In C++, `def` is not a keyword; however, you can define functions using the `return type functionName(parameters) { /* code */ }` syntax to encapsulate reusable code.
Here’s a simple example of defining a function in C++:
#include <iostream>
int add(int a, int b) {
return a + b;
}
int main() {
std::cout << "Sum: " << add(3, 4) << std::endl;
return 0;
}
Understanding Function Definitions
What is a Function?
A function is a reusable block of code that performs a specific task. In programming, functions enhance modularity, allow code to be reused efficiently, and improve readability. By breaking down code into smaller, manageable sections, functions make it easier to debug and maintain.
Components of a Function Definition
Return Type
The return type of a function defines the type of value that will be returned after the function execution. This can vary; common return types include `int`, `float`, `char`, and `void`.
For example, if a function is intended to return an integer value, you would define it as follows:
int square(int number) {
return number * number;
}
This function calculates the square of a number and returns an integer result.
Function Name
Function names are identifiers that must be unique within a given scope. Good naming conventions in programming allow the code to be self-explanatory. Avoid using generic names; instead, opt for descriptive names that communicate the function's purpose clearly, such as `calculateSum` instead of `func1`.
Parameters
Parameters are inputs that a function can take. They allow functions to be flexible and reusable. There are two main types of parameter passing:
- Pass by Value: A copy of the argument is passed to the function. Changes made to the parameter value do not affect the original argument.
- Pass by Reference: A reference to the original argument is passed, allowing the function to modify the argument's value.
Here’s an example demonstrating both types:
void modifyByValue(int value) {
value = 10; // This won't affect the original variable
}
void modifyByReference(int &value) {
value = 10; // This will affect the original variable
}
Function Body
The function body contains the set of statements that define what the function will do. It is enclosed within braces `{}`. Here's an example of a function body in context:
int multiply(int a, int b) {
return a * b; // Function body performing multiplication
}
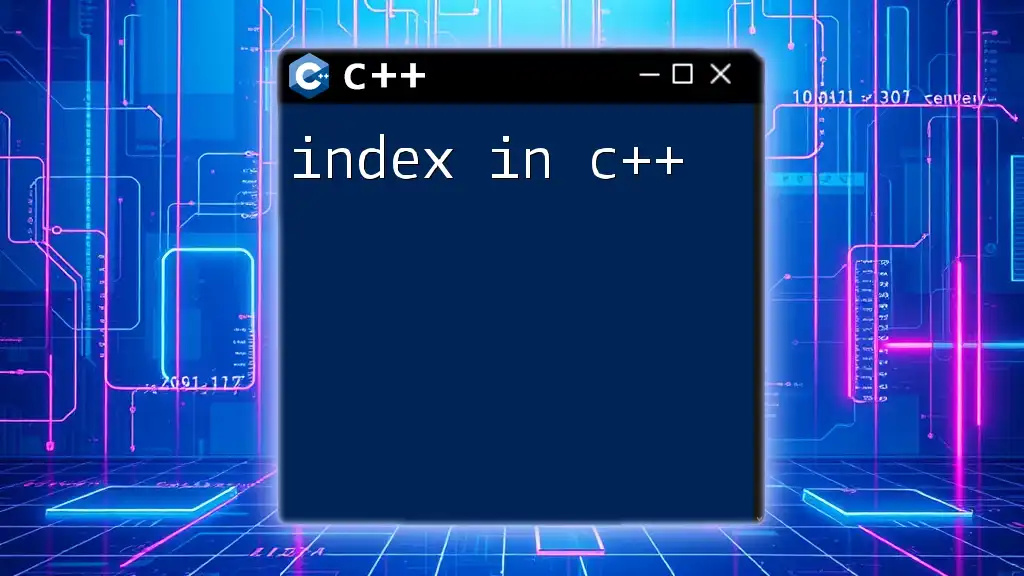
Syntax of Function Definition in C++
The basic syntax for defining a function in C++ is structured as follows:
return_type function_name(parameter_list) {
// function body
}
This concise definition captures the essentials of defining functions in C++.
