C++ File I/O allows you to read from and write to files using the standard library's `ifstream` and `ofstream` classes for input and output operations, respectively.
Here's a simple code snippet demonstrating both reading from and writing to a file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
if (outFile.is_open()) {
outFile << "Hello, World!" << std::endl;
outFile.close();
}
// Reading from the same file
std::ifstream inFile("example.txt");
std::string line;
if (inFile.is_open()) {
while (getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
}
return 0;
}
Understanding File Streams
What Are File Streams?
In C++, file streams are essential abstractions that allow programs to read from and write to files. They provide a way to perform file I/O operations using a consistent interface, similar to how you handle standard input and output.
Types of File Streams
C++ provides three main types of file streams:
- ifstream: This is the input file stream, used specifically for reading data from files.
- ofstream: This is the output file stream, dedicated to writing data to files.
- fstream: This stream can handle both input and output, allowing reading from and writing to the same file.

Opening and Closing Files
How to Open a File in C++
To work with files in C++, you first need to open them. You can use the `open()` member function of file stream objects or specify the filename directly when creating the file stream object.
#include <fstream>
// Opening a file using ifstream
std::ifstream inputFile("example.txt");
// Opening a file using ofstream
std::ofstream outputFile("output.txt");
Handling Different File Modes
When opening a file, you can specify the mode in which the file should be opened. Here are some commonly used modes:
- ios::in: Opens a file for reading.
- ios::out: Opens a file for writing. If the file already exists, it is truncated.
- ios::app: Opens a file for writing at the end of the file, preserving existing data.
- ios::binary: Opens a file in binary mode, which is crucial for non-text files.
Here’s how to specify a mode when opening a file:
std::ifstream file("data.txt", std::ios::in);
std::ofstream file("data.txt", std::ios::out | std::ios::app);
Closing a File
Closing a file is equally important to prevent memory leaks and data corruption. Use the `close()` method to free the resources associated with the file:
inputFile.close();
outputFile.close();

Reading from Files
Using ifstream to Read Data
The `ifstream` stream provides several methods to read data from files. A common way to read data line by line is by using the `getline()` function.
Here’s a simple example of how to read from a file:
#include <fstream>
#include <iostream>
int main() {
std::ifstream inputFile("example.txt");
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
inputFile.close();
return 0;
}
This code reads each line from the "example.txt" file and outputs it to the console until the end of the file is reached.
Techniques for Reading Data
You can also read data character by character using the `get()` function, or formatted data using the stream extraction operator (`>>`). Error handling, such as checking for end-of-file or stream failures, is important for robustness.
char ch;
while (inputFile.get(ch)) {
std::cout << ch;
}

Writing to Files
Using ofstream to Write Data
Writing to files with `ofstream` is straightforward. You can simply use the `<<` operator to write data into a file.
Example code for writing data to a file:
#include <fstream>
#include <iostream>
int main() {
std::ofstream outputFile("output.txt");
outputFile << "Hello, File I/O in C++!";
outputFile.close();
return 0;
}
This code will create (or overwrite) "output.txt" and write the specified string to it.
Techniques for Writing Data
You can write different types of data using the same syntax. You can also format the output using manipulators provided by the C++ standard library. Ensuring data integrity involves checking stream states to confirm successful writes.
outputFile << std::fixed << std::setprecision(2) << 123.456; // Writing a float with precision

Random Access and Binary Files
What Are Binary Files?
Binary files differ from text files in that they store data in a format that can be directly read by the machine, making them more efficient for certain applications. Common uses for binary files include multimedia files, databases, and custom data formats.
Random Access with fstream
With the `fstream` type, you can perform random access reads and writes, allowing you to seek to specific positions in a file and operate on that data.
Here’s an example demonstrating random access:
#include <fstream>
#include <iostream>
int main() {
std::fstream file("data.bin", std::ios::in | std::ios::out | std::ios::binary);
file.seekp(0); // Move to the beginning
int data = 100;
file.write(reinterpret_cast<const char*>(&data), sizeof(data));
file.seekg(0); // Move back to the beginning to read
int readData;
file.read(reinterpret_cast<char*>(&readData), sizeof(readData));
std::cout << "Read Data: " << readData << std::endl;
file.close();
return 0;
}
Here, the file is opened in binary mode, and data is written and read back, demonstrating the concept of random access.

