In this post, we will explore practical examples of C++ while loops to help you understand their structure and use effectively.
#include <iostream>
using namespace std;
int main() {
int count = 1;
while (count <= 5) {
cout << "Count is: " << count << endl;
count++;
}
return 0;
}
What is a While Loop in C++?
Definition of a While Loop
A while loop is a control flow statement used in C++ programming that allows code to be executed repeatedly based on a boolean condition. As long as the condition evaluates to true, the code inside the loop will keep running.
How Does a While Loop Work?
At its core, a while loop consists of three main parts:
- Condition Checking: The condition is evaluated before each iteration. If it evaluates to true, the loop executes; if false, the loop terminates.
- Execution: If the condition is true, the statements inside the loop body are executed.
- Repetition: After executing the loop body, it goes back to re-evaluate the condition.
It's crucial to update the condition during the loop’s execution to prevent an infinite loop, a programming error where the loop runs endlessly due to an unresolved condition.
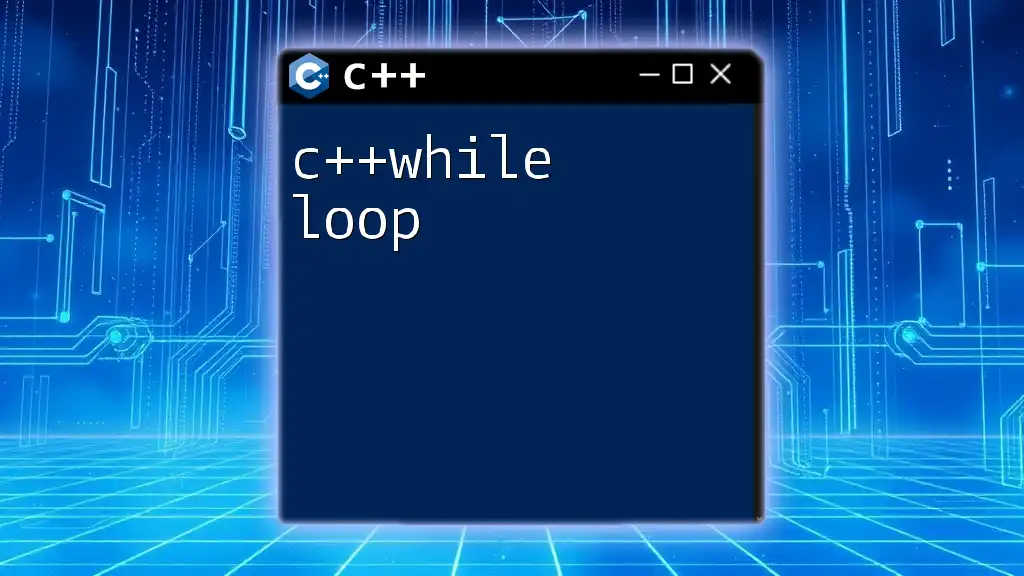
Basic Syntax of a While Loop
Understanding the syntax is essential for writing correct while loops in C++. The general structure is as follows:
while (condition) {
// Code to be executed
}
Here, `condition` is a boolean expression that decides whether the loop should continue executing. The code within the braces `{}` will execute repeatedly as long as the condition remains true.
