Borland C++ 2.0 is a development environment released in the early '90s that provided an integrated programming interface for creating DOS applications using the C++ language.
Here's a simple code snippet demonstrating how to print "Hello, World!" in Borland C++ 2.0:
#include <iostream.h>
void main() {
cout << "Hello, World!" << endl;
}
Installation of Borland C++ 2.0
System Requirements
Before diving into the features and functionalities of Borland C++ 2.0, it's important to ensure that your system meets the necessary requirements. This version, released in the early 1990s, may not demand much compared to modern software, but checking compatibility is crucial.
- Minimum Hardware Requirements: Typically, a compatible IBM PC or compatible system with at least 640KB of RAM and adequate disk space is needed.
- Software Requirements: Ideally, you should be running a DOS-compatible operating system. It's worth noting that modern operating systems may require additional setups, such as running DOS emulators.
Downloading the Software
Finding a legitimate source for Borland C++ 2.0 can be challenging due to its age. Look for reputable repositories or archives that focus on legacy software to download the installation files safely.
Installation Process
Once you've obtained Borland C++ 2.0, follow these steps for installation:
- Extract the Files: If the files are compressed, ensure you extract them to an accessible directory.
- Access the Setup Program: Run the `INSTALL.EXE` file from the command prompt.
- Follow On-Screen Instructions: The installer will guide you through the installation process. You’ll typically define the destination directory and other settings.
- Common Issues: In case of installation failures, ensure that your system settings, such as memory and drive formats, are correctly configured.
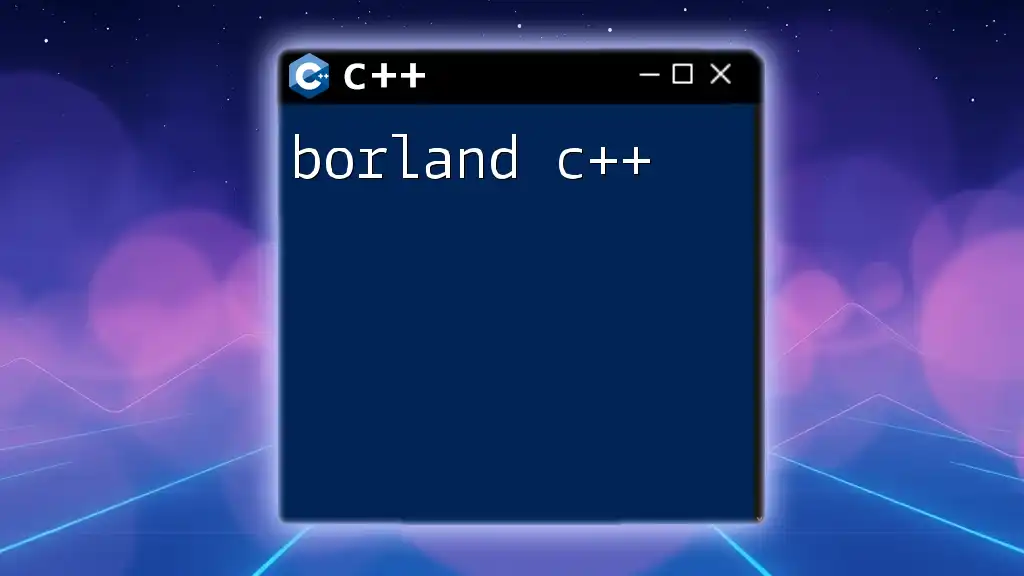
Features of Borland C++ 2.0
Integrated Development Environment (IDE)
The Borland C++ 2.0 IDE is intuitive and user-friendly, designed to enhance programming efficiency.
Overview of the IDE Layout
Upon launching the IDE, you will notice various panels, including:
- Menu Bar: Access all primary functions like file management, editing, and project configuration.
- Toolbars: Quick access to frequently used tools like compile and debug.
- Project Manager: A panel for creating and managing projects efficiently, allowing for better organization of your code files.
Key Components
- Code Editor: The main area for writing and editing C++ code, supporting basic features such as color coding and line numbering.
