In C++, a for loop with an index allows you to iterate through a range of values, typically using an integer variable to access elements in an array or to execute a block of code a specific number of times.
Here’s an example code snippet:
#include <iostream>
int main() {
for (int i = 0; i < 5; i++) {
std::cout << "Index: " << i << std::endl;
}
return 0;
}
Understanding Loops in C++
What is a Loop?
In programming, a loop is a fundamental concept that allows developers to execute a block of code multiple times until a specified condition is met. By utilizing loops, you can effectively handle repetitive tasks with minimal redundancy and enhanced efficiency.
Why Use a For Loop?
Among the various types of loops available in C++, the for loop stands out for its ability to execute a sequence of commands a predetermined number of times. This predictability makes for loops particularly useful in scenarios such as iterating over arrays, performing calculations a specific number of times, or controlling program flow in structures like tables.
In comparison to other looping constructs, such as while loops and do-while loops, for loops provide a more compact syntax that integrates initialization, condition-checking, and iteration progression in a single line. This clarity can significantly improve the readability of your code.
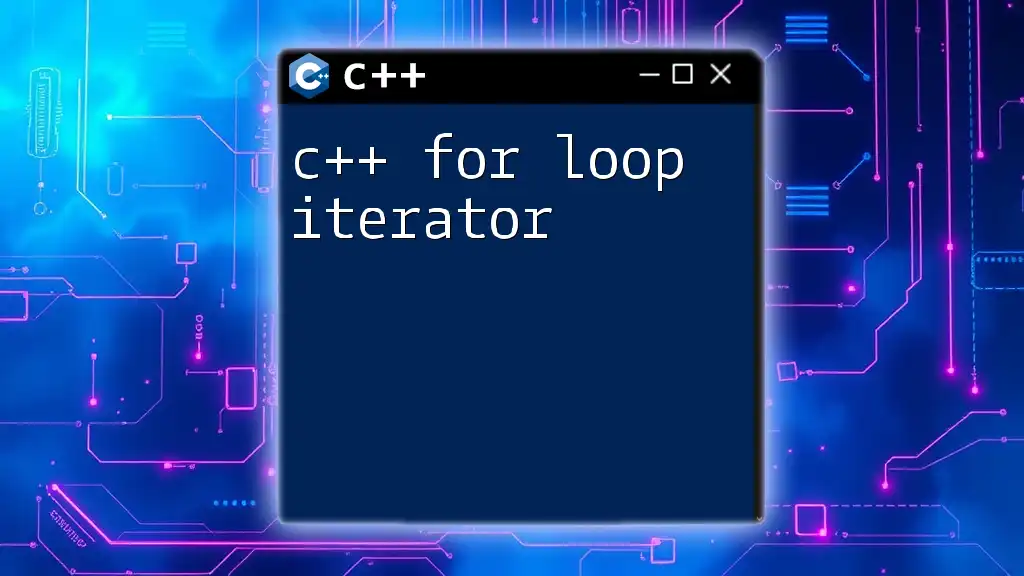
The Anatomy of a For Loop
Structure of a For Loop
The fundamental syntax of a for loop consists of three key components:
for(initialization; condition; increment/decrement) {
// code block to be executed
}
-
Initialization: This step sets up any necessary variables for the loop, often defining the loop counter.
-
Condition: This is a Boolean expression evaluating whether the loop should continue executing. If the condition evaluates to `true`, the loop continues; if it evaluates to `false`, the loop terminates.
-
Increment/Decrement: This portion updates the loop variable, typically increasing or decreasing its value on each iteration.