Error Handling During File I/O
Common File I/O Errors
File I/O operations may encounter several issues, including:
- File not found: Occurs if the specified file does not exist at the given path.
- Permission denied: Happens when the program lacks permission to access the file.
- Reading/Writing issues: This may arise if you attempt to read from or write to an improperly opened file.
How to Handle Errors Gracefully
It’s crucial to implement error handling to ensure that your program can respond to unexpected situations:
if (!inputFile) {
std::cerr << "Error opening file!" << std::endl;
}
Using methods like `fail()`, `eof()`, and `bad()` can help determine the state of the stream and gracefully handle errors.
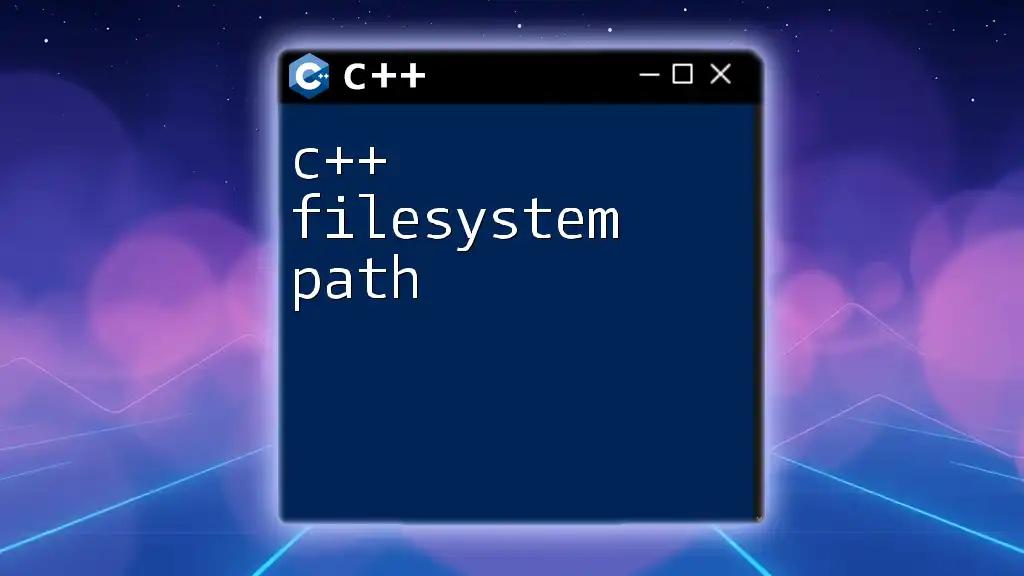
Conclusion
Mastering C++ file I/O is vital for developing programs that interact with data beyond memory. From understanding file streams and modes to implementing reading, writing, and error handling techniques, this comprehensive guide provides a solid foundation for using file operations in C++. Practice with the given examples to gain confidence in your file handling skills, and continue exploring various use cases in real-world applications.
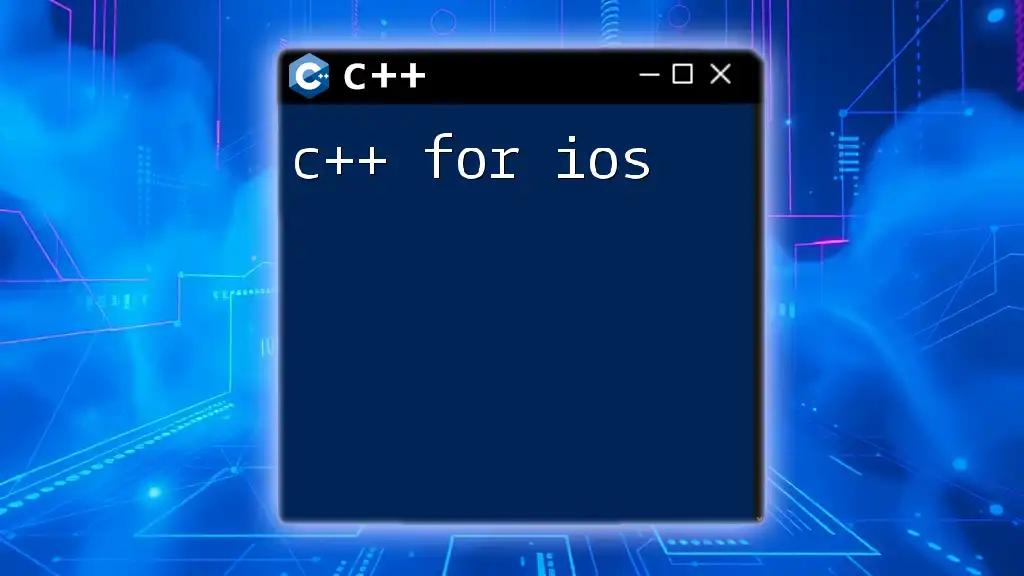
Additional Resources
For those eager to deepen their understanding of C++ file I/O, many resources are available:
- Books: Look for titles focused on C++ programming and file handling.
- Online courses: Enroll in specialized C++ courses that include file I/O modules.
- Community Forums: Engage with platforms dedicated to C++ development for peer support and knowledge sharing.
- Code Repositories: Explore GitHub and other sites for practical examples and projects.

Call to Action
For more tutorials on C++ and to further your learning in file I/O, subscribe to our newsletter. Share your experiences and challenges with file handling in C++, and let’s grow together in this journey of programming!