In C++, file handling is accomplished using streams, allowing you to read from and write to files easily.
Here's a simple example of writing to a file and then reading from it:
#include <iostream>
#include <fstream>
int main() {
// Writing to a file
std::ofstream outFile("example.txt");
outFile << "Hello, C++ File Handling!" << std::endl;
outFile.close();
// Reading from the file
std::ifstream inFile("example.txt");
std::string line;
while (std::getline(inFile, line)) {
std::cout << line << std::endl;
}
inFile.close();
return 0;
}
Understanding File Streams
What are File Streams?
In C++, file streams are used to facilitate interactions between a program and files on the disk. A file stream allows programs to read data from files or write data to files, essentially bridging the gap between the program's data structures and persistent storage.
Types of File Streams
C++ provides three primary types of file streams, allowing various operations related to file handling:
-
ifstream: This is used for input file stream operations, mainly to read data from files.
-
ofstream: This is used for output file stream operations, mainly to write data to files.
-
fstream: This allows both reading and writing to and from a file, making it versatile for diverse file operations.
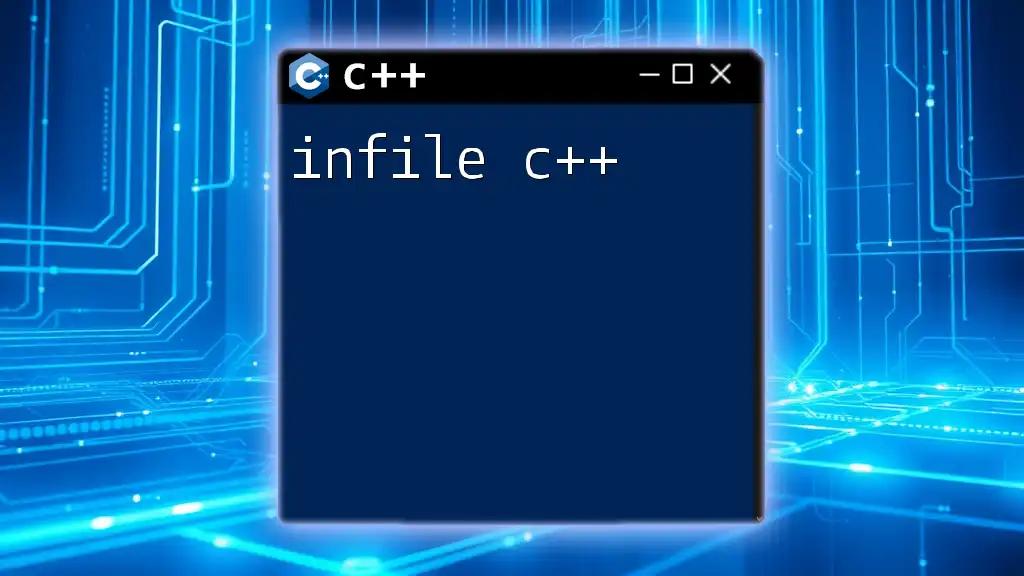
Basic File Operations
Opening a File
To start working with files, you must first open them. An essential step when dealing with files in C++ is to create an instance of the appropriate stream class (`ifstream`, `ofstream`, or `fstream`), specifying the file name you want to access.
Here’s a straightforward example using `ifstream` to open a file:
#include <fstream>
#include <iostream>
std::ifstream inputFile("example.txt");
if (!inputFile) {
std::cerr << "Error opening file!" << std::endl;
}
The above snippet checks if the file has opened successfully. If not, it prints an error message. Handling errors is a crucial step to ensure that your program behaves predictably and doesn't encounter unexpected runtime failures.
Closing a File
Just as opening a file is important, so is closing a file properly once you are finished working with it. This releases system resources and ensures that all buffered output content is written to the file.
You can close a file as follows:
inputFile.close();
Neglecting to close files can lead to memory leaks or data corruption.
Reading from Files
Reading Single Characters
To read data from a file one character at a time, the `get()` method of an ifstream object can be employed. This method allows you to read each character until the end of the file is reached. Here is how you can achieve this:
char ch;
while (inputFile.get(ch)) {
std::cout << ch;
}
In this code, `ch` is a character variable, and the while loop continues until the end of the file is encountered, outputting each character on the console.
Reading Lines
Sometimes, you may want to read an entire line from a file at once. The `getline()` function is particularly useful for this purpose. Here’s a quick demonstration:
std::string line;
while (std::getline(inputFile, line)) {
std::cout << line << std::endl;
}
This loop reads one line at a time until the end of the file, storing each line in a `std::string` variable called `line`.
Writing to Files
Writing Strings
To write strings (or any other type of data) to a file using `ofstream`, simply follow this example:
std::ofstream outputFile("output.txt");
outputFile << "Hello, World!" << std::endl;
outputFile.close();
This code opens (or creates) a file named `output.txt`, writes a string to it, and subsequently closes the file.
Appending to Files
If you wish to append content to an existing file rather than overwrite it, you need to open the file in append mode using `std::ios::app`. Here’s how:
std::ofstream outputFile("output.txt", std::ios::app);
outputFile << "Appending this line." << std::endl;
This ensures that any new data is added to the end of the file instead of replacing the original content.

Advanced File Handling Techniques
Error Handling in File Operations
Robust programs include error handling to manage unexpected situations. For file operations, it’s prudent to check if the file was successfully opened before proceeding. Here's an example of how to enforce this:
if (!inputFile) {
std::cerr << "File could not be opened or does not exist." << std::endl;
}
This simple check prevents your program from running into issues when accessing non-existent files.
Working with Binary Files
When working with files, you may come across binary files, which store data in a format that is not human-readable, unlike text files. Working with binary files requires a slightly different approach, particularly in terms of reading and writing data.
Here’s a way to write an integer to a binary file:
std::ofstream binaryOut("binary.dat", std::ios::binary);
int number = 42;
binaryOut.write(reinterpret_cast<char*>(&number), sizeof(number));
binaryOut.close();
And here’s how to read that integer back from the binary file:
std::ifstream binaryIn("binary.dat", std::ios::binary);
int readNumber;
binaryIn.read(reinterpret_cast<char*>(&readNumber), sizeof(readNumber));
Using `reinterpret_cast` allows you to treat the address of `number` as a pointer to a character type, which is necessary for the write and read methods.
File Positioning
Seeking in Files
File positioning pointers are vital for navigating through stored data, especially in long files where you may need to skip portions of data. You can manipulate the pointer using functions like `seekg()` and `tellg()`. Here’s how to seek to the end of a file and get the position:
inputFile.seekg(0, std::ios::end);
std::streampos end = inputFile.tellg();
This snippet moves the file pointer to the end of the file and retrieves the position for potential operations.

Conclusion
In summary, understanding files in C++ is fundamental for any programmer interested in storing and retrieving data effectively. Mastering file handling enables you to create applications capable of persistent data storage, thus enhancing their functionality. By practicing these concepts through small projects, you'll gain confidence in managing files and handling diverse data types in your C++ applications.

Further Reading and Resources
For those keen to learn more, there are numerous resources available, including books on C++ programming, online tutorials, and the official C++ documentation. These materials can provide deeper insights and additional examples that will further enhance your understanding of file handling in C++.