A C++ cheatsheet is a quick reference guide that summarizes essential C++ commands, syntax, and functions to help streamline the learning process for beginners and professionals alike.
Here's a simple example of a C++ program that outputs "Hello, World!" to the console:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
C++ Basics
Variables and Data Types
Defining Variables
In C++, variables must be declared before they can be used. A variable declaration includes a data type and a variable name. Here’s how you can define variables:
int age = 25;
float salary = 50000.00;
Choosing appropriate names is crucial for readability. Following naming conventions—like using camelCase or snake_case—will help maintain clarity in your code.
Common Data Types
C++ offers several fundamental data types that you should be familiar with:
- `int` - Used for integers.
- `char` - Represents a single character.
- `float` - A single-precision floating point.
- `double` - A double-precision floating point, providing more precision than float.
- `bool` - Represents boolean values: `true` or `false`.
Type Modifiers
In addition to the basic types, you can modify them:
- `signed` / `unsigned` - Determines whether the variable can hold negative values.
- `short` / `long` - Specifies the size of the variable, affecting the range of values it can store.
Input and Output
Using `cin` and `cout`
C++ provides `cin` and `cout` from the `<iostream>` library for input and output operations. Here’s a basic example:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl; // Output to the console
int number; // Declare a variable
cin >> number; // Input from the user
return 0;
}
String Output
You can easily manipulate and output strings in C++:
string name = "Alice";
cout << "Hello, " << name << "!" << endl; // Prints: Hello, Alice!