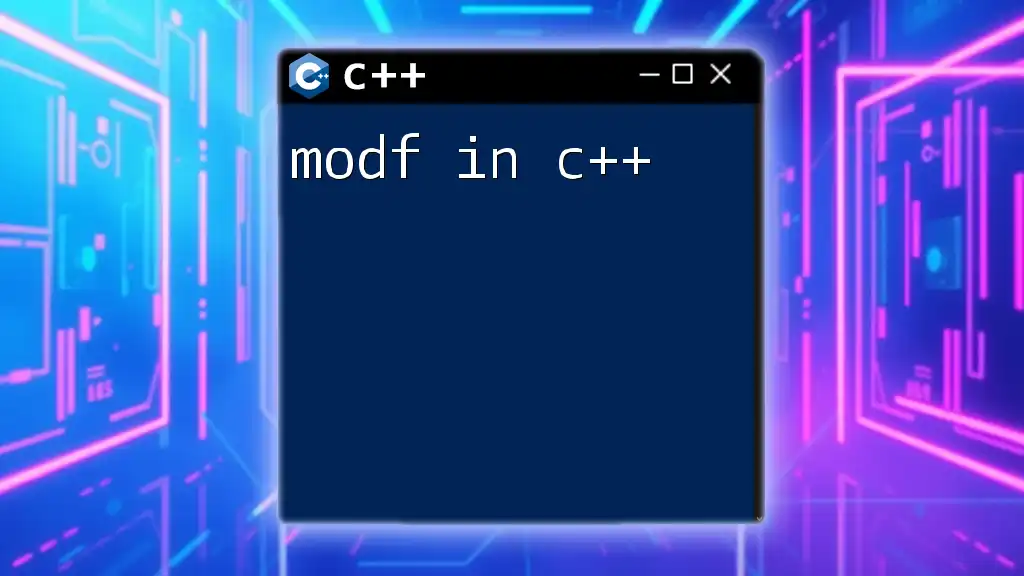
Creating Your First Function
Step-by-Step Example
To create your first function, let’s consider a simple example where we define a function that adds two numbers.
int add(int a, int b) {
return a + b; // Return the sum of a and b
}
Calling Your Function
Once the function is defined, you can call it in your main program.
#include <iostream>
using namespace std;
int add(int a, int b) {
return a + b;
}
int main() {
int result = add(5, 3); // Calling the function
cout << "The sum is: " << result << endl; // Output: The sum is: 8
return 0;
}
In this example, the function `add` takes two integers as input and returns their sum, demonstrating the complete process of function definition and invocation in C++.
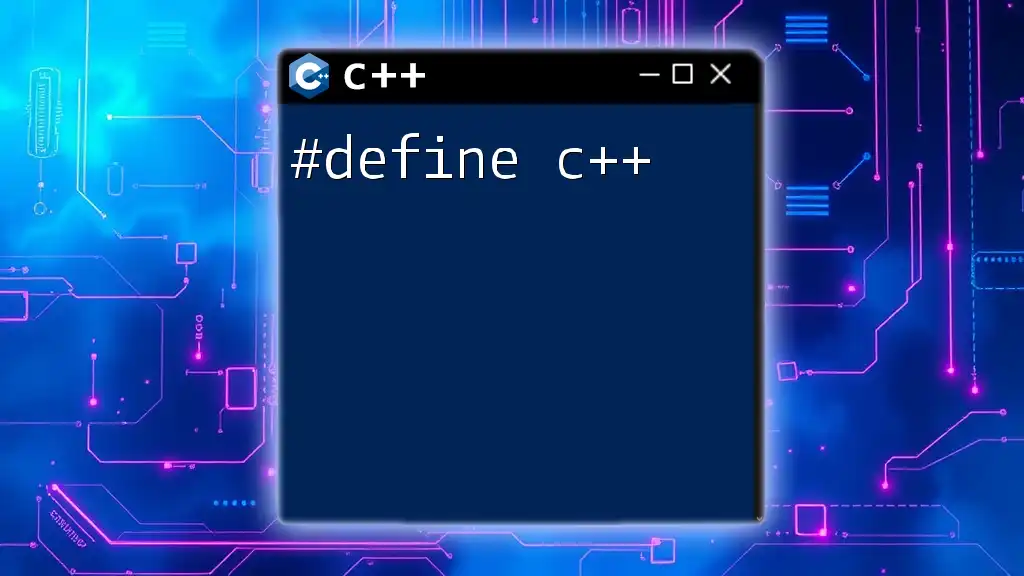
Types of Functions
Built-in vs User-defined Functions
C++ includes a variety of built-in functions that simplify development, such as mathematical operations and string manipulations. In contrast, user-defined functions are created by the programmer to perform specific tasks tailored to the application’s needs.
