A C++ `while` loop repeatedly executes a block of code as long as a specified condition evaluates to true.
Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
int count = 0;
while (count < 5) {
cout << "Count is: " << count << endl;
count++;
}
return 0;
}
Understanding the While Loop in C++
A while loop is a fundamental control structure in C++ that enables you to execute a block of code repeatedly as long as a specified condition evaluates to true. This loop continues its iterations until the condition becomes false. The basic syntax for a while loop looks like this:
while (condition) {
// code to execute
}
In this formulation:
- Condition: This is a boolean expression that the while loop checks before each iteration. If the condition is true, the loop executes the statements in the loop body.
- Loop Body: This is the block of code that gets executed repeatedly as long as the condition holds true.
How the While Loop Works
The while loop operates based on a simple flow:
- Before each iteration, the condition is evaluated.
- If the condition returns true, the code inside the loop executes.
- Once the code block has executed, control returns to the condition check, continuing this cycle until the condition is false.
This means that if your condition is set up correctly, the while loop can be a powerful tool for performing repetitive tasks, such as iterating over user input or processing collections of data.
Example of Simple While Loop
Here’s a basic example demonstrating the use of a while loop in C++:
#include <iostream>
using namespace std;
int main() {
int i = 0; // Initialization
while (i < 5) { // Condition
cout << "Number: " << i << endl; // Executed while condition is true
i++; // Incrementing loop variable
}
return 0;
}
In this example, the loop prints numbers 0 through 4. The variable `i` is incremented with each iteration, eventually making the condition false when `i` reaches 5, at which point the loop terminates.
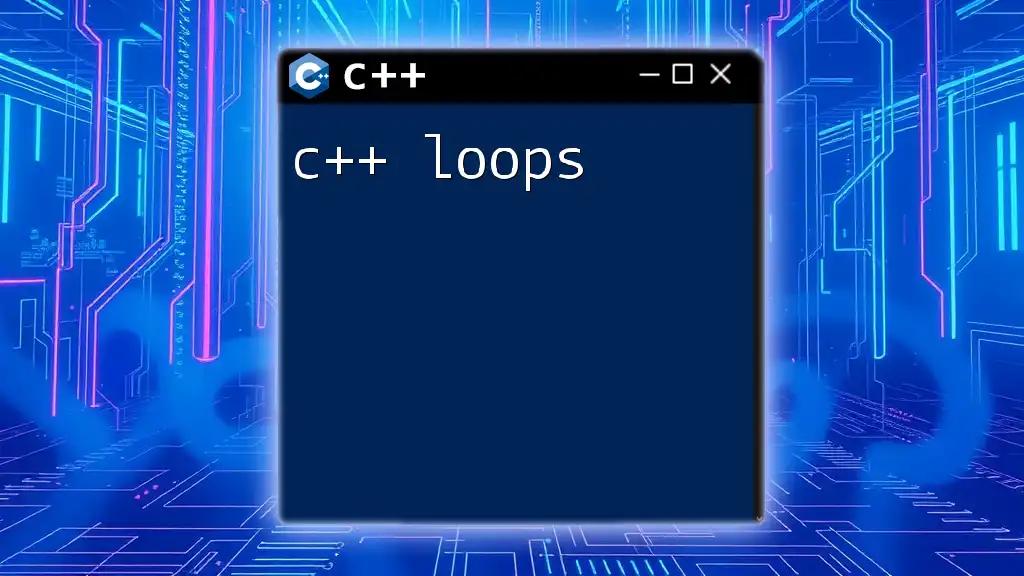
When to Use a While Loop
The while loop is appropriate when the number of iterations is not known beforehand and depends on a condition that could change during execution. For example, consider scenarios like:
- User Input: When you need to keep prompting a user until valid input is received.
- Indeterminate Iterations: Situations like reading data from a file or processing user commands in a game, where the number of steps may vary.
Comparison with Other Loops
While loops are often compared with other loop constructs like `for` loops and `do-while` loops. The key difference lies in:
- For Loop: Best suited for counting scenarios where the number of iterations is predetermined.
- Do-While Loop: Executes the code block at least once before checking the condition, making it useful when the initial execution is a requirement.
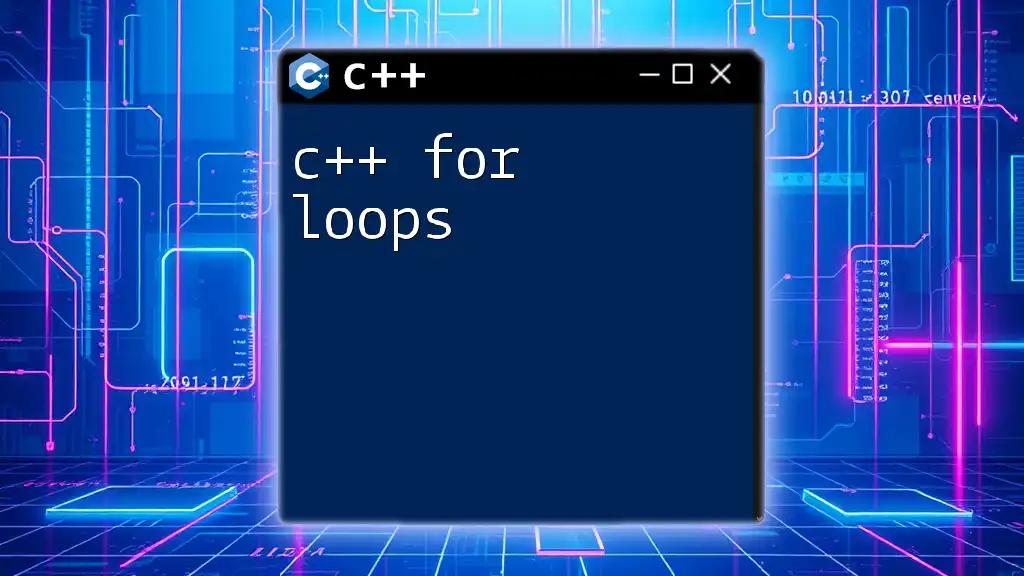
Common Mistakes with While Loops
Infinite Loops
One of the most common pitfalls when writing while loops is creating an infinite loop. An infinite loop occurs when the loop's condition never evaluates to false, causing the program to execute the loop body indefinitely. For example:
while (true) {
// This loop runs forever
}
To avoid infinite loops, ensure that the loop variable is properly updated inside the loop, and logically verify that the condition will eventually become false.
Forgetting to Update the Loop Variable
Failure to update the loop variable can also lead to infinite loops or unintended behavior. Take a look at this incorrect implementation:
int i = 0;
while (i < 5) {
cout << "Hello" << endl; // i is never updated
}
Since `i` is never updated within the loop, the condition remains true, and the loop would spin endlessly while constantly printing "Hello." Always remember to modify the loop variable within the loop to maintain control.

