The C++ Rule of Three states that if a class manages dynamic resources, you should explicitly define a destructor, copy constructor, and copy assignment operator to ensure proper resource management.
Here’s a simple implementation demonstrating the Rule of Three:
#include <cstring>
#include <iostream>
class String {
private:
char* data;
public:
// Constructor
String(const char* value) {
data = new char[strlen(value) + 1];
strcpy(data, value);
}
// Destructor
~String() {
delete[] data;
}
// Copy Constructor
String(const String& other) {
data = new char[strlen(other.data) + 1];
strcpy(data, other.data);
}
// Copy Assignment Operator
String& operator=(const String& other) {
if (this != &other) {
delete[] data;
data = new char[strlen(other.data) + 1];
strcpy(data, other.data);
}
return *this;
}
// Display method
void display() const {
std::cout << data << std::endl;
}
};
Understanding the C++ Rule of Three
The Three Special Member Functions
Destructor
The destructor in C++ is a special member function that is invoked when an object goes out of scope or is explicitly deleted. Its primary role is to release resources—such as memory, file handles, and network connections—that were allocated during the lifetime of an object. Properly defining a destructor prevents resource leaks and ensures that the memory occupied by an object is correctly reclaimed.
Here’s a simple example demonstrating a destructor:
class Resource {
public:
Resource(const char* name) {
resourceName = new char[strlen(name) + 1];
strcpy(resourceName, name);
}
~Resource() {
delete[] resourceName; // Cleanup allocated memory
}
private:
char* resourceName;
};
In this code, when an object of the `Resource` class is destroyed, the destructor is automatically called, freeing the allocated memory for `resourceName`.
Copy Constructor
The copy constructor creates a new object as a copy of an existing object. It ensures that a deep copy is made, meaning that dynamically allocated resources are duplicated rather than merely copied by reference. This step is crucial when an object manages its own memory.
A simple copy constructor is shown below:
Resource(const Resource& other) {
resourceName = new char[strlen(other.resourceName) + 1];
strcpy(resourceName, other.resourceName);
}
In this example, the copy constructor allocates new memory for `resourceName` and copies the content of `other.resourceName`. This guarantees that two distinct objects can exist simultaneously without interfering with each other.
Copy Assignment Operator
The copy assignment operator is used to assign the contents of one object to another existing object. It's important to implement this operator to prevent memory leaks and ensure proper resource management during assignments.
Here’s how to implement a copy assignment operator:
Resource& operator=(const Resource& other) {
if (this != &other) { // Self-assignment check
delete[] resourceName; // Release existing resources
resourceName = new char[strlen(other.resourceName) + 1];
strcpy(resourceName, other.resourceName);
}
return *this;
}
In this code, the copy assignment operator first checks for self-assignment, then releases any previously allocated memory to prevent leaks before duplicating the resource from `other`.
When to Implement the Rule of Three
Identifying Resource Management Needs
The Rule of Three comes into play mainly when you have a class that handles dynamic resources or complex types like pointers, dynamic arrays, or file streams. If your class does any of the following, you should consider implementing all three of the special member functions:
- Allocating memory dynamically.
- Managing resources owned by the class.
- Managing resources that require specific cleanup procedures.
Complex Types and Resource Ownership
In C++, ownership of resources is a critical concept. If a class does not properly manage its resources, it can lead to severe issues such as memory leaks, data corruption, or crashes. The Rule of Three ensures that resources are correctly copied and destructed, maintaining the integrity of your program.