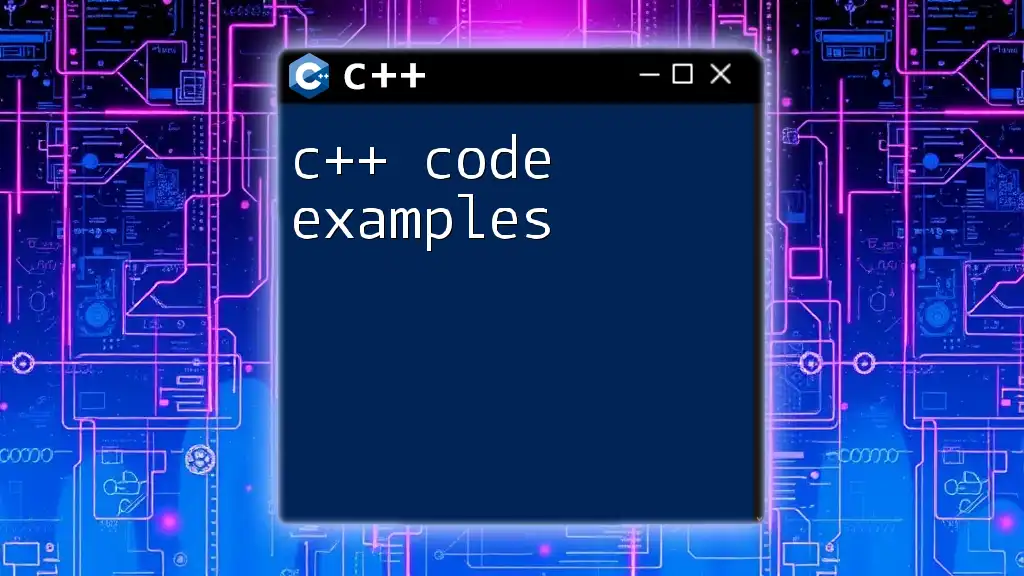
Simple While Loop Examples
Example 1: Basic Count from 1 to 5
In this example, we'll write a simple program that counts from 1 to 5:
#include <iostream>
using namespace std;
int main() {
int count = 1;
while (count <= 5) {
cout << count << endl;
count++;
}
return 0;
}
Explanation: Here, we initialize `count` to 1. While `count` is less than or equal to 5, the loop will output the value of `count`. After each output, we increment `count` by 1. This loop will print numbers 1 through 5, demonstrating a straightforward use of the while loop.
Example 2: Sum of Numbers
Let's create a program that calculates the sum of numbers from 1 to 10 using a while loop:
#include <iostream>
using namespace std;
int main() {
int sum = 0, number = 1;
while (number <= 10) {
sum += number;
number++;
}
cout << "Sum: " << sum << endl;
return 0;
}
Explanation: This example initializes `sum` to 0 and `number` to 1. During each iteration, `number` is added to `sum`, and `number` is incremented. The loop continues until `number` exceeds 10. In the end, it displays the total sum, which is 55.
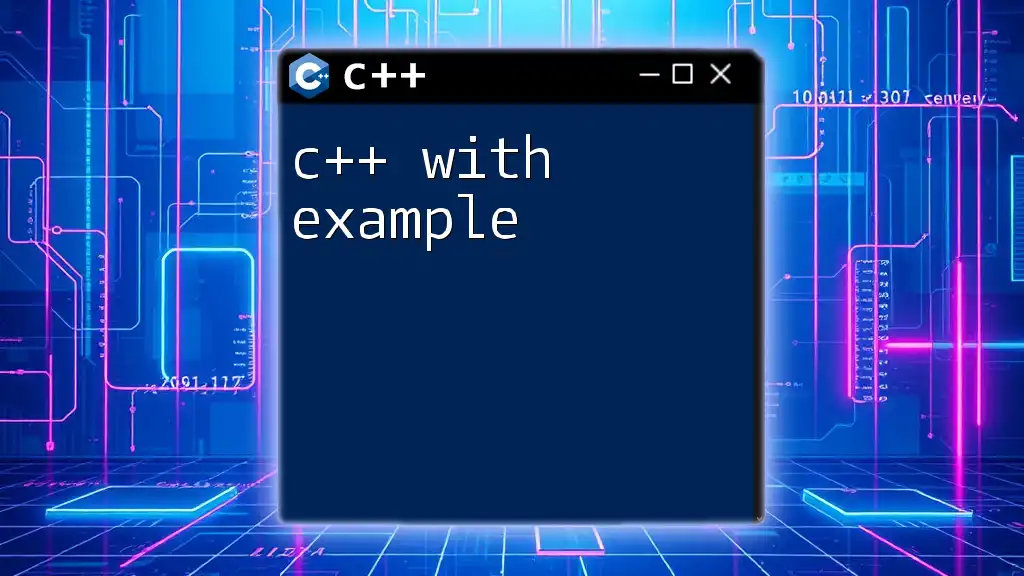
Practical While Loop Examples
Example 3: Input Validation
Input validation is essential in programming. Here’s a scenario to ensure that user input is within a valid range:
#include <iostream>
using namespace std;
int main() {
int age;
cout << "Enter your age: ";
cin >> age;
while (age < 0 || age > 120) {
cout << "Please enter a valid age: ";
cin >> age;
}
cout << "Your age is: " << age << endl;
return 0;
}
Explanation: Initially, the program prompts the user to enter their age. If the input age is less than 0 or greater than 120, it triggers the while loop, prompting the user to re-enter their age until a valid value is received. This demonstrates how while loops can effectively validate user input.
