In C++, `#define` is a preprocessor directive used to create macros, allowing you to define constants or functions that can be easily reused throughout your code.
#define PI 3.14
#define SQUARE(x) ((x) * (x))
#include <iostream>
int main() {
std::cout << "The value of PI is: " << PI << std::endl;
std::cout << "The square of 5 is: " << SQUARE(5) << std::endl;
return 0;
}
Understanding `#define`
What is `#define`?
The `#define` directive is one of the preprocessor commands in C++. It allows developers to define macros, which can be simple constants or more complex expressions that can substitute values during the preprocessing stage of compilation. Preprocessor directives, including `#define`, are evaluated before the actual compilation takes place, meaning they can influence how the code behaves without being part of the compiled output.
Syntax of `#define`
The basic syntax of a `#define` statement is straightforward:
#define NAME VALUE
Here, `NAME` represents the name of the macro, and `VALUE` is the expression or value that `NAME` will be replaced with in the code.
For example, you can define PI as follows:
#define PI 3.14
In this case, whenever `PI` is encountered in the code, it will be replaced with `3.14` before the compilation process begins.
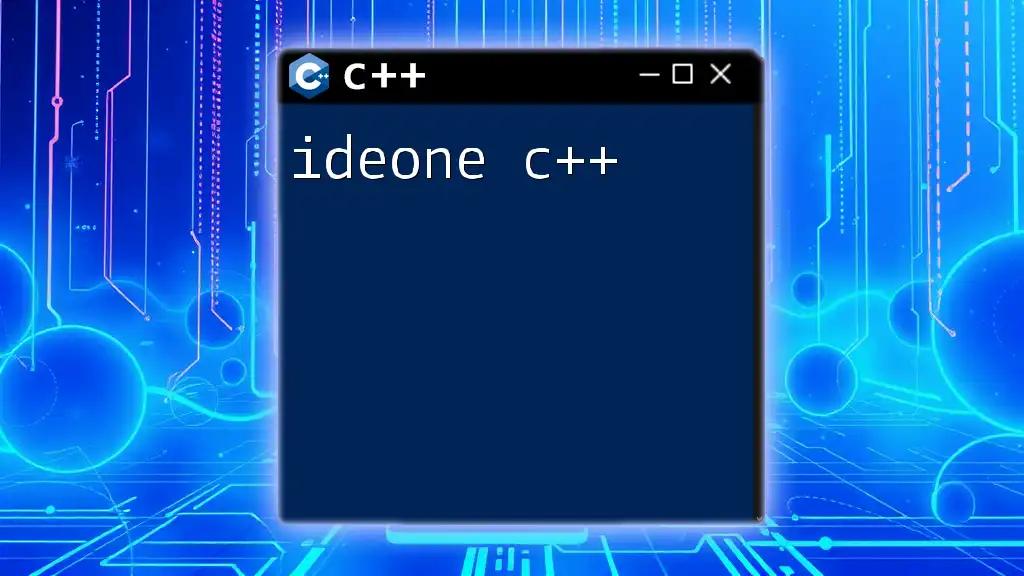
How `#define` Works in C++
Preprocessor Directives
Preprocessor directives are commands in C++ that give instructions to the compiler to preprocess the code before actual compilation happens. When you use `#define`, the preprocessor scans your code and substitutes occurrences of the defined name with its corresponding value, allowing for flexible code management and readability.
Use Cases of `#define`
Constants: One common use of `#define` is to create constant values that are used throughout your program. For example, if you need to define a constant maximum value for an array, you could do:
#define MAX_VALUE 100
This makes it easy to update the value in one place rather than hunting through the entire codebase for occurrences of `100`.
Code readability: By using descriptive macros, you can improve the readability of your code significantly. For example, instead of using numbers or less descriptive terms:
#define SPEED_OF_LIGHT 299792458 // in meters per second
You make it clear what the constant refers to, which is immensely beneficial for anyone reviewing or maintaining the code.
Conditional Compilation
`#define` can also be useful for conditional compilation, controlling which parts of your code get compiled based on certain conditions. This is particularly helpful for debugging or differentiating configurations.
For example, you can use it with `#ifdef` to include debugging code only when a specific macro is defined:
#define DEBUG
#ifdef DEBUG
std::cout << "Debugging Mode" << std::endl;
#endif
If `DEBUG` is defined, the output will show "Debugging Mode" during compilation; otherwise, it will be excluded.

