The `infile` in C++ is used to handle input file streams, allowing you to read data from a file into your program.
Here’s a simple example of how to use `infile` to read data from a text file:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream infile("data.txt");
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
return 0;
}
What is `infile` in C++?
The term `infile` in C++ refers primarily to the input file stream that is used for reading data from files into a program. It serves as a bridge between the file stored on the disk and the program's run-time environment. Utilizing `infile` effectively allows developers to load data, configuration settings, or any type of relevant information from an external source, thus enabling greater flexibility and functionality within their applications.
Usage Context
You might consider using `infile` in several scenarios, such as:
- Reading user input data that is stored in a file.
- Loading configuration data for an application.
- Processing data files like CSVs, logs, or text documents.
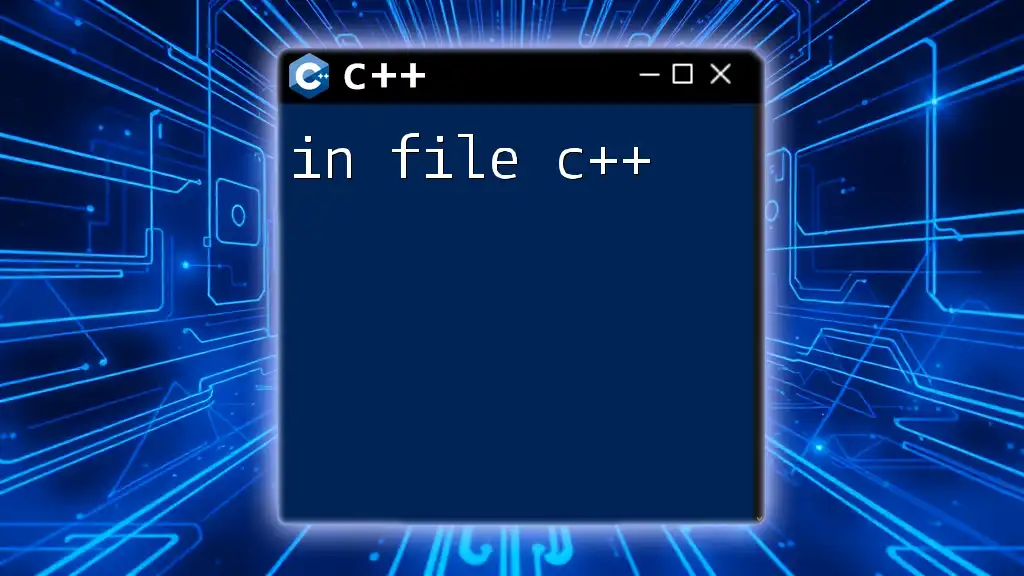
Setting Up for File Input
Including Necessary Libraries
Before we can work with `infile`, it's essential to include the appropriate standard library that provides the requisite functions and classes for file handling:
#include <fstream>
This inclusion grants access to the `ifstream` class, which is the input file stream class used for reading files.
Defining the Input File Stream
To read from a file, you need to define an `ifstream` object. This object acts as a channel through which data flows from the file into your program. Here’s how you would typically declare it:
std::ifstream infile("example.txt");
This line not only creates an `ifstream` instance but also opens `example.txt` for reading.
