In C++, you can pause the execution of a thread for a specified duration using the `std::this_thread::sleep_for` function from the `<thread>` header.
Here’s a simple example:
#include <iostream>
#include <thread>
#include <chrono>
int main() {
std::cout << "Sleeping for 2 seconds..." << std::endl;
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Woke up!" << std::endl;
return 0;
}
Understanding the Need for Sleep in Multithreading
The Role of Sleep in Thread Management
In a multithreaded environment, efficient thread management is crucial. One of the fundamental aspects of managing threads is knowing when to pause their execution. Thread sleep helps mitigate unnecessary CPU cycles by preventing threads from continuously executing when they don't need to. By allowing a thread to sleep, you avoid busy-waiting, which can lead to resource exhaustion and diminish overall system performance.
Real-World Scenarios to Use Thread Sleep
Consider a gaming application where multiple threads manage different aspects, such as rendering graphics and processing user inputs. If the input processing thread were to run continuously without any pause, it would consume CPU resources unnecessary while waiting for user actions, thus degrading performance. By using c++ thread sleep, you ensure that this thread efficiently waits for events, reducing the load on the CPU and improving responsiveness.

C++ Thread Sleep Mechanisms
Using std::this_thread::sleep_for
In C++, you can pause the execution of a thread using the `std::this_thread::sleep_for` function. This function allows you to specify how long the thread should sleep by providing a duration.
Syntax:
#include <chrono>
#include <thread>
std::this_thread::sleep_for(std::chrono::milliseconds(1000)); // sleeps for 1 second
Example for Demonstration:
#include <iostream>
#include <thread>
#include <chrono>
void sleepDemo() {
std::cout << "Thread will sleep for 2 seconds.\n";
std::this_thread::sleep_for(std::chrono::seconds(2));
std::cout << "Thread woke up!\n";
}
int main() {
std::thread t(sleepDemo);
t.join();
return 0;
}
In this example, a thread is created to run the `sleepDemo` function. The thread sleeps for 2 seconds before outputting a message. This demonstrates how to manage execution timing effectively.
Using std::this_thread::sleep_until
Another option for putting a thread to sleep is the `std::this_thread::sleep_until` function, which sleeps the current thread until a specified time point.
Syntax:
#include <chrono>
#include <thread>
std::this_thread::sleep_until(std::chrono::steady_clock::now() + std::chrono::seconds(1));
Example for Demonstration:
#include <iostream>
#include <chrono>
#include <thread>
void sleepUntilDemo() {
auto wake_time = std::chrono::steady_clock::now() + std::chrono::seconds(3);
std::cout << "Thread will sleep until a specific time in the future.\n";
std::this_thread::sleep_until(wake_time);
std::cout << "Thread woke up after sleeping until the specified time!\n";
}
int main() {
std::thread t(sleepUntilDemo);
t.join();
return 0;
}
In this example, the thread sleeps until a defined time point, demonstrating flexibility in managing thread behavior.
