C++ for Dummies simplifies the foundational concepts of C++ programming, making it accessible for beginners to quickly grasp and implement basic commands.
Here's a simple "Hello, World!" program in C++:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
What is C++?
C++ is a general-purpose programming language that emerged as an extension of the C language. Developed by Bjarne Stroustrup in the early 1980s, C++ incorporates both high-level and low-level language features, allowing for efficient memory management and manipulation.
History and Evolution
C++ evolved from C in response to the need for object-oriented programming (OOP) features. The first edition of "The C++ Programming Language" was published in 1985, establishing C++ as a fundamental language in software development.
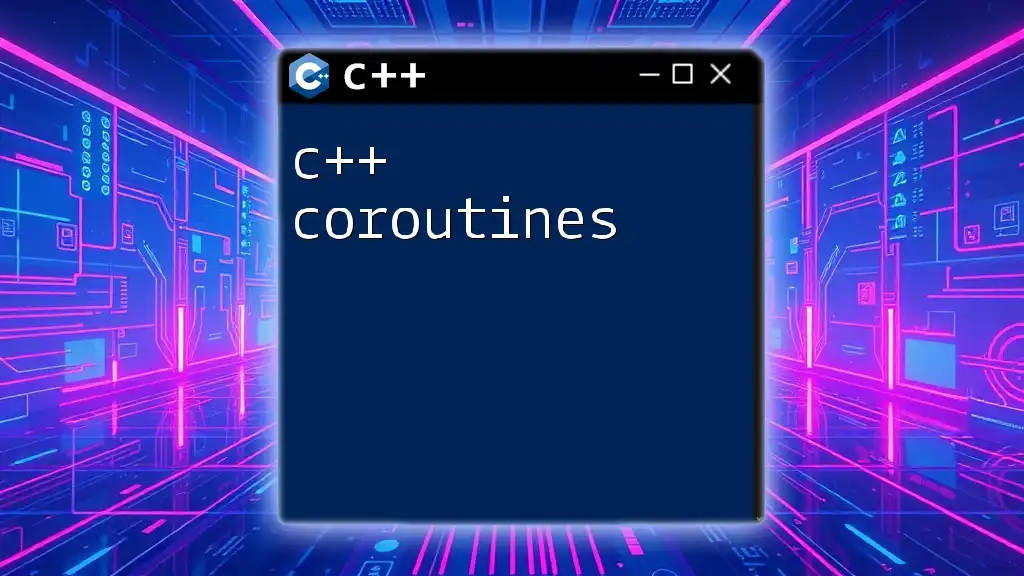
Why Learn C++?
Learning C++ opens doors to various opportunities in computer programming.
C++ is widely used in many fields, including:
- System/software development: Operating systems and compilers.
- Game development: Popular game engines like Unreal Engine are built on C++.
- Embedded systems: C++ helps program microcontrollers and embedded systems devices.
The benefits of learning C++ include:
- A deep understanding of how computers work.
- Transferable skills to other programming languages.
- A strong foundation for advanced concepts in computer science.
