In C++, the `sin` function is used to calculate the sine of an angle (in radians) and requires the `<cmath>` header for proper functionality.
#include <iostream>
#include <cmath>
int main() {
double angle = 1.0; // Angle in radians
double sineValue = sin(angle);
std::cout << "The sine of " << angle << " is: " << sineValue << std::endl;
return 0;
}
What is the Sine Function?
Definition of the Sine Function
The sine function is a fundamental trigonometric function that relates the angle of a right triangle to the ratio of the length of its opposite side to the hypotenuse. It is mathematically defined as:
\[ \text{sine}(\theta) = \frac{\text{opposite}}{\text{hypotenuse}} \]
This function can also be understood through the unit circle, where the sine of an angle corresponds to the y-coordinate of the point on the circle.
Applications of the Sine Function
The sine function is vital across various fields:
- In physics, it models oscillatory phenomena such as sound waves, light waves, and mechanical vibrations.
- In engineering, it is used in signal processing and electrical engineering to analyze alternating current circuits.
- In computer graphics, sine functions help in creating animations and modeling rotations, adding realism to animations through smooth transitions.
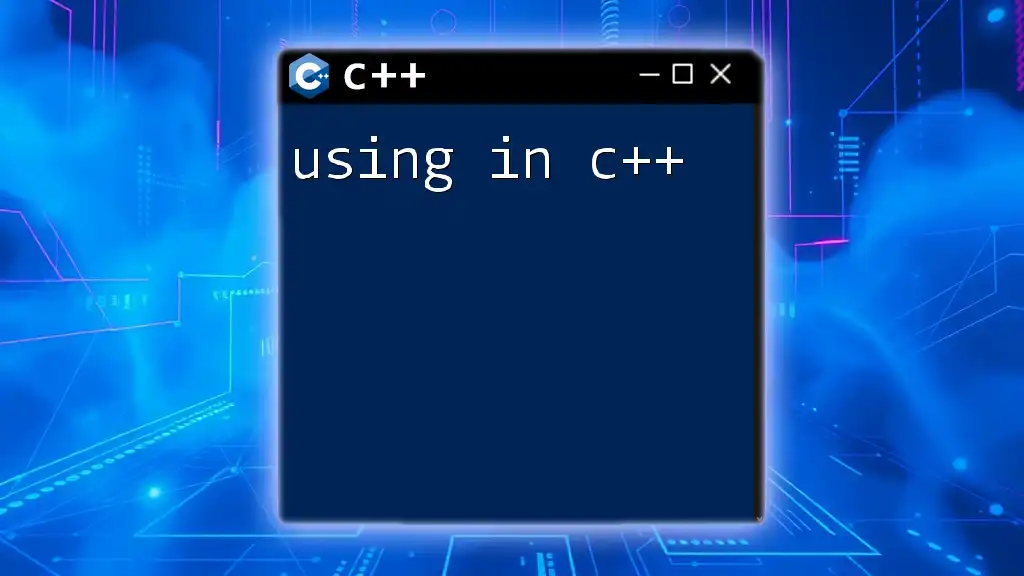
The sin Function in C++
Understanding the C++ sin Function
In C++, the sine function can be accessed using the `sin` function, which is part of the cmath header file. The function takes an angle in radians as an argument and returns its sine.
To use the `sin` function, ensure you include the header at the beginning of your code:
#include <cmath>
Syntax and Return Type
The syntax of the C++ sine function is straightforward:
double sin(double x);
- Parameter: `x` is the angle in radians.
- Return Type: The function returns a `double` that represents the value of the sine of the angle.
