"C++ fix" typically refers to correcting errors or inefficiencies in C++ code to ensure proper functionality and performance.
Here's a simple code snippet demonstrating a fix for a common issue, such as an uninitialized variable:
#include <iostream>
int main() {
// Fixing uninitialized variable warning
int x = 0; // Initialize the variable
std::cout << "The value of x is: " << x << std::endl; // Output the value of x
return 0;
}
Understanding Common C++ Errors
Syntax Errors
A syntax error occurs when you violate the rules of the C++ language. It could be as simple as a missing semicolon at the end of a statement. Syntax errors are typically caught by the compiler, which gives you error messages pointing to the line where the error occurred.
Examples of syntax errors include:
int main() {
cout << "Hello World" // Missing semicolon
}
To fix syntax errors, carefully read the error messages provided by your compiler. They usually include the line number and a brief description of what went wrong. Make it a habit to check your brackets, semicolons, and data types consistently.
Runtime Errors
Runtime errors are not detected until the program runs, making them trickier to spot. These errors occur during execution, often due to logical mistakes or invalid operations, such as dividing by zero.
For example:
int main() {
int a = 5;
int b = 0;
cout << a / b; // This will cause a runtime error
}
To debug runtime errors, you can use various methods like inserting print statements to understand variable values in real-time or employing modern debugging tools such as GDB.
Logic Errors
A logic error occurs when your program runs but produces incorrect results. Unlike syntax errors, your code may be perfectly syntactically correct, but the logic you've built into the program may not yield the expected output.
For example, consider this simple miscalculation:
int main() {
int a = 5, b = 10;
cout << a + b * 2; // Misplaced operations; would yield 25 instead of 30 if the intent was to sum first
}
To resolve logic errors, step through your code with a debugger or carefully review your calculations, conditions, and problem-solving approaches to identify where you’ve strayed from your intended logic.
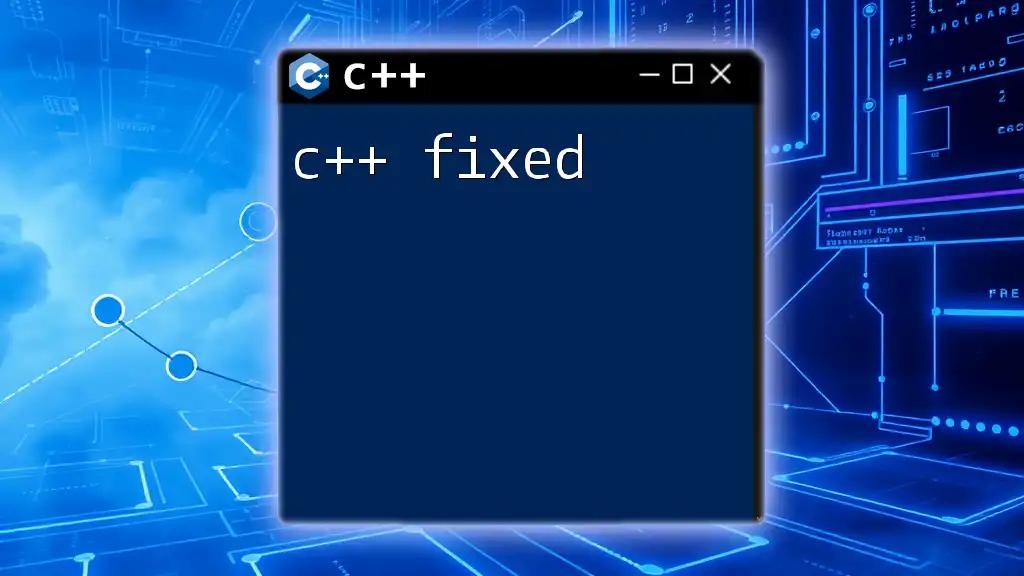
Using Tools for C++ Fix
IDEs and Compilers
Utilizing appropriate integrated development environments (IDEs) can significantly ease the debugging process. Popular IDEs like Visual Studio, Code::Blocks, and CLion offer built-in error highlighting and debugging tools that simplify finding and fixing mistakes.
Moreover, understanding compiler flags can enhance the error-checking capabilities. For instance, using flags like `-Wall` will enable the compiler to show all warnings, while `-Werror` promotes warnings to errors.
Debugging Tools
Built-in debuggers are a part of most IDEs. They allow you to set breakpoints, step through code execution, and watch variable values. GDB is a powerful debugger for managing C++ applications from the command line.
To start debugging with GDB, you can use the following command:
gdb ./your_program
This will open GDB and allow you to run your program, inspect variables, and step through code.
