C++ pthread is a POSIX (Portable Operating System Interface) library used for creating and managing threads in C++ applications, allowing for concurrent execution of code.
Here's a simple code snippet demonstrating the creation of a thread using pthreads in C++:
#include <iostream>
#include <pthread.h>
void* threadFunction(void* arg) {
std::cout << "Hello from the thread!" << std::endl;
return nullptr;
}
int main() {
pthread_t thread;
pthread_create(&thread, nullptr, threadFunction, nullptr);
pthread_join(thread, nullptr);
return 0;
}
What are PThreads?
POSIX Threads, commonly referred to as PThreads, is a standardized C library for implementing multi-threading in software applications. This library provides a set of APIs for developers to create and manage threads, enabling concurrent execution of tasks. Utilizing PThreads in C++ allows for efficient utilization of CPU resources, enhancing application performance and responsiveness.
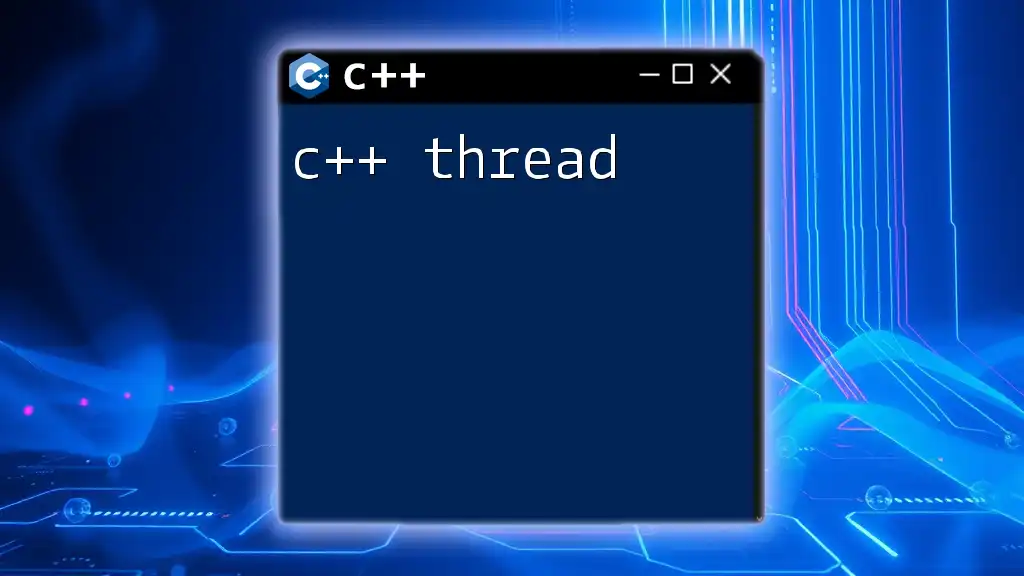
Why Use PThreads in C++?
Choosing PThreads over other threading libraries, such as C++11 threads or platform-specific APIs, offers several advantages. Firstly, PThreads is a widely supported and standardized library, making it portable across different Unix-like operating systems. Secondly, it provides fine-grained control over threads, including thread attributes, scheduling, and synchronization methods. This level of control is crucial for developing complex applications, such as server applications, simulations, and real-time processing.
