A C++ template class allows you to create a blueprint for a class that can operate with any data type, enabling code reusability and type safety.
Here's a simple example of a C++ template class:
template <typename T>
class Container {
public:
Container(T value) : value(value) {}
T getValue() const { return value; }
private:
T value;
};
What is a Template Class in C++?
A template class in C++ is a blueprint for creating classes that can operate with any data type. Rather than defining multiple classes for different types, a template allows you to write a single class definition. This feature embodies the principle of code reusability—you get to use the same code with different data types without sacrificing type safety.
The key features of template classes include:
- Code Reusability: Write once, use with any data type.
- Type Safety: Templates allow for compile-time checking, reducing runtime errors.
- Performance Benefits: Template specialization can lead to optimized code.
Through these features, template classes allow you to create highly generic and flexible code.
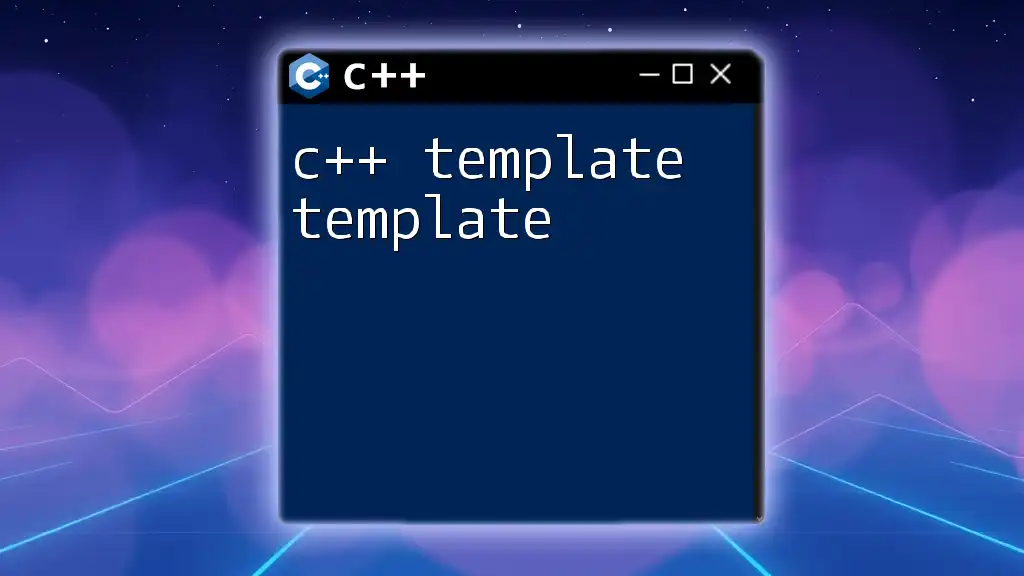
Syntax of Template Class
Understanding the syntax of a template class is essential for using it in your programs. A basic template class is declared as follows:
template <typename T>
class ClassName {
// Class definition goes here
};
In this definition:
- `template <typename T>` indicates that `T` is a placeholder for any data type.
- `ClassName` is the name of your class.
Consider a practical example where you might need a template class. For instance, you might want to create a generic container to hold any type of object. This is an ideal use case for a class template.
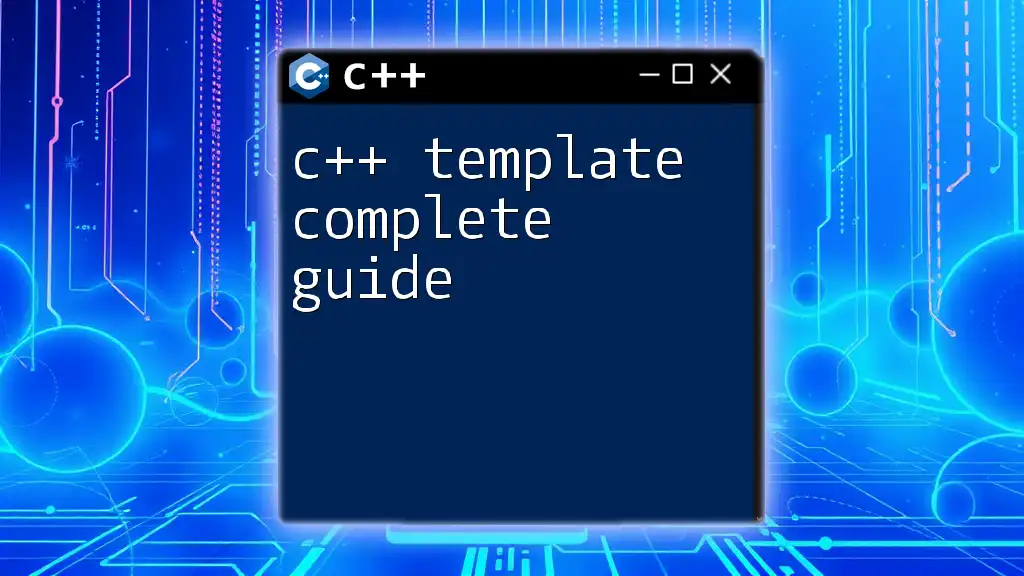
Example of Template Class in C++
Creating a Simple Template Class
Let's create a simple template class named `Box`. This class can hold a single value of any type.
