A lexical analyzer in C++ is a program that reads input characters to produce tokens, which are the building blocks for syntactic analysis in compilers.
Here's a simple code snippet that demonstrates how to implement a basic lexical analyzer in C++:
#include <iostream>
#include <cctype>
#include <string>
#include <vector>
class Lexer {
public:
Lexer(const std::string& src) : source(src), currentIndex(0) {}
std::vector<std::string> tokenize() {
std::vector<std::string> tokens;
while (currentIndex < source.size()) {
if (isspace(source[currentIndex])) {
currentIndex++;
} else if (isalpha(source[currentIndex])) {
tokens.push_back(parseIdentifier());
} else {
tokens.push_back(std::string(1, source[currentIndex]));
currentIndex++;
}
}
return tokens;
}
private:
std::string source;
size_t currentIndex;
std::string parseIdentifier() {
std::string identifier;
while (currentIndex < source.size() && isalnum(source[currentIndex])) {
identifier += source[currentIndex++];
}
return identifier;
}
};
int main() {
Lexer lexer("int main() { return 0; }");
std::vector<std::string> tokens = lexer.tokenize();
for (const auto& token : tokens) {
std::cout << token << std::endl;
}
return 0;
}
What is Lexical Analysis?
Lexical analysis is the first phase of the compilation process, where the source code is divided into meaningful units known as tokens. It involves scanning the input text (source code) and converting it into a sequence of tokens that can be understood by the compiler or interpreter. This process is critical as it simplifies the parsing phase that follows and allows for the efficient processing of source code.
The importance of lexical analysis in compilers and interpreters cannot be overstated. It helps in identifying different components of a programming language, ensuring that the syntax is correctly adhered to. In C++, lexical analysis aids in the conversion of code into a format that can be utilized by the parser.
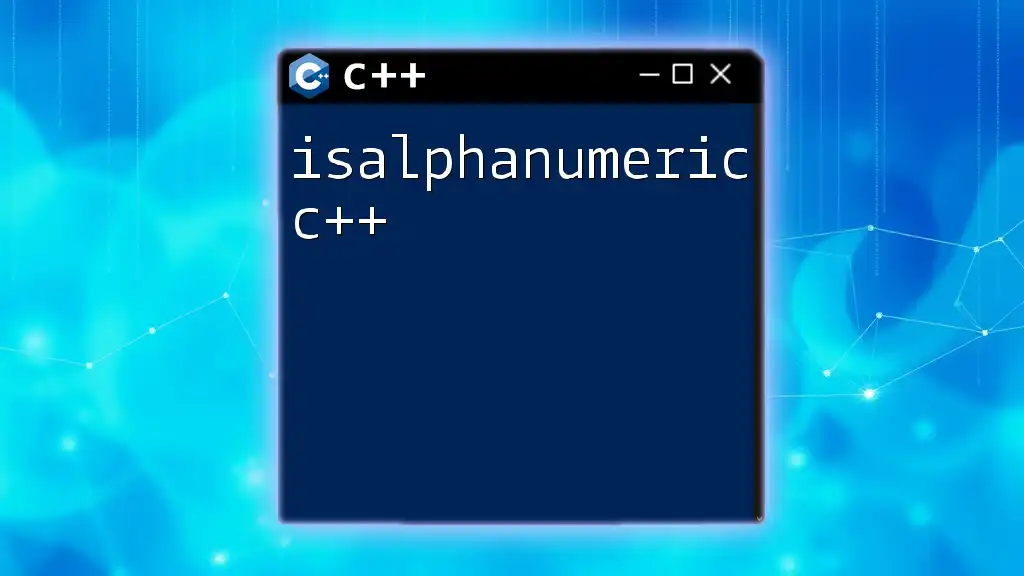
Overview of the C++ Lexical Analyzer
In C++, a lexical analyzer acts as an intermediary that transforms raw source code into a structured format using tokens. The C++ lexical analyzer identifies distinct tokens such as keywords, literals, identifiers, and operators, creating a token stream that serves as input for the parser.
