C++ on Mac allows developers to efficiently write and compile programs using tools like Xcode or command-line utilities, enabling a seamless coding experience.
Here’s a simple code snippet that demonstrates a basic "Hello, World!" program in C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Understanding C++ and Its Importance
C++ is a powerful programming language known for its flexibility and performance. It belongs to the family of object-oriented languages and adds features from both procedural and object-oriented programming paradigms. This makes C++ a versatile choice for a variety of applications, from system software to game development.
The unique features of C++ that make it particularly appealing include:
- High Performance: C++ is often used in applications where speed and resource efficiency are critical, such as game engines and real-time simulations.
- Portability: Code written in C++ can be compiled and run on multiple platforms, including macOS, Windows, and Linux, making it a great choice for cross-platform development.
- Rich Standard Library: The C++ Standard Library provides many built-in functions and classes, which simplifies coding and enhances productivity.
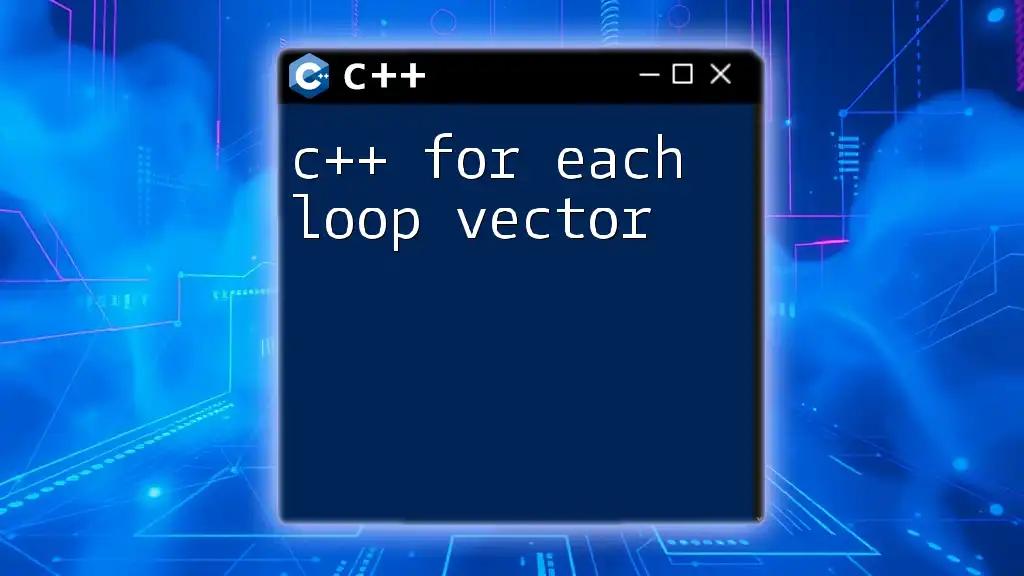
Why Use C++ on macOS?
Choosing C++ for development on macOS comes with several advantages:
- Performance: macOS is built on Unix, allowing C++ applications to run efficiently and take advantage of the powerful hardware architecture of Apple devices.
- Native App Development: For developers looking to create native macOS applications, C++ offers integration with macOS APIs and frameworks, resulting in more responsive and resource-friendly applications.
- Industry Relevance: Many industries, including gaming, finance, and scientific research, utilize C++ for its speed and reliability. As such, learning C++ can open doors to numerous career opportunities.
