In C++, the size of a char array can be determined using the `sizeof` operator, which returns the total number of bytes allocated for the array.
#include <iostream>
int main() {
char myArray[50];
std::cout << "Size of char array: " << sizeof(myArray) << " bytes" << std::endl;
return 0;
}
Understanding Char Arrays
What is a Char Array?
A char array in C++ is a fundamental data structure used to store a sequence of characters. It's an essential component for handling strings within the language. When you declare a char array, you allocate contiguous memory that can hold characters, including the null terminator (`\0`), which indicates the end of the string.
For example:
char example[] = "Hello";
In this case, the `example` array will contain five characters, plus one for the null terminator, totaling six bytes.
Memory Allocation for Char Arrays
Memory allocation for char arrays can occur on the stack or the heap, depending on how the array is declared. Stack allocation is used for local variables, and memory is automatically released when they go out of scope. In contrast, heap allocation requires manual management.
Understanding where your char arrays are allocated is vital as it influences the size determination and potential memory leaks, especially in larger applications.
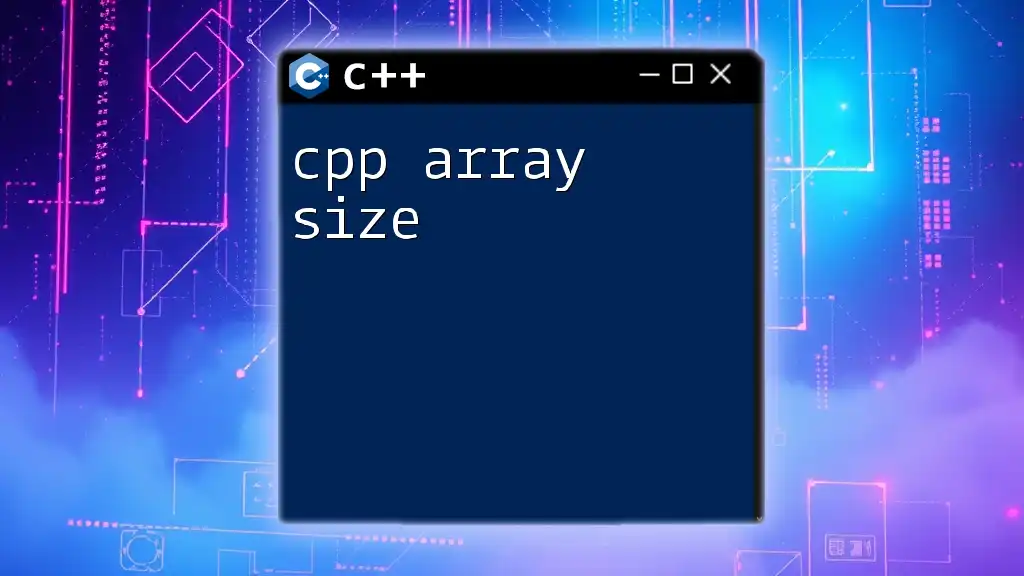
Determining Char Array Size
Using the `sizeof` Operator
The `sizeof` operator is a powerful tool in C++ that can be used to determine the size of a char array at compile time. It calculates the total number of bytes allocated for the array. The syntax is straightforward:
char arr[] = "Hello";
std::cout << "Size of arr: " << sizeof(arr) << std::endl; // Outputs the size
This code will return `6`, accounting for the five characters and the null terminator. However, it's crucial to note that `sizeof` behaves differently when used with pointers. Consider the following example:
char *ptr = "Hello";
std::cout << "Size of ptr: " << sizeof(ptr) << std::endl; // Outputs size of pointer, not string
In this context, `sizeof(ptr)` returns the size of the pointer itself (typically 4 or 8 bytes, depending on the architecture), not the size of the string it points to.
Using `strlen` Function
The `strlen` function serves a different purpose: it calculates the length of a string, excluding the null terminator. To illustrate its use:
char str[] = "Hello";
std::cout << "Length of str: " << strlen(str) << std::endl; // Outputs length excluding null terminator
This will output `5`, which is the length of the string. It's essential to remember that `strlen` does not count the null terminator, making it unreliable if you need the total size of the array including the null character.
When comparing `sizeof` and `strlen`, note that while `sizeof` provides the total size including the null terminator for arrays, `strlen` purely gives the count of characters in the string. This distinction is crucial when managing char arrays properly.