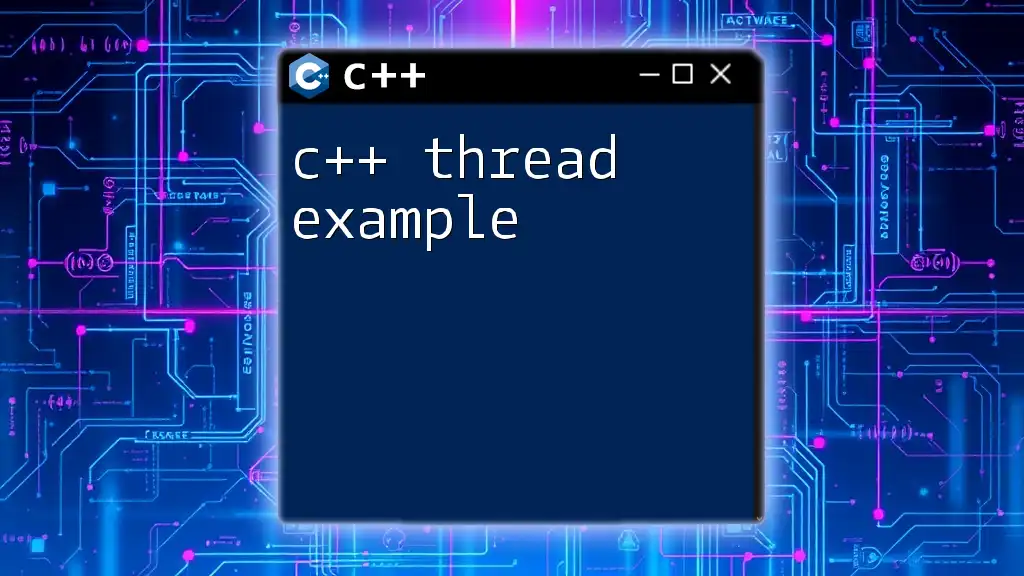
Setting Up Your Development Environment
Required Libraries and Tools
To begin working with PThreads in C++, you need to ensure your development environment is properly set up. Install the GCC and G++ compilers, which come with built-in support for PThreads. On Debian-based systems, you can run:
sudo apt-get install build-essential
Compiling with PThreads
When you compile a C++ program that uses PThreads, you must link against the PThreads library explicitly. You can do this by adding the `-pthread` flag to your compilation command. Here’s an example:
g++ -o my_program my_program.cpp -pthread
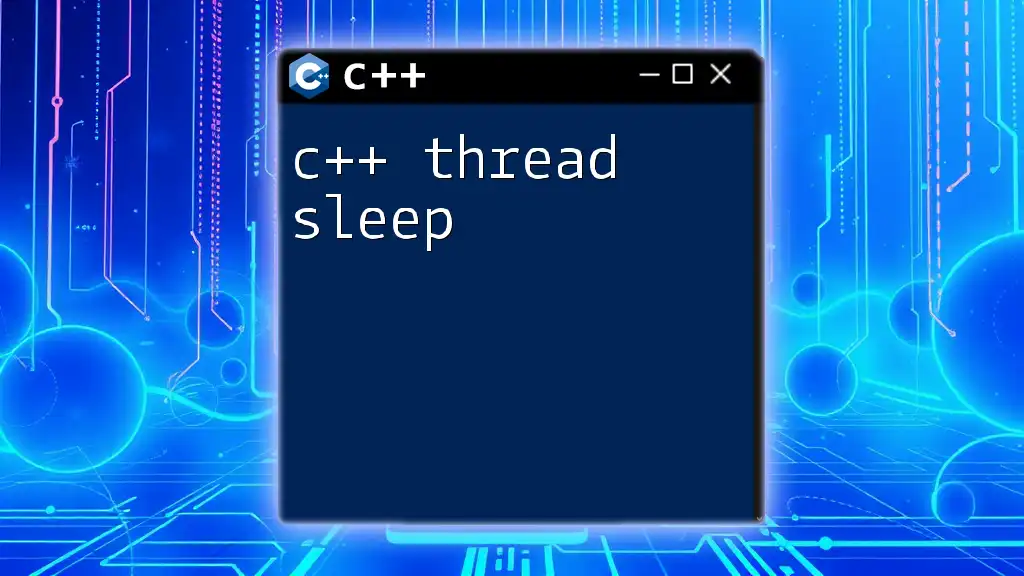
Key Concepts in PThreads
Thread Creation
Creating a thread in PThreads is straightforward with the `pthread_create` function. This function takes four parameters: a pointer to the thread identifier, thread attributes (which can be set to NULL for default attributes), a function pointer to the thread's start routine, and a pointer to any argument you want to pass to that function.
Here is a basic example of creating a thread:
#include <iostream>
#include <pthread.h>
void* threadFunction(void* arg) {
std::cout << "Hello from thread!" << std::endl;
return nullptr;
}
int main() {
pthread_t threadId;
pthread_create(&threadId, nullptr, threadFunction, nullptr);
pthread_join(threadId, nullptr); // Wait for the thread to complete
return 0;
}
Thread Attributes
PThreads allows you to customize thread behavior using thread attributes. The `pthread_attr_t` type can be used to specify various attributes such as stack size, scheduling policy, and more. To modify thread attributes, follow these steps:
- Initialize the attributes object using `pthread_attr_init`.
