In C++, floor division can be achieved using the division operator for integers, which automatically rounds down to the nearest whole number. Here's a code snippet demonstrating floor division using integer types:
#include <iostream>
int main() {
int a = 7;
int b = 3;
int result = a / b; // Floor division
std::cout << "Result of floor division: " << result << std::endl; // Outputs 2
return 0;
}
Understanding the Concept of Floor Division
Floor division in C++ is a unique operation that translates mathematical division into programming syntax, yielding results that accommodate integer behavior. Floor division can be defined fundamentally as the process of dividing two numbers and returning the largest integer that is less than or equal to the quotient. This is particularly useful in scenarios where the precise value of division is not necessary, but rather the lowest integral result is desired.
To emphasize the difference, consider the contrasting behaviors between standard division and floor division. Standard division could yield a floating-point number, while floor division guarantees an integer output.
Mathematically, floor division can be represented using the formula:
- floor(a / b)
Consider some examples to cement this concept:
- Performing floor division on integers, such as `9 / 4`, results in 2, because 2 is the largest integer less than or equal to 2.25.
- For negative integers, floor division behaves differently. For instance, `-9 / 4` would result in -3, since -3 is the largest integer less than or equal to -2.25.
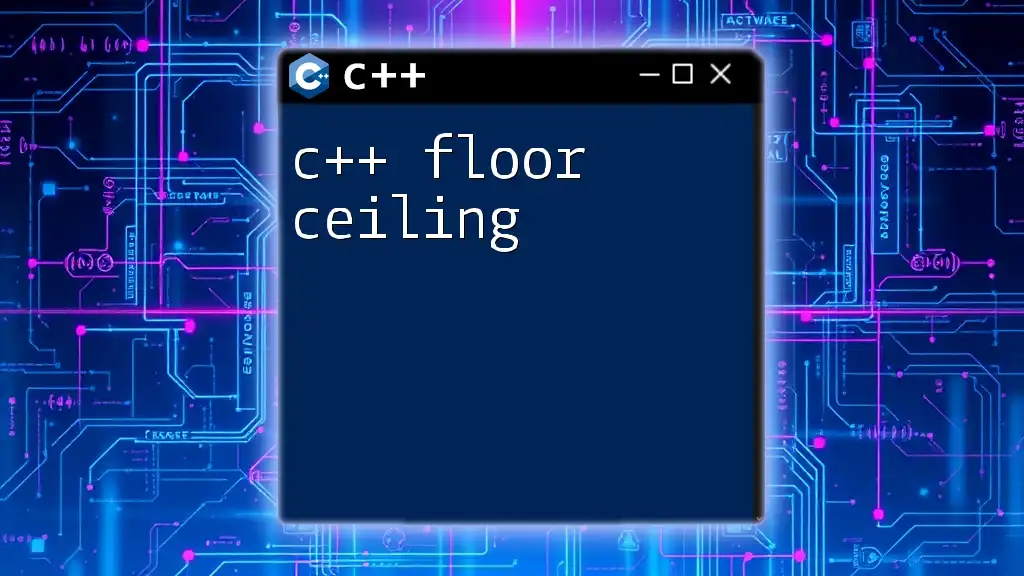
Syntax and Implementation
Floor Division Using Standard Operators
C++ allows for simple implementations of floor division using the division operator. Although the `/` operator defaults to standard division, when both operands are integers, it naturally results in an integer division.
#include <iostream>
int main() {
int a = 9;
int b = 4;
std::cout << "Floor Division: " << a / b << std::endl; // Outputs 2
return 0;
}
In this scenario, since both `a` and `b` are integers, C++ performs an implicit floor division, resulting in 2.
Implementing Floor Division with Type Casting
For cases involving floating-point numbers where you need an accurate floor division result, type casting helps. By using `static_cast`, we can transform floats to integers, effectively truncating any decimal values.
#include <iostream>
int main() {
double a = 9.0;
double b = 4.0;
int floor_division = static_cast<int>(a / b);
std::cout << "Floor Division via Casting: " << floor_division << std::endl; // Outputs 2
return 0;
}
Here, by casting the result of `a / b` to `int`, we eliminate any decimal portion, yielding the correct floor division of 2.
