A circular array queue in C++ is an efficient data structure that uses a fixed-size array to implement a queue, allowing for the wrapping-around of the end of the array to the beginning, thus utilizing all available space.
Here’s a simple implementation of a circular array queue in C++:
#include <iostream>
using namespace std;
class CircularQueue {
int* arr;
int front, rear, capacity;
public:
CircularQueue(int size) {
arr = new int[size];
capacity = size;
front = rear = 0;
}
~CircularQueue() {
delete[] arr;
}
void enqueue(int value) {
if ((rear + 1) % capacity == front) {
cout << "Queue is Full\n";
return;
}
arr[rear] = value;
rear = (rear + 1) % capacity;
}
void dequeue() {
if (front == rear) {
cout << "Queue is Empty\n";
return;
}
front = (front + 1) % capacity;
}
void display() {
if (front == rear) {
cout << "Queue is Empty\n";
return;
}
cout << "Queue elements: ";
for (int i = front; i != rear; i = (i + 1) % capacity) {
cout << arr[i] << " ";
}
cout << "\n";
}
};
int main() {
CircularQueue cq(5);
cq.enqueue(10);
cq.enqueue(20);
cq.enqueue(30);
cq.display();
cq.dequeue();
cq.display();
return 0;
}
What is a Queue?
A queue is a fundamental data structure that follows the FIFO (First In, First Out) principle. This means that the first element added to the queue will be the first one to be removed. Queues are essential in various scenarios, such as task scheduling, resource sharing, and handling requests in multi-threaded environments.
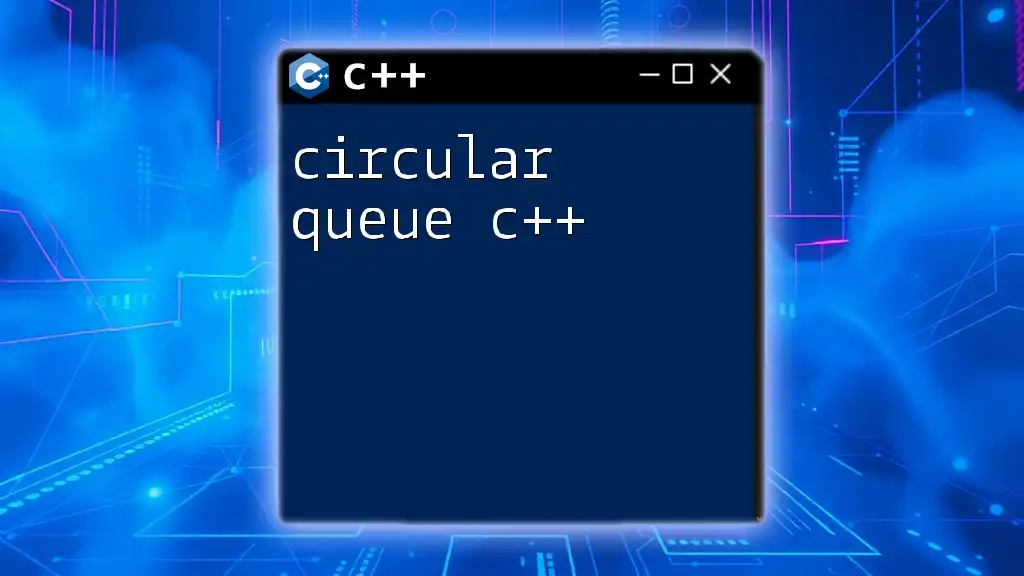
Need for a Circular Array Queue
While linear queues implemented with arrays are simple, they have a significant limitation: once the rear pointer reaches the end of the array, it cannot enqueue any new elements even if there are vacant spaces at the beginning of the array. This results in inefficient space utilization. A circular array queue solves this issue by treating the array as circular, allowing the front and rear to "wrap around" to the beginning when they reach the end of the array.
