The `#define` directive in C++ is used to create macros, allowing you to define constants or shorthand for frequently used expressions.
#define PI 3.14159
What is `#define`?
`#define` is a preprocessor directive in C++ that creates symbolic constants or macros. It allows developers to define constants or functions that can be used throughout their code without having to repeatedly specify the same value or expression. While `#define` is widely used, it is important to note that it operates at a textual level, which means that every instance of the defined identifier will be replaced by its corresponding value or expression before compilation.
It’s also crucial to differentiate between `#define` and other preprocessor directives like `#include`. While `#include` is used to include files into your program, `#define` is strictly about defining constants or macros for use in your code.
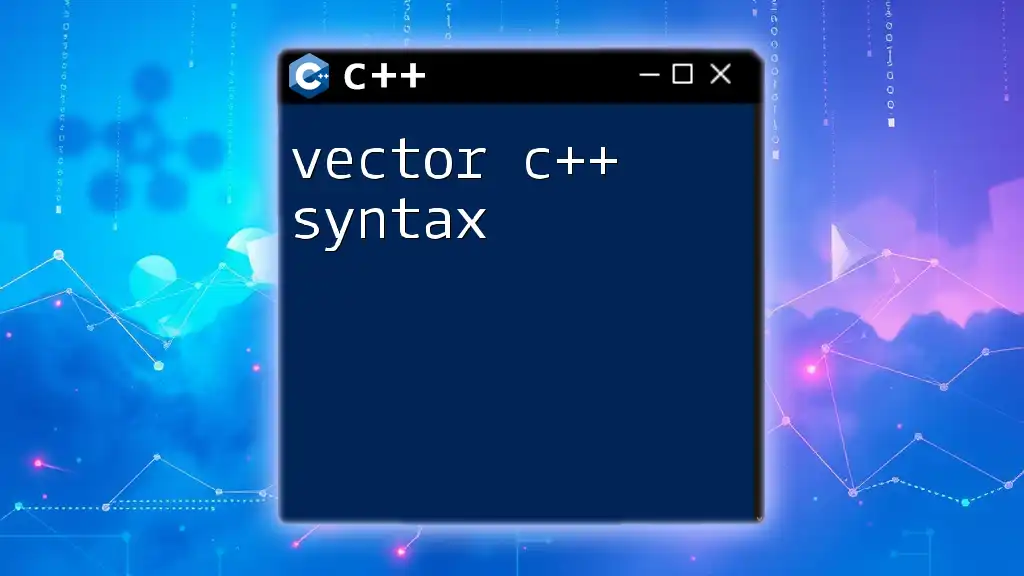
Syntax of `#define`
The basic syntax for the `#define` directive is straightforward:
#define identifier replacement
Here, `identifier` is the name you give to your macro or constant, and `replacement` is the value or expression you want to use in its place.
It’s essential to remember that identifiers defined with `#define` are case-sensitive. As for the `replacement`, it can be any expression, making `#define` versatile. Additionally, `#define` can also take arguments, creating function-like macros. A common practice is to name constants in uppercase letters to differentiate them from regular variables.
Let’s see an example of defining a constant:
#define PI 3.14
In the example above, every occurrence of `PI` in the code will be replaced by `3.14` during preprocessing.
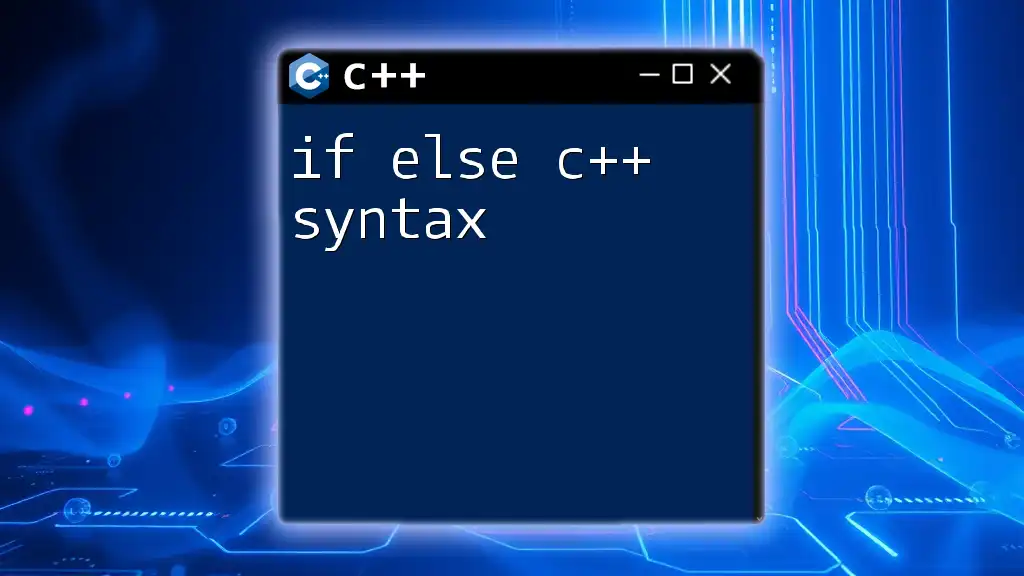
Types of Macros
Object-like Macros
Object-like macros are the simplest form of macros. They serve primarily as constants or variable values. By defining a constant, you eliminate magic numbers from your code, which aids clarity and ease of maintenance.
