In this post, we'll explore engaging C++ project ideas that encourage practical learning through hands-on coding experiences.
Here’s a simple "Guess the Number" game to get you started:
#include <iostream>
#include <cstdlib>
#include <ctime>
int main() {
srand(static_cast<unsigned>(time(0))); // Seed for random number
int number = rand() % 100 + 1; // Random number between 1 and 100
int guess;
std::cout << "Guess a number between 1 and 100: ";
do {
std::cin >> guess;
if (guess > number) {
std::cout << "Too high! Try again: ";
} else if (guess < number) {
std::cout << "Too low! Try again: ";
}
} while (guess != number);
std::cout << "Congratulations! You've guessed the correct number: " << number << std::endl;
return 0;
}
Introduction to C++ Projects
Hands-on experience is vital for mastering programming concepts, and engaging in practical projects helps solidify that knowledge. When choosing to build C++ projects, you are not only practicing coding syntax but also exploring the diverse applications of this powerful language. C++ is favored for its versatility in various domains such as system programming, game development, and software development, offering robust performance advantages in terms of speed and memory efficiency.
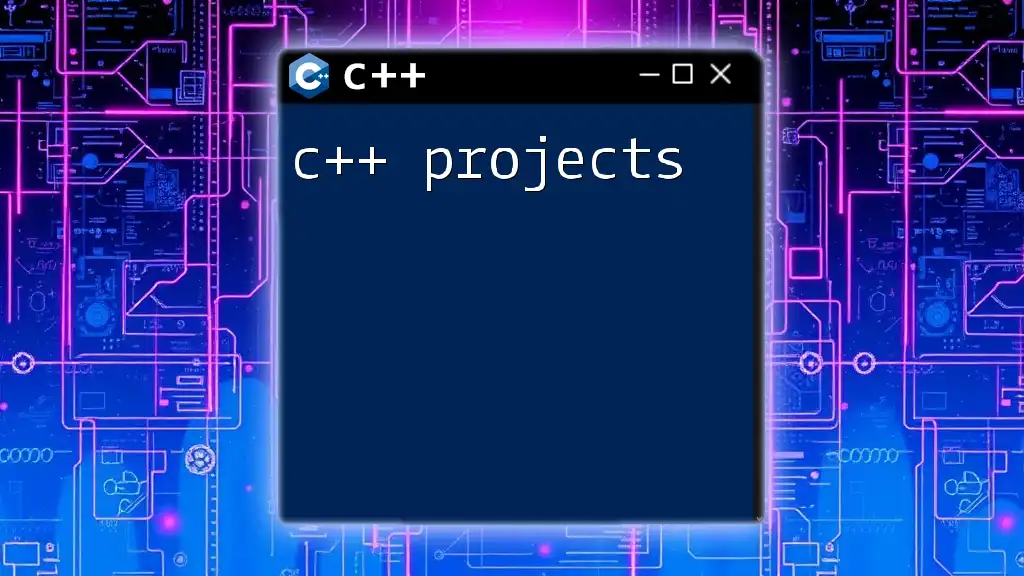
Getting Started with C++ Projects
Setting Up Your Development Environment
Before embarking on your C++ projects journey, setting up an appropriate development environment is crucial. Recommended IDEs such as Visual Studio, Code::Blocks, or CLion provide user-friendly interfaces and tools designed to enhance the coding experience. Begin by download and installation:
- Install the IDE of your choice: Visit the official website and follow the instructions for your operating system.
- Set up a compiler: Most IDEs come with built-in compilers, but ensure you check that your setup is correctly configured for C++.
- Create a sample project: Familiarize yourself with the IDE by creating a simple "Hello World" project to ensure everything is functioning as expected.
Choosing the Right Project for Your Skill Level
C++ project ideas can range from very simple to highly complex. It’s essential to choose projects that match your skill level to maintain motivation and maximize learning.
- Beginner projects provide a great starting point, allowing you to grasp foundational concepts.