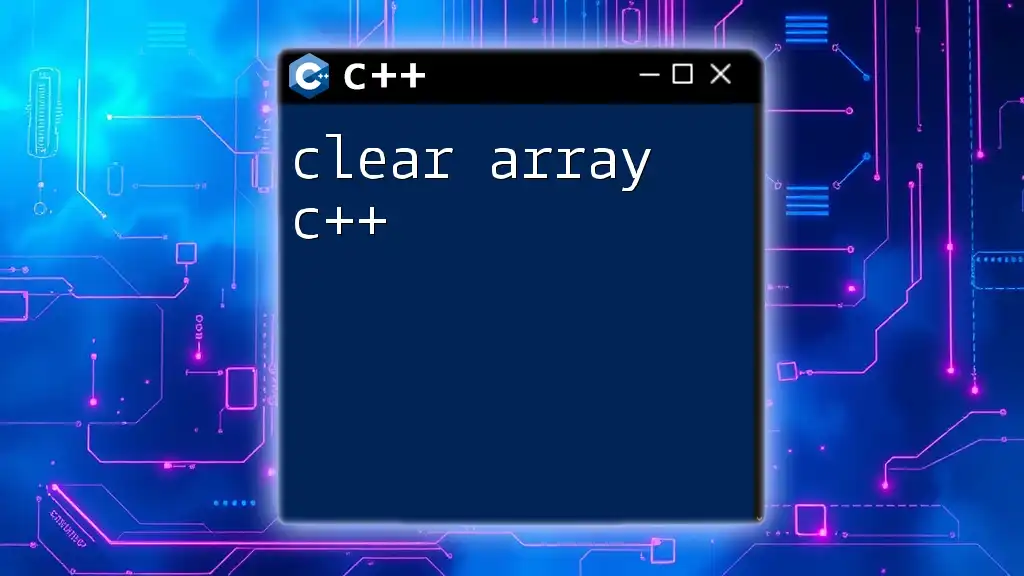
Understanding Circular Array Queues
What is a Circular Array Queue?
A circular array queue is a type of queue that uses a circularly linked array to manage its elements, thus enabling better use of the available space. In this structure, when the rear pointer reaches the end of the array, it can continue from the beginning if there is available space.
Key Concepts
In a circular array queue:
- Elements: Represent the data items being queued.
- Front Pointer: Indicates the position from where the next element will be dequeued.
- Rear Pointer: Indicates the position at which the next element will be enqueued.
These concepts are central to the functioning of circular queues.
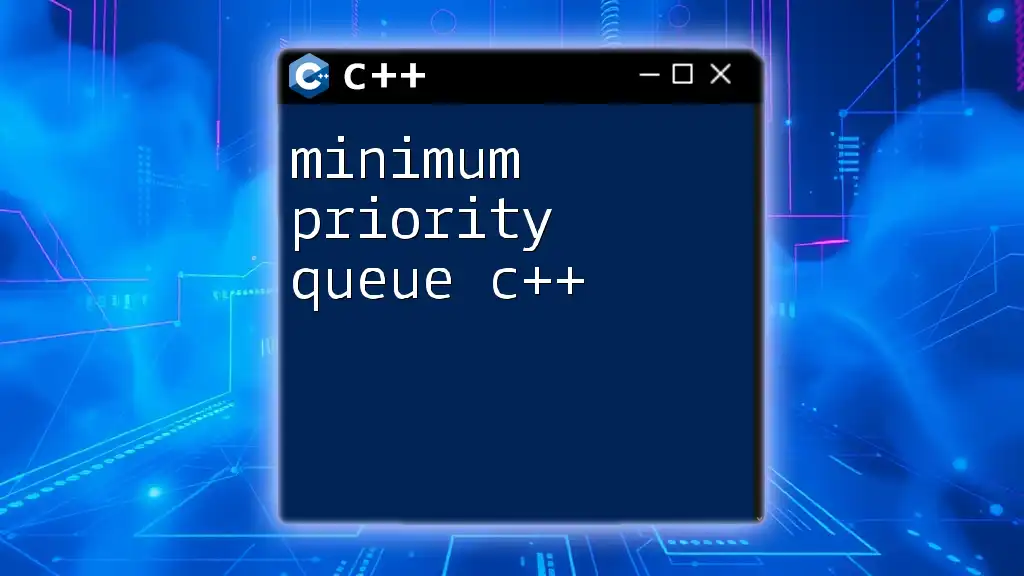
Implementing a Circular Array Queue in C++
To implement a circular array queue in C++, we need to define a class that encapsulates the data structure and its operations.
