The "Hello, World!" program is a simple way to demonstrate the basic syntax of C++ and serves as an introductory example for beginners.
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
What is "Hello World"?
The "Hello World" program is typically the first program that programmers write when learning a new programming language. It serves not just as a simple example but as an essential introduction to the syntax and structure of the language being learned. Historically, it has been used to demystify programming, allowing beginners to see their first successful output quickly.
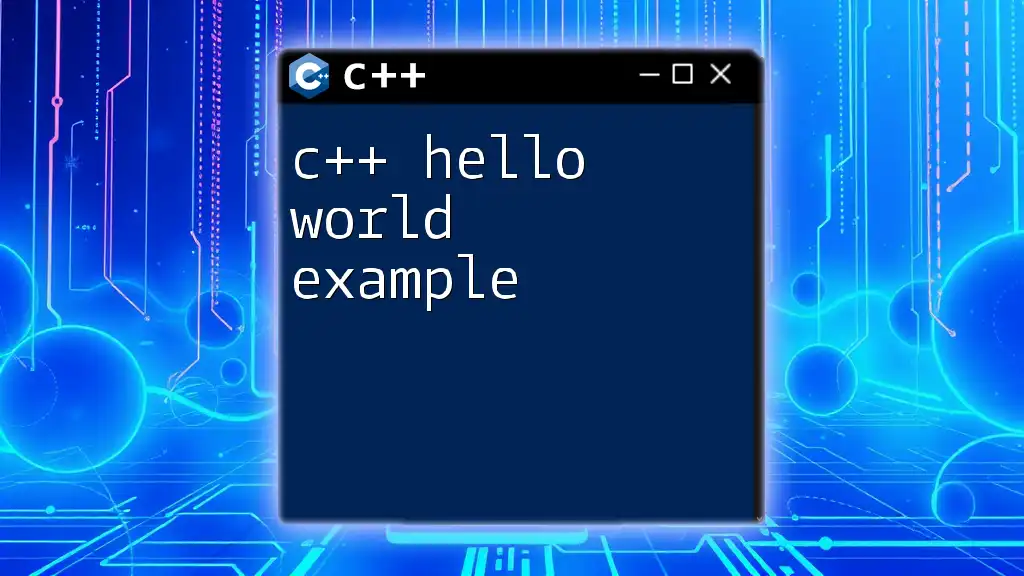
Setting Up the C++ Environment
Installing a C++ Compiler
Before you can start programming in C++, you need a C++ compiler. Different operating systems use various compilers. Here are some common options:
- GCC (GNU Compiler Collection): Widely used for Linux environments.
- Clang: Known for its fast compilation and excellent diagnostics, used in macOS and Linux.
- MSVC (Microsoft Visual C++): Mainly used for Windows development.
To install a compiler:
- Windows: Use the Microsoft Store to install Visual Studio, which includes the MSVC compiler. Alternatively, you can install MinGW to use GCC.
- macOS: You can install Xcode from the App Store, which includes Clang.
- Linux: Most distributions come with GCC pre-installed, or you can easily install it via your package manager (e.g., `sudo apt install g++` for Ubuntu).
Choosing an Integrated Development Environment (IDE)
An IDE streamlines the coding process with features like syntax highlighting, debugging, and project management. Here are a few popular choices for C++ development:
- Code::Blocks: Lightweight and open-source with easy project setup.
- Visual Studio: Comprehensive and feature-rich, ideal for Windows users.
- CLion: A powerful IDE from JetBrains, great for cross-platform development.
- Eclipse: Open-source and customizable, suitable for larger projects.
Once you've installed the IDE, create a new project and select C++ as the programming language to start coding.
