The `std::min_element` function in C++ is used to find the smallest element in a range of elements, returning an iterator to that element.
Here’s a code snippet demonstrating its usage:
#include <iostream>
#include <algorithm>
#include <vector>
int main() {
std::vector<int> numbers = {5, 3, 8, 1, 4};
auto min_it = std::min_element(numbers.begin(), numbers.end());
std::cout << "The minimum element is: " << *min_it << std::endl;
return 0;
}
What is `min_element`?
`min_element` is a powerful function in C++ that is part of the Standard Template Library (STL). It is used to find the minimum value in a range, making it an essential tool for developers when working with various data structures like arrays, vectors, and lists.
Finding the minimum value in a collection can be crucial for numerous applications such as statistical analysis, data processing, and algorithm optimizations.
By the end of this guide, you will have a comprehensive understanding of how to utilize `min_element` effectively and in different contexts in C++.

The `min_element` Function
Syntax
The syntax of the `min_element` function is simple yet concise. It is defined as follows:
template<class ForwardIt>
ForwardIt min_element(ForwardIt first, ForwardIt last);
Parameters
`first`: This parameter is an iterator that points to the first element in the range.
`last`: This parameter is an iterator that points to one past the last element in the range. It helps to define the range to search for the minimum element.
Return Value
The function returns an iterator pointing to the minimum element found within the specified range. If the range is empty, it returns the `last` iterator.

How to Use `min_element` in C++
Including Necessary Libraries
To use `min_element`, you need to include the `<algorithm>` library in your code, along with other necessary libraries:
#include <algorithm>
#include <vector>
#include <iostream>
Basic Example
Finding Minimum in a Vector
Here is a straightforward example of using `min_element` to find the minimum value in a vector:
std::vector<int> numbers = {5, 2, 9, 1, 5, 6};
auto min_it = std::min_element(numbers.begin(), numbers.end());
std::cout << "The minimum element is: " << *min_it << std::endl;
In this example:
- A vector named numbers is initiated with several integers.
- The `min_element` function is called with the beginning and end iterators of the vector.
- The iterator returned by `min_element` points to the lowest number in the collection, which is then dereferenced and printed.
Finding Minimum in an Array
You can also use `min_element` with arrays. Here’s how:
int arr[] = {3, 1, 4, 1, 5, 9, 2, 6, 5};
auto min_it_arr = std::min_element(std::begin(arr), std::end(arr));
std::cout << "The minimum element in the array is: " << *min_it_arr << std::endl;
In this snippet:
- An array `arr` is defined.
- The `min_element` function is invoked using `std::begin()` and `std::end()` to specify the range.
- The minimum element found is outputted to the console.
![Effortless Memory Management: Delete[] in C++ Explained](/_next/image?url=%2Fimages%2Fposts%2Fd%2Fdelete-cpp.webp&w=1080&q=75)
Custom Comparator with `min_element`
Using a Lambda Function
`min_element` can be enhanced with a custom comparison function to find the minimal element based on specific criteria. This can be accomplished using a lambda function.
Here is an example where we have a vector of pairs, representing people and their ages:
std::vector<std::pair<std::string, int>> people = {{"Alice", 30}, {"Bob", 25}, {"Charlie", 35}};
auto min_age = std::min_element(people.begin(), people.end(),
[](const std::pair<std::string, int>& a, const std::pair<std::string, int>& b) {
return a.second < b.second;
});
std::cout << "The youngest person is: " << min_age->first << " aged " << min_age->second << std::endl;
In this example:
- A vector of pairs, people, is defined.
- `min_element` is used with a lambda function as the third argument, comparing the second element (age) of the pairs.
- The code outputs the name and age of the youngest person.
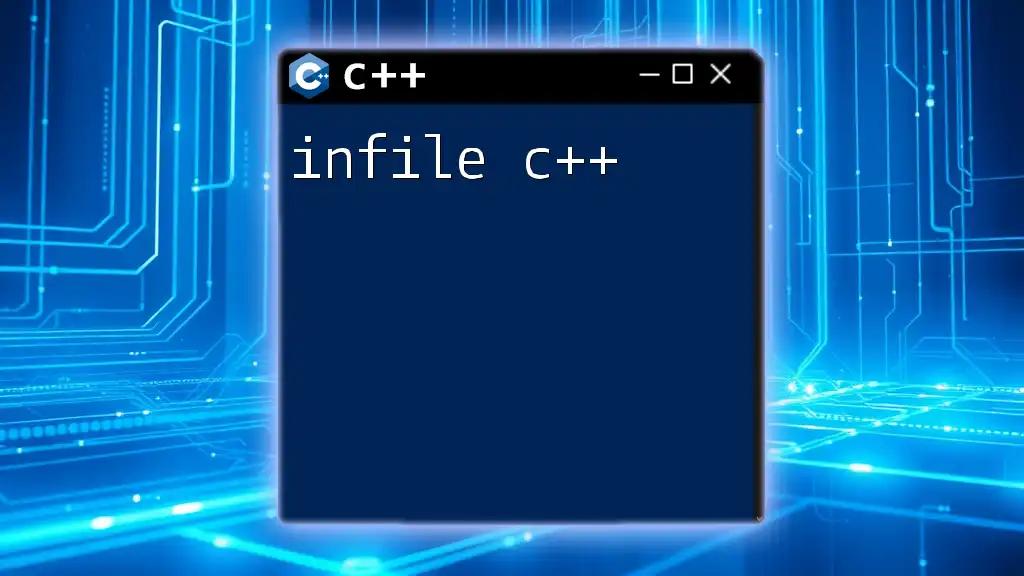
Common Errors to Avoid
Misunderstanding Iterators
One common error when using `min_element` is misunderstanding how iterators work. Developers might mix up `begin()` and `end()` iterators, leading to undefined behavior or incorrect results. Ensure that the `first` iterator is always less than the `last` iterator.
Data Types Consideration
When using `min_element`, it’s essential to note that the function works with primitives as well as custom types and objects. If you attempt to find a minimum element with objects, you need to ensure that the relevant comparison operators are defined for those objects.
For example, consider this class definition without an operator overloading:
class Person {
public:
std::string name;
int age;
// No operator overload for less than
};
std::vector<Person> persons = {{"Alice", 30}, {"Bob", 25}};
auto min_it = std::min_element(persons.begin(), persons.end()); // This will cause an error
To fix it, you need to overload the `<` operator in the `Person` class.

