"Codebeauty C++" refers to techniques and practices that enhance the readability and aesthetics of C++ code, making it more efficient and easier to maintain.
Here's a simple example of a C++ function that demonstrates clean coding practices:
#include <iostream>
using namespace std;
// Function to calculate the square of a number
int square(int number) {
return number * number;
}
int main() {
int num = 5;
cout << "The square of " << num << " is " << square(num) << endl;
return 0;
}
What is CodeBeauty C++?
CodeBeauty C++ refers to the practices and tools associated with beautifying C++ code to enhance its readability and maintainability. Code beauty is significantly pivotal in software development, as it not only improves the visual formatting of the code but also plays a critical role in debugging, collaboration, and long-term project sustainability.
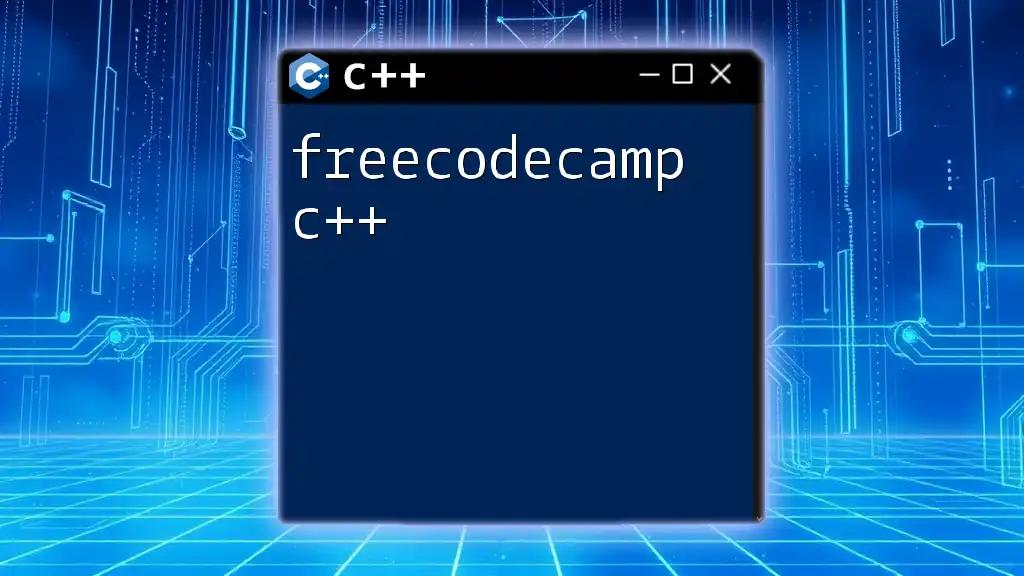
Understanding Code Beautification
What is Code Beautification?
Code beautification is the process of restructuring and formatting code to make it cleaner and more aesthetically pleasing. This involves adjustments such as consistent indentation, appropriate line breaks, and enforcing naming conventions. Ultimately, beautified code is easier to read and understand, which reduces the cognitive load required for developers when trying to comprehend complex logic.
Benefits of Beautifying C++ Code
-
Improved Readability: Clean code following established patterns allows developers to quickly grasp the purpose and mechanics of the code without getting lost in inconsistent formatting.
// Before beautification int main() {int a=5; int b=10; if(a<b){cout<<"a is less than b";} else {cout<<"a is greater than or equal to b";} return 0;}
// After beautification #include <iostream> using namespace std; int main() { int a = 5; int b = 10; if (a < b) { cout << "a is less than b"; } else { cout << "a is greater than or equal to b"; } return 0; }
-
Easier Debugging: Well-structured code makes it easier to identify errors. Instead of searching through a misformatted mess, developers can quickly locate logical errors or typos.
-
Collaboration with Team Members: Projects often involve multiple developers. Code beautification standardizes the format, ensuring everyone reads and edits the code uniformly, minimizing misunderstandings.
