The `boolalpha` manipulator in C++ allows you to output boolean values as "true" or "false" instead of 1 or 0 when using streams.
#include <iostream>
#include <iomanip> // for std::boolalpha
int main() {
bool myBool = true;
std::cout << std::boolalpha << myBool << std::endl; // Outputs: true
return 0;
}
What is boolalpha?
`boolalpha` is an I/O manipulator in C++ that allows you to control the way boolean values (`true` and `false`) are represented when outputting to streams. Instead of the default outputs, which are the integers `1` (for true) and `0` (for false), using `boolalpha` enables the textual representation, making your output more human-readable. This feature enhances clarity, particularly in debugging sessions or when presenting results to end-users.
Historically speaking, `boolalpha` was introduced in the C++ standard library to provide developers with a more intuitive method to display boolean values, aligning with the language's goal of maintaining readability in code.
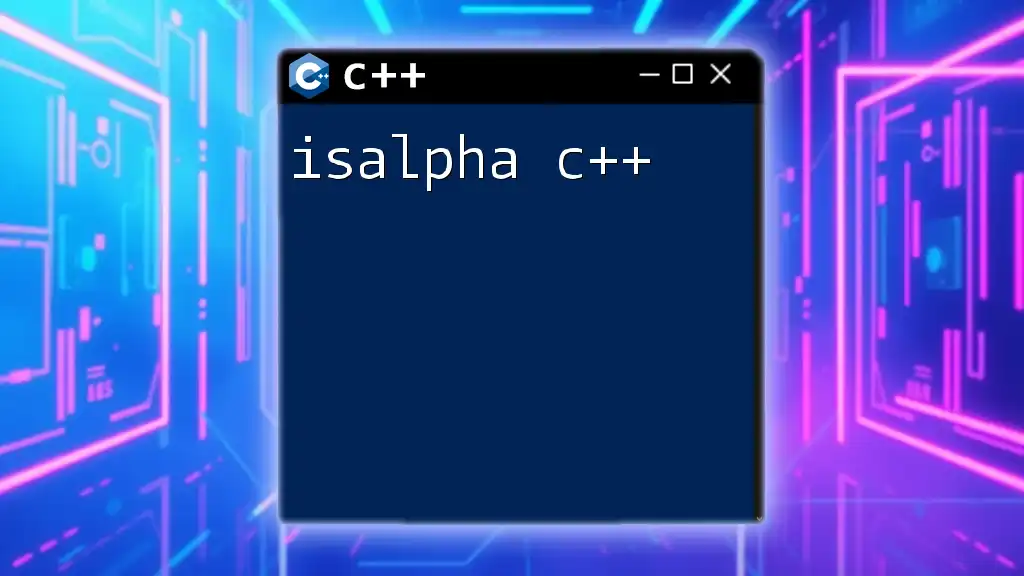
Purpose of boolalpha
Using `boolalpha` serves an essential purpose in programming by converting boolean output from numerical representations to their string equivalents. The benefits of this approach are clear:
-
Readability: Textual representations like `true` and `false` are much easier to understand at a glance, especially for those who might not be as familiar with the code.
-
Debugging: When debugging, it’s crucial to grasp variable states quickly. Seeing `true` and `false` eliminates the ambiguity that comes with numeric outputs and helps you spot problems swiftly.