Manual Calculation of Array Size
When to Manually Calculate Size
Occasionally, you may need to manually compute the size of a char array, especially when dealing with dynamically allocated arrays or when certain constraints apply.
How to Calculate Size
You can perform manual calculations using pointer arithmetic. Here’s how:
char arr[] = "Hello";
int size = (sizeof(arr) / sizeof(arr[0])); // Size calculation
std::cout << "Manual Size of arr: " << size << std::endl;
This snippet divides the total size of the entire array by the size of a single element (character), yielding the total number of elements in the array. For the given example, this will also produce `6`, factoring in the null terminator.
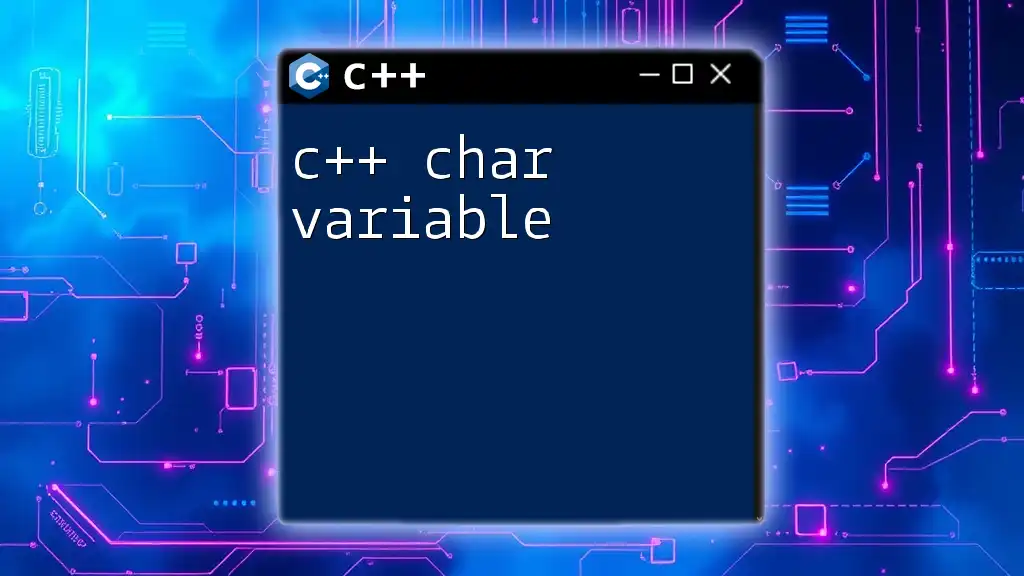
Char Array Size in Different Contexts
Local vs. Global Char Arrays
When defining char arrays, the context significantly impacts their size determination. Local arrays utilize stack memory, which automatically handles memory management but limits size based on stack capacity. Conversely, global char arrays use static storage and remain allocated throughout the program's lifecycle. Understanding these distinctions helps ensure effective memory use.
Char Arrays and Function Parameters
Char arrays can lose their size context when passed to functions. When you pass an array to a function, it decays into a pointer, meaning you lose the information regarding its size. For instance:
void printArray(char arr[])
{
std::cout << "Size of received array: " << sizeof(arr) << std::endl; // Always outputs size of pointer
}
Despite passing a char array, `sizeof(arr)` will return the size of the pointer instead of the actual array size. To work around this limitation, an additional parameter can be passed representing the size.