Overloaded Functions
Function overloading allows multiple functions to have the same name but different parameter lists. This is useful for performing similar operations with different types or numbers of arguments.
int add(int a, int b) {
return a + b;
}
double add(double a, double b) {
return a + b;
}
Here, we have two functions named `add`, one for integers and another for doubles. C++ differentiates between them based on the types of parameters passed.
Inline Functions
Defining inline functions allows the compiler to insert the function's code directly into the calling code, which can reduce the overhead of a function call.
inline int square(int x) {
return x * x; // Directly replace calls to this function with this expression
}
Using inline functions can optimize performance, especially for small, frequently called functions.
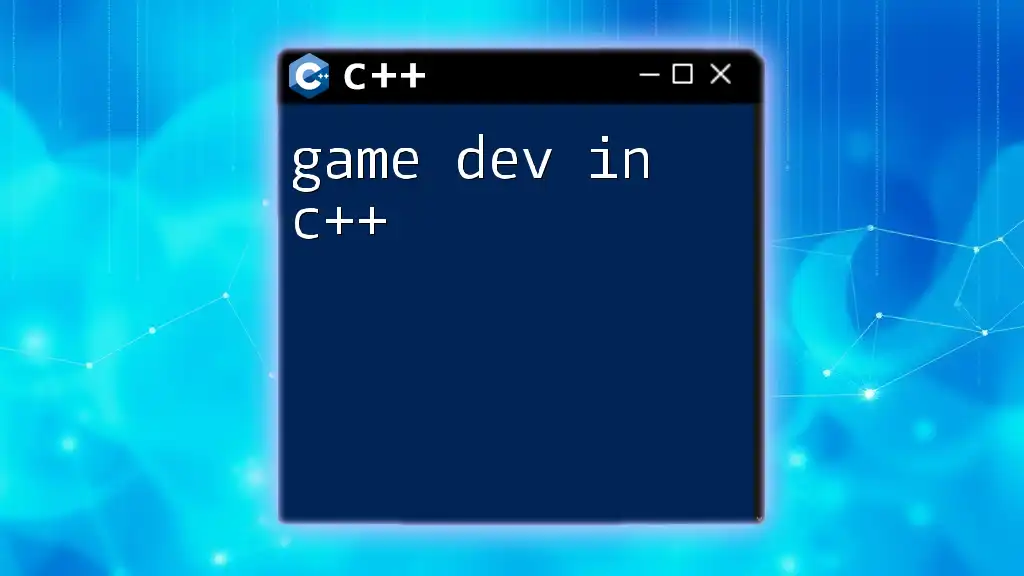
Best Practices for Function Definitions
Naming Conventions: Develop a consistent naming strategy. Using verbs in function names can clarify their purpose, such as `computeTotal` or `fetchData`.
Avoiding Global Variables: Keep functions self-contained. Minimizing the reliance on global variables enhances function reusability and reduces unintended side effects.
