C# is a high-level, object-oriented programming language designed for rapid application development on the .NET framework, while C++ is a low-level, multi-paradigm programming language that offers greater control over system resources and performance.
Here's a simple code snippet in both languages highlighting a basic "Hello, World!" program:
// C++
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
// C#
using System;
class Program {
static void Main() {
Console.WriteLine("Hello, World!");
}
}
Background of C# and C++
Origins of C#
C# was developed by Microsoft as part of the .NET framework, introduced in the early 2000s. The language was designed with the goal of making application development easier and more efficient. C# is influenced by several programming languages such as C, C++, and Java. It offers modern programming features that enhance developer productivity and promote robust coding practices. Its emphasis on simplicity and usability makes it a popular choice for beginners and seasoned developers alike.
Origins of C++
C++ was created by Bjarne Stroustrup in the early 1980s as an extension of the C programming language. With a primary focus on object-oriented programming, C++ allows developers to write complex and high-performance applications. It was designed to be efficient and to provide more control over system resources compared to its predecessors. C++ has evolved significantly over the years and is widely used in system programming, game development, and performance-critical applications.
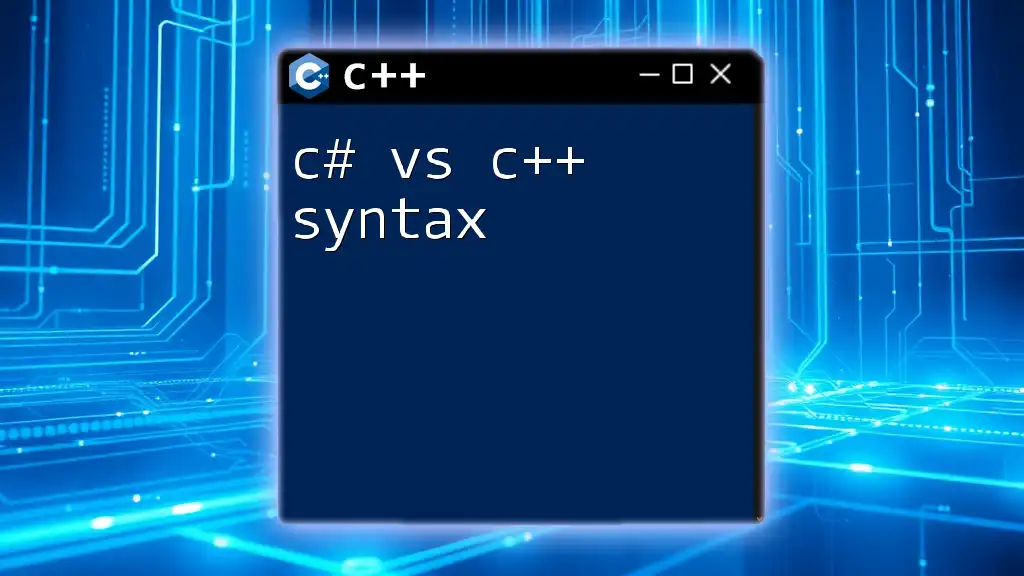
Core Differences Between C# and C++
Language Paradigms
One of the primary distinctions between C# and C++ lies in their language paradigms:
- C++ supports multiple paradigms, including procedural, object-oriented, and functional programming. This flexibility allows developers to choose the approach that best fits their project requirements.
- C#, on the other hand, primarily emphasizes object-oriented programming (OOP). While C# has introduced some functional programming features, its core design revolves around objects and classes, promoting encapsulation, inheritance, and polymorphism.
Memory Management
Memory management is a crucial aspect that separates C# and C++:
C++ Memory Management
In C++, memory management is primarily manual. Developers are responsible for allocating and deallocating memory, which allows for fine-grained control but also increases the likelihood of memory leaks.
For example, consider the following code snippet for dynamic memory allocation:
int* arr = new int[10]; // Allocate an array of 10 integers
delete[] arr; // Deallocate the memory once done
In this case, failing to call `delete` can lead to memory leaks, making robust error handling necessary.
C# Memory Management
C# utilizes automatic garbage collection, which simplifies memory management. The runtime automatically reclaims memory that is no longer in use, reducing the burden on developers and minimizing memory-related errors.
This can be particularly beneficial for projects needing rapid development and frequent updates, as developers can focus more on functionality rather than memory allocation details.