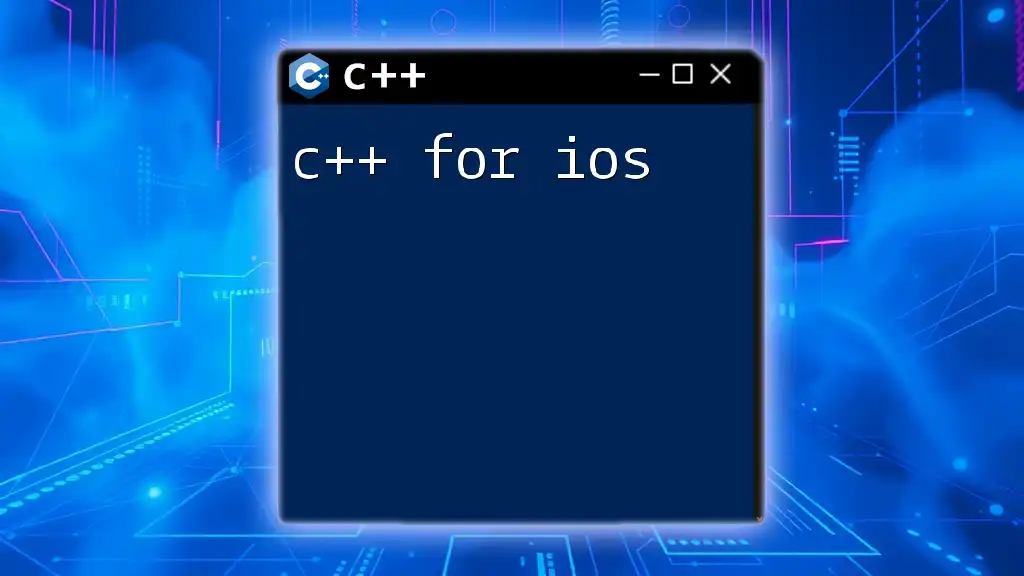
Understanding Syntax and Structure
C++ syntax can initially seem daunting, but grasping its basic structure will ease the learning process.
Basic Structure of a C++ Program
A simple C++ program generally includes the following components:
- Preprocessor Directives: Lines beginning with `#` that inform the compiler to include particular files.
- Main Function: The entry point of a C++ program where execution begins.
- Statements: Instructions that the program executes.
Here’s a code snippet illustrating a straightforward C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
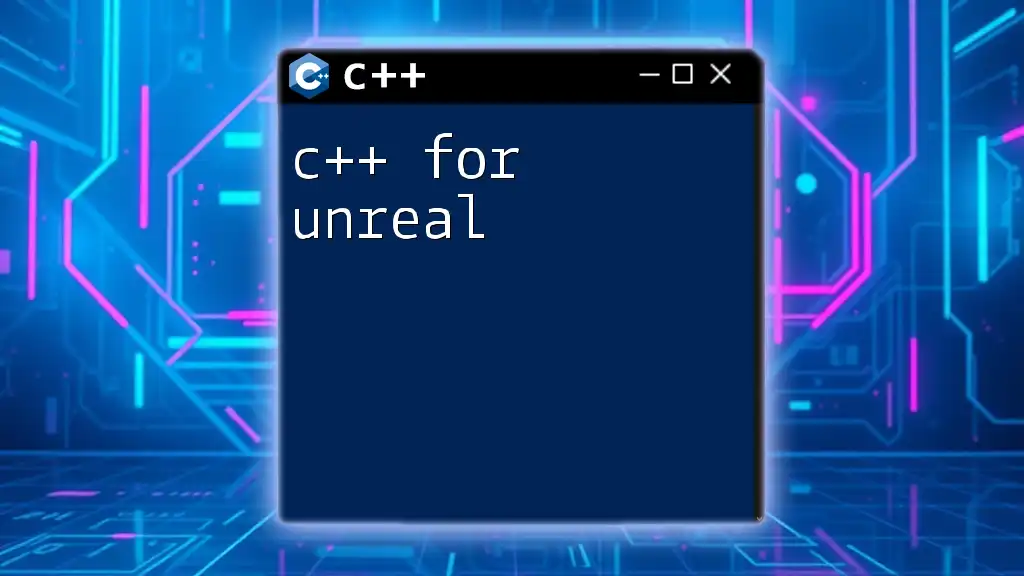
Data Types in C++
C++ has several fundamental data types that allow you to store various kinds of information.
Overview of Fundamental Data Types
- int: Used for integers.
- float: For floating-point numbers (decimals).
- char: Represents single characters.
- bool: Boolean data type, representing true or false.