- Set any desired attributes with corresponding setter functions (like `pthread_attr_setstacksize`).
- Pass the attributes object to `pthread_create`.
Example:
pthread_attr_t attr;
pthread_attr_init(&attr);
pthread_attr_setstacksize(&attr, 16384); // Set stack size to 16KB
pthread_create(&threadId, &attr, threadFunction, nullptr);
pthread_attr_destroy(&attr); // Clean up the attributes object
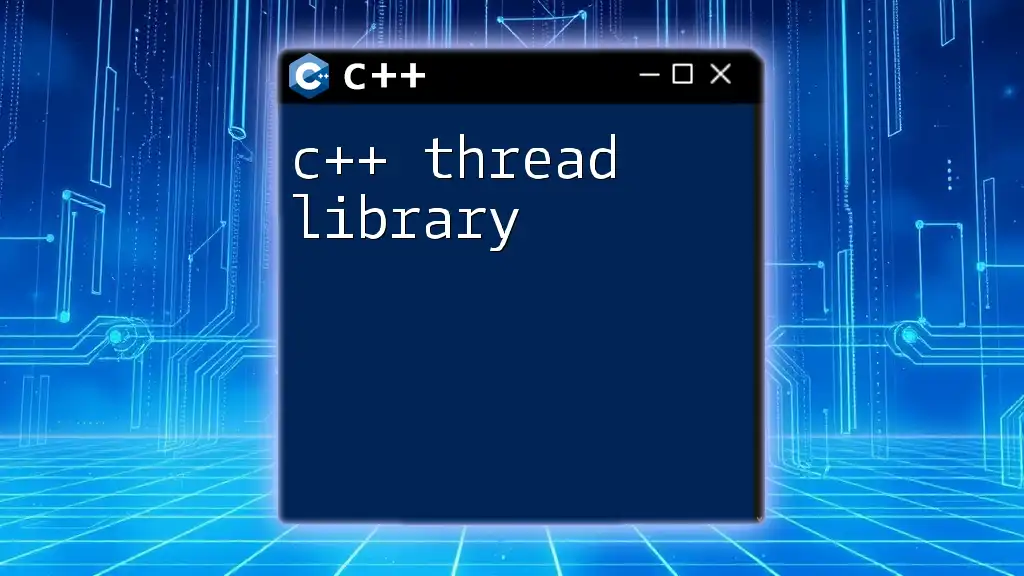
Managing Threads
Joining and Detaching Threads
Once a thread has been created, you must determine its termination strategy. Joining a thread using `pthread_join` ensures that the calling thread waits for the specified thread to finish executing. In contrast, detaching a thread with `pthread_detach` allows it to run independently, freeing resources automatically once it finishes.
Example of joining a thread:
pthread_join(threadId, nullptr);
Example of detaching a thread:
pthread_detach(threadId);
Thread Cancellation
Thread cancellation allows you to terminate a running thread. You can request cancellation by using the `pthread_cancel` function. It is essential to manage thread state to ensure that cancellation does not leave shared resources in an inconsistent state.
