In C++, `void` is a keyword used to specify that a function does not return a value.
#include <iostream>
void greet() {
std::cout << "Hello, World!" << std::endl;
}
What is `void` in C++?
In C++, `void` is a keyword that signifies the absence of a value. It is primarily used in the context of function declarations and helps to define the return type of a function. When a function returns `void`, it indicates that the function does not return any data to the calling code. This is in contrast to standard data types like `int`, `float`, or `char`, which specify that the function will return a value of that type.
For example, a function declared with a return type of `int` is expected to return an integer value, while a function declared with a return type of `void` signals that it completes some operations without yielding a return value.
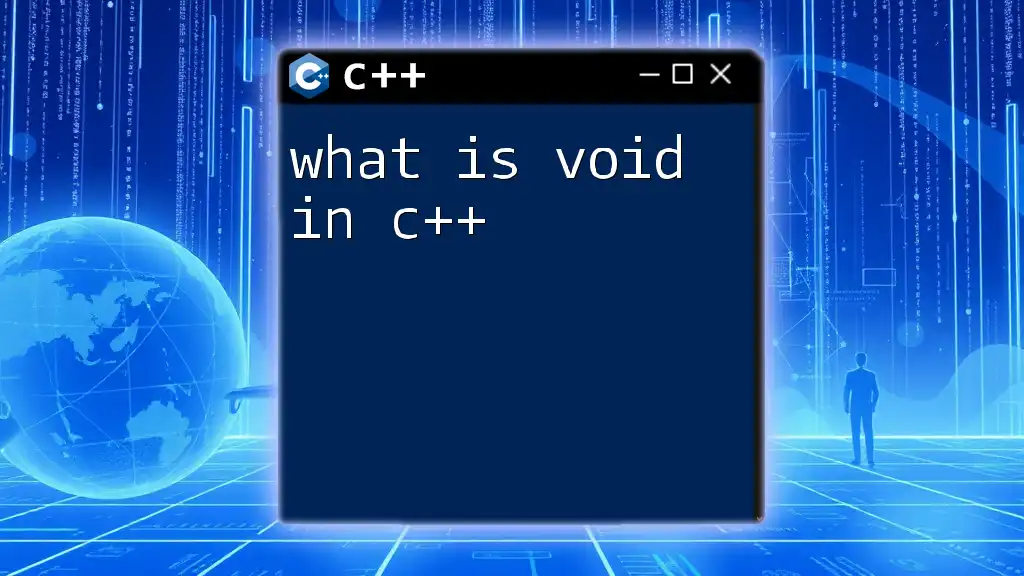
What Does `void` Do in C++?
The primary role of `void` in C++ is to define function signatures, indicating that a function will not return any value to the caller. This is useful for functions that perform tasks such as displaying output or modifying data without needing to send a result back.
Example: A Simple Function that Uses `void`
void printMessage() {
std::cout << "Hello, world!" << std::endl;
}
In this example, the `printMessage` function does not return any value; it simply performs an action—printing a message to the console.
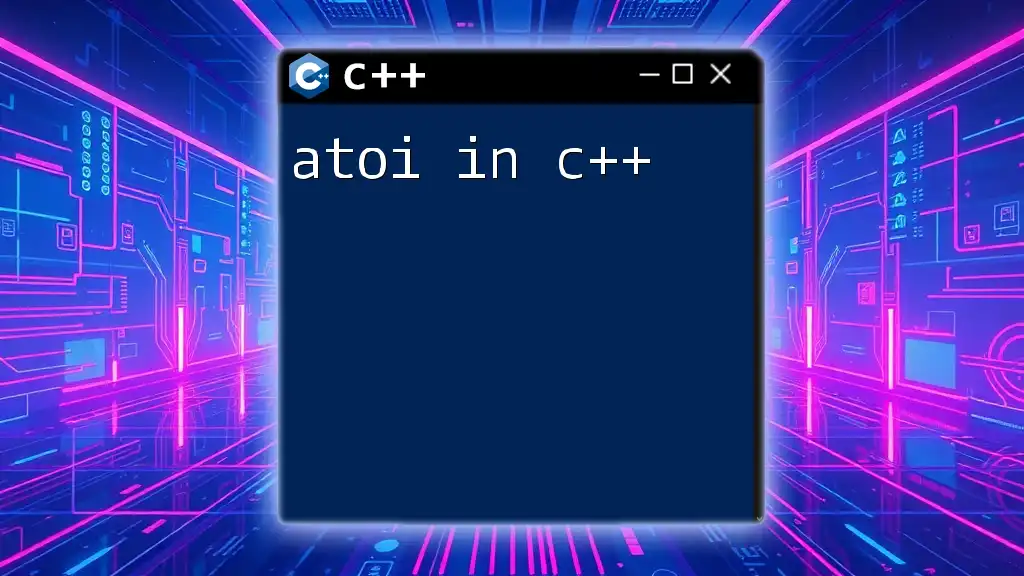
Using `void` in Function Definitions
A function's signature, including its return type, is essential for indicating how the function behaves. By using `void` as the return type, you clarify to other developers and future maintainers of your code that the function is intended purely for side effects, not value computation.