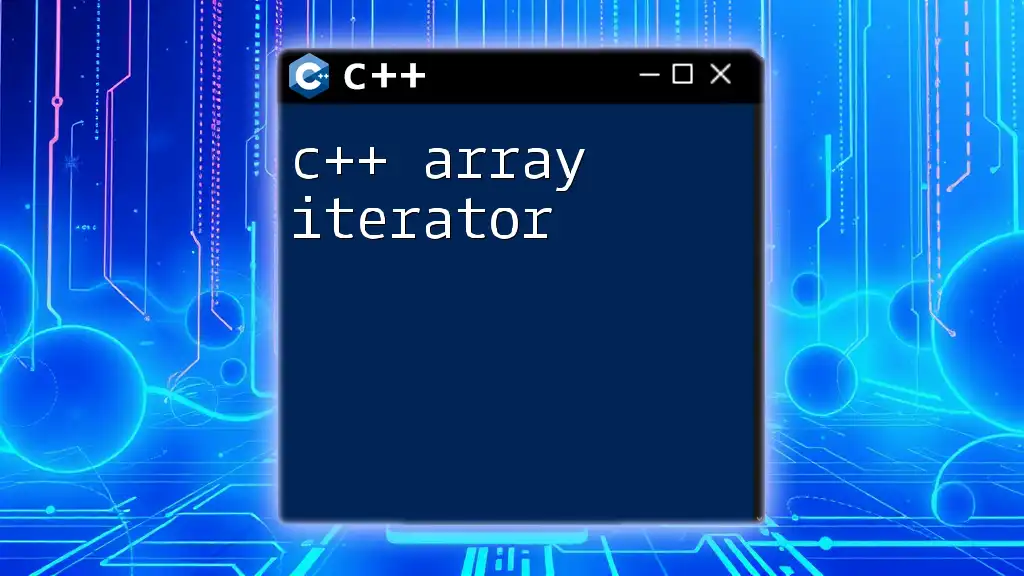
Best Practices for Working with Char Arrays
Initialize Arrays Properly
Proper initialization of char arrays is critical to prevent undefined behavior from uninitialized memory. A poorly initialized char array may have unpredictable sizes or values. For example:
char arr[5]; // No initialization, can lead to undefined behavior
It's best to initialize arrays at the time of declaration or through constructor-style syntax.
Avoiding Buffer Overflows
Buffer overflow is a common pitfall with char arrays, especially when using functions like `strcpy` or `strcat`. You should always ensure your destination array has enough space to accommodate the source and the null terminator. One strategy is to utilize safer alternatives like `strncpy` to prevent overflows:
char source[] = "Hello, World!";
char dest[20];
strncpy(dest, source, sizeof(dest) - 1); // Leave one byte for null terminator
dest[sizeof(dest)-1] = '\0'; // Ensure null termination
This way, you minimize the risk of writing outside the bounds of your allocated memory.
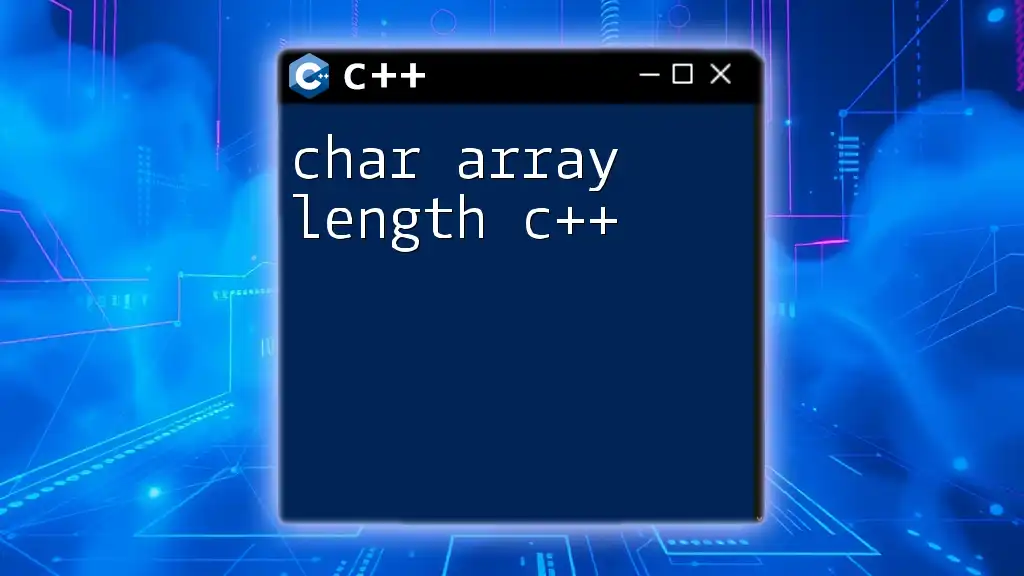
Conclusion
Understanding C++ char array size is essential for effective memory management and programming practices. The `sizeof` operator and `strlen` function serve different purposes, and knowing when to use each effectively can prevent common pitfalls in C++. Confidence in managing char arrays will lead to fewer bugs and more robust applications. Be sure to practice these concepts by implementing them in your coding exercises.