Here’s how you can implement cancellation:
void* cancellableThreadFunction(void* arg) {
while (true) {
// Perform work
pthread_testcancel(); // Check for cancellation
}
}
pthread_t threadId;
pthread_create(&threadId, nullptr, cancellableThreadFunction, nullptr);
pthread_cancel(threadId); // Request cancellation
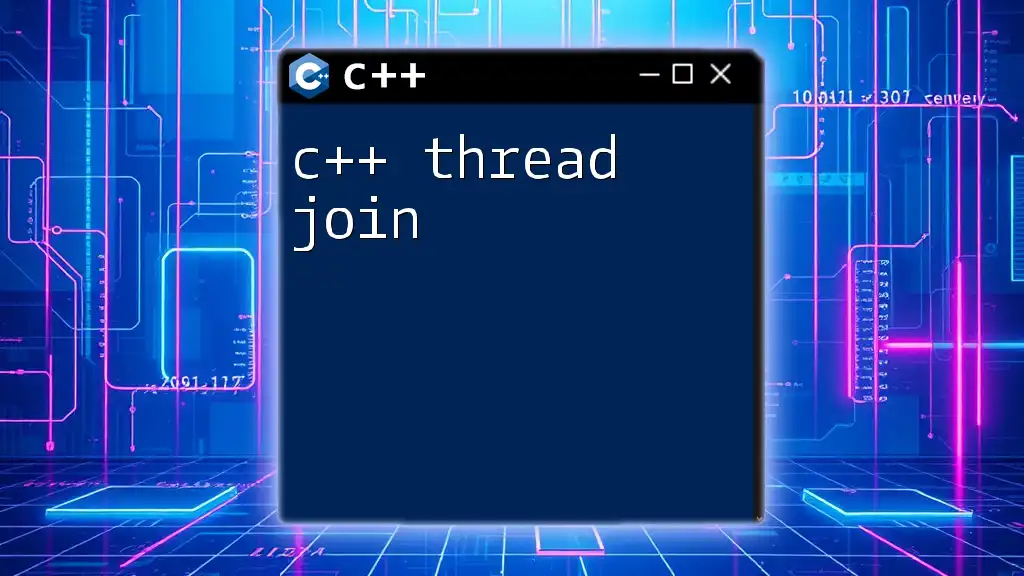
Synchronization Mechanisms
Mutexes
To prevent data races when multiple threads access shared resources, you can use mutexes. A mutex (mutual exclusion) ensures that only one thread can access the resource at a time. To utilize a mutex, you need to:
- Initialize the mutex using `pthread_mutex_init`.
- Lock the mutex with `pthread_mutex_lock` before accessing the critical section.
- Unlock the mutex with `pthread_mutex_unlock` after the critical section.
Example:
pthread_mutex_t mutex;
pthread_mutex_init(&mutex, nullptr);
pthread_mutex_lock(&mutex);
// Critical section
pthread_mutex_unlock(&mutex);
pthread_mutex_destroy(&mutex); // Clean up the mutex
Condition Variables
Condition variables allow threads to wait for certain conditions to be met. They are used in conjunction with mutexes. A thread can wait on a condition variable using `pthread_cond_wait`, and another thread can signal the condition using `pthread_cond_signal`.
Example of a producer-consumer scenario:
pthread_mutex_t mutex;
pthread_cond_t cond;
bool ready = false;
void* consumer(void* arg) {
pthread_mutex_lock(&mutex);
while (!ready) {
pthread_cond_wait(&cond, &mutex);
}
// Consume the product
pthread_mutex_unlock(&mutex);
}
void* producer(void* arg) {
pthread_mutex_lock(&mutex);
// Produce a product
ready = true;
pthread_cond_signal(&cond); // Notify consumer
pthread_mutex_unlock(&mutex);
}
Read-Write Locks
Read-write locks allow multiple threads to read data simultaneously while ensuring exclusive write access. This is particularly useful in scenarios where reading is more frequent than writing.
You can implement read-write locks using `pthread_rwlock_t`, which provides functions for reading (`pthread_rwlock_rdlock`) and writing (`pthread_rwlock_wrlock`) access.
Example:
pthread_rwlock_t rwlock;
pthread_rwlock_init(&rwlock, nullptr);
// Reading
pthread_rwlock_rdlock(&rwlock);
// Perform read operation
pthread_rwlock_unlock(&rwlock);
// Writing
pthread_rwlock_wrlock(&rwlock);
// Perform write operation
pthread_rwlock_unlock(&rwlock);
pthread_rwlock_destroy(&rwlock); // Clean up the lock
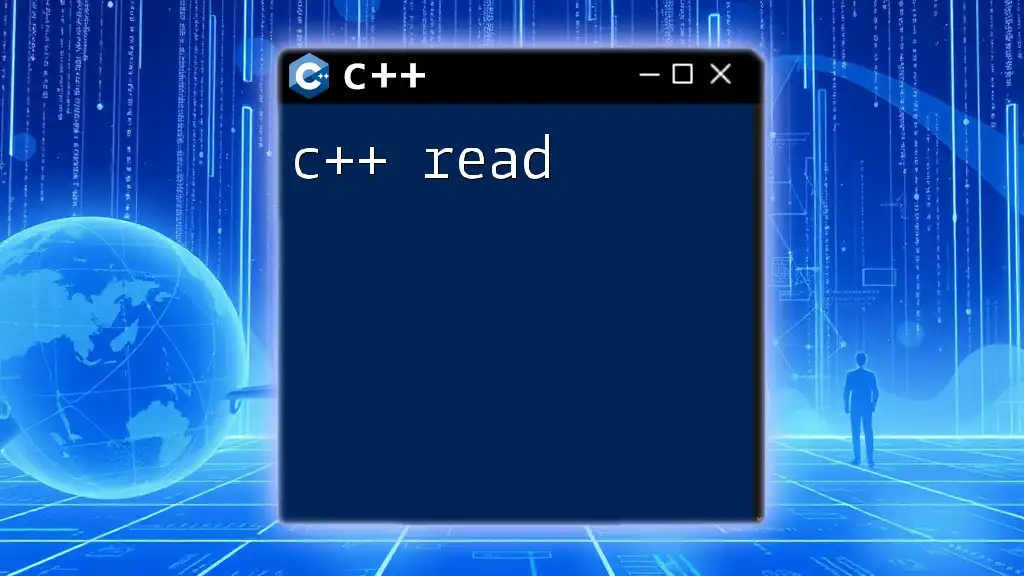
Error Handling
Common PThread Errors
Error handling in PThreads is critical to ensure that your application behaves correctly under exceptional conditions. After any PThread function call, it is essential to check its return value; most of these functions return zero on success and an error code on failure.