Syntax and Usability
Code Structure
Overall, C# and C++ share similarities in syntax, but there are distinct differences that can impact usability:
A simple "Hello World" example illustrates this contrast:
// C++ Hello World
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
// C# Hello World
using System;
class Program {
static void Main() {
Console.WriteLine("Hello, World!");
}
}
In C++, the syntax requires including headers like `<iostream>`, while C# uses namespaces (`using System;`) to incorporate libraries. Also, note that C# requires defining a class, whereas C++ allows procedural programming without requiring you to define a class for every function.
Error Handling
Error handling approaches in C++ and C# also vary significantly:
C++ Error Handling
In C++, error handling primarily employs exceptions and error codes. This gives developers flexibility but requires careful management of exceptions to avoid crashes. For instance:
try {
throw std::runtime_error("An error occurred");
}
catch (const std::runtime_error& e) {
std::cerr << e.what();
}
The above snippet demonstrates how to catch a runtime error and take appropriate action, emphasizing the need for robust exception handling practices.
C# Error Handling
C# simplifies error handling with a consistent try-catch-finally structure, making it easier to read and understand:
try {
throw new Exception("An error occurred");
}
catch (Exception e) {
Console.WriteLine(e.Message);
}
This clarity can significantly speed up development and debugging processes, as it is easier for developers to identify and address issues.
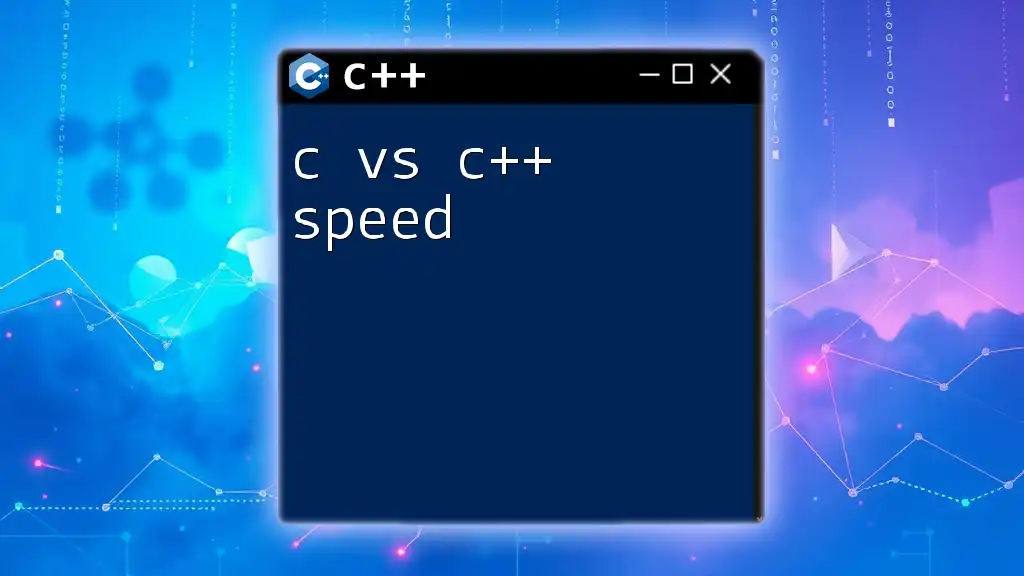
Performance and Efficiency
C++ Performance
C++ shines in performance-critical scenarios, such as system programming and game development. Low-level operations allow developers to interact directly with hardware, offering unparalleled control over performance optimizations. Applications that require high efficiency, such as real-time simulations or video games, often rely on C++ to maximize resource utilization.
C# Performance
C# operates within a managed environment, which sometimes leads to slower performance compared to C++. However, the .NET framework adds significant features and optimizations that can benefit enterprise applications. For example, C# applications can often be developed and deployed faster than their C++ counterparts, making it a suitable choice for web applications and business software where development speed is a priority.
