SFINAE (Substitution Failure Is Not An Error) is a C++ feature that allows function templates to be conditionally enabled or disabled based on the types of their template parameters, enabling more flexible and context-specific template specialization.
Here's a simple example demonstrating SFINAE:
#include <iostream>
#include <type_traits>
// Function template that is enabled only if T is an integral type
template<typename T>
typename std::enable_if<std::is_integral<T>::value, void>::type
process(T value) {
std::cout << value << " is an integral type." << std::endl;
}
// Overload for floating point types
template<typename T>
typename std::enable_if<std::is_floating_point<T>::value, void>::type
process(T value) {
std::cout << value << " is a floating point type." << std::endl;
}
int main() {
process(42); // Calls the integral version
process(3.14); // Calls the floating point version
return 0;
}
Understanding the Basics of Templates
Templates in C++
In C++, templates are a powerful feature that allows developers to create generic and reusable code. A template can be thought of as a blueprint for creating functions or classes. Here are the main types of templates:
-
Function Templates: These allow you to define a function without specifying the exact type of its arguments. The compiler generates the function code for each specific type used.
-
Class Templates: Similar to function templates, class templates provide a way to define classes that can operate with any data type.
Templates enable you to create code that is more flexible and capable of handling various data types without sacrificing type safety.
The Role of Type Deduction
Type deduction occurs when the compiler infers the type of template parameters based on the function's or class's arguments. In C++, type traits can help developers apply conditions to the operations that must be performed, depending on the type being processed. This aspect of templates plays a crucial role in implementing SFINAE.
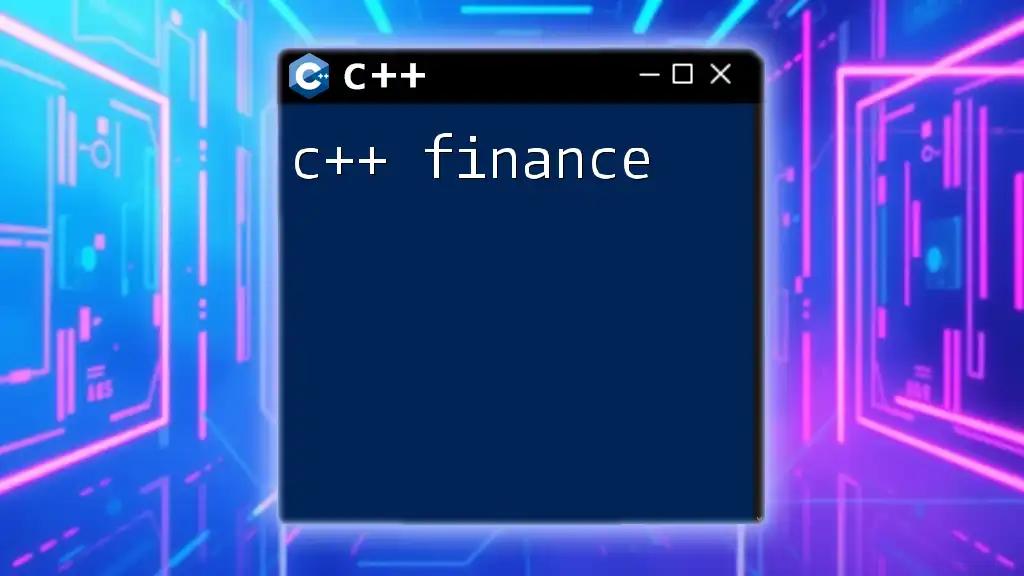
The Mechanism Behind SFINAE
How SFINAE Works
SFINAE, or Substitution Failure Is Not An Error, is a principle that enables the compiler to ignore particular template instantiations that are incompatible with given types while not resulting in a compile-time error. This means that if a substitution in a template argument results in an invalid type, the compiler will not generate an error; it will simply look for other potential candidates.
When leveraging SFINAE, the compiler uses a mechanism called overload resolution. This mechanism prioritizes which template function applies to the given types and allows for graceful degradation when an instantiation fails.
Conditionally Excluded Functions
One of the key features of SFINAE is the ability to create functions that are conditionally excluded based on the template parameters provided. This can be extremely powerful when you want certain functions to only be available for specific types. An example of such a function can be seen here:
template<typename T, typename = std::enable_if_t<std::is_integral<T>::value>>
void process(T value) {
// This function is only callable if T is an integral type
}
In this example, the `process` function will only be instantiated for integral types (like `int`, `short`, etc.). Any attempt to call `process<float>(3.5)` would lead to that specialization being excluded, rather than throwing a compile-time error.

