C++ hashing involves using hash functions to convert data into a fixed-size value, often for efficient data retrieval in data structures like hash tables.
Here’s a simple code snippet demonstrating how to use the `std::hash` function in C++:
#include <iostream>
#include <functional>
int main() {
std::string key = "example";
std::hash<std::string> hash_fn;
std::size_t hash_value = hash_fn(key);
std::cout << "Hash value of '" << key << "' is: " << hash_value << std::endl;
return 0;
}
What is Hashing?
Hashing is a fundamental concept in computer science that involves transforming input data (or keys) into a fixed-size numerical value called a hash code. This process is crucial for various applications, including data integrity verification, fast data retrieval, and efficient storage. In the context of C++, hashing provides efficient ways to handle dynamic datasets through structures like hash tables and hash maps.
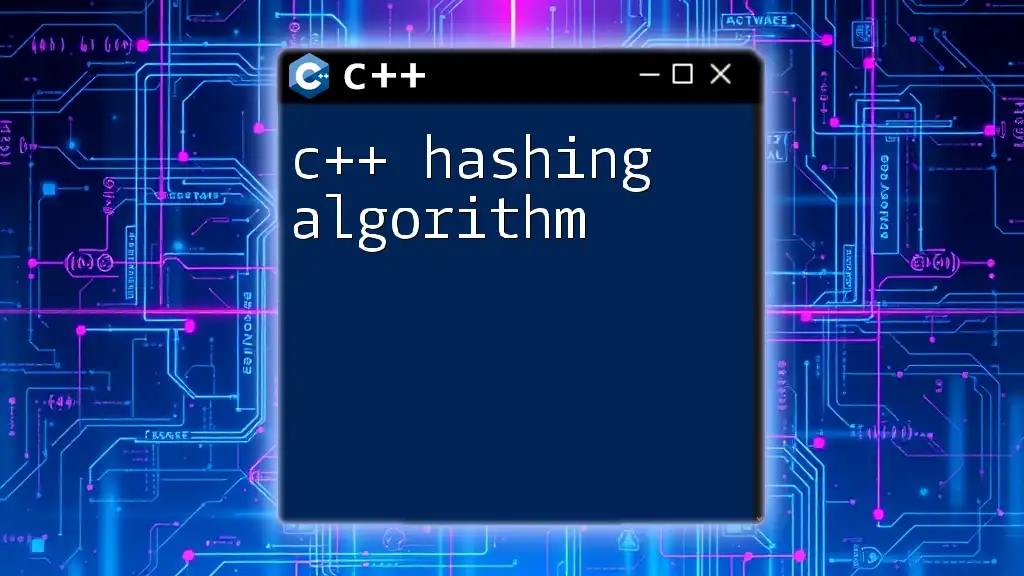
Applications of Hashing
The applications of hashing are extensive and varied:
- Data Integrity and Verification: Hash functions help ensure that data has not been altered. By comparing hash codes before and after data transfer, discrepancies can be detected.
- Password Storage: Securely storing user passwords involves converting them into hash codes so that the original text cannot be easily retrieved.
- Data Structures: Hash tables and hash maps leverage hashing to enable quick access to data, typically in constant time.
- Caching and Indexing: Hashing simplifies data retrieval. By using hash codes as keys, large datasets can be indexed and accessed more rapidly.