Example 4: Infinite Loop with a Break Condition
In this example, we will see how to create an infinite loop with a condition to exit:
#include <iostream>
using namespace std;
int main() {
int input;
while (true) {
cout << "Enter a number (0 to exit): ";
cin >> input;
if (input == 0) {
break;
}
cout << "You entered: " << input << endl;
}
return 0;
}
Explanation: This program continuously prompts the user for input and prints it. The loop is designed to run indefinitely (due to `while (true)`), but it includes an exit condition using `break`. When the user enters `0`, the loop terminates, showcasing how to manage infinite loops safely.
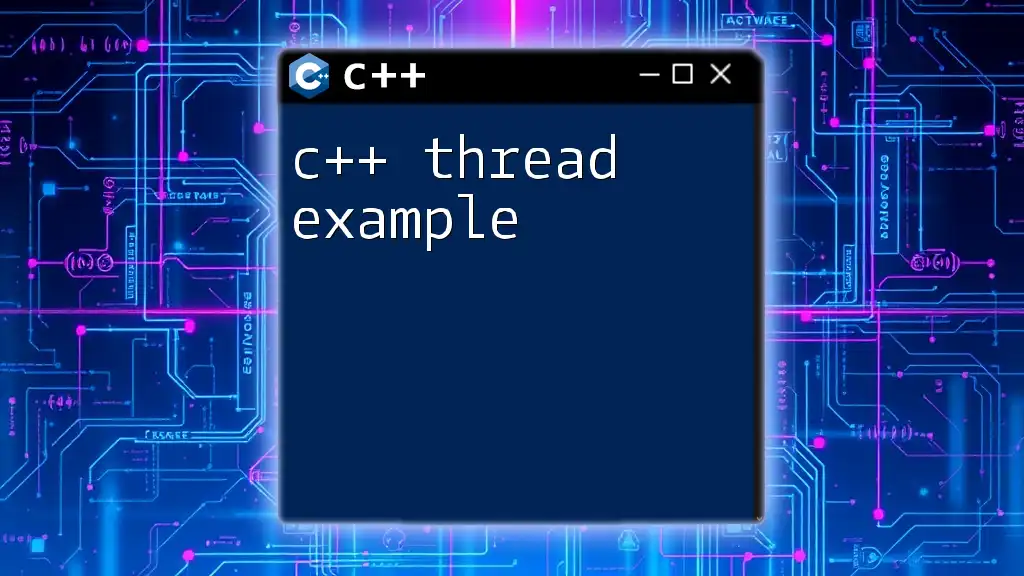
Advanced While Loop Examples
Example 5: Nested While Loops
Nested loops allow execution of a loop within another loop. Here's how it works:
#include <iostream>
using namespace std;
int main() {
int i = 1;
while (i <= 3) {
int j = 1;
while (j <= 3) {
cout << "i: " << i << ", j: " << j << endl;
j++;
}
i++;
}
return 0;
}
Explanation: In this example, the outer loop runs three times (for `i` from 1 to 3). The inner loop also runs three times for every iteration of `i`. This results in a total of nine outputs, demonstrating how nested while loops function together.
