Topological sort in C++ is an algorithm used to order the vertices of a directed acyclic graph (DAG) in a linear sequence, ensuring that for every directed edge from vertex `u` to vertex `v`, `u` comes before `v` in the ordering.
Here's a simple implementation of topological sort using DFS in C++:
#include <iostream>
#include <vector>
#include <stack>
void topologicalSortUtil(int v, std::vector<bool>& visited, std::stack<int>& Stack, const std::vector<std::vector<int>>& adj) {
visited[v] = true;
for (int i : adj[v]) {
if (!visited[i]) {
topologicalSortUtil(i, visited, Stack, adj);
}
}
Stack.push(v);
}
void topologicalSort(int V, const std::vector<std::vector<int>>& adj) {
std::stack<int> Stack;
std::vector<bool> visited(V, false);
for (int i = 0; i < V; i++) {
if (!visited[i]) {
topologicalSortUtil(i, visited, Stack, adj);
}
}
while (!Stack.empty()) {
std::cout << Stack.top() << " ";
Stack.pop();
}
}
int main() {
int V = 6; // Number of vertices
std::vector<std::vector<int>> adj(V);
// Adding edges to the adjacency list
adj[5].push_back(2);
adj[5].push_back(0);
adj[4].push_back(0);
adj[4].push_back(1);
adj[2].push_back(3);
adj[3].push_back(1);
std::cout << "Topological Sort: ";
topologicalSort(V, adj);
return 0;
}
What is Topological Sort?
Topological sort is a method of ordering the vertices in a directed acyclic graph (DAG) in such a way that for every directed edge UV from vertex U to vertex V, vertex U comes before vertex V in the ordering. This ordering is essential in scenarios where certain tasks depend on others, making it a critical concept in graph theory.
The importance of topological sorting extends to numerous practical applications, including scheduling tasks, programming language compilation sequence, and even managing workflow dependencies.
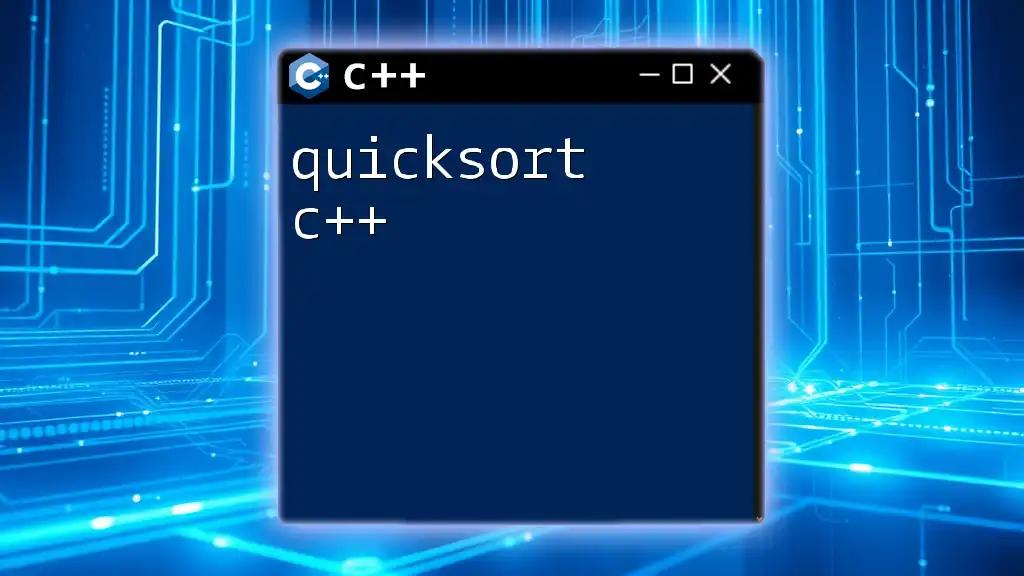
Understanding Graphs
What is a Graph?
A graph is a mathematical structure used to model pairwise relations between objects. It consists of vertices (or nodes) and edges that connect these vertices.
Types of Graphs
- Directed Graphs: Here, edges have a direction, indicating a one-way relationship.
- Undirected Graphs: Edges represent mutual relationships.
- Weighted Graphs: Edges carry weights, often used to depict costs or distances.
For example, consider the directed graph below:
A → B
A → C
B → D
C → D
In this graph, A has directed edges to B and C, while both B and C direct edges to D.