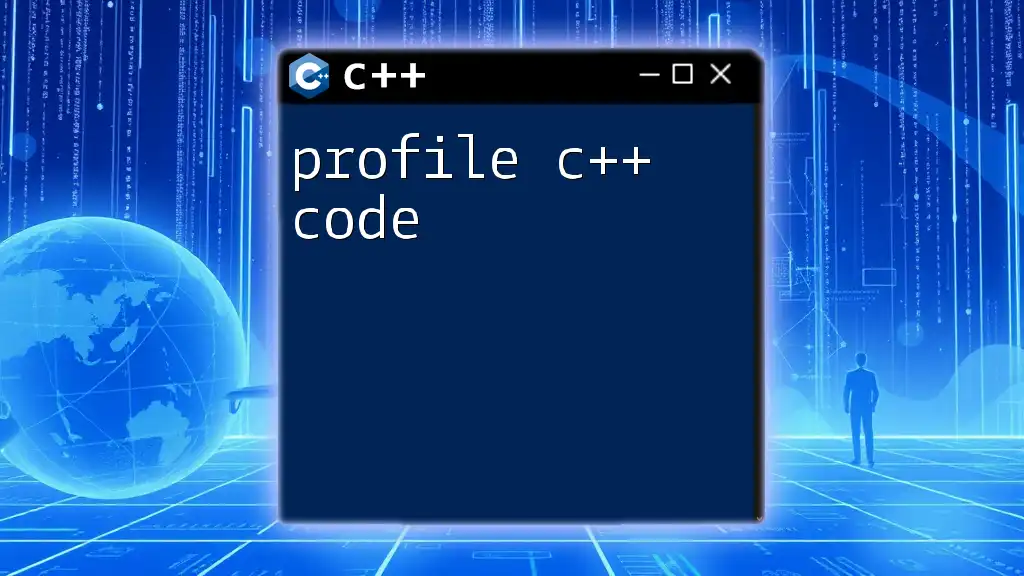
Opening a File with `infile`
Using the `open()` Method
You may explicitly open a file using the `open()` method of the `ifstream` class. This method allows for more control, such as when you need to open a file after creating the stream object:
infile.open("data.txt");
Automatic File Opening
Alternatively, you can open a file automatically when creating the `ifstream` object. This approach is more concise and often favored for simplicity:
std::ifstream infile("data.txt");
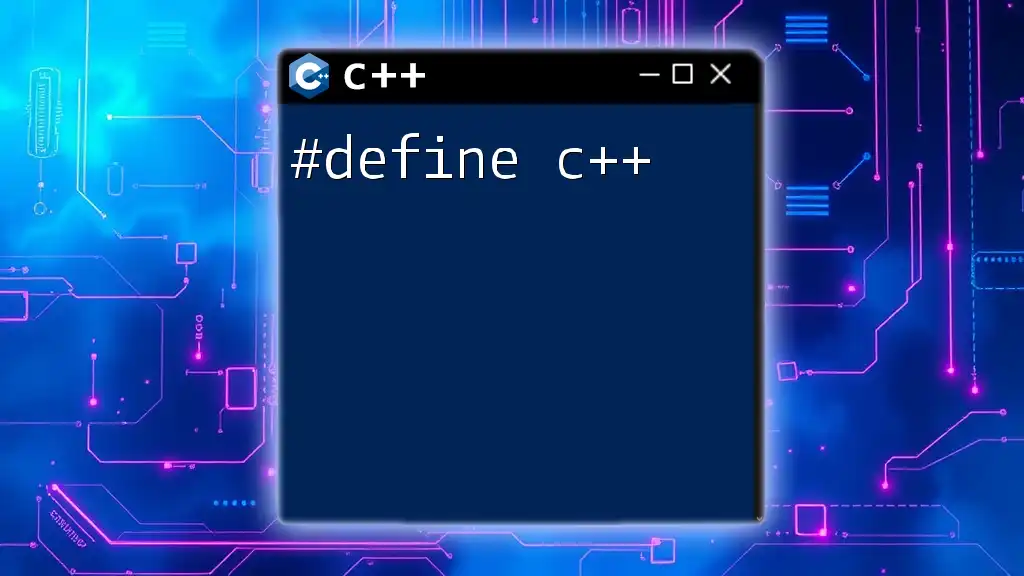
Checking if the File is Open
Upon attempting to open a file, it is crucial to confirm whether the file is indeed open. This prevents potential runtime errors that could arise from trying to read from an unopened file. Use the `is_open()` method to check the file's status:
if (!infile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
}
If the file opening fails, the message will notify you of the error, guiding you to troubleshoot the file path or permissions.
