To calculate the sum of an array in C++, you can iterate through the array elements and accumulate their values into a single variable. Here's an example:
#include <iostream>
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < sizeof(arr)/sizeof(arr[0]); i++) {
sum += arr[i];
}
std::cout << "Sum of array: " << sum << std::endl;
return 0;
}
Understanding Arrays in C++
What is C++?
C++ is a powerful programming language that extends the capabilities of the original C language by adding object-oriented features. It is widely used for system/software development, games, and high-performance applications. Mastering C++ opens the doors to a variety of career opportunities and helps you tackle complex programming paradigms.
Understanding Arrays in C++
An array is a collection of elements of the same type stored in a contiguous memory location. The syntax for declaring arrays in C++ is straightforward:
data_type array_name[array_size];
For instance, to declare an integer array with five elements:
int numbers[5];
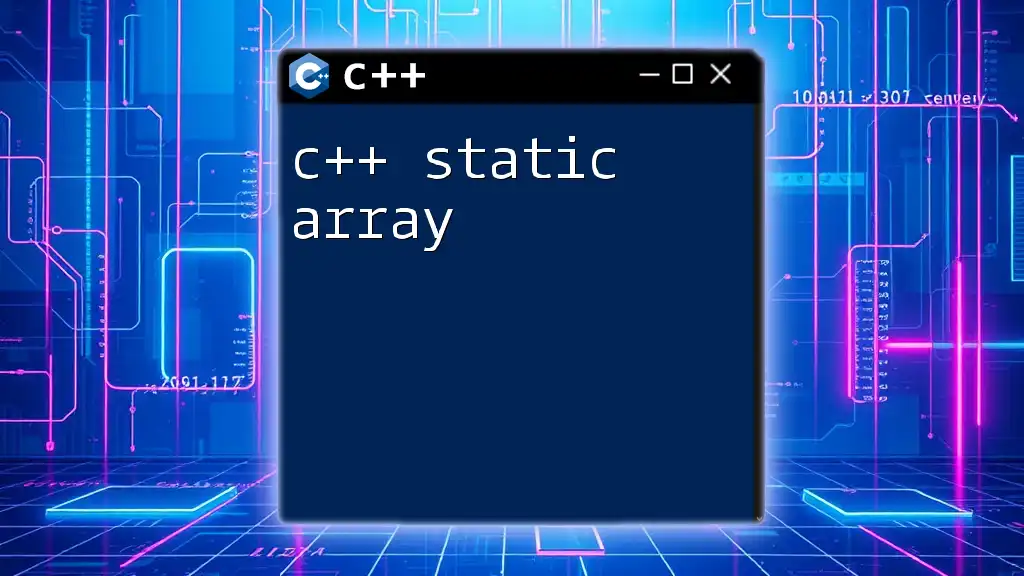
The Concept of Summing an Array
Why Sum Arrays?
The sum of an array serves multiple real-world applications such as statistical analysis, calculating totals, and optimizing algorithms. Summing arrays is often the first step in more complex operations, making it a fundamental skill in programming.