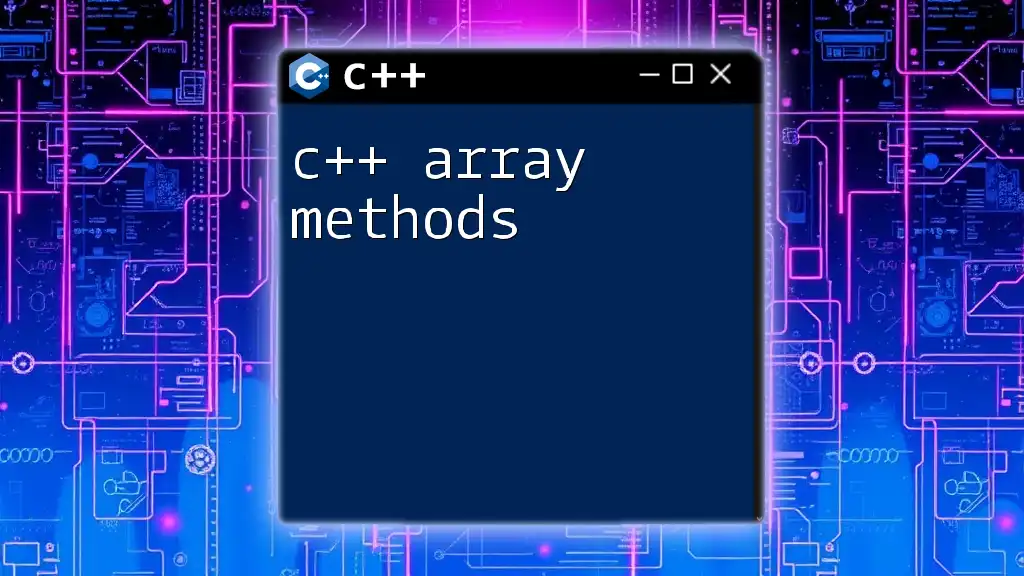
Additional Resources
For further reading, you can refer to the official C++ documentation or explore books focused on advanced C++ programming techniques. These resources will help deepen your knowledge of arrays and memory management, essential topics for proficient C++ developers.
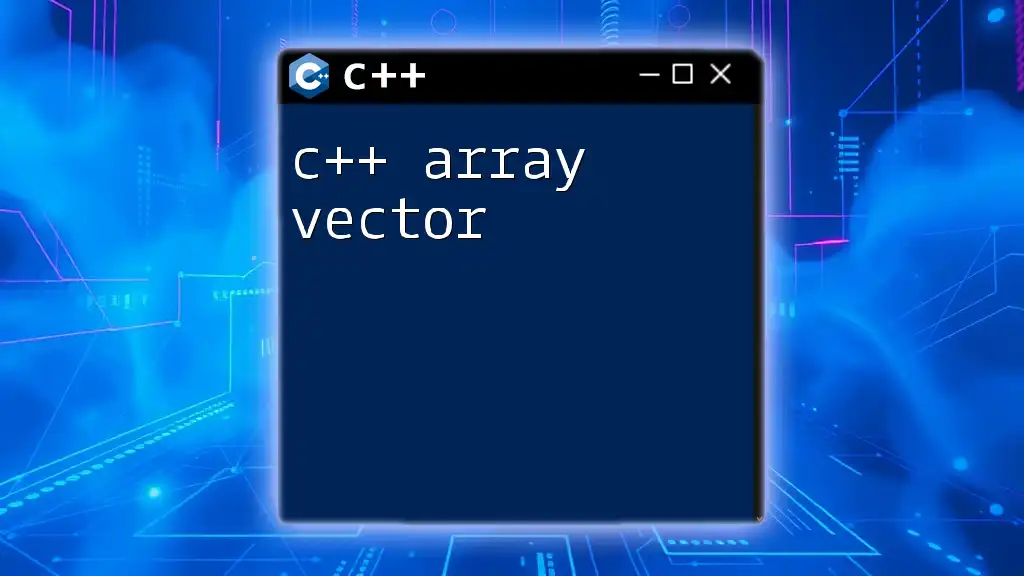
FAQs
What is the difference between `char *` and `char[]`?
`char *` declares a pointer to a character, which may or may not point to an actual string (it could point to a specific location in memory or be uninitialized). In contrast, `char[]` declares an array of characters, allocating memory for the array size determined at compile time.
Can I define a char array without specifying its size?
Yes, you can implicitly define a char array size by providing a string literal, as shown below:
char arr[] = "Hello"; // Size automatically determined as 6
This initializes `arr` with a size sufficient to hold the string plus the null terminator.