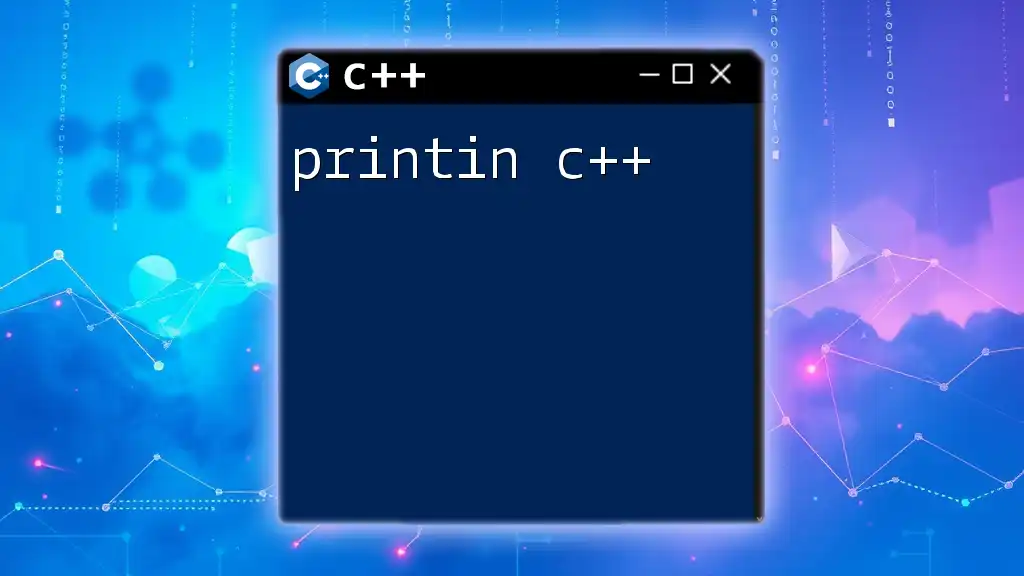
How to Use the Sine Function in C++
Basic Example of Using the sin Function
To effectively use the sine function, you often need to convert degrees to radians because the `sin` function in C++ requires the input to be in radians. Here's a simple example:
#include <iostream>
#include <cmath>
int main() {
double angle = 30.0; // degrees
double radians = angle * (M_PI / 180.0); // convert to radians
double sineValue = sin(radians);
std::cout << "Sine of " << angle << " degrees is: " << sineValue << std::endl;
return 0;
}
In this example, the angle of `30 degrees` is converted to radians by multiplying it by \(\frac{\pi}{180}\). The returned sine value is then displayed.
Advanced Examples
Example: Sine Wave Generation
The sine function can be used to generate a set of values that represent a sine wave. This can be especially useful in physics simulations or graphic visualizations. Below is an example that prints out the sine values for angles from `0` to `2π`:
#include <iostream>
#include <cmath>
int main() {
for (double x = 0; x < 2 * M_PI; x += 0.1) {
std::cout << "sin(" << x << ") = " << sin(x) << std::endl;
}
return 0;
}
This loop iterates over values of `x`, printing the sine of each value, which illustrates how the sine function behaves in a complete cycle.
Example: Sine Function in Animation
In animation, the sine function can animate the smooth motion of an object, such as creating a simple back-and-forth motion. Below is a conceptual example that illustrates this concept. Note that in a real-world scenario, this might be integrated into a graphics rendering loop rather than as a standalone program.
#include <iostream>
#include <cmath>
#include <unistd.h> // for sleep function
int main() {
for (double t = 0; t < 10; t += 0.1) {
double position = 10 * sin(t); // amplitude of 10
std::cout << "Object position at time " << t << ": " << position << std::endl;
usleep(100000); // wait for 0.1 seconds
}
return 0;
}
In this example, the object's position varies sinusoidally over time, demonstrating the potential of the `sin` function in simulating periodic motion.
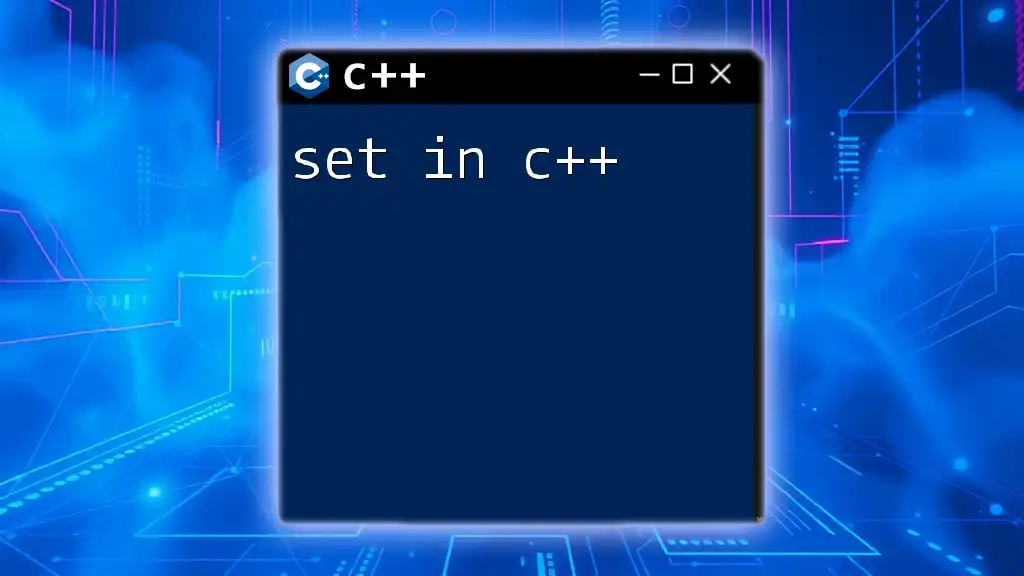
Understanding Trigonometric Functions in C++
Other Trigonometric Functions
Aside from the sine function, C++ provides several other trigonometric functions, including `cos` (cosine) and `tan` (tangent). These functions can be utilized similarly to calculate the respective values for given angles.
