data types without repeating code.
What are Function Templates?
A function template is defined using the `template` keyword, followed by template parameters, typically enclosed in angle brackets. The function itself has the same syntax as a regular function but utilizes the template parameters as types.
Example of a Function Template
Here's a simple example to demonstrate:
template<typename T>
T add(T a, T b) {
return a + b;
}
In this example, the `add` function takes two parameters of type `T`, which will be defined when the function is instantiated. This allows `add` to work with `int`, `double`, or any other data type that supports the `+` operator.
Class Templates
Class templates allow you to create classes that can handle different data types.
Definition of Class Templates
Like function templates, class templates utilize the `template` keyword. They enable you to define a class blueprint that can be instanced with various types.
Example of a Class Template
Consider the following:
template<typename T>
class Box {
public:
T value;
Box(T v) : value(v) {}
};
In this example, the `Box` class is designed to hold a value of type `T`. The constructor accepts a value of type `T`, demonstrating how class templates can store and manipulate different data types.
Variadic Templates
Variadic templates enable templates to accept an arbitrary number of template parameters.
What are Variadic Templates?
Variadic templates allow you to define templates that can take any number of parameters. This feature is particularly useful for functions or classes that need to handle multiple data types without excessive overloads.
Example of a Variadic Template
Here's a practical example:
template<typename... Args>
void print(Args... args) {
(cout << ... << args) << endl;
}
In this example, `print` can accept any number of arguments, print them, and showcases the power of fold expressions introduced in C++17.
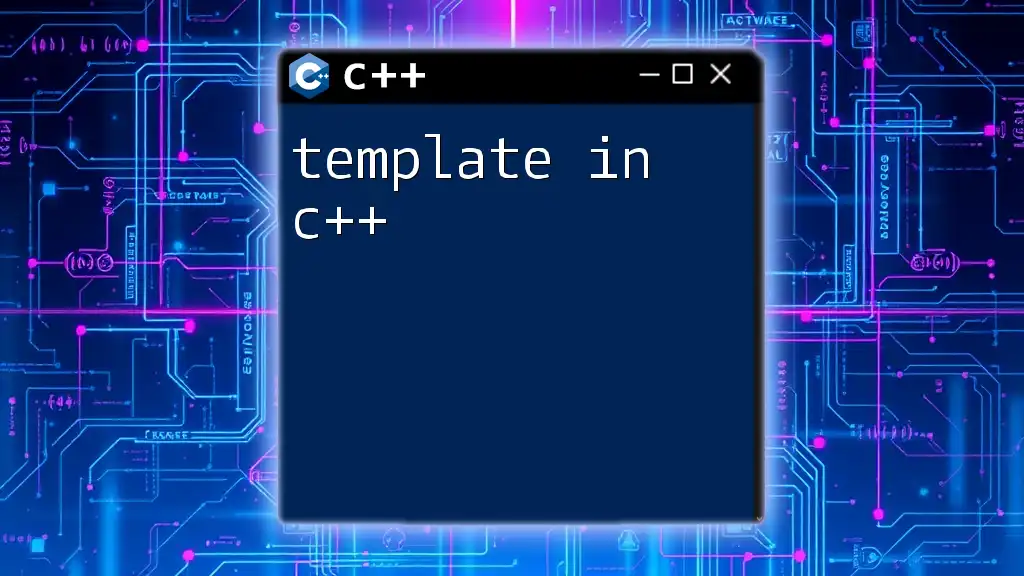
Advanced Concepts in C++ Templates
Template Specialization
Template specialization allows for customization of templates based on specific types.
Understanding Template Specialization
There are two main types of template specialization: full specialization, which provides a specific implementation for a concrete type, and partial specialization, which provides behavior for a subset of types.
Example of Template Specialization
Full specialization can be seen below:
template<>
class Box<int> {
public:
int value;
Box(int v) : value(v) {}
void display() { cout << "Integer Box: " << value << endl; }
};
In this code, we define a specialized version of `Box` for the `int` type. The `display` method is only relevant for this specialization, showcasing how you can tailor functionality for specific types.
SFINAE (Substitution Failure Is Not An Error)
SFINAE is a technique in templates that allows the compiler to ignore template instantiations that would fail due to substitution failure, rather than generating an error.
What is SFINAE?
This powerful feature can enable more complex template behaviors, such as conditional expressions based on type traits.
Example of SFINAE Use Case
Consider this example:
template<typename T>
typename std::enable_if<std::is_integral<T>::value, T>::type
square(T value) {
return value * value;
}
In this case, the function `square` will only be instantiated for integral types. If `T` is not an integral type, the function does not exist, effectively preventing incorrect usage of the function.
