NetBeans is an integrated development environment (IDE) that provides tools for developing C++ applications, allowing programmers to write, compile, and debug their code efficiently.
#include <iostream>
int main() {
std::cout << "Hello, NetBeans C++!" << std::endl;
return 0;
}
Installing NetBeans for C++ Development
System Requirements
Before diving into the installation, it’s essential to ensure that your environment meets the necessary specifications.
Hardware Requirements:
To effectively run NetBeans for C++ development, your machine should have:
- A processor with at least a dual-core.
- A minimum of 4 GB of RAM (8 GB recommended).
- At least 1 GB of available hard disk space to accommodate the IDE and additional libraries.
Software Requirements:
You will also need:
- A compatible operating system (Windows, macOS, or Linux).
- Java Development Kit (JDK) since NetBeans is Java-based; the JDK version should be compatible with your NetBeans IDE version.
Downloading and Installing NetBeans
Step-by-Step Installation Guide:
To get started with NetBeans for C++, follow these steps:
- Visit the official NetBeans download page.
- Select the version compatible with your operating system.
- Download the installer and run it.
- Follow the on-screen instructions to complete the installation.
Installing on Different Operating Systems:
- Windows: Simply run the installer .exe file, agreeing to the terms and choosing your installation path.
- macOS: Open the downloaded .dmg file and drag the NetBeans icon to your Applications folder.
- Linux: Execute the downloaded .sh file in the terminal with necessary permissions.
Configuring C++ Development Environment
Setting Up the C++ Compiler
To compile and run C++ code effectively, you need a C++ compiler. Popular choices include GCC or MinGW for Windows users:
- GCC (GNU Compiler Collection): It's widely used and works well on Unix/Linux systems. Follow online guides to install it.
- MinGW: A minimalist version tailored for Windows. You can download it from the MinGW website.
Configuring NetBeans
Once the compiler is installed, launch NetBeans and configure it to use the compiler:
- Open Tools from the menu bar.
- Navigate to Options, then click on the C/C++ tab.
- Specify the paths to your compiler and debugger.
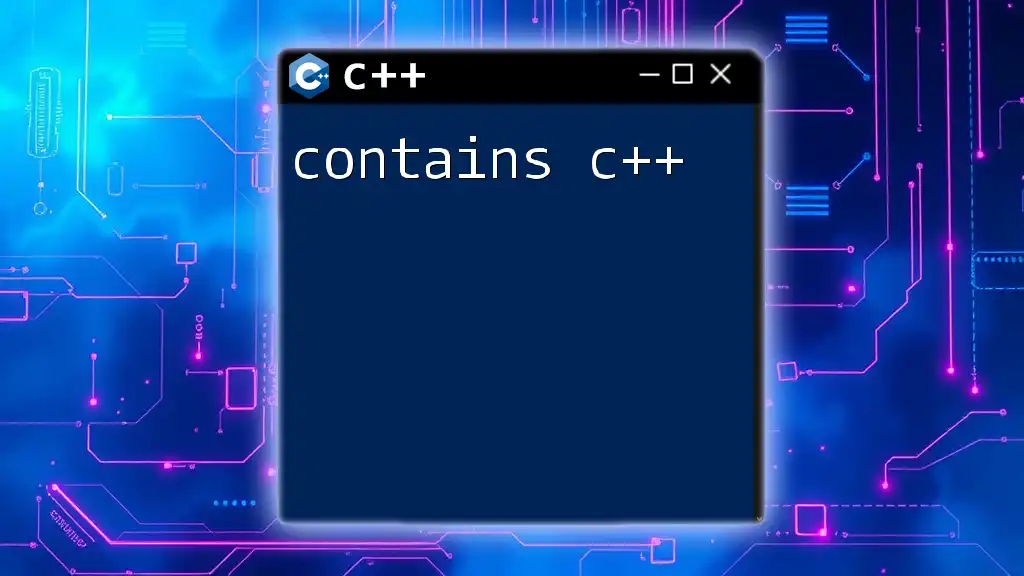
Creating Your First C++ Project in NetBeans
Starting a New Project
To begin writing C++ code, you must create a new project. Here's how:
- Launch NetBeans and select File > New Project.
- In the project wizard, choose C/C++ as the project category.
- Select C/C++ Application and click Next.
- Name your project and specify the location to save it, then click Finish.
Writing Your First Program
Now that your project is set up, it’s time to write your first C++ program.