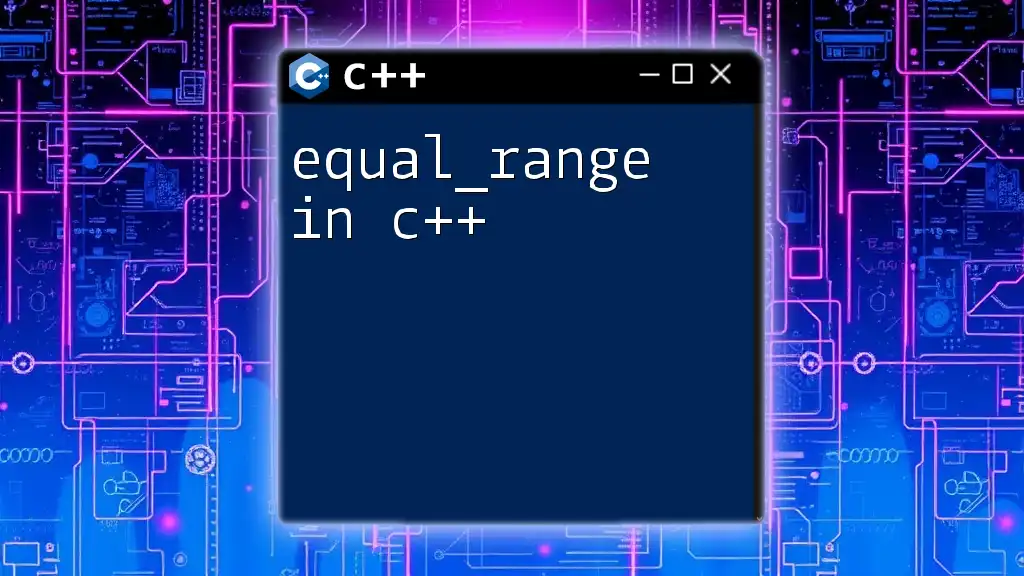
Understanding the Components of a Lexical Analyzer
Tokens: The Building Blocks
Tokens are the basic elements identified during lexical analysis. Each type of token serves a specific purpose within C++ code. Here are the main types of tokens:
- Keywords: Reserved words that have special meaning, such as `int`, `return`, and `if`.
- Identifiers: Names given to variables, functions, or other user-defined items.
- Literals: Fixed values not subject to change, such as numeric literals (`10`, `3.14`) or string literals (`"Hello, World!"`).
- Operators: Symbols that represent operations, such as `+`, `-`, `*`, and `/`.
Regular Expressions and Finite Automata
Regular expressions are vital in lexical analysis as they provide a formal way to specify the patterns of tokens. They enable the lexical analyzer to recognize tokens in the source code efficiently.
Finite automata, a theoretical model of computation, play a significant role in tokenization. They allow the construction of a state machine that processes the input character by character, transitioning between states based on the recognized patterns. This approach is foundational in the design of a C++ lexical analyzer.