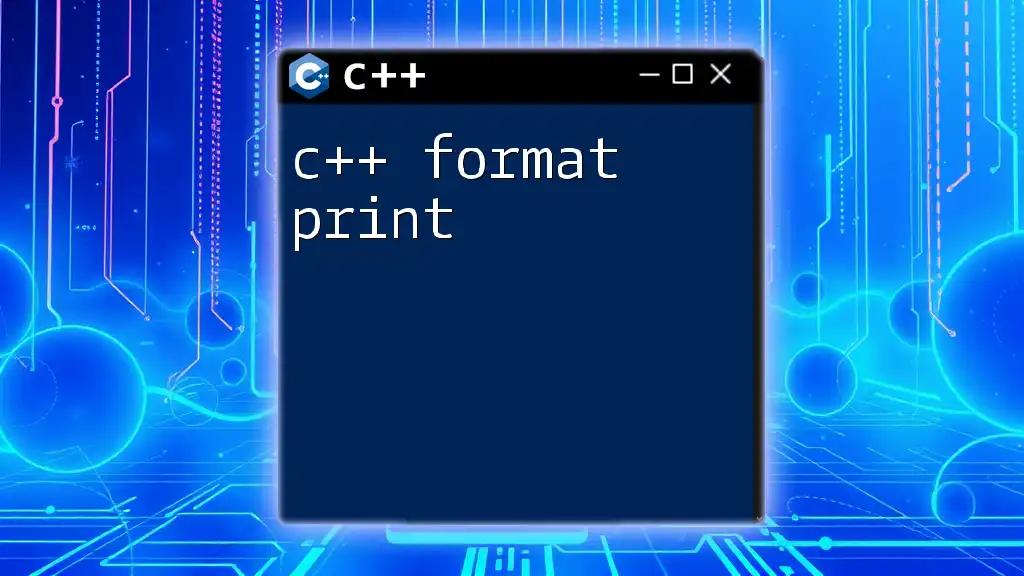
Setting Up Your Development Environment
Installing Xcode
To start developing C++ applications on macOS, the first step is to install Xcode, which is Apple's official integrated development environment (IDE).
- Download Xcode: You can download Xcode for free from the Mac App Store.
- Install: Once downloaded, follow the installation prompts. After installation, open Xcode and agree to the license agreement.
Installing Command Line Tools
Xcode comes with a robust set of tools, but you'll also need the Command Line Tools for compiling C++ code directly in the terminal.
- Open your Terminal application and enter the following command:
xcode-select --install
- This command initiates the installation of the necessary command-line tools, which include compilers and libraries vital for C++ development.
Using Homebrew to Install Additional Tools
Homebrew is a popular package manager for macOS that simplifies the process of managing software. It allows you to install additional development tools for C++.
- Install Homebrew: Open Terminal and execute:
/bin/bash -c "$(curl -fsSL https://raw.githubusercontent.com/Homebrew/install/HEAD/install.sh)"
- Install gcc and clang: After Homebrew is set up, you can install the GNU Compiler Collection (gcc) and clang, which are essential for compiling C++ code:
brew install gcc brew install llvm
Using these tools provides greater flexibility and control over your C++ programming environment.

Writing Your First C++ Program on macOS
Creating a Simple C++ Project
Now that your development environment is set up, let’s create a simple C++ project using Xcode. This will familiarize you with the development process on macOS.
- Open Xcode: Launch Xcode and select "Create a new Xcode project".
- Choose Template: Select the "Command Line Tool" under MacOS.
- Set Project Details: Give your project a name (e.g., `HelloWorld`) and ensure the language is set to C++.
Example: Hello World Program
The first program most developers write is the classic "Hello, World!" program. Here’s how to do it:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Explanation:
- `#include <iostream>`: This preprocessor directive includes the input/output stream library required for using `std::cout`.
- `int main()`: This is the main function where program execution begins.
- `std::cout`: This is used to output text to the console.
- `return 0;`: This line indicates that the program finished successfully.
Compiling and Running Your Program
To compile and run your C++ program in Xcode:
- Build the Project: Click on the 'Product' menu and select 'Build' (or simply press `Command + B`).
- Run the Program: To run the compiled program, click the 'Run' button or press `Command + R`.