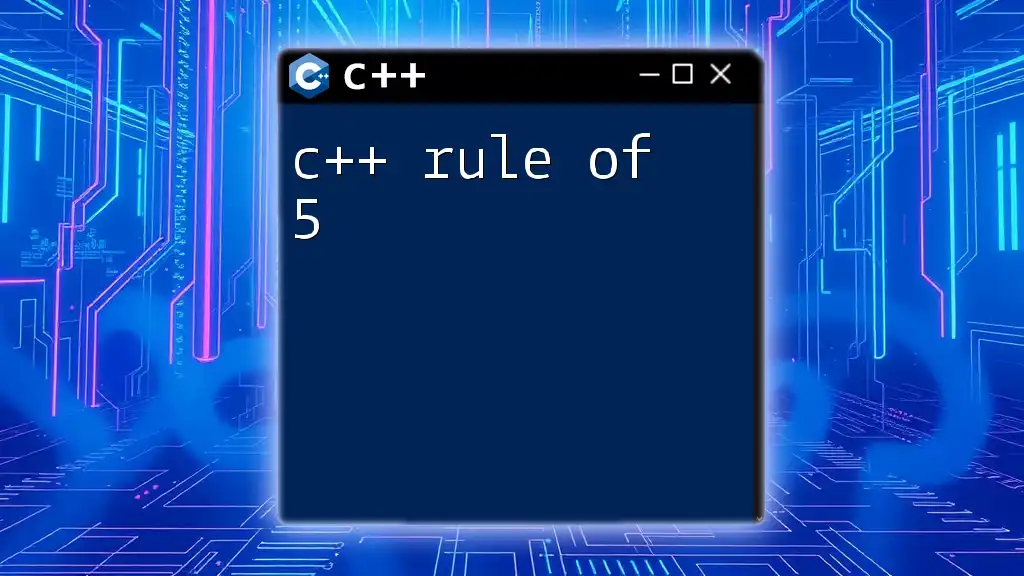
Detailed Examples to Illustrate the Rule of Three
Example Class Implementation
To see the full implementation of a resource-managed class, here's how you could combine the destructor, copy constructor, and copy assignment operator into one example:
class Resource {
public:
Resource(const char* name) {
resourceName = new char[strlen(name) + 1];
strcpy(resourceName, name);
}
~Resource() {
delete[] resourceName; // Cleanup allocated memory
}
Resource(const Resource& other) {
resourceName = new char[strlen(other.resourceName) + 1];
strcpy(resourceName, other.resourceName);
}
Resource& operator=(const Resource& other) {
if (this != &other) { // Self-assignment check
delete[] resourceName; // Release existing resource
resourceName = new char[strlen(other.resourceName) + 1];
strcpy(resourceName, other.resourceName);
}
return *this;
}
private:
char* resourceName;
};
Testing the Rule of Three
Let’s see how to use the `Resource` class effectively in a sample program:
int main() {
Resource res1("Resource 1");
Resource res2 = res1; // Invokes copy constructor
Resource res3("Resource 2");
res3 = res1; // Invokes copy assignment operator
}
In this example, `res2` is created using the copy constructor, ensuring that it has its own copy of `resourceName`. Meanwhile, `res3` takes on the values of `res1` via the copy assignment operator. Both instances reliably retain their resources, demonstrating the effectiveness of the Rule of Three.
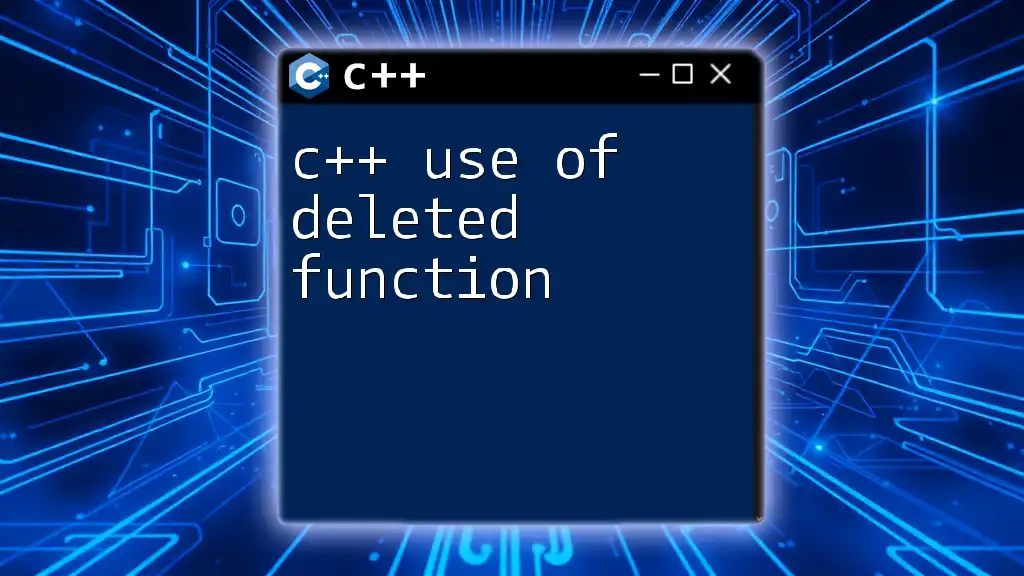
Common Pitfalls in the Rule of Three
Forgetting to Implement One of the Three Functions
One common mistake developers make is neglecting to implement one of the three special member functions. Failing to do so can lead to issues such as memory leaks or undefined behavior. For instance, if the destructor is omitted in a class that allocates memory, that memory will never be released, causing a memory leak with every object created.