Using `errno` for Enhanced Error Reporting
Using `errno` alongside PThread calls can improve your error handling, providing detailed insight into the nature of the issue. Example:
if (pthread_create(&threadId, nullptr, threadFunction, nullptr) != 0) {
std::cerr << "Error creating thread: " << strerror(errno) << std::endl;
}
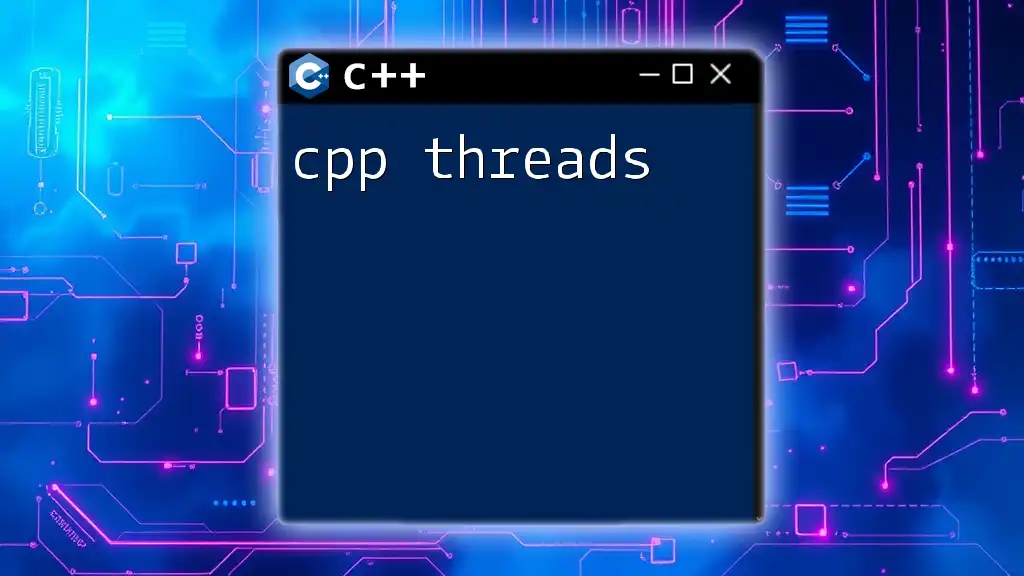
Best Practices for Using PThreads in C++
Design Patterns for Multi-threading
Implementing appropriate design patterns for your multi-threaded applications enhances maintainability and performance. Common patterns include:
- Thread Pool: Manage a fixed number of threads for executing tasks, reducing the overhead of thread creation.
- Producer-Consumer: Employing condition variables and mutexes to synchronize tasks between producing and consuming threads.
Testing and Debugging Multi-threaded Applications
Testing multi-threaded applications can be challenging due to timing issues and race conditions. Utilize tools like Valgrind with the Helgrind module or ThreadSanitizer to detect data races. Ensure you thoroughly test your application under various loads and conditions to uncover hidden issues.
