Fortran, primarily used in scientific computing for its performance with numerical data, contrasts with C++, which offers a more versatile object-oriented approach, allowing for better code organization and modularity.
Here’s a simple comparison of a "Hello, World!" program in both languages:
Fortran:
program hello
print *, 'Hello, World!'
end program hello
C++:
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl;
return 0;
}
History of Fortran and C++
Background of Fortran
Fortran, short for "Formula Translation," is one of the oldest programming languages, developed in the 1950s. It was specifically designed for scientific and engineering calculations, quickly becoming the language of choice for high-performance computing. The language has evolved through several versions — from Fortran 77, known for its procedural capabilities, to later standards like Fortran 90, 95, and the more modern 2003 and 2008 iterations.
Each version introduced new features aimed at improving readability, usability, and performance. Fortran 90 brought free-form syntax and constructs for modular programs, while Fortran 2003 added object-oriented programming capabilities.
Background of C++
Developed in the early 1980s by Bjarne Stroustrup, C++ emerged as an enhancement of the C programming language. It added features such as classes and inheritance, enabling developers to write object-oriented code, which is particularly useful in complex software systems. C++ has since become a cornerstone in various fields, including system/software development, game programming, and real-time simulations, thanks to its flexibility and performance.
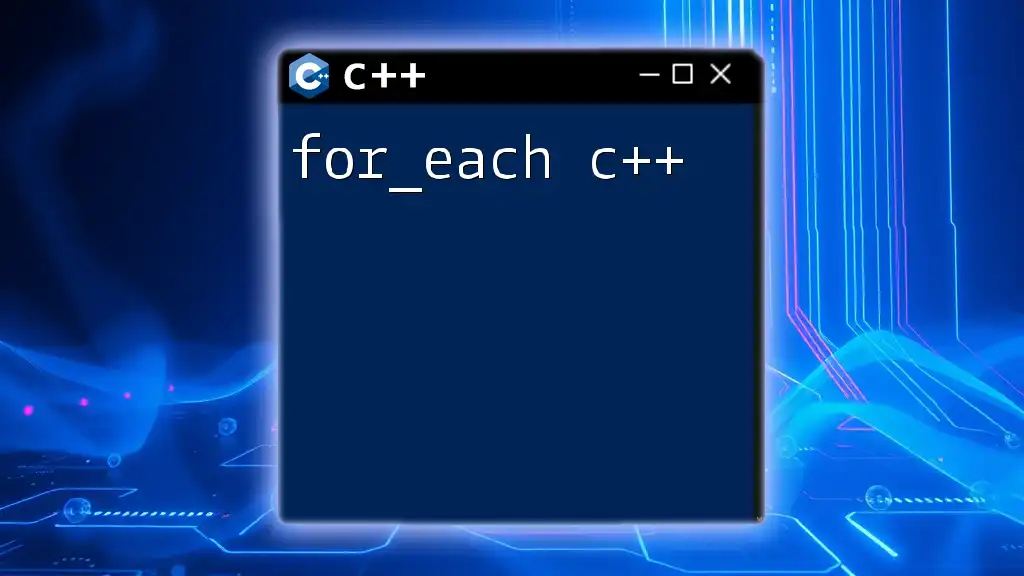
Language Syntax and Structure
Fortran Syntax
Fortran's syntax is unique, with a focus on mathematical expressions. Its structure emphasizes readability and ease of use for scientific applications. Here’s a simple Fortran program that outputs a greeting:
program hello
print *, "Hello, World!"
end program hello
In this snippet, the `print` statement is used to output text to the console, showcasing how straightforward Fortran aims to be.
C++ Syntax
C++ incorporates both procedural and object-oriented programming features, allowing for a balanced approach in solving various programming challenges. Below is an example of a simple C++ program:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, World!" << endl;
return 0;
}
This code snippet highlights the use of libraries (like `iostream`), namespaces (`std`), and the syntax structure typical of C++. The `cout` object is used for outputting data, similar to Fortran's `print`.
