C# syntax is designed to be more user-friendly and integrates seamlessly with the .NET framework, while C++ offers more flexibility and control over system resources, leading to differences in how variables are declared and functions are defined.
Here’s a simple code snippet comparing variable declaration in both languages:
// C++ syntax for declaring a variable
int number = 10;
// C# syntax for declaring a variable
int number = 10;
Understanding C# and C++
What is C++?
C++ is a general-purpose programming language that emphasizes performance and efficiency. Initially developed as an extension of the C programming language, C++ supports both object-oriented and procedural programming paradigms, making it a versatile choice for a wide range of applications. Its key features include low-level memory manipulation, which allows developers to interact directly with hardware, making it ideal for systems programming.
What is C#?
C#, on the other hand, was developed by Microsoft as part of the .NET framework. It is designed to be easy to use and highly productive, particularly in enterprise environments. C# is a strongly typed, object-oriented language that benefits from a rich library support, automatic memory management, and powerful development tools, all of which enhance developer productivity and software quality.
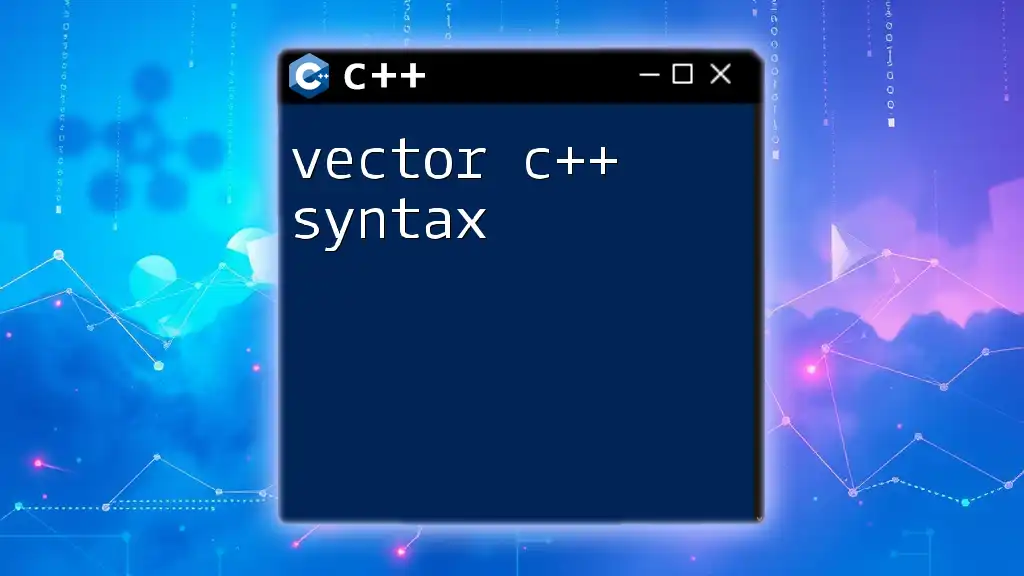
Differences in Syntax
Basic Syntax Comparisons
Declaration of Variables
In both C# and C++, variable declaration syntax is quite similar. You define a variable type, followed by the variable name. For example, in C++:
int age = 25;
In C#, you would use a similar syntax:
int age = 25;
The similarity here indicates that both languages support strong typing, which helps to catch errors at compile time.
Control Structures
If Statements
Control structures such as conditional statements largely resemble one another in both languages, yet with slight variations in how output is handled. In C++, the syntax looks like this:
if (age > 18) {
cout << "Adult";
}
In C#, it becomes:
if (age > 18) {
Console.WriteLine("Adult");
}
Here, `cout` is replaced by the more descriptive `Console.WriteLine`, demonstrating C#'s focus on readability and self-explanatory syntax.
Loops
The `for` loop also retains a similar structure between the two languages. For instance, a simple `for` loop in C++ appears as follows:
for (int i = 0; i < 10; i++) {
cout << i;
}
In comparison, the C# version is:
for (int i = 0; i < 10; i++) {
Console.WriteLine(i);
}
Both languages allow you to iterate over a sequence easily, reinforcing their comparable syntax.
Object-Oriented Concepts
Class Definition
Defining classes showcases how both languages support object-oriented programming. In C++, a class definition is structured like this:
class Dog {
public:
void bark() {
cout << "Woof!";
}
};
C# follows a similar approach, but the method and class setups look slightly more streamlined:
class Dog {
public void Bark() {
Console.WriteLine("Woof!");
}
}
Notably, C# promotes PascalCase naming for methods and classes, which adds to the clarity of the code.
Inheritance
Inheritance is another cornerstone of object-oriented programming, and both C++ and C# implement it effectively. A base class and derived class in C++ would look like this:
class Animal {
// Base class
};
class Dog : public Animal {
// Derived class
};
In C#, it is similarly structured, but without the `public` keyword preceding the base class:
class Animal {
// Base class
}
class Dog : Animal {
// Derived class
}
The syntax for inheritance remains consistent between the two languages, demonstrating how both leverage inheritance to extend functionality.