Code Examples
Declaring and initializing variables can be performed as follows:
int age = 30;
float temperature = 36.5;
char initial = 'A';
bool isHappy = true;
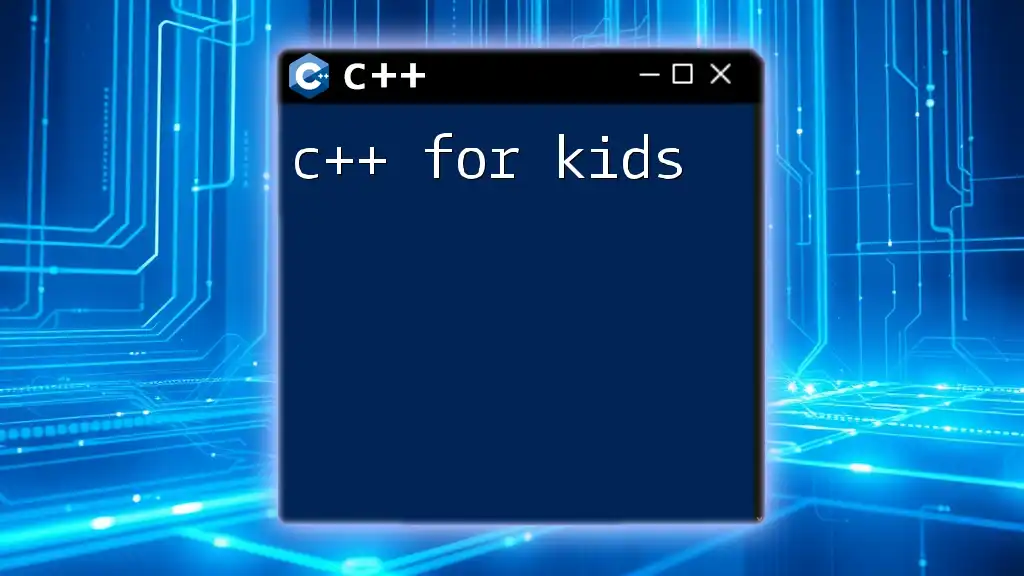
Operators in C++
C++ provides various operators to perform arithmetic, comparison, and logical operations.
Arithmetic Operators
Basic arithmetic operators include `+`, `-`, `*`, `/`, and `%`. Here’s how they function:
int a = 10, b = 20;
int sum = a + b; // 30
Comparison Operators
These operators compare two values, returning a boolean result:
bool isEqual = (a == b); // false
Logical Operators
Logical operators like `&&` and `||` are crucial for controlling program flow:
bool result = (a < b) && (a > 5); // true
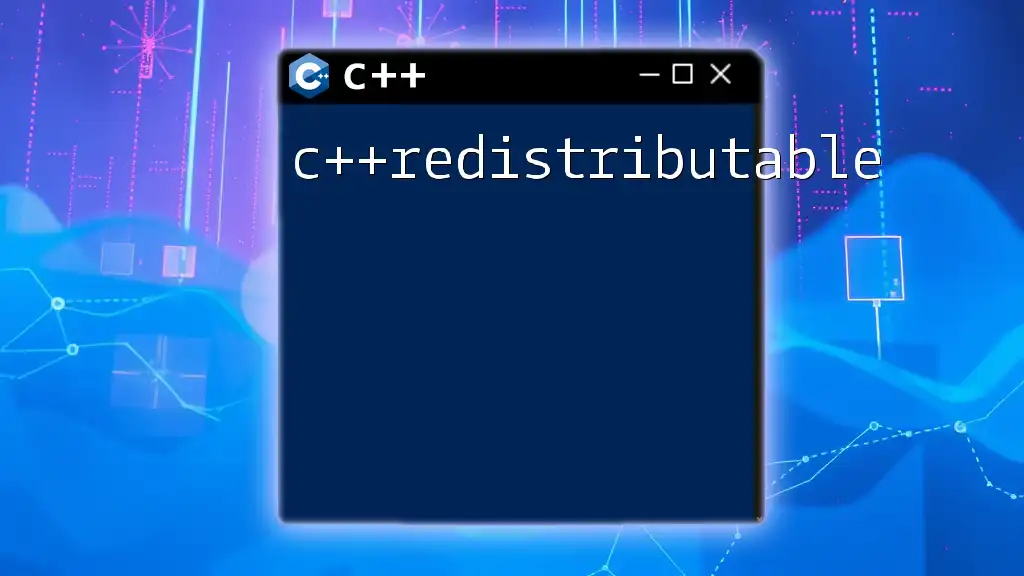
Control Flow in C++
Control flow statements direct the execution of code based on conditions.
