The `fixed` manipulator in C++ is used to set the floating-point output format to fixed-point notation, which means that numbers will be displayed with a specified number of decimal places.
Here's a code snippet demonstrating its use:
#include <iostream>
#include <iomanip>
int main() {
double num = 123.456789;
std::cout << std::fixed << std::setprecision(2) << num << std::endl; // Output: 123.46
return 0;
}
What is `fixed`?
The term `fixed` in C++ refers to a stream manipulator that alters the behavior of output for floating-point types. When applied, it forces the output stream to display numbers in a fixed-point notation rather than scientific notation. This capability is particularly useful in scenarios where precision and readability are paramount. In contexts like financial reporting or scientific measurements, presenting data in a clear and consistent manner can make a significant difference.
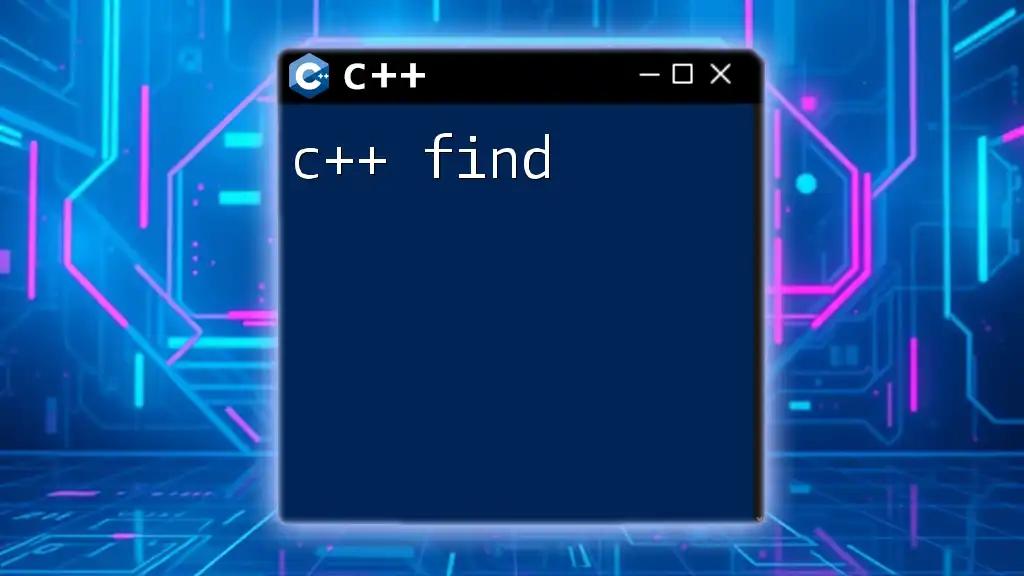
Why Use `fixed` in C++?
Using `fixed` enhances your formatting capabilities, allowing you to manage the precision in your numerical outputs effectively. When working in applications like finance or engineering, the ability to control how many digits appear after the decimal point in your outputs is essential.
Another area where `fixed` shines is in comparison to other formatting options. Without it, numbers may automatically be displayed in scientific notation when they exceed certain limits, which can make them harder to read for typical users or stakeholders.
