C++ for iOS allows developers to leverage the power of C++ for performance-critical code, enabling seamless integration with Apple’s frameworks through the use of Objective-C++.
// Example of a C++ function used in an iOS application
#include <iostream>
extern "C" void displayMessage() {
std::cout << "Hello from C++!" << std::endl;
}
Understanding C++ on iOS
What is C++?
C++ is a powerful general-purpose programming language that has become a staple in software development due to its performance and versatility. It succeeds C and adds features such as classes, polymorphism, and operator overloading, enabling developers to write complex applications efficiently. With its rich standard library, C++ allows developers to manage low-level system resources while still benefiting from high-level abstractions, making it ideal for systems programming, game development, and applications requiring intricate hardware interaction.
Why Use C++ on iOS?
Using C++ for iOS development brings several advantages:
- Cross-Platform Compatibility: By writing core logic in C++, you can reuse code across multiple platforms, including Android and Windows, making your workflow more efficient.
- Performance Efficiency: C++ is known for its speed and low-level performance optimization, which is critical for computationally intensive applications like games and multimedia processing.
- Code Reuse in Large Projects: When working in teams or on large projects, C++ allows multiple developers to work on different components concurrently without significant integration issues, ensuring a modular and scalable architecture.
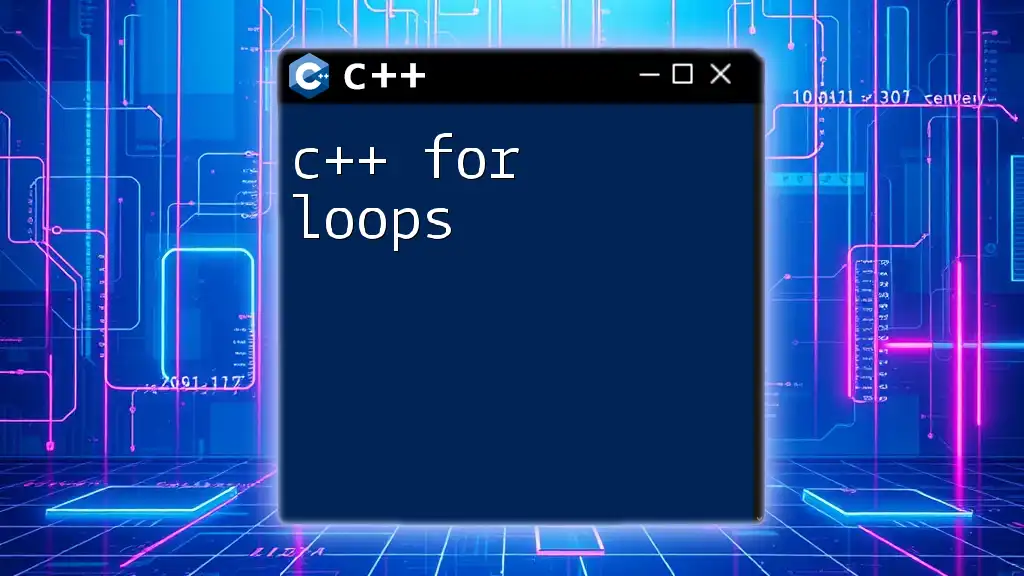
Setting Up Your Environment
Required Tools for C++ Development on iOS
To develop C++ for iOS, you need specific tools:
- Xcode: Apple's official IDE for macOS that supports development for all Apple platforms, including iOS. It comes with robust support for C++.
- Command Line Tools: Command line tools are essential for building and running C++ applications, allowing for flexibility and automation through scripts.
- Additional Libraries and Frameworks: Depending on your project's needs, you might need libraries such as Boost for advanced features or Qt for cross-platform GUIs.
Creating a New Xcode Project with C++
Setting up a new Xcode project for C++ on iOS is straightforward:
- Starting Xcode: Launch Xcode and select "Create a new Xcode project."