Conditional Statements
if, else if, and else statements allow the program to execute specific blocks of code based on conditions:
if (a > b) {
cout << "A is greater than B";
} else {
cout << "A is not greater than B";
}
Switch Statements
The switch statement simplifies multiple conditions:
switch (a) {
case 1:
cout << "One";
break;
case 2:
cout << "Two";
break;
default:
cout << "Not One or Two";
}
Loops in C++
C++ supports various looping constructs for repeated execution of code.
for Loop
The for loop is used when the number of iterations is known:
for (int i = 0; i < 5; i++) {
cout << i << " ";
}
while Loop
The while loop continues until its condition evaluates to false:
int counter = 0;
while (counter < 5) {
cout << counter << " ";
counter++;
}
do...while Loop
The do...while loop guarantees that the code block executes at least once:
int i = 0;
do {
cout << i << " ";
i++;
} while (i < 5);
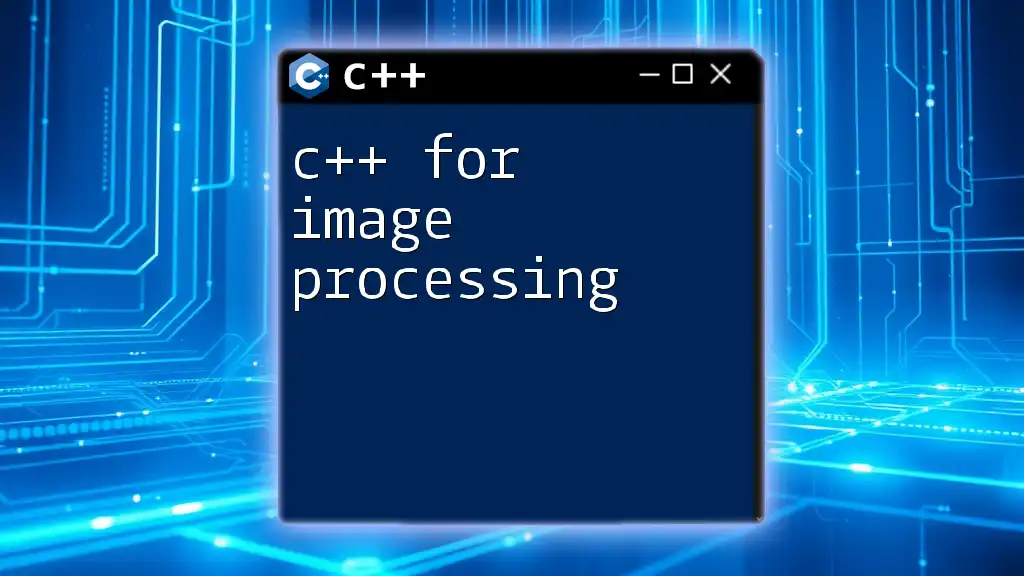
Functions in C++
Functions are reusable blocks of code that make your program modular and organized.
What are Functions?
Functions can receive inputs, perform actions, and return outputs, promoting code reuse.