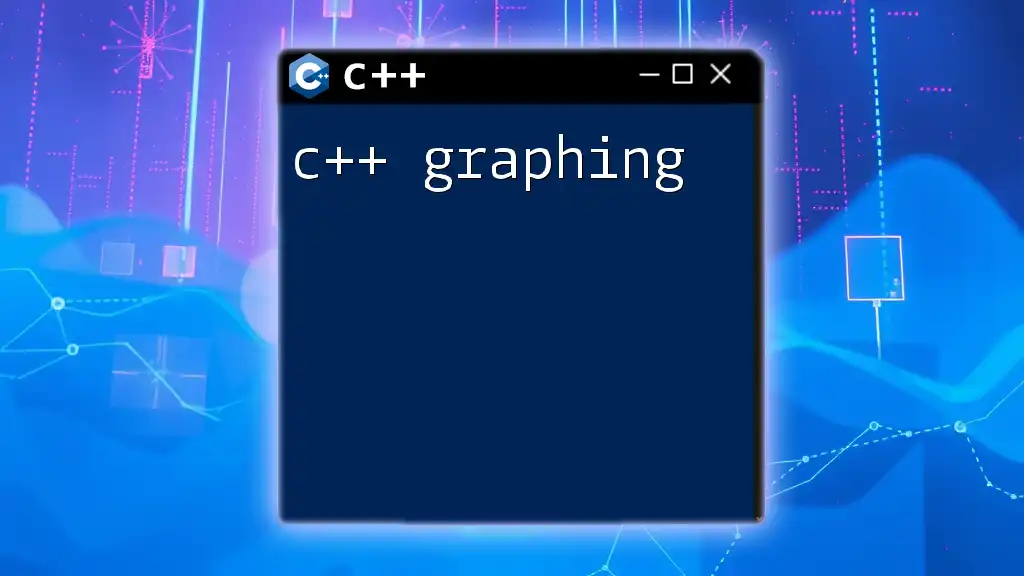
Understanding Hash Functions
What is a Hash Function?
A hash function is a specific algorithm that converts data into a fixed-size string of characters, which appears random. Key characteristics of an effective hash function include:
- Deterministic: The same input will always produce the same output.
- Fast Computation: The function should compute the hash quickly.
- Uniform Distribution: The hash values should be spread evenly across the output range to minimize collisions.
Example of a Simple Hash Function
Creating a basic hash function in C++ can be straightforward. Here’s an example using string input:
int simpleHash(const std::string &key) {
int hash = 0;
for (char c : key) {
hash += c; // Simple accumulation of ASCII values
}
return hash;
}
In this `simpleHash` function, each character in the input string contributes to the end hash value, making it easy to understand yet effective for simple applications.
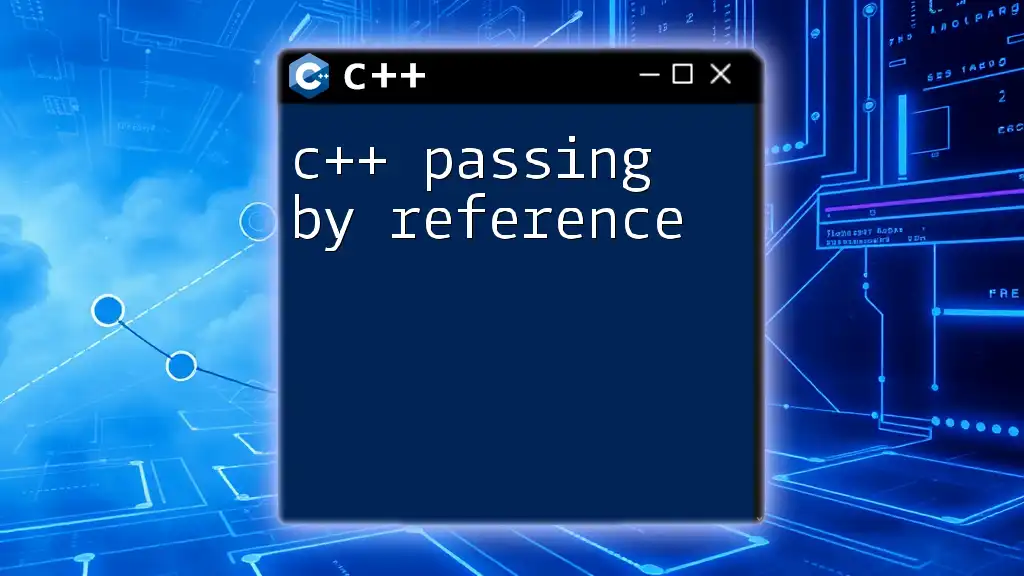
Hashing Techniques in C++
Common Hashing Algorithms
Several popular hashing algorithms are widely used for various purposes:
- MD5: A widely known hashing algorithm primarily used for data integrity checks. However, it's not suitable for secure password hashing due to vulnerabilities.
