A circular buffer in C++ is a fixed-size data structure that efficiently manages the overwriting of the oldest data when new data is added, functioning like a queue that wraps around upon reaching its capacity.
Here's a basic implementation of a circular buffer in C++:
#include <iostream>
#include <array>
class CircularBuffer {
public:
CircularBuffer(size_t size) : buffer(size), head(0), tail(0), full(false) {}
void push(int value) {
buffer[head] = value;
if (full) {
tail = (tail + 1) % buffer.size(); // overwrite the oldest
}
head = (head + 1) % buffer.size();
full = head == tail;
}
int pop() {
if (isEmpty()) throw std::out_of_range("Buffer is empty");
int value = buffer[tail];
full = false;
tail = (tail + 1) % buffer.size();
return value;
}
bool isEmpty() const { return !full && (head == tail); }
private:
std::array<int, 5> buffer; // Fixed size 5 for demonstration
size_t head, tail;
bool full;
};
int main() {
CircularBuffer cb(5);
cb.push(1);
cb.push(2);
cb.push(3);
std::cout << cb.pop() << std::endl; // Outputs: 1
cb.push(4);
cb.push(5);
cb.push(6); // Overwrites the oldest (2)
std::cout << cb.pop() << std::endl; // Outputs: 3
return 0;
}
Understanding Circular Buffers
What is a Circular Buffer?
A circular buffer is a data structure that utilizes a fixed-size buffer in a circular (ring) manner. This means that when the buffer reaches its end, the next element insertion occurs at the front, effectively creating a loop. This is distinct from a linear buffer where the beginning and end have clear boundaries.
One of the primary characteristics of a circular buffer is that it maintains a constant size. When it is full, any new data can overwrite the oldest data, which is particularly useful in real-time applications that continuously produce and consume data, such as audio processing or streaming applications.
Advantages of Using Circular Buffers
The benefits of using circular buffers include:
- Memory efficiency: Since the buffer size is fixed, you can prevent dynamic memory allocation issues, resulting in predictable memory usage.
- Performance benefits: Circular buffers often provide better cache locality, which leads to improved performance in time-sensitive applications.
- Use cases in real-world applications: They are particularly useful for buffering streams of data such as video or audio or for managing data between producer and consumer entities in multithreaded applications.

Implementing a Circular Buffer in C++
Structure of a Circular Buffer
A typical circular buffer structure includes the following key components:
- head: The index position where the next element will be inserted.
- tail: The index position from where the next element will be removed.
- capacity: The maximum number of elements that can be stored in the buffer.
- size: The current number of elements in the buffer.
These components work together to manage the data flow efficiently as elements are added and removed.
Basic Operations
Insertion
To insert elements into a circular buffer, you will typically check if the buffer is full. If it is not, the element is added at the `head` index, and `head` is then advanced using modulo arithmetic for the circular wrapping.
Here’s an example of how to implement the insertion operation within a Circular Buffer class:
class CircularBuffer {
private:
int *buffer;
int head;
int tail;
int capacity;
int size;
public:
CircularBuffer(int cap) : capacity(cap), head(0), tail(0), size(0) {
buffer = new int[capacity];
}
void insert(int value) {
if (size == capacity) {
throw std::overflow_error("Buffer is full");
}
buffer[head] = value;
head = (head + 1) % capacity;
size++;
}
};
In this code, if the buffer is at full capacity, it throws an overflow error. Otherwise, it inserts the new value and updates the `head`.
Deletion
For the deletion operation, you retrieve the element at the `tail` index and then increment the `tail`, also using modulo arithmetic to account for the circular structure. If the buffer is empty, you throw an underflow error:
int remove() {
if (size == 0) {
throw std::underflow_error("Buffer is empty");
}
int value = buffer[tail];
tail = (tail + 1) % capacity;
size--;
return value;
}
In this deletion method, the oldest element is removed, and the size of the buffer decreases accordingly.
Peeking
To access the front element without removing it, implement a peek operation. Ensure that the buffer is not empty before accessing the `tail` position:
int peek() {
if (size == 0) {
throw std::underflow_error("Buffer is empty");
}
return buffer[tail];
}
This allows you to check what’s next to be removed without altering the state of the buffer.
Full Implementation of a Circular Buffer Class
To have a fully functional Circular Buffer, it's crucial to manage memory correctly and deallocate it when the buffer is no longer needed. Here's a complete class implementation, including a destructor:
class CircularBuffer {
private:
int *buffer;
int head;
int tail;
int capacity;
int size;
public:
CircularBuffer(int cap) : capacity(cap), head(0), tail(0), size(0) {
buffer = new int[capacity];
}
void insert(int value) {
if (size == capacity) {
throw std::overflow_error("Buffer is full");
}
buffer[head] = value;
head = (head + 1) % capacity;
size++;
}
int remove() {
if (size == 0) {
throw std::underflow_error("Buffer is empty");
}
int value = buffer[tail];
tail = (tail + 1) % capacity;
size--;
return value;
}
int peek() {
if (size == 0) {
throw std::underflow_error("Buffer is empty");
}
return buffer[tail];
}
~CircularBuffer() {
delete[] buffer;
}
};
This complete code provides a clear structure for a circular buffer, including memory management.