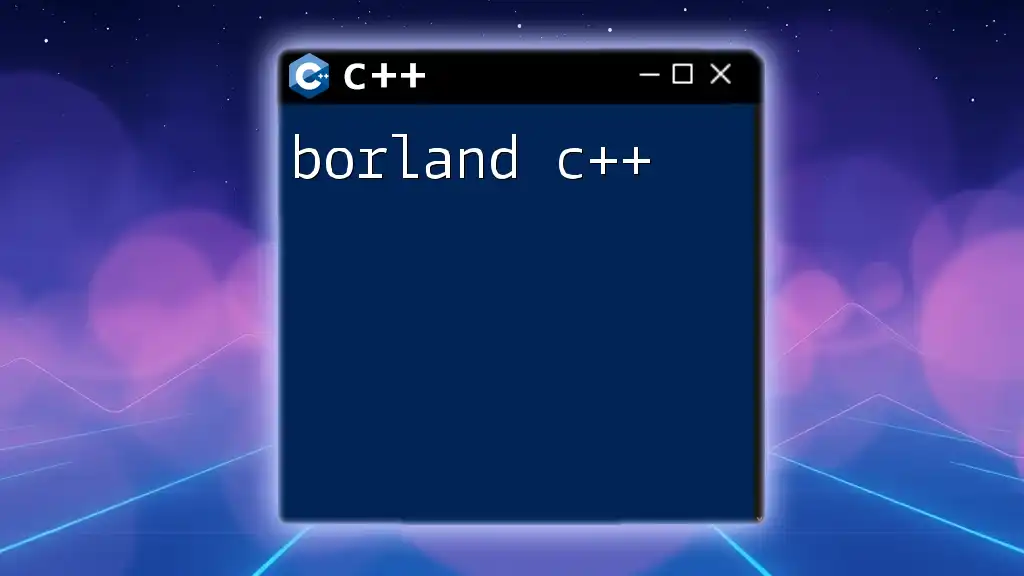
Paradigms and Approaches
Procedural Programming in Fortran
Fortran primarily follows a procedural programming paradigm, allowing developers to write subroutines and functions for code reuse. Subroutines enable modular design, making it easy to handle complex calculations in manageable chunks. For instance, below is a simple function definition in Fortran to calculate the square of a number:
function square(x)
real :: square
real :: x
square = x * x
end function square
Object-Oriented Programming in C++
C++ shines with its support for object-oriented programming (OOP), encompassing concepts like encapsulation, inheritance, and polymorphism. This allows for more structured code that can be easily maintained and reused. Here’s a C++ example demonstrating these OOP principles:
class Animal {
public:
virtual void sound() { cout << "Some sound" << endl; }
};
class Dog : public Animal {
public:
void sound() override { cout << "Bark" << endl; }
};
In this example, the `Animal` class serves as a base class, and `Dog` derives from it, overriding the `sound` method. This demonstrates how C++ facilitates designing flexible and extensible systems.
