Unreal C++ provides a powerful programming approach for fine-tuned control and performance in game development, while Blueprints offer a visual scripting alternative that allows for rapid prototyping and easier iteration without extensive coding knowledge.
Here's a simple code snippet in C++ for spawning an actor:
AActor* SpawnedActor = GetWorld()->SpawnActor<AActor>(ActorClass, SpawnLocation, SpawnRotation);
Understanding Unreal Engine Programming
What is Unreal C++?
Unreal C++ refers to the programming language used to develop games and applications within Unreal Engine. C++ is a powerful object-oriented language that provides developers with a high degree of control over system resources, performance optimizations, and memory management. This makes it particularly suitable for large-scale projects and tasks that require complex calculations or custom algorithms.
Advantages of using C++ include:
- Performance: C++ is compiled into machine code, allowing for optimized execution speeds. This means that game mechanics that rely on heavy computation, like physics simulations or AI behavior, can run more efficiently.
- Control: With C++, developers can manipulate system resources directly, which is crucial for customizing low-level features or algorithms that require extensive optimization.
Example Code Snippet:
void AMyActor::BeginPlay()
{
Super::BeginPlay();
UE_LOG(LogTemp, Warning, TEXT("BeginPlay called"));
}
In this example, the function `BeginPlay()` is overridden in an actor class to add custom functionality upon the start of the game. The log output helps with debugging and understanding when the code executes.
What are Unreal Blueprints?
Blueprints represent a visual scripting language within Unreal Engine that allows developers to create gameplay elements without writing conventional code. This makes it particularly accessible to non-programmers, such as designers and artists. Blueprints enable users to create complex gameplay interactions through a graphical interface that illustrates the flow of logic visually.
Advantages of using Blueprints include:
- Visual Scripting: The node-based nature allows rapid comprehension of gameplay logic, making it easy to design and implement new features without needing extensive programming knowledge.
- Rapid Prototyping: Blueprints support faster iteration, enabling developers to quickly test new ideas and receive real-time feedback.
Blueprint Example: Imagine a simple Blueprint setup that handles character movement. The visual representation would consist of various nodes connected by lines that show how input from the player corresponds to actions in the game. For instance, an "Input Key" node could connect to a "Move Forward" node, illustrating how pressing a key triggers forward movement.
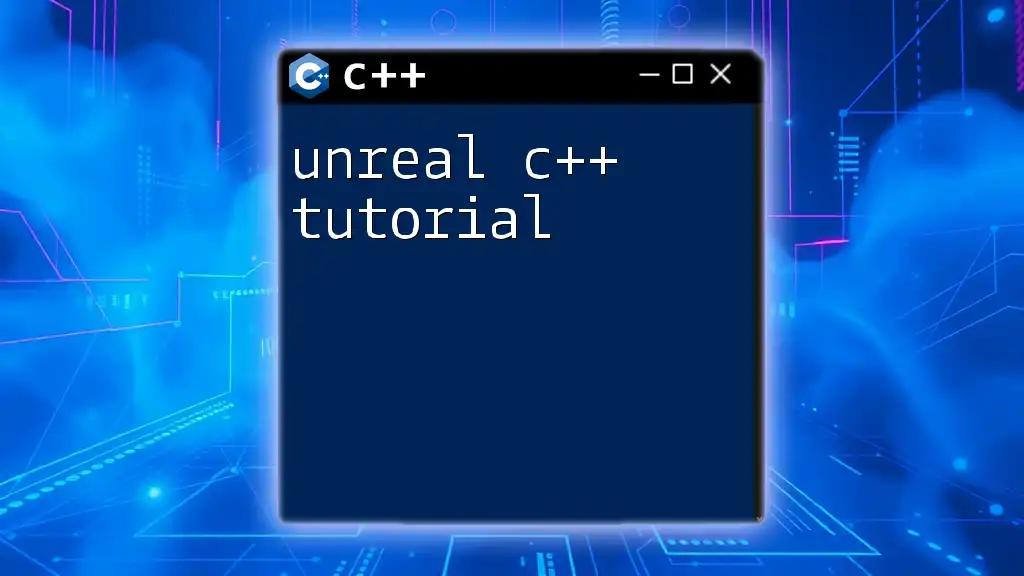
Unreal Blueprint vs C++
Workflow Differences
One of the major distinctions between unreal C++ vs blueprint is the workflow involved in each.
In C++, developers often need to write, compile, and debug their code, which can be time-consuming, especially for larger projects. However, this approach rewards developers with robust performance and flexibility.
On the other hand, Blueprints allow for immediate visual feedback. Developers can tweak gameplay mechanics on-the-fly without needing to stop, compile, or restart the game. This enables a faster and more iterative approach to design that some may find less frustrating.