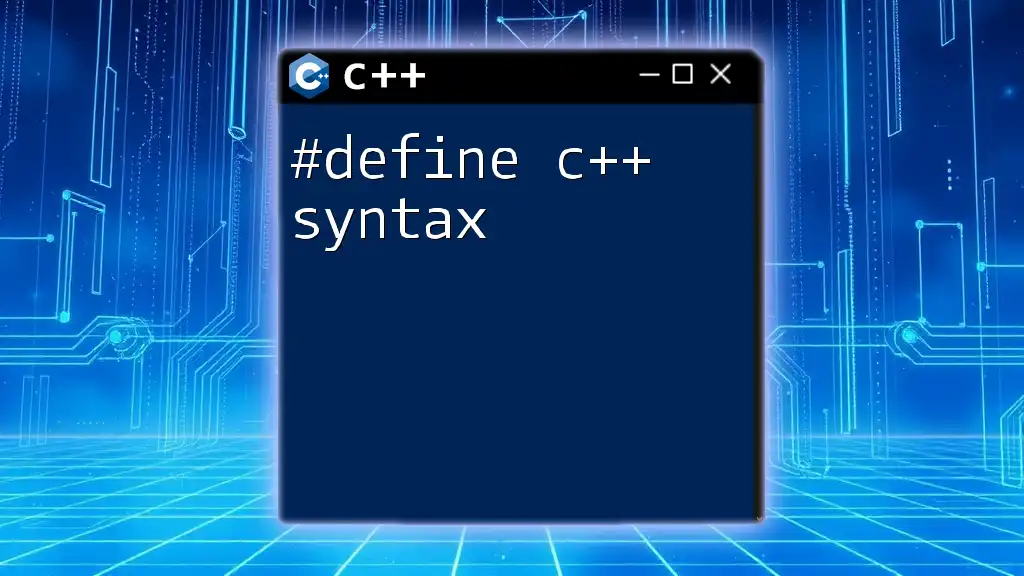
Memory Management
Pointers vs. References
Memory management displays one of the most significant differences between C++ and C#. C++ allows for direct memory manipulation through pointers, as illustrated here:
int* p = &age; // Pointer to age
In contrast, C# abstracts this aspect for safety; variables are typically passed by reference. Thus, you'd see something like:
int age = 25;
int reference = age; // A copy of the value
The absence of pointers in C# emphasizes its goal of being more secure and user-friendly while still supporting robust reference types for advanced programming.
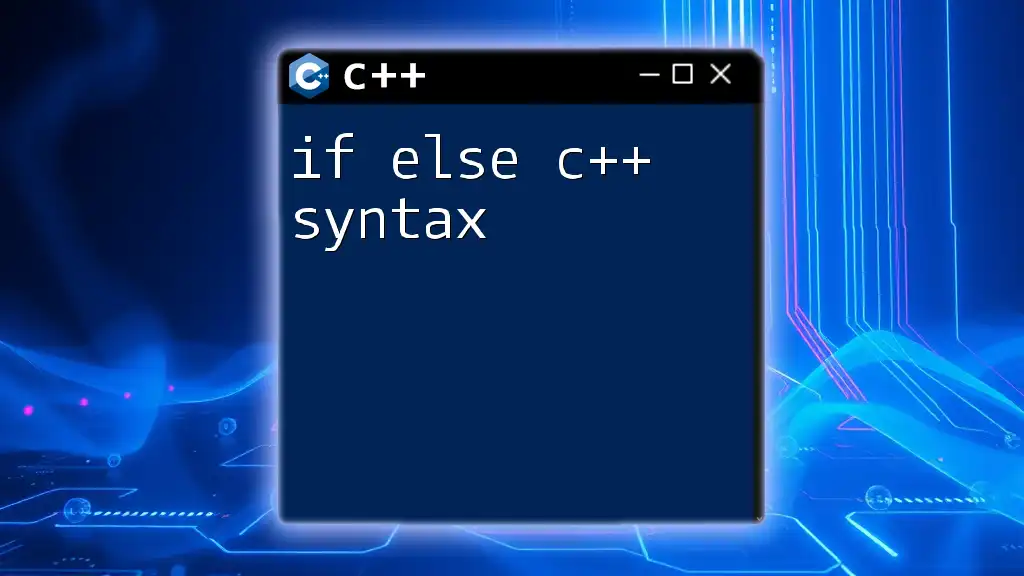
Error Handling
Exception Handling Syntax
Both languages include exception handling mechanisms, but their syntax varies slightly. In C++, exceptions are managed as follows:
try {
// Code block
} catch (exception& e) {
// Handle error
}
In C#:
try {
// Code block
} catch (Exception e) {
// Handle error
}
While both use a `try-catch` block for error management, C# leverages a more consistent naming convention with `Exception`, enhancing readability.