Example: Creating and Calling a `void` Function
void greetUser(std::string name) {
std::cout << "Welcome, " << name << "!" << std::endl;
}
int main() {
greetUser("Alice"); // Output: Welcome, Alice!
return 0;
}
In this example, `greetUser` is a `void` function that takes a `string` parameter and displays a greeting. The absence of a return value is clear to anyone reading the code.
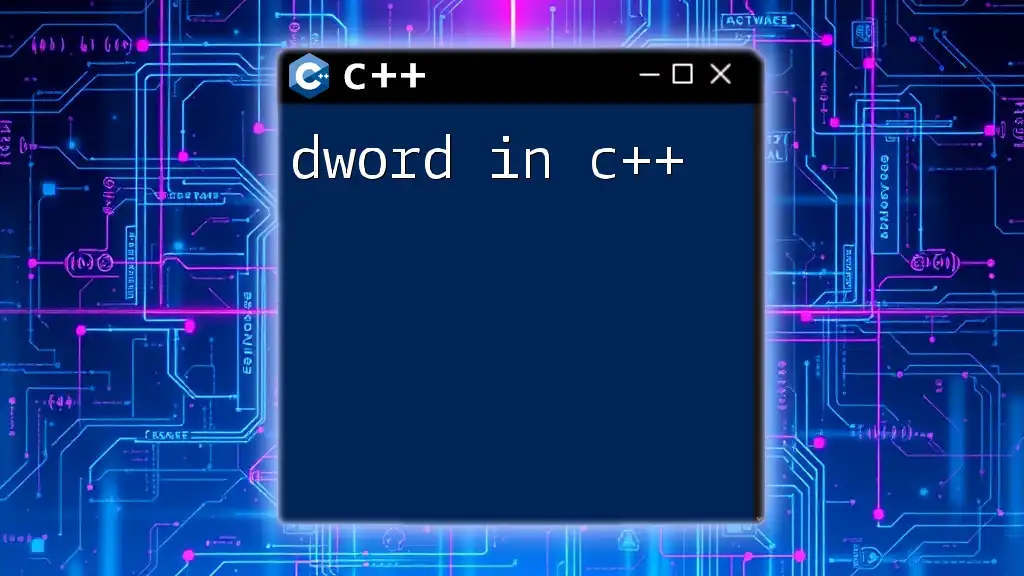
The Role of `void` in Function Arguments
Functions can also have `void` as a parameter type, although it is rare. More commonly, you might encounter `void` pointers, which can point to any data type. However, the concept of using `void` in function arguments can signify that the function does not require parameters.
Example: A Function That Doesn't Take Any Parameters
void showMenu() {
std::cout << "1. Start Game\n2. Exit\n";
}
Here, the `showMenu` function does not take any parameters and simply displays menu options.