- Selecting the Appropriate Project Template: Choose a template such as "iOS App" and configure for C++ usage by selecting the relevant options.
- Configuring Project Settings for C++ Compatibility: Make sure to set the project file type to use Objective-C++ if you plan to combine C++ with Objective-C.
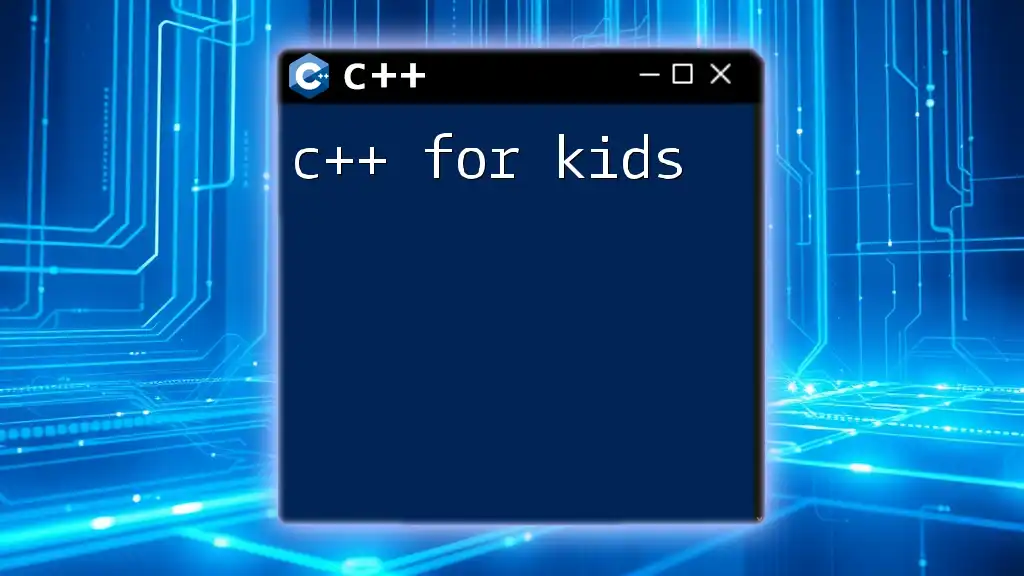
Writing C++ Code in Xcode
Basic C++ Syntax Refresher
Before delving deeper, let’s review some fundamental syntax features in C++:
- Variables: C++ has strong typing; every variable must be declared with a data type, such as int, float, or std::string.
- Control Structures: Use if-else statements, for loops, and while loops to manage the flow of your program.
- Functions: Functions can return values and can take parameters of different types.
Here’s a simple example of a C++ program that prints "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
Interfacing C++ with Objective-C
Objective-C is the primary programming language for iOS applications, and integrating it with C++ can enhance functionality. Objective-C++ enables you to mix both languages by renaming your `.m` files to `.mm`.
Using Objective-C++, you can call C++ functions seamlessly within your Objective-C code. Below is an example of how to bridge C++ and Objective-C:
// Objective-C Code:
#import "YourClass.mm"
@implementation YourClass {
MyCPPClass *cppObj;
}
- (void)useCPP {
cppObj = new MyCPPClass();
cppObj->cppFunction();
}
In this example, `MyCPPClass` is a standard C++ class, and `cppFunction()` is a method within that class. This approach paves the way for creating hybrid applications that leverage the strengths of both languages.