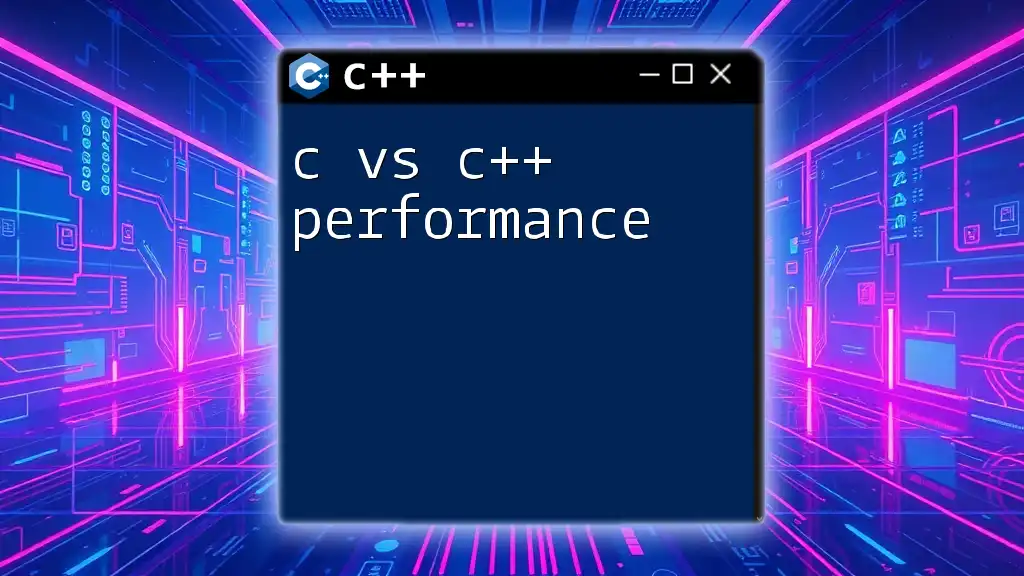
Libraries and Frameworks
C++ Libraries
C++ boasts a powerful Standard Template Library (STL), providing data structures and algorithms that facilitate efficient coding. The STL includes templates for vectors, lists, maps, and other data types, empowering developers to write reusable code. Here’s an example of creating a vector:
#include <vector>
std::vector<int> numbers = {1, 2, 3, 4, 5};
With these powerful libraries at their disposal, developers can focus more on algorithms and applications rather than reinventing data structures.
C# Libraries
C# benefits from the extensive .NET framework, which encompasses a vast array of libraries that extend functionality. The framework supports everything from web development (ASP.NET) to data access (Entity Framework). An example of using a generic list in C# is as follows:
using System.Collections.Generic;
List<int> numbers = new List<int> {1, 2, 3, 4, 5};
The .NET framework's built-in libraries not only provide tools for development but also promote best practices through established patterns and conventions.
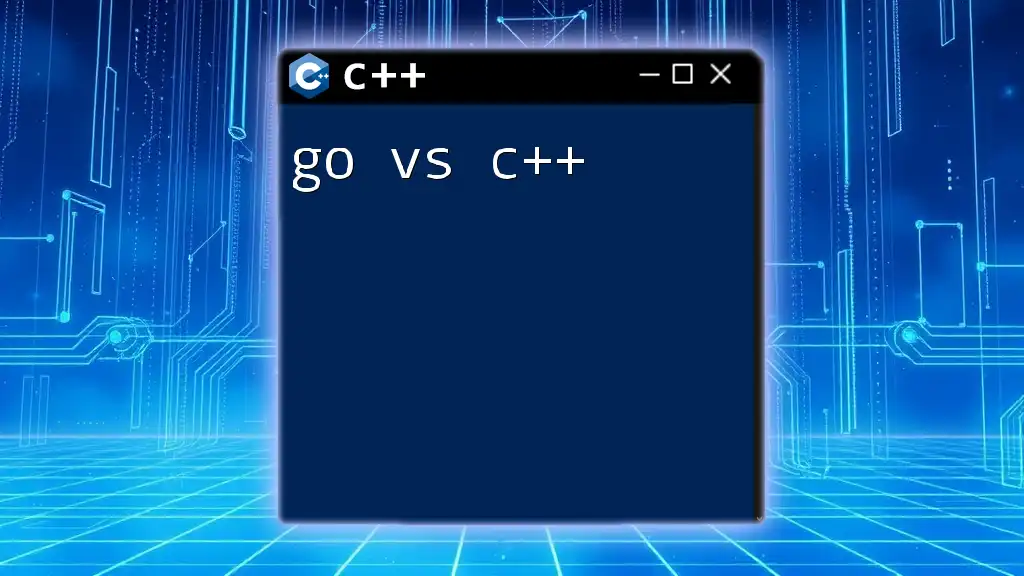
Use Cases and Applications
When to Use C++
C++ is ideal for applications requiring extensive performance optimizations, such as video games, graphic engines, and system-level software. If your project involves lower-level programming with a focus on resource management, C++ may be the superior choice.
When to Use C#
Conversely, C# excels in environments that benefit from rapid development and a plethora of built-in frameworks. If the project targets enterprise applications, web services, or mobile applications using Xamarin, C# typically presents a more efficient route due to its developer-friendly architecture.