Example Code Snippet
In the newly created project, navigate to the Main.cpp file and replace its content with the following code:
#include <iostream>
using namespace std;
int main() {
cout << "Hello, NetBeans!" << endl;
return 0;
}
Breaking Down the Code:
This simple program introduces basic C++ syntax:
- `#include <iostream>` allows you to use standard input and output streams.
- `using namespace std;` enables access to standard library names without prefixing them with `std::`.
- In `main()`, `cout << "Hello, NetBeans!" << endl;` outputs the text to the terminal, and `return 0;` indicates successful execution.
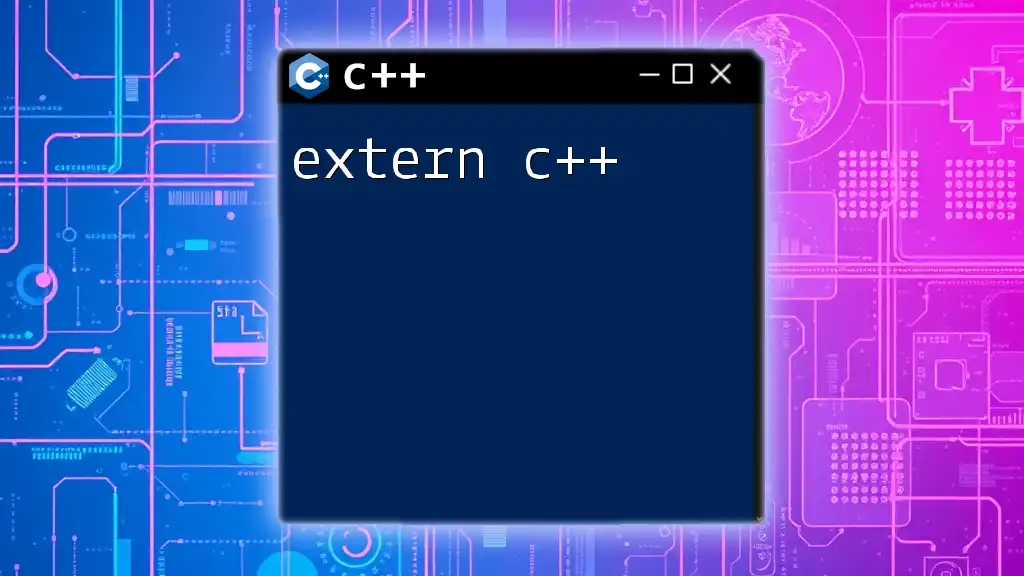
Navigating the NetBeans Interface
Key Features of the IDE
NetBeans offers several features to enhance your development experience.
Project Explorer:
The Project Explorer displays your project structure, making file navigation intuitive. You can expand folders to access headers, source files, and resources.
Code Editor:
The built-in code editor provides functionalities such as:
- Syntax Highlighting: Different colors for keywords, variables, and syntax, enhancing readability.
- Auto-completion: Suggestions for coding help, which saves time and reduces errors.
Output Window:
The Output Window is crucial for viewing messages, errors, and program output during execution. It helps you track your program's behavior in real time.
Toolbars and Shortcuts
Utilizing toolbars and keyboard shortcuts can significantly improve productivity. Here are some key aspects:
Commonly Used Toolbars:
- Build Toolbar: Contains buttons for compiling and building projects.
- Run Toolbar: Offers options to execute your program directly.
Keyboard Shortcuts:
Familiarizing yourself with shortcuts can streamline your workflow:
- F6: Builds and runs the project.
- Ctrl + Space: Triggers code completion suggestions.
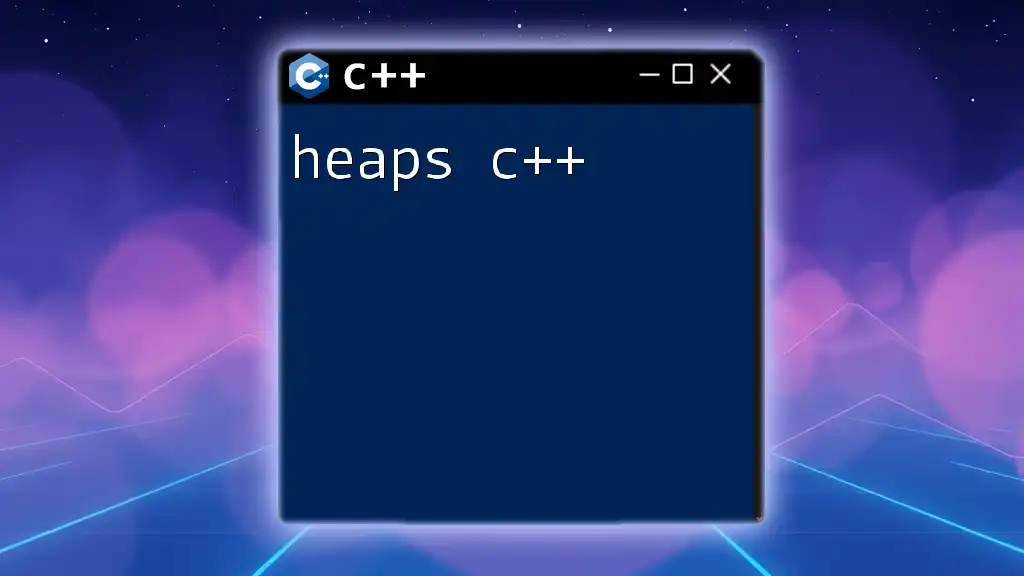
Building and Running Your C++ Program
Compiling Your Project
Once your code is ready, it’s essential to compile the project to identify errors.