Misuse of the Copy Assignment Operator
The distinction between deep and shallow copies is often misconstrued. A shallow copy merely copies the address of dynamically allocated memory rather than duplicating it. This can cause multiple objects to point to the same memory, leading to double deletions or corrupting data.
To safeguard against these pitfalls, ensure that you implement all three special member functions together whenever your class manages dynamic resources.
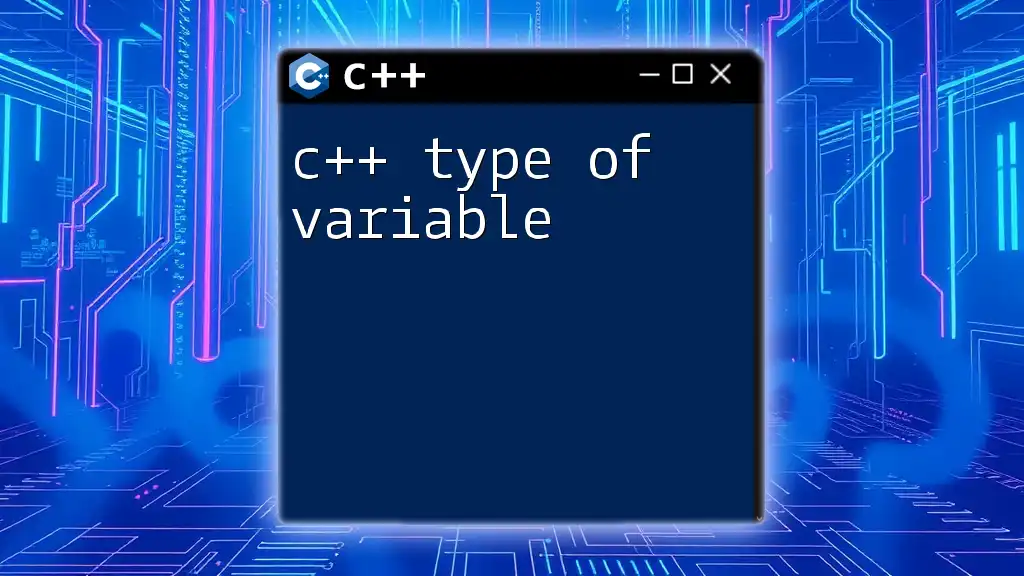
Alternatives to the Rule of Three: Rule of Five
Introduction to Rule of Five
As modern C++ has evolved, the introduction of move semantics has led to the Rule of Five. This rule includes everything in the Rule of Three plus two additional functions: the move constructor and the move assignment operator.
Move Constructor and Move Assignment Operator allow you to transfer ownership of resources without the overhead of copying, preserving the performance efficiency of your applications.
Here’s a brief outline for the additional functions:
Resource(Resource&& other) noexcept {
resourceName = other.resourceName; // Steal the resource
other.resourceName = nullptr; // Leave other in a safe state
}
Resource& operator=(Resource&& other) noexcept {
if (this != &other) {
delete[] resourceName; // Clean up current resource
resourceName = other.resourceName; // Steal resource
other.resourceName = nullptr; // Leave other in a safe state
}
return *this;
}
These move functions enable performance optimizations in C++ by avoiding unnecessary copies of objects.