While the sine function gives insight into the vertical component of a triangle, the cosine function provides the horizontal component, whereas tangent represents the slope. Understanding all three functions enhances the mathematical capabilities of your programs.
Inverse Sine Function
C++ also offers the inverse sine function, commonly referred to as `asin`. This function can find the angle (in radians) corresponding to a given sine value:
#include <iostream>
#include <cmath>
int main() {
double value = 0.5; // sine value
double angleInRadians = asin(value);
std::cout << "Angle (in radians) corresponding to sine value " << value << " is: " << angleInRadians << std::endl;
return 0;
}
The `asin` function is significant when you need to determine angles from sine values, especially in applications like solving triangles.
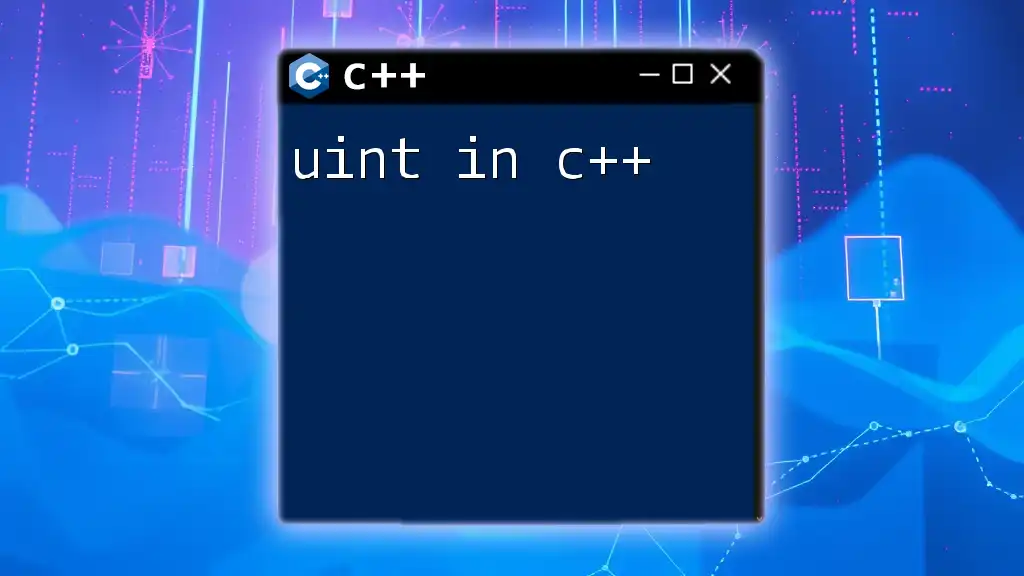
Common Errors and Debugging Tips
Common Mistakes When Using the Sine Function
When using the sine function, beginners often overlook essential details:
- Degree to Radian Conversion: Forgetting to convert degrees into radians is a common mistake, leading to incorrect sine values.
- Input Value Limits: Misunderstanding the range of sine outputs, which always remains between -1 and 1, can lead to logic errors in calculations.
Debugging Tips
To help avoid and correct mistakes, consider implementing the following strategies:
- Use output statements throughout your code to check intermediate values, ensuring your calculations are correct and aligned with expectations.
- Always ensure you're aware of the mathematical domain and range of the sine function, which can help prevent logical errors in larger algorithms or simulations.