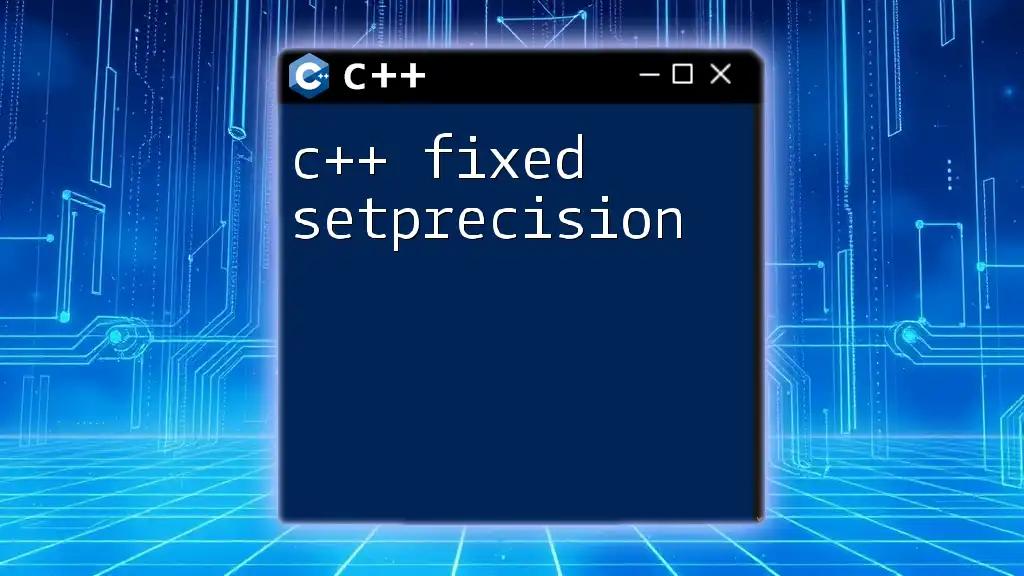
Writing Better Code to Minimize Errors
Code Organization
Organizing your code into smaller, reusable functions can help you locate and fix errors efficiently. Modularity not only facilitates easier debugging but also enhances code readability. Keeping your functions focused on a single task ensures that if something goes wrong, you know where to look.
Example of Modular Code:
void printResult(int result) {
cout << "The result is: " << result << endl;
}
int main() {
int a = 5, b = 10;
int result = a + b;
printResult(result);
}
Commenting and Documentation
Well-commented code is easier to understand for both the original programmer and others who may read it later. Adding comments can clarify your logic and help in tracking what your code is intended to accomplish.
Document any changes made during the debugging process, as this provides context for why certain fixes were necessary. This practice not only aids you but also serves others who may inherit the codebase.
Code Reviews
Peer reviews are invaluable for catching oversights. Engaging other developers to review your code before it moves to production can catch mistakes that you might not have noticed. Different perspectives help in ensuring code quality, functionality, and adherence to coding standards.
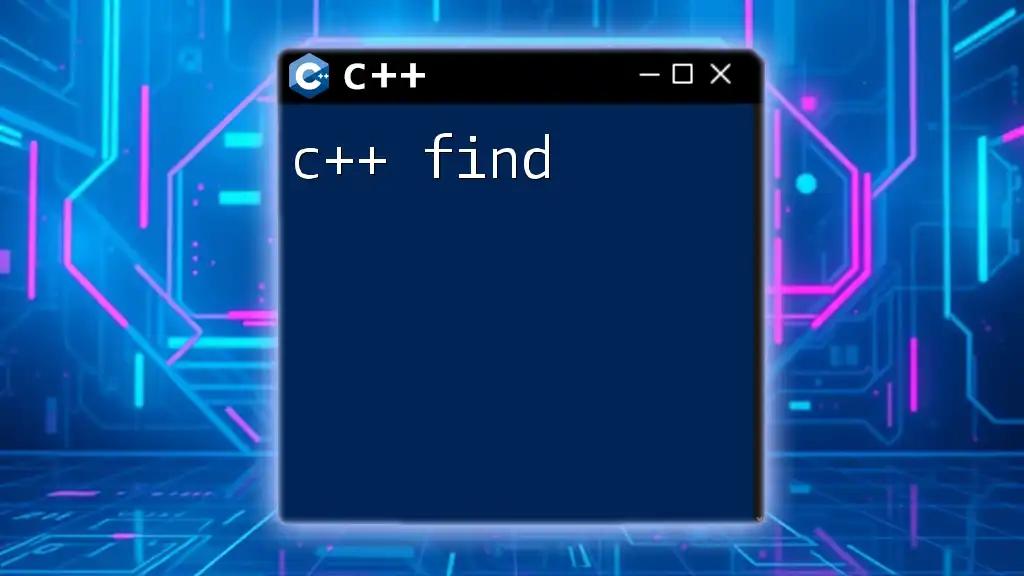
Best Practices for C++ Fix
Consistent Coding Standards
Adhering to established coding standards, such as the Google C++ Style Guide, fosters consistency. Consistent code is generally easier to read and maintain, reducing the likelihood of making errors in the first place.