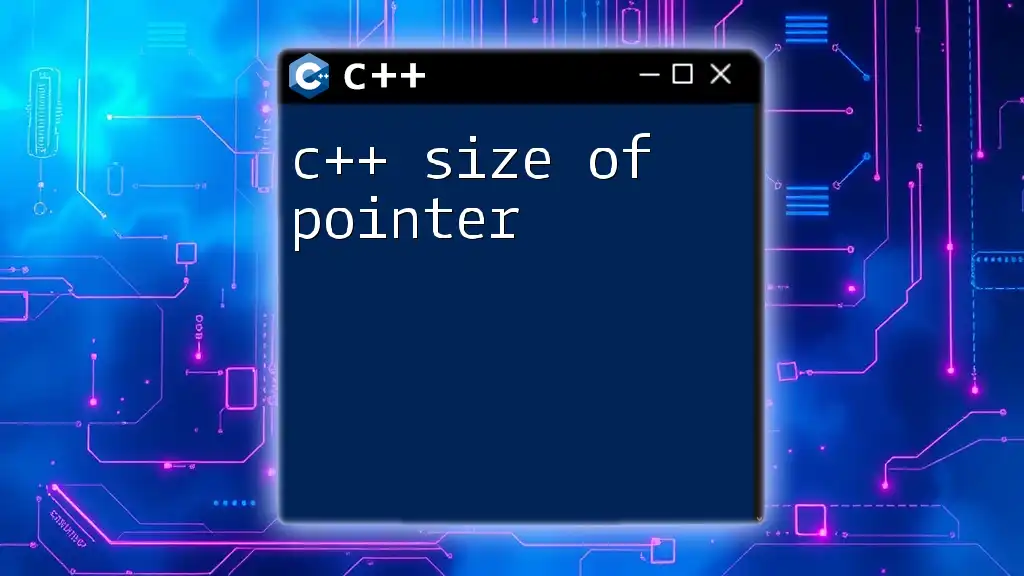
Conclusion
The C++ Rule of 3 emphasizes the importance of proper memory management in classes that handle dynamic resources. By implementing the Destructor, Copy Constructor, and Copy Assignment Operator, developers can prevent memory leaks and ensure robustness in their software applications. Always strive to implement best practices in your C++ code, particularly as you manage resources.
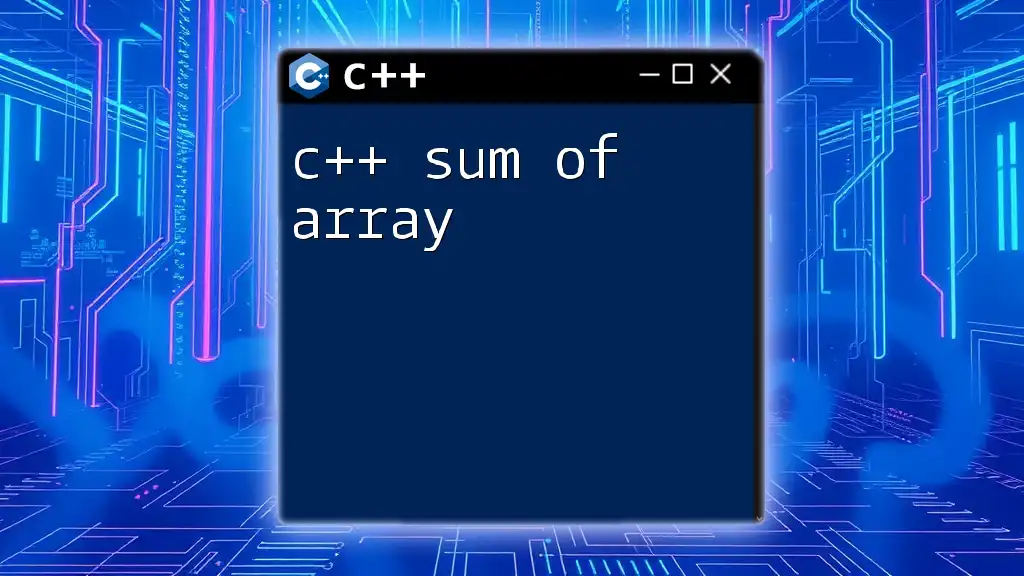
Further Reading and Resources
To deepen your understanding of the C++ Rule of 3, consider exploring reputable books on C++ programming or reputable online resources and tutorials. Engaging with communities and forums dedicated to C++ can also provide invaluable support and insight as you continue your programming journey.