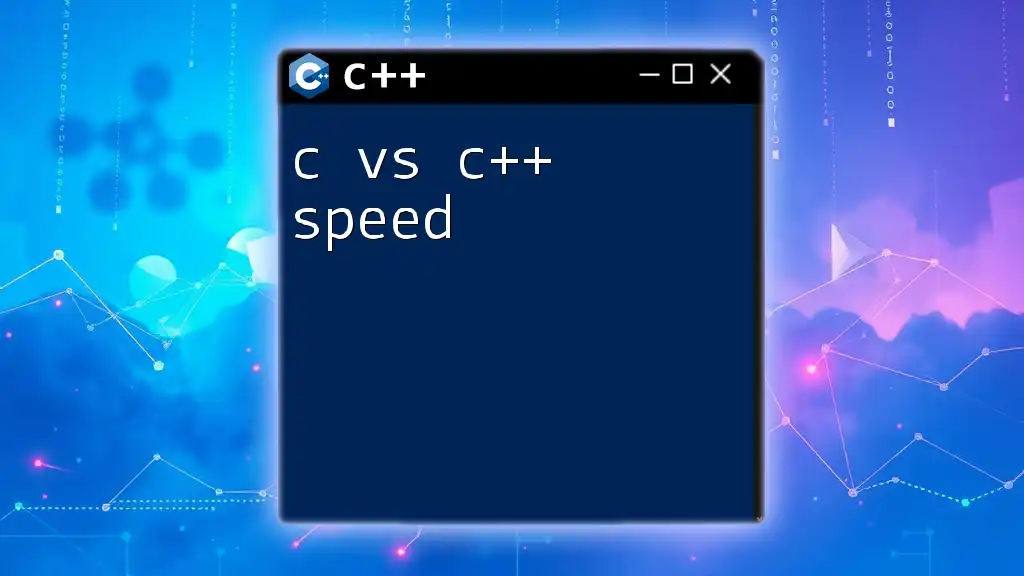
Performance and Use Cases
Performance Comparison
Performance is a notable differentiator in the C# vs C++ syntax discourse. C++ generally provides higher efficiency due to system-level access, allowing developers to optimize their applications right down to the hardware level. This makes it suitable for applications where performance is critical, such as game engines and real-time systems.
In contrast, C# operates within a managed environment (via the .NET framework), placing more emphasis on development speed and ease of use. Although it is typically slower than C++ due to its reliance on a Garbage Collector, the trade-off is often worthwhile for enterprise applications where development time is crucial.
Typical Use Cases
C++ is frequently used in scenarios demanding maximum performance. Common examples include:
- Game development
- Systems software
- Embedded systems
In contrast, C# shines in:
- Web applications
- Enterprise-level software development
- Game development using Unity
These contrasting use cases highlight the strengths that each language brings to different areas of software development.
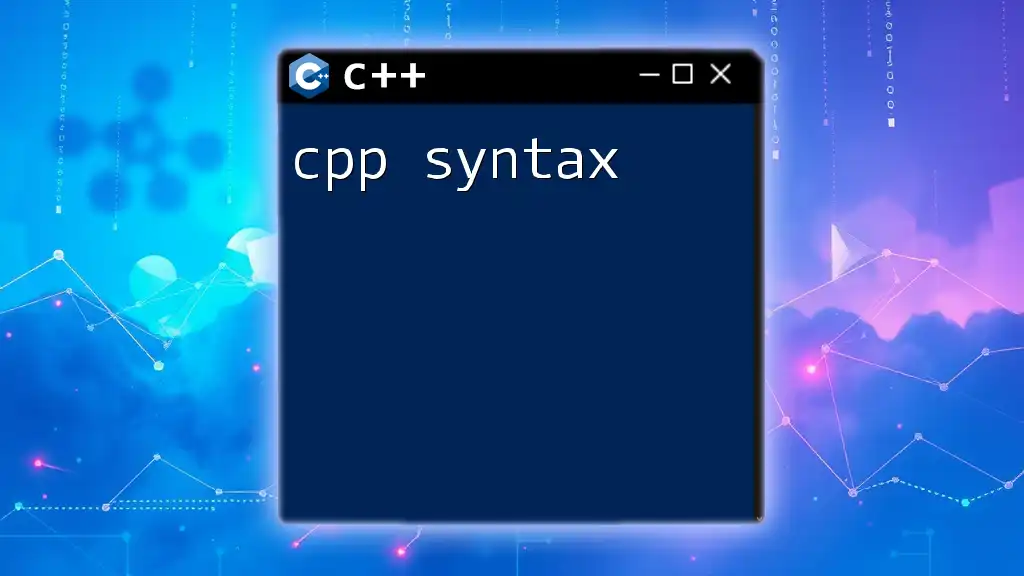
Conclusion
Through a detailed exploration of C# vs C++ syntax, we can see that while both languages share similarities, they cater to different programming paradigms and priorities. C++ provides granular control and efficiency, ideal for performance-intensive applications, while C# emphasizes readability, productivity, and automated memory management, making it more suitable for business applications.
Whether you're deciding to learn one or both, it's essential to understand their unique features and strengths to choose the right tool for your software development needs. Exploring both languages will open new avenues in your programming journey, enriching your skill set and adaptability in the ever-evolving tech landscape.