- Output Window: Displays compilation results, error messages, and other notifications.
Compiler Capabilities
Borland C++ 2.0 offers a robust compiler that is compliant with the C++ standards of its time. A few notable features include:
- Syntax Highlighting: Enhances readability and debugging by color-coding different code components.
- Code Completion: Speeds up coding with context-aware suggestions for functions and variables.
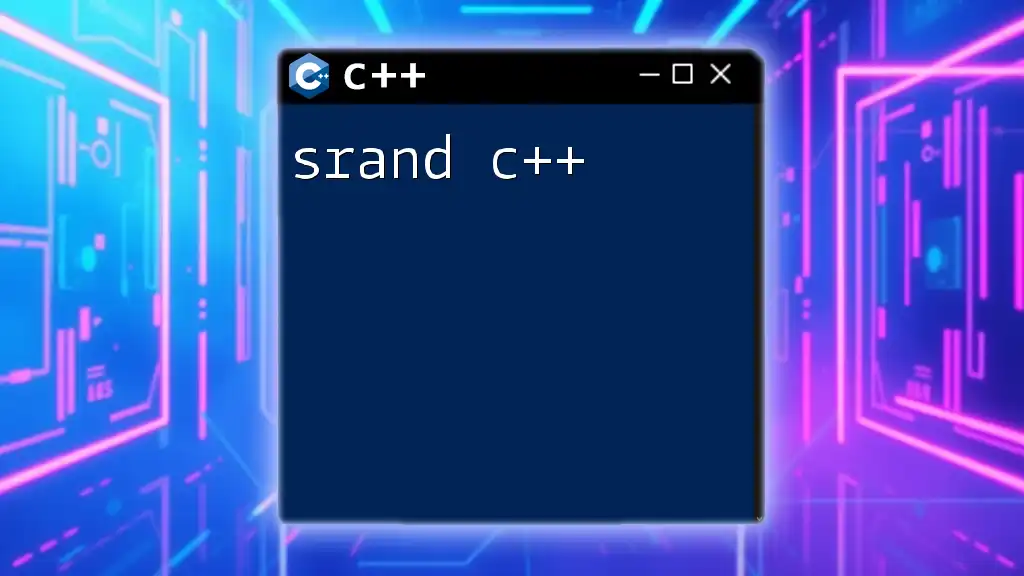
Basic Syntax in Borland C++ 2.0
Overview of C++ Syntax
Understanding C++ syntax is essential for effective programming. Key components include:
- Data types: Use fundamental types like `int`, `char`, and `float` to define the kind of data you will be working with.
- Variables and Constants: Declare variables for storing data and constants for fixed values.
- Operators: Use arithmetic operators like `+`, `-`, `*`, and `/`, as well as logical operators like `&&` and `||`.
Writing Your First Program
To illustrate the basic syntax, let's write a simple program that displays "Hello, World!" on the screen:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
In this code snippet:
- `#include <iostream>` includes the Input/Output stream library.
- `using namespace std;` allows us to use standard functions without the prefix.
- `int main() { ... }` represents the main function where execution starts.
Compiling and Running the Program
To compile and run this simple program in Borland C++ 2.0, follow these steps:
- Open the IDE and create a new project.
- Enter the Code: Input your C++ code into the code editor.
- Compile the Program: Use the compile button or access it through the menu to check for errors.
- Run the Program: Once compiled without errors, execute the program from the IDE.