Example of a Simple For Loop
Consider this basic implementation of a for loop in C++:
#include<iostream>
using namespace std;
int main() {
for(int i = 0; i < 5; i++) {
cout << "Index: " << i << endl;
}
return 0;
}
In this example, the loop initializes an integer variable `i` to 0. It checks whether `i` is less than 5, and if so, it executes the block of code that outputs the current value of `i`. After each execution, `i` is incremented by 1. The expected output will be:
Index: 0
Index: 1
Index: 2
Index: 3
Index: 4
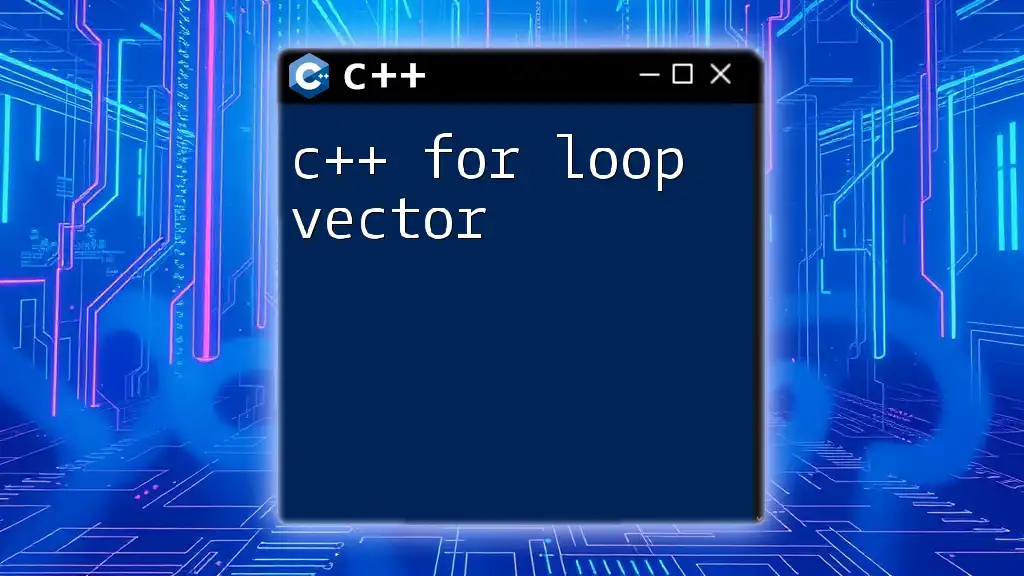
Using Indices in For Loops
What is an Index?
An index is a critical concept in programming, particularly when working with data structures like arrays, vectors, and other collections. It allows you to access specific elements or positions within these collections. In C++, indices are zero-based, meaning that the first element is accessed with an index of 0.
Accessing Array Elements with For Loops
One of the prominent uses of the C++ for loop with index is to iterate over arrays. By using indices, you can efficiently traverse each element. For example:
#include<iostream>
using namespace std;
int main() {
int arr[] = {10, 20, 30, 40, 50};
for(int i = 0; i < 5; i++) {
cout << "Element at index " << i << ": " << arr[i] << endl;
}
return 0;
}
In this example, the program initializes an array of integers. The for loop uses the index `i` to access each element, iterating from 0 through 4. The output will demonstrate the traversal of the array, indicating the element at each index:
Element at index 0: 10
Element at index 1: 20
Element at index 2: 30
Element at index 3: 40
Element at index 4: 50
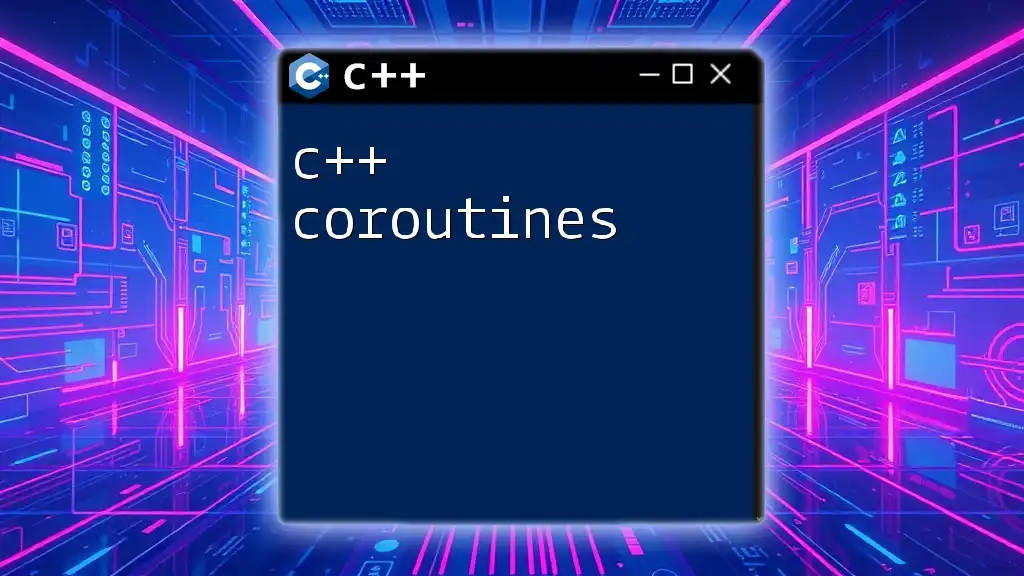
Common Use Cases for For Loops
Iterating Over Collections
For loops are excellent for iterating over different types of collections in C++, such as std::vector, std::array, and std::list. These data structures frequently utilize indices to access their contained elements.