Creating Functions in C++
Here is how to create a simple function in C++:
int add(int a, int b) {
return a + b;
}
Function Overloading
C++ supports function overloading, allowing you to define multiple functions with the same name but different parameters:
int add(int a, int b) {
return a + b;
}
float add(float a, float b) {
return a + b;
}
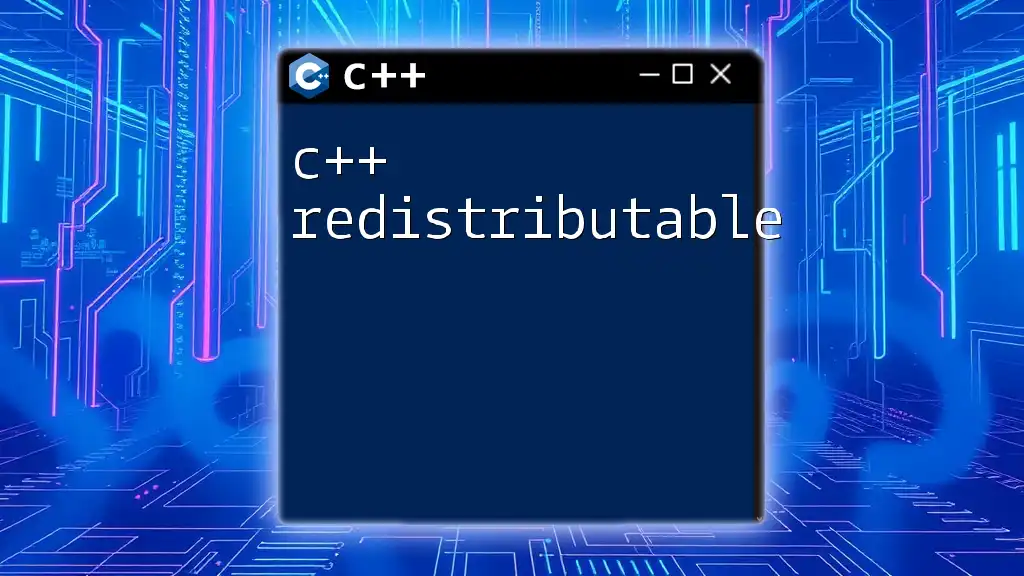
Object-Oriented Programming (OOP) in C++
OOP is a programming paradigm that empowers developers to create classes and objects.
Classes and Objects
A class is a blueprint for creating objects. Here’s a basic example:
class Car {
public:
string model;
int year;
void display() {
cout << "Model: " << model << ", Year: " << year << endl;
}
};
Inheritance
Inheritance allows one class to inherit properties from another:
class Vehicle {
public:
void info() {
cout << "This is a vehicle.";
}
};
class Bike : public Vehicle {
// Bike inherits from Vehicle
};
Polymorphism
Polymorphism allows objects to be treated as instances of their parent class, promoting flexibility in the code:
class Animal {
public:
virtual void sound() {
cout << "Some generic animal sound";
}
};
class Dog : public Animal {