Basic Structure of the Circular Queue
Here’s a simplified class definition for a circular queue:
class CircularQueue {
private:
int *arr; // Pointer to the array that holds the queue elements
int front; // Index of front element
int rear; // Index of rear element
int capacity; // Maximum number of elements the queue can hold
public:
CircularQueue(int size);
~CircularQueue();
};
Constructor
The constructor initializes the circular queue by allocating memory for the array and setting the front and rear pointers:
CircularQueue::CircularQueue(int size) {
arr = new int[size]; // Allocate an array of given size
capacity = size; // Set the capacity
front = rear = -1; // Initialize front and rear to indicate an empty queue
}
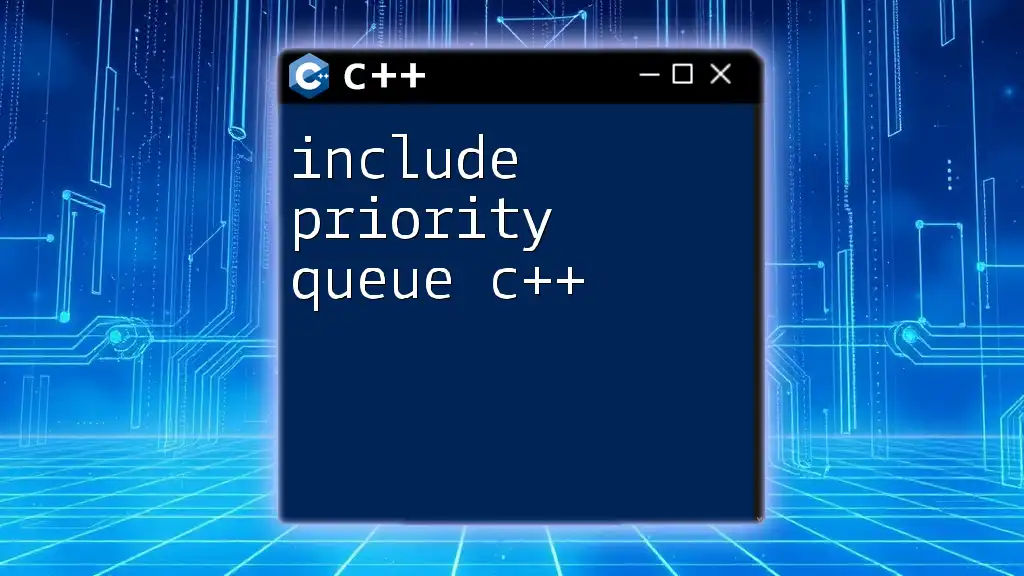
Circular Queue Operations
Enqueue Operation
The enqueue operation adds an element to the rear of the queue. It needs to check for overflow conditions before adding a new element.