Practical Use Cases for While Loops
Real-World Applications
While loops are frequently used in situations like:
- Input Validation: Here’s an example where a while loop is used to validate user input until a correct value is entered:
int num;
cout << "Enter a positive number: ";
cin >> num;
while (num <= 0) {
cout << "Invalid input. Please enter a positive number: ";
cin >> num; // Continue prompting until valid input is received
}
In this code, the loop ensures the user enters a valid positive number, repeatedly requesting input until the requirement is met.
Applying to Game Loops
While loops can also serve as the foundation for game loops where the game runs continuously until a specific condition is met (like an exit command). This involves continuously processing user input, updating the game state, and rendering graphics within the while loop.

Nesting While Loops
Explanation of Nested Loops
C++ allows you to nest while loops, meaning you can have one loop inside another. This might be necessary when dealing with multi-dimensional data or performing more complex iterations. The syntax for nested while loops looks like this:
while (outer_condition) {
while (inner_condition) {
// code
}
}
Example of Nested While Loops
Consider this example where we loop through two indices:
int i = 0, j;
while (i < 3) { // Outer loop
j = 0; // Reset j for each iteration of i
while (j < 2) { // Inner loop
cout << "i: " << i << ", j: " << j << endl; // Print indices
j++;
}
i++;
}
This code will print pairs of values for `i` and `j`, demonstrating how inner loops can operate independently while being controlled by an outer loop.