Example 6: Using While Loops with Arrays
While loops can also be utilized to traverse arrays. In this example, we will print all elements of an array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int index = 0;
while (index < 5) {
cout << arr[index] << " ";
index++;
}
return 0;
}
Explanation: The program initializes an array of integers and uses a while loop to iterate through the elements. The condition checks that `index` is less than the array size, allowing it to print all five elements sequentially.
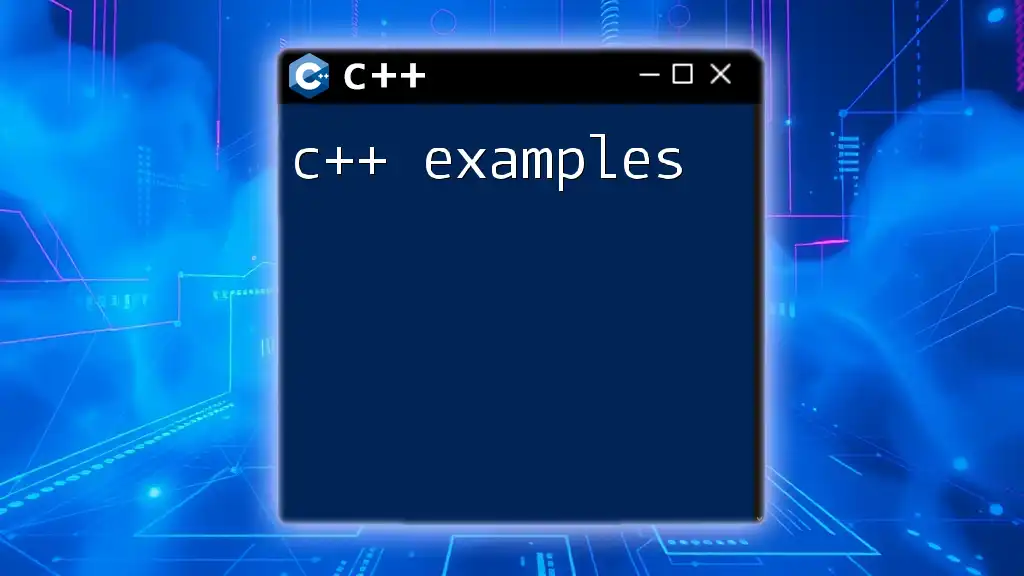
Common Mistakes with While Loops
Infinite Loops
One of the most common mistakes made with while loops is creating infinite loops. This often occurs when the condition is never updated. For instance, if we forget to increment `count` in the first example, it will run indefinitely. To avoid this, always ensure that the loop condition can eventually become false.
Misunderstanding Conditions
Another frequent mistake arises from incorrectly setting conditions. A common error is using the wrong logical operators, which can lead to unexpected behavior. For example, confusing `&&` (AND) with `||` (OR) can alter the loop’s expected execution path. Always double-check the condition's logic to ensure it aligns with the intended purpose of the loop.