The Mathematical Approach
From a mathematical standpoint, summing an array is simply the process of adding all its elements together. If you have an array A defined as:
\[ A = [a_1, a_2, a_3, \ldots, a_n] \]
The sum can be represented as:
\[ \text{Sum}(A) = a_1 + a_2 + a_3 + \ldots + a_n \]
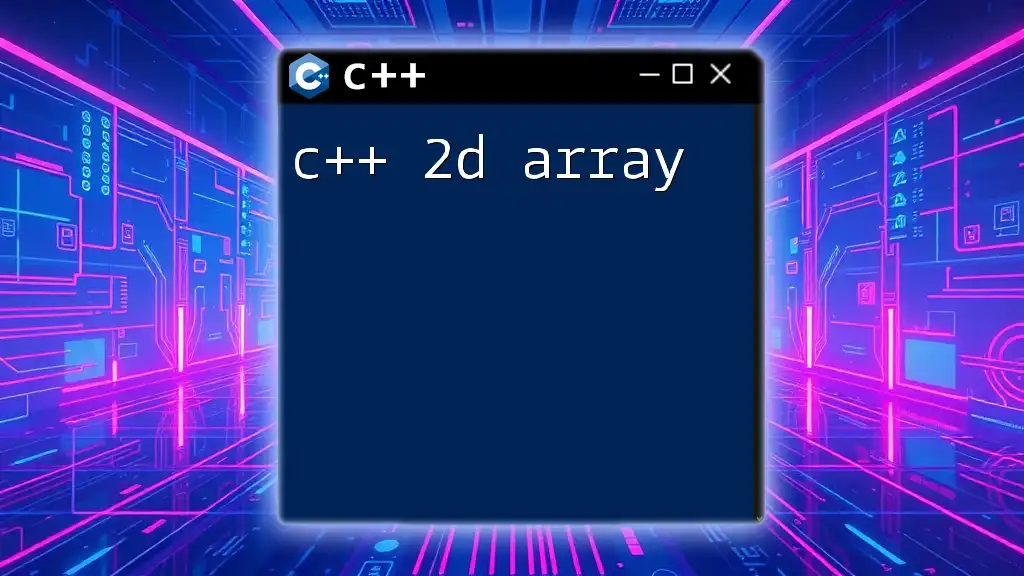
Implementing the Sum of Array in C++
Using a Simple Loop
One of the most straightforward methods to calculate the c++ sum of array elements is to use a loop. Here’s an example demonstrating how to sum an integer array:
#include <iostream>
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = 0;
for (int i = 0; i < 5; i++) {
sum += arr[i];
}
cout << "Sum of array: " << sum << endl;
return 0;
}
In this code snippet, we declare an integer array and initialize `sum` to zero. By iterating through the array using a for loop, we add each element to `sum`, which is then displayed. This method is simple and intuitive, making it suitable for beginners.
Using the `std::accumulate` Function
C++ offers a more elegant solution for array summation through the Standard Library. The `std::accumulate` function simplifies the process:
#include <iostream>
#include <numeric> // for std::accumulate
using namespace std;
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = accumulate(arr, arr + 5, 0);
cout << "Sum of array: " << sum << endl;
return 0;
}
Here, `accumulate` takes three arguments: the beginning of the array, the end of the array, and the initial value for summation (which is zero in this case). This function abstracts away the loop, providing cleaner and more readable code.
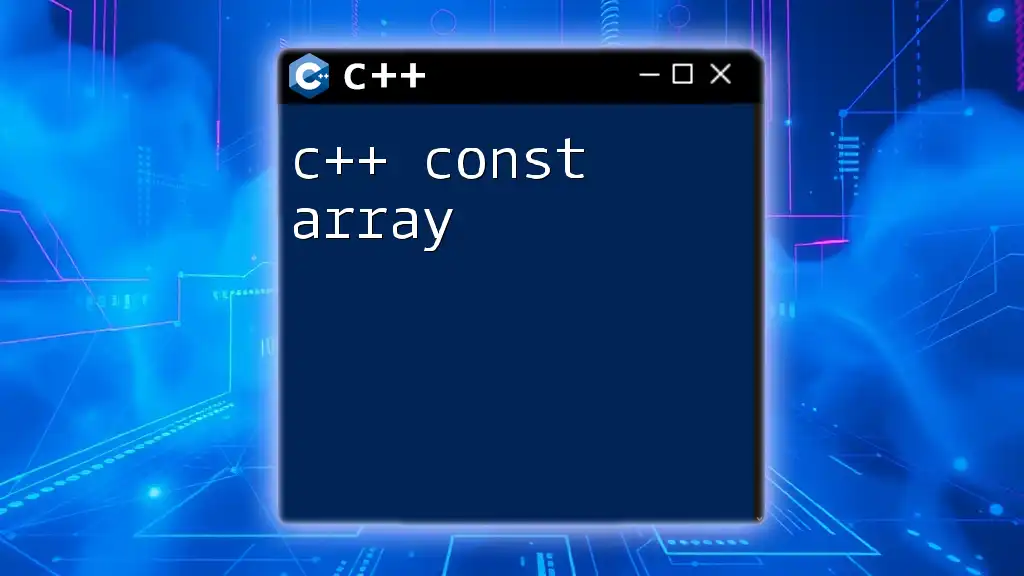
Summing Arrays of Different Data Types
Summing Integer Arrays
The summing method illustrated above can straightforwardly apply to integer arrays. When working with integer data types, the concepts remain the same, allowing you to adapt the same loop or `std::accumulate` method.