Advantages of Using `#define`
Performance Benefits
Using `#define` can have significant performance implications. Since the preprocessor replaces the macro with its value directly in the code prior to compilation, it eliminates the overhead associated with variable storage and access at runtime. This is especially useful for constant values compared to using variables through the `const` keyword.
# define PI 3.14
const float constantPi = 3.14f; // This has storage overhead
In performance-critical sections, using `#define` can lead to minor efficiency gains.
Code Maintainability
One of the strongest advantages of using `#define` is the simplification of code maintenance. If you have a defined macro in your code and need to make a change, you do so in just one spot. For example, if you define a constant error code:
#define ERROR_OK 0
#define ERROR_NOT_FOUND 404
If you need to change `ERROR_NOT_FOUND` to another value, you only do it here once, instead of changing multiple occurrences throughout your code.

Limitations and Pitfalls of `#define`
No Type Safety
One critical limitation of `#define` is the lack of type safety. The preprocessor performs simple textual replacement without any regard for the underlying data types. This can lead to unintended consequences and bugs.
Consider the following example:
#define SQUARE(x) x * x
int area = SQUARE(5 + 1); // Misleading output due to operator precedence
In this case, the output of `area` might not yield the expected result because of how the expression is expanded. The recommended way to avoid this pitfall is using parentheses:
#define SQUARE(x) (x) * (x)
Debugging Challenges
Debugging macros defined by `#define` can be tricky. If a macro expands to many lines of code, it can obscure the source of errors. During debugging, the lack of proper stack traces or error messages associated with macros can complicate the process. To mitigate this, it's often a better practice to use `inline` functions or `const` variables when possible.

Alternatives to `#define`
Using `const`
While `#define` is powerful, it's not always the best option. For defining constants, using `const` provides type safety. Unlike `#define`, `const` enforces type consistency during compilation. Here's an example:
const float PI = 3.14f;
This effectively creates a constant float variable that cannot be modified.
Using `constexpr`
A more modern alternative is `constexpr`, which allows you to define variables that are both constant and can be evaluated at compile time, making them more versatile than `const`.
constexpr float PI = 3.14f;
With `constexpr`, the variable is treated like a compile-time constant, and the compiler performs checks that prevent unintended usage.
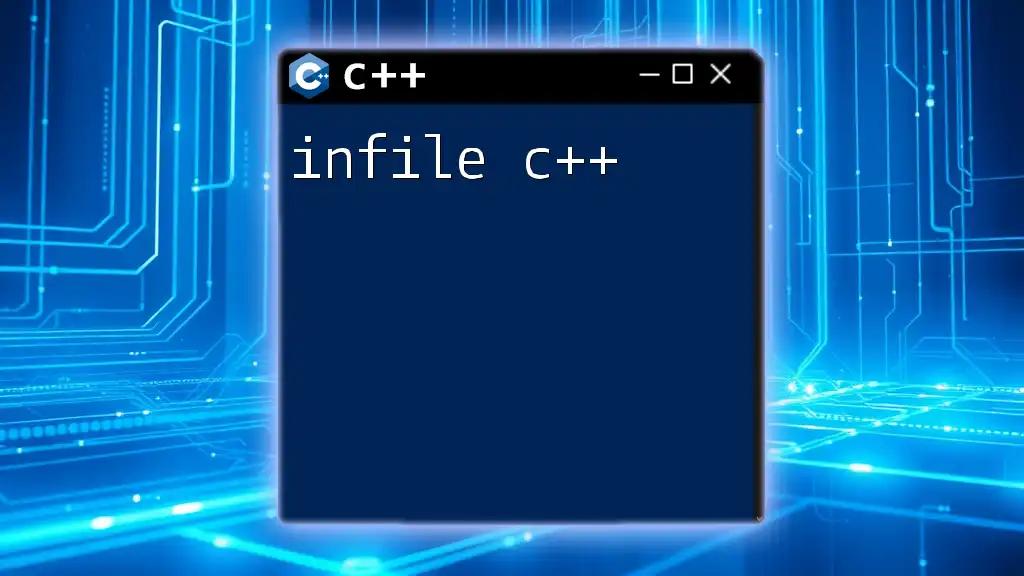
Best Practices for Using `#define`
Naming Conventions
When using `#define`, it is crucial to adopt proper naming conventions. Typically, uppercase letters and underscores (like `MAX_BUFFER_SIZE`) are used to distinguish macros from regular variables. This convention increases the likelihood of readability and reduces naming conflicts.
