In C++, a common way to handle failures, such as an unsuccessful memory allocation, is to check if the pointer is `nullptr` after attempting to allocate memory.
Here's a simple example:
#include <iostream>
int main() {
int* ptr = new(std::nothrow) int[10]; // Attempt to allocate memory
if (ptr == nullptr) { // Check for failure
std::cerr << "Memory allocation failed!" << std::endl;
return 1; // Exit the program indicating failure
}
// Code to use the allocated memory…
delete[] ptr; // Don't forget to free memory
return 0;
}
Common Types of Failures in C++
Syntax Errors
Syntax errors are the most elementary type of errors programmers encounter when coding in C++. These errors stem from violations of the language's grammar rules and can prevent the code from compiling altogether. Common examples include forgetting to add semicolons at the end of statements or mismatching brackets.
Example of a syntax error:
int main() {
int a = 5
return 0;
}
In this snippet, the omission of the semicolon after `int a = 5` once attempted to compile will result in an error due to the incomplete statement.
Semantic Errors
Semantic errors occur when syntax is correct, but the program executes incorrectly due to logical mistakes, meaning the program does not do what the programmer intended. These can sometimes be more troublesome than syntax errors since they may only manifest during runtime.
Example of a semantic error:
int multiply(int x, int y) {
return x + y; // Incorrect operation
}
In this case, while the syntax is correct, the logic is flawed; the function intending to multiply integers is incorrectly coded to add them instead.
Runtime Errors
Runtime errors are unexpected problems that occur during program execution, often leading to crashes or unexpected behavior. Common causes include division by zero, improper file access, or dereferencing null pointers.
Example of a runtime error:
int main() {
int* ptr = nullptr;
*ptr = 10; // Dereferencing null pointer
return 0;
}
Here, dereferencing a null pointer results in undefined behavior and leads to a crash, effectively stopping the program.
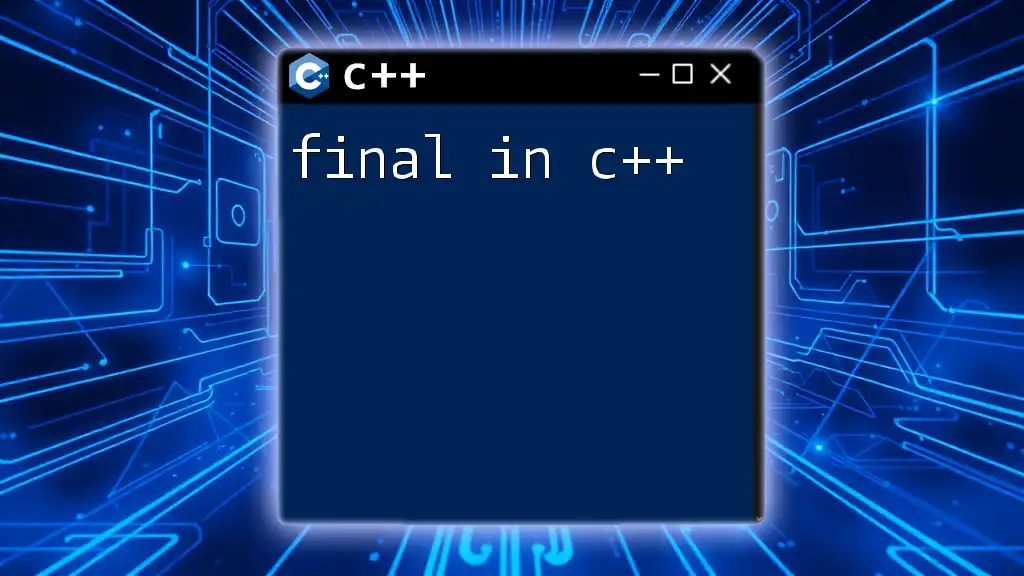
Debugging Techniques for Failures in C++
Using Compiler Warnings
Compiler warnings are essential indicators that can help detect potential errors before runtime. By enabling compiler warnings, programmers can catch subtle mistakes that might not trigger outright compilation errors but could lead to problematic behavior.
For instance, developers using GCC can enable warnings by adding the `-Wall` flag during the compilation process. Observing these warnings allows developers to address issues proactively.
Utilizing Debuggers
Debuggers like gdb (GNU Debugger) play a fundamental role in diagnosing issues in C++ applications. Debuggers allow practitioners to step through their code, inspect variable values, and determine where failures occur in the flow of execution.
Example of using gdb:
gdb ./my_program
This command opens the debugger for `my_program`, allowing you to set breakpoints, examine variable states, and analyze the execution process thoroughly, facilitating the identification of failures.
Print Debugging
Print debugging is a straightforward yet effective technique. By inserting print statements into the code, developers can track variable values and control flow, allowing for a clearer understanding of what is happening at any given moment during execution.
Example with `std::cout`:
std::cout << "Value of x: " << x << std::endl;
This simple statement can reveal the value of `x` at critical points, helping to trace through logical problems.