Summing Floating-Point Arrays
To sum arrays of floating-point numbers, you can use the same techniques, adjusting the data type in the declaration:
#include <iostream>
#include <numeric>
using namespace std;
int main() {
double arr[] = {1.1, 2.2, 3.3, 4.4, 5.5};
double sum = accumulate(arr, arr + 5, 0.0);
cout << "Sum of floating-point array: " << sum << endl;
return 0;
}
Here, we use `double`, and it’s important to initialize `sum` to `0.0` to match the data type, ensuring precision in the calculation.
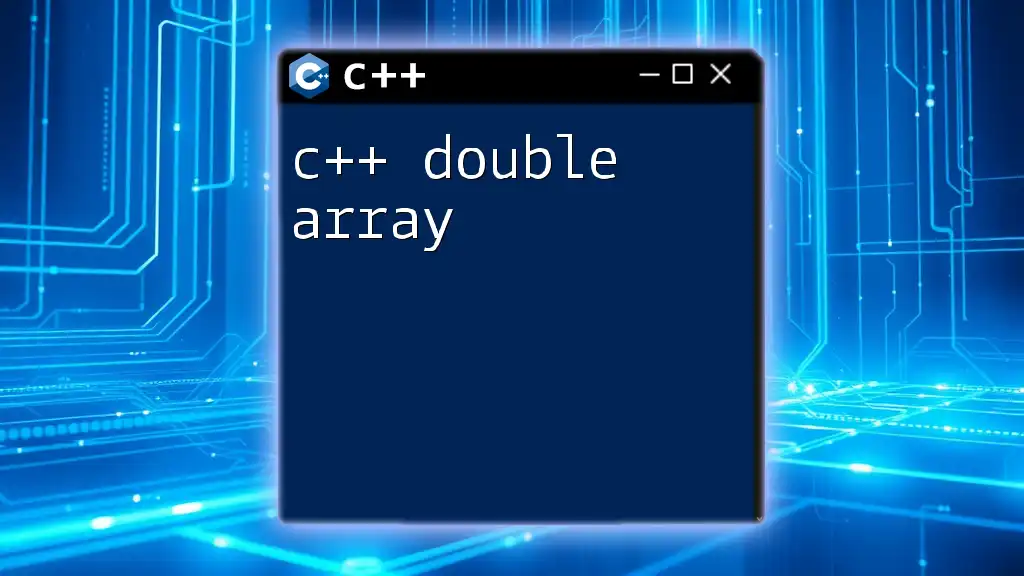
Error Handling and Edge Cases
Dealing with Empty Arrays
Handling edge cases such as empty arrays is important to prevent runtime errors. Checking for an empty array involves verifying the size before performing a sum:
#include <iostream>
using namespace std;
void sumArray(int arr[], int size) {
if (size == 0) {
cout << "Array is empty!" << endl;
return;
}
int sum = 0;
for (int i = 0; i < size; i++) {
sum += arr[i];
}
cout << "Sum of array: " << sum << endl;
}
int main() {
int arr[] = {};
sumArray(arr, sizeof(arr) / sizeof(arr[0]));
return 0;
}
This code checks the size of the array and outputs a message if it’s empty, ensuring a smooth user experience.