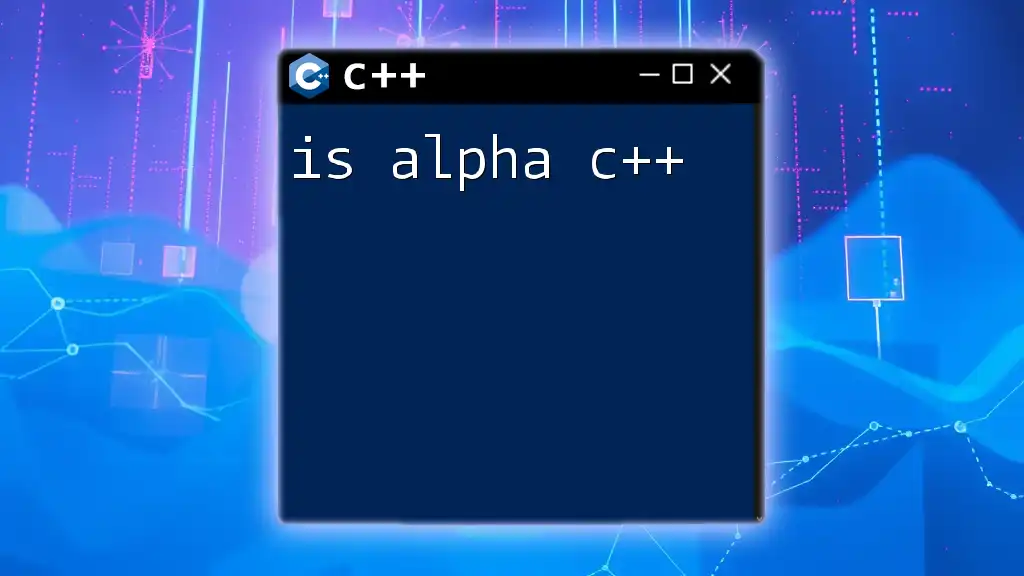
Enabling boolalpha
How to Use boolalpha
To enable `boolalpha`, you must include it within the scope of your output subsequent to including the necessary headers. Here’s a straightforward example to illustrate its use:
#include <iostream>
int main() {
bool flag = true;
std::cout << std::boolalpha << flag << std::endl; // Outputs: true
return 0;
}
In this code, the statement `std::cout << std::boolalpha << flag` directs the program to print `true` instead of `1`. This simple change can drastically improve the clarity of your output, especially beneficial when working with various boolean variables in your application.
Setting boolalpha in Streams
When you set `boolalpha` for a stream, it remains effective until you either change it or reset the stream. This capability allows you to feed outputs seamlessly:
#include <iostream>
int main() {
std::cout << std::boolalpha;
std::cout << true << " " << false << std::endl; // Outputs: true false
std::cout << std::noboolalpha;
std::cout << true << " " << false << std::endl; // Outputs: 1 0
return 0;
}
In this example, the first part of the output uses `boolalpha`, which prints `true` and `false`. The second part, after switching to `noboolalpha`, reverts to numeric representation. It’s crucial to recognize how these manipulators interact and the impact they have on subsequent outputs.
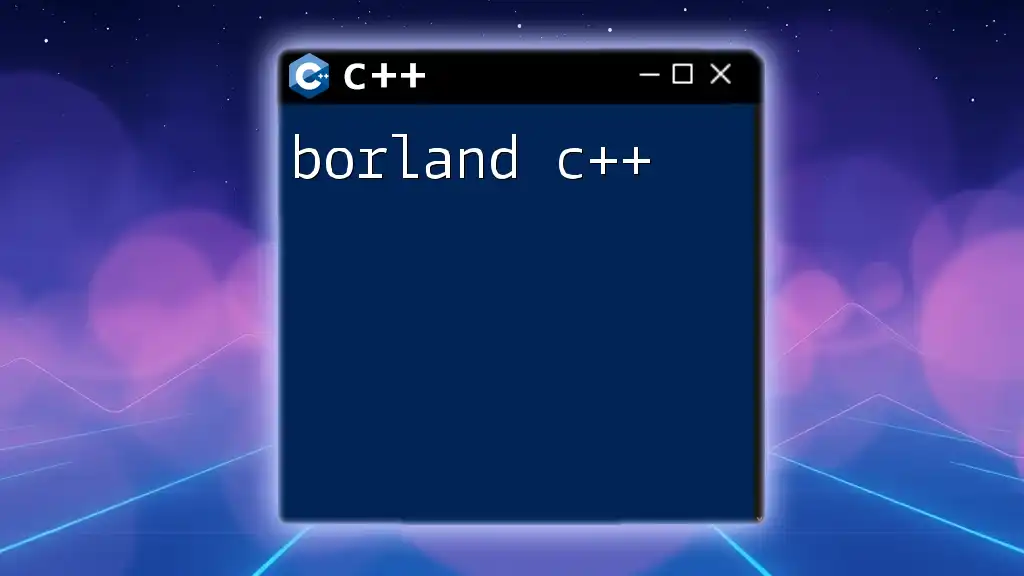
Combining boolalpha with Other I/O Manipulators
Using with Additional Formatters
You can leverage `boolalpha` alongside other formatters to achieve more complex outputs. For example, consider this code snippet that combines `boolalpha` with formatting functions:
#include <iostream>
#include <iomanip>
int main() {
bool flag = true;
double pi = 3.14;
std::cout << std::boolalpha << flag << std::endl;
std::cout << std::fixed << std::setprecision(2) << pi << std::endl;
return 0;
}
In this instance, `std::boolalpha` converts the boolean output, while `std::fixed` and `std::setprecision(2)` control the floating-point display. This shows how easily one can combine formatting to create clear and structured console output.