- SHA-1: Offers better security than MD5, but it has fallen out of favor due to discovered vulnerabilities as well.
- SHA-256: Part of the SHA-2 family, it offers one of the most secure hashing methods currently available, making it suitable for sensitive data.
Implementing a Hash Function in C++
Building a custom hash function is often necessary. Here’s how you can create a more structured hash using a class:
struct CustomHash {
size_t operator()(const std::string &key) const {
size_t hash = 0;
for (char c : key) {
hash = hash * 31 + c; // Using polynomial rolling hash technique
}
return hash;
}
};
In this `CustomHash` structure, we use the polynomial rolling hash technique, which minimizes collisions by distributing hash values evenly across a range.
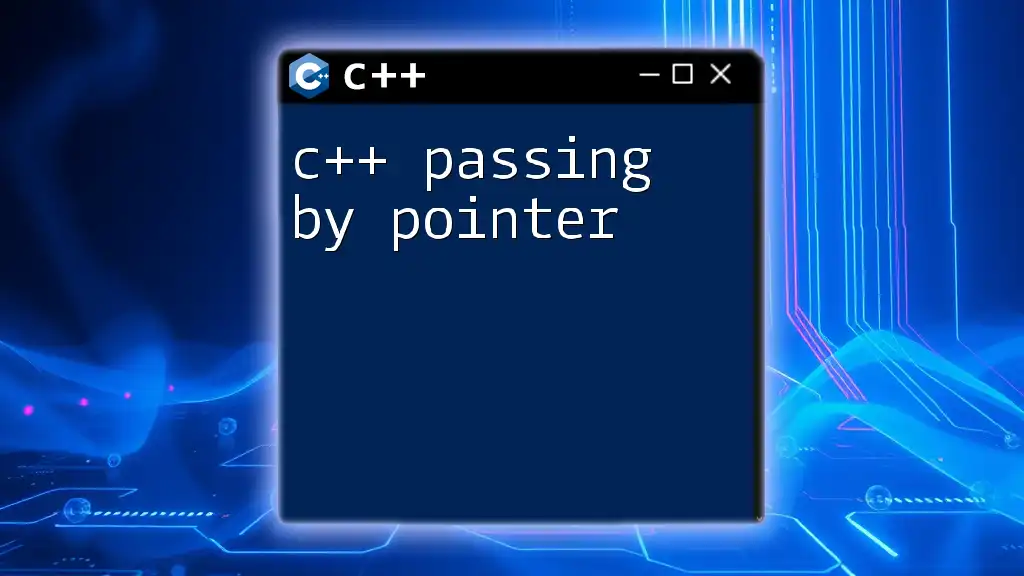
Hash Tables in C++
What is a Hash Table?
A hash table is a data structure that pairs keys to values for efficient data access. It supports essential operations, including:
- Insert: Adding a new key-value pair.
- Delete: Removing a key and its associated value.
- Search: Finding a value associated with a key.
Implementing a Hash Table
Creating a basic hash table involves setting its structure and operations. Here’s an example:
#include <vector>
#include <list>
#include <utility>
#include <string>
class HashTable {
private:
std::vector<std::list<std::pair<std::string, int>>> table;
size_t size;
public:
HashTable(size_t size) : size(size) {
table.resize(size);
}
void insert(const std::string &key, int value) {
size_t index = simpleHash(key) % size;
table[index].emplace_back(key, value);
}
// Additional methods such as search and delete would go here...
};
In this `HashTable` class, we've initialized a vector of lists, allowing for separate chaining to handle collisions. Each list can store multiple key-value pairs.
Collision Resolution Techniques
Collisions occur when two keys hash to the same index. Effective handling techniques are crucial for maintaining the performance of hash tables:
- Separate Chaining: Each index in the hash table holds a list of entries. When a collision occurs, the new entry is simply appended to this list.