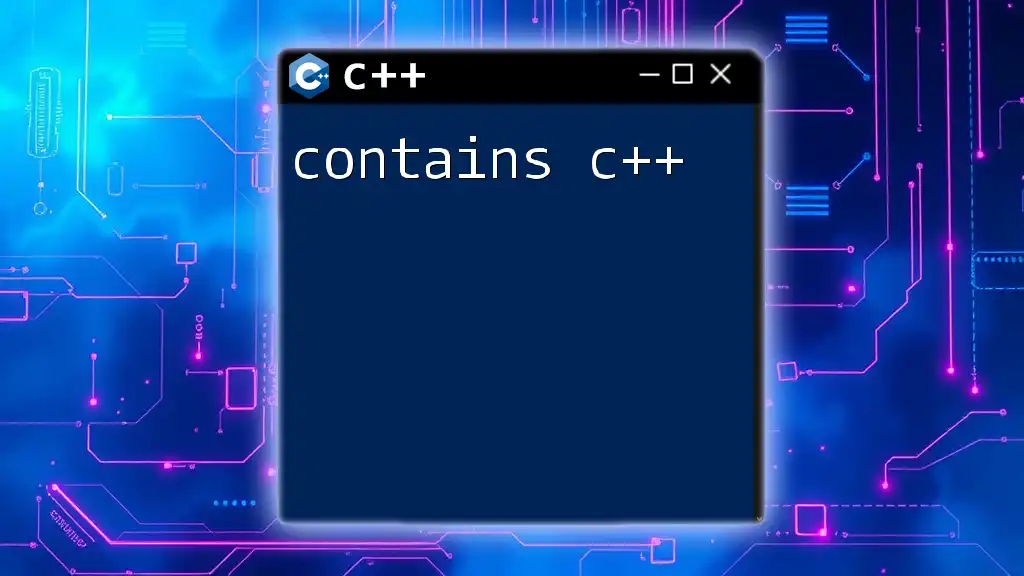
Performance and Efficiency
Fortran's Performance in Numerical Computing
Fortran is renowned for its performance in numerical computing. Due to its long history in scientific computing, it has been heavily optimized for operations involving floating-point arithmetic. As an example, many scientific computation benchmarks show Fortran's advantages when handling large arrays and matrix operations, often outperforming other languages due to its optimizations.
C++ and Performance Optimization Techniques
C++ also offers superior performance, but it requires developers to be more proactive about optimization. Techniques such as using inline functions and templates can significantly enhance run-time efficiency. Below is an example of an inline function defined with templates:
template<typename T>
inline T add(T a, T b) {
return a + b;
}
This function optimizes performance by reducing the overhead associated with function calls, showcasing how developers can leverage the language's capabilities for high-performance applications.
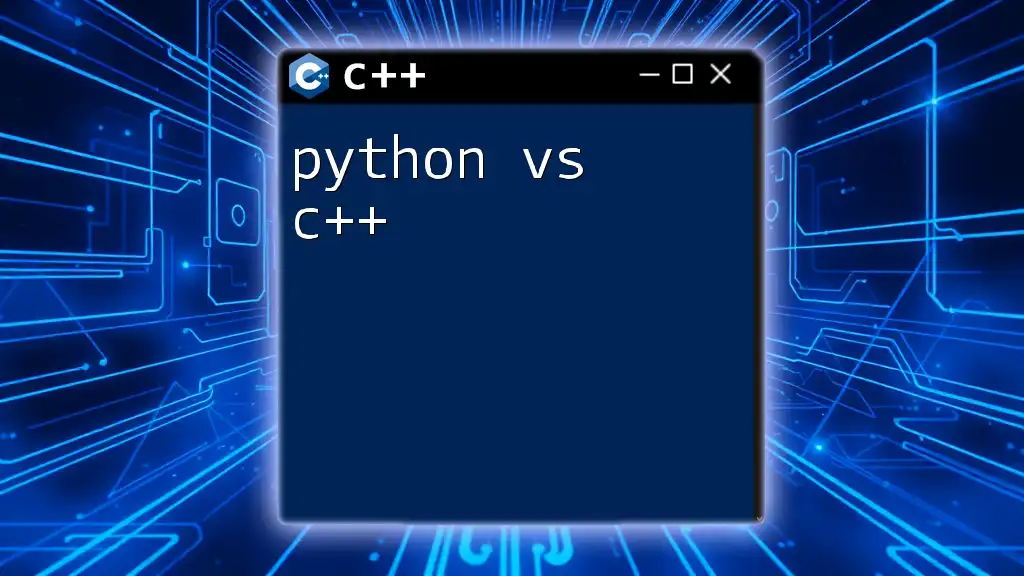
Standard Libraries and Ecosystem
Libraries and Frameworks in Fortran
Fortran has a robust ecosystem, particularly in scientific computing. Libraries such as LAPACK and BLAS offer powerful routines for linear algebra and other mathematical operations. While the community is not as large as that of C++, it still provides ample resources for learners and professionals alike.