void CircularQueue::enqueue(int item) {
if ((rear + 1) % capacity == front) {
// Queue is full
throw std::overflow_error("Queue is full");
}
if (front == -1) { // If the queue is empty
front = 0;
}
rear = (rear + 1) % capacity; // Move rear to the next position (circularly)
arr[rear] = item; // Place the new item at the rear position
}
Dequeue Operation
The dequeue operation removes an element from the front of the queue. If the queue is empty, it should inform the user:
int CircularQueue::dequeue() {
if (front == -1) {
// Queue is empty
throw std::underflow_error("Queue is empty");
}
int item = arr[front]; // Get the item at front
if (front == rear) { // If the queue has only one element
front = rear = -1; // Reset to indicate empty queue
} else {
front = (front + 1) % capacity; // Move front to the next position (circularly)
}
return item; // Return the dequeued item
}
Front and Rear Functions
To peek the elements at the front and rear without removing them, we can implement the following methods:
int CircularQueue::peekFront() {
if (front == -1) throw std::underflow_error("Queue is empty");
return arr[front];
}
int CircularQueue::peekRear() {
if (rear == -1) throw std::underflow_error("Queue is empty");
return arr[rear];
}
Checking Queue Status
Understanding the status of the queue (whether it is empty or full) is essential for proper operation:
bool CircularQueue::isEmpty() const {
return front == -1; // Queue is empty if front is -1
}
bool CircularQueue::isFull() const {
return (rear + 1) % capacity == front; // Queue is full if rear is right next to front
}
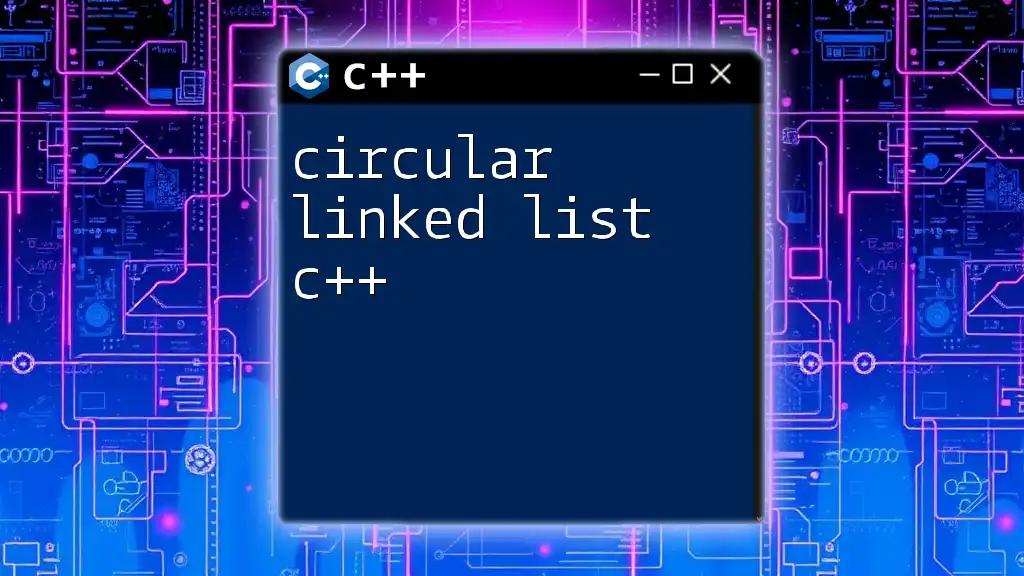
Advantages of Using Circular Array Queues
One of the main advantages of a circular array queue is its optimal space usage. When implemented correctly, it can efficiently utilize available memory without waste. Additionally, it provides constant time complexity (O(1)) for both enqueue and dequeue operations, making it a highly efficient choice for handling data.
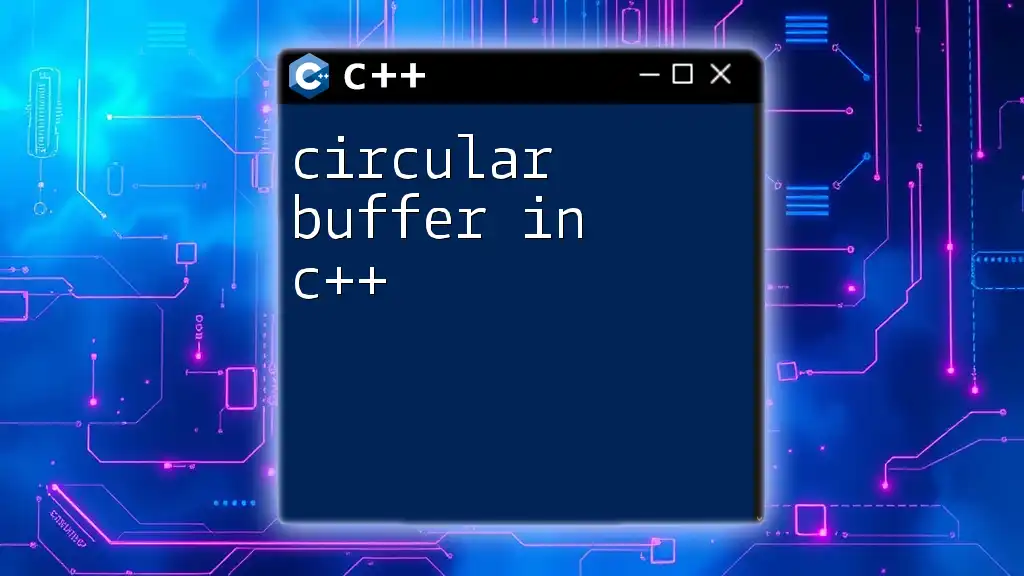
Applications of Circular Queues
Real-World Usage
Circular queues are widely used in several practical scenarios, such as:
- CPU Scheduling: Managing processes in a round-robin manner.