- Open Addressing: When a collision occurs, the hash table probes for the next available index.
- Linear Probing: This is one form of open addressing where consecutive slots are checked until an empty one is found.
Code Example: Handling Collisions
Here's how you would implement insertion using separate chaining:
void insert_with_chaining(const std::string &key, int value) {
size_t index = simpleHash(key) % size;
table[index].emplace_back(key, value); // Insert in the list at the computed index
}
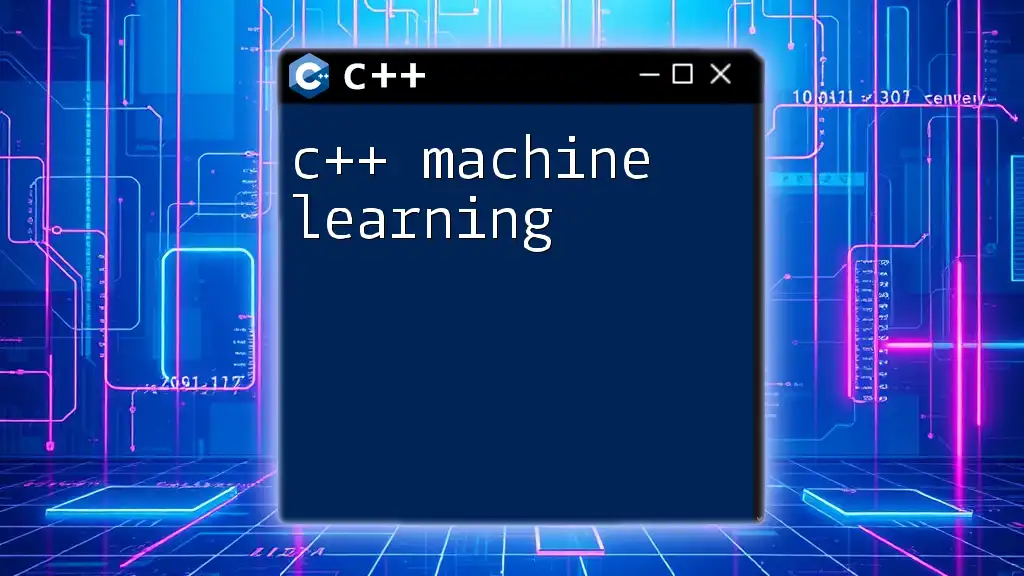
Performance Considerations
Time Complexity of Hash Operations
The average time complexity for basic hash table operations is typically O(1). However, this can degenerate to O(n) in the worst case, particularly when many collisions occur.
Factors Affecting Hash Table Performance
- Load Factor: This metric indicates how full the hash table is. A higher load factor can lead to more collisions.
- Choosing an Appropriate Size: A poorly sized hash table can hinder performance due to excessive collisions.
- Quality of the Hash Function: A well-designed hash function minimizes collisions and ensures quicker access.
