To read data in C++, you can utilize the standard input stream `cin` to capture user input directly from the console. Here's a simple code snippet demonstrating this:
#include <iostream>
using namespace std;
int main() {
int number;
cout << "Enter a number: ";
cin >> number;
cout << "You entered: " << number << endl;
return 0;
}
Understanding Input and Output in C++
C++ is a powerful programming language that emphasizes performance and efficiency. At its core, understanding how to manage Input and Output (I/O) operations is crucial. I/O operations allow you to interact with users and external data, making your applications dynamic and responsive.
C++ Standard Input/Output Library
To perform any I/O operations in C++, you need to include the iostream header. This library provides essential functionalities to read from and write to standard input and output.
#include <iostream>
By including this header, you'll have access to the basic I/O commands like `cin` and `cout`.
Additionally, utilizing the std namespace simplifies the code by allowing you to write commands without prefixing them with `std::`.
using namespace std;

The `cin` Command for Input
The `cin` command is your gateway to reading user input. It allows you to gather various types of data, whether they be integers, characters, or strings.
Inputting Different Data Types
Reading Integers
Reading integers using `cin` is straightforward and commonly used. Here’s how you can ask a user for their age:
int age;
cout << "Enter your age: ";
cin >> age;
With this code snippet, the program will wait for the user to input their age. Type safety is crucial here; if the input is an invalid character (like a string), it will lead to runtime errors.
Reading Strings
When it comes to reading strings, using `getline()` offers more flexibility by reading an entire line of text, including spaces. Here's how you can use it:
string name;
cout << "Enter your name: ";
getline(cin, name);
This command reads everything until the newline character, ensuring you capture full names, including those with spaces.

The `cout` Command for Output
The `cout` command is used for displaying output to the console. It is essential for providing feedback to users or presenting information.
Formatting Output
To polish your output, you can make use of manipulators. Manipulators are built-in functions that allow control over how data is displayed.
For instance, the `endl` manipulator is used to insert a new line and flush the output buffer, while `setw()` can set the width of the next output value. Here’s an example:
#include <iomanip>
cout << "Formatted output: " << setprecision(2) << fixed << number << endl;
This code formats the `number` variable to display two decimal places, enhancing the display of floating-point values.
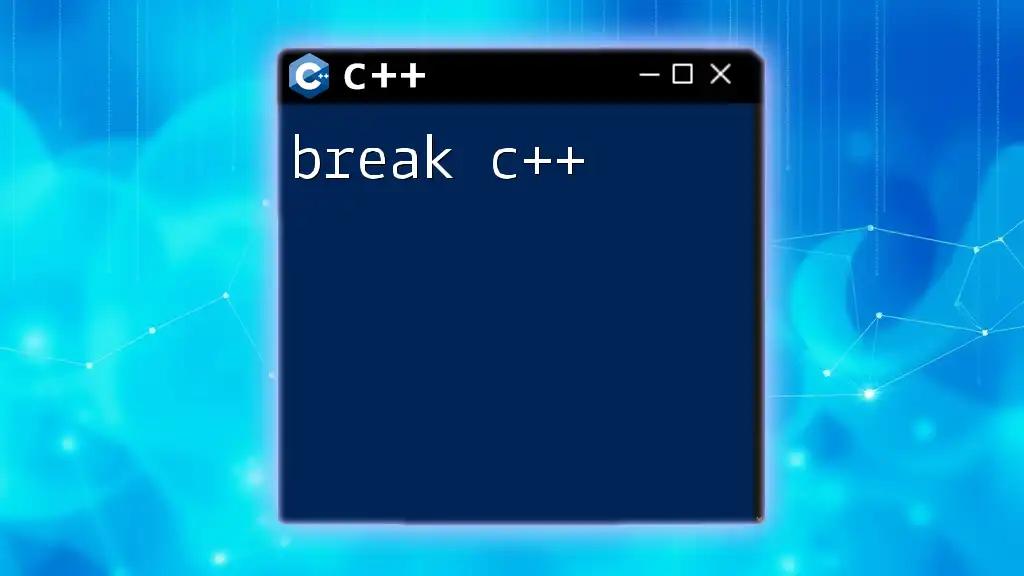
Error Handling with Input/Output
When dealing with user input, errors can occur. Understanding common I/O errors and how to handle them is vital for creating robust applications.