- Intermediate projects introduce more complexity and real-world scenarios, integrating multiple programming concepts.
- Advanced projects challenge your skills and encourage you to learn more advanced techniques like multi-threading and networking.
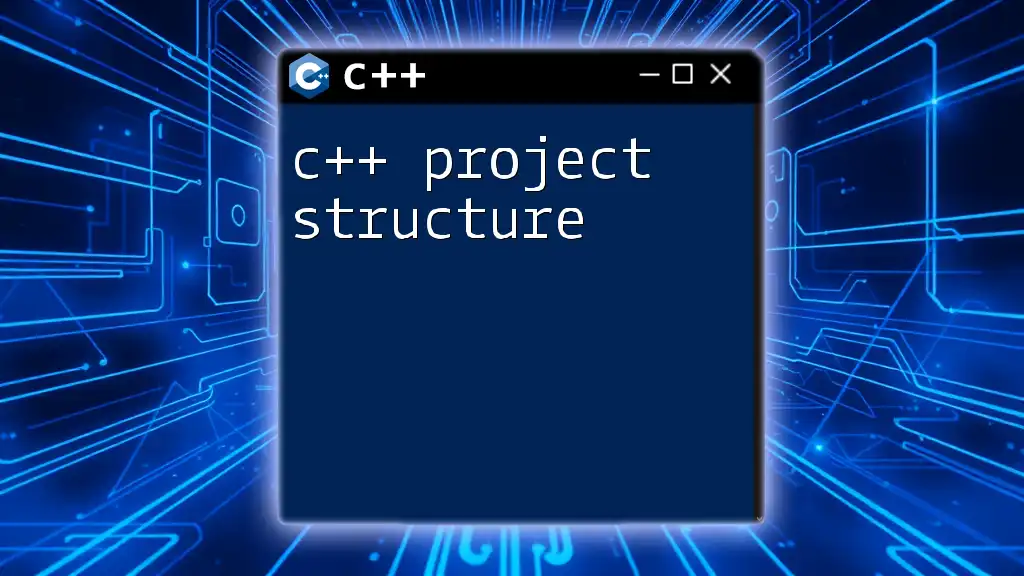
C++ Project Ideas
Beginner Project Ideas in C++
Simple Calculator
Creating a command-line calculator offers an excellent opportunity to practice basic programming concepts such as input/output, data types, and control flow.
Description: This calculator will perform basic arithmetic operations on two numbers provided by the user.
Code Snippet:
#include <iostream>
using namespace std;
int main() {
int a, b;
char op;
cout << "Enter first number: ";
cin >> a;
cout << "Enter operator (+, -, *, /): ";
cin >> op;
cout << "Enter second number: ";
cin >> b;
switch(op) {
case '+': cout << a + b; break;
case '-': cout << a - b; break;
case '*': cout << a * b; break;
case '/': cout << a / b; break;
default: cout << "Invalid operator";
}
return 0;
}
Learning Outcomes: Variables, user input handling, switch statements, and basic arithmetic logic.
Tic-Tac-Toe Game
Developing a simple console-based Tic-Tac-Toe game allows you to work with arrays and loops.
- Description: Implement the game where two players take turns marking spaces in a 3x3 grid.
Potential Enhancements: Incorporate error handling for invalid moves and the ability to play against a rudimentary AI.
Intermediate Project Ideas for C++
Bank Management System
A Bank Management System application will give you insights into data structures, classes, and object-oriented programming.
Description: This application will manage bank account details along with transactions.
- Components to Implement:
- Account creation and deletion features.
- Balance inquiries with detailed transaction history.
- Fund transfer functions between accounts.
Advanced Features: Implement file handling to save account data, allowing for persistence between application sessions.
Library Management System
Building a Library Management System offers an opportunity to work with data organization and user interaction.
Description: This application will manage a collection of books and the records of borrowers.
- Functionality Aspects:
- Add and remove books from the inventory.