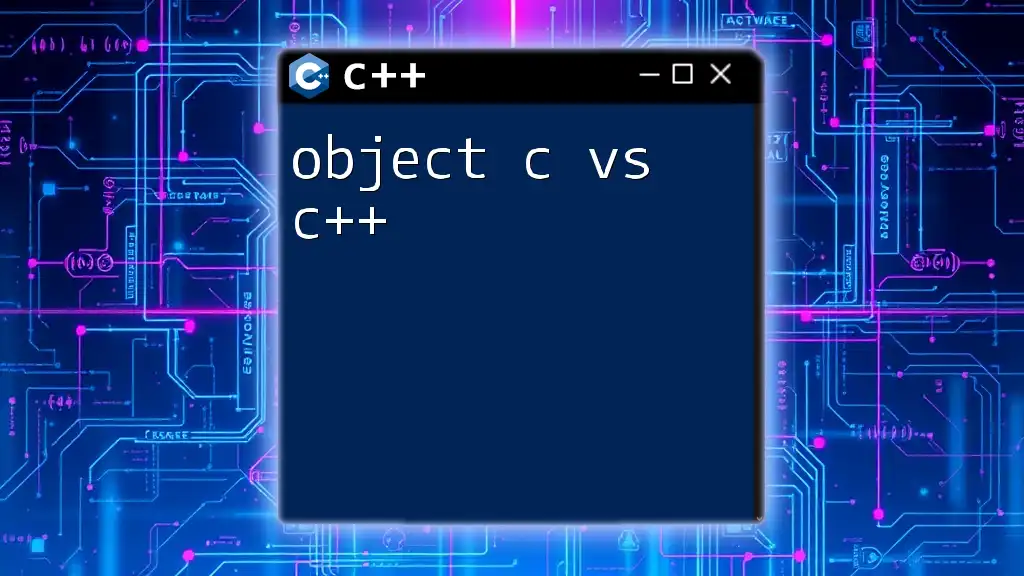
Conclusion
In summary, both C# and C++ are powerful programming languages tailored for different scenarios. C++ offers unparalleled performance and control, making it suitable for resource-intensive applications, while C# emphasizes productivity and ease of use, ideal for rapid application development. Understanding the differences between "C# vs C++" is critical for choosing the right tool for your specific project needs. Whether you are a novice developer or a seasoned professional, both languages present unique opportunities and challenges that can significantly influence your programming journey.
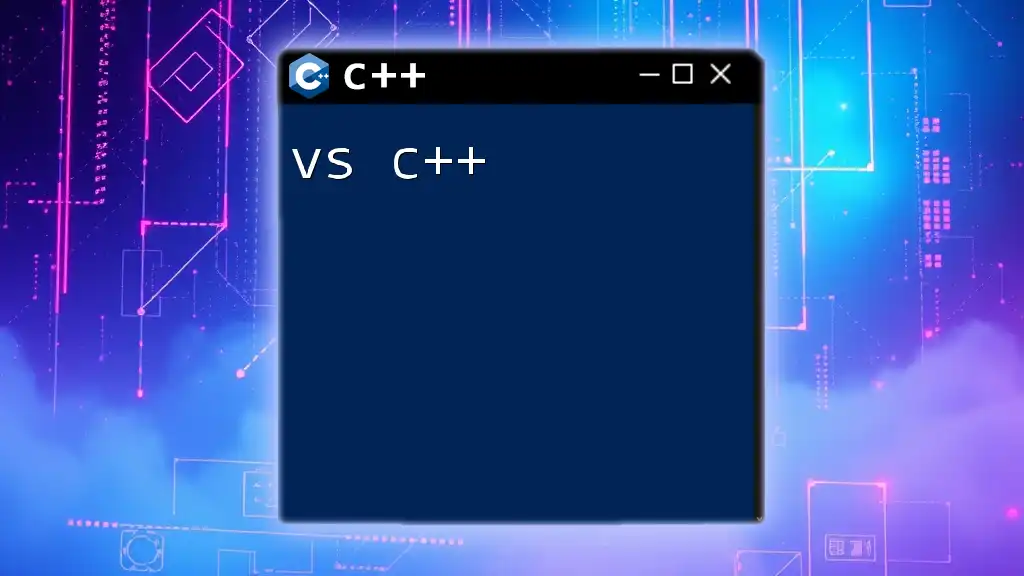
Call to Action
What are your experiences with C# and C++? Share your thoughts in the comments below! If you found this article useful, don’t forget to subscribe to our blog for more insights and guides on programming languages. Happy coding!