Libraries and Frameworks in C++
C++ benefits from one of the most extensive collections of libraries and frameworks available in programming today. The Standard Template Library (STL) provides collections of data structures and algorithms that are both efficient and easy to use. Additionally, libraries like Boost offer additional utilities that can simplify tasks across various domains. The wealth of resources available makes C++ a versatile choice for developers.
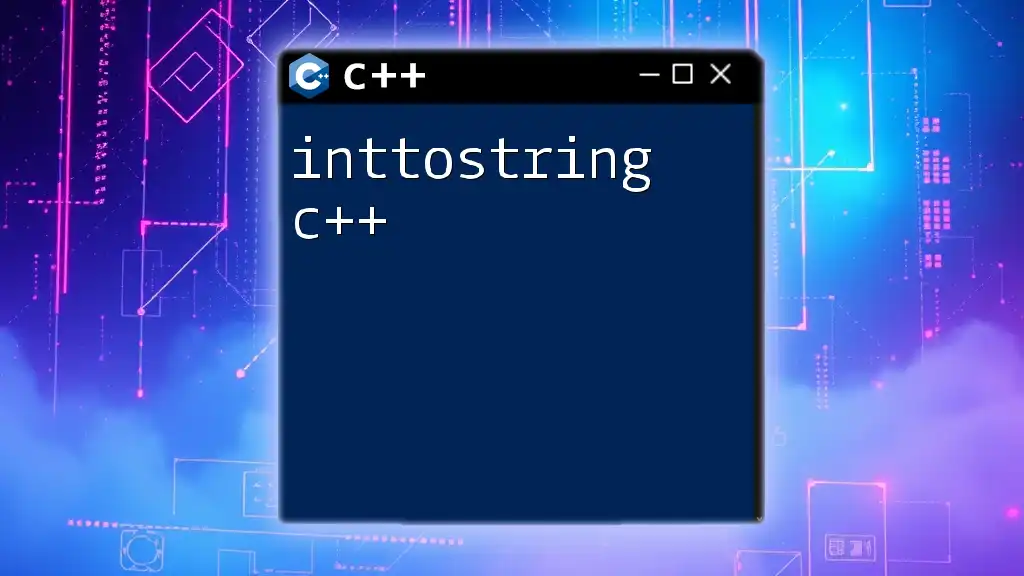
Use Cases and Applications
Typical Use Cases for Fortran
Fortran is still predominantly used in fields that rely heavily on numerical computations. Typical applications include:
- Weather modeling and simulation
- Computational fluid dynamics
- Scientific research in physics and chemistry
Its specialized capabilities make it the go-to language for high-performance calculations required in these domains.
Typical Use Cases for C++
C++ is extensively used in various realms, including:
- Game development, leveraging its performance for real-time graphics
- System and application software that require direct hardware manipulation
- Real-time systems, where time efficiency is critical
The adaptability of C++ makes it friendly for both new programmers and experienced developers tackling complex software challenges.