- Click on the Build button in the toolbar, or use F11 to compile the code. NetBeans will display any compilation errors in the Output Window, so you can troubleshoot them easily.
Running Your Project
After a successful build, you can run your program:
- Click the Run button or press F6 to execute the application. The Output Window will display "Hello, NetBeans!" confirming that your program operates as intended.
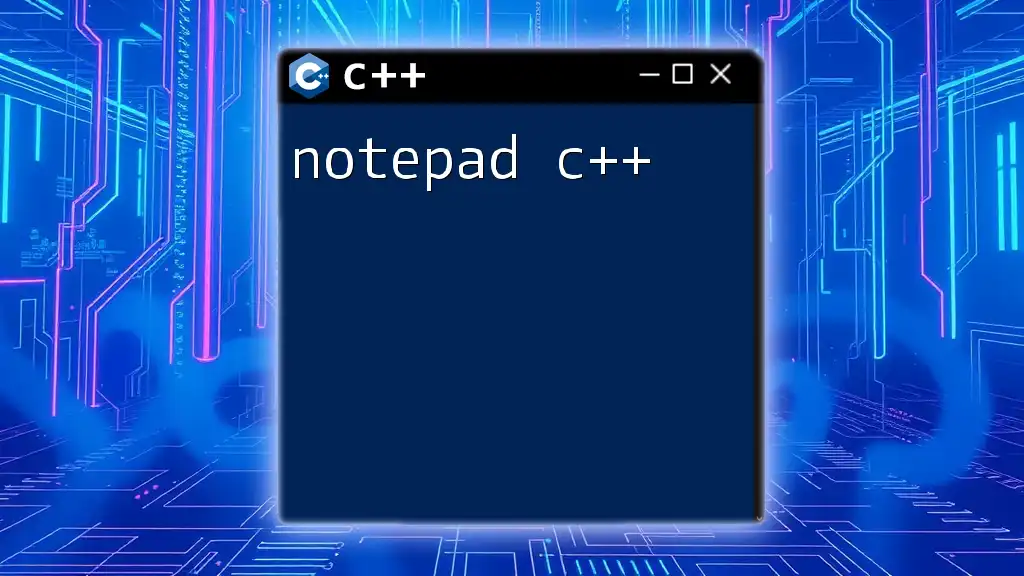
Debugging in NetBeans
Using the Debugger
Debugging is vital for finding and fixing defects. NetBeans provides robust debugging tools:
Setting Breakpoints:
Click in the left margin next to a code line to set a breakpoint. This will pause program execution at that line, allowing you to inspect the program's state.
Step Through Code Execution:
Use the debugger controls to step into, over, or out of function calls, enabling you to follow the code flow systematically.
Common Debugging Techniques
Debugging can be made easier with effective techniques:
Inspecting Variables:
Hover over variables during debugging to view their current values. This helps confirm whether your program behaves as expected.
Using the Call Stack:
The Call Stack window displays active function calls, allowing you to track the sequence of execution, which is invaluable for identifying logical errors.