Performance Considerations
Performance is a central consideration in the discussion of blueprint vs c++. C++ often shines in performance-critical applications. For example, if you're developing a game with complex physics or AI, using C++ would typically yield better performance due to its lower-level control and optimizations.
Conversely, while Blueprints may introduce some performance overhead, for many gameplay elements, this overhead is negligible. Thus, for smaller projects or elements that don’t require intense processing, Blueprints could be sufficient.
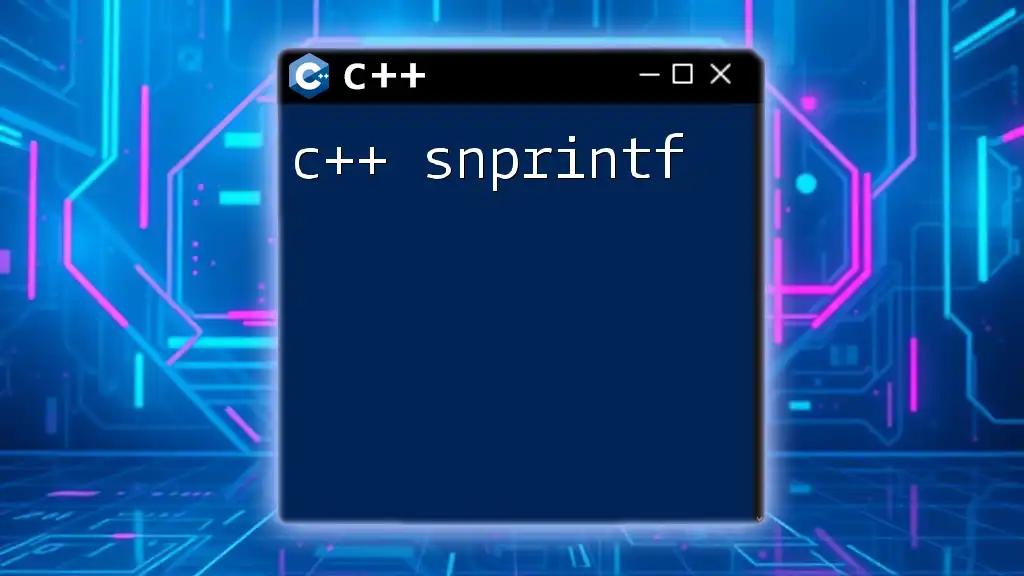
The Case for Using Both
Hybrid Approach
Many developers find success in a hybrid approach, integrating both C++ and Blueprints. This allows them to exploit the best of both worlds. Core mechanics can be built in C++ for performance, while user interface elements or level design can leverage Blueprints to simplify the workflow.
Example Scenario: Imagine a scenario where a game leverages C++ for intricate character movement and AI systems, but uses Blueprints for level design and triggers. In this instance, you have the performance and control that C++ offers while maintaining the ease and speed of development that Blueprints provide.
Best Practices for Integration
Integrating C++ with Blueprints effectively can lead to a more powerful development experience. To expose C++ functions to Blueprints, developers need to use specific macros.
Example Code Snippet for Function Exposure:
UFUNCTION(BlueprintCallable, Category = "MyFunctions")
void MyFunction();
In this code, `UFUNCTION` declares that `MyFunction` can be called from Blueprints, allowing designers to leverage C++ code without diving deep into programming.
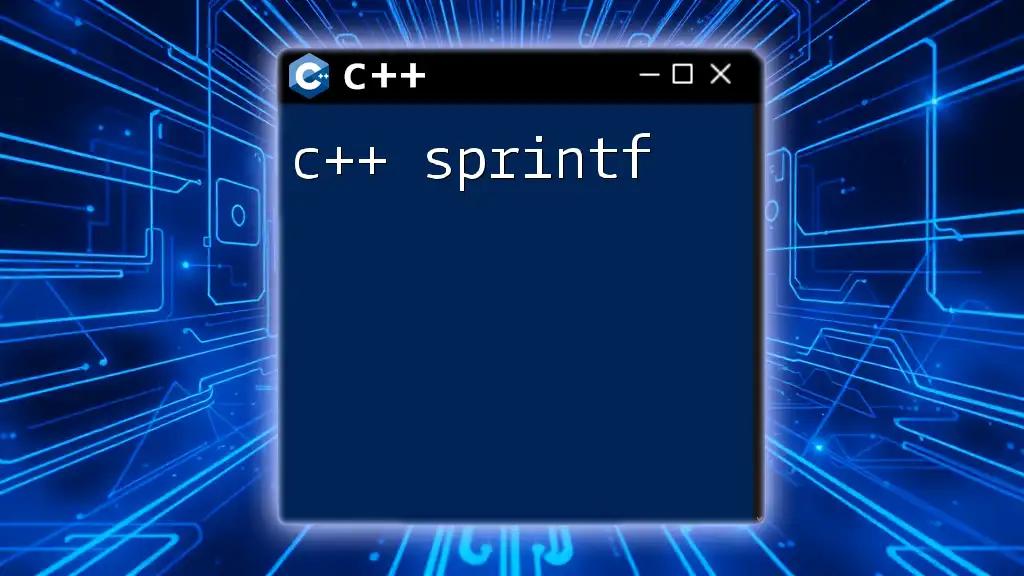
Blueprint vs C++: Pros and Cons
Advantages of Each
Advantages of C++
- Performance: C++ outperforms Blueprints, particularly in resource-intensive scenarios.