- Search books by title or author efficiently.
- Keep track of availability and reservations of books.
Advanced Project Ideas in C++
Chat Application
Developing a chat application using the client-server architecture will familiarize you with networking concepts.
Description: This project entails creating a console-based chat system where multiple clients can communicate with a server.
- Key Concepts:
- Learn about sockets and TCP/IP networking.
- Use multi-threading to handle multiple client connections simultaneously.
Game Development: Snake Game
Creating a Snake game is a fun way to delve into game design using a graphics library like SFML (Simple and Fast Multimedia Library).
Description: The objective is to grow a snake by collecting points while avoiding collisions.
- Features to Implement:
- Score tracking to keep the player engaged.
- Implement difficulty levels that increase gameplay complexity.
- Design game-over scenarios and allow restarts.
Code Snippet: (Please include basic pseudocode or instructions on how to set up the graphics for drawing the snake and handling user inputs).
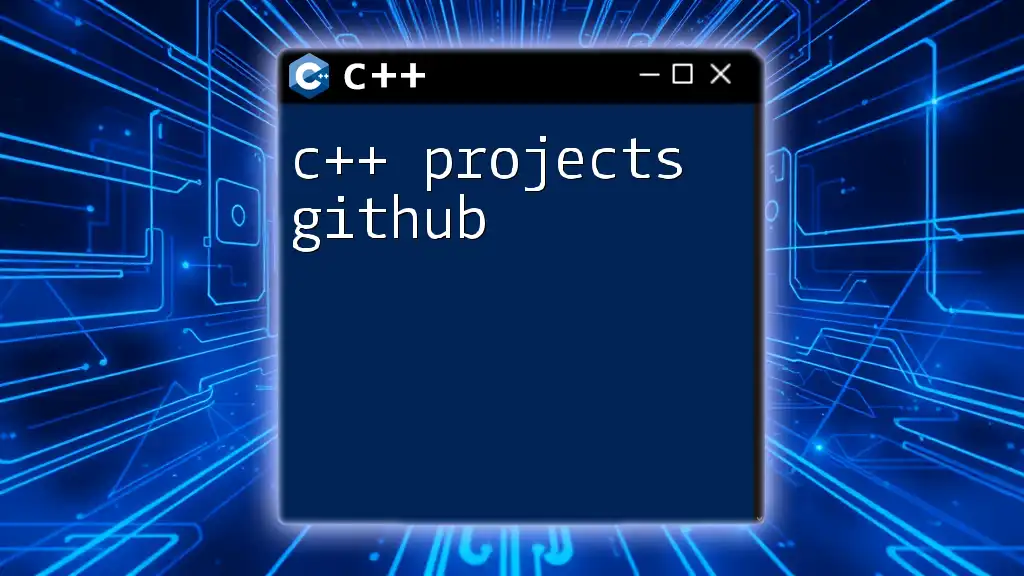
Tips for Successfully Completing C++ Projects
Breaking Down Projects into Manageable Tasks
When approaching any C++ project, it’s beneficial to break it down into smaller, manageable tasks. This encourages modular programming, which not only helps with organization but also makes debugging easier. Prioritize your tasks by creating a roadmap or checklist before you start coding, which will guide you through the development process.
Utilizing Online Resources and Communities
Do not hesitate to lean on online communities and resources as you advance with your projects. Collaborating with others, asking questions, and seeking feedback are essential components of the learning process. Utilize platforms such as GitHub, Stack Overflow, and specialized C++ forums where you can share your ideas and receive constructive criticism.