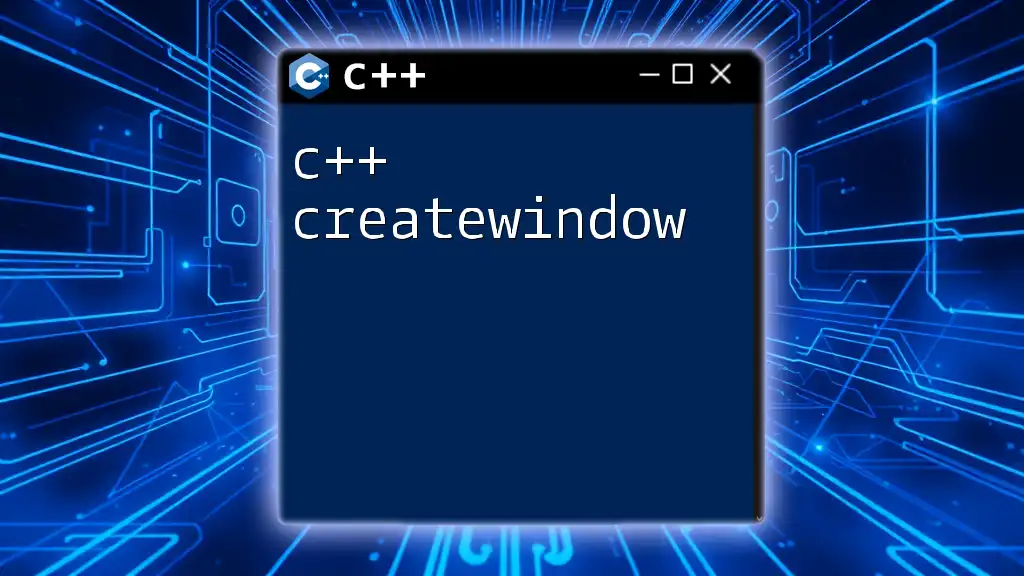
Control Structures
Conditional Statements
if, else if, and else
Conditional statements control the flow based on conditions. Here’s how you can structure them:
if (age >= 18) {
cout << "Adult";
} else {
cout << "Minor";
}
Switch-case Statement
Sometimes a switch-case statement is more efficient than multiple `if-else` statements, especially with many cases:
switch (day) {
case 1:
cout << "Monday";
break;
case 2:
cout << "Tuesday";
break;
// Additional cases for the rest of the week
}
Loops
For Loop
Loops allow you to execute code multiple times. The `for` loop is commonly used when the number of iterations is known:
for (int i = 0; i < 10; i++) {
cout << i << endl; // Prints numbers from 0 to 9
}
While Loop
A `while` loop continues until a specified condition is false:
int i = 0;
while (i < 10) {
cout << i << endl; // Prints numbers from 0 to 9
i++;
}
Do-While Loop
A `do-while` loop guarantees at least one iteration:
int i = 0;
do {
cout << i << endl; // Executes at least once
i++;
} while (i < 10);
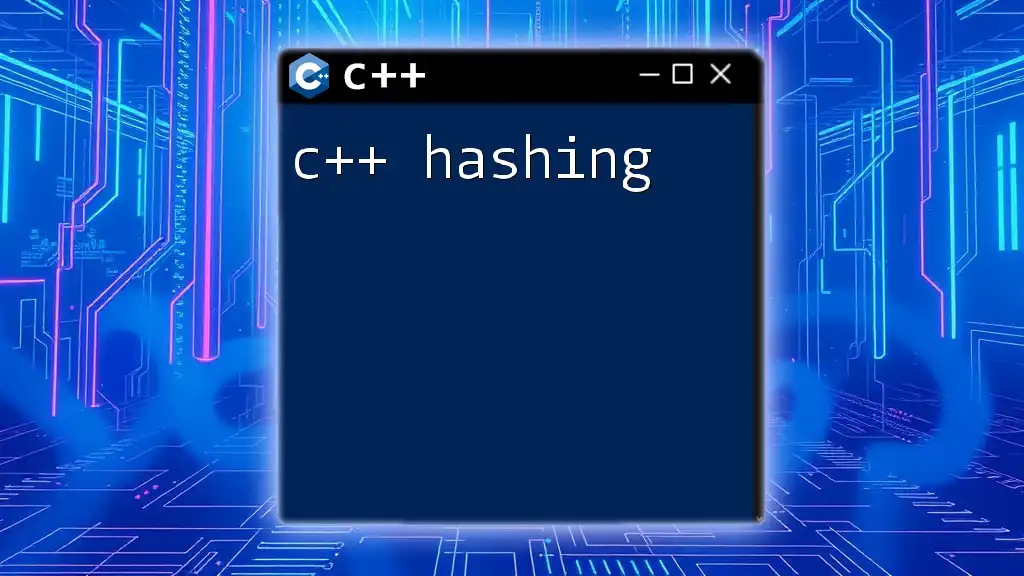
Functions
Defining and Calling Functions
Basic Function Syntax
Functions encapsulate code for reuse. Here’s a simple function example:
int add(int a, int b) {
return a + b; // Returns the sum of a and b
}
Function Overloading
You can have multiple functions with the same name differing by parameters:
int add(int a, int b);
double add(double a, double b);
Return Values
Understanding Return Types
Functions can return values, and you must specify the return type:
double divide(double a, double b) {
return a / b; // Returns the quotient
}
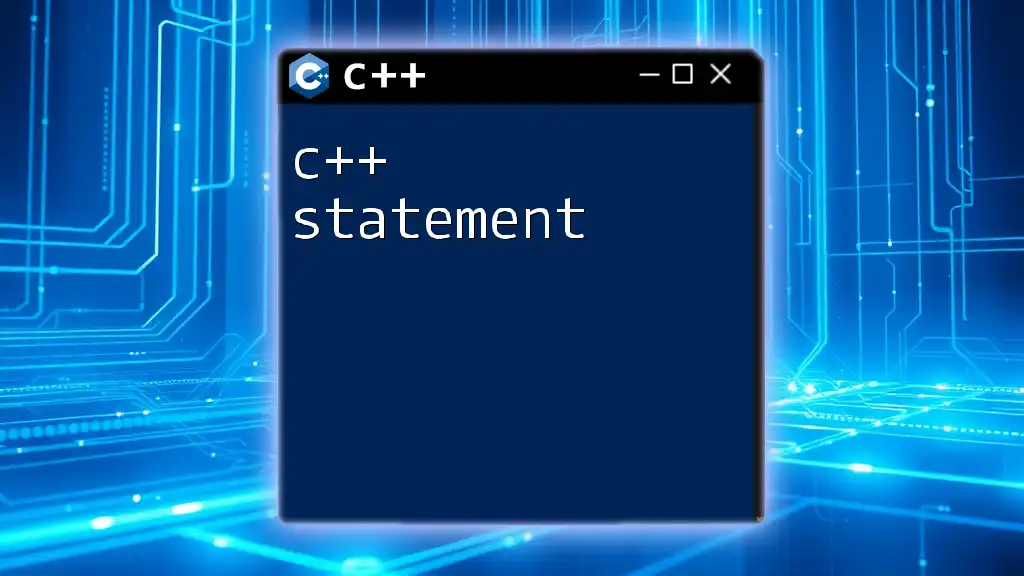
Object-Oriented Programming in C++
Classes and Objects
Defining a Class
C++ is an object-oriented language, and defining a class is straightforward. Here’s a simple class structure:
class Car {
public:
string brand; // Class member variable
void honk() { // Member function
cout << "Beep Beep!";
}
};
Creating Objects
Once a class is defined, you can create instances (objects) of it:
Car myCar; // Create an object of Car
myCar.brand = "Toyota";
myCar.honk(); // Calls the honk() method
Inheritance
Understanding Inheritance
Inheritance allows a class to inherit attributes and methods from another class, promoting reusability. Here’s a basic example:
class Vehicle {
public:
void start() {
cout << "Vehicle starting...";
}
};
class Bike : public Vehicle {
// Inherits from Vehicle
public:
void pedal() {
cout << "Pedaling...";
}
};
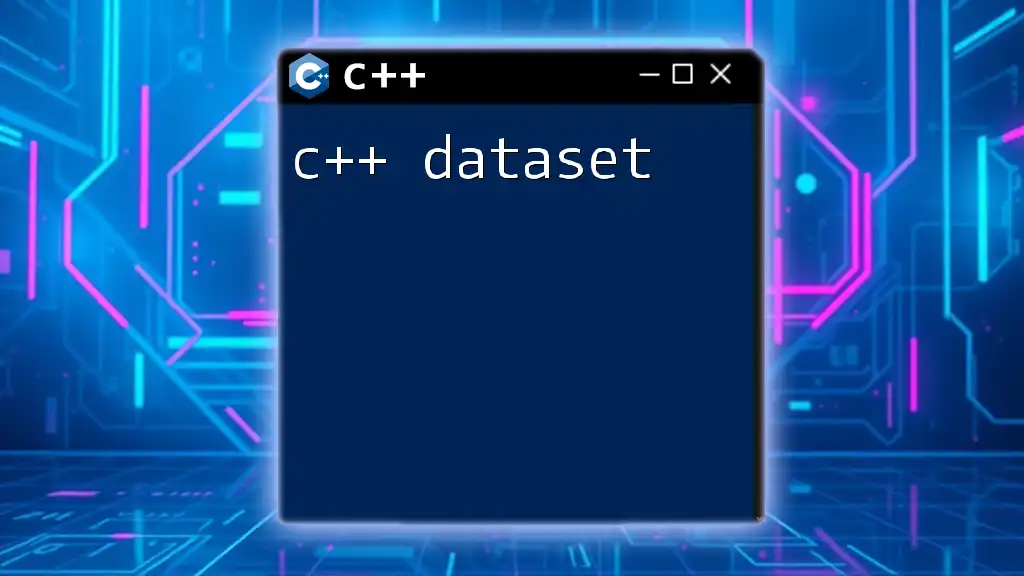
Advanced Topics
Templates
Function Templates
Templates allow functions to operate with different data types. Here's an example of a function template:
template <typename T>
T add(T a, T b) {
return a + b; // Function works with any type
}
Exception Handling
Try-Catch Blocks
Handling errors gracefully is crucial. C++ uses try-catch blocks for this:
try {
// Code that may throw an exception
int a = 10, b = 0;
if (b == 0) throw "Division by zero!";
cout << a / b;
} catch (const char* msg) {
cout << msg; // Handle the error
}
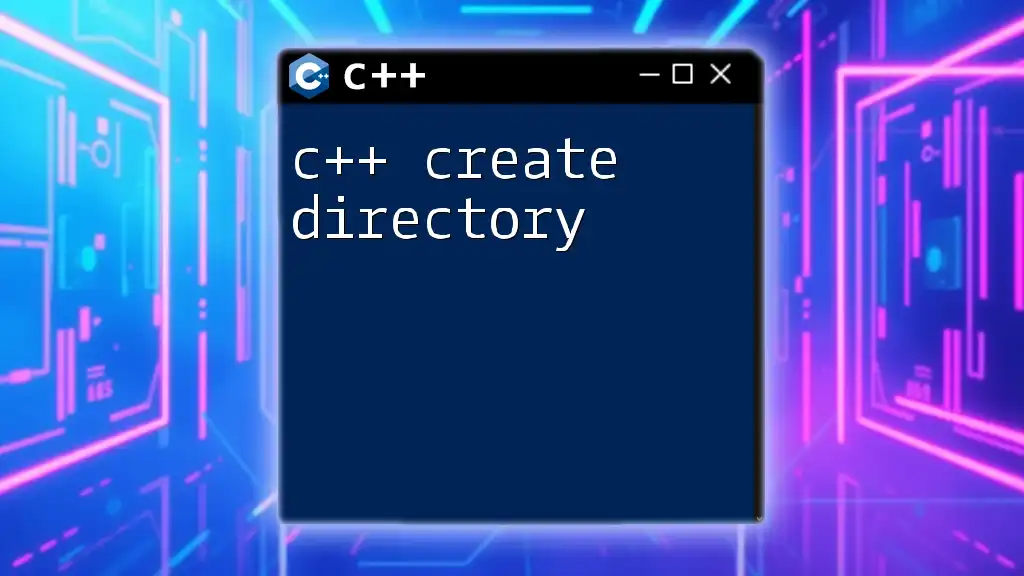
Conclusion
In this C++ cheatsheet, you've covered the core concepts that are essential for both beginners and seasoned programmers. From understanding data types, control structures, and functions, to mastering OOP and advanced topics like templates and exception handling—this guide provides a strong foundation to dive deeper into C++ programming.
As you continue your learning journey, consider exploring more advanced resources, participating in community discussions, and practical coding exercises to hone your skills. Happy coding!