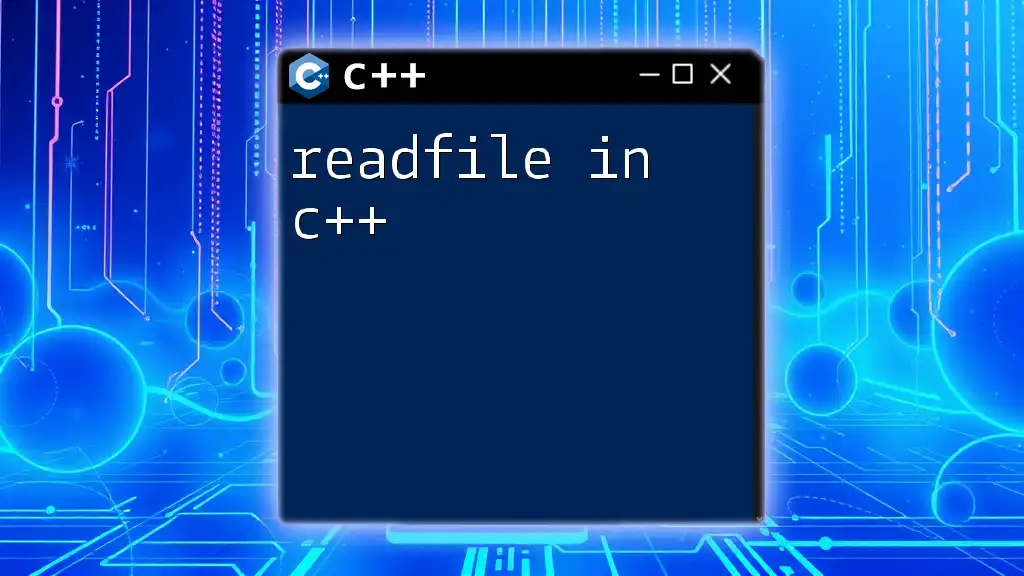
Common Logical Failures in C++
Misunderstanding Control Structures
Control structures such as loops and conditional statements often lead to logical mistakes. Common pitfalls include incorrect loop conditions leading to infinite loops or incorrect handling of edge cases in conditional statements.
Example of an infinite loop:
for (int i = 0; i >= 0; i++) { /* Infinite Loop */ }
In the above example, the loop condition will always evaluate to true, causing an infinite execution that drains resources.
Incorrect Function Usage
Functions can lead to errors if they are not properly understood or applied. Mismatched types or incorrect parameters can lead to unexpected behaviors, especially if the programmer has assumed incorrect types or values.
Example of incorrect function usage:
int add(int a, double b) { return a + b; } // Loss of precision
In this instance, passing a double to a function expecting an integer could lead to potential loss of precision, resulting in unexpected results.
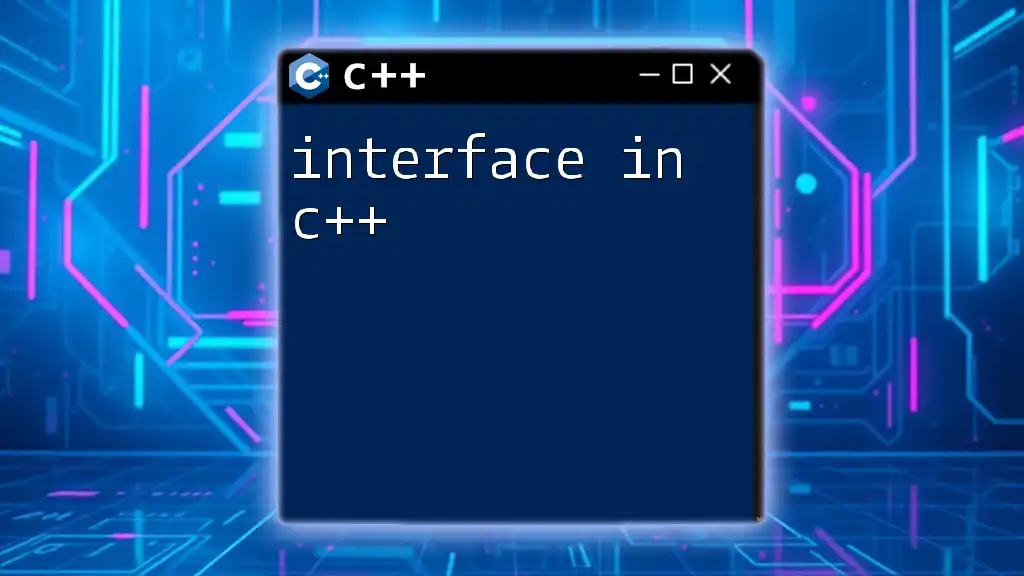
Memory Management Failures
Understanding Memory Leaks
Memory leaks occur when dynamically allocated memory is not properly deallocated. These leaks gradually consume system resources, leading to performance degradation and potential crashes over time.
Example of a memory leak:
int* arr = new int[10];
// Forgetting to delete arr causes memory leak
In this case, the allocated memory for `arr` remains inaccessible without proper deletion, consuming system resources without being utilized.
Segmentation Faults
Segmentation faults often arise from improper memory access, usually due to dereferencing invalid or uninitialized pointers. They signal unintended access to memory areas, leading to program termination.
Example of a segmentation fault:
int* arr = new int[5];
arr[10] = 1; // Out of bounds access
Accessing an array out of bounds yields unpredictable behavior and typically causes a segmentation fault.