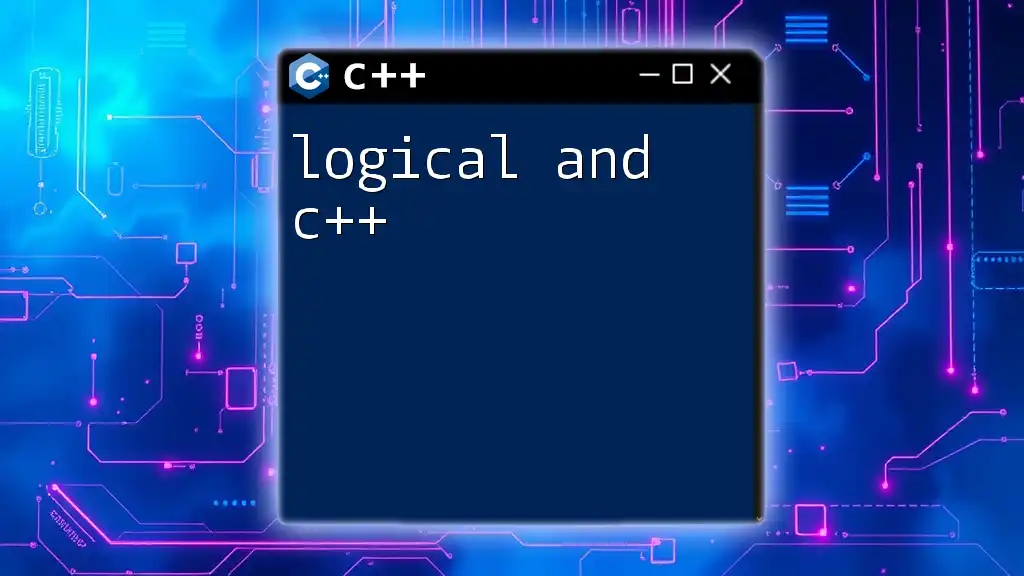
Building a Simple Lexical Analyzer in C++
Setting Up the Development Environment
To build a lexical analyzer in C++, you need to set up your development environment. Ensure that you have a C++ compiler like GCC or Clang installed along with a text editor or an integrated development environment (IDE) such as Visual Studio or Code::Blocks.
A suggested project structure might look like this:
/lexical_analyzer
|-- main.cpp
|-- LexicalAnalyzer.h
|-- LexicalAnalyzer.cpp
Implementation of a C++ Lexical Analyzer
Here’s a basic outline of a simple lexical analyzer in C++:
// LexicalAnalyzer.h
#ifndef LEXICALANALYZER_H
#define LEXICALANALYZER_H
#include <string>
class LexicalAnalyzer {
public:
void analyze(const std::string &sourceCode);
// Other member functions
};
#endif // LEXICALANALYZER_H
// LexicalAnalyzer.cpp
#include "LexicalAnalyzer.h"
#include <iostream>
void LexicalAnalyzer::analyze(const std::string &sourceCode) {
// Analysis code goes here
std::cout << "Analyzing source code: " << sourceCode << std::endl;
}
In this code snippet, the `LexicalAnalyzer` class sets the foundation for the analyzer, with a member function `analyze` to process the source code.
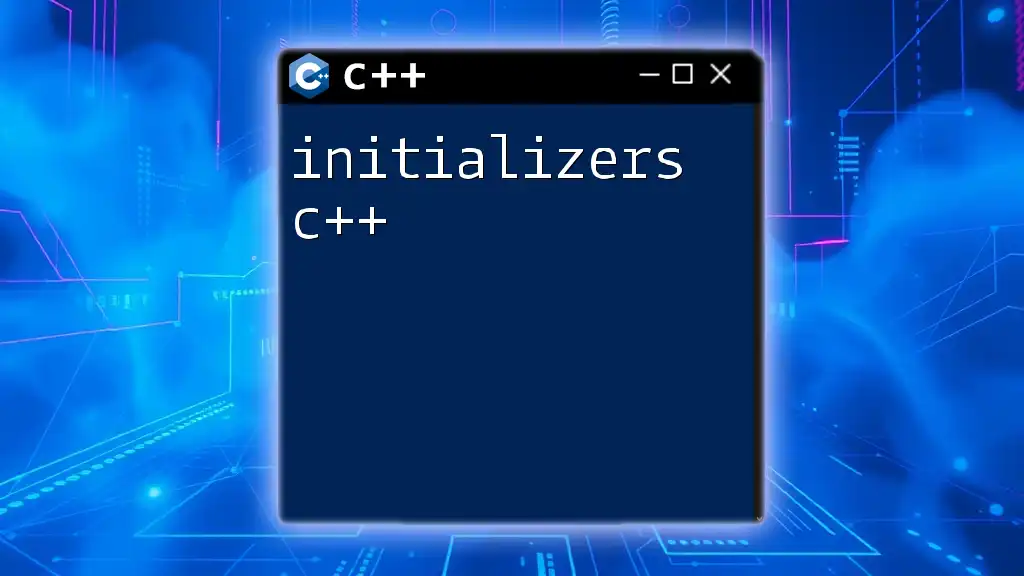
Breaking Down the Lexer Logic
Input Handling
The lexical analyzer must effectively read the source code. Implement a function to handle file reading and manage end-of-file (EOF) conditions gracefully, ensuring that the entire source is processed correctly. It is crucial to skip whitespace and comments, as they do not contribute to the token stream.