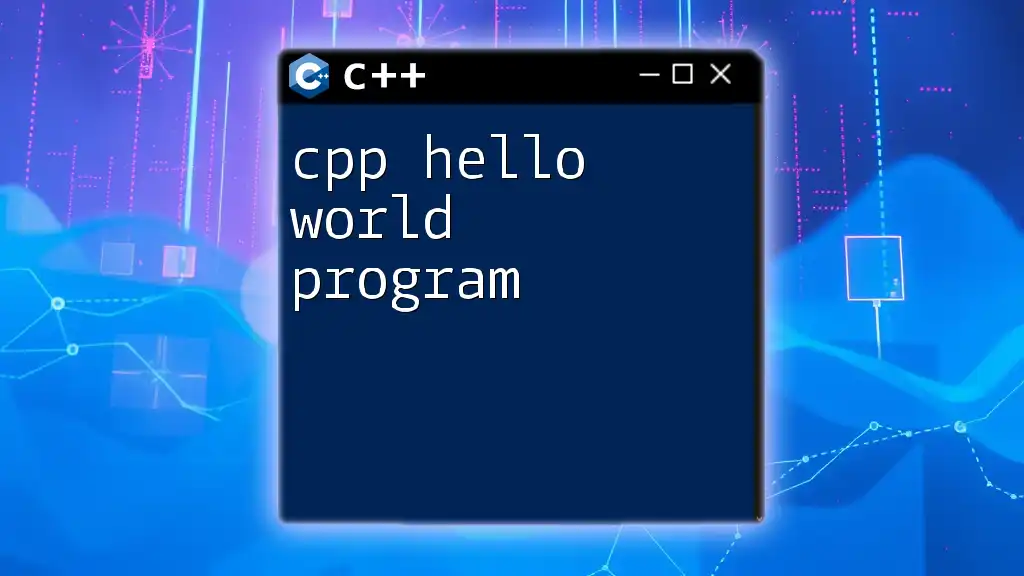
Writing Your First "Hello World" Program
The Syntax of a C++ Program
Understanding the basics of C++ syntax is crucial for writing your first program. A typical C++ program consists of the following components:
- Preprocessor Directives: Lines that begin with `#`, such as `#include <iostream>`, which tell the compiler to include standard libraries.
- Main Function: Indicated by `int main()`, it serves as the entry point for execution.
- Return Statement: Typically `return 0;`, signaling successful completion of the program.
Code Example: A Simple "Hello World"
Here is a simple "Hello World" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation of Each Line of Code:
-
`#include <iostream>`: This directive includes the Input/Output stream library, crucial for using `std::cout` to display output.
-
`int main()`: This denotes the start of the main function. Every C++ program must have a `main` function where execution begins.
-
`std::cout << "Hello, World!" << std::endl;`:
- `std::cout`: Represents the standard output stream used to print text to the console.
- `<<`: The insertion operator, which sends the string `"Hello, World!"` to the output stream.
- `"Hello, World!"`: The message to be printed.
- `std::endl`: This manipulator is used to insert a newline character and flush the output buffer, ensuring that the message appears in the console immediately.
-
`return 0;`: This statement indicates that the program has been executed successfully and returns control back to the operating system.