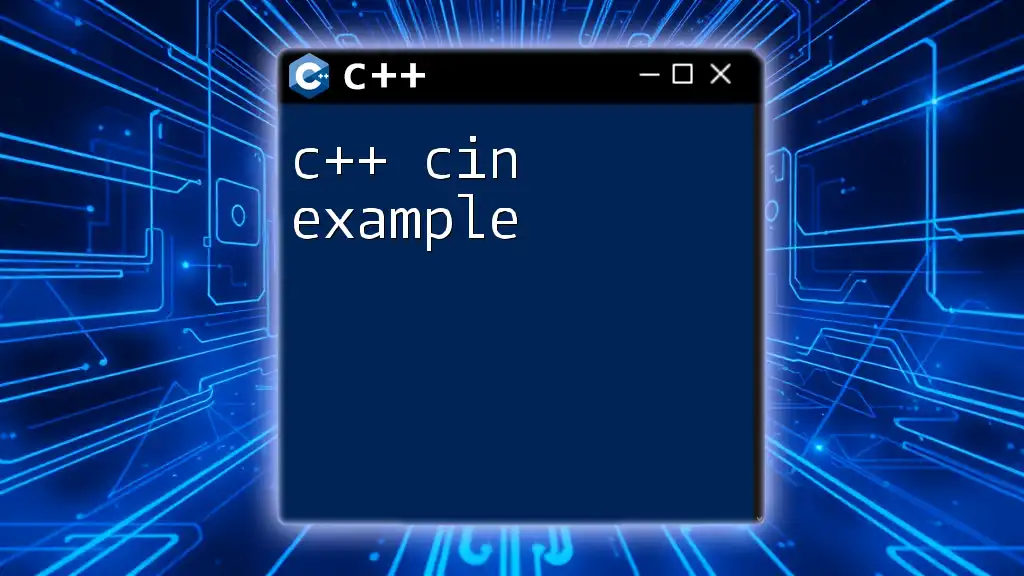
Conclusion
In summary, understanding C++ while loop examples is fundamental for any programmer looking to harness the power of control flow in their code. While loops provide a versatile way to execute code based on condition checks, and by mastering this construct, programmers can efficiently handle repetitive tasks, validate user inputs, and perform subsequent actions until a specific condition is met. As you practice these examples and experiment with while loops, you'll enhance your problem-solving skills in programming.
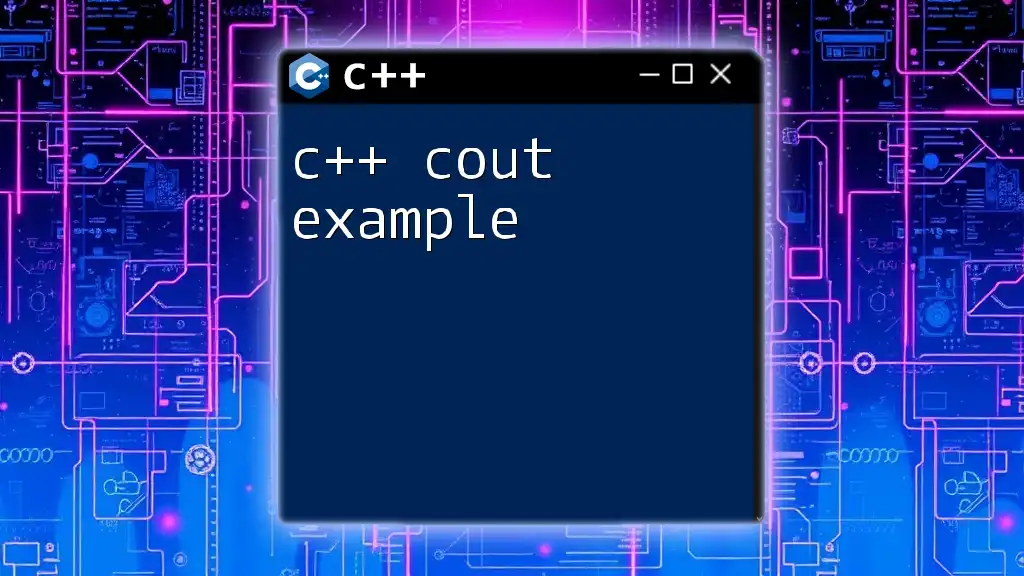
Call to Action
Feel free to share your own examples or questions in the comments below. If you want more tips and insights into C++ programming, consider joining our mailing list for future updates. Happy coding!
Additional Resources
For further learning, consider checking out the official C++ documentation or exploring recommended books and courses that delve into loops and structured programming practices.