C++ can be used to interact with PostgreSQL databases by leveraging libraries like libpq, allowing you to execute SQL queries efficiently.
Here's a simple example of how to connect to a PostgreSQL database and execute a query using C++:
#include <iostream>
#include <pqxx/pqxx>
int main() {
try {
pqxx::connection C("dbname=test user=postgres password=secret");
if (C.is_open()) {
std::cout << "Opened database successfully: " << C.dbname() << std::endl;
} else {
std::cout << "Can't open database" << std::endl;
return 1;
}
pqxx::work W(C);
W.exec("SELECT * FROM my_table;");
W.commit();
C.disconnect();
} catch (const std::exception &e) {
std::cerr << e.what() << std::endl;
return 1;
}
return 0;
}
What is PostgreSQL?
PostgreSQL is an advanced, open-source relational database management system (RDBMS) that has gained immense popularity among developers for its powerful features and robust performance. Its support for standards compliance, extensibility, and reliability makes it an ideal choice for applications that require a strong backend.
Features of PostgreSQL
PostgreSQL boasts a host of features that enhance its usability and efficiency:
- Open-source and Free to Use: Being open-source, PostgreSQL allows developers to utilize it without licensing fees, making it a cost-effective option.
- ACID Compliance: PostgreSQL ensures data integrity with its adherence to ACID properties (Atomicity, Consistency, Isolation, Durability).
- Support for Advanced Data Types: PostgreSQL supports various data types like JSON, XML, and arrays, facilitating complex data structures.
- Extensibility: Developers can create their own data types, operators, and index types, enabling customized functionalities.
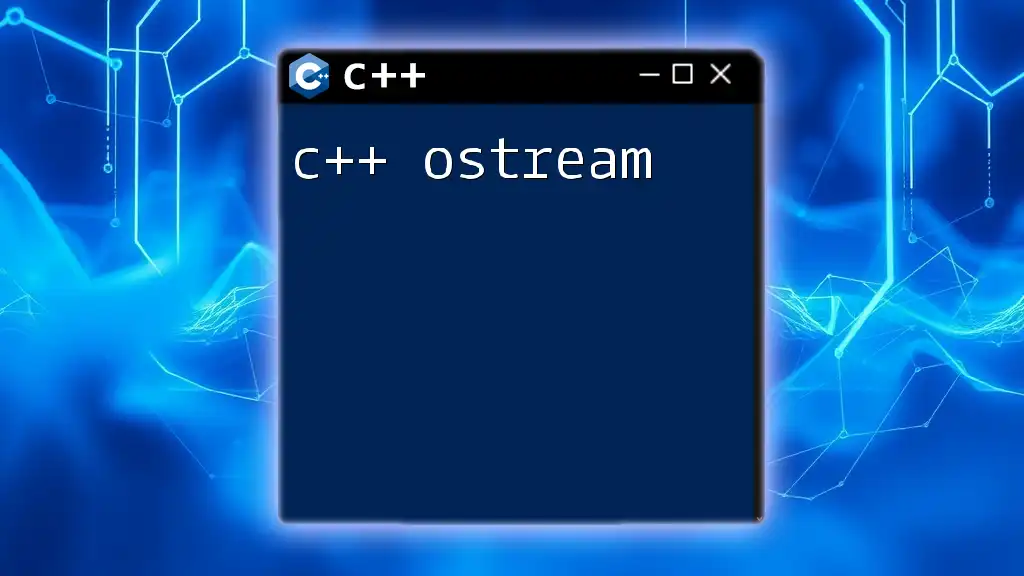
Setting Up PostgreSQL for C++ Development
To begin using PostgreSQL with C++, follow these steps for a successful setup.
Installation of PostgreSQL
Installing PostgreSQL can vary slightly based on your operating system. Here are the steps for common platforms:
- Windows: Download the installer from the official PostgreSQL website and follow the setup wizard.
- Mac: Use Homebrew with the following command:
brew install postgresql
- Linux: On Debian/Ubuntu systems, the following command will install PostgreSQL:
sudo apt-get install postgresql postgresql-contrib
After installation, ensure to configure PostgreSQL’s `pg_hba.conf` file to allow necessary connections.