Advanced Concepts
Resizing a Circular Buffer
Resizing a circular buffer is sometimes necessary when the fixed capacity does not meet the application’s needs anymore. To implement this, you can create a new larger buffer, copy existing elements over while maintaining their order, and then update the head and tail indices accordingly.
Here’s a simple approach to resizing:
void resize(int new_capacity) {
int *new_buffer = new int[new_capacity];
for (int i = 0; i < size; i++) {
new_buffer[i] = buffer[(tail + i) % capacity];
}
delete[] buffer;
buffer = new_buffer;
capacity = new_capacity;
head = size; // New head will be at the end of new elements
tail = 0; // Reset tail
}
After resizing, the elements are retained in the same order, and `head` and `tail` are updated accordingly.
Thread Safety in Circular Buffers
When using circular buffers in multithreaded environments, you must consider thread safety to avoid race conditions. Utilizing a mutex can help protect your buffer from concurrent modifications:
std::mutex mtx;
void threadSafeInsert(int value) {
std::lock_guard<std::mutex> lock(mtx);
insert(value);
}
This `threadSafeInsert` function ensures that only one thread can modify the buffer at a time, preventing unexpected behavior.

Use Cases of Circular Buffers
Audio Processing
Circular buffers are widely utilized in audio processing systems. For example, when streaming audio, data is continuously written to the buffer while playback occurs from it. When the buffer runs low, the system can refill it seamlessly, ensuring smooth, uninterrupted playback.
Data Streaming Applications
In data streaming, especially with network communication, circular buffers are crucial for managing incoming packets. They allow for efficient buffering and processing of network packets without losing data due to overflow.
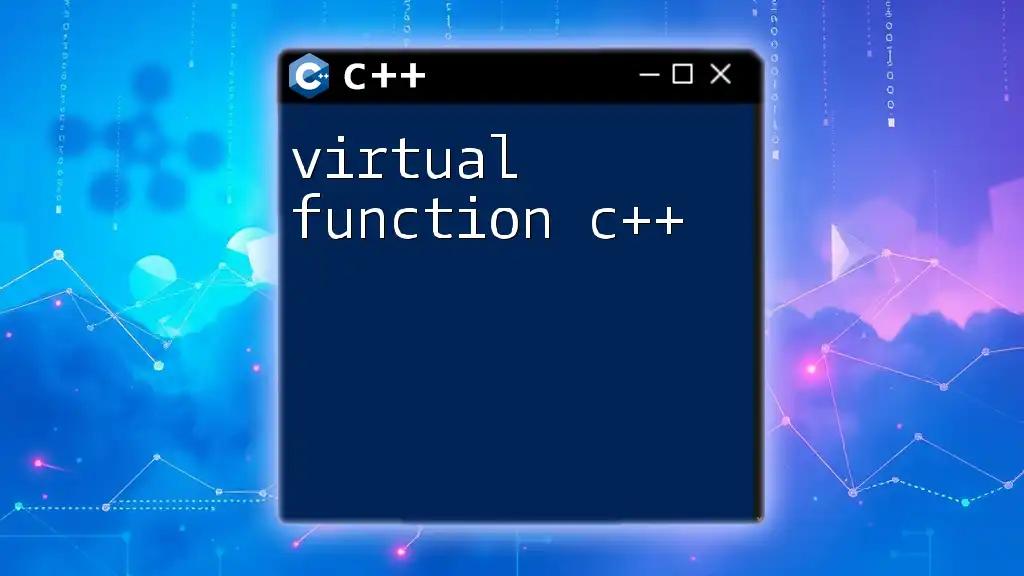
Best Practices for Using Circular Buffers in C++
Performance Optimization
To ensure optimal performance of circular buffers, consider the following best practices:
- Minimize Locking: In multithreaded applications, try to minimize the time locks are held.
- Use Efficient Data Types: Choose the most appropriate data types for your use case to reduce overhead.
Memory Management
Avoid memory leak issues by ensuring the buffer is deallocated correctly when no longer needed. Always implement proper destructors and ensure that dynamic memory allocations are managed accurately.
Testing Your Circular Buffer Implementation
Testing is crucial to validate the functionality of your circular buffer. Some effective strategies include:
- Implement unit tests for insertion, deletion, and resizing.
- Create scenarios that simulate real-world usage, especially under high-load conditions.
Example test cases should consider edge cases such as inserting into a full buffer and removing from an empty one.

Conclusion
Understanding and implementing a circular buffer in C++ is a vital skill for developers working with data streaming and real-time processing. By leveraging this efficient data structure, you can manage data reliably while optimizing memory and performance. As you implement circular buffers, be mindful of best practices, testing, and potential pitfalls in multithreaded environments to ensure robust applications.

Additional Resources
For those looking to deepen their knowledge further, consider exploring additional reading materials and resources, online courses, and programming documentation. Engaging with programming communities, forums, and contributing to open-source projects can also expand your understanding of circular buffers and optimize your C++ programming skills.