public:
void sound() override {
cout << "Bark";
}
};
Encapsulation
Encapsulation restricts access to certain components of an object:
class Account {
private:
double balance;
public:
void deposit(double amount) {
balance += amount;
}
double getBalance() {
return balance;
}
};
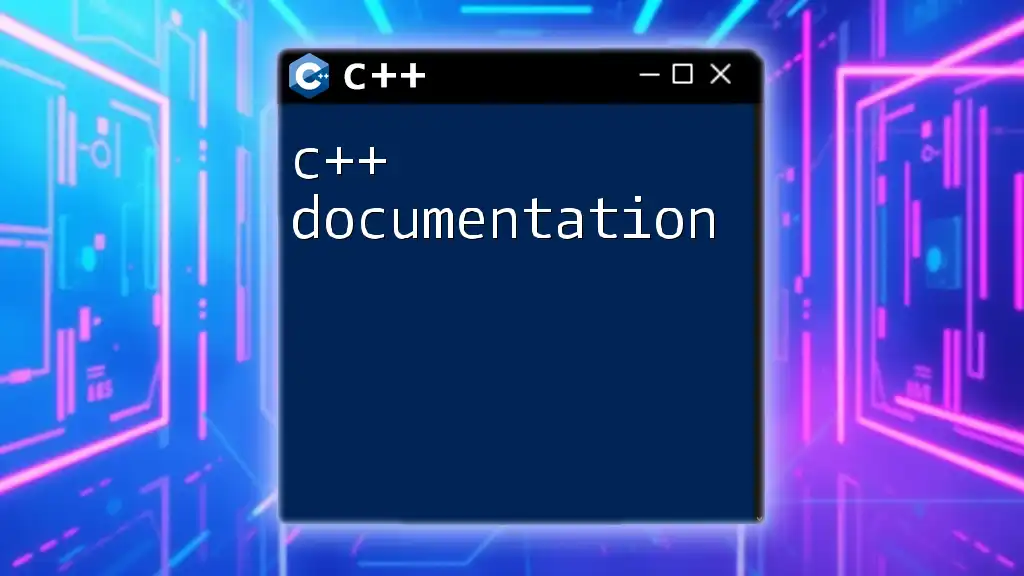
Memory Management in C++
Managing memory is crucial for efficient use of resources in C++.
Pointers and References
Pointers store memory addresses, while references provide an alias to a variable. Here's a pointer example:
int x = 10;
int* ptr = &x; // ptr holds the address of x
Dynamic Memory Allocation
C++ allows dynamic memory allocation using `new` and `delete`:
int* arr = new int[5]; // allocate an array of 5 integers
delete[] arr; // free the allocated memory
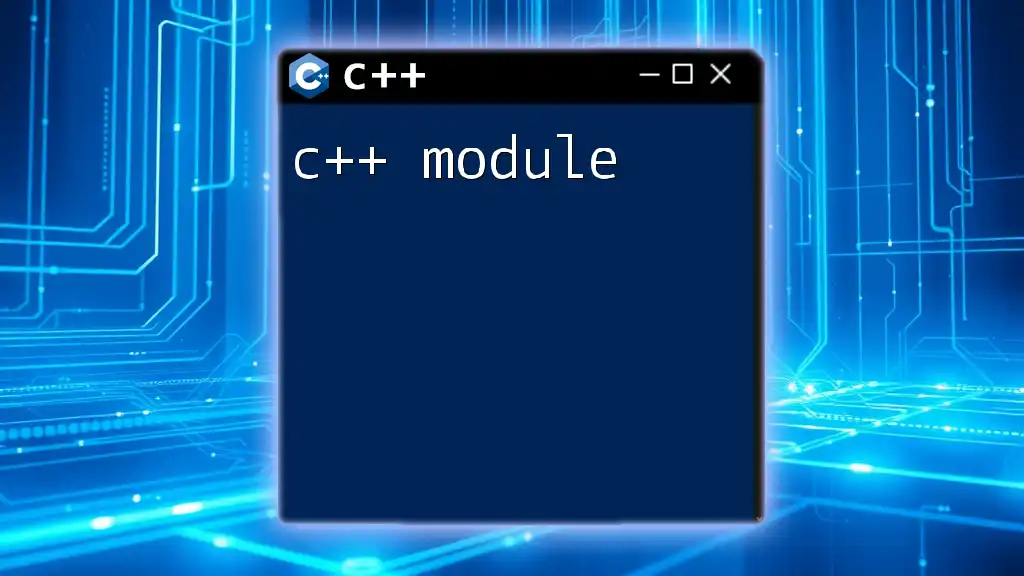
Standard Template Library (STL)
The Standard Template Library is a powerful feature of C++ that provides generic classes and functions.
What is STL?
STL includes a collection of algorithms and data structures such as vectors, lists, and maps.
Containers and Algorithms
- Vectors: Dynamic arrays that can resize themselves.