Installing Development Libraries for C++
To compile and link your C++ projects with PostgreSQL, you'll need the development libraries.
For most Linux distributions, you can install the `libpq` library with this command:
sudo apt-get install libpq-dev # For Debian/Ubuntu
This library provides the necessary APIs for establishing connections and executing SQL queries in your applications.
Connecting C++ to PostgreSQL
Before you can interact with your PostgreSQL database, you must configure the connection parameters such as the database name, user, password, host, and port. The connection string typically looks like this:
const char *conninfo = "dbname=testdb user=postgres password=secret hostaddr=127.0.0.1 port=5432";
Utilizing this string, you can establish a connection within your C++ application using the `libpq` or `pqxx` library.
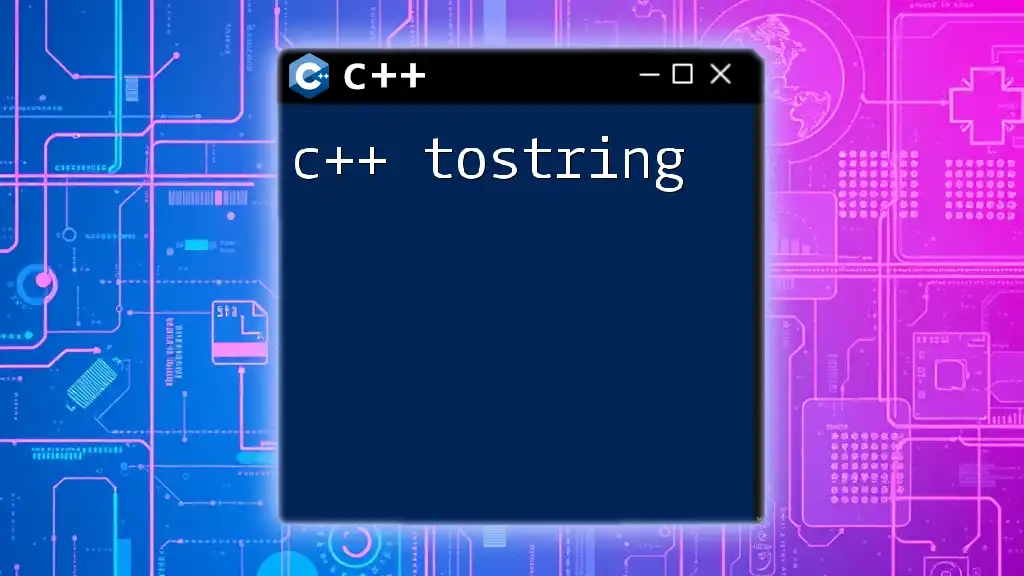
Writing Your First C++ PostgreSQL Application
To start writing your C++ application that interacts with PostgreSQL, you'll need to include the necessary headers and libraries.
Including Necessary Headers
You'll need to include the following headers at the beginning of your program:
#include <iostream>
#include <pqxx/pqxx>
pqxx is a C++ client library specifically designed to work with PostgreSQL, providing a user-friendly interface for database operations.
Establishing Database Connection
Here's a simple code snippet that demonstrates how to connect to a PostgreSQL database:
try {
pqxx::connection C("dbname=testdb user=postgres password=secret");
if (C.is_open()) {
std::cout << "Opened database successfully: " << C.dbname() << std::endl;
} else {
std::cout << "Can't open database" << std::endl;
return 1;
}
} catch (const pqxx::sql_error &e) {
std::cerr << "SQL error: " << e.what() << std::endl;
}
In this example, if the connection is successful, you'll receive a confirmation message; otherwise, it catches any SQL error that occurs during the connection process.