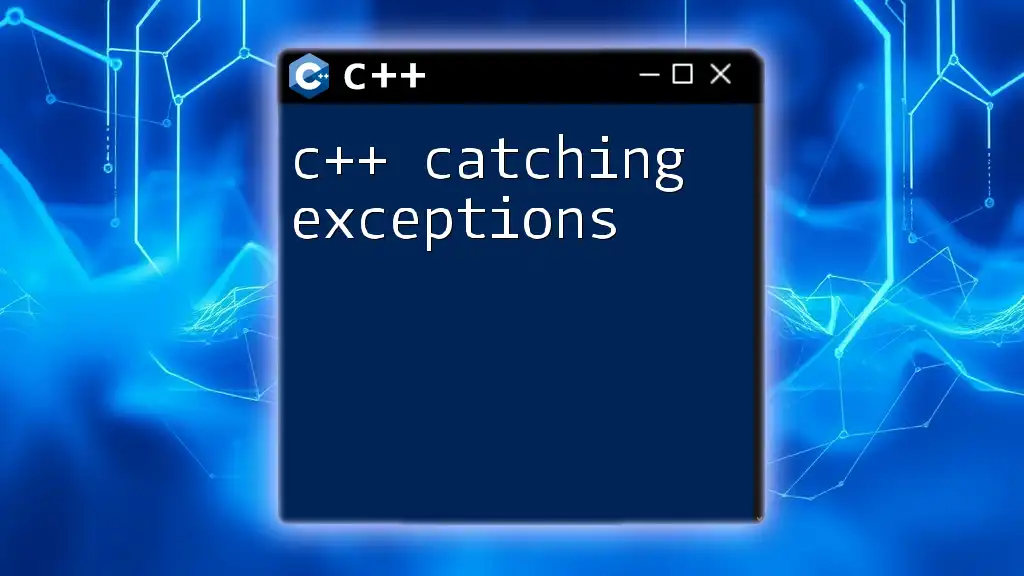
Real-world Use Cases of Hashing in C++
Use Case 1: Caching
Caching is a strategy to speed up data retrieval by storing copies of frequently accessed data. Hash maps are often used to implement caches due to their high-speed access times. In C++, you can leverage `std::unordered_map` for this purpose:
#include <unordered_map>
std::unordered_map<std::string, int> cache;
Use Case 2: Password Hashing
In modern applications, it is critical to store passwords securely. A basic password hashing strategy in C++ might look like this:
std::string hashPassword(const std::string &password) {
return hashFunction(password); // hashFunction would be defined earlier
}
This approach ensures that even if the database is compromised, the actual passwords remain safe since only the hash is stored.
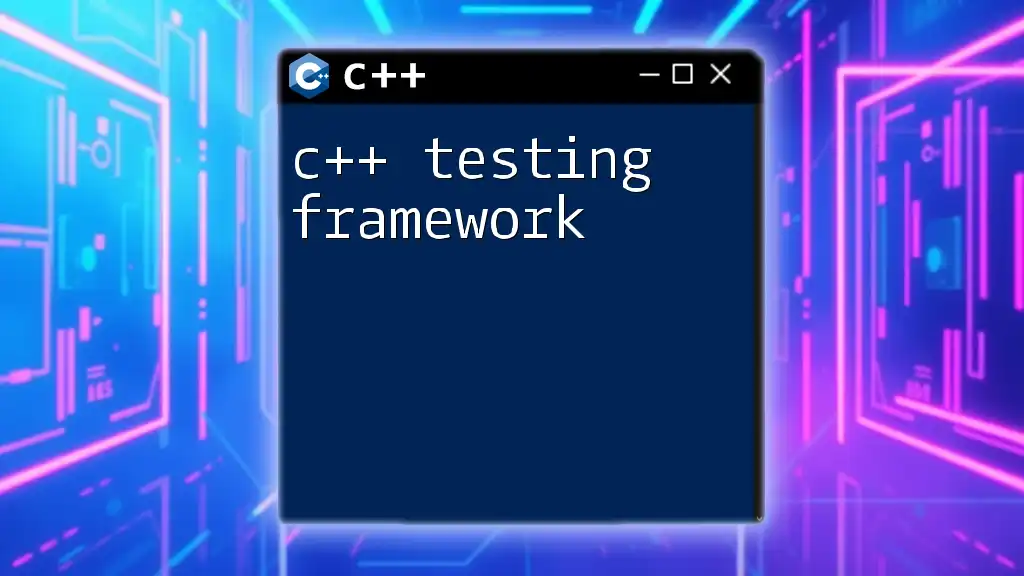
Conclusion
In this comprehensive guide to C++ hashing, we explored the fundamental aspects of hashing, the characteristics of effective hash functions, the implementation of hash tables, and various practical applications. Understanding hashing is essential for any programmer looking to build efficient and reliable software solutions.