Performance Considerations
Complexity Analysis
The time complexity of `min_element` is O(n), where n is the number of elements in the range. It performs a linear search, making it efficient for modestly sized collections. However, for larger datasets or sorted collections, you might need to explore more efficient algorithms when performance is crucial.
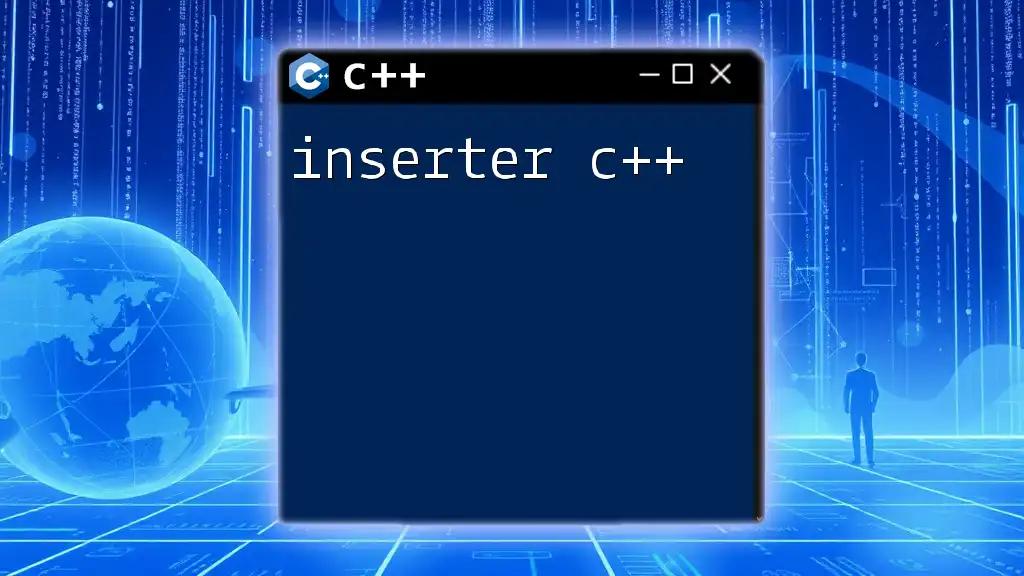
Conclusion
In summary, `min_element` is a versatile function in C++ that allows developers to efficiently find the minimum element in various data structures. Its straightforward syntax and ability to work with custom comparators make it a valuable tool in the C++ programmer's toolkit.
Practice using `min_element` through various coding exercises to solidify your understanding of its applications and behaviors.

FAQ Section
What is the difference between `min_element` and other min-finding methods?
`min_element` is a part of STL and works directly with iterators over collections, allowing for easy integration with algorithms. Other methods may not provide the same level of flexibility or may necessitate extra code for iterating through the collection.
Can `min_element` handle empty containers?
No. Attempting to call `min_element` on an empty container results in undefined behavior. It is essential to check if the range is empty before calling this function.
How does `min_element` behave with equal elements?
If two or more elements are equal, `min_element` will return the iterator pointing to the first occurrence of the minimum element in the specified range.