Alternatively, you can compile and run your program from the terminal:
- Navigate to your project directory:
cd path/to/HelloWorld
- Compile the code using g++:
g++ main.cpp -o hello
- Execute the compiled program:
./hello
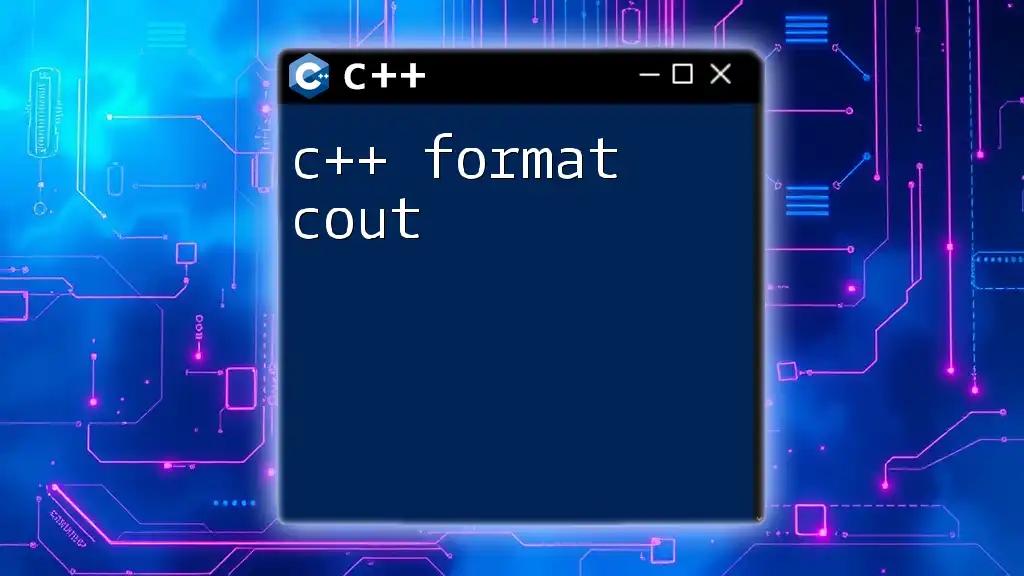
Understanding C++ Syntax and Features
Data Types and Variables
C++ offers several built-in data types:
- int: Used for integer values.
- float: Represents floating-point numbers.
- char: Used for character data.
Variable declaration and initialization are straightforward in C++. For instance:
int age = 25;
float height = 5.9;
char initial = 'A';
Control Structures
C++ supports various control structures that dictate the flow of execution.
- If-Else Statements:
if (age >= 18) {
std::cout << "Adult" << std::endl;
} else {
std::cout << "Minor" << std::endl;
}
- Loops:
- For Loop:
for (int i = 0; i < 5; i++) { std::cout << i << std::endl; }
- While Loop:
int count = 0; while (count < 5) { std::cout << count << std::endl; count++; }
Functions in C++
Functions are reusable blocks of code in C++. They allow you to encapsulate logic and can either return a value or just perform an action.
Example of a Simple Function:
int add(int a, int b) {
return a + b;
}
This function takes two integers as input and returns their sum.
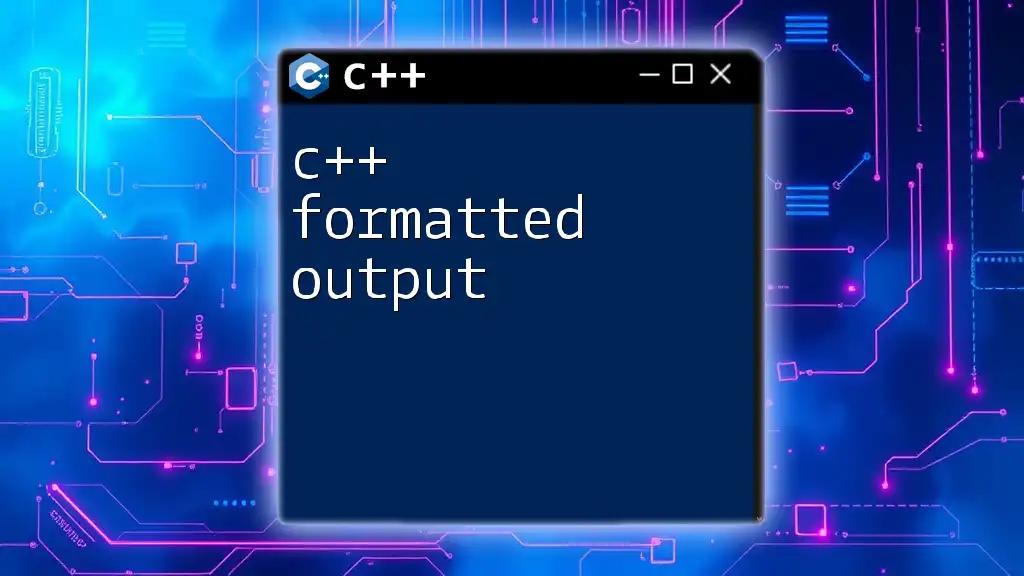
Advanced Topics in C++ on macOS
Object-Oriented Programming (OOP) Concepts
Object-oriented programming is a powerful paradigm supported by C++. It emphasizes concepts such as:
- Classes and Objects: Class is a blueprint for creating objects.