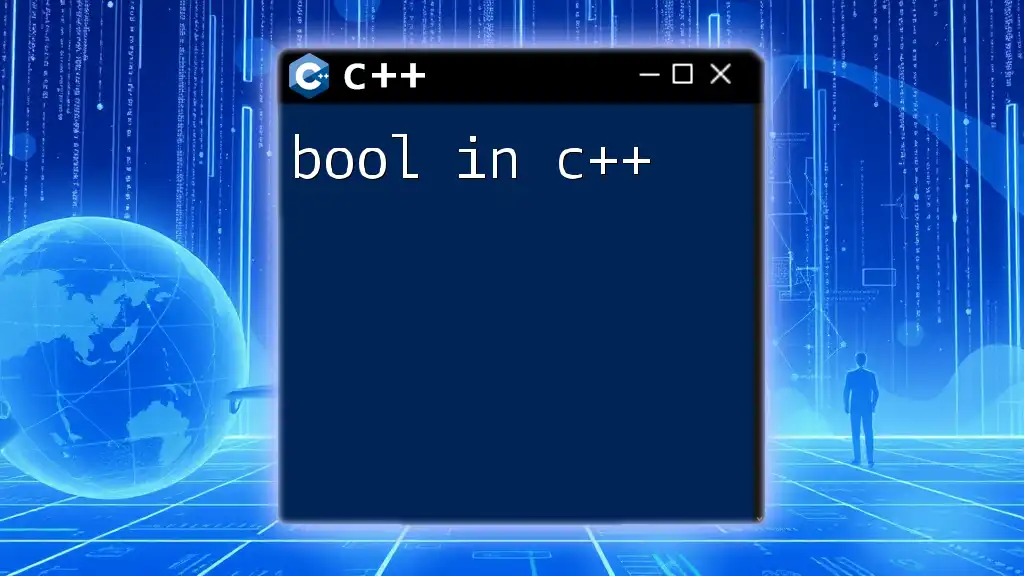
Disabling boolalpha
Returning to Default Behavior
After working with `boolalpha`, you may find yourself needing to revert to the default numeric representation. By using the `noboolalpha` manipulator, you can switch back effortlessly:
#include <iostream>
int main() {
std::cout << std::boolalpha << true << std::endl; // Outputs: true
std::cout << std::noboolalpha << true << std::endl; // Outputs: 1
return 0;
}
The code demonstrates how easy it is to toggle between representations. Always remember to revert to default where necessary, especially in larger software projects where visual consistency might be critical.
Importance of Resetting Stream Formatters
When working on extensive applications, resetting your stream formatters, including `boolalpha`, can prevent confusion and ensure all outputs maintain their intended format, leveraging clarity and consistency throughout your code.
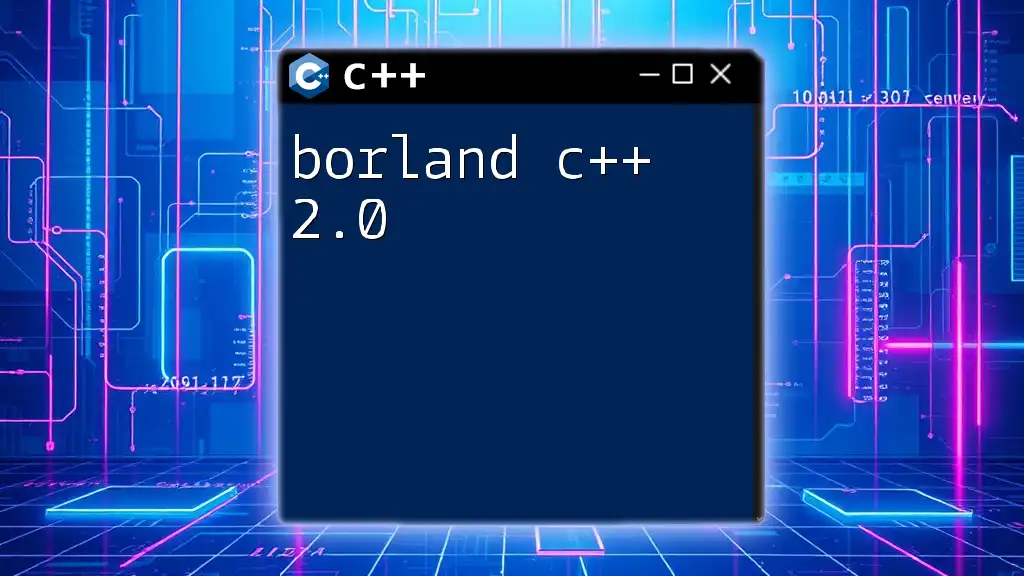
Common Use Cases for boolalpha
Debugging Outputs
Using `boolalpha` becomes invaluable in debugging scenarios where tracking boolean states is essential. A practical example is creating a logging function that utilizes the textual representation of boolean values:
#include <iostream>
void logStatus(bool status) {
std::cout << "Current status: " << std::boolalpha << status << std::endl;
}
int main() {
logStatus(true);
return 0;
}
In this instance, logging boolean states is more straightforward with `boolalpha`. Not only is this output more user-friendly, but it also allows for quicker comprehension of what’s occurring in your application without needing to interpret numeric values.