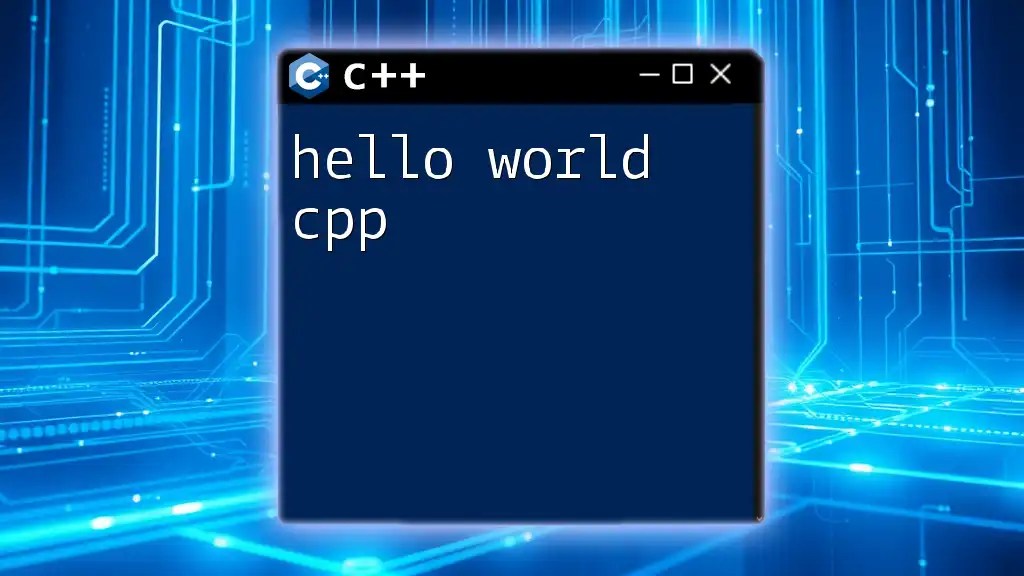
Compiling and Running the Program
Compilation Process
Compiling is the process where the compiler translates your C++ code into machine-readable format. Missing syntax or errors can lead to compilation failures, so it is essential to write syntactically correct code.
Running the Program
Once you've written your code, it’s time to compile and run it. Here's how to do that in various environments:
-
Windows (using Command Prompt):
- Open Command Prompt.
- Navigate to your code’s directory.
- Compile using: `g++ hello.cpp -o hello.exe`
- Run the program with: `hello.exe`
-
macOS (using Terminal):
- Open Terminal.
- Navigate to your code's directory.
- Compile using: `g++ hello.cpp -o hello`
- Run the program with: `./hello`
-
Linux (using Terminal):
- Open Terminal.
- Navigate to your code's directory.
- Compile using: `g++ hello.cpp -o hello`
- Run the program with: `./hello`
Common Errors and How to Troubleshoot Them
-
Compilation Errors: These might arise from syntax mistakes, such as missing semicolons or typos in function names. The compiler typically provides error messages that can guide you to the problem line.
-
Linker Errors: These occur when the necessary libraries are not found. Ensure all required libraries are linked correctly, particularly if you include additional headers.
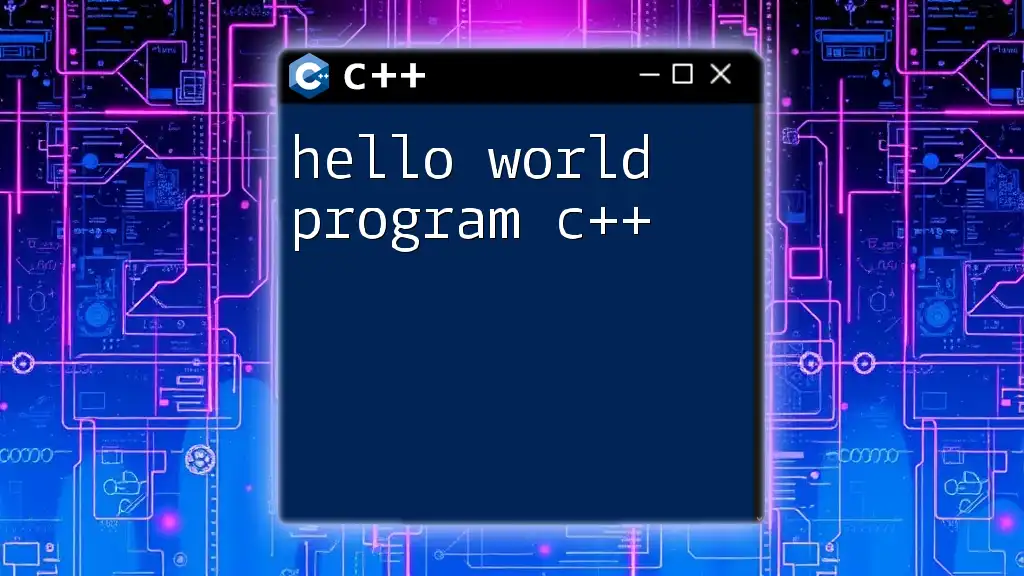
Variations of "Hello World"
Customizing Output
The "Hello World" program can be easily modified to provide different outputs or even accept user input. Here’s how to modify your program to greet the user by name:
#include <iostream>
#include <string>
int main() {
std::string name;
std::cout << "Enter your name: ";
std::cin >> name; // User input
std::cout << "Hello, " << name << "!" << std::endl; // Custom greeting
return 0;
}
Explanation of the Code:
- `std::string name;`: Declares a string variable to store the user’s name.