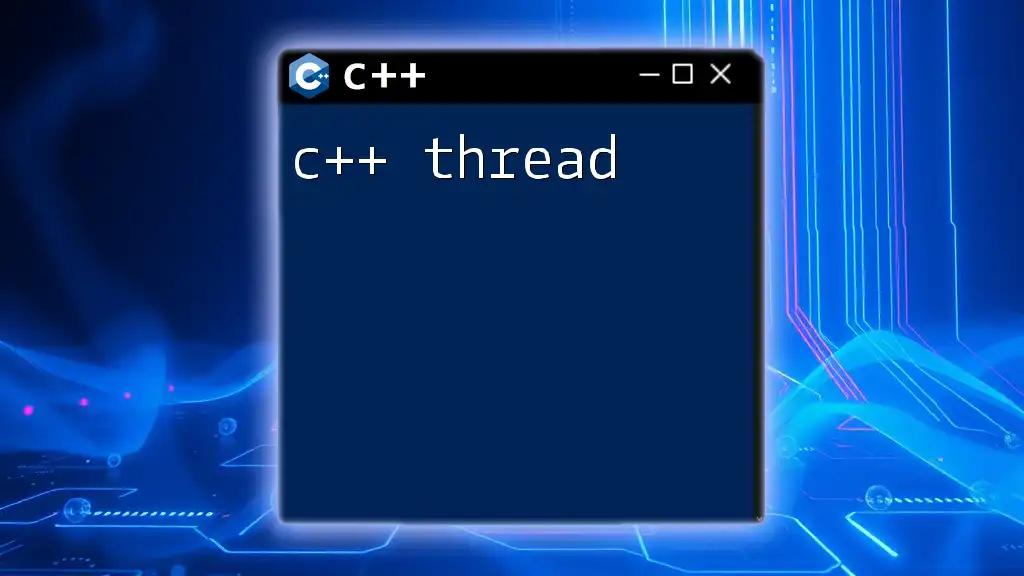
Comparing Different Sleep Functions
Sleep_for vs. Sleep_until
While both functions serve a similar purpose, they are used in different scenarios. `sleep_for` is intuitive for situations where you want a thread to sleep for a predefined length of time, making it straightforward. On the other hand, `sleep_until` is beneficial when you need the thread to wake up at a specific time, allowing for more precise control.
Performance Considerations
Choosing the right sleep function can significantly impact your program's efficiency. Using `sleep_for` can be appropriate for tasks that need periodic work, whereas `sleep_until` is more suitable for operations that must align with real-time events, like timers or scheduled tasks.

The Importance of Precision in Sleep Functions
Factors Influencing Timer Precision
When dealing with sleep functions, several factors can affect their precision. Operating system contention and thread scheduling policies can cause delays that may not be accounted for in your code. For critical applications, understanding these influences is crucial.
Achieving High-Precision Sleep
For high-resolution sleep needs, leveraging libraries that support finer timer resolutions can be valuable. The `std::chrono` library in C++ offers high-resolution facilities, aiding you in achieving the desired timing precision.

Common Pitfalls with Thread Sleep
Misusing Thread Sleep
A common mistake in multithreading is overusing thread sleep. While it can prevent busy-waiting, excessive use can lead to inefficiencies and sluggish user experiences. It's essential to use sleep wisely—keeping the number of sleep calls to a minimum and ensuring they are condition-based rather than static.
Debugging Common Thread Sleep Issues
When using `c++ thread sleep`, issues may arise such as waking up too early or too late. These scenarios can often be traced back to misunderstandings about how your system handles thread scheduling or timing inaccuracies. To troubleshoot, it’s helpful to log timestamps around sleep calls to understand the actual behavior.

Best Practices for Using C++ Thread Sleep
Guidelines for Effective Thread Management
To optimize performance, balance responsiveness and resource use when implementing sleep. Use conditional sleep statements that halt thread execution only when necessary — for example, waiting for user input rather than continuously iterating in a loop.
Alternatives to Sleep for Thread Synchronization
While sleep functions are valuable, consider alternative synchronization techniques where applicable. Using mutexes or condition variables can provide better control in scenarios requiring thread synchronization over mere waiting periods.