Outputting Boolean Results from Functions
When functions return boolean values, displaying these results using `boolalpha` benefits any developer looking for clarity. Here’s a code example:
#include <iostream>
bool isEven(int number) {
return number % 2 == 0;
}
int main() {
int testNumber = 10;
std::cout << "Is number " << testNumber << " even? " << std::boolalpha << isEven(testNumber) << std::endl;
return 0;
}
Observe how the output is more comprehensible and straightforward for anyone reading it. Utilizing `boolalpha` in this context simplifies the checking of conditions and their outcomes.
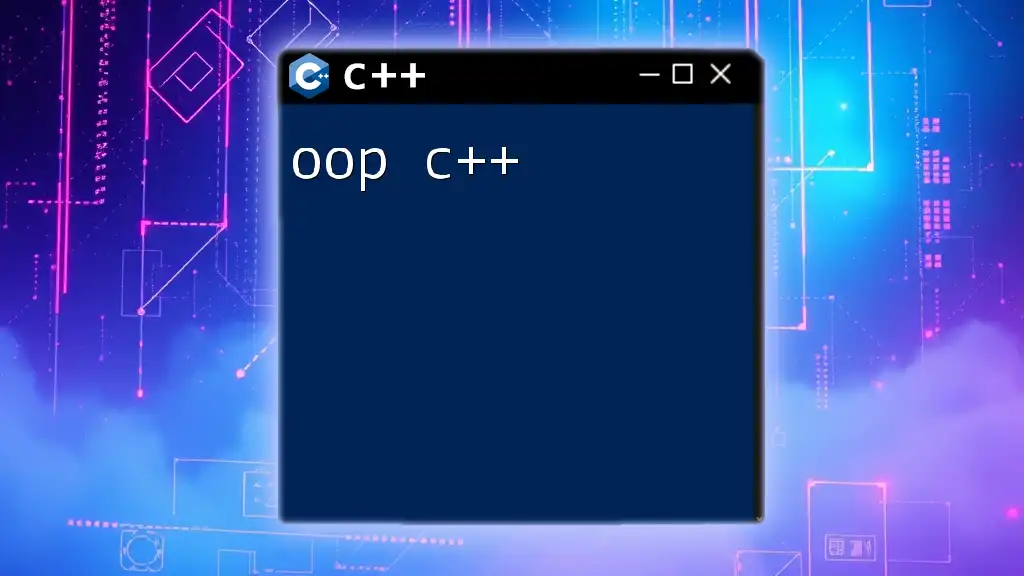
Tips and Best Practices
Consistency in Output
In collaborative environments, maintaining a consistent output style is paramount. Using `boolalpha` for boolean outputs creates uniformity in your codebase. Establishing standards for how outputs are printed helps reduce misunderstandings among team members.
Performance Considerations
While `boolalpha` offers readability advantages, it’s vital to be aware of its potential performance implications. In performance-sensitive sections of code or in high-frequency output situations, the overhead introduced by using manipulators should be weighed against the benefits of clearer output.
