In C++, comments are used to provide explanations or annotations in the code, which are ignored by the compiler and can be added using either single-line (`//`) or multi-line (`/* ... */`) comment syntax.
Here's an example:
// This is a single-line comment
/*
This is a
multi-line comment
*/
#include <iostream>
int main() {
std::cout << "Hello, World!" << std::endl; // Print message
return 0;
}
What is a C++ Comment?
A comment in C++ is a line or a block of text in the source code that the compiler ignores. Comments serve an essential purpose: they provide context, explanations, and insights into what specific sections of code are doing. This improves the ability to maintain and understand code, both for the original author and collaborators.
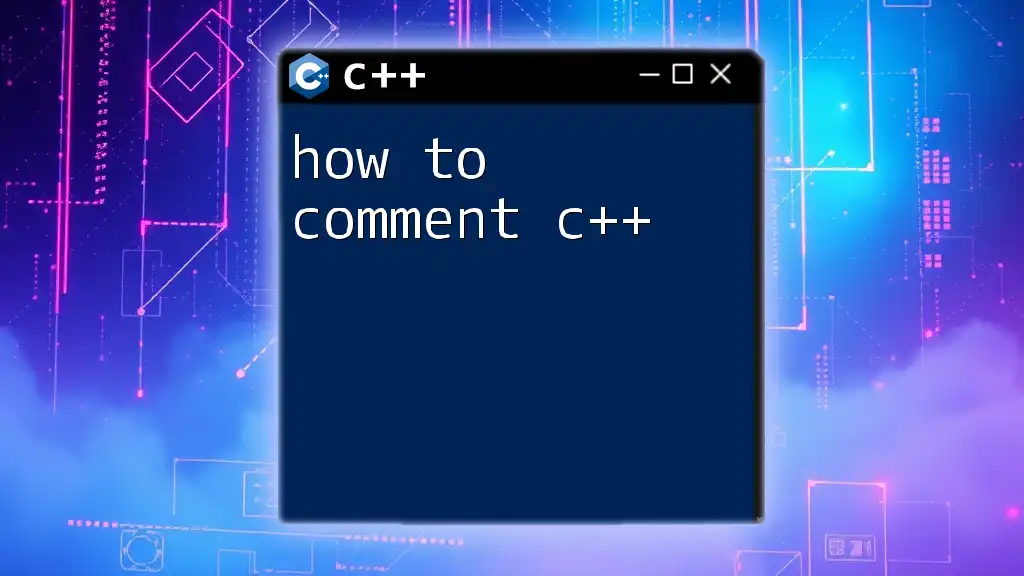
Types of Comments in C++
Single-Line Comments
To create a single-line comment in C++, you can use two forward slashes (`//`). Everything following these slashes on that line will be treated as a comment and will not be executed by the compiler.
Example Code Snippet:
// This variable stores the age of the user
int age = 30;
Single-line comments are particularly useful for brief notes or explanations. They help clarify the purpose of variables or specific lines of code without overwhelming the reader with extensive documentation.
Multi-Line Comments
When you need to include a comment that spans multiple lines, you can use the multi-line comment syntax, which consists of `/` to start the comment and `/` to end it.
Example Code Snippet:
/* This is a multi-line comment
that spans multiple lines. */
int year = 2023;
Multi-line comments are beneficial for providing detailed explanations or when you want to temporarily disable sections of your code during testing or debugging.
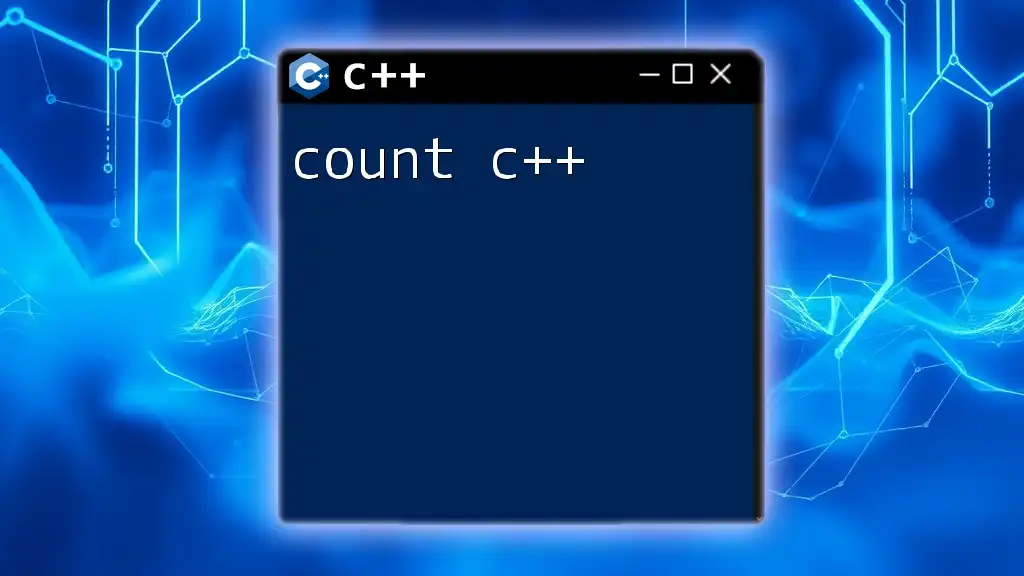
Block Comments in C++
Block comments are very similar to multi-line comments and serve the same purpose. The terminology can vary in practice, but it usually indicates a larger section of comments.