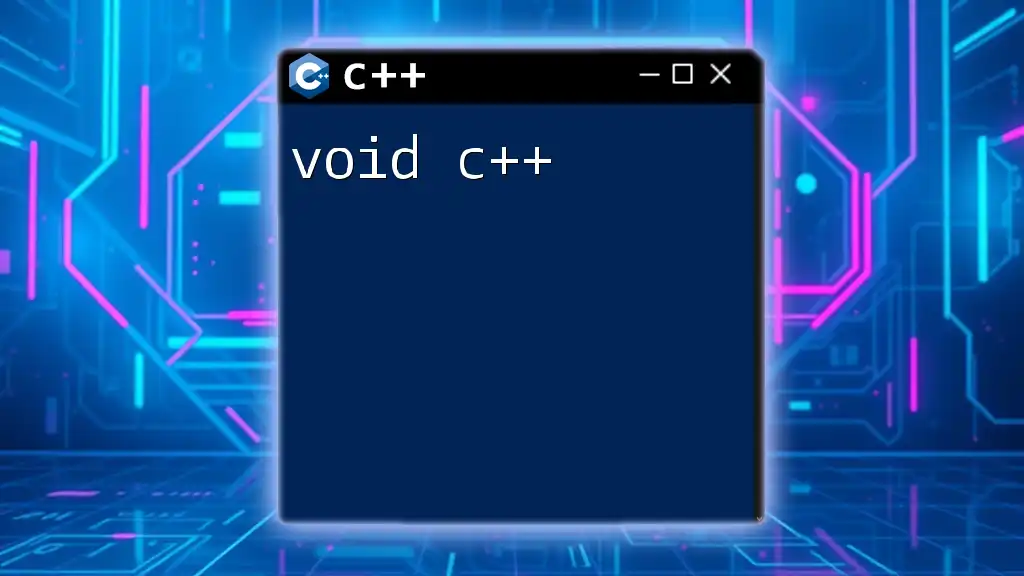
Understanding `void` Pointers
A more advanced use of `void` in C++ is its role as a pointer. A `void*` pointer can hold the address of any data type, providing a flexible mechanism for generic programming. However, when using `void` pointers, caution is necessary since the compiler does not know the type it is pointing to without explicit casting.
Example: Using a `void*` Pointer
void* ptr;
int value = 10;
ptr = &value; // A void pointer can hold the address of any data type
In this example, `ptr` is a `void` pointer that can refer to an `int`, but you would need a cast to perform operations on it:
int dereferencedValue = *static_cast<int*>(ptr); // Correctly casting and dereferencing
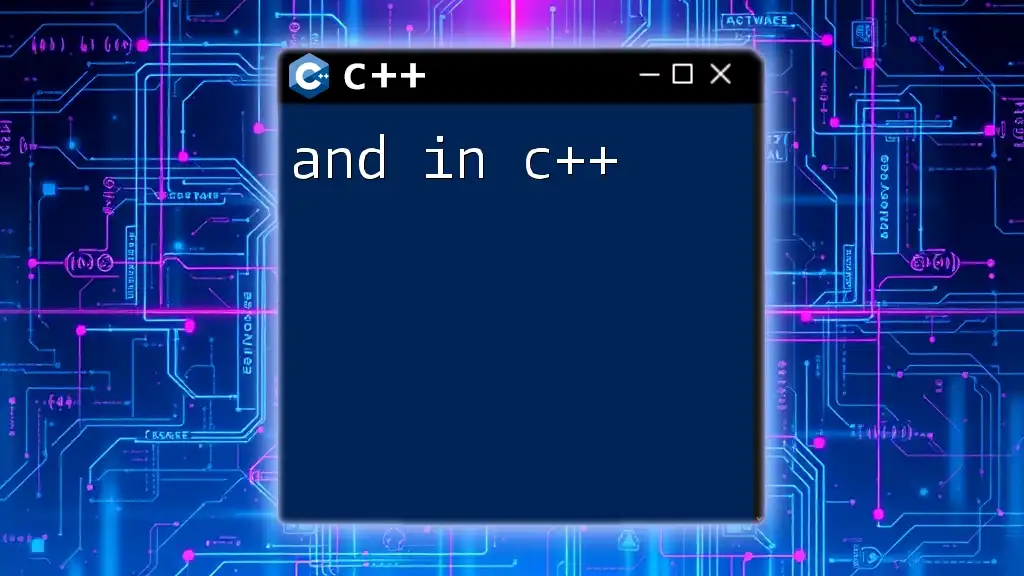
Advantages of Using `void`
Using `void` in C++ functions offers several advantages:
- Flexibility: Functions declared as `void` can be focused purely on side effects like printing to the console or modifying global state without the need to return a value.
- Code Readability: The use of `void` can improve the readability of the code by clearly distinguishing between functions that return values and those that do not.
Example: Using `void` for Callback Functions
Callbacks are functions passed as arguments to other functions, and they often use `void` for their return type since they are typically executed for their side effects.
void callback() {
std::cout << "Callback executed!" << std::endl;
}
void executeCallback(void (*func)()) {
func();
}
int main() {
executeCallback(callback); // Output: Callback executed!
return 0;
}
In this scenario, `executeCallback` accepts a function pointer to a `void` function, illustrating how `void` supports flexible function behavior.