Common I/O Errors
When using `cin`, if the input does not match the expected data type, an error state is triggered. You can check and handle these scenarios effectively:
if (cin.fail()) {
cout << "Invalid input. Please enter a number." << endl;
cin.clear(); // Clear the error state
cin.ignore(numeric_limits<streamsize>::max(), '\n'); // Discard invalid input
}
This code snippet effectively clears any erroneous input and resets the state of `cin`, allowing the user to try again.
Using Exceptions for Robust Programs
For more complex applications, using exceptions can provide a structured error handling mechanism. Here’s an example to illustrate:
try {
// Code that may throw an exception
} catch(exception& e) {
cout << "Error: " << e.what() << endl;
}
By using `try` and `catch`, you can manage unexpected scenarios gracefully, informing the user without crashing the application.

Advanced Input and Output Techniques
For more complex operations, C++ offers advanced techniques such as file I/O, allowing you to read data from or write data to files.
File Input and Output
To perform file I/O, you need to include the fstream library. This provides functionalities to manage files in a way similar to standard I/O streams.
#include <fstream>
fstream file("example.txt", ios::out);
if (file.is_open()) {
file << "Writing to the file.\n";
file.close();
}
In this example, we open a file for writing. If it's successfully opened, we can write some content to it before closing the file.
Serialization: Saving Object States
For object-oriented programming, serialization involves converting an object into a format that can be stored or transmitted and then reconstructed later. This is particularly useful in saving application state or user data.
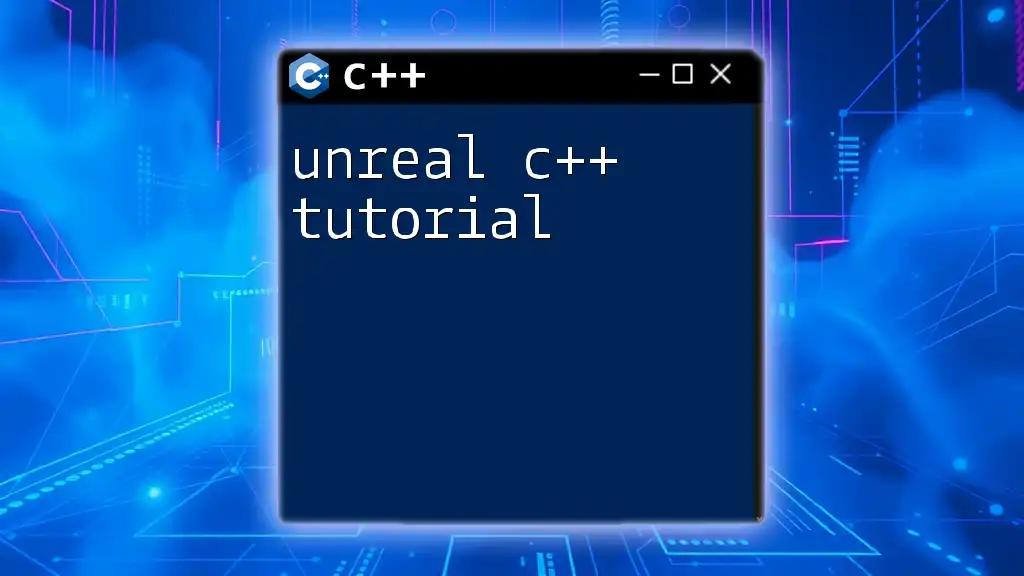
Best Practices for Command Usage in C++
When learning how to read C++ commands, adhering to certain best practices will improve the quality of your code.
Being Consistent with Syntax
Maintain consistent syntax throughout your code. Avoid unnecessary complexity and strive for clarity. Commenting your code to explain key operations increases maintainability and readability, especially for more complex I/O operations.
Optimizing Performance
I/O operations can sometimes be a bottleneck in your applications. Optimize performance by minimizing the number of I/O calls and buffering data whenever possible. Techniques like reading large chunks of data at once can significantly improve efficiency.