For example:
#define MAX_VALUE 100
This means that throughout your code, wherever you write `MAX_VALUE`, it will be replaced with `100`. This practice not only makes the code self-documenting but also makes it easy to change values if necessary.
Function-like Macros
Function-like macros are those that can take arguments, similar to functions. However, unlike functions, they are expanded inline, making them a powerful, albeit sometimes dangerous feature.
Here’s an example of a function-like macro:
#define SQUARE(x) ((x) * (x))
If you call `SQUARE(5)`, during preprocessing, it gets replaced with `((5) * (5))`, which evaluates to `25`.
While function-like macros can appear efficient, they carry potential pitfalls, such as multiple evaluations of arguments, leading to unexpected results:
int a = 5;
int result = SQUARE(a++); // a is increased twice!
In this scenario, `result` would not just be `36` but might yield `37` or more, as `a` is incremented more than once.
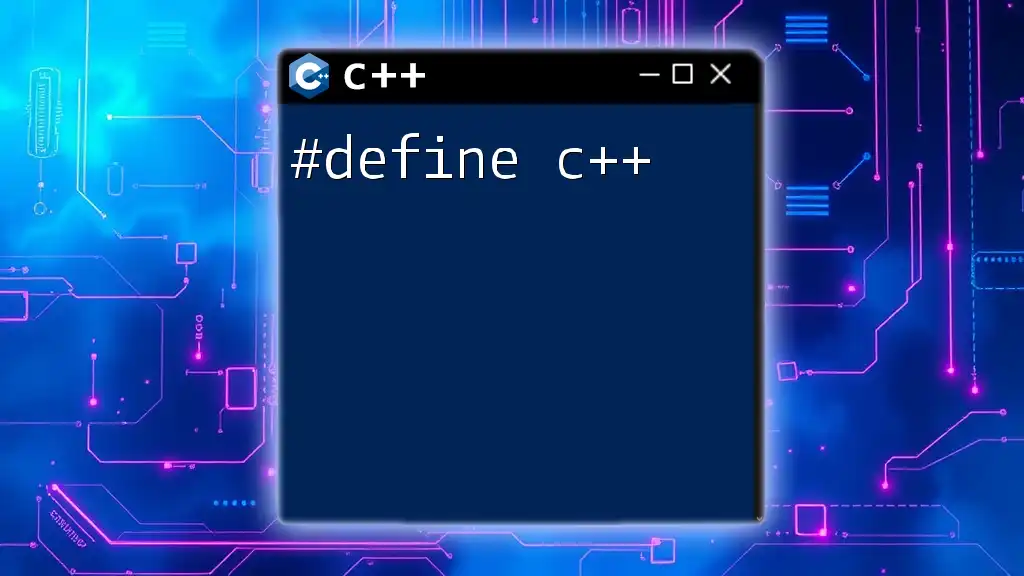
Advantages of Using `#define`
Using `#define` has several notable advantages:
-
Performance Improvement: Since `#define` operates at the preprocessing stage, macros can lead to faster compilation times compared to functions that require calls at runtime.
-
Simplification: `#define` allows programmers to manage constants and repeated expressions easily. Changing a single definition will automatically propagate throughout the codebase without requiring manual changes.
-
Code Clarity: When properly named, `#define` can make code clearer. For instance, using descriptive macro names can provide context that enhances understanding when reading or maintaining code.