Topological Sorting Explained
Definition of Topological Sorting
Topological sorting facilitates the arrangement of nodes in a linear sequence based on their dependency relationships, making it indispensable in scenarios where certain tasks must be completed before others.
Properties of Topological Sort
- Directed Acyclic Graph (DAG): Topological sorting is only applicable to DAGs. If a graph contains a cycle, it's impossible to perform a topological sort as it creates conflicting dependencies.
- Uniqueness of Order: Depending on the graph, there can be multiple valid topological sorts or just one unique ordering.

Algorithms for Topological Sorting
Depth-First Search (DFS) Approach
The DFS-based approach to topological sorting utilizes recursive traversal of the graph. It marks nodes as visited and pushes them onto a stack after all their adjacent nodes have been processed, ensuring that dependencies are respected.
Step-by-step breakdown:
- Initialize a visited array to track visited nodes.
- Traverse each node using DFS, marking nodes as visited.
- After finishing the exploration of all adjacent nodes, push the node onto a stack.
- Finally, pop nodes from the stack to produce the sorted order.
Code Snippet: DFS Based Topological Sort
#include <iostream>
#include <vector>
#include <stack>
void dfs(int v, std::vector<bool>& visited, std::stack<int>& Stack, const std::vector<std::vector<int>>& adj) {
visited[v] = true;
for (int neighbor : adj[v]) {
if (!visited[neighbor]) {
dfs(neighbor, visited, Stack, adj);
}
}
Stack.push(v);
}
std::vector<int> topologicalSortDFS(int V, const std::vector<std::vector<int>>& adj) {
std::stack<int> Stack;
std::vector<bool> visited(V, false);
for (int i = 0; i < V; i++) {
if (!visited[i]) {
dfs(i, visited, Stack, adj);
}
}
std::vector<int> result;
while (!Stack.empty()) {
result.push_back(Stack.top());
Stack.pop();
}
return result;
}
Kahn's Algorithm (BFS Approach)
Kahn's algorithm utilizes a breadth-first approach to achieve topological sorting. It works by repeatedly removing nodes with zero incoming edges, ensuring that once a node is removed, its dependent nodes are correctly processed.
Step-by-step breakdown:
- Compute the in-degree of each vertex (number of incoming edges).
- Initialize a queue and enqueue all vertices with zero in-degrees.
- While the queue is not empty, dequeue a vertex and add it to the result.
- Decrease the in-degree of its adjacent nodes; if any nodes’ in-degree becomes zero, enqueue them.
Code Snippet: Kahn's Algorithm Based Topological Sort
#include <iostream>
#include <vector>
#include <queue>
std::vector<int> topologicalSortKahn(int V, const std::vector<std::vector<int>>& adj) {
std::vector<int> in_degree(V, 0);
for (const auto& neighbors : adj) {
for (int neighbor : neighbors) {
in_degree[neighbor]++;
}
}
std::queue<int> q;
for (int i = 0; i < V; i++) {
if (in_degree[i] == 0) {
q.push(i);
}
}
std::vector<int> result;
while (!q.empty()) {
int node = q.front();
q.pop();
result.push_back(node);
for (int neighbor : adj[node]) {
in_degree[neighbor]--;
if (in_degree[neighbor] == 0) {
q.push(neighbor);
}
}
}
return result;
}

Practical Applications
Use Cases of Topological Sorting
-
Task Scheduling and Dependencies: It is particularly useful in project management, where certain tasks must be completed before others. Topological sorting ensures that tasks are performed in the correct order.
-
Compilation Order in Programming Languages: When multiple files depend on each other, topological sorting determines the optimal order for compiling them.
-
Resolving Package Dependencies: For package managers, a topological sort can help resolve the installation order based on dependencies.

Common Mistakes and Troubleshooting
Identifying Cyclic Graphs
One of the most common mistakes when dealing with topological sorting is attempting to sort a graph that contains cycles. If you suspect a cycle exists, you can implement a cycle detection step before performing a topological sort.
Performance Considerations
Understanding the efficiency of the algorithms is crucial. A DFS-based approach generally runs in O(V + E) time, where V is the number of vertices and E is the number of edges. Kahn's algorithm also runs in O(V + E) time but has different space complexities due to the use of queues.

Conclusion
In summary, topological sorting is essential for managing dependencies in graphs, particularly in directed acyclic graphs. Whether using a DFS or Kahn's algorithm, understanding these methods can significantly enhance problem-solving skills in programming and data structuring.
For further exploration, delving into algorithmic complexities and experimenting with varied graph structures can solidify your understanding. Additionally, consider practicing coding challenges that involve topological sort to master the topic and apply your knowledge effectively.