- Resource Sharing: Allocating resources like bandwidth and memory in networking.
Use in Algorithms
Circular queues also play a vital role in various algorithms, notably in breadth-first search (BFS), where it facilitates layer-wise exploration of nodes in a graph.
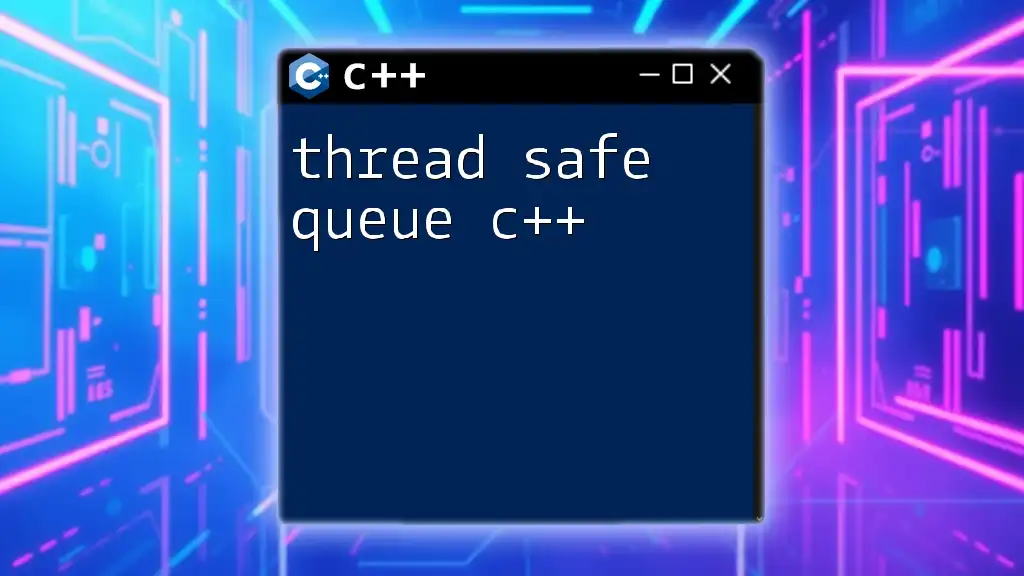
Common Challenges and Troubleshooting
Dealing with Queue Overflow and Underflow
Handling queue overflow and underflow is critical in implementation. The overflow condition occurs when the rear pointer wraps around and meets the front pointer, indicating that the queue is full. Conversely, underflow occurs when a dequeue operation is attempted on an empty queue. Both should be handled gracefully, often by throwing exceptions.
Debugging Tips
Common pitfalls when implementing circular queues include incorrect pointer management and boundary conditions. Thoroughly testing with edge cases can help identify these issues. Utilize debugging tools and print statements to monitor the values of front and rear pointers during enqueue and dequeue operations.