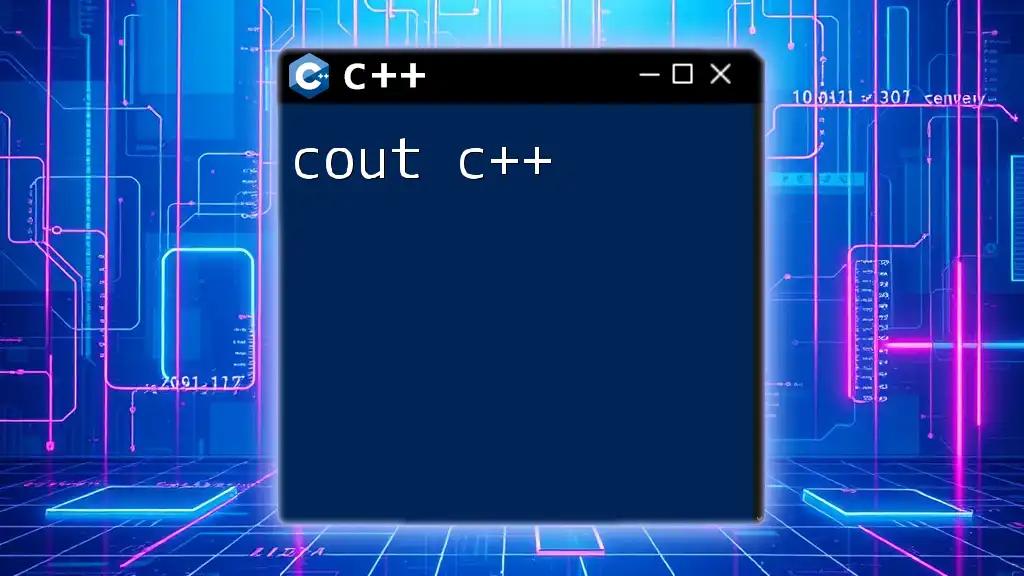
Tools and Techniques for Code Beautification in C++
Popular C++ Code Beautifiers
-
ClangFormat: This tool is part of the Clang project and provides a powerful mechanism for formatting C++ code. To utilize ClangFormat, first, install it via package managers or from the official Clang website. The following is a basic example configuration that can be placed in a file named `.clang-format`:
BasedOnStyle: LLVM IndentWidth: 4 UseTab: Always BreakBeforeBraces: Attach
Applying ClangFormat on the sample code from before results in formatted code that follows the specified style.
-
Artistic Style (Astyle): Another popular option is Astyle, which supports multiple programming languages, including C++. Similar to ClangFormat, it can be configured to adhere to specific style guidelines. An example command for beautifying a file is:
astyle --style=linux your_code.cpp
Integrated Development Environment (IDE) Settings
-
Configuring Visual Studio: Visual Studio provides built-in settings for formatters. Navigate to `Tools > Options > Text Editor > C/C++ > Formatting` and adjust your preferences for indentation, spacing, and more.
-
Using Code::Blocks: For users of Code::Blocks, beautifying code can be done through external tools or by setting up specific project preferences ensuring that coding styles are consistent.

Essential C++ Code Beautification Techniques
Consistent Indentation
Using a consistent indentation style, such as 4 spaces or a tab, is essential. Indentation not only enhances readability but also visually represents code blocks, making logic structures clearer. Different indentation styles can be reflected in code formatting and lead to major differences in readability.
// Example of poor indentation
int sum(int a,int b){return a+b;}
// Example of consistent indentation
int sum(int a, int b) {
return a + b;
}
Naming Conventions
In C++, it is crucial to follow naming conventions for variables and functions, improving the readability and meaning behind the code's structure. The use of CamelCase for class names and snake_case for variable names can contribute to clear understanding.
// Good naming conventions
class PlayerCharacter {};
int player_health;
// Poor naming conventions
class pc {};
int ph;
Structuring Code with Comments
Comments are a vital part of code beautification. They should clarify code functionality rather than restate it. A good practice is placing comments above complex lines or sections of code.
// Calculate the factorial of a number
int factorial(int n) {
if (n == 0) {
return 1;
}
return n * factorial(n - 1);
}
Code Organization
File Structure Recommendations: Organizing files logically by grouping related classes or functionalities promotes clarity. For instance, follow a structure like:
/src
/main.cpp
/utils
/helper.cpp
/helper.h
/models
/Player.cpp
/Player.h
Function and Class Design: Aim to keep functions short and with a single responsibility. As a rule of thumb, if a function exceeds twenty lines, consider refactoring it into smaller sub-functions.