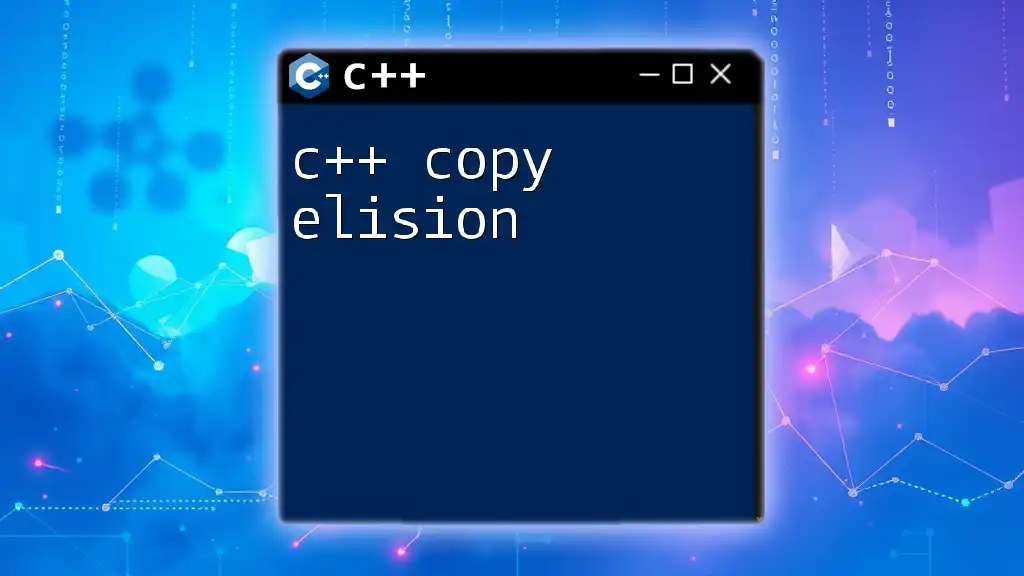
Customized Floor Division Function
Occasionally, it’s beneficial to have a customized floor division function, particularly when dealing with various data types or incorporating additional logic to handle edge cases.
To create a custom floor division function, one must consider how integer division behaves with negative values. Below is an example of a basic implementation:
#include <iostream>
int floor_divide(int x, int y) {
return (x < 0 && x % y != 0) ? (x / y) - 1 : (x / y);
}
int main() {
std::cout << "Custom Floor Division: " << floor_divide(-9, 4) << std::endl; // Outputs -3
return 0;
}
This function checks whether the numerator is negative and whether there is a remainder. If so, it decrements the quotient, ensuring it adheres to the floor division's definition.
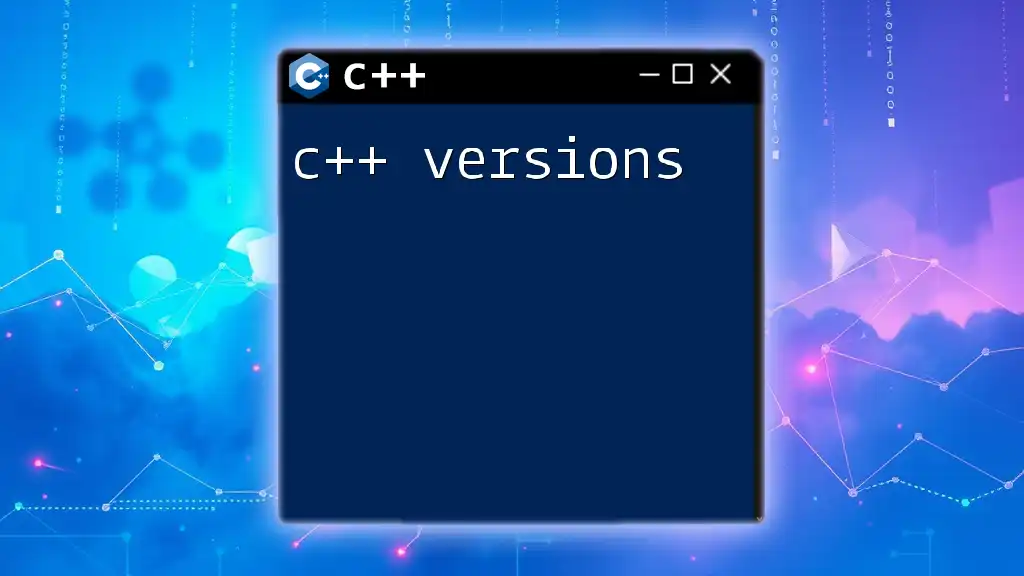
Handling Edge Cases
Division by Zero
When implementing floor division, one must handle the possibility of division by zero gracefully. In C++, attempting to divide by zero results in runtime errors or undefined behavior. It is essential to validate inputs before performing any division operation. Here’s how one might handle this situation:
#include <iostream>
#include <stdexcept>
int safe_floor_divide(int x, int y) {
if (y == 0) {
throw std::invalid_argument("Division by zero is not allowed.");
}
return (x < 0 && x % y != 0) ? (x / y) - 1 : (x / y);
}
int main() {
try {
std::cout << "Safe Floor Division: " << safe_floor_divide(10, 0) << std::endl; // Throws an error
} catch (const std::invalid_argument& e) {
std::cerr << e.what() << std::endl;
}
return 0;
}
In this snippet, the `safe_floor_divide` function checks for a zero denominator and throws an exception if an attempt to divide by zero occurs, promoting safer coding practices.