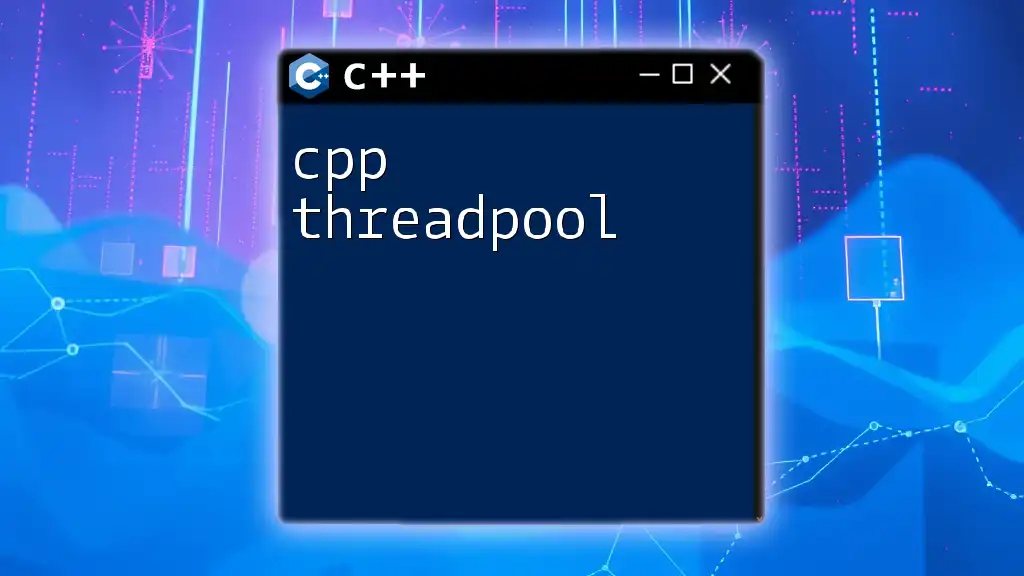
Conclusion
PThreads in C++ provides a powerful means of implementing multi-threading in your applications. By mastering this library, you can develop efficient, responsive software that harnesses the full power of modern multi-core processors. Continue experimenting with the examples provided, and build upon this knowledge to tackle complex threading scenarios with confidence.
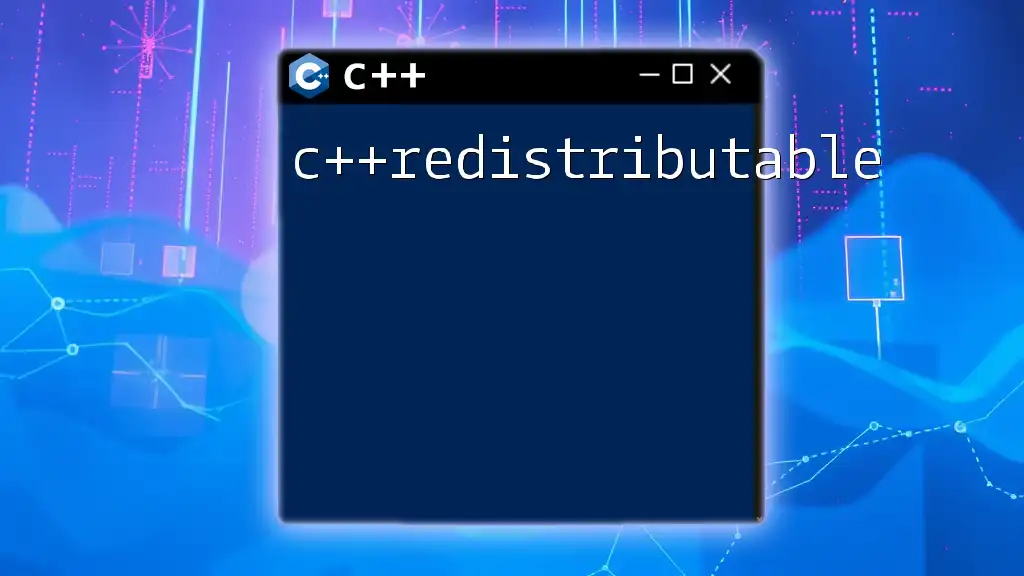
Further Resources
To further enhance your understanding of C++ PThreads, consider consulting books such as "Programming with POSIX Threads" by David R. Butenhof and online tutorials that cover both basic and advanced topics. Engaging in community forums like Stack Overflow can also provide valuable insights and support as you delve deeper into multi-threading in C++.