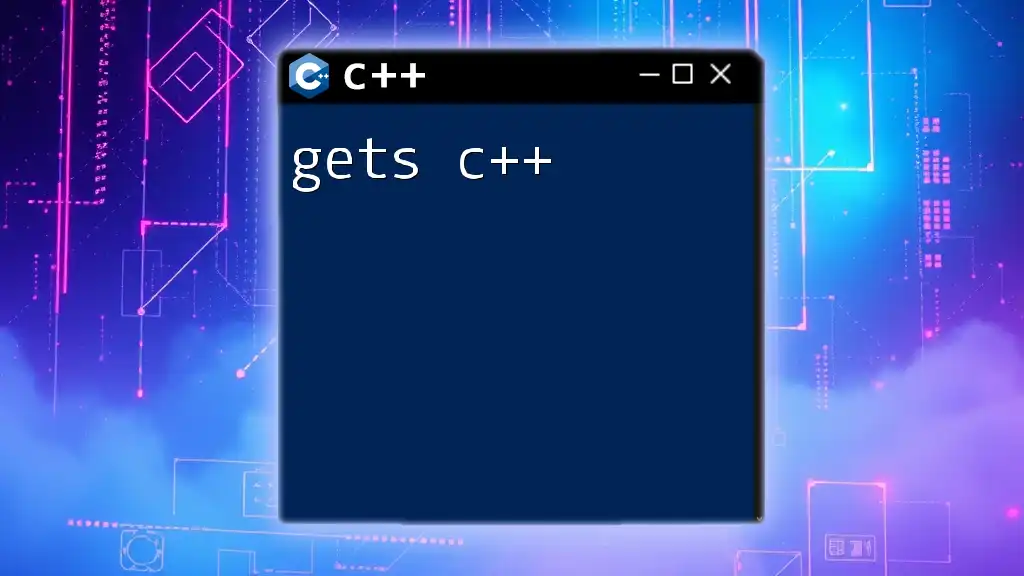
Enhancing Your Development Experience
Customizing NetBeans
Tailoring NetBeans to suit your workflow is essential for comfort and efficiency:
Themes and Appearance:
To change the IDE’s visuals:
- Navigate to Tools > Options > Appearance. Here, you can select themes, modify font sizes, and adjust other display preferences.
Useful Plugins for C++ Development
Plugins can enhance your NetBeans experience:
Top Plugins to Consider:
- Boost C++ Libraries: Improves functionality and supports advanced programming techniques.
- CPPcheck: A static code analysis tool that identifies bugs and potential issues in your C++ code.
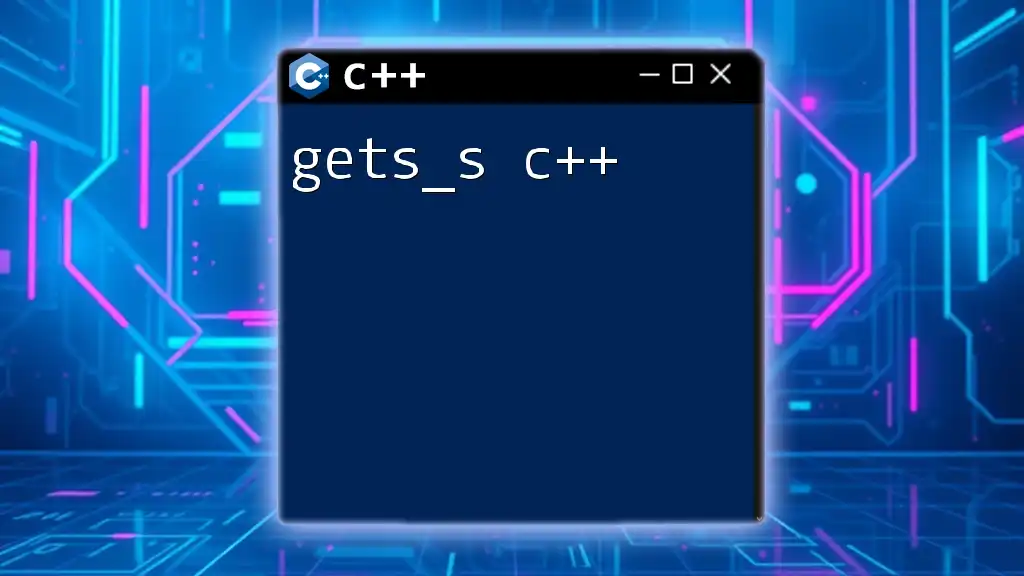
Best Practices for C++ Development in NetBeans
Code Organization
Organizing your code can help maintain clarity and efficiency:
Project Structure:
- Group related source files into folders.
- Keep headers in a include directory and source files in a src directory.
Version Control
Integrating version control systems ensures your coding history is preserved:
Integrating Git:
Set up Git within NetBeans for code versioning:
- Create a repository via Team > Git > Initialize Repository.
- Use Git functions from the Teams menu for commits, pushes, and merges.