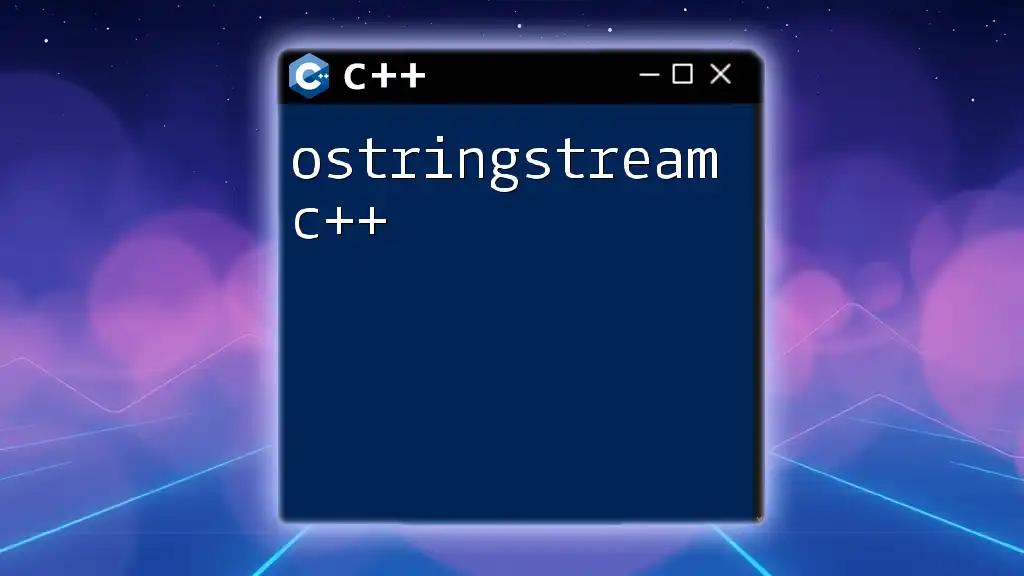
Conclusion
Mastering how to "read C++" effectively is foundational for creating interactive and user-friendly applications. This involves not just using `cin` and `cout`, but also handling errors, formatting output, and engaging with advanced techniques like file I/O and serialization. By applying the concepts discussed in this guide, you'll be well-prepared to manage input and output operations in your C++ programs confidently.
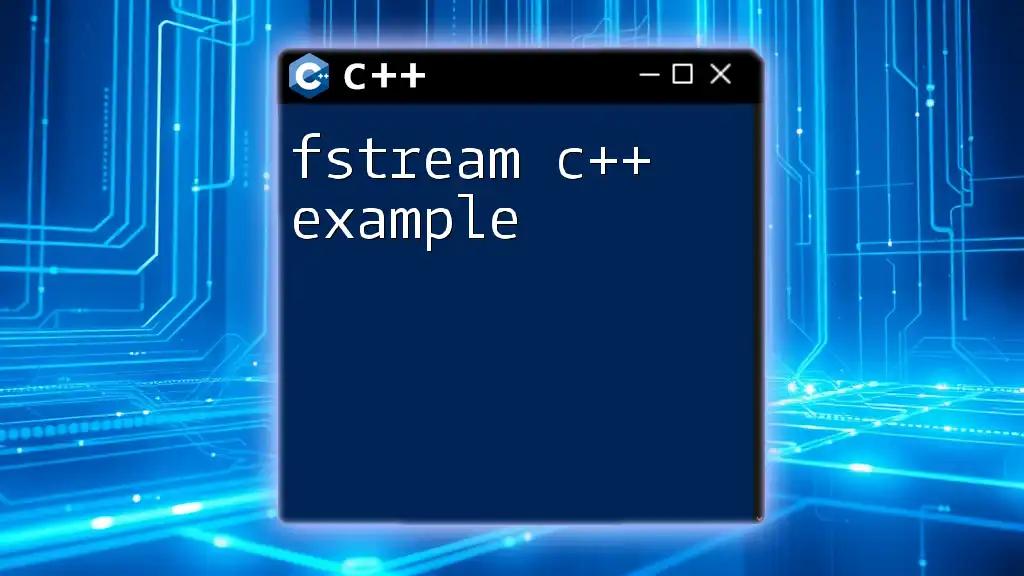
Additional Resources
To deepen your understanding, consider exploring recommended C++ books, tutorials, and online courses focused on C++ I/O operations. Practice exercises can further reinforce your learning, enabling you to apply the principles discussed in real-world scenarios.