Best Practices when Using While Loops
To maximize the effectiveness of your while loops and ensure robust code, consider these best practices:
- Keep Conditions Simple and Clear: Complex conditions can lead to confusion and errors. Aim for readability.
- Always Update the Loop Variable: Ensure the loop variable changes within the loop to avoid infinite loops.
- Minimize the Logic Inside the Loop: Keep the logic straightforward to maintain performance and ease of debugging.
- Use Comments for Clarity: Comment on your code to explain the logic behind conditions and actions, especially if implementing complex loops.
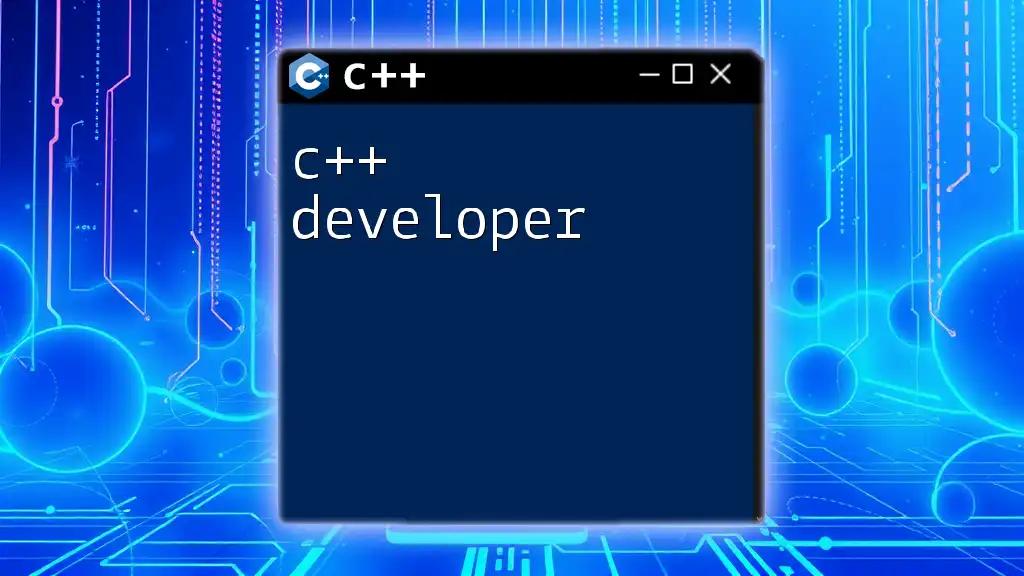
Conclusion
In conclusion, the c++ while loop is an essential construct for developers, providing the ability to execute code repeatedly under a specified condition. Understanding its syntax, structure, and best practices can empower programmers to leverage this tool effectively in various applications. Practice with examples, and don't hesitate to explore different scenarios where while loops shine!

Additional Resources
For further learning, please explore authoritative C++ documentation and recommended online courses that can deepen your understanding of loops and conditional structures in programming.

FAQs Section
What happens if the condition is always false?
If the condition in a while loop is always false from the beginning, the code block inside the loop will never execute. This means that any code following the loop will run immediately. Always ensure that your loops can evaluate to true when you expect them to.
Can you use a while loop without initializing a variable?
Technically, yes; however, if you do not properly initialize or manage your loop variables, you risk creating infinite loops or unintended behavior. It's always best practice to have your variables correctly initialized before use in your loop conditions.
How to break out of a while loop early?
You can use the `break` statement within your while loop to exit it prematurely when a certain condition is met. For example:
while (true) {
// some code
if (condition) {
break; // exit the loop
}
}
This allows flexibility in program flow and efficient handling of looping constructs based on dynamic conditions.