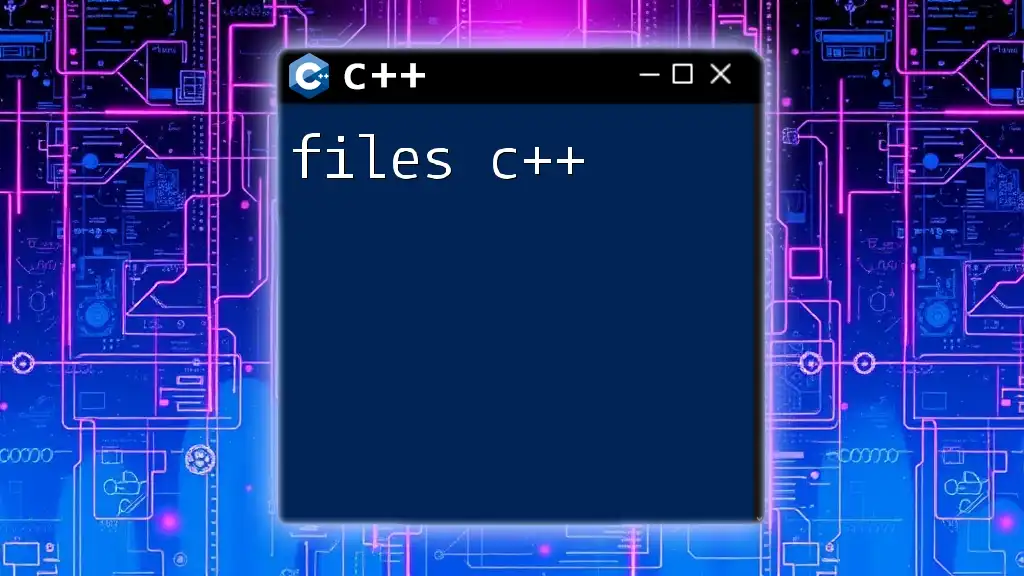
Reading Data from the File
Using Extraction Operator (`>>`)
C++ offers an intuitive way to read data from files using the extraction operator `>>`. This is particularly useful for reading formatted data such as integers, floats, or strings:
int number;
infile >> number;
This code will read an integer value from the file, provided the number is formatted correctly.
Reading Lines with `getline()`
For scenarios where you need to read entire lines of text, `getline()` is your go-to function. This function reads data until a newline character is encountered, enabling you to capture long strings:
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
In this example, the loop continues until the end of the file, outputting each line sequentially.
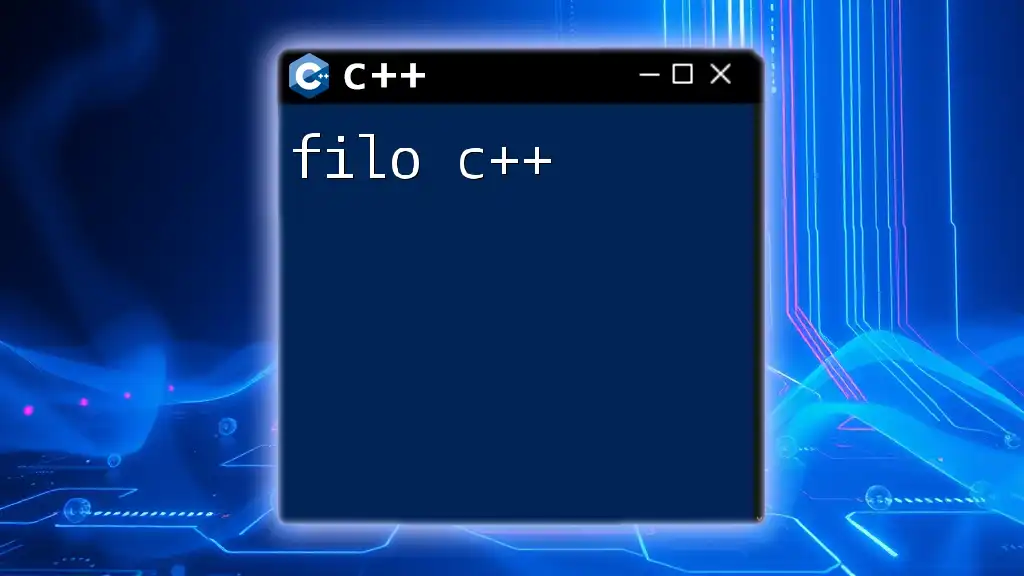
Closing the File
Importance of Closing File Streams
After completing your read operations, it is paramount to close the file. Not only does this free system resources, but it also helps prevent data corruption and ensures that all data is flushed from the buffer.