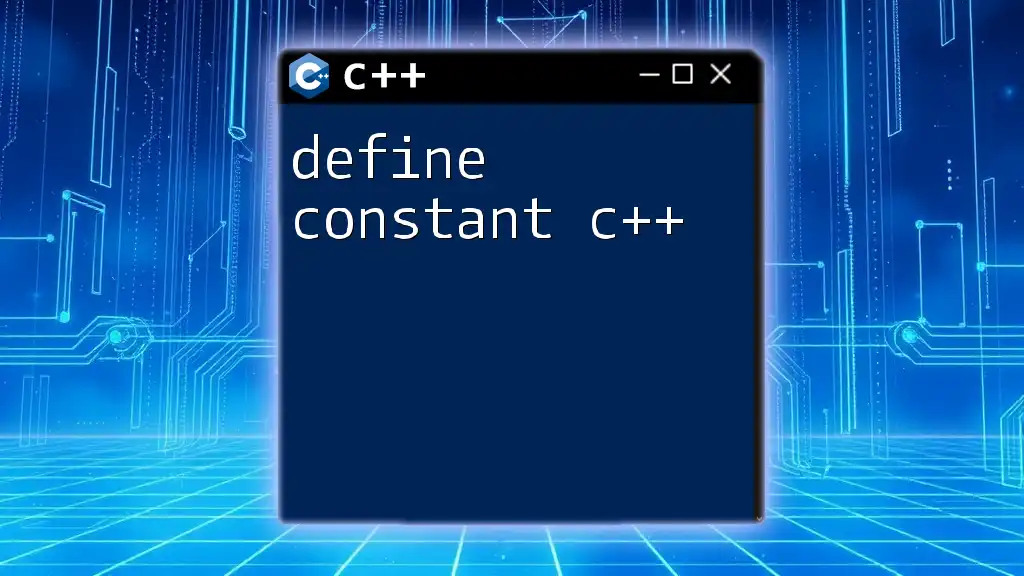
Disadvantages of Using `#define`
However, using `#define` is not without its disadvantages:
-
Lack of Type Safety: Macros do not have types, which means there’s no type checking during compilation, leading to potential runtime errors.
-
Debugging Difficulties: Debugging macros can be tricky because the errors may not point to the line where the macro is expanded.
-
Global Scope: Macros defined with `#define` exist globally and can lead to naming conflicts. It’s easy to inadvertently overwrite a macro, causing bugs that can be very subtle.
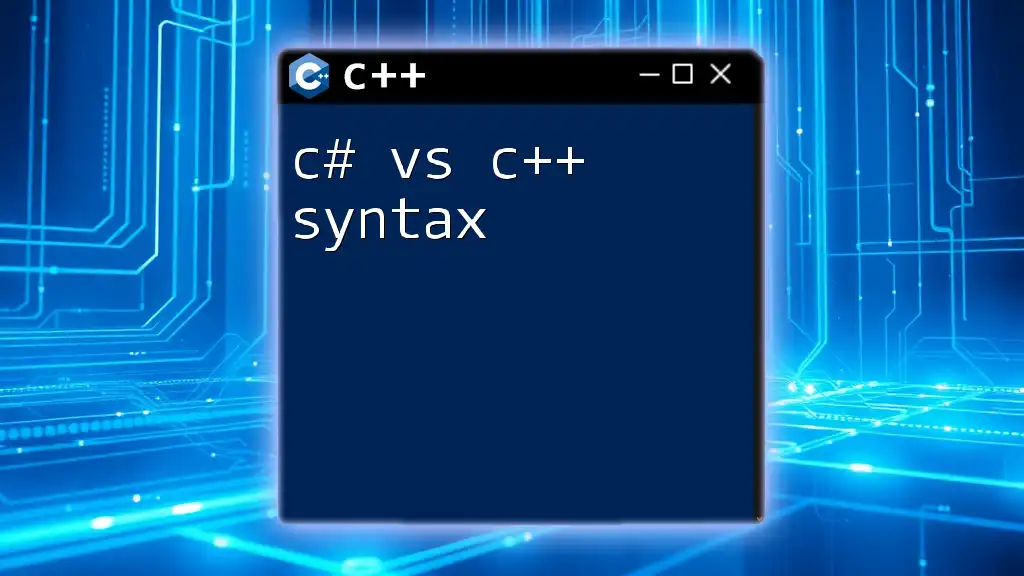
Best Practices When Using `#define`
To maximize the benefits and minimize pitfalls when using `#define`, consider the following best practices:
-
Namespacing: Ensure that your identifier names are unique to avoid conflicts. Some developers use prefixes (e.g., `MYLIB_MAX_VALUE`) to avoid name clashes.
-
Using Parentheses: Always wrap argument expressions in parentheses to avoid issues with operator precedence:
#define SQUARE(x) ((x) * (x))
- Alternative Approaches: In many cases, it is preferable to use `constexpr` or `inline` functions over `#define`, as these offer type safety, better tooling support, and scope management.