Summing Large Arrays
When dealing with large data sets, performance becomes a consideration. Techniques to improve efficiency include minimizing memory allocation and avoiding copy operations. Always choose the appropriate data types to reduce memory overhead.
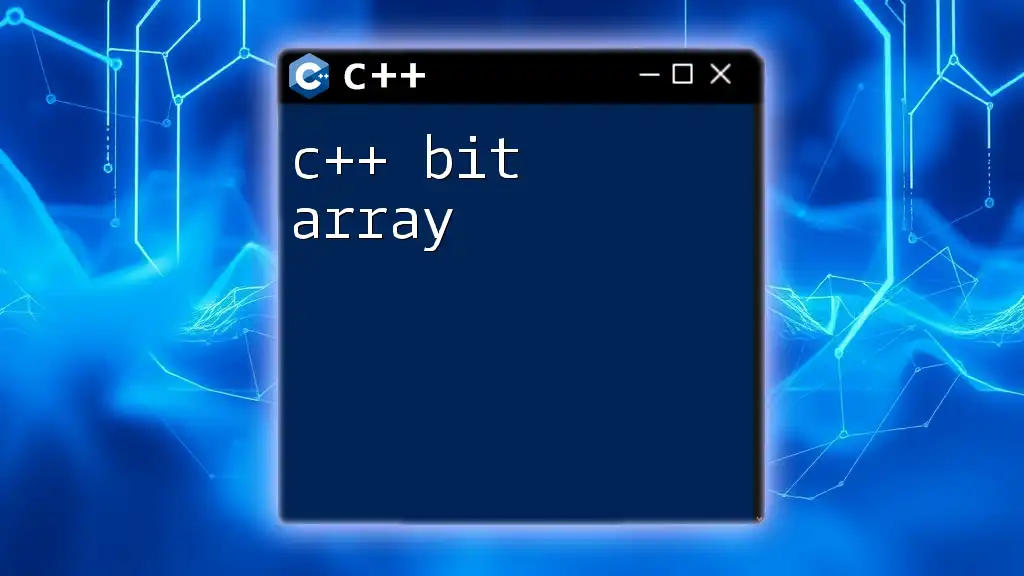
Advanced Summation Techniques
Parallel Summation
For significantly large arrays, you might consider parallelizing the summation process, thus making use of multi-threading capabilities in C++. This allows dividing the array into segments that can be summed concurrently, greatly enhancing performance.
Recursive Approach
As a different approach, you can sum an array using recursion:
int recursiveSum(int arr[], int n) {
if (n <= 0) return 0;
return arr[n-1] + recursiveSum(arr, n-1);
}
int main() {
int arr[] = {1, 2, 3, 4, 5};
int sum = recursiveSum(arr, 5);
cout << "Sum of array using recursion: " << sum << endl;
return 0;
}
In this example, `recursiveSum` calls itself, adding the last element of the current segment to the sum of the remainder. This technique highlights the flexibility of C++, showcasing how both iterative and recursive approaches can achieve the same goal.