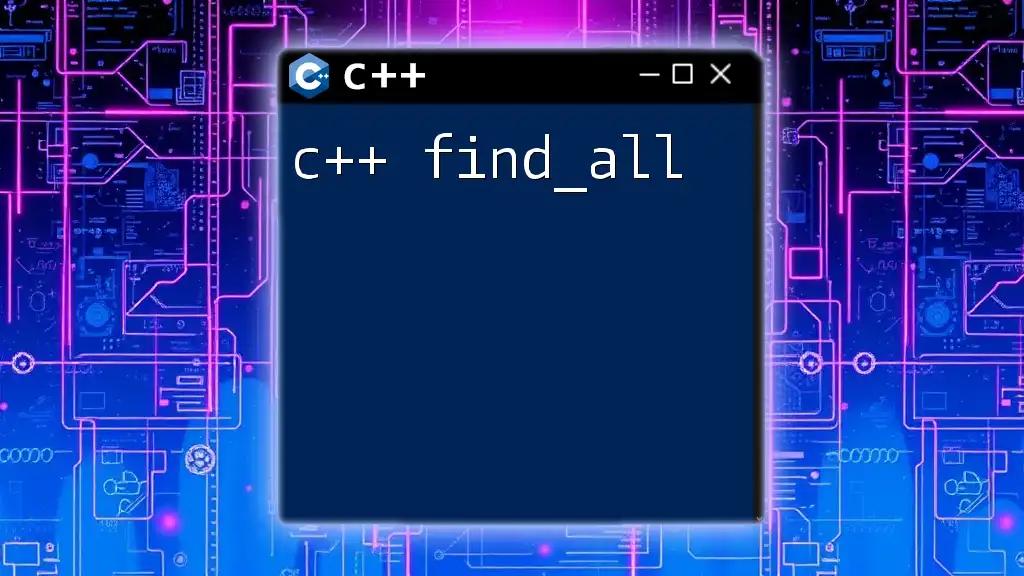
Understanding the C++ Fixed Manipulator
Basic Syntax of `fixed`
The `fixed` manipulator is part of the `<iomanip>` library, which provides various formatting options for input and output streams. To use `fixed`, you must ensure to include the appropriate header:
#include <iostream>
#include <iomanip>
The basic syntax of using `fixed` is straightforward. It is commonly used in combination with the `std::cout` object to display formatted output:
std::cout << std::fixed; // Activates fixed-point notation
Example of Using `fixed`
Here’s an example demonstrating the use of `fixed` in a simple C++ program:
#include <iostream>
#include <iomanip> // For std::fixed
int main() {
double value = 123.456789;
std::cout << std::fixed << value << std::endl; // Output: 123.457
return 0;
}
In this example, we declare a double variable `value` and use `fixed` to ensure that it is printed as a fixed-point number. The output rounds the number to three decimal places by default, even though it has many more digits in its value.

Format Specifiers with `fixed`
Controlling Decimal Places
One of the most powerful features of the `fixed` manipulator is its ability to control the number of decimal places displayed in your output. You can achieve this using the `setprecision` manipulator, which adjusts the total number of digits to appear after the decimal point.
Here's an example demonstrating how to set precision:
#include <iostream>
#include <iomanip>
int main() {
double value = 123.456789;
std::cout << std::fixed << std::setprecision(2) << value << std::endl; // Output: 123.46
return 0;
}
In this case, by setting the precision to 2, we round the number to two decimal places, which results in `123.46`. This is incredibly useful when displaying monetary amounts or other metrics that require specific decimal formats.
Fixed vs Scientific Notation
Understanding the difference between `fixed` and scientific notation is crucial. When you don't apply `fixed`, large or very small floating-point numbers may automatically be displayed in scientific form, making them harder to interpret. For instance, a number like `123456.789` would appear as `1.234568e+05` if you haven’t set the output to `fixed`.
Choosing the correct format is vital based on context—financial reports might require fixed-point formatting, while scientific analysis may occasionally necessitate scientific notation.

Practical Applications of `fixed` in C++
Financial Calculations
In financial applications, presenting values with appropriate decimal places is a necessity. The `fixed` manipulator is perfect for displaying currency values consistently. Here’s an example:
#include <iostream>
#include <iomanip>
int main() {
double amount = 1500.567;
std::cout << "Amount: $" << std::fixed << std::setprecision(2) << amount << std::endl; // Output: Amount: $1500.57
return 0;
}
When you run this program, it formats the monetary value as `1500.57`, helping financial analysts present a professional-looking output.
Engineering Data Representation
In engineering, precision in measurements is critical. The `fixed` manipulator makes it easy to display precise measurement values consistently. Consider this example:
#include <iostream>
#include <iomanip>
int main() {
double length = 1234.56789;
std::cout << "Length: " << std::fixed << std::setprecision(3) << length << " units" << std::endl; // Output: Length: 1234.568 units
return 0;
}
Here, setting the precision to 3 helps communicate a precise measure accurately. In scenarios where accuracy is critical, such formatting avoids misunderstandings.