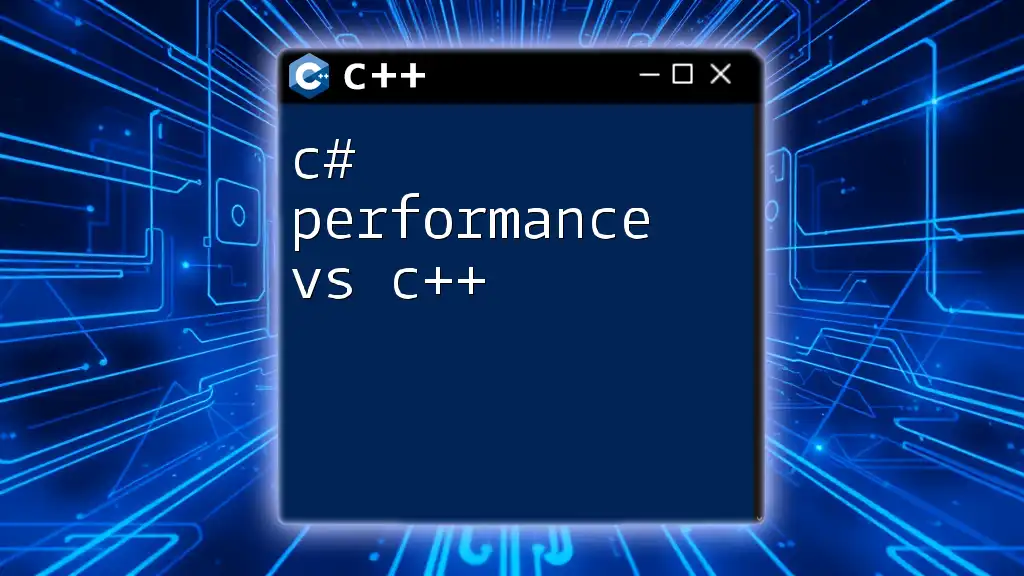
Community and Support
Fortran Community and Resources
While the Fortran community may be more niche, it includes dedicated forums, mailing lists, and literature vital for those interested in the language. Notable resources like the Fortran Wiki and community discussions can offer assistance and foster learning.
C++ Community and Support
In contrast, the C++ community is vast and active, with platforms like Stack Overflow and Reddit providing arenas to discuss problems and share solutions. Resources like cplusplus.com and cppreference.com are invaluable for both beginners and seasoned developers looking for quick references.
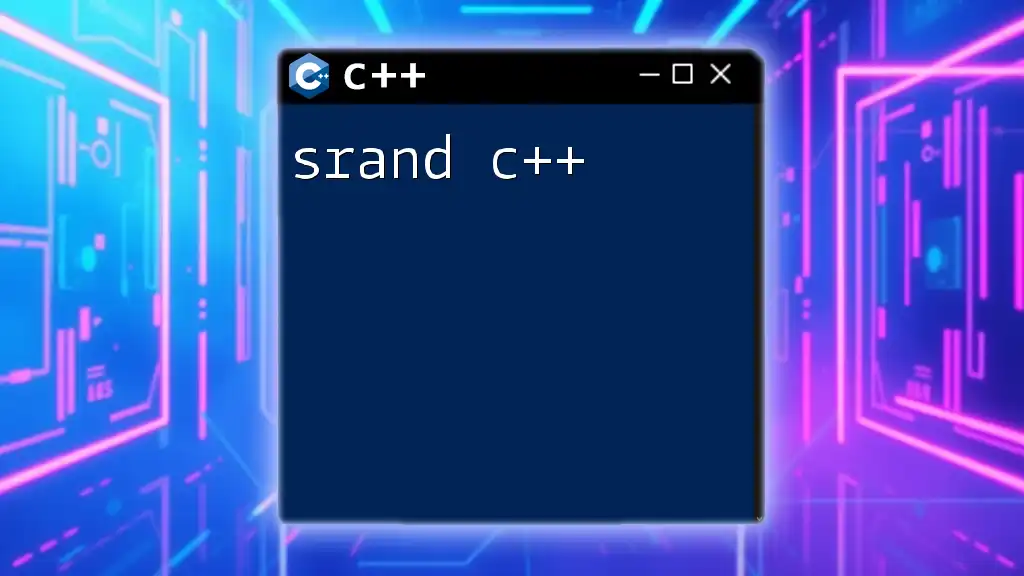
Conclusion
In summary, the "Fortran vs C++" debate often leads to a deeper understanding of the requirements for specific projects. Fortran may excel in numerical computing and scientific applications, while C++ provides versatility across different domains through object-oriented principles and extensive libraries. Choosing the right language depends on your goals, project requirements, and familiarity with the language features.