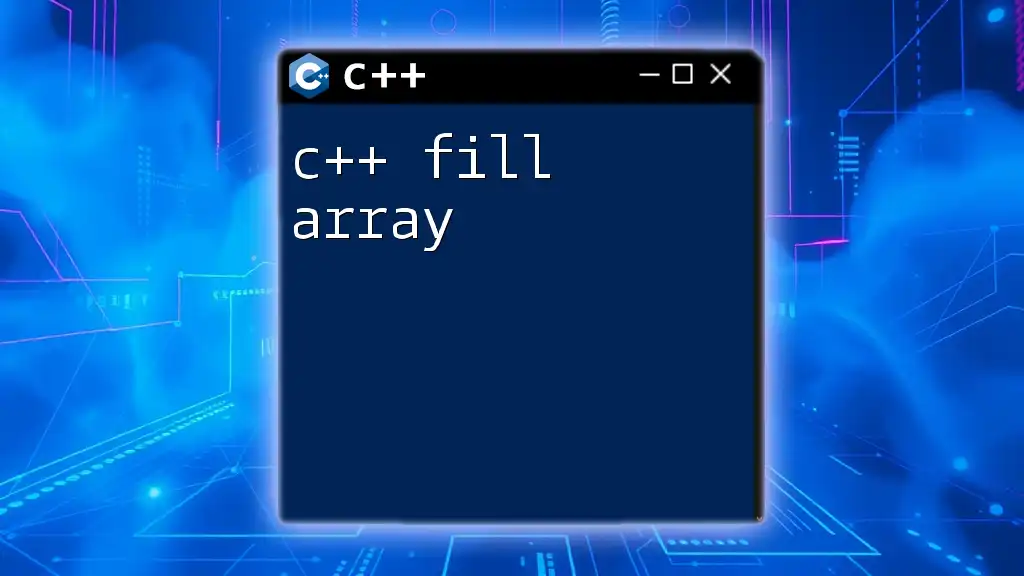
Conclusion
Recap of Key Points
In this guide, we explored the various methods to calculate the c++ sum of array elements. We discussed using loops, the `std::accumulate` function, handling of various data types, error management for empty arrays, and advanced techniques like recursion and parallel summation.
Encouraging Further Learning
C++ is a rich language with vast applications. For those interested in expanding their knowledge further, consider diving into more complex data structures, algorithms, or even exploring design patterns within C++. The skills you build today will be invaluable in your programming journey.
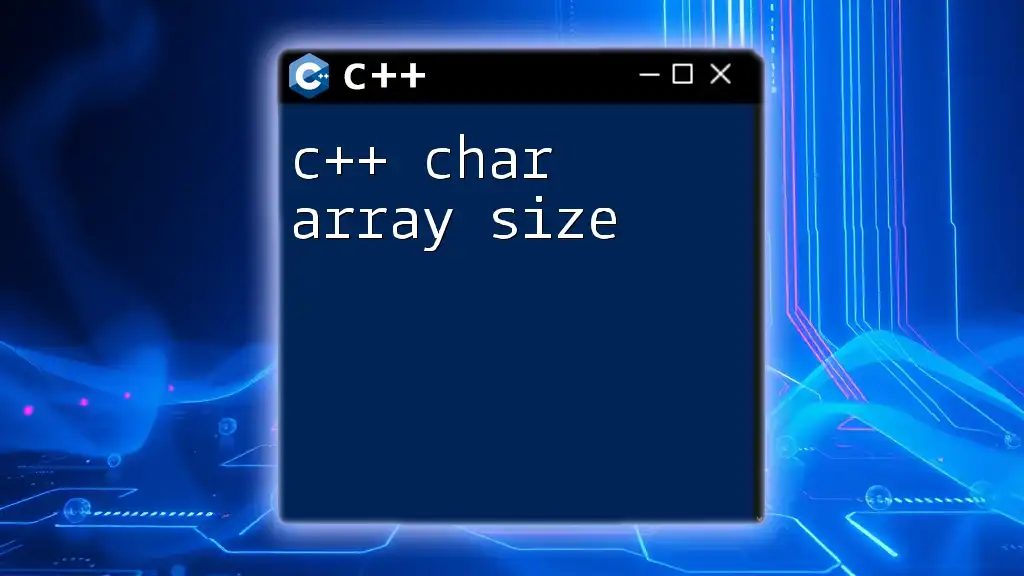
FAQs about Summing Arrays in C++
Common Questions
- Can arrays hold different data types? No, standard C++ arrays are homogeneous, meaning all elements must be of the same data type.
- What is the maximum size of an array in C++? The maximum size of an array is compiler-dependent and often limited by system memory. Consider using dynamic arrays or vectors if you need flexibility.
Additional Resources
For further study, explore books on C++ programming, online tutorials, and courses that cover both foundational and advanced topics relevant to arrays and data manipulation.