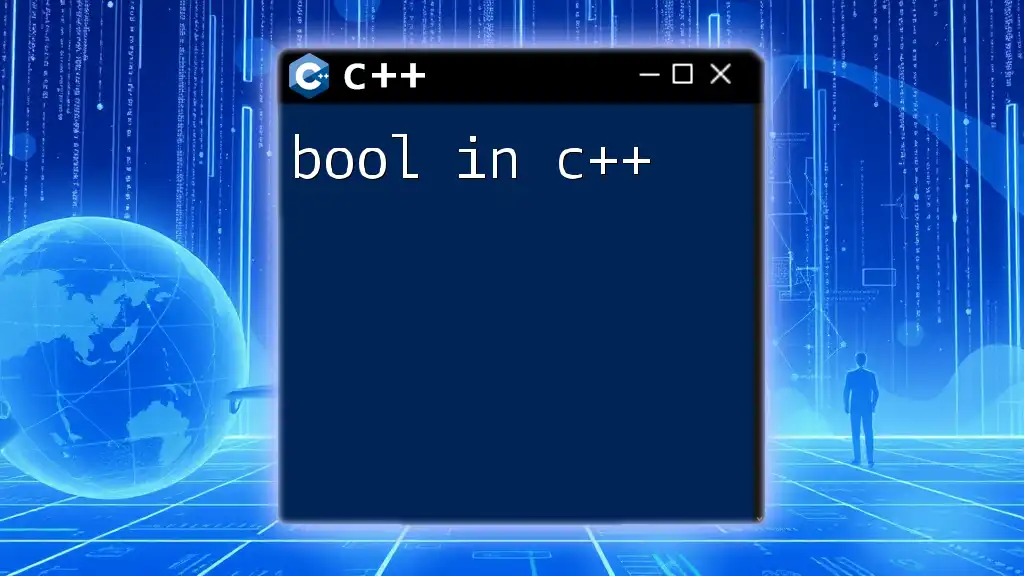
Limitations of `void`
While `void` is useful, it does come with certain limitations. One key point is that a `void` function cannot return a value. Attempting to return a value from a `void` function will result in a compilation error.
Example: Avoiding the Return of Values from a Void Function
void returnValueExample() {
return 42; // This will cause a compilation error
}
Here, trying to return an integer from a `void` function is invalid and should be corrected by either removing the return statement or changing the function's return type.
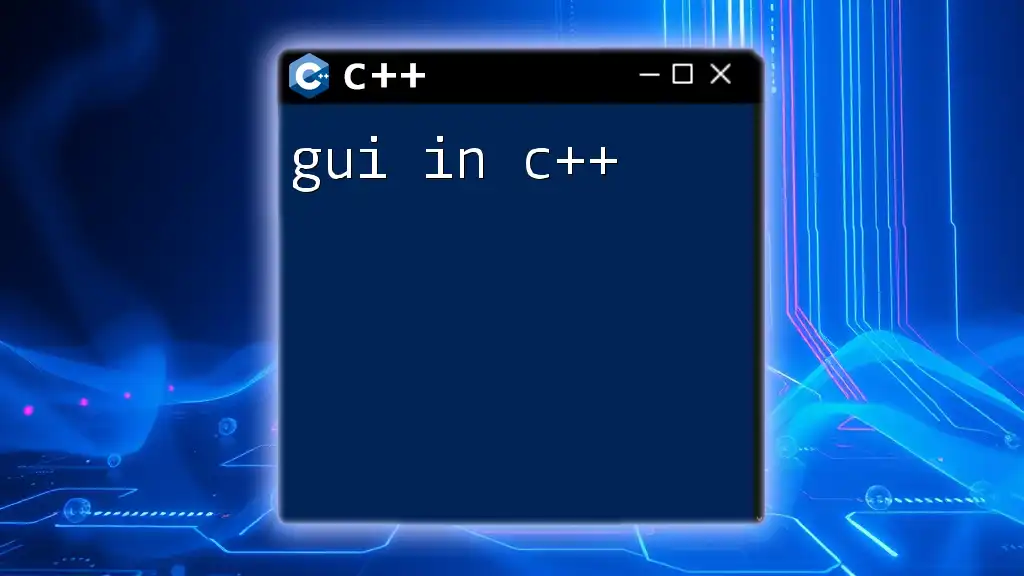
Summary of `void` in C++
In summary, understanding `void` in C++ is crucial for programmers who want to master function definitions and usage. It clarifies the intention of a function—whether it performs actions without returning data. From defining function signatures to implementing flexible function pointers, `void` enhances the expressiveness of your code.