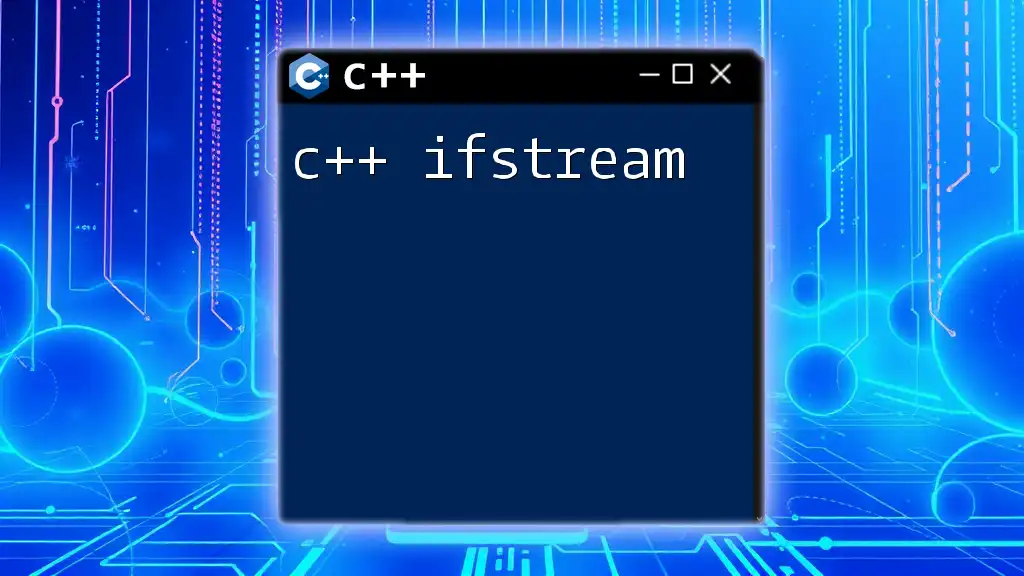
CRUD Operations in C++ with PostgreSQL
Once your connection to the database is established, you can perform CRUD (Create, Read, Update, Delete) operations seamlessly.
Create Operation
To insert data into a PostgreSQL table, you can use the following code snippet:
pqxx::work W(C);
W.exec("INSERT INTO COMPANY (ID, NAME, AGE, ADDRESS) VALUES (1, 'Paul', 32, 'California')");
W.commit();
This snippet creates a new entry in the `COMPANY` table. Always remember to call `commit()` to save the changes to the database.
Read Operation
To read data from the database, employ the following code:
pqxx::nontransaction N(C);
pqxx::result R(N.exec("SELECT * FROM COMPANY"));
for (auto row : R) {
std::cout << "ID = " << row[0].as<int>() << std::endl;
}
This example fetches all records from the `COMPANY` table and iterates through them, printing the ID of each entry.
Update Operation
Updating existing records is just as straightforward:
pqxx::work W(C);
W.exec("UPDATE COMPANY SET AGE = 33 WHERE ID = 1");
W.commit();
This command updates the `AGE` for the record where `ID` is `1`. Always ensure you `commit()` after making changes.
Delete Operation
To remove a record from the database, use this code:
pqxx::work W(C);
W.exec("DELETE FROM COMPANY WHERE ID = 1");
W.commit();
It’s important to carefully manage deletions to avoid losing important data.