Example code for vector usage:
#include <vector>
vector<int> numbers;
numbers.push_back(10); // adding an element
- Lists and Maps: `list` provides a linked list, while `map` stores key-value pairs efficiently.
Iterators
Iterators provide a way to access elements in a container without exposing the underlying structure. Here’s an example of using iterators with a vector:
vector<int>::iterator it;
for (it = numbers.begin(); it != numbers.end(); ++it) {
cout << *it << " "; // accesses the value pointed to by the iterator
}
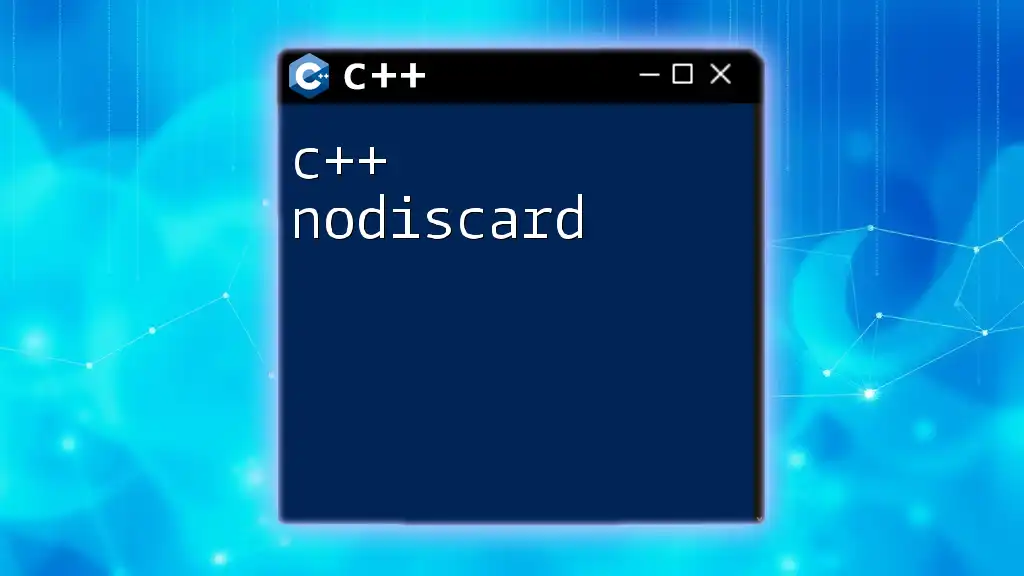
Common C++ Mistakes and Troubleshooting
As a beginner in C++, making mistakes is part of the learning experience. Here’s how to handle common errors.
Syntax Errors vs. Logic Errors
Syntax Errors occur when the language rules are violated, while Logic Errors happen without any syntax problems but produce incorrect results. For example, missing a semicolon (`;`) leads to a syntax error.
Debugging Techniques
Debugging is essential to find and fix issues in your code. Here’s how you can effectively debug:
- Use print statements to trace your code execution.
- Leverage debugging tools like GDB or integrated debuggers in IDEs.
Frequently Encountered Issues
Common issues include:
- Forgetting to initialize variables.
- Mismatched data types in function calls.
- Misusing pointers leading to segmentation faults.