Here’s how you might define this class:
template <typename T>
class Box {
private:
T content;
public:
Box(T c) : content(c) {}
T getContent() { return content; }
};
In this code:
- `T content;` defines a member variable that can hold any type specified when the class is instantiated.
- The constructor `Box(T c)` initializes the `content`.
- The member function `getContent()` returns the value of `content`.
This implementation exemplifies how to implement a template class that is both simple and versatile.
Using the Template Class
To utilize the `Box` template class, let’s instantiate it with a few different data types.
int main() {
Box<int> intBox(123);
Box<std::string> strBox("Hello, Templates!");
std::cout << intBox.getContent() << std::endl; // Output: 123
std::cout << strBox.getContent() << std::endl; // Output: Hello, Templates!
return 0;
}
In this usage example:
- `Box<int>` creates an instance specifically for integers.
- `Box<std::string>` creates another instance for strings.
The ability to instantiate `Box` with various types showcases the power and flexibility of template classes.
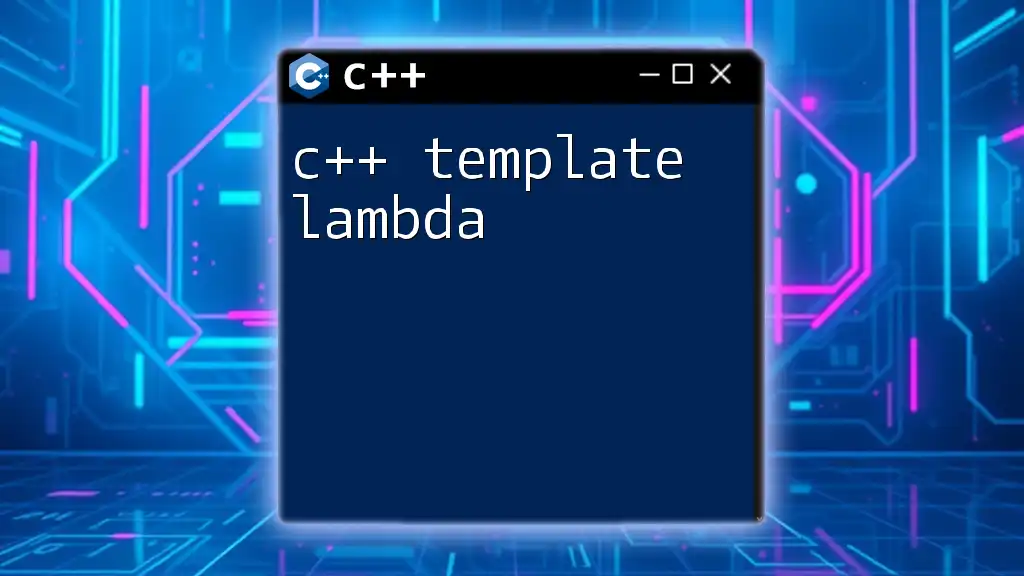
Advanced Concepts in Template Classes
Template Specialization
Template specialization is a technique that allows you to define specific behaviors for a particular data type. Let's rewrite the `Box` class for strings to demonstrate this.
template <>
class Box<std::string> {
private:
std::string content;
public:
Box(std::string c) : content(c) {}
std::string getContent() { return "String Content: " + content; }
};
In this specialized version:
- We define how `Box` behaves specifically when `std::string` is the datatype.
- The `getContent()` function prepends a label to the output.
Specialization can enhance functionality based on the type being worked with, thus providing different behaviors as needed.
Template Parameter Packs
Template parameter packs allow you to work with multiple types in a single template class. Here’s an example of how you can implement this using `std::tuple`.
template <typename... Args>
class MultiBox {
private:
std::tuple<Args...> contents;
public:
MultiBox(Args... args) : contents(std::make_tuple(args...)) {}
};
In this example:
- `typename... Args` allows for an arbitrary number of template parameters.
- `std::tuple<Args...>` can hold any number of parameters of varied types.
- This enables the creation of a `MultiBox` that can hold multiple items of potentially different types.
Using parameter packs, you can create even more dynamic classes, increasing the capability of template programming in C++.