Tokenizing the Input
To identify tokens, the tokenizer logic can be implemented in the `tokenize` method. Below is a foundational structure of this logic:
void LexicalAnalyzer::tokenize(const std::string &sourceCode) {
for (char c : sourceCode) {
// Sample logic to identify tokens
if (std::isalpha(c)) {
// Handle identifiers and keywords
} else if (std::isdigit(c)) {
// Handle literals
}
// Handle other character types
}
}
This logic should include conditions for successfully recognizing and categorizing different token types.
Error Handling in Lexical Analysis
Error handling during lexical analysis is crucial—common errors arise from invalid tokens or unexpected characters.
Implement mechanisms to report errors effectively, providing feedback such as:
- Line and column number at which the error occurred.
- An informative error message describing the issue.
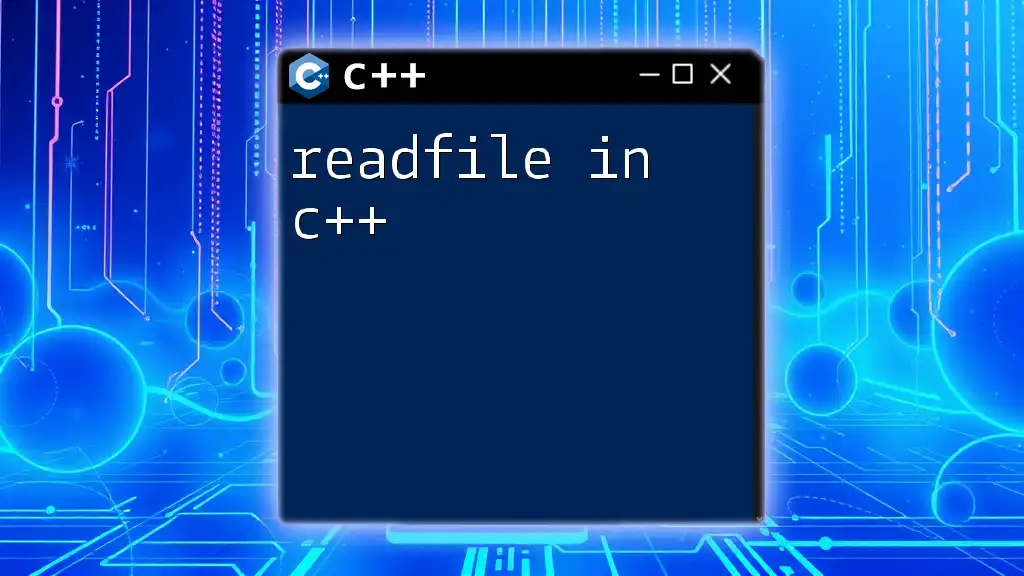
Advanced Concepts in Lexical Analysis
Finite State Machines in Lexical Analyzers
Finite State Machines (FSMs) can effectively manage the states of the lexer during token recognition. An FSM can transition between various states based on input characters, which allows you to maintain organized control over the tokenization process. Here’s a basic outline of an FSM for lexical analysis:
class FSM {
private:
// FSM states and transitions
public:
void processInput(char c);
// Transition and state management
};
Performance Considerations
As the input size increases, performance becomes critical. Implement optimizations such as:
- Buffering inputs to minimize file I/O operations.