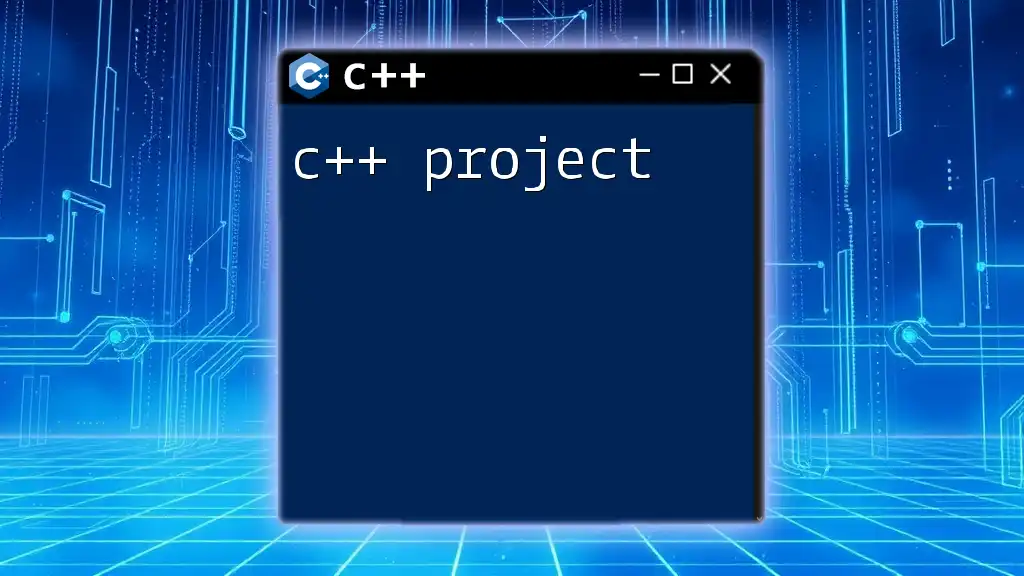
Conclusion
Engaging in C++ projects not only enhances your programming skills but also builds confidence in your ability to tackle real-world challenges. Remember that the goal is not just to complete these projects but to learn from the process, iterating on your work to enhance and expand your skills further.
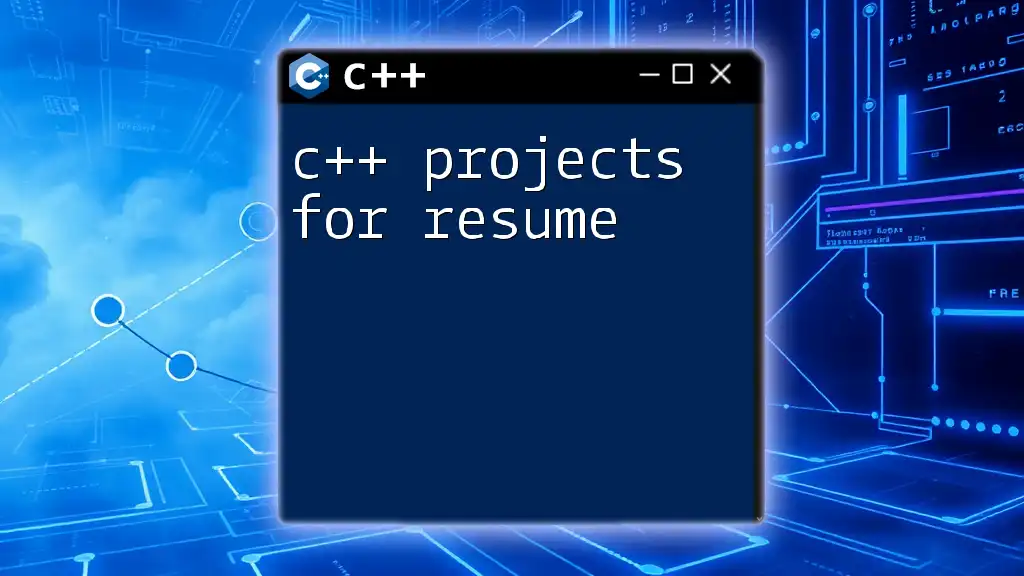
Call to Action
Join our community of learners! Subscribe to receive more tips and ideas on C++ programming. We invite you to share your personal C++ project ideas and experiences with us!