- Encapsulation: Hides the internal state of an object and only exposes a controlled interface.
- Inheritance: A mechanism to create a new class based on an existing class, promoting code reusability.
Example of a Class Implementation:
class Animal {
public:
void speak() {
std::cout << "Animal speaks!" << std::endl;
}
};
class Dog : public Animal {
public:
void bark() {
std::cout << "Dog barks!" << std::endl;
}
};
Using Libraries and Frameworks
C++ has a rich ecosystem of libraries that developers can leverage to accelerate development. The Standard Template Library (STL) is widely used for data structures and algorithms.
Example of Including and Using a Library:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
In this example, we've included the STL `vector` library, which simplifies array handling by providing dynamic array capabilities.
Debugging C++ Programs on macOS
Debugging is an essential skill for any developer. Xcode provides a sophisticated debugging tool that allows you to set breakpoints, watch variables, and inspect memory. Using terminal tools like `gdb` is also viable for debugging C++ code:
gdb ./hello
You can then use debugging commands to step through your code and inspect values.
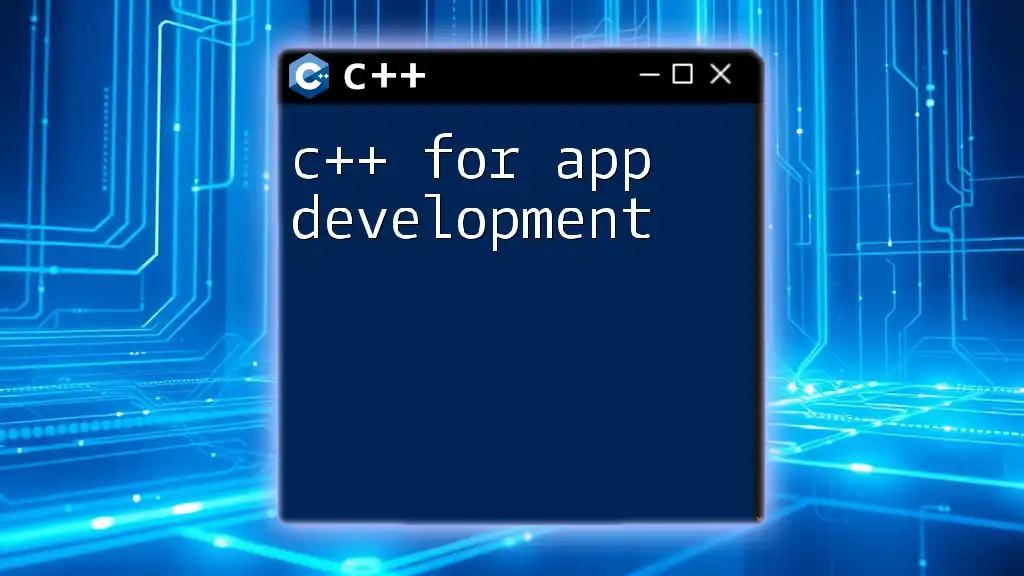
Resources for Learning C++ on macOS
Recommended Books and Tutorials
To continue your learning, consider exploring reputable books and online tutorials:
- Books: Look for classics like "C++ Primer" by Lippman or "Effective C++" by Meyers.
- Online Tutorials: Websites like Codecademy, GeeksforGeeks, and the official C++ documentation provide tutorials and exercises.
Online Communities and Forums
Joining online communities can significantly boost your learning. Resources like Stack Overflow and Reddit's r/cpp provide platforms to ask questions, share knowledge, and engage with fellow C++ enthusiasts.