Common Mistakes When Using `fixed`
Forgetting to Include Necessary Headers
One of the most common mistakes when using `fixed` is failing to include the proper header, `<iomanip>`. Without this header, the compiler won’t recognize `setprecision` and `fixed`, leading to compilation errors. Always remember to include it at the top of your source file.
Misunderstanding the Scope of `fixed`
Another common pitfall is misunderstanding the scope of the `fixed` manipulator. It's essential to know that `fixed` affects only the subsequent outputs following its declaration. If you desire a consistent application across all outputs, you should set it before all output statements.
Not Setting the Right Precision
An often-overlooked aspect of using `fixed` is the appropriate setting of precision. Using too high or too low a precision can lead to confusion and misinterpretation of data. Be mindful of the levels of precision necessary for your particular application.

Conclusion: Mastering C++ Fixed
The `fixed` manipulator in C++ provides programmers with robust formatting capabilities essential for presenting floating-point numbers clearly and accurately. Mastering its use, along with the complementary `setprecision` manipulator, will significantly improve your ability to format output in a variety of applications.
By practicing `fixed` in practical scenarios—like financial analysis or engineering calculations—you can ensure that your data is presented professionally, facilitating better understanding and decision-making.
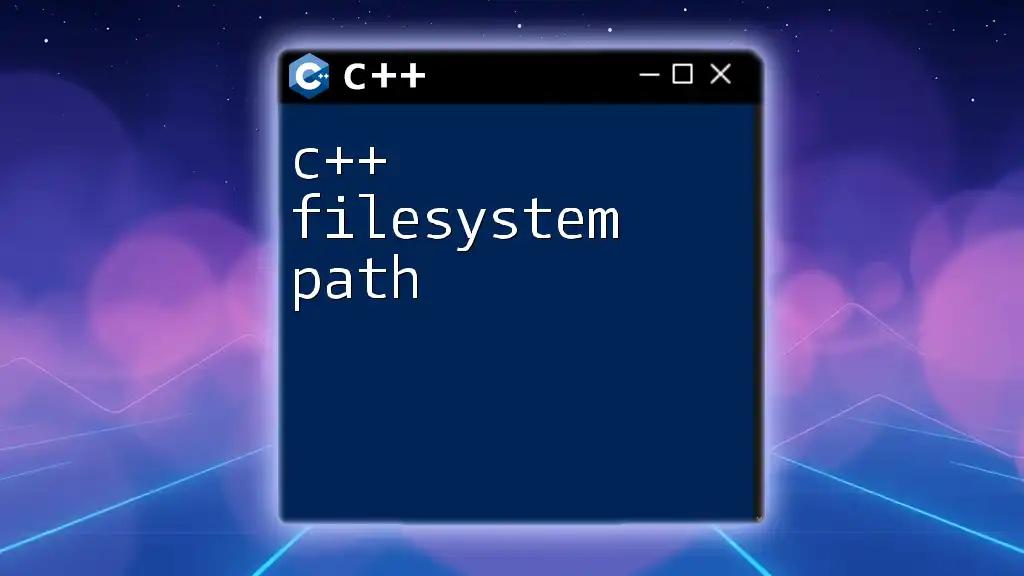
FAQs About Fixed in C++
How does `fixed` affect floating-point types?
The `fixed` manipulator primarily affects the manner in which floating-point numbers are presented. It displays them in a consistent decimal format rather than in scientific notation.
Can `fixed` be used in input operations?
While `fixed` is handy for output, it does not directly influence input operations. Its primary role is in determining how numbers are displayed in streams.
What happens if I forget to use `setprecision` with `fixed`?
If you skip `setprecision`, the output produced by `fixed` will still have a default precision (usually six decimal places), which may or may not meet your needs, especially in situations requiring specific formatting.
Best Practices for Using `fixed` in C++
- Always include the necessary headers: `<iomanip>`.
- Use `setprecision` alongside `fixed` to control the number of decimal places effectively.
- Familiarize yourself with the context to decide whether to use `fixed` or scientific notation based on your needs.
- Test outputs frequently to ensure you’re receiving the desired formatting.
- Make it a habit to comment on your code, clarifying the use of manipulators like `fixed` and `setprecision` for others who may read your code later.
By following these guidelines, you can enhance your C++ coding skills and output formatting in a significant way.