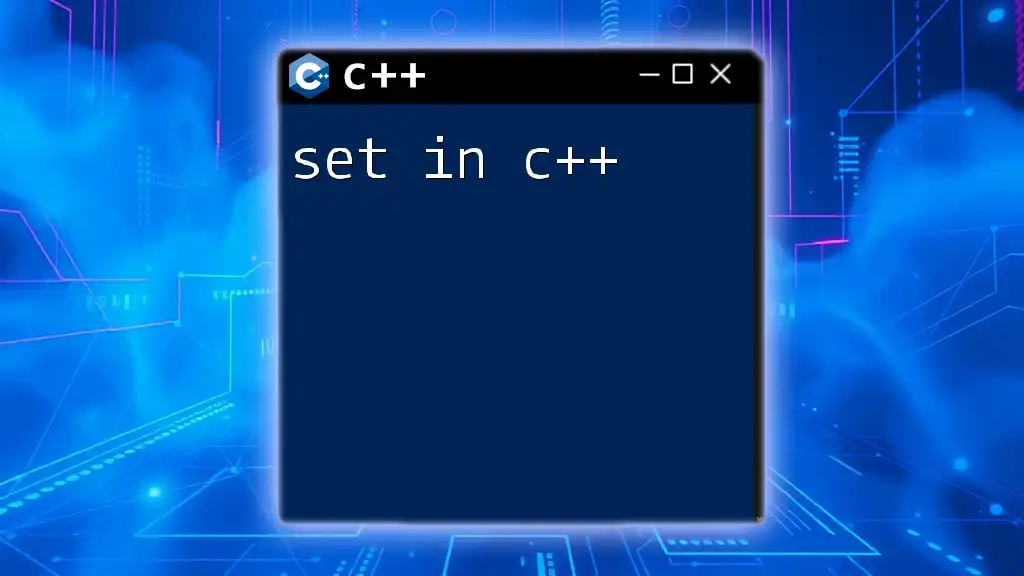
Common Errors in Function Definitions
Compile-time Errors
Compile-time errors often arise from mismatched return types, incorrect parameters, or syntax mistakes. For example, forgetting to specify the return type may lead to compilation failure:
function errorFunction() { // Missing return type
return 42;
}
Runtime Errors
Runtime errors may occur when a function is called with invalid arguments. Always validate inputs to prevent errors during execution.
int divide(int a, int b) {
if (b == 0) {
throw runtime_error("Division by zero not allowed."); // Handle potential runtime error
}
return a / b;
}
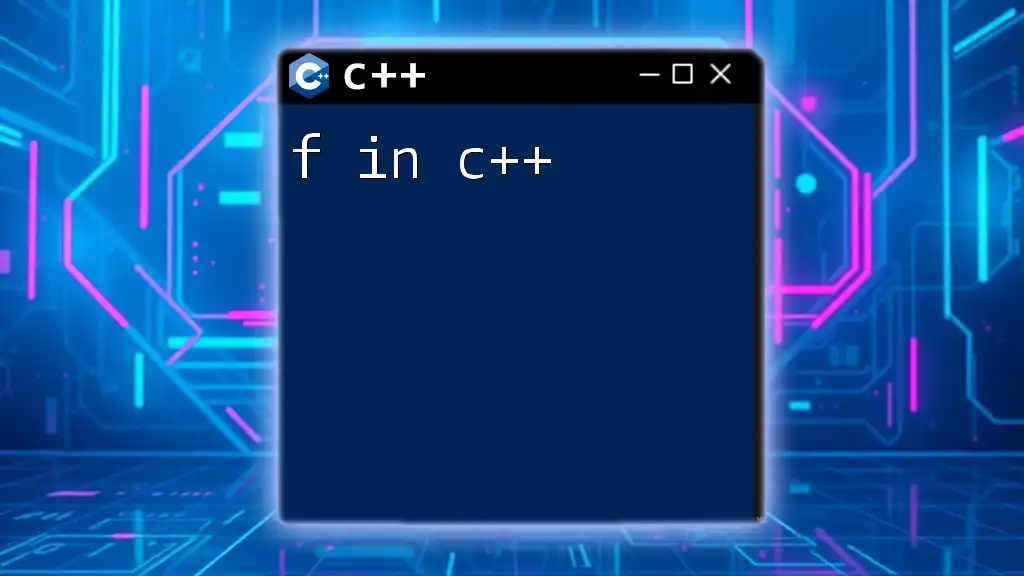
Advanced Topics
Lambda Functions
C++ introduced lambda functions for defining unnamed functions in a concise way. Lambdas are particularly useful for passing functions as arguments.