- `std::cin >> name;`: Uses `std::cin` to take input from the user.
- `std::cout << "Hello, " << name << "!"`: Combines static text with user input for a personalized greeting.
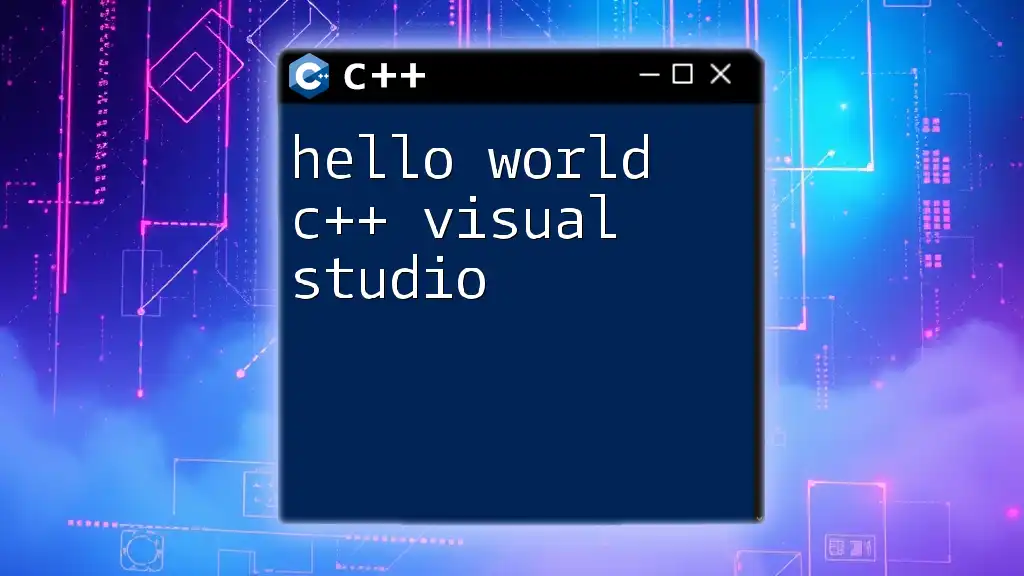
Conclusion
The "Hello World" program may seem simple, but it lays the groundwork for deeper understanding in C++. It illustrates the fundamental components of a C++ program and helps you get started with coding. As you become comfortable with this, consider exploring more C++ features such as loops, functions, and data structures to build more complex applications.