Example Code Snippet:
/*
This block of code performs a check
and outputs results based on conditions.
*/
if (age > 18) {
cout << "Adult";
}
Block comments facilitate the organization of code, especially when you need to provide context on multiple lines. They are great for documenting larger sections of functionality, giving viewers insight into the logic behind a block of code.
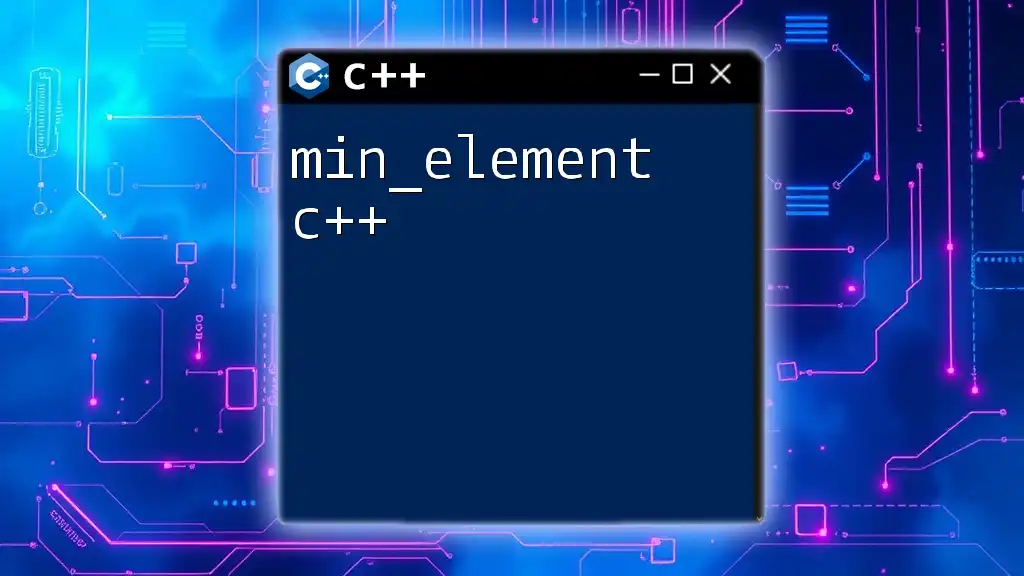
How to Comment in C++: Best Practices
Importance of Clear Comments
Clear and precise comments are vital in programming. They guide readers through complex logic and enhance the overall readability of the code. Whether you're explaining the purpose of a function or clarifying a particular algorithm, understanding comes first.
Avoiding Redundant Comments
One common mistake is to comment sections of code that are already self-explanatory. Redundant comments can clutter your code and lessen its readability.
Example Code Snippet:
// Increment age by 1
age++; // Not needed
Only comment when the intention may not be immediately clear. This approach keeps your code concise and clean.
Using Comments for Documentation
Comments are also instrumental for code documentation. When documenting functions, it's good practice to explain the parameters, return types, and overall functionality.
For instance:
/*
* Function: CalculateSum
* Purpose : Adds two integers
* Params : int a - first integer
* int b - second integer
* Returns : int - sum of a and b
*/
int CalculateSum(int a, int b) {
return a + b;
}
This documentation serves as a reference point for anyone who reads the code, making it easier to understand how to use the function effectively.