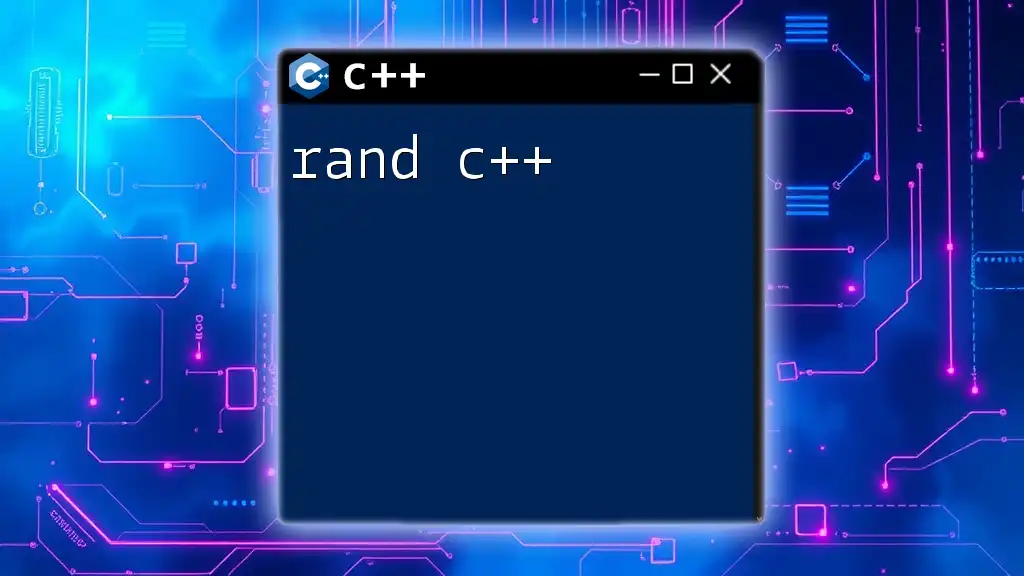
Advanced Features of Borland C++ 2.0
Object-Oriented Programming (OOP) Support
One of the most powerful features of Borland C++ 2.0 is its support for Object-Oriented Programming (OOP).
Classes and Objects
C++ allows you to create classes that encapsulate data and functions:
class Animal {
public:
void sound() {
cout << "Animal makes a sound" << endl;
}
};
class Dog : public Animal {
public:
void sound() {
cout << "Dog barks" << endl;
}
};
In this example:
- `Animal` is a base class with a method for producing sound.
- `Dog` inherits from `Animal` and overrides the `sound` method.
Inheritance and Polymorphism
The ability to inherit functionalities and modify behaviors enhances code reusability and flexibility. You can define various data types using a common interface.
Template Programming
Templates enable you to write generic functions to operate on different data types:
template <typename T>
T getMax(T a, T b) {
return (a > b) ? a : b;
}
With this template function, you can find the maximum of two values, regardless of their type (assuming they support comparison).
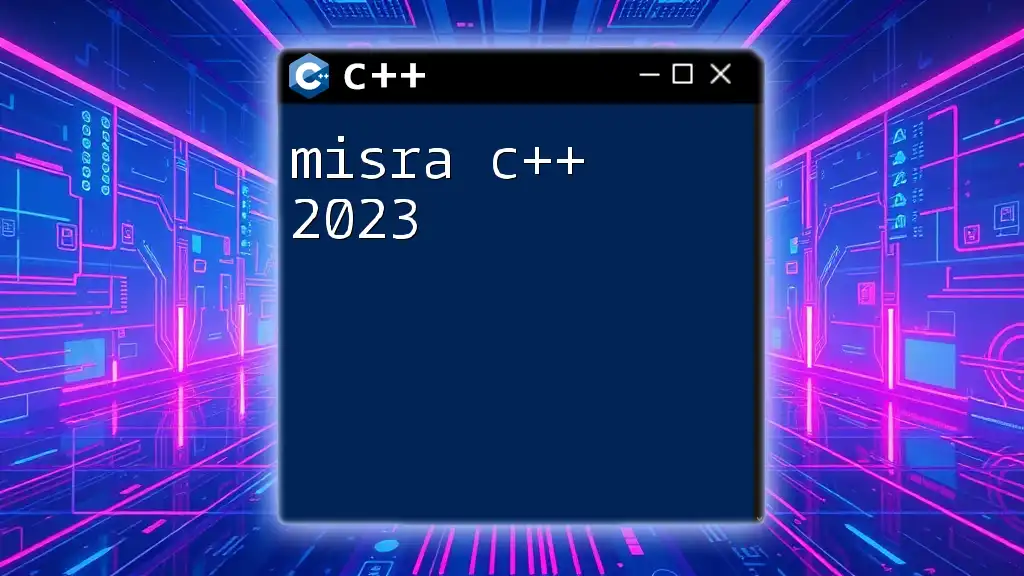
Debugging in Borland C++ 2.0
Setting Breakpoints
Utilize breakpoints to pause program execution at specific points. This allows you to inspect the state of variables before proceeding.