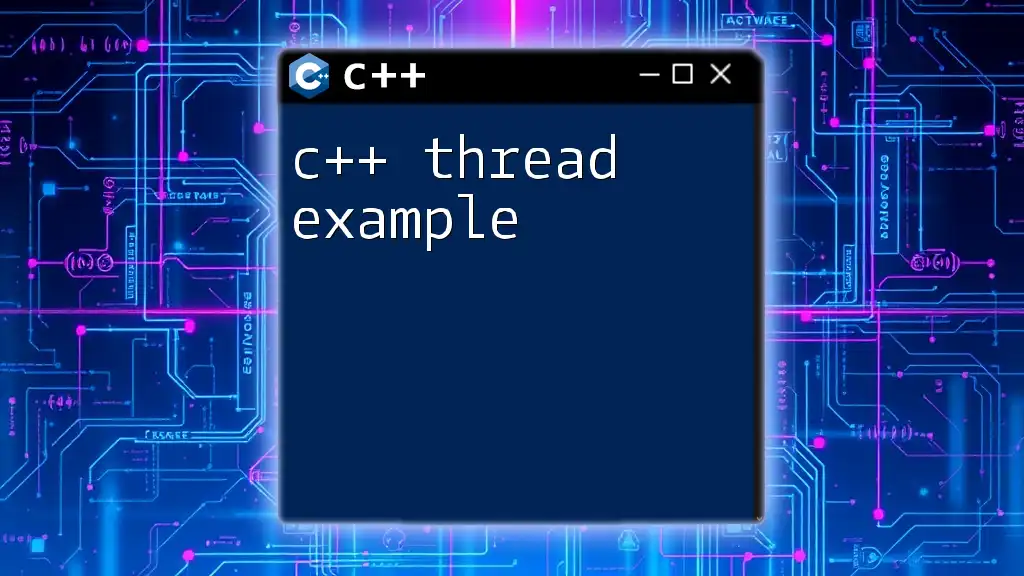
Best Practices for Using Template Classes
Code Organization
Keeping template classes well-organized is crucial for maintenance and readability. Here are some best practices:
- Separate Declarations and Implementations: Consider implementing your template classes in header files only. This keeps source files tidy and emphasizes reusable components.
- Clear Naming Conventions: Use descriptive names for template parameters to convey intent, such as `T` for type or `Size` for integral values.
Performance Considerations
While templates boost reusability and flexibility, they can also lead to template bloat—a scenario where excessive instantiation leads to increased binary size. To mitigate this:
- Use explicit specializations wisely and only when necessary.
- Make sure to profile the performance impact of template-heavy code, ensuring that the advantages outweigh any drawbacks.
By following these practices, you can maximize the efficiency and effectiveness of your C++ template classes while maintaining clean and readable code.
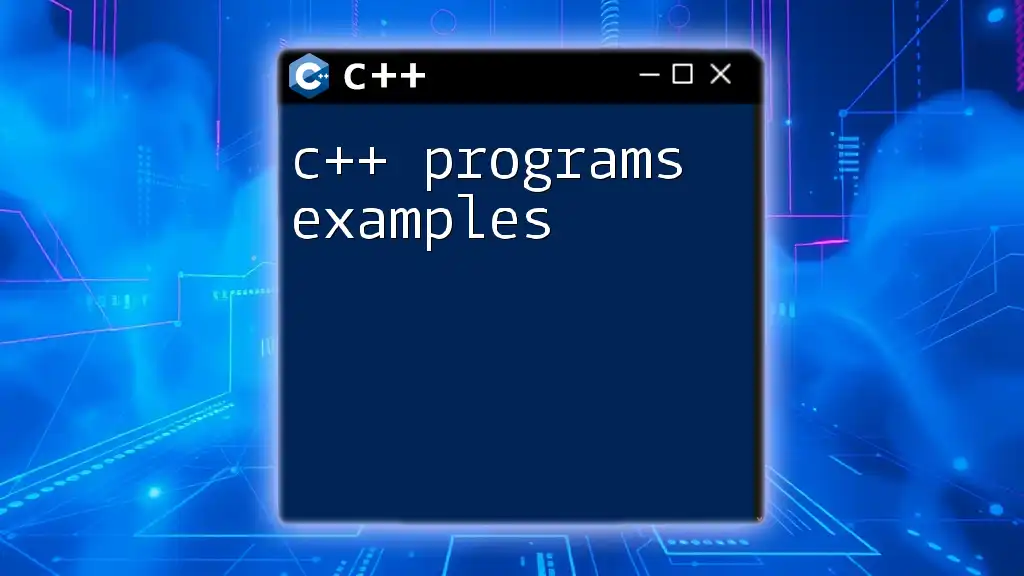
Conclusion
Understanding `c++ template class example` is essential for modern C++ programming. Template classes provide an invaluable method for achieving code reusability without sacrificing type safety or performance. By practicing how to create and utilize templates, and exploring advanced concepts like specialization and parameter packs, you can become adept at writing flexible and efficient C++ code.
For those eager to learn more, consider exploring additional resources or enrolling in lessons on advanced C++ techniques, including the intricate world of template programming.