Testing Your Code
Testing is critical to finding and fixing bugs. Implementing unit tests allows you to check that individual pieces of code function as expected. For example, you can create a simple unit test as follows:
void testFunction() {
assert(someFunction(5) == expectedValue);
}
Engaging in thorough testing prevents many bugs from making it to production.
Learning from Mistakes
After fixing errors, engage in a post-mortem analysis. Reflect on what went wrong, how it was identified, and discuss ways to prevent similar issues in the future. This practice promotes a culture of learning and continuous improvement among programmers.
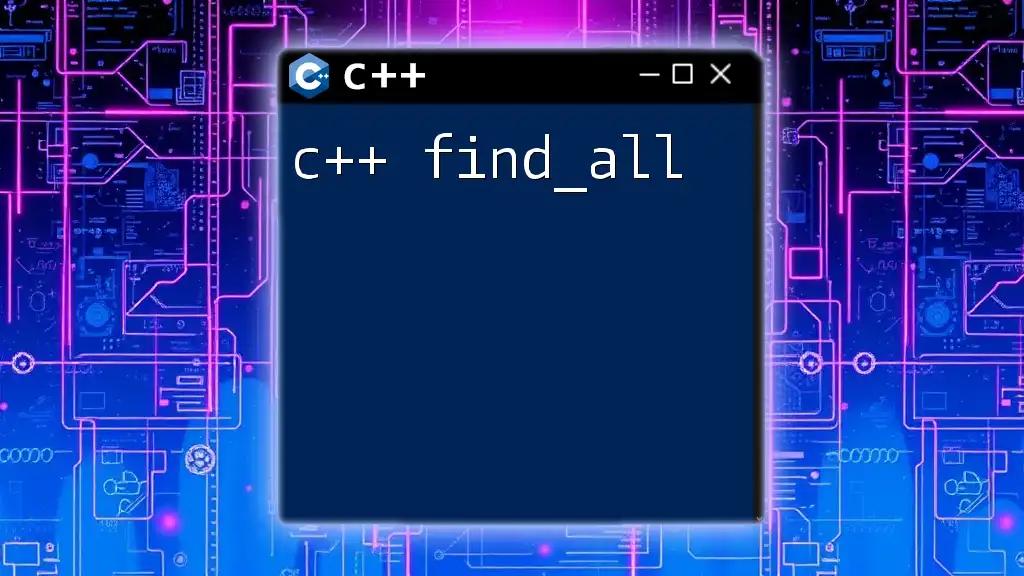
Conclusion
Efficiently addressing issues in C++ programming involves understanding the types of errors you may encounter, utilizing robust tools, and adopting best practices when coding. By focusing on organized code, diligent commenting, and peer reviews, you create a pathway to minimize errors. Each mistake becomes a stepping stone to improved programming competency.
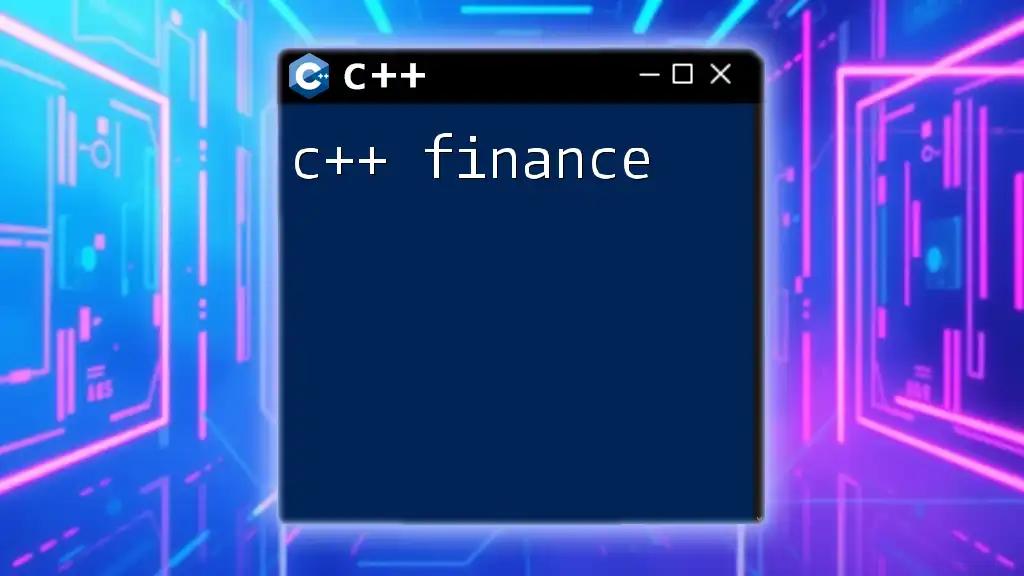
Additional Resources
To enhance your understanding further, explore tutorials, online courses, and official C++ documentation. Engaging with community forums can also provide real-world insights into specific problems and solutions encountered by other developers.