- Extensive Control and Flexibility: With C++, developers can craft custom classes and complex patterns.
Advantages of Blueprints
- Ease of Use for Beginners: The visual interface decreases the entry barrier for new developers.
- Faster Iteration and Visualization: Blueprints facilitate a rapid feedback loop that is invaluable for prototyping.
Disadvantages of Each
Disadvantages of C++
- Steeper Learning Curve: Learning C++ can be daunting for those unaccustomed to programming.
- Longer Development Time for Simple Tasks: Writing code, debugging, and compiling can slow down development, especially for straightforward tasks.
Disadvantages of Blueprints
- Potential Performance Overhead: For numerous complex interactions, Blueprints might introduce latency.
- Limited Control Over Memory Management: Developers have less granular control compared to C++.

Choosing Between C++ and Blueprints
Considerations for Beginners
For beginners, it’s crucial to understand your learning style and career objectives. If you aspire to be a technical designer or a programmer, starting with C++ might provide you with a strong foundation. However, if you’re more interested in level design or gameplay mechanics, starting with Blueprints could be advantageous.
Scenarios for Each
When to choose C++ over Blueprints: You’re developing a large-scale multiplayer game where performance and optimization are critical. C++ is likely the best choice.
When to choose Blueprints for rapid development: If you’re rapidly prototyping a mobile game or indie project where speed of delivery is more important than raw performance, Blueprints are often suitable.
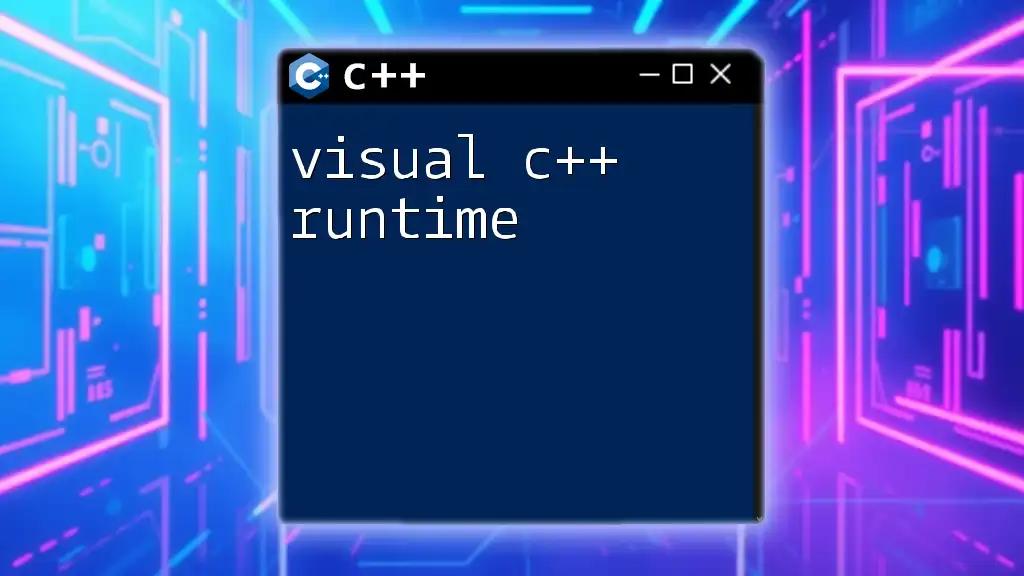
Conclusion
In navigating the terrain of unreal C++ vs blueprint, it's clear that both avenues have their advantages and disadvantages. C++ excels in performance and control, while Blueprints simplify the game development process with their visual scripting capabilities. A balanced skill set incorporating both strategies will empower developers to leverage the best tools available, allowing for greater creativity and efficiency in game development.
By embracing both C++ and Blueprints, you’ll not only enrich your skill set but also enhance your productivity in Unreal Engine. For more tutorials and resources to further your understanding, we invite you to explore our offerings.