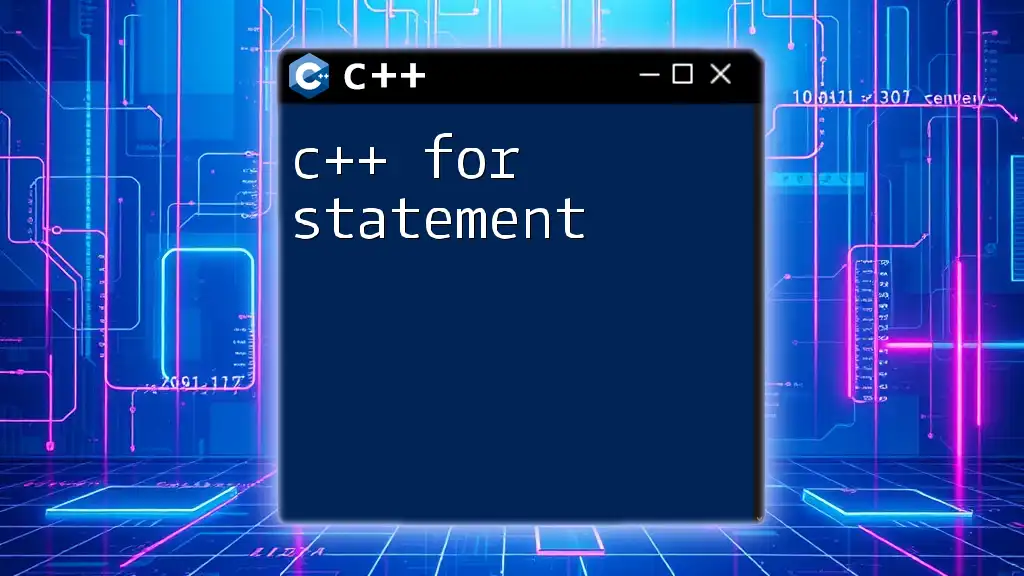
Building and Compiling C++ Projects for iOS
Compiling C++ Code in Xcode
Compiling C++ code in Xcode requires familiarity with the build system. When you create or add a new C++ file to your project, ensure that:
- The file has a `.cpp` or `.mm` extension.
- The Compile Sources build phase includes your C++ files.
- C++11 or C++14 standards are selected in your project settings for modern features.
Debugging C++ Code on iOS
Xcode offers sophisticated debugging tools, including breakpoints, a watch window, and code stepping. Use these tools to diagnose issues quickly. Ensure you utilize logging effectively when working with complex C++ code, making it easier to track program flow and variable states.
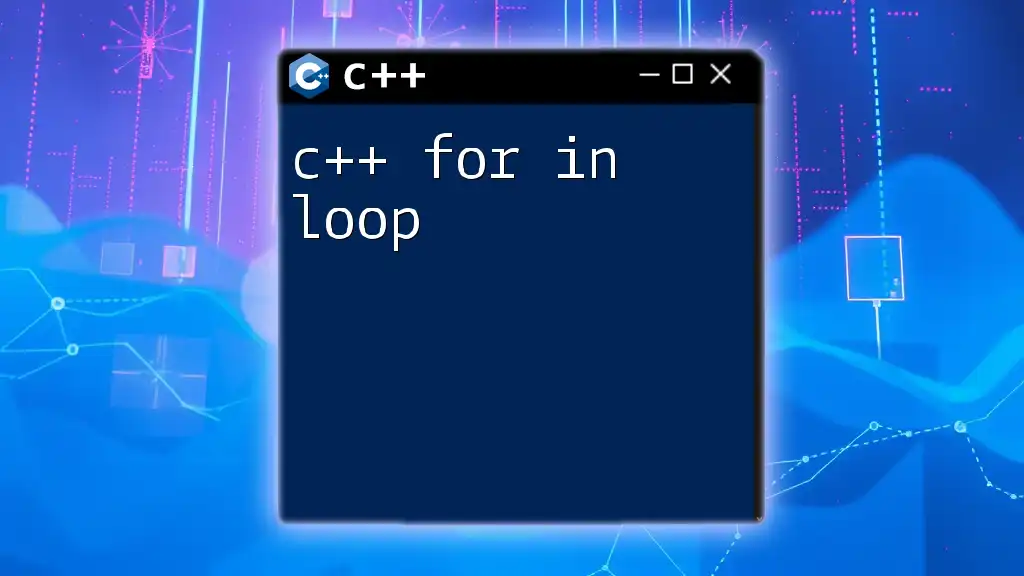
Best Practices for C++ Development on iOS
Code Organization
Organizational strategies are critical for maintaining a clear structure in larger projects. Adopt a modular approach; separate classes or functions into distinct files and use namespaces to prevent naming collisions.