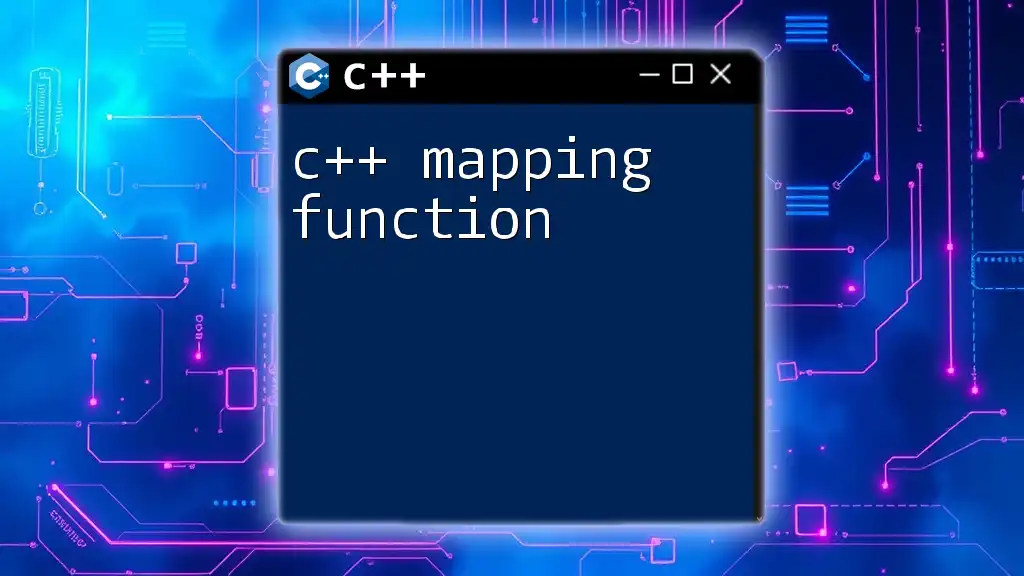
Additional Resources
For those eager to delve deeper into the topic of C++ hashing, consider exploring advanced C++ libraries, books on algorithm design, and online tutorials focusing on data structures.
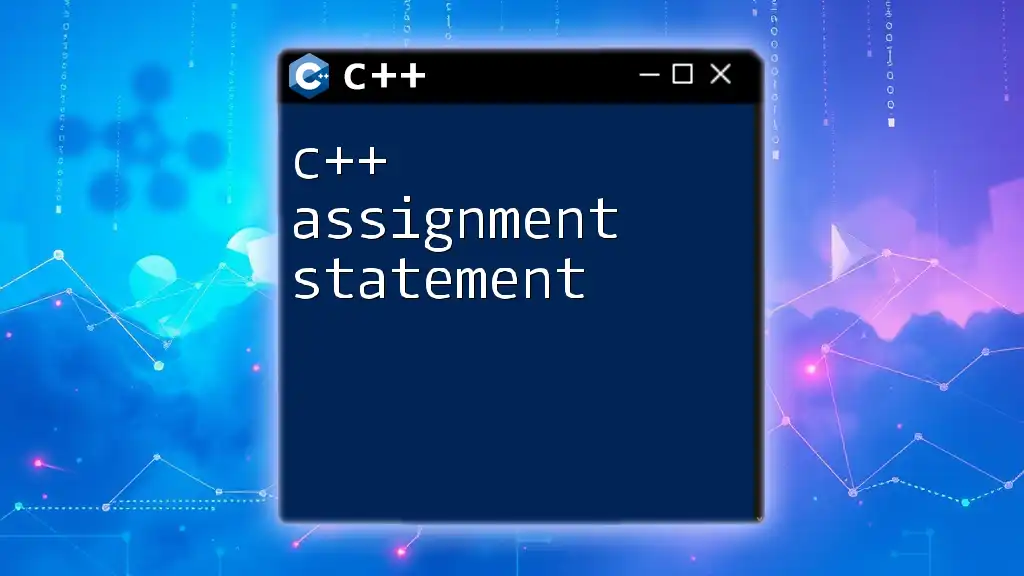
Call to Action
Now that you have a solid foundation in C++ hashing, it's time to practice. Implement your own hash function or create a hash table to cement these concepts in your memory. Feel free to share your experiences or any questions you may have, as hashing is a vast and intriguing subject!