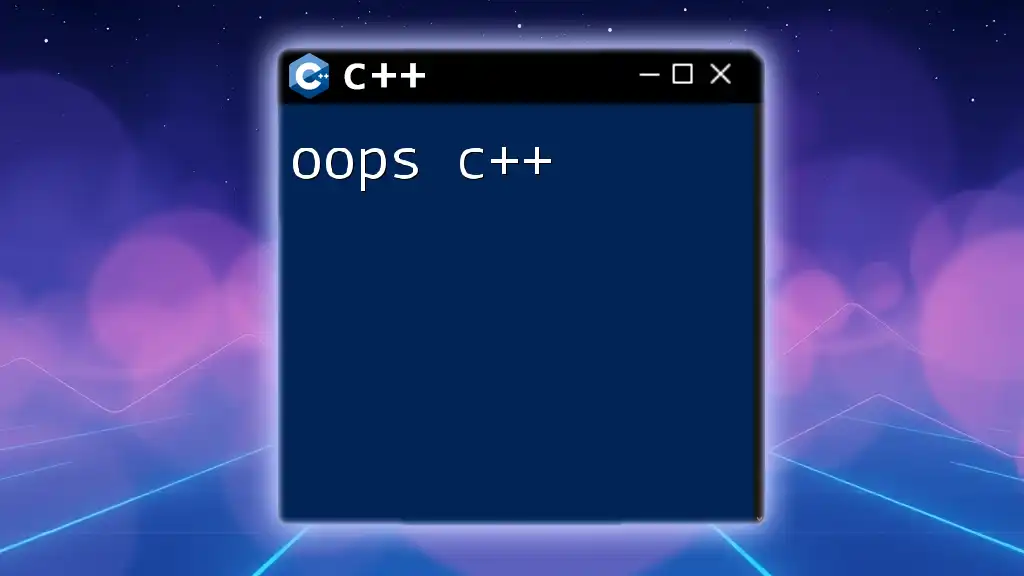
Conclusion
In conclusion, adopting `boolalpha` in your C++ projects can significantly enhance the clarity and readability of boolean outputs. By utilizing this simple yet powerful manipulator, you provide a better experience for anyone interacting with your code, from future developers to end-users. Embrace its benefits not only for your own clarity but also to deliver well-documented and understandable software.
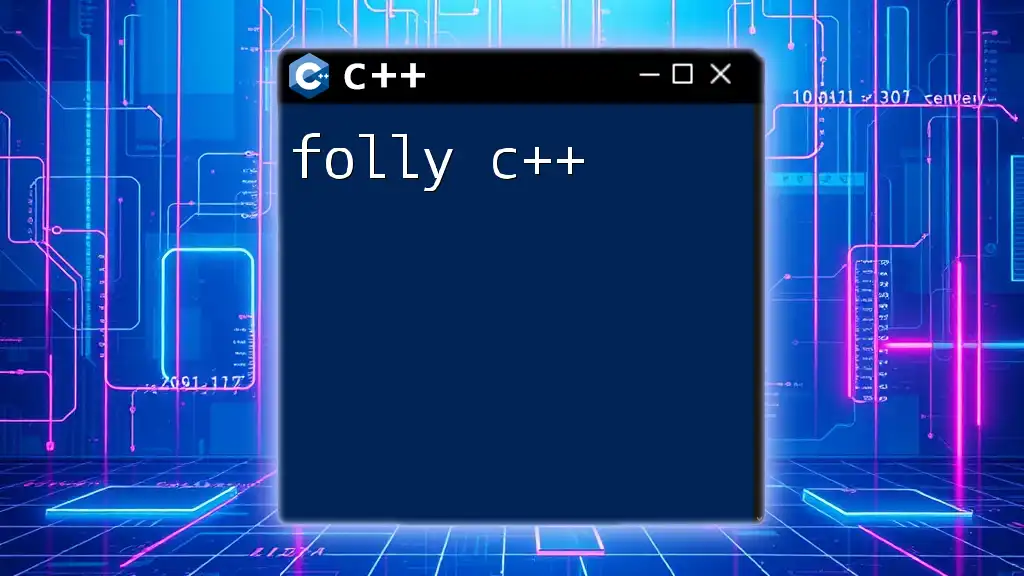
Additional Resources
To deepen your understanding of `boolalpha`, check out the official C++ documentation and consider exploring additional literature or online resources focusing on C++ programming techniques. By continuously learning and applying these principles, you can improve your coding skills and code quality significantly.