Nested For Loops
A nested for loop is a powerful construct that allows you to use one for loop inside another. This is particularly useful when handling multi-dimensional arrays or matrices. Consider the following example:
#include<iostream>
using namespace std;
int main() {
for (int i = 1; i <= 3; i++) {
for (int j = 1; j <= 3; j++) {
cout << "i: " << i << ", j: " << j << endl;
}
}
return 0;
}
Here, the outer loop iterates over `i`, while the inner loop iterates over `j`. Each time the outer loop executes, the inner loop runs through its complete cycle, leading to a total of 9 iterations in the output:
i: 1, j: 1
i: 1, j: 2
i: 1, j: 3
i: 2, j: 1
i: 2, j: 2
i: 2, j: 3
i: 3, j: 1
i: 3, j: 2
i: 3, j: 3
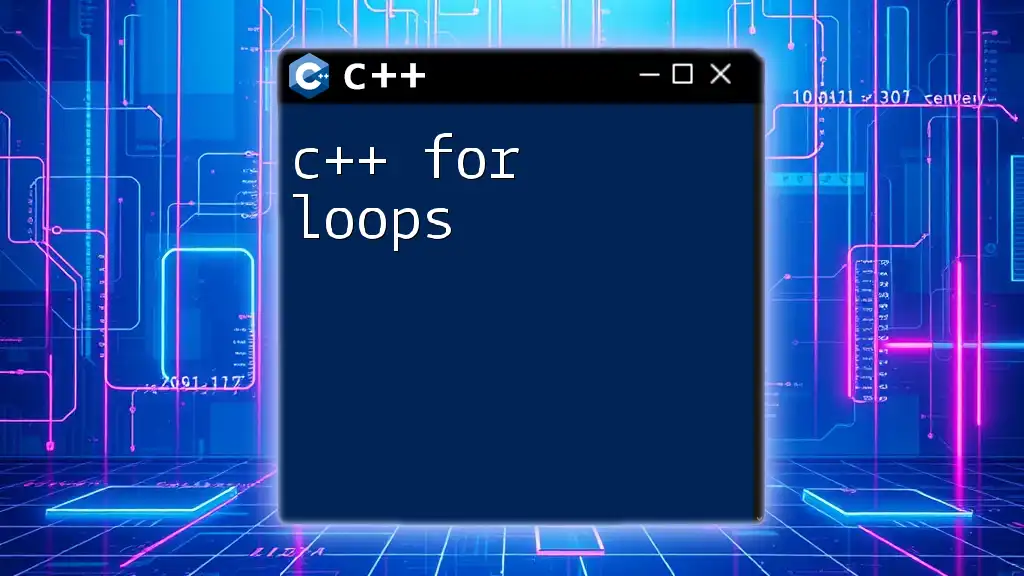
Practical Tips for Using For Loops
Performance Considerations
While loops are powerful, proper utilization is paramount for optimal performance. Always ensure your loop has a clear terminating condition to prevent infinite loops. Additionally, strive to minimize the work done inside the loop to improve performance.
Debugging and Testing
Debugging for loops can sometimes be tricky. Use debugging tools to step through your code and confirm that your loop behaves as expected. It is crucial to test the boundaries of your loops to ensure robustness—make sure to validate that your indices do not exceed the limits of the data structures you’re working with.