- Using efficient data structures to store tokens and error messages.
Managing memory can also minimize overhead—consider using smart pointers in C++ to avoid memory leaks and ensure efficient use of resources.
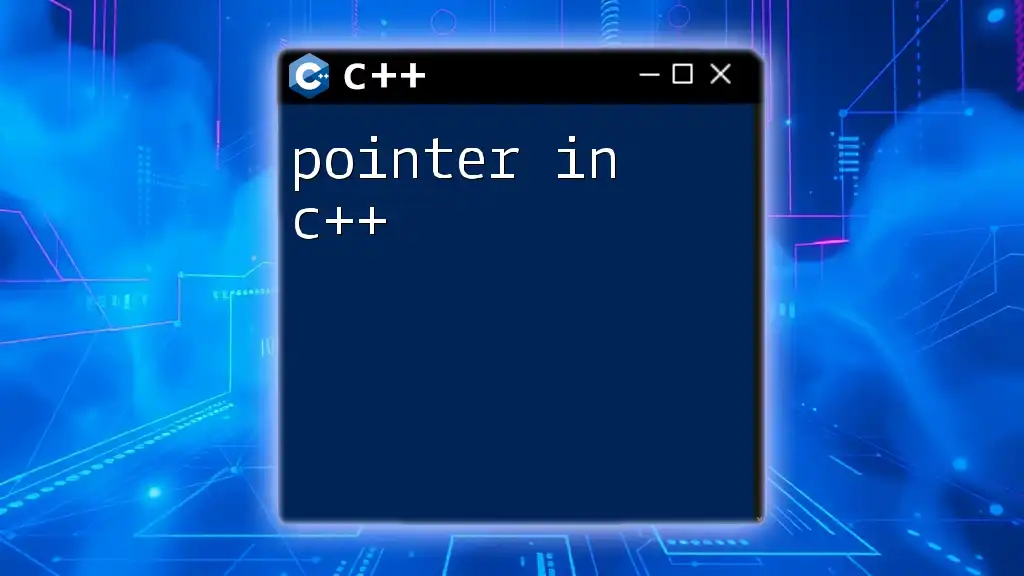
Testing Your Lexical Analyzer
Writing Test Cases
Robust unit tests will help confirm the accuracy and reliability of your lexical analyzer. Design test cases to validate various scenarios, including:
- Multiple token types in different orders.
- Invalid input that should trigger error handling.
Debugging Techniques
To maintain a stable codebase, employ effective debugging techniques:
- Use debugging tools integrated into your IDE, such as breakpoints and variable watches.
- Implement logging mechanisms to track the lexer’s internal state during execution, allowing for easier identification of bugs.