Practical Applications of SFINAE
Usage in Template Specialization
SFINAE can be beneficial for template specialization, allowing you to define different behaviors depending on type characteristics dynamically. Here’s how you might specialize a template based on type traits:
template<typename T, typename = std::enable_if_t<std::is_floating_point<T>::value>>
void process(T value) {
// This function is only callable if T is a floating-point type
}
In this case, the `process` function is designed explicitly for floating-point types. Attempting to pass an integer to `process` would exclude it gracefully.
Detecting Function Availability
SFINAE can also help in detecting whether a particular function or member type exists. This can be achieved using `decltype` and `std::void_t`. Below is an example that checks if a type has a specific member function:
template<typename T, typename = void>
struct has_member_function : std::false_type {};
template<typename T>
struct has_member_function<T, std::void_t<decltype(std::declval<T>().member_function())>> : std::true_type {};
This construct checks if the type `T` has a member function called `member_function` and allows you to conditionally enable or disable template instantiation options based on its existence.
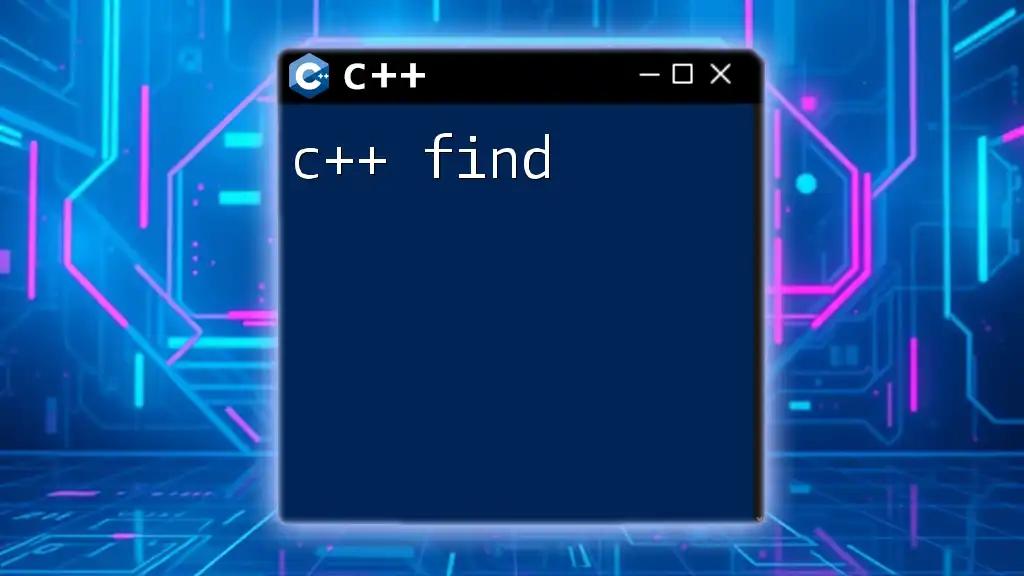
Advanced SFINAE Techniques
Nested SFINAE
Nested SFINAE refers to the use of SFINAE within SFINAE to create complex validations. You can create multilayered checks that offer intricate resolutions for template arguments. Here is a simple illustration:
template<typename T, typename Enable = void>
struct process_helper;
template<typename T>
struct process_helper<T, std::enable_if_t<std::is_integral<T>::value>> {
// Specialization for integral types
static void process(T value) {
// Handle integral value
}
};
template<typename T>
struct process_helper<T, std::enable_if_t<std::is_floating_point<T>::value>> {
// Specialization for floating-point types
static void process(T value) {
// Handle floating-point value
}
};
In this example, `process_helper` specializes handling for integral and floating-point types but allows for expansion in different ways as necessary.
SFINAE vs. Concepts
While SFINAE has been a cornerstone in template programming, C++20 introduces concepts, which provide a more concise way to specify template requirements. Concepts allow developers to express constraints more clearly.
For instance, instead of using `std::enable_if`, you could define a concept like this:
template<typename T>
concept Integral = std::is_integral_v<T>;
template<Integral T>
void process(T value) {
// This will only accept integral types
}
Concepts enhance code readability and maintainability, making the intent clearer than what SFINAE often accomplishes with more complex syntax.