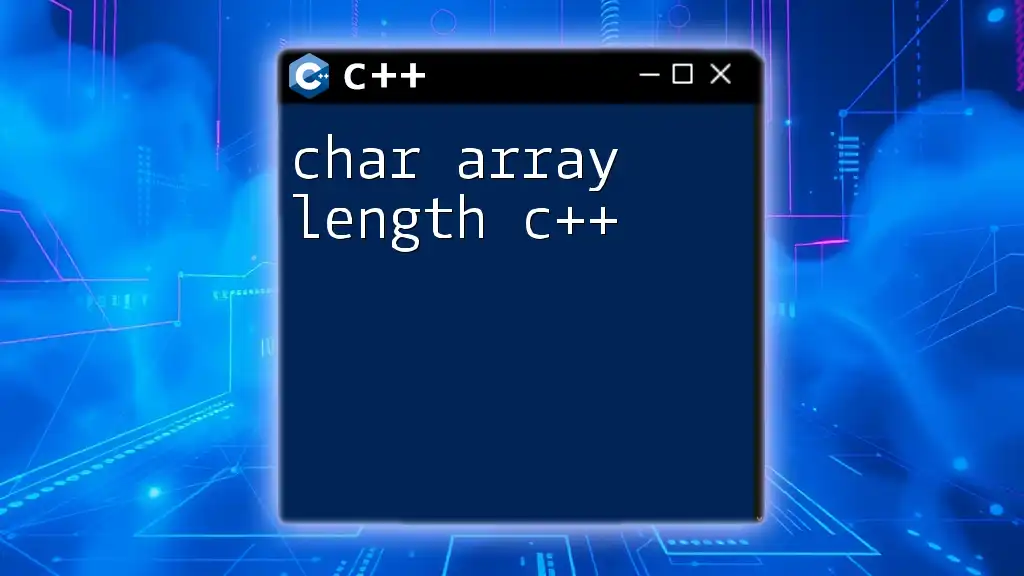
Conclusion
In summary, a circular array queue in C++ is an efficient data structure that overcomes the limitations of traditional queues by maximizing space and ensuring constant time operations. Understanding its implementation, operations, and applications can significantly enhance your ability to manage data efficiently in various programming scenarios. Delving into further resources will help round out your knowledge for practical application and implementation.
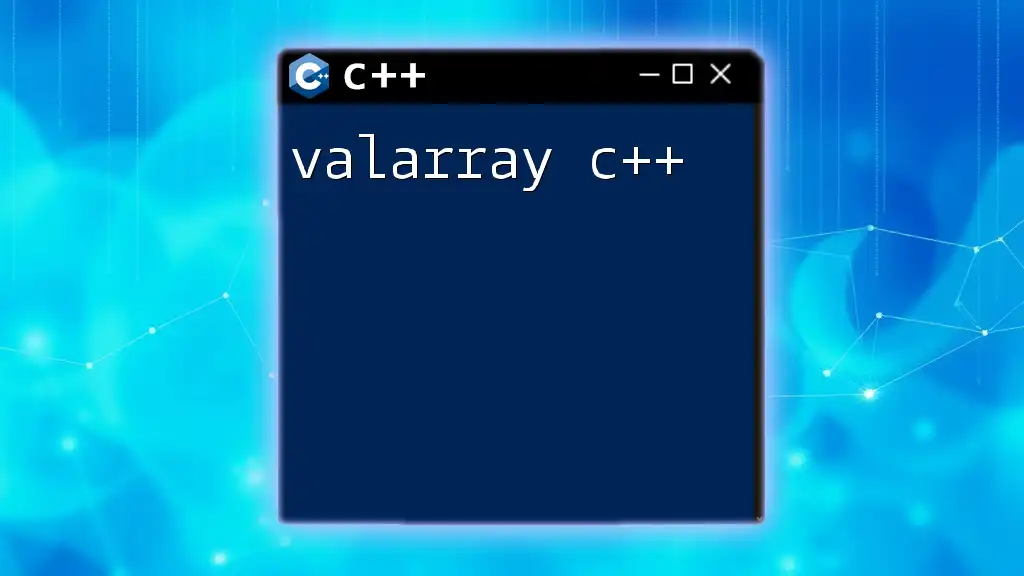
Additional Resources
For further learning, consider exploring:
- Sample Code Repository: A collection of code snippets and full implementations.
- Interactive Examples: Online environments for experimenting with your circular queue implementations.