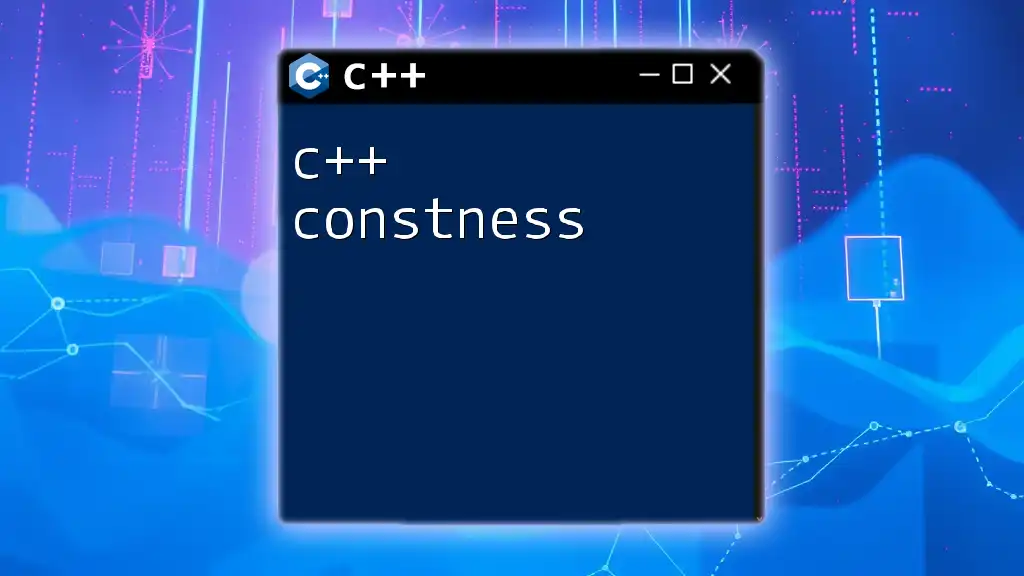
Error Handling in C++ PostgreSQL
When working with databases, it’s crucial to implement comprehensive error handling. The `pqxx` library provides exceptions that can help identify issues.
Common Errors and Exceptions
For instance, you could use a `try-catch` block to handle exceptions like so:
catch (const pqxx::sql_error &e) {
std::cerr << "SQL error: " << e.what() << std::endl;
}
This approach allows you to capture SQL-related errors, giving you insights into what might have gone wrong during execution.
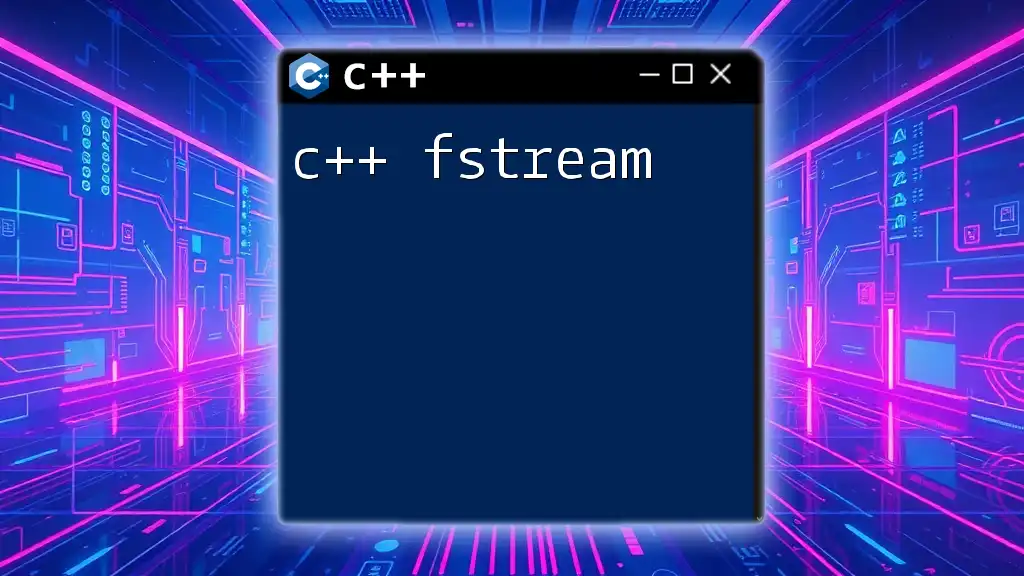
Advanced Operations
As you become more familiar with C++ and PostgreSQL, you may want to explore advanced database operations.
Transactions in PostgreSQL
Transactions are crucial for maintaining data integrity. To use transactions in C++, wrap your database commands in a `work` block:
pqxx::work W(C);
// Execute SQL statements here
W.commit();
This approach ensures that all operations within the block are executed as a single unit, either committing all changes or rolling them back if there’s an error.