Error Handling and Memory Management
Efficient error handling is vital. Use exception handling judiciously. For memory management, prefer smart pointers (like `std::unique_ptr` and `std::shared_ptr`) over raw pointers to prevent memory leaks and ensure automatic resource management.
Performance Optimization Tips
Profiling your C++ code is essential for identifying bottlenecks. Xcode's instruments can help track CPU usage and memory allocation. Use efficient algorithms and data structures that are optimized for performance, such as hash tables for quick access.
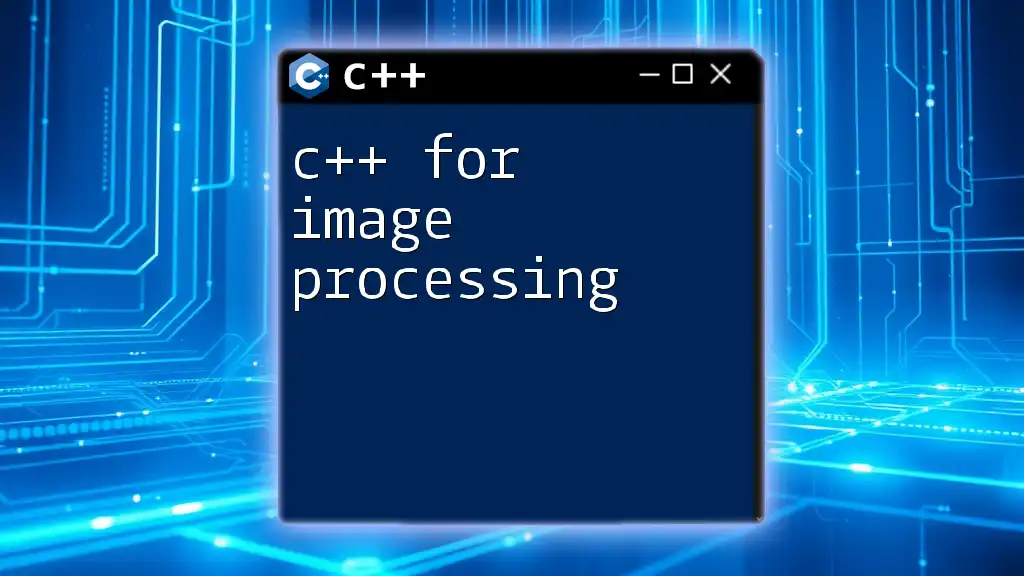
Advanced Topics
Using C++ Libraries on iOS
Integrating third-party libraries can amplify the capabilities of your C++ projects. Libraries such as Boost or OpenCV bring powerful features and save development time. Consult the library's documentation for specific integration steps to ensure compatibility with Xcode.
Game Development with C++ on iOS
C++ shines in game development, particularly with engines like Unreal Engine that are optimized for high performance. Understanding the game loop is crucial in this area:
void gameLoop() {
while (playing) {
handleInput();
updateGame();
renderFrame();
}
}
In this basic structure, the game loop constantly processes input, updates game logic, and renders the display, allowing for dynamic and responsive gameplay.