Using the Debugger
The integrated debugger in Borland C++ 2.0 is a valuable tool. You can:
- Step Through Code: Execute your program line by line to monitor how variables change.
- Inspect Variables and Memory: Check the values of variables at any point in your code, which helps track down bugs.
Common Debugging Techniques
Familiarize yourself with common debugging strategies, such as printing debug statements to the console or using assert statements to verify assumptions.
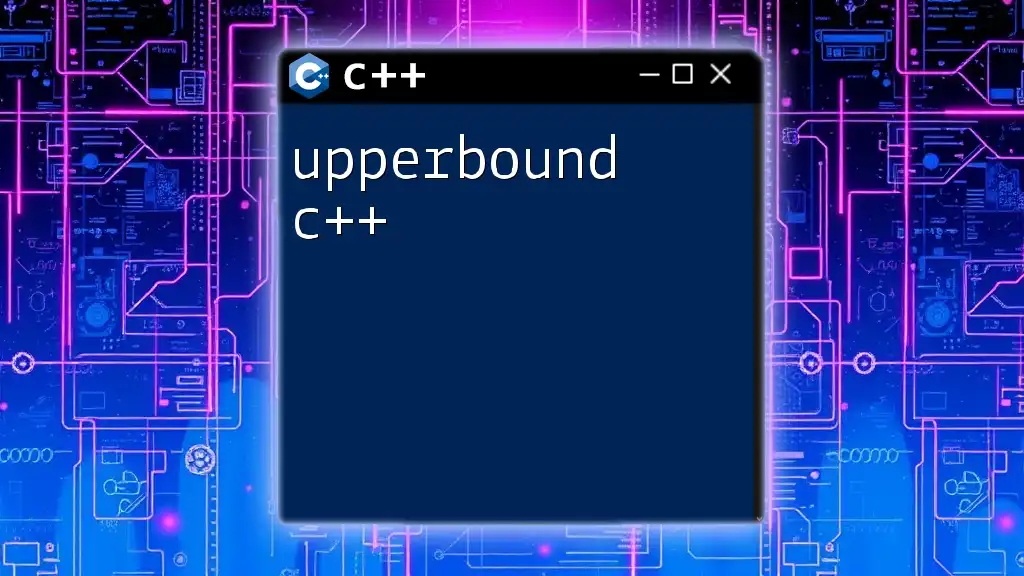
Best Practices when Using Borland C++ 2.0
Coding Standards
Establishing a clear coding standard is crucial for maintainable code. Follow these principles:
- Naming Conventions: Use descriptive names for variables and functions, following camelCase or snake_case styles as appropriate.
- Code Organization: Group related functions and classes logically and comment generously to explain code functionality.
Performance Optimization
To write efficient applications in Borland C++ 2.0, understand performance optimization techniques:
- Avoid Unnecessary Calculations: Store results of expensive operations to prevent duplicate computations.
- Memory Management: Be mindful of dynamic memory allocation and deallocation to avoid leaks.