Using the `close()` Method
You can close an `ifstream` object using the `close()` method:
infile.close();
This practice is vital to maintain the integrity of your application and manage resources efficiently.
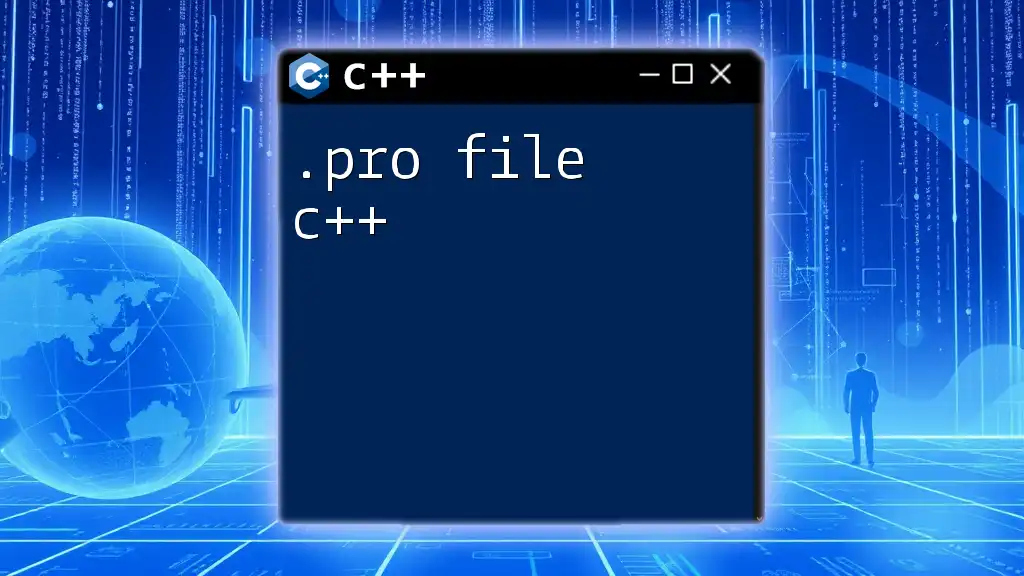
Handling Errors in File Input
Checking Stream Status with `fail()`
While reading from a file, errors may occur, such as trying to read data that doesn’t exist or is formatted incorrectly. By using `fail()`, you can determine if an error has occurred in the input stream:
if (infile.fail()) {
std::cerr << "Failed to read from file." << std::endl;
}
This step enhances the robustness of your file handling code.
Using Try-Catch Structure
In C++, exception handling is a vital part of dealing with file input. Although standard input file operations do not throw exceptions by default, using a try-catch block can help manage unforeseen occurrences gracefully:
try {
// file reading code
} catch (const std::exception& e) {
std::cerr << "Exception: " << e.what() << std::endl;
}
This structure allows you to catch any exceptions thrown during runtime and handle them appropriately, thus preventing crashes and maintaining a stable flow in your program.