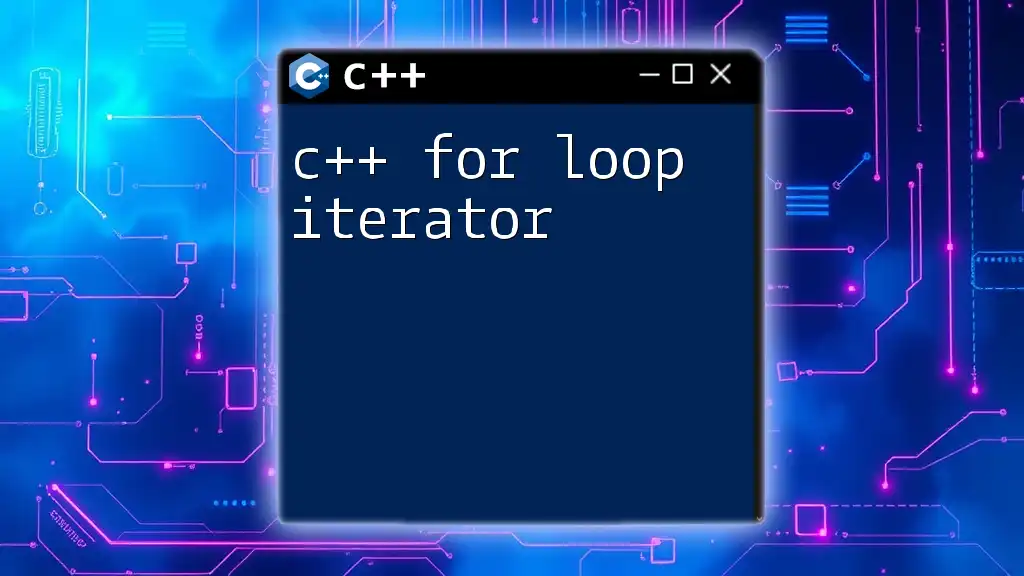
Conclusion
Embracing C++ for iOS development can significantly enhance your programming capabilities. The cross-platform efficiency, performance, and rich ecosystem of libraries provide a solid foundation for building powerful applications. As you explore and practice more with C++, you will discover its numerous applications within the iOS development environment.
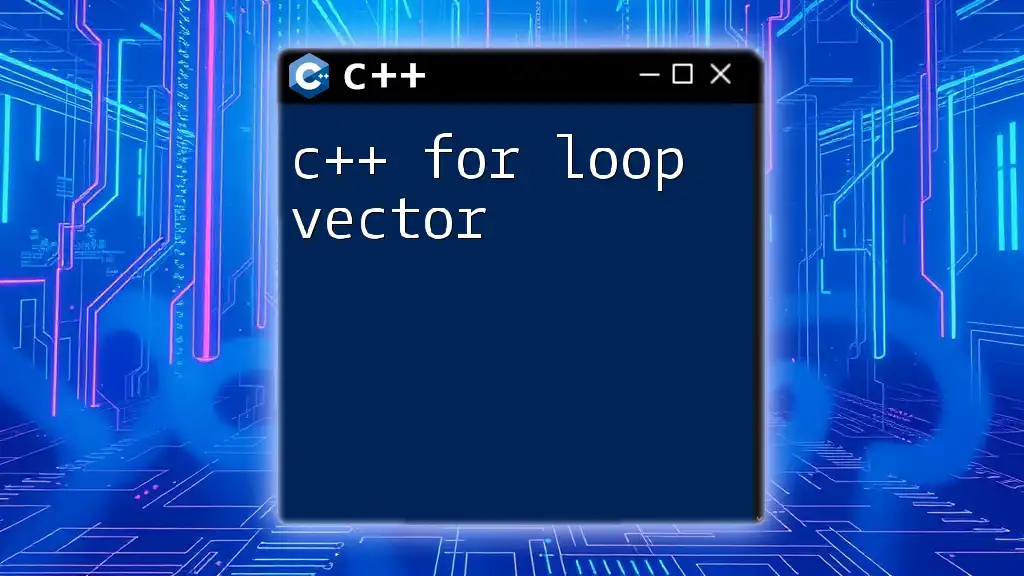
Call to Action
To continue your journey in mastering C++ and iOS development, subscribe to our blog for more articles and join our community discussions to share insights and best practices with fellow developers. Dive into the world of C++ for iOS and unlock the potential of your programming skills!