Advanced Beautification Techniques
Leveraging Modern C++ Features
Modern C++ introduces features that enhance both functionality and aesthetics, such as range-based loops, which can replace traditional for-loops for improved clarity.
// Traditional loop
for (std::vector<int>::iterator it = vec.begin(); it != vec.end(); ++it) {
std::cout << *it << " ";
}
// Range-based loop
for (const auto& value : vec) {
std::cout << value << " ";
}
Refactoring Techniques
Removing Dead Code: Identify and eliminate unused functions or variables that clutter the code. This can often be achieved through analysis tools or performing regular code reviews.
Code Reusability Practices: Reuse code by extracting repeated logic into reusable functions or classes. This not only minimizes redundancy but also enhances maintainability.
// Redundant code
void drawCircle(int radius) {
// Draw circle logic...
}
void drawCircle2(int radius) {
// Draw circle logic...
}
// Refactored code
void drawCircle(int radius) {
// Draw circle logic...
}

Best Practices for Maintaining Code Beauty
Regular Code Reviews
Conduct regular code reviews as part of the development process. During reviews, pay close attention to adherence to your formatting guidelines, identifying areas where code does not comply with beautification standards.
Continuous Integration Tools
Implement linters and continuous integration (CI) tools, such as CPPcheck or SonarQube, which automatically flag issues or discrepancies in style, aiding in maintaining coding standards across the team.
Educational Approach
Investing in ongoing training and workshops for your team is invaluable. Staying updated on best practices helps maintain a culture of clean coding. Numerous online platforms provide resources and structured courses to keep skills sharp.
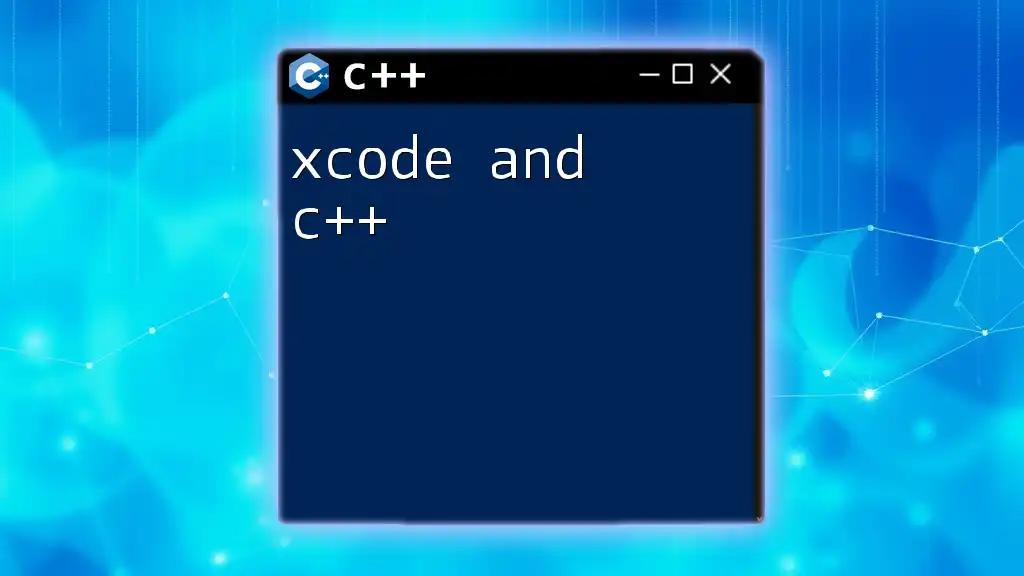
Conclusion
The practice of codebeauty in C++ is not just a matter of aesthetics; it directly influences the maintainability and sustainability of software projects. By adopting and promoting code beautification practices, developers can significantly improve their coding efficiency and effectiveness. Embracing tools and techniques designed for beautification can lead to better collaborative environments, ultimately enhancing productivity.