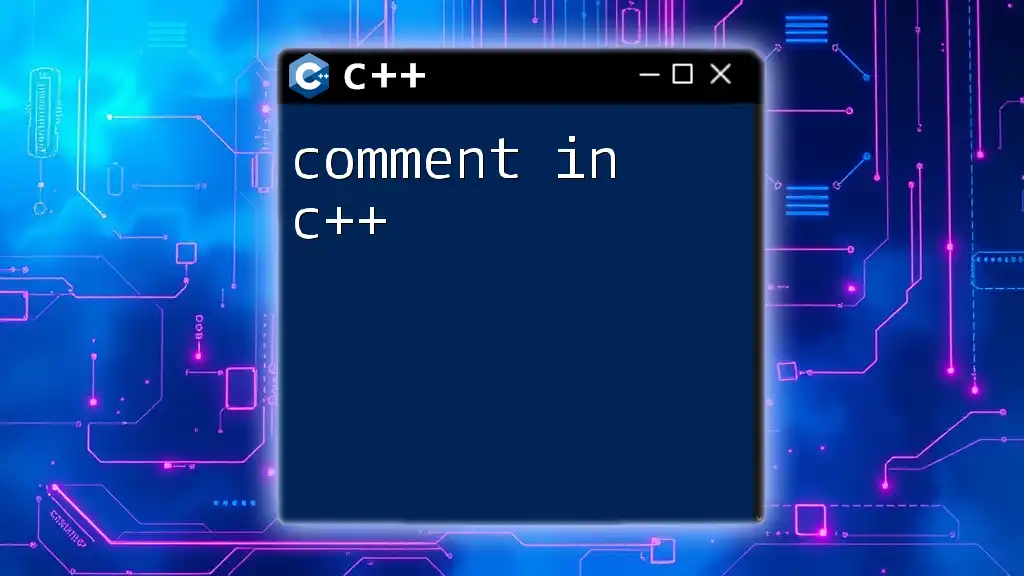
How to Comment Out Multiple Lines in C++
When you want to comment out multiple lines of code, you have a couple of different methods to choose from.
Method 1: Use multi-line comments.
Example Code Snippet:
/*
cout << "This line will not run";
cout << "This line will also not run";
*/
Method 2: Use individual single-line comments.
Example Code Snippet:
// cout << "This line will not run";
// cout << "This line will also not run";
While both methods are valid, using multi-line comments is often more efficient when dealing with larger blocks of code. It makes it clearer that a specific section has been intentionally disabled.
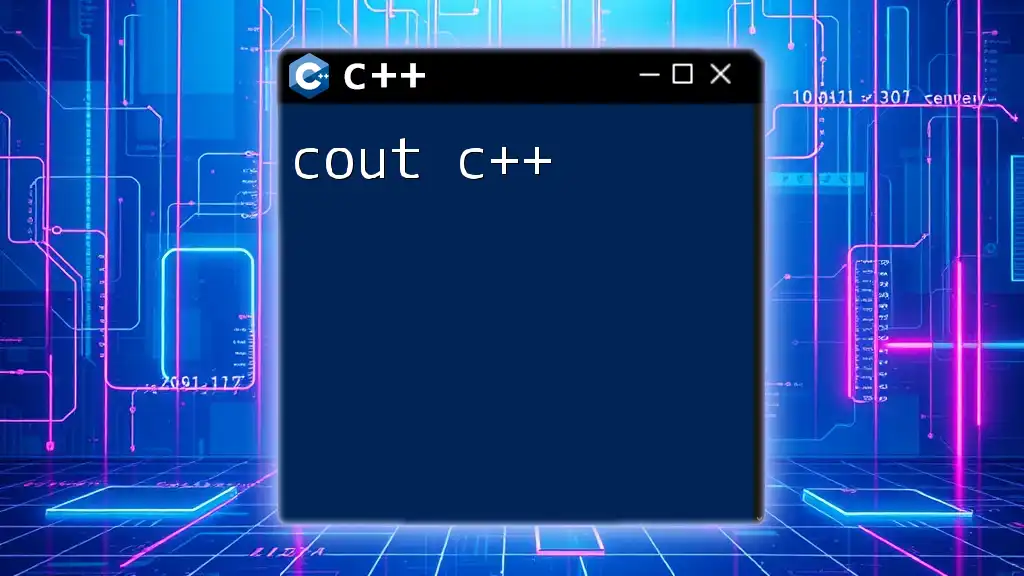
Common Mistakes to Avoid with C++ Comments
Misplacing Comment Syntax
Incorrectly placed comments can lead to compile errors or unintended behaviors in your code.
Example Code Snippet:
// Uncommenting this line will cause an error /* cout << "Error";
Always ensure that your comment syntax is accurate to avoid these pitfalls.