Prepared Statements
For improved performance and security, especially against SQL injection attacks, use prepared statements. Here's an example:
pqxx::work W(C);
pqxx::prepare::declaration P(W, "INSERT INTO COMPANY (ID, NAME) VALUES ($1, $2)");
P(1, "John Doe");
W.exec(P);
W.commit();
Prepared statements separate SQL logic from data inputs, enhancing the robustness of your applications.
Using C++ PostgreSQL with Multi-threading
If your application is multi-threaded, you'll need to be careful with connection handling. Each thread should have its own connection object to avoid potential data corruption and ensure thread safety.
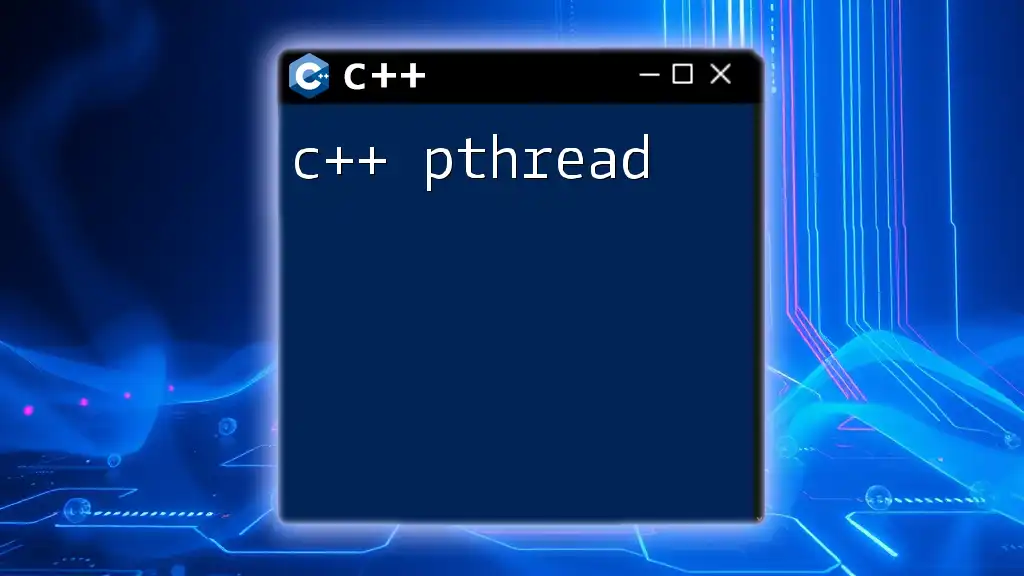
Best Practices for Using C++ with PostgreSQL
Maximizing performance and security in your C++ applications interacting with PostgreSQL requires following best practices.
Performance Optimization Tips
- Database Indexing: Index frequently queried columns to speed up read operations.
- Query Optimization: Use `EXPLAIN` to analyze and optimize your SQL commands.
- Connection Pooling: Reuse existing connections instead of establishing new ones for every request.
Securing PostgreSQL Databases
To secure your PostgreSQL database, consider implementing these measures:
- Use SSL for Connections: Encrypt data transmitted over the network.
- Limit User Privileges: Give users only the access they need for their tasks.
- Regularly Update PostgreSQL: Keep your database up-to-date with the latest security patches.