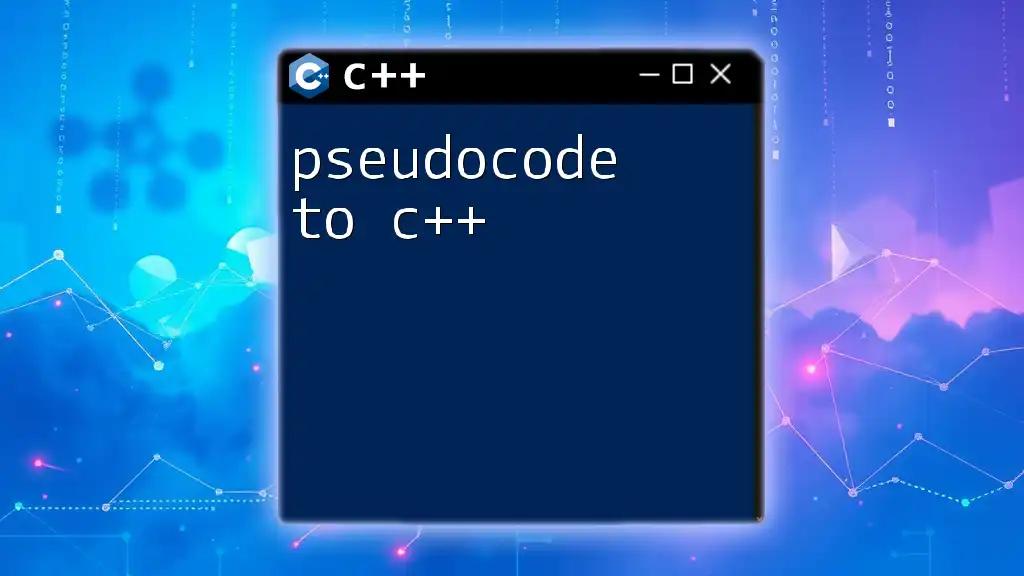
Additional Resources
- Online platforms such as Stack Overflow, CodeProject, and GitHub repositories focused on C++ coding standards.
- Recommended books focusing on code quality and best practices in C++ that can enhance learning and application.
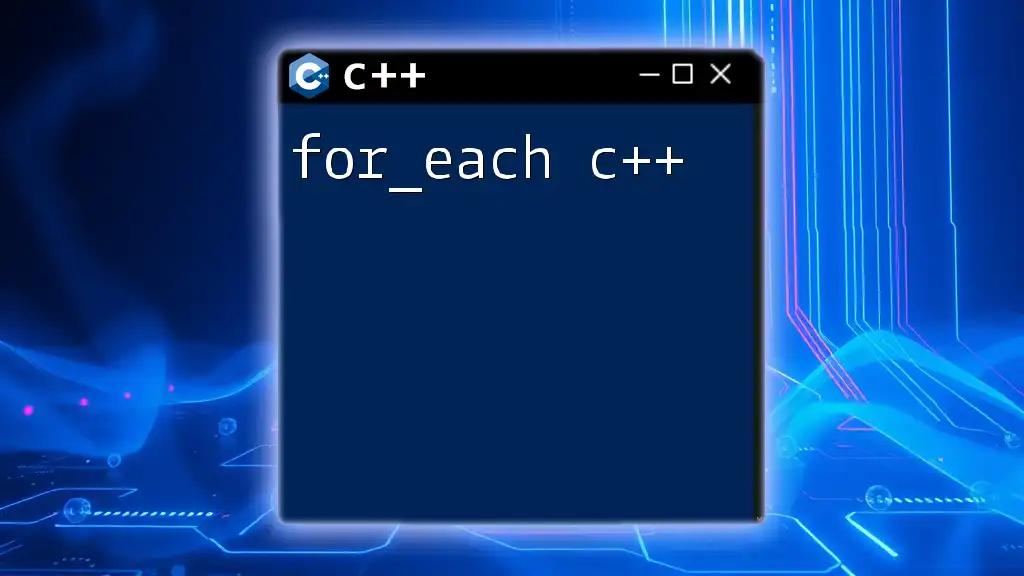
Call to Action
Share your favorite code beautification tools or techniques in the comments, and consider enrolling in workshops offered by our company to elevate your C++ skills to the next level. Together, let’s make coding beautiful!