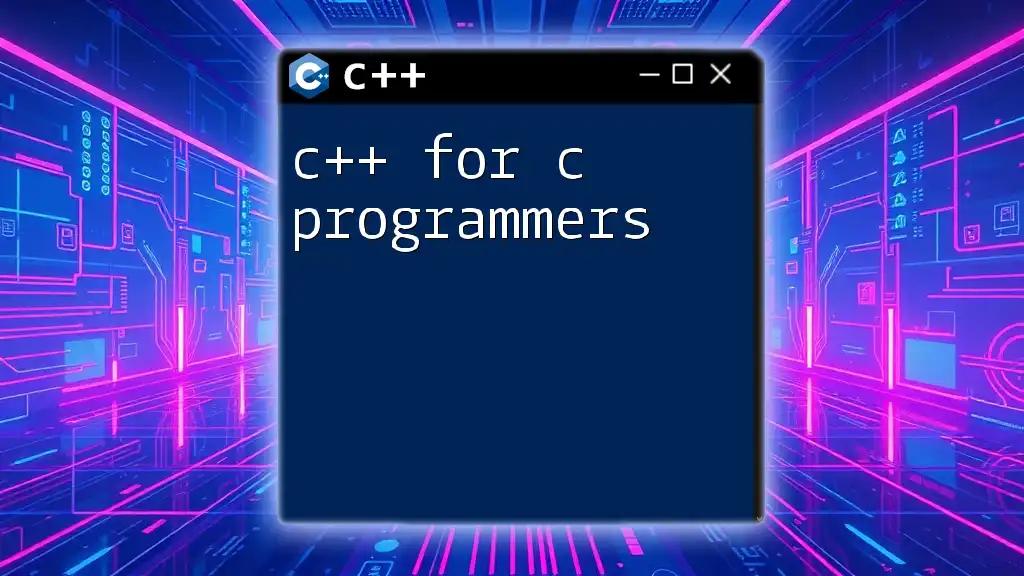
Final Thoughts
Mastering C++ on macOS can be a rewarding experience. With its vast capabilities and industry relevance, C++ knowledge is a valuable asset in the tech world. Keep practicing, explore advanced topics, and don’t hesitate to engage with the community for support.
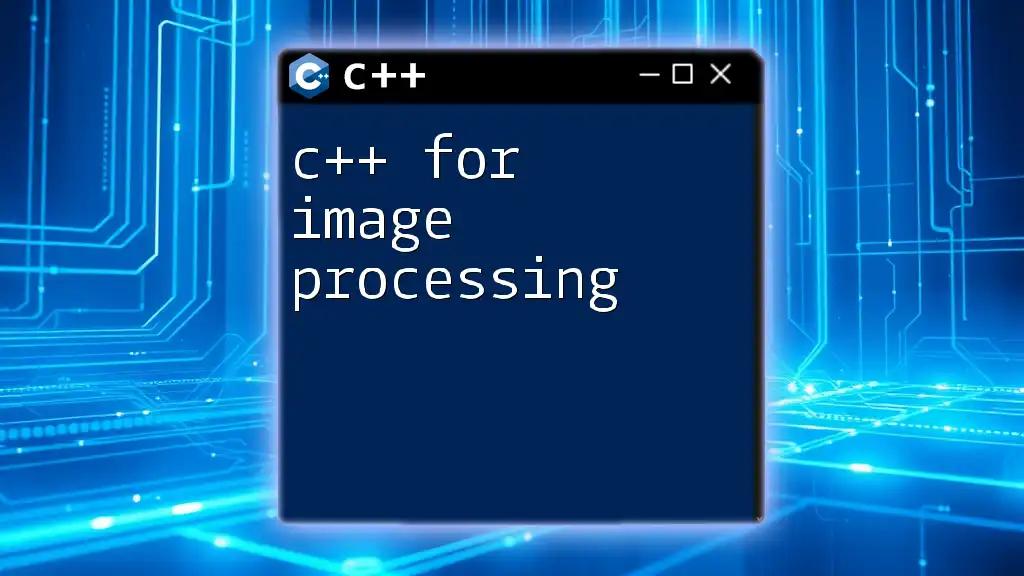
Call to Action
Consider subscribing to newsletters and following forums dedicated to C++ development. Engaging with the community will keep you updated on the latest trends and best practices. Feel free to leave comments or ask questions about your C++ journey on macOS, and take the first step toward becoming a proficient C++ developer!