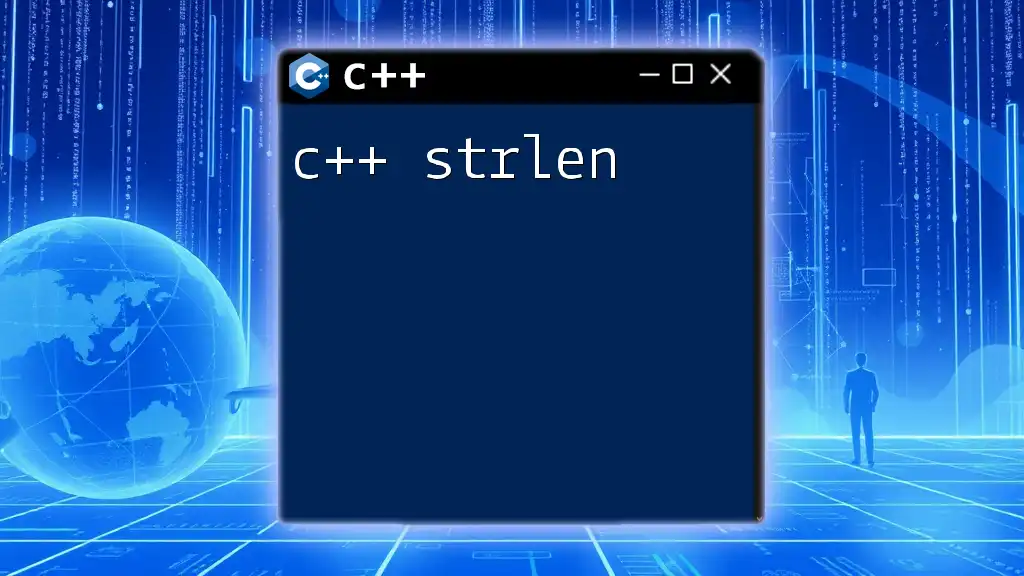
Conclusion
In conclusion, using C++ with PostgreSQL opens up a world of possibilities for building efficient and reliable applications. As you've learned, establishing connections, performing CRUD operations, handling errors, and adhering to best practices are foundational elements to becoming proficient in this integration.
Encouragement for Further Learning
To further your understanding of C++ and PostgreSQL, consider exploring additional resources such as the official documentation, C++ library guides, and online courses targeting database management. The more you practice, the more confident you'll become in harnessing the power of PostgreSQL in your C++ applications.
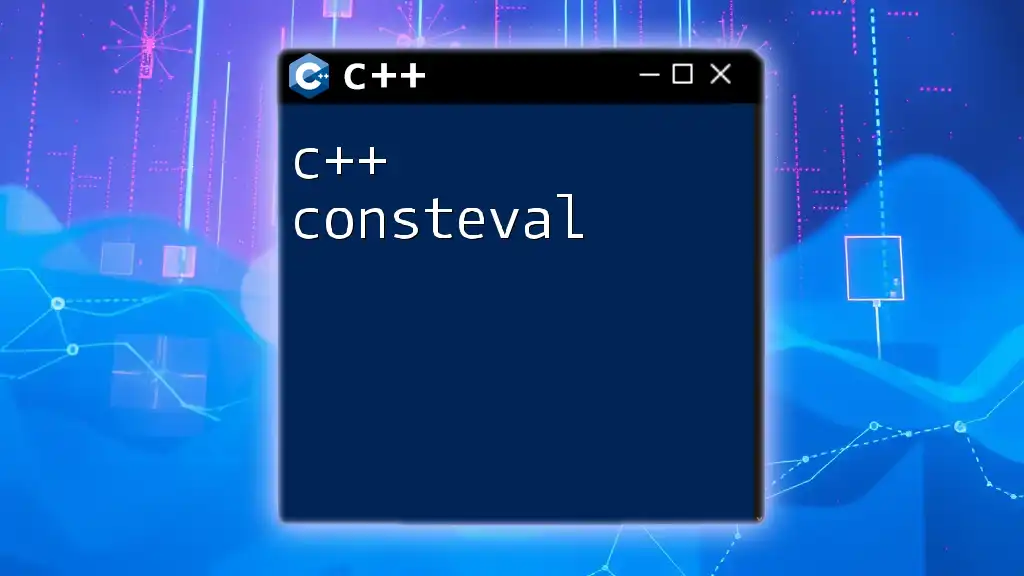
FAQs
Common Questions About C++ and PostgreSQL
-
What is the difference between `pq` and `pqxx`?
`pq` is the C API for PostgreSQL, while `pqxx` is a C++ wrapper that provides a more user-friendly interface. -
Can I use PostgreSQL with other programming languages?
Yes, PostgreSQL supports a wide range of programming languages including Python, Java, and Ruby, among others. -
Do I need to know SQL to work with C++ and PostgreSQL?
Yes, a solid understanding of SQL is essential for effectively querying and manipulating data in PostgreSQL.