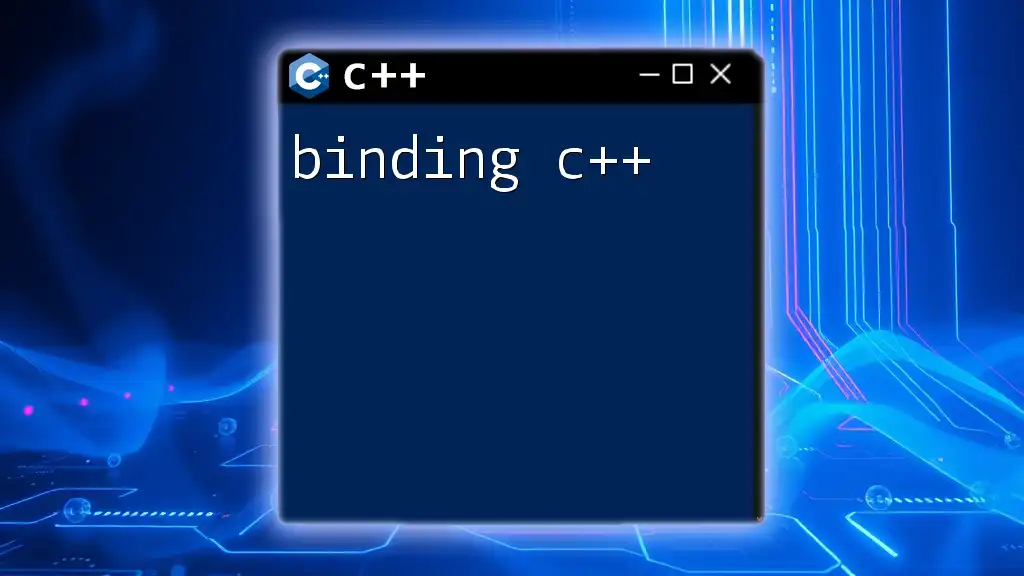
Example Using `infile` in a Complete Program
To illustrate the entire process of using `infile`, let’s look at a complete example that incorporates the concepts discussed:
#include <iostream>
#include <fstream>
#include <string>
int main() {
std::ifstream infile("data.txt");
if (!infile.is_open()) {
std::cerr << "Error opening file!" << std::endl;
return 1;
}
std::string line;
while (std::getline(infile, line)) {
std::cout << line << std::endl;
}
infile.close();
return 0;
}
In this example, the program attempts to open `data.txt`, checks if it was successful, and then reads and outputs each line to the console. Finally, it closes the file to preserve resources.
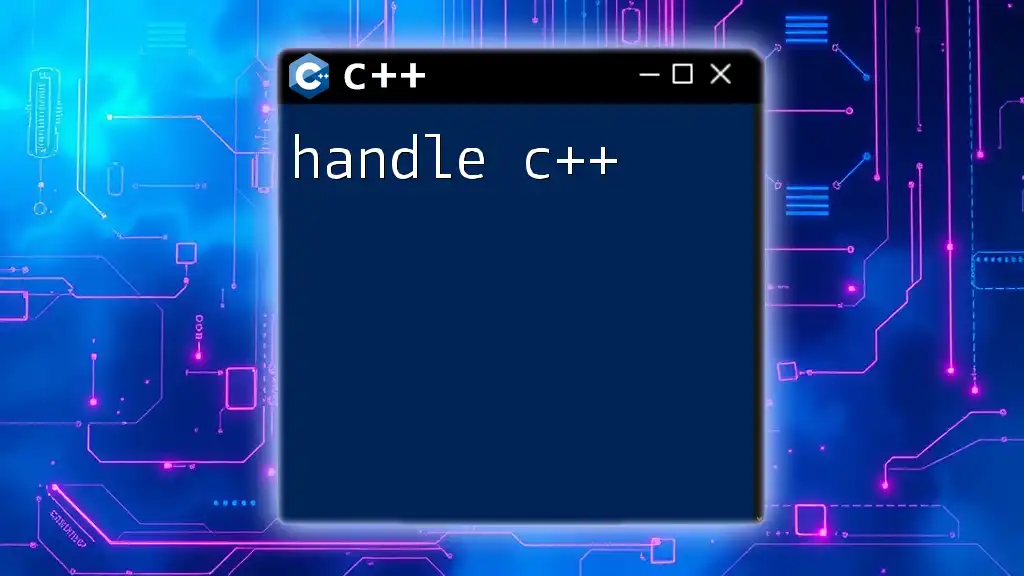
Best Practices for File Input in C++
Avoiding Common Pitfalls
When working with `infile`, there are several common errors to be wary of:
- Failing to check if the file opened successfully can lead to runtime errors and unexpected behavior.
- Forgetting to close file streams can cause memory leaks and other resource management issues that affect your application's performance.
Enhancing Code Readability
- Using descriptive naming conventions for your input files enhances readability and maintainability.
- Commenting on your code helps others (and your future self) understand your logic and decisions effortlessly.