When to Use `#define`
Emphasize that `#define` is best used in specific scenarios, such as defining symbolic constants or enabling conditional compilation. If you find yourself needing complex expressions or type checks, consider using `const` or `constexpr` instead.
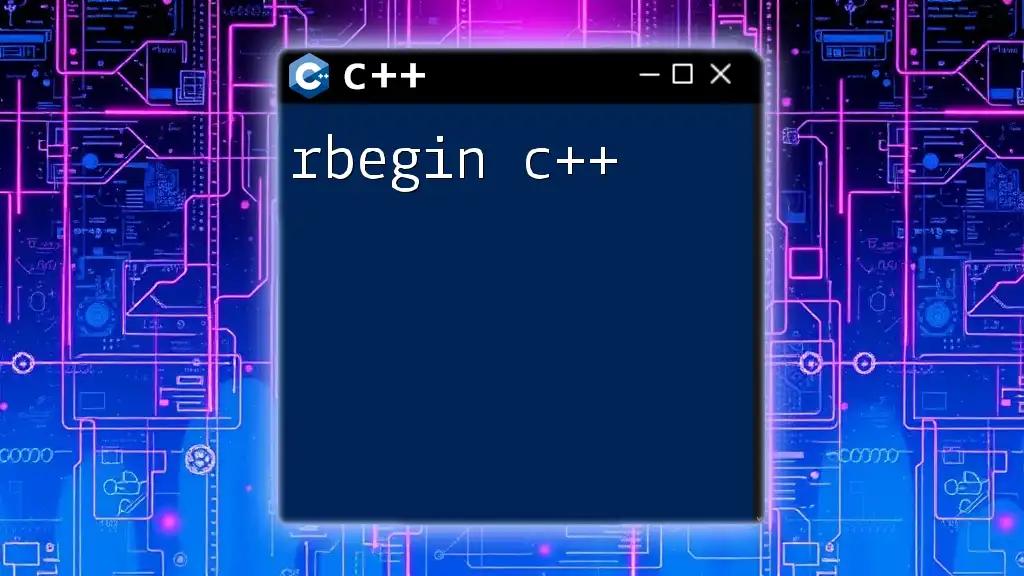
Conclusion
Understanding the functioning of the `#define` directive in C++ is critical for writing effective and maintainable code. While it offers flexibility and performance benefits, it also brings challenges, such as type safety issues and debugging complexities. Knowing when and how to use `#define`, along with its alternatives, can greatly enhance your programming skills and code quality.
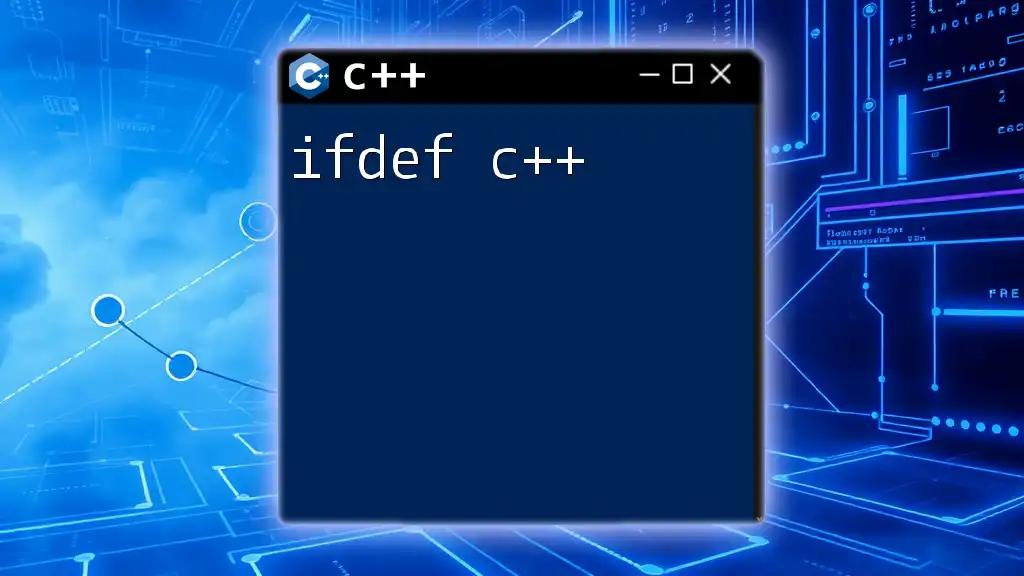
Additional Resources
For further learning on `#define` and preprocessor directives, refer to the official C++ documentation or explore various programming books and tutorials that focus on advanced C++ features to deepen your expertise.