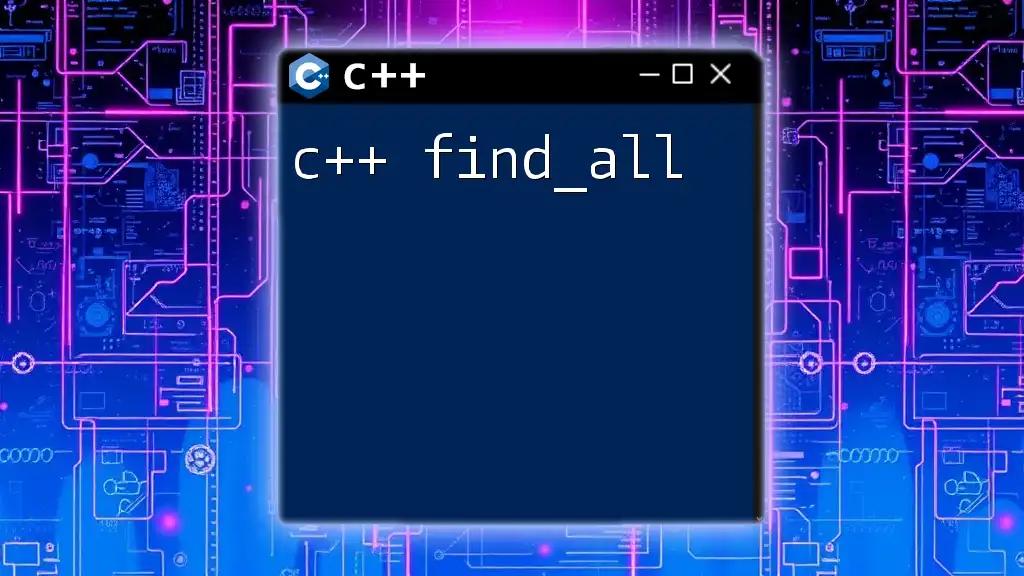
Common Pitfalls in Using SFINAE
Mistakes to Avoid
One common misunderstanding is assuming SFINAE can provide complete type safety without consideration of all potential overloads. In specific scenarios, it might miss certain type validations or inadvertently provide the wrong candidate.
Additionally, developers often forget that SFINAE only applies to certain types of substitution failures. If an issue arises unrelated to type substitution, the compiler will still throw an error.
Performance Considerations
While SFINAE is powerful, it can introduce template bloat—a situation where many distinct instantiations are created. Each unique set of template parameters can lead to increased compile times and larger binaries.
To optimize, use SFINAE judiciously and avoid excessive complexity that can lead to compile-time overhead. Where possible, consider using simpler alternatives or profiling your code to identify performance bottlenecks.
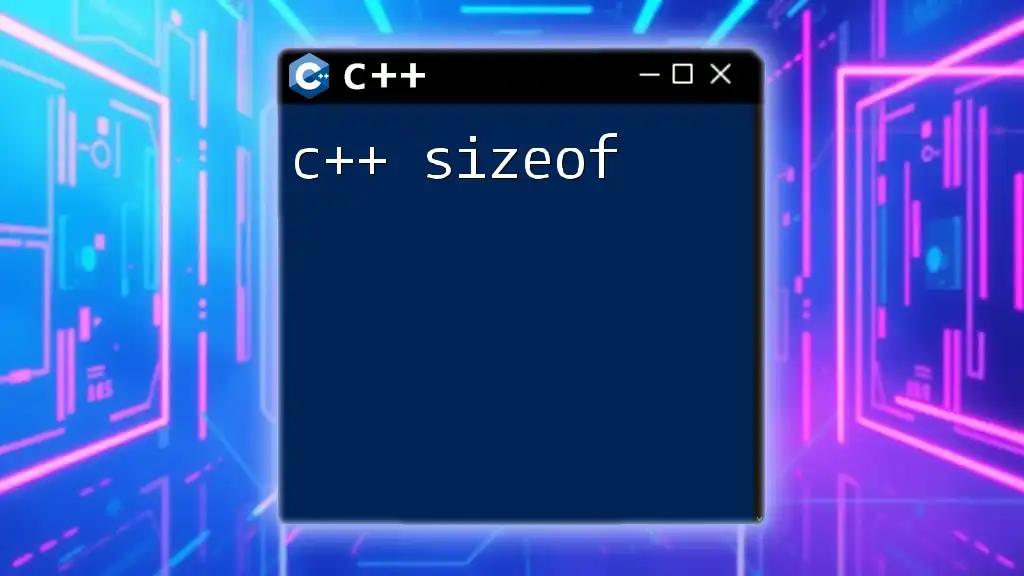
Conclusion
SFINAE (Substitution Failure Is Not An Error) is a vital concept in C++ that enables developers to write more flexible and powerful template code. Mastering SFINAE allows you to create conditions for type traits, specialize behavior based on type characteristics, and detect member functions seamlessly.