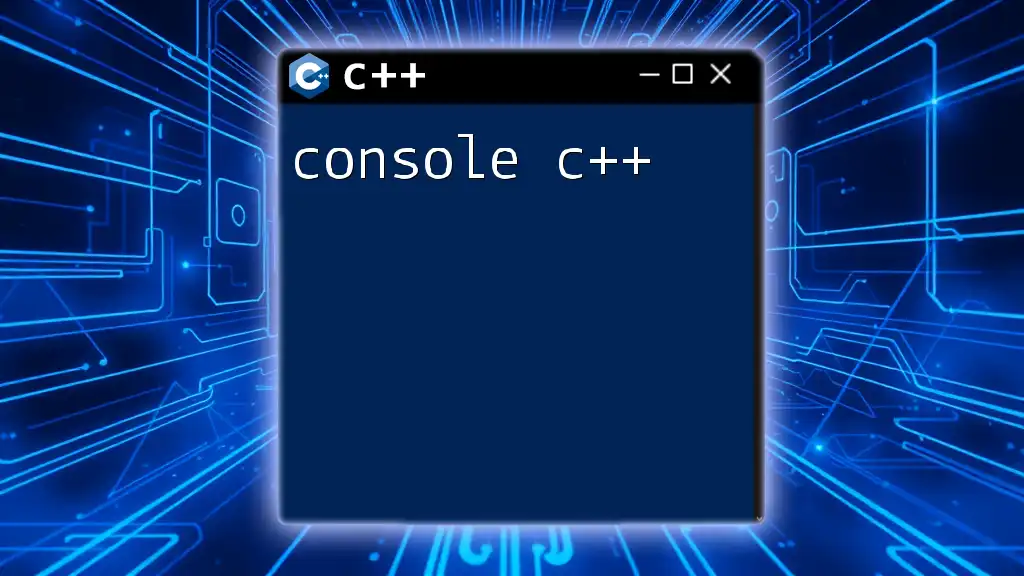
Conclusion
In summary, understanding how to effectively use `infile` in C++ will significantly enhance your ability to read and manipulate data from files. This knowledge empowers you to create more dynamic and flexible applications that can adapt to varying input scenarios. Practice using these techniques in your projects to gain mastery over file input in C++.
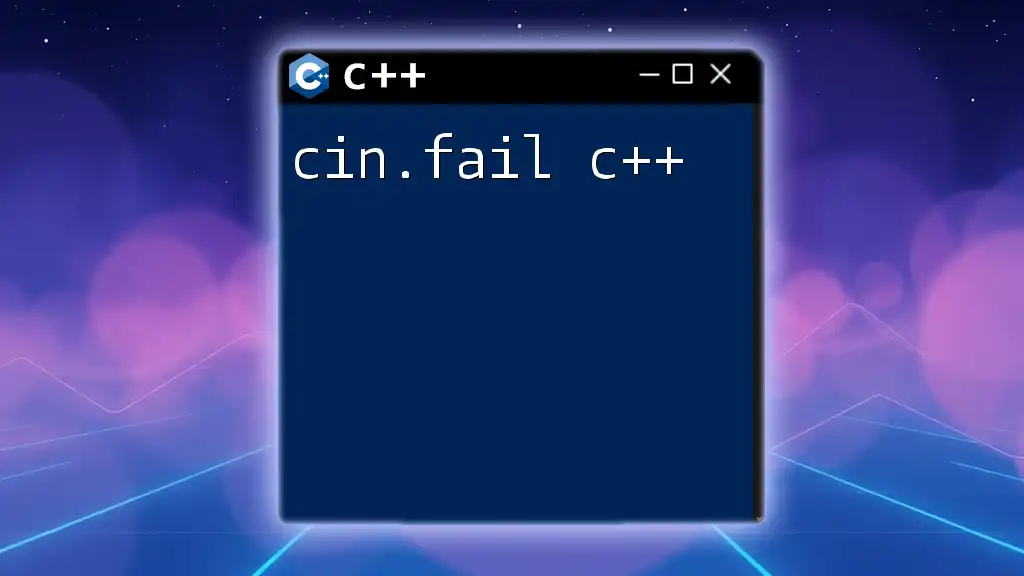
Further Reading and Resources
To continue your journey in mastering file handling and C++, you can explore recommended books, tutorials, and online courses that delve deeper into these topics. These resources will broaden your understanding and skills in C++ file management.