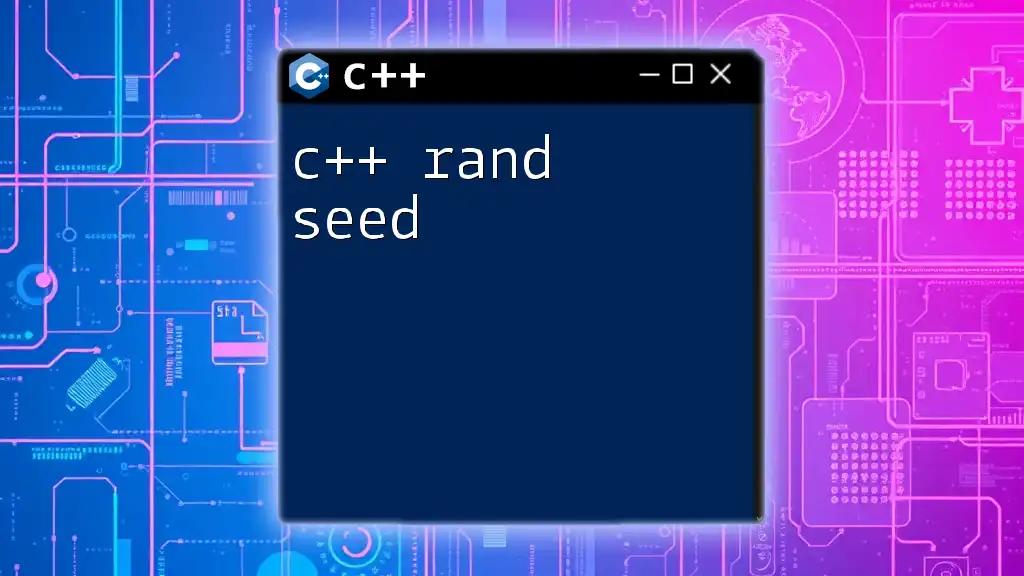
Conclusion
In this article, we explored the fundamentals of c++ thread sleep, focusing on its mechanisms, use cases, and best practices. By incorporating the right sleep strategies into your multithreaded applications, you can achieve more efficient performance and better resource management. Experiment with the concepts discussed and continue to explore the rich world of C++ multithreading!
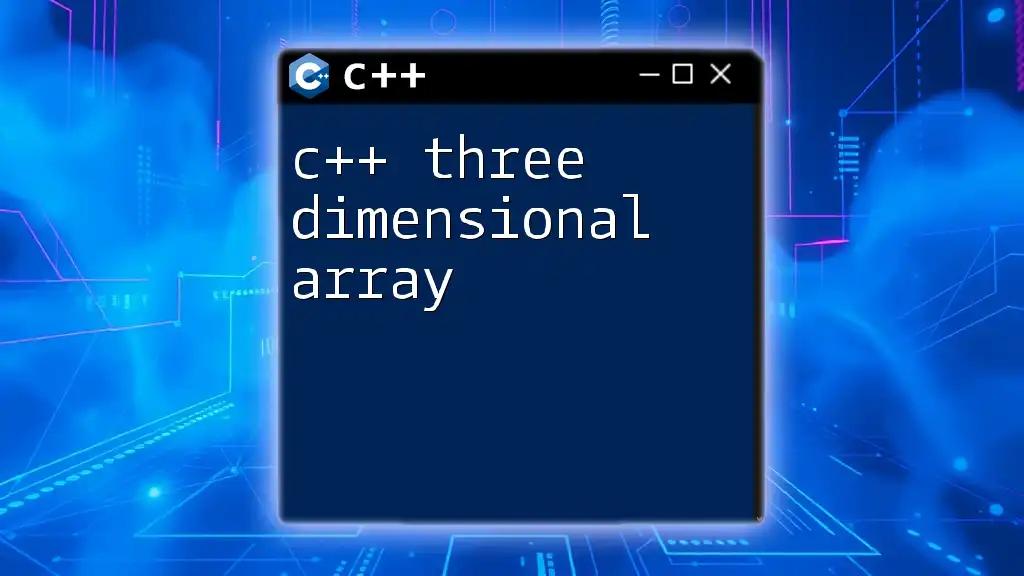
FAQs about C++ Thread Sleep
What is the maximum sleep time for `std::this_thread::sleep_for`?
While `std::this_thread::sleep_for` does not have a predetermined maximum duration, it is limited by the system’s capabilities and the precision of the clock being used.
Can you sleep a thread indefinitely in C++?
Yes, you can effectively create an indefinite sleep by using an extremely long duration, although this is generally not recommended for resource management.
Are there platform-specific limitations to sleeping threads?
Yes, platform-specific limitations exist regarding sleep precision and thread scheduling. It is crucial to test and consider these factors while developing multithreaded applications across different operating systems.