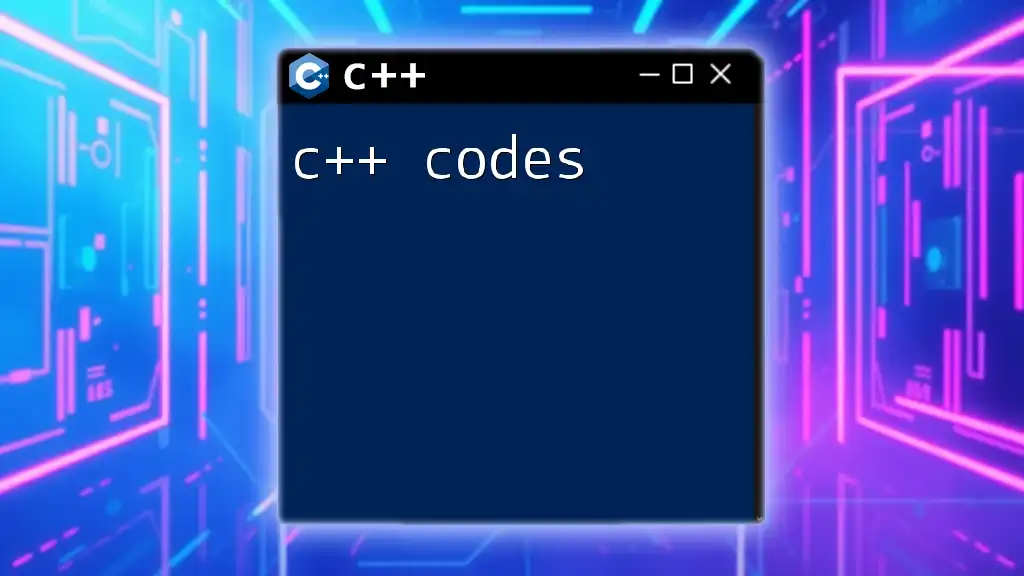
Conclusion
C++ is a powerful and versatile language, essential for many fields in software development. Understanding its syntax, data types, control structures, and various concepts like OOP and pointers is crucial for budding programmers. Don't forget to practice consistently and explore the wealth of resources available to deepen your knowledge of C++. The journey may seem daunting at times, but mastering C++ will significantly enhance your programming capabilities and career prospects.
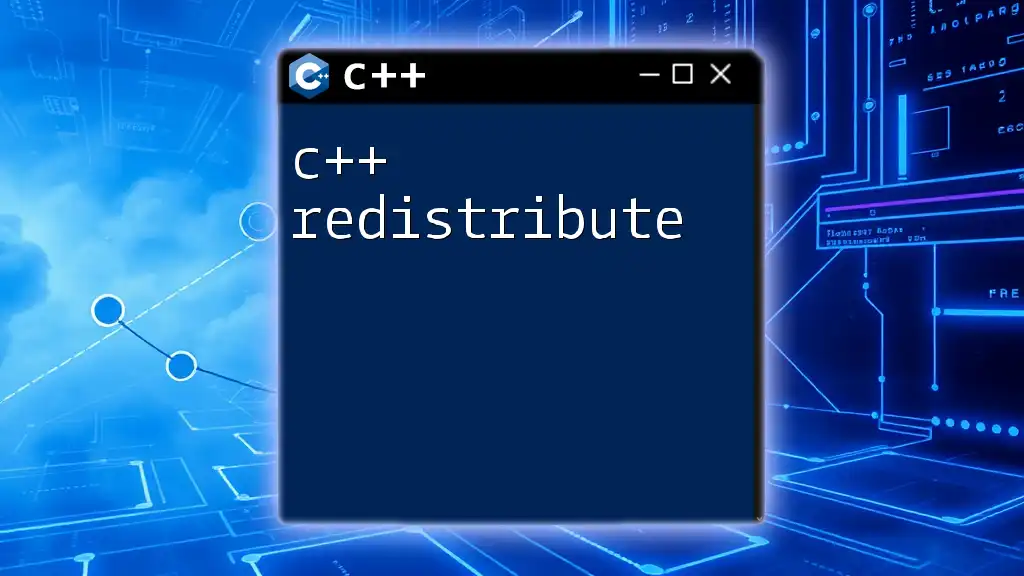
Additional Resources
For further learning, consider exploring the following resources:
- Books: "The C++ Programming Language" by Bjarne Stroustrup.
- Websites: TutorialsPoint, GeeksforGeeks.
- Online Courses: Coursera, edX, and Udemy offer excellent C++ courses.
- Community: Engage with C++ forums and support groups for help and networking.