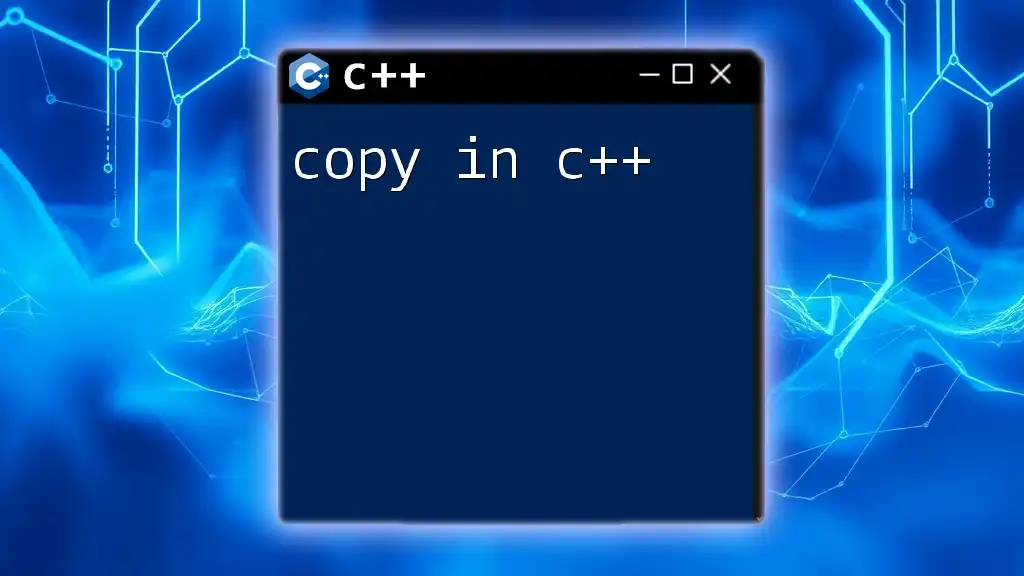
Conclusion
The concept of `void` in C++ is not just a syntactical detail; it plays an important role in shaping how functions interact and behave in your programs. Grasping how to utilize `void` effectively can lead to better-designed functions and cleaner, more maintainable code.
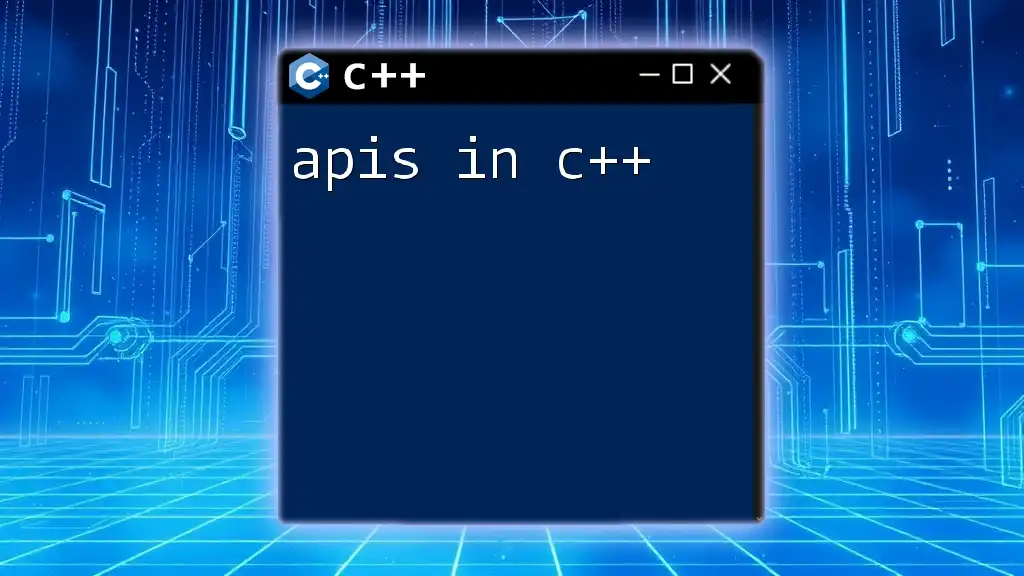
Call to Action
Now that you have a foundational understanding of `void` in C++, I encourage you to experiment with this keyword in your own projects. Try creating `void` functions, using `void` pointers, and implementing callbacks to see firsthand the power and utility they can provide. Continue your exploration of C++ to deepen your knowledge of functions and data types!