Overusing Comments
While comments are invaluable, excessive commenting can clutter your code. An overload of comments can distract from the code itself. Strive for balance: aim for self-explanatory code wherever possible, using comments for clarity only when necessary.
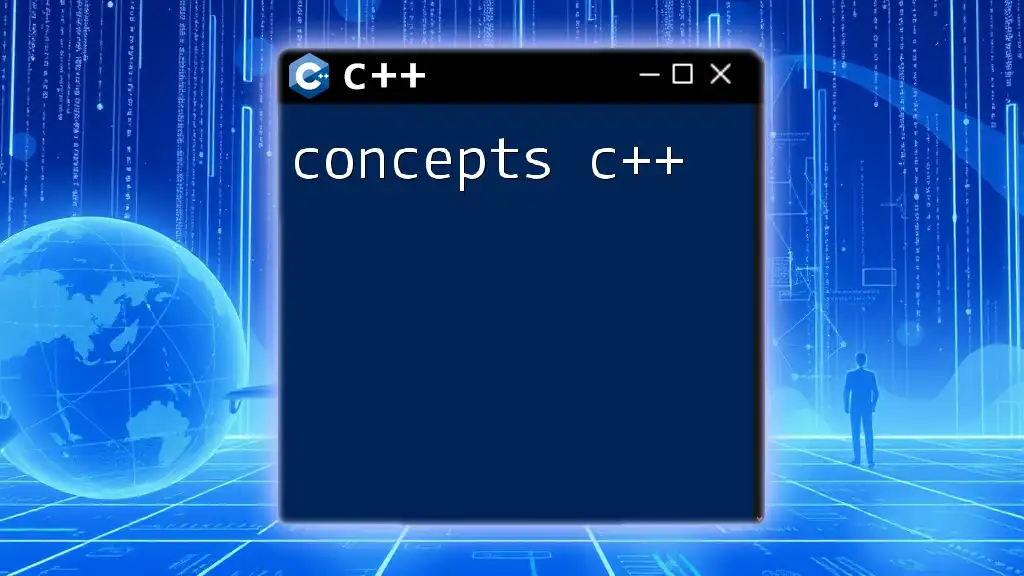
Conclusion
In summary, effectively using comments in C++ can greatly enhance code readability and maintainability. By understanding the types of comments available and following best practices, you can make your code not only functional but also accessible to others. Embrace the power of comments to create a collaborative and efficient programming environment!
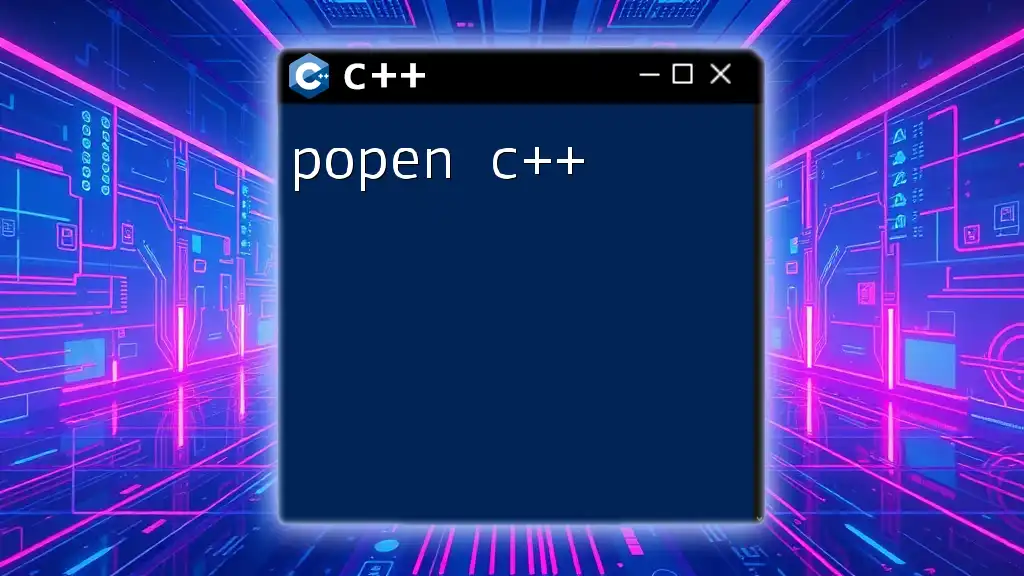
Additional Resources
For further learning on how to implement comments effectively in your C++ code, consider exploring official C++ documentation, online tutorials, and community forums dedicated to best coding practices. With consistent practice, your ability to utilize comments wisely will become a valuable asset in your programming toolkit.