auto add = [](int a, int b) {
return a + b; // Lambda function for addition
};
Templates in Function Definitions
Function templates allow you to define functions that can operate on different data types. This feature makes your code more flexible and reusable.
template <typename T>
T add(T a, T b) {
return a + b; // Implementing a function template
}
Using templates, this single `add` function can operate with any data type that supports the addition operator, showcasing the power of generic programming.
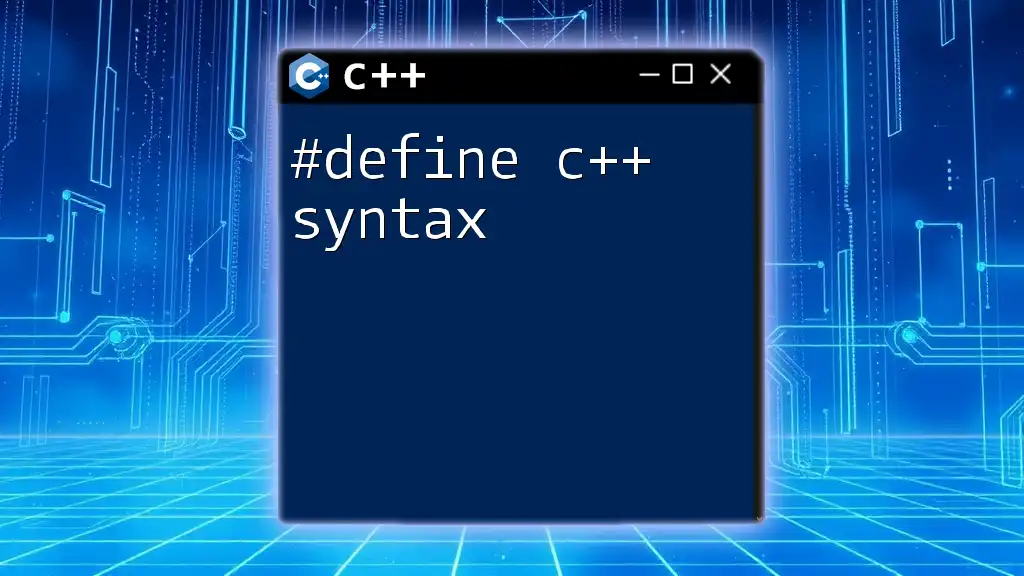
Conclusion
Function definitions in C++ are fundamental to creating efficient and maintainable code. Understanding the components of a function, the correct syntax, and best practices will help you leverage the power of C++. Continuous practice in defining and using functions will strengthen your programming skills and increase your fluency in C++.