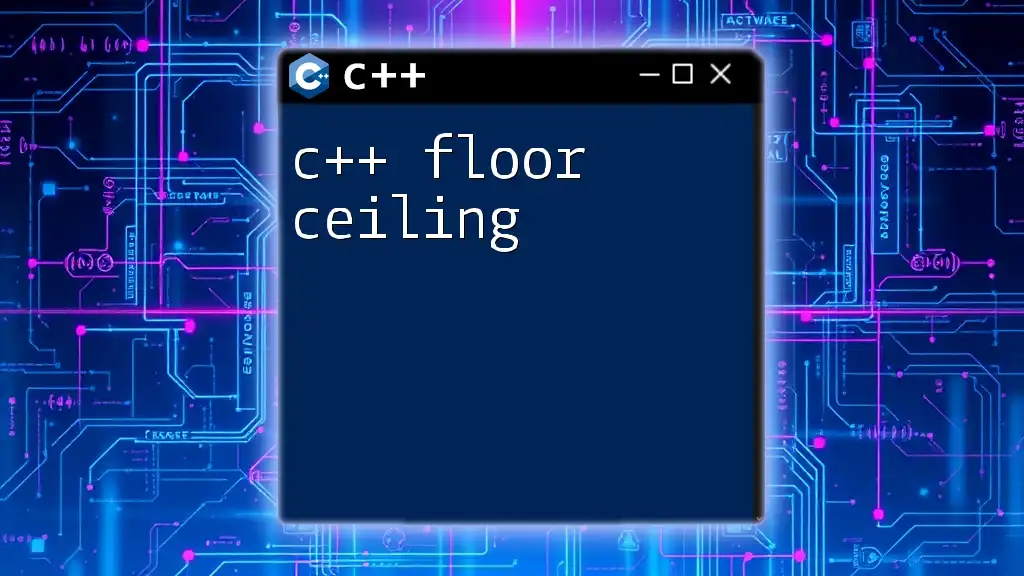
Conclusion
In summary, the C++ for loop with index is a fundamental and powerful programming construct that allows for organized iterations through collections and arrays. By mastering the for loop, programmers can improve not only their code efficiency but also its readability. Practicing with a variety of for loop implementations will help solidify these concepts. Explore additional resources and exercises to continue your journey in C++ programming!
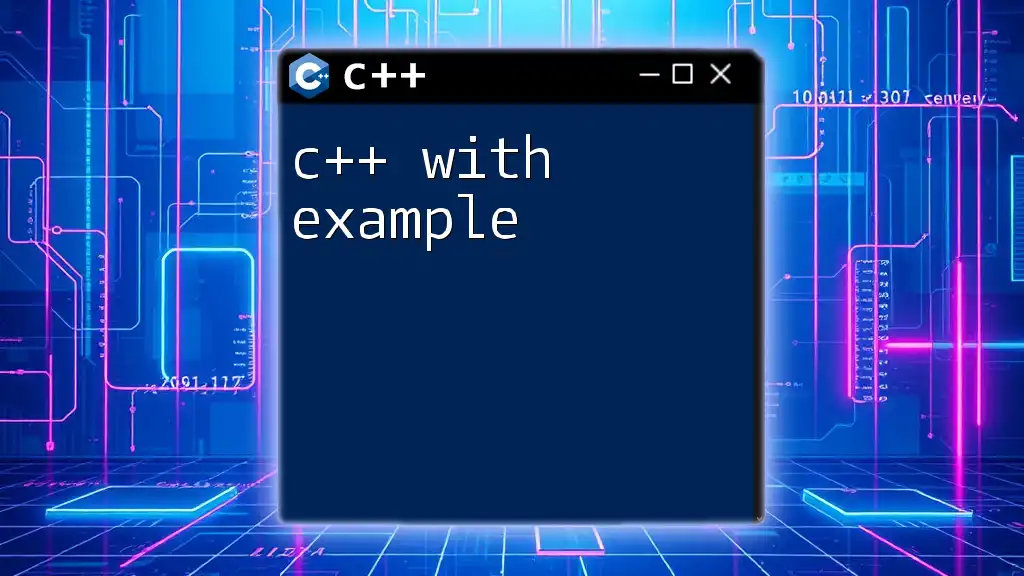
Additional Resources
Consider seeking out tutorials, documentation, and community forums where you can deepen your understanding of C++ loops and indices. Tools like integrated development environments (IDEs) can also help streamline your coding process as you practice.