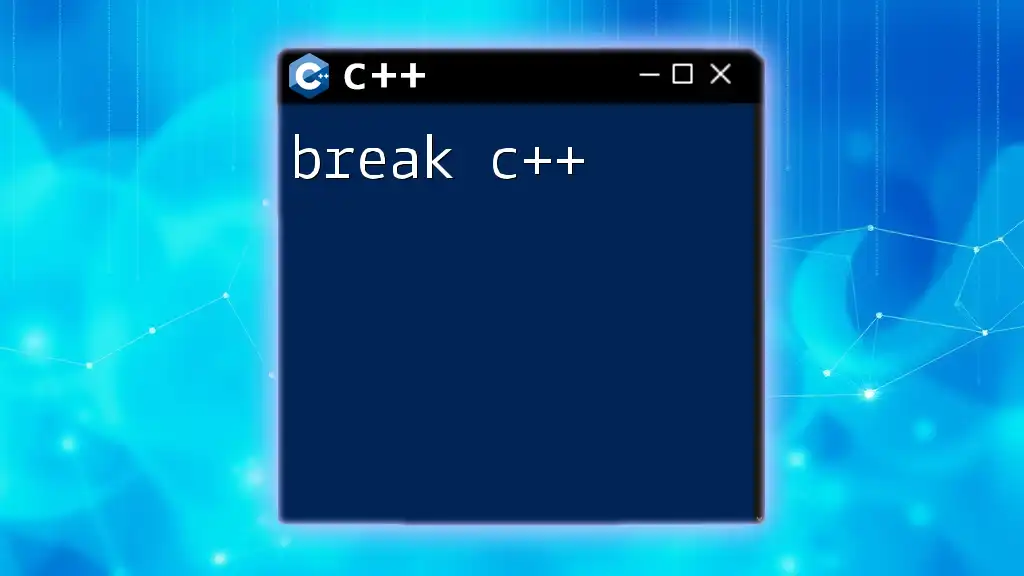
Tutorials and Resources
Online Communities and Forums
Engage with online communities dedicated to C++ programming. Many forums allow users to ask questions, share knowledge, and collaborate on projects. Consider exploring platforms like Stack Overflow and Reddit's r/Cplusplus.
Recommended Books and Materials
Invest in learning materials dedicated to Borland C++ 2.0 and C++ in general. Classic books by authors like Bjarne Stroustrup provide foundational knowledge, while specialized books may offer targeted guidance for Borland.
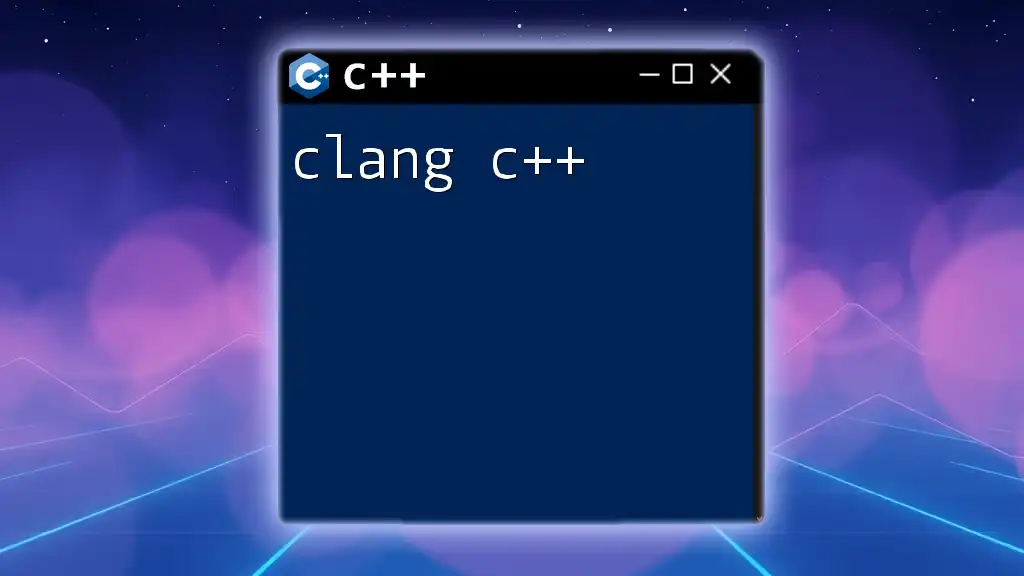
Conclusion
In this comprehensive guide, we explored various aspects of Borland C++ 2.0, from installation to advanced programming features. By mastering the tools and techniques available in this environment, you're well on your way to becoming a proficient C++ programmer. Don't hesitate to dive deeper into the functionalities of Borland C++ 2.0 and uncover even more of what this powerful tool can do. Join our community of learners to enhance your coding skills and share your journey!