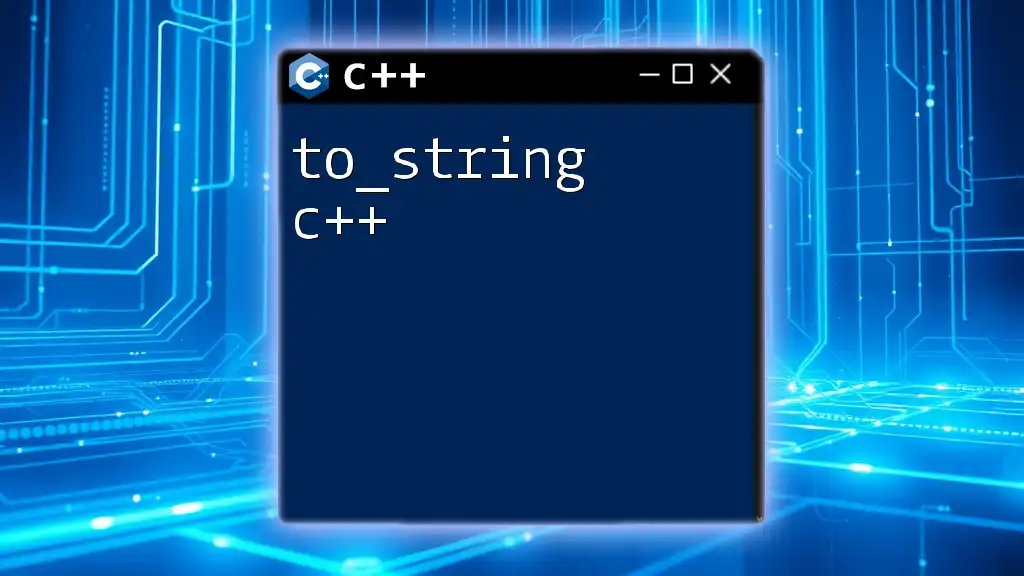
Additional Resources
If you’re interested in diving deeper into either language, consider exploring educational books, online tutorials, and courses. Engaging with community forums can also enhance your learning experience and provide real-time help from peers.
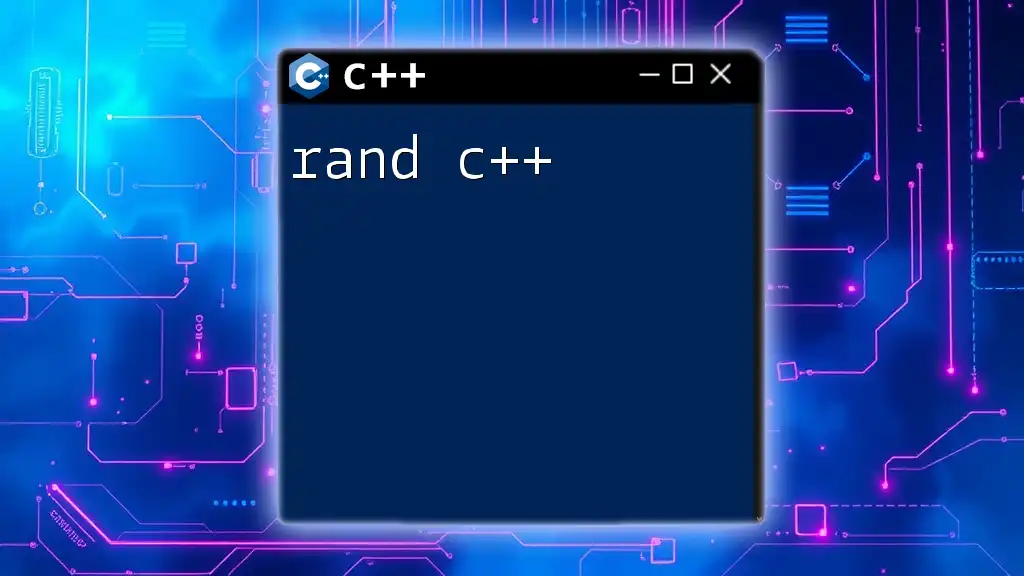
Call to Action
If you're eager to master C++ commands quickly and concisely, connect with us. Our training programs focus on brevity and clarity, making it easy for you to become proficient in C++ programming!