Negative Numbers in Division
Understanding how floor division processes negative numbers is crucial, as the results can sometimes be counterintuitive. Floor division with negative numbers behaves differently than with positive numbers.
Consider the following examples:
std::cout << floor_divide(-5, 2) << std::endl; // Outputs -3
std::cout << floor_divide(5, -2) << std::endl; // Outputs -3
In both cases, floor division adheres to the same logic of yielding the largest integer that is less than or equal to the result of the division.
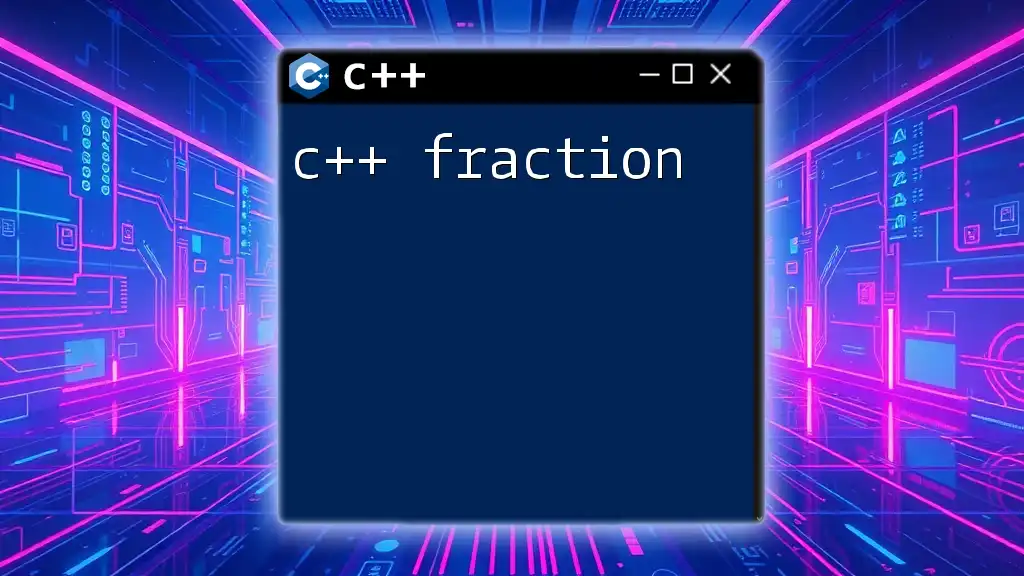
Practical Applications of Floor Division
The utility of C++ floor division extends beyond mere mathematical calculations; it significantly serves in various algorithmic contexts. For example, when implementing pagination in an application, floor division can effectively determine the total number of pages required to display a given number of items.
A practical algorithm might utilize this feature to split data or allocate resources evenly amongst available entities, ensuring that the appropriate integer values are generated regardless of whether the original data consisted of whole or fractional values.