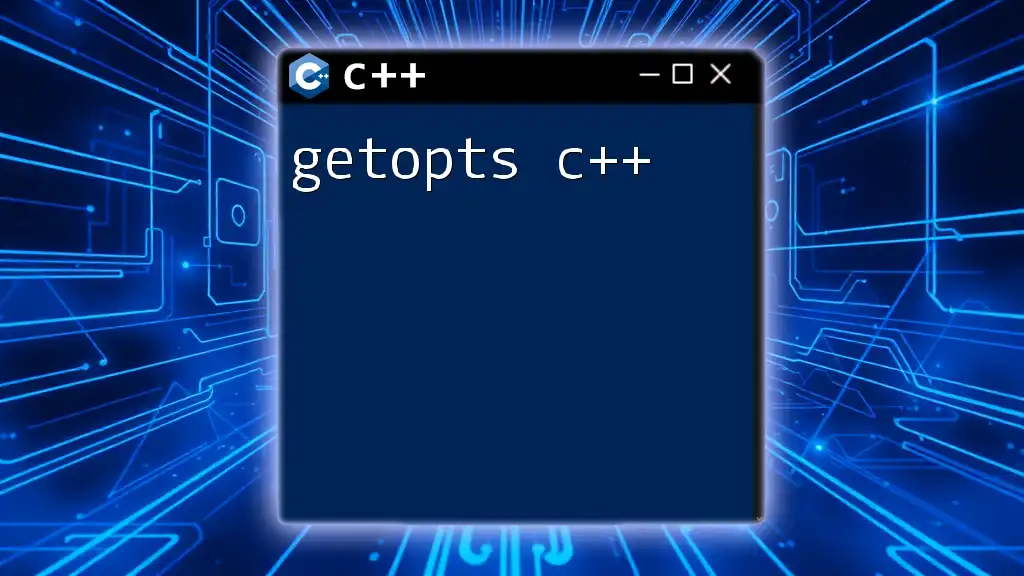
Conclusion
In summary, mastering the use of NetBeans for C++ development opens doors to enhanced productivity and coding efficiency. From installation to debugging, the IDE provides a rich set of features tailored for both beginners and seasoned developers. As you continue to explore C++ and NetBeans, embrace experimentation and continually refine your skills, paving the way for successful programming projects.
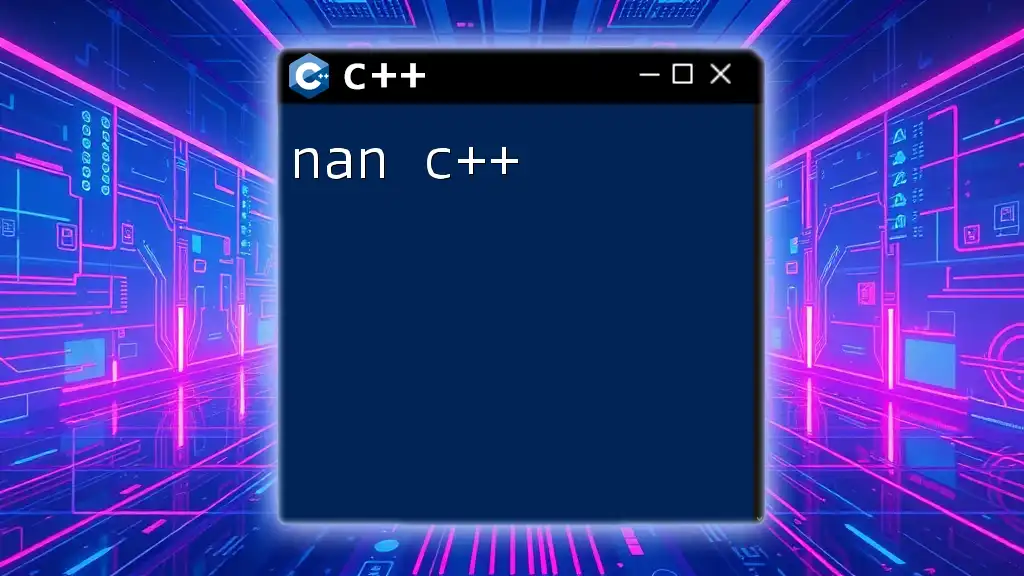
Additional Resources
To further enhance your C++ development skills, explore:
Official NetBeans Documentation:
Visit the NetBeans website for comprehensive resources, tutorials, and FAQs.
Community and Support Forums:
Engage with peers and seek help through platforms like Stack Overflow to expand your knowledge and resolve coding challenges.