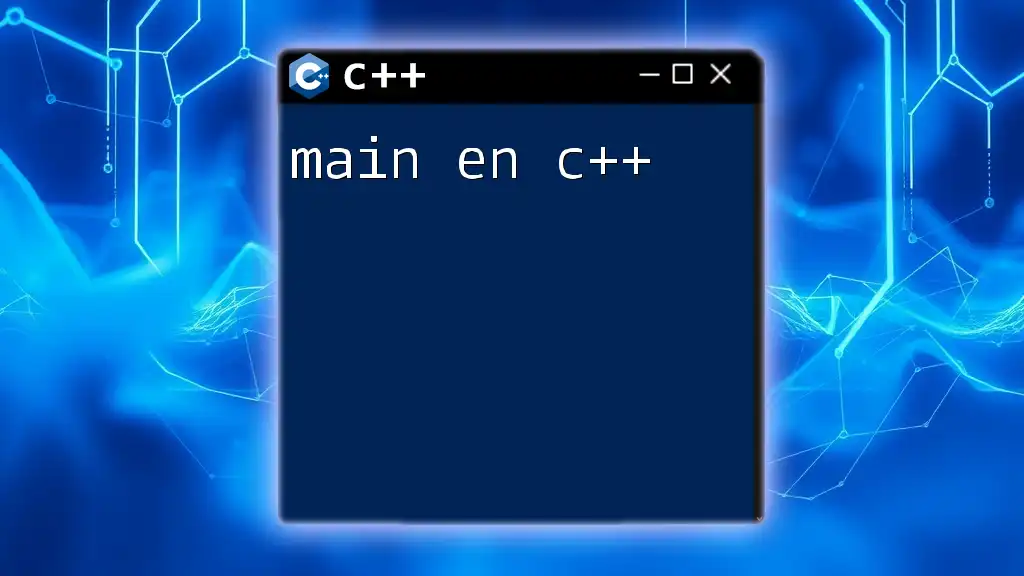
Conclusion
The sine function, represented as sin in C++, is a powerful tool for both mathematical computations and practical applications in various fields. Understanding and utilizing this function effectively allows for robust programming, particularly in physics simulations and animations.
Encouraging further experimentation with the sine function not only solidifies your grasp of trigonometry in programming but also opens up new avenues for creativity and problem-solving in your projects. Whether in graphics or simulations, mastering the sine function is crucial for any aspiring C++ developer.
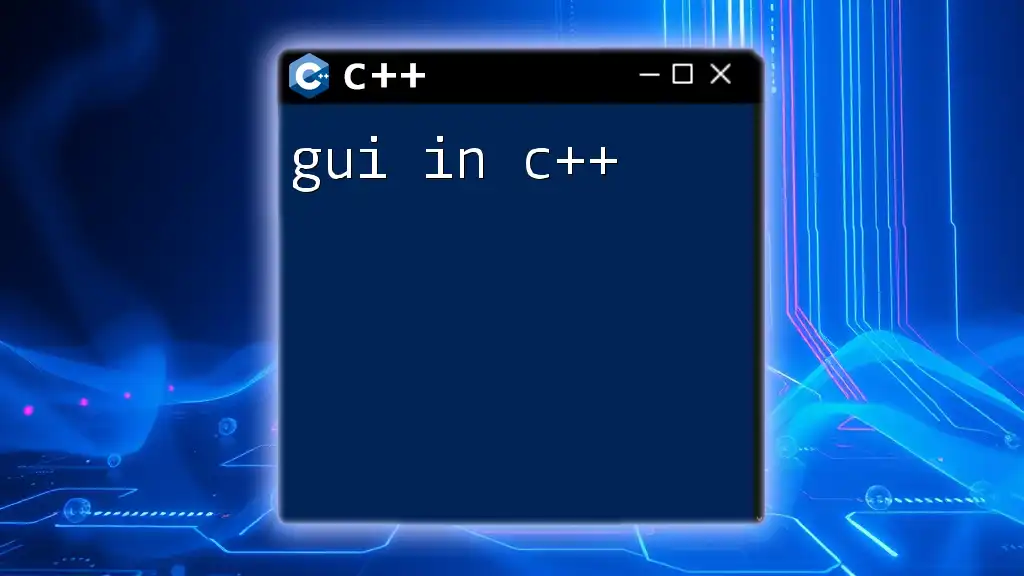
Additional Resources
Recommended Reading
For additional learning, consider exploring the official documentation on the C++ standard library, which provides extensive information on mathematical functions.
Online Tools for Practice
Utilize online compilers to practice C++ syntax and functionality for the sine function. Interactive graphing tools can also help visualize sine waves, enriching your understanding of its behavior.
By actively engaging with these resources, you can deepen your knowledge and enhance your programming skills in C++.