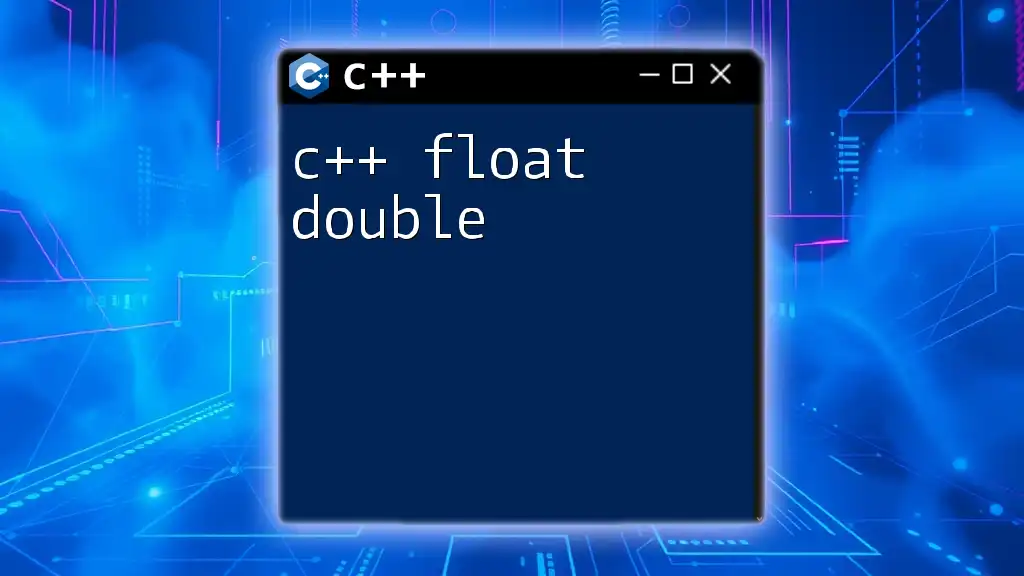
Conclusion
Mastering C++ floor division is crucial for any programmer looking to optimize code and streamline functionality. By understanding and implementing various techniques—be it through standard operators, custom functions, or careful handling of edge cases—you can enhance your ability to control integral results in mathematical operations effectively.
CCall to exploration: dive deeper into other C++ features to expand your toolkit and elevate your programming skills. The journey through C++ offers endless opportunities for optimization and improved performance. Keep experimenting and practicing!
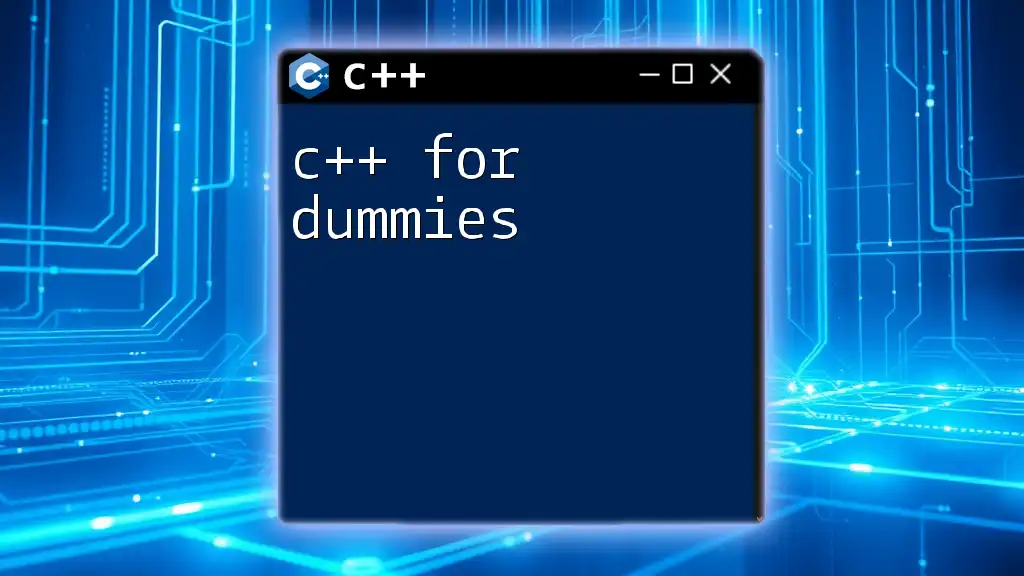
Additional Resources
To further your understanding, consider exploring recommended texts on C++ programming and online tutorials focused on mathematical operations, error handling, and advanced data types. Exploring related topics will deepen your competency, enabling you to tackle more complex programming challenges.