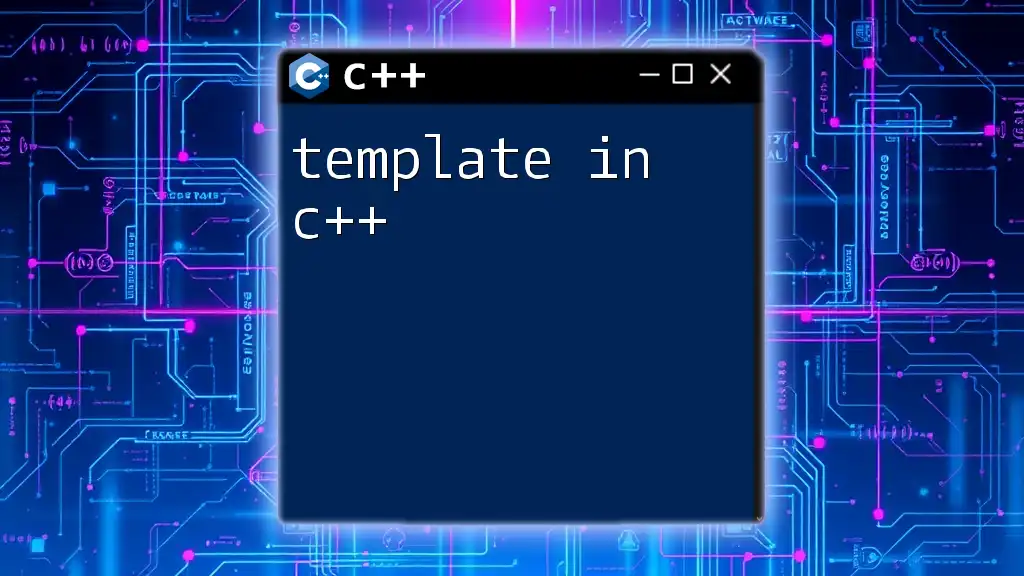
Conclusion
In summary, the role of a lexical analyzer in C++ is foundational in processing source code efficiently. By understanding how to identify tokens, implement FSMs, and manage input effectively, you can create robust lexical analysis tools that serve as the backbone for any C++ parser or compiler. For those looking to deepen their understanding, numerous resources and literature are available that explore lexical analysis concepts further, providing a richer foundation to build upon.
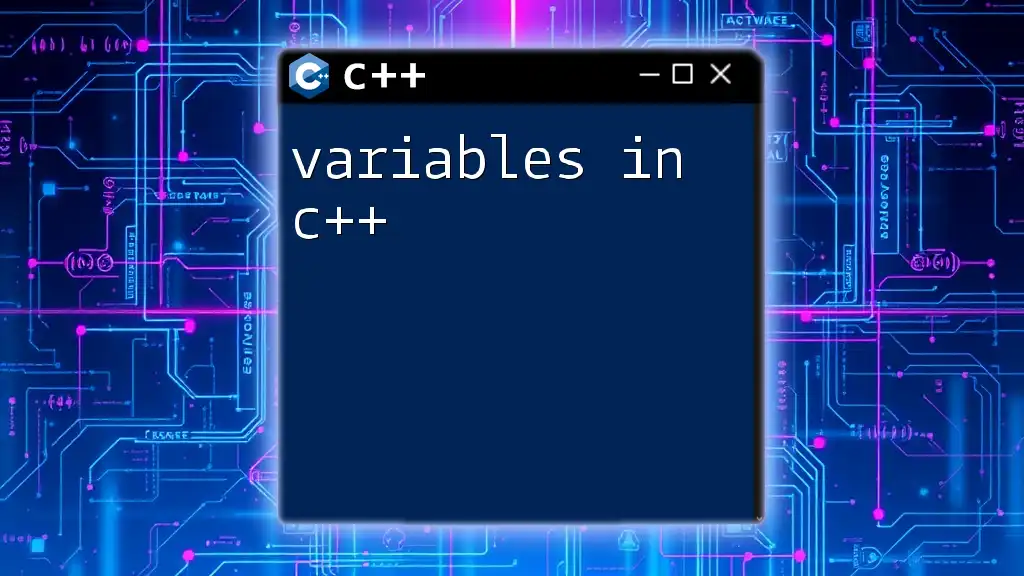
FAQs about Lexical Analyzers in C++
What is the difference between a lexical analyzer and a parser?
A lexical analyzer processes input to convert it into tokens, while a parser takes those tokens to build a syntax tree representing the grammatical structure of the code.
How do I handle different character encodings in a lexical analyzer?
Utilize libraries that support various encodings, such as UTF-8, to ensure your lexical analyzer can process different character sets effectively.
Can I use existing libraries for lexical analysis in C++?
Yes, libraries such as Flex or ANTLR can greatly simplify the process of building a lexical analyzer and save development time.