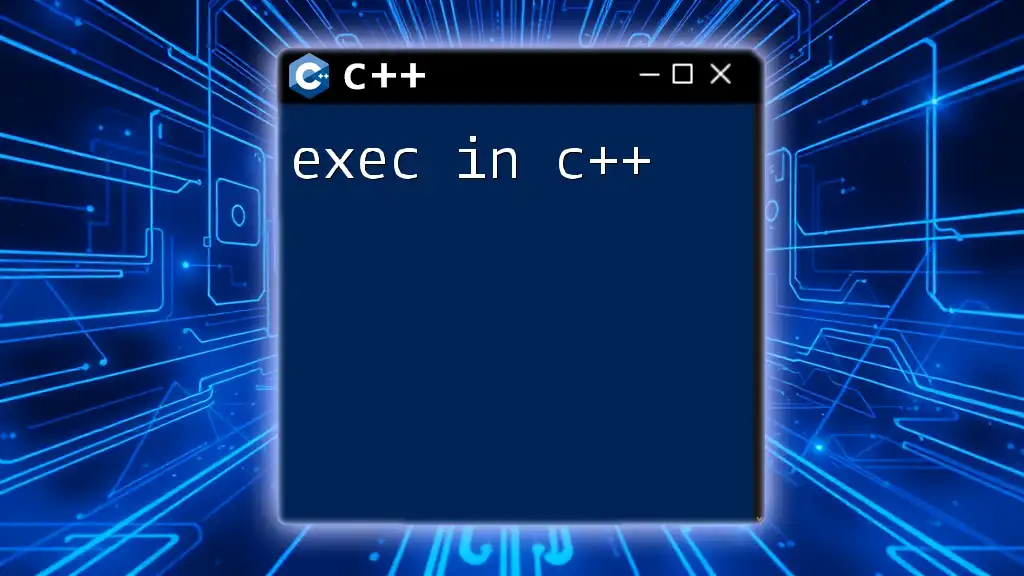
Additional Resources
For further learning, refer to recommended books, courses, and online platforms focusing on C++. Engaging with community problems on platforms like LeetCode or HackerRank can also enhance your understanding of C++ functions.
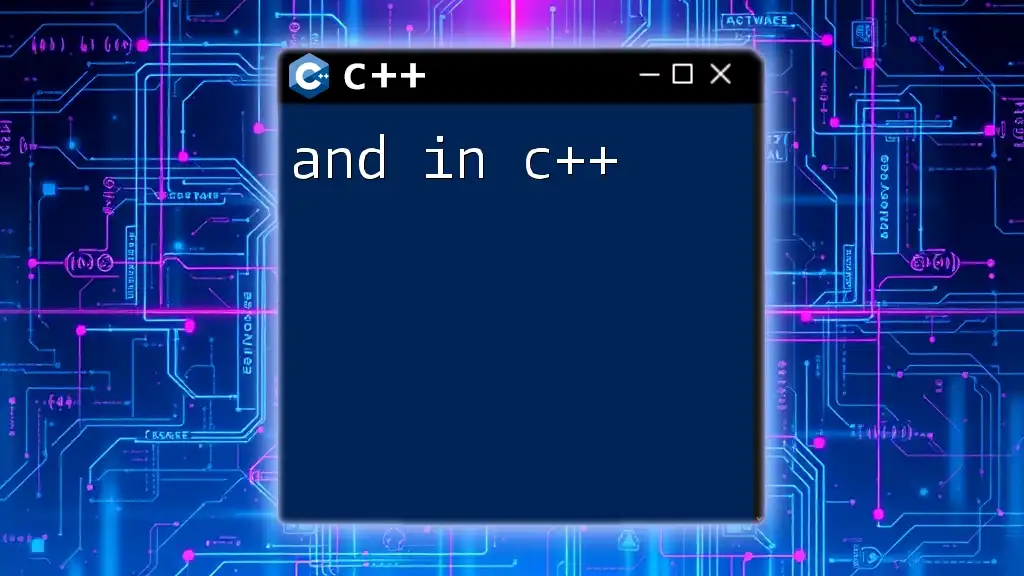
FAQs
Commonly Asked Questions About Functions in C++
-
What is the difference between a function declaration and definition? A declaration specifies the function's name, return type, and parameters, while a definition provides the implementation.
-
Can functions be defined inside other functions? Yes, C++ allows nested functions, but it is not commonly used as it may lead to complex and hard-to-maintain code.
This comprehensive guide on "def in C++" should provide you with key insights and practical examples to understand and apply function definitions effectively in your C++ programming endeavors.