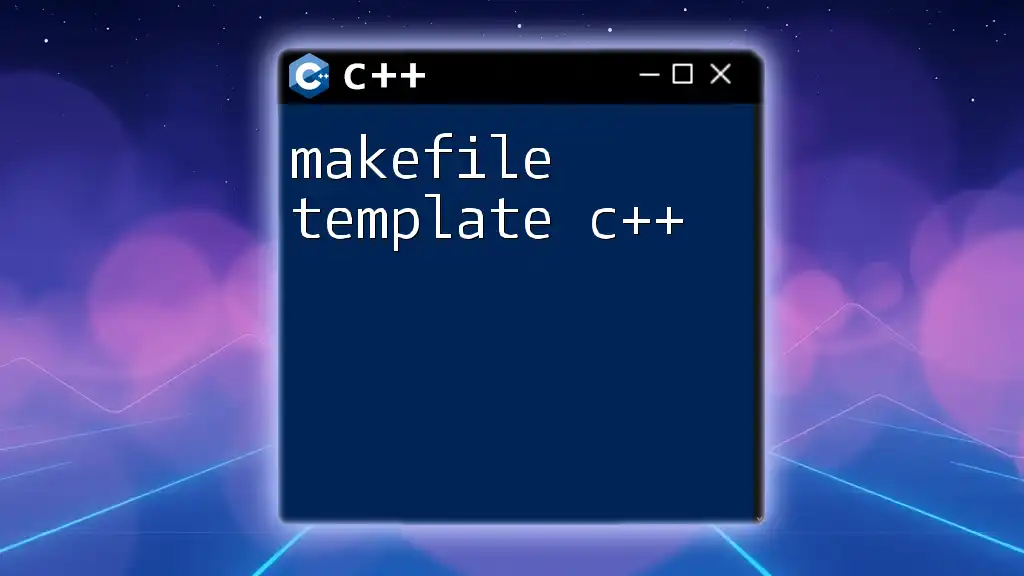
Best Practices for C++ Templates
Choosing Template Parameters Wisely
It is crucial to select template parameters carefully to optimize code usability and performance. Overusing templates can lead to confusion and overly complex code.
Template Code Organization
Organizing your template code for clarity and readability can make a big difference. Use descriptive names for template parameters and maintain consistent formatting to help others (and yourself) understand the code.
Documentation and Usage
Documenting your template functions and classes is vital. Providing clear examples and descriptive comments helps ensure that anyone using your template understands its purpose, usage, and limitations.
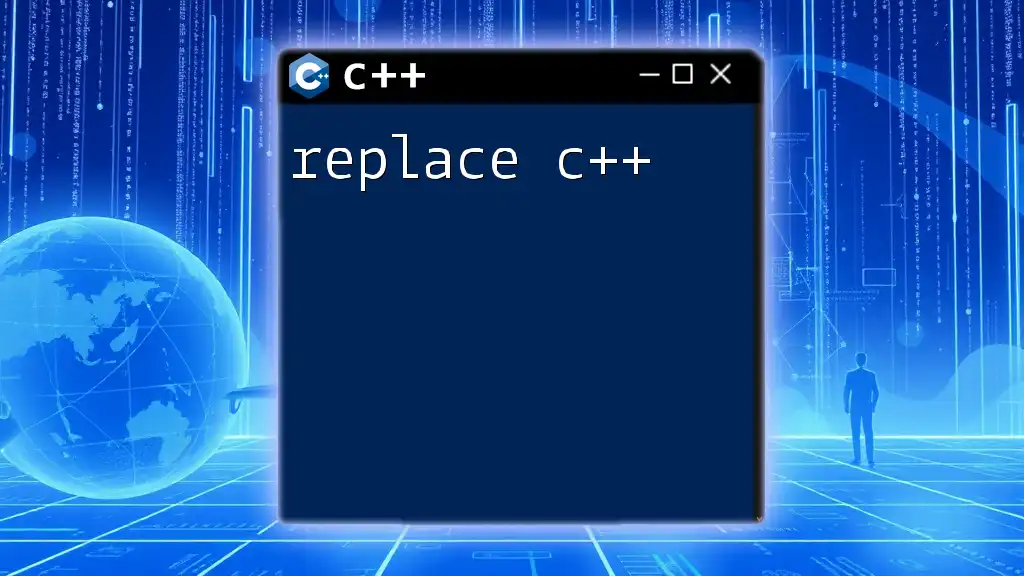
Common Mistakes with C++ Templates
Overusing Templates
While they are powerful, misusing templates can lead to complex and hard-to-maintain code. It’s important to assess when a template is genuinely necessary versus when a simple function or class would suffice.