Through careful implementation and awareness of its nuances, SFINAE can lead to cleaner, safer, and more efficient C++ code. As the language continues to evolve with the introduction of concepts and improved template facilities, understanding SFINAE will remain invaluable for leveraging the power of C++.
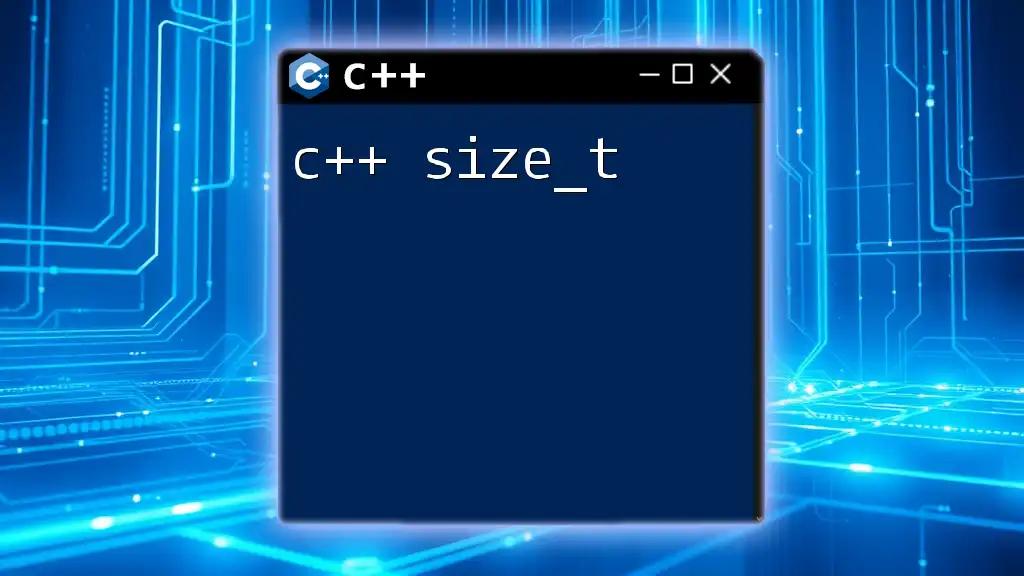
Further Learning Resources
For those looking to deepen their understanding of C++ SFINAE, consider exploring the following resources:
- Books: "Effective Modern C++" by Scott Meyers
- Articles: Tutorials on sites like cppreference.com or Stack Overflow
- Online Courses: Platforms such as Coursera and Udemy that offer specialized C++ programming classes
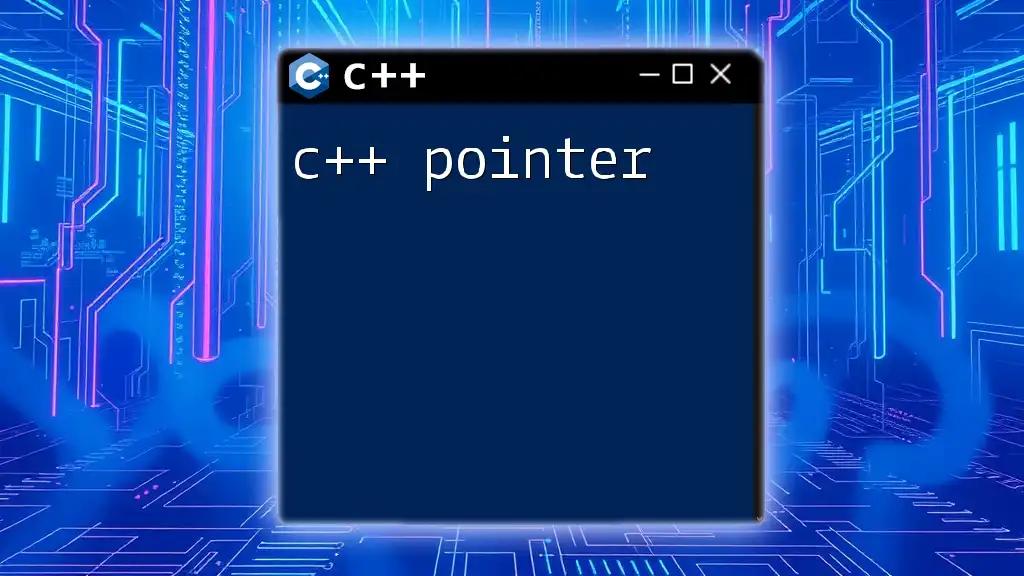
Call to Action
We encourage you to share your experiences with C++ SFINAE and engage with our community. If you found this guide helpful, consider signing up for our courses to further enrich your knowledge in C++. Happy coding!