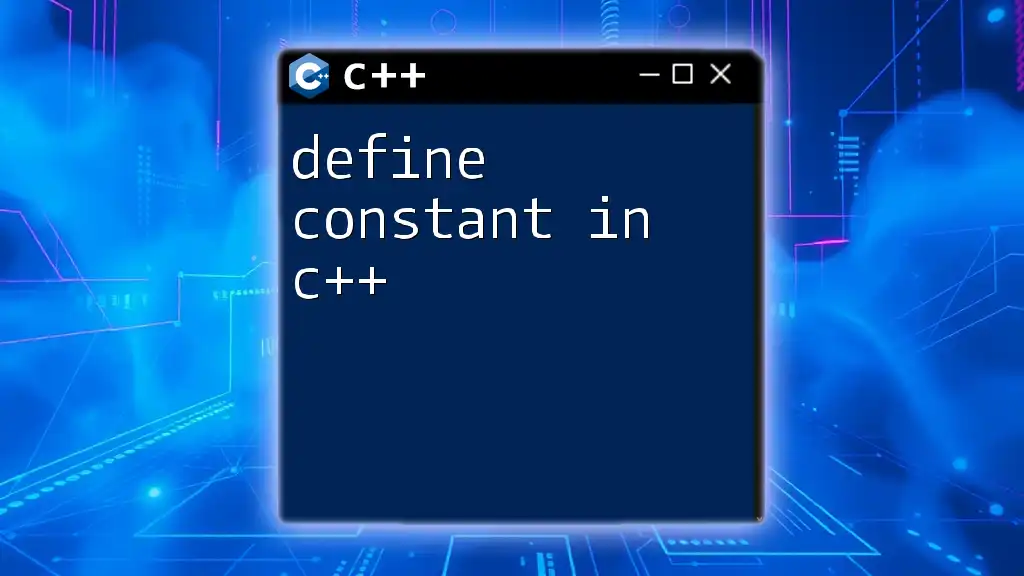
Examples of `#define` in Real-World Scenarios
Constants in Mathematical Calculations
Using `#define` can simplify mathematical computations. For instance, you might define the mathematical constant `E` for natural logarithms as follows:
#define E 2.71828
With this, you can use `E` throughout your code without repeatedly writing its value.
Conditional Compilation
`#define` can also play a crucial role in conditional compilation. It allows developers to enable or disable features based on the defined macro. This is especially useful for debugging or creating version-specific code.
Here’s an example of a debug feature toggle:
#define DEBUG
#ifdef DEBUG
std::cout << "Debugging Information" << std::endl;
#endif
In this case, if `DEBUG` is defined, the debugging information will be printed; if not, that section of the code is ignored, reducing unnecessary output in a production environment.
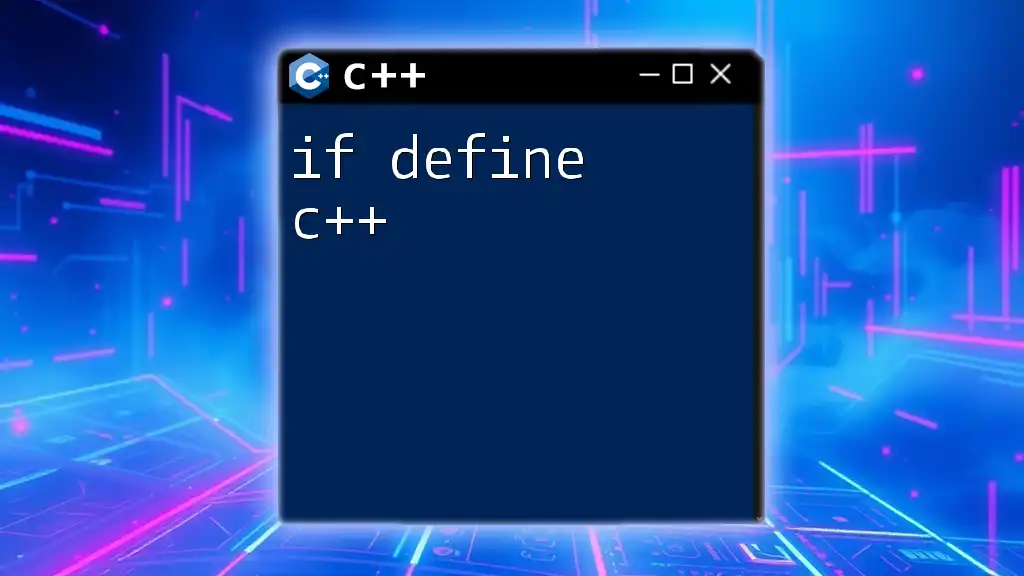
Conclusion
In summary, understanding `#define c++ syntax` is essential for any C++ programmer. Utilizing `#define` can simplify your coding practices, enhance readability, and foster a more organized development process. While it does come with its set of challenges, being mindful of its advantages, disadvantages, and best practices can significantly improve your programming efficiency.
Experimenting with `#define` in your own projects will deepen your understanding and aid in mastering its application. To stay updated on C++ best practices and tips, consider following dedicated C++ communities and forums.