Inefficient Template Instantiation
Understanding the costs associated with instantiating templates is key to maintaining performance. Each unique instance of a template results in generated code, so be mindful when creating templates for many different types.
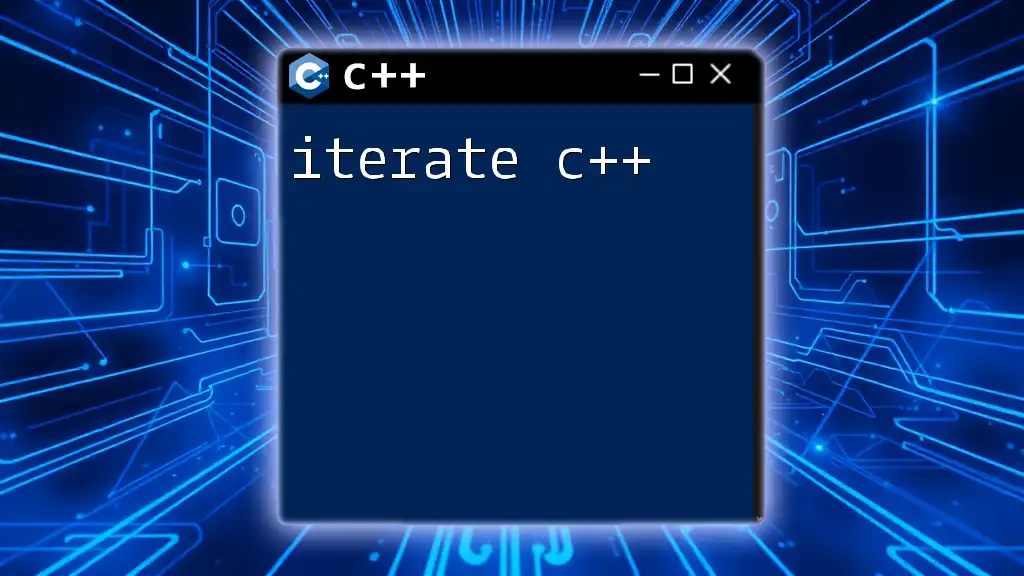
Recap of Key Points
Templates in C++ are a robust feature that enables type-safe, reusable, and flexible code. They can take various forms, including function templates, class templates, and variadic templates. Advanced concepts like template specialization and SFINAE add further power and functionality to template programming.
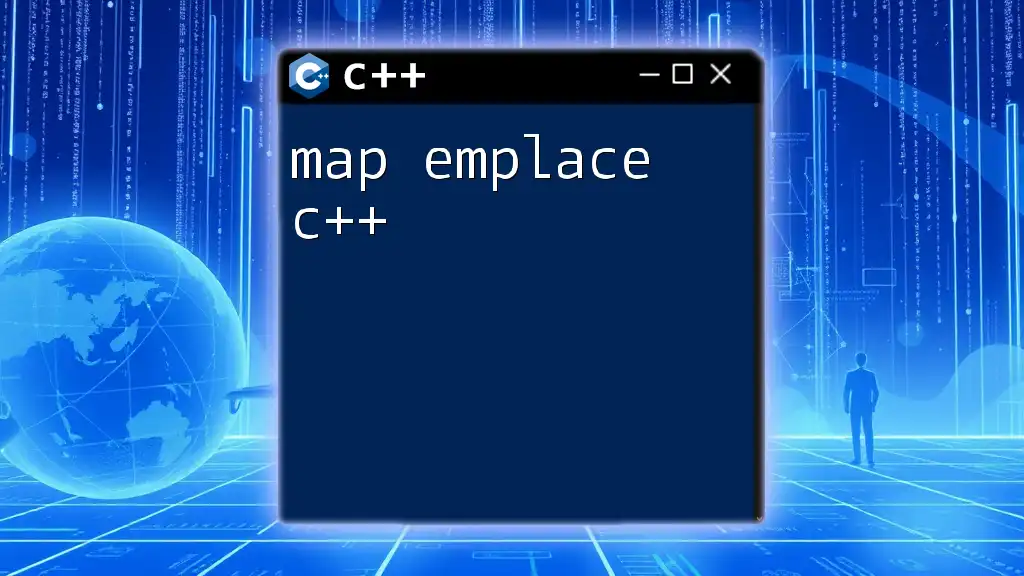
Encouragement to Explore Templates Further
There are numerous resources available for those wishing to dive deeper into templates in C++. Books, online courses, and community forums are excellent starting points to expand your knowledge. Whether you are new to C++ or looking to enhance your skills, leveraging templates is a critical part of becoming an effective C++ developer.