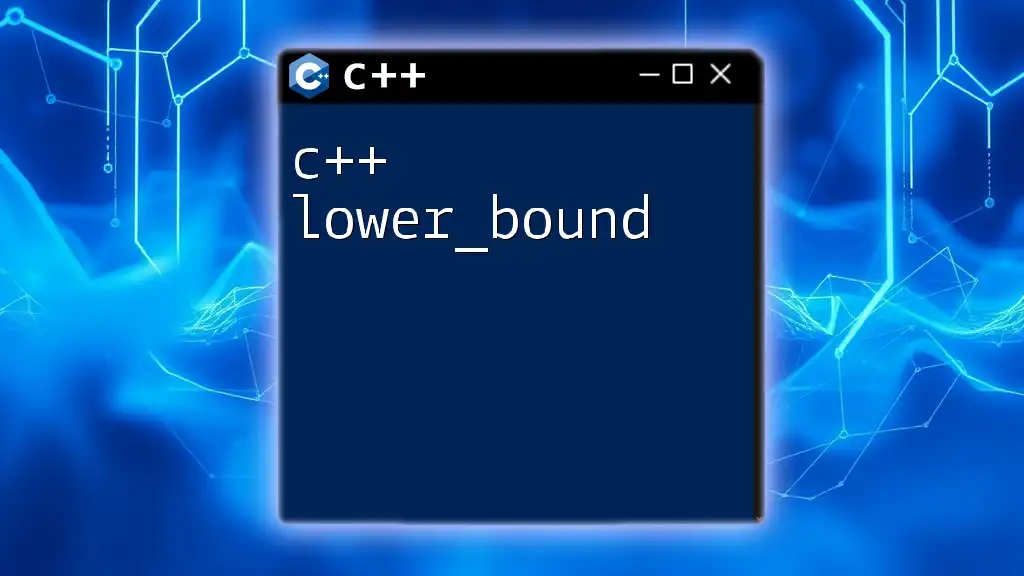
Frequently Asked Questions (FAQs)
Why is "Hello World" important in programming?
The "Hello World" program serves as a rite of passage for new programmers. It introduces essential concepts, provides an immediate sense of accomplishment, and demonstrates how to write and run a program.
What can C++ be used for beyond "Hello World"?
C++ is a versatile language widely used in various domains like game development, systems programming, embedded systems, and high-performance applications. It offers control over system resources and memory management, making it ideal for resource-intensive applications.
How can I troubleshoot compilation errors?
Common errors can typically be resolved by carefully reading the compiler’s error messages, checking for syntax errors, and ensuring all necessary libraries are included. Online forums and documentation can also be valuable resources for debugging.
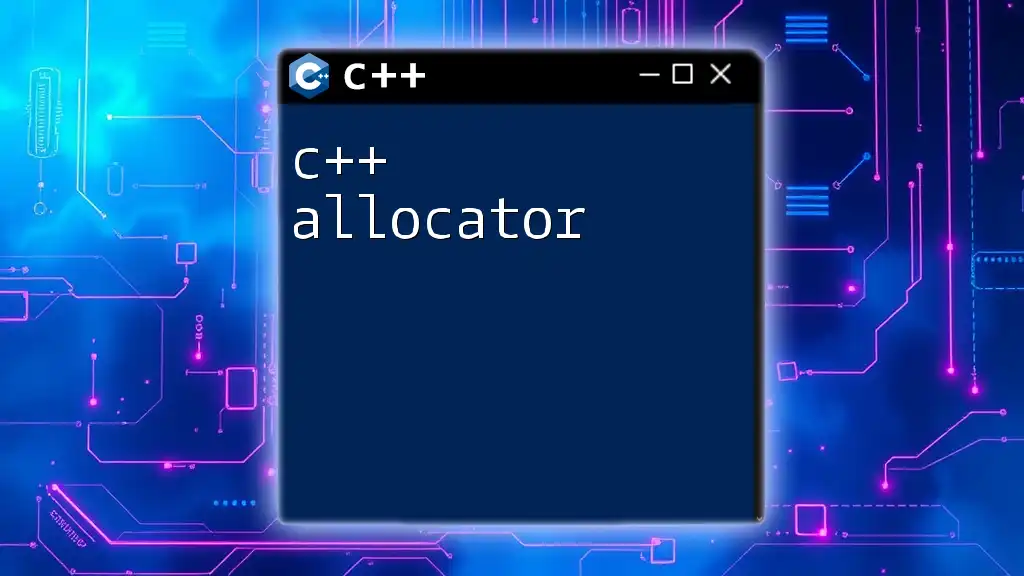
Call to Action
Now that you’ve executed your first C++ hello world program, challenge yourself by writing more complex programs! Experiment with user inputs, explore C++ features, and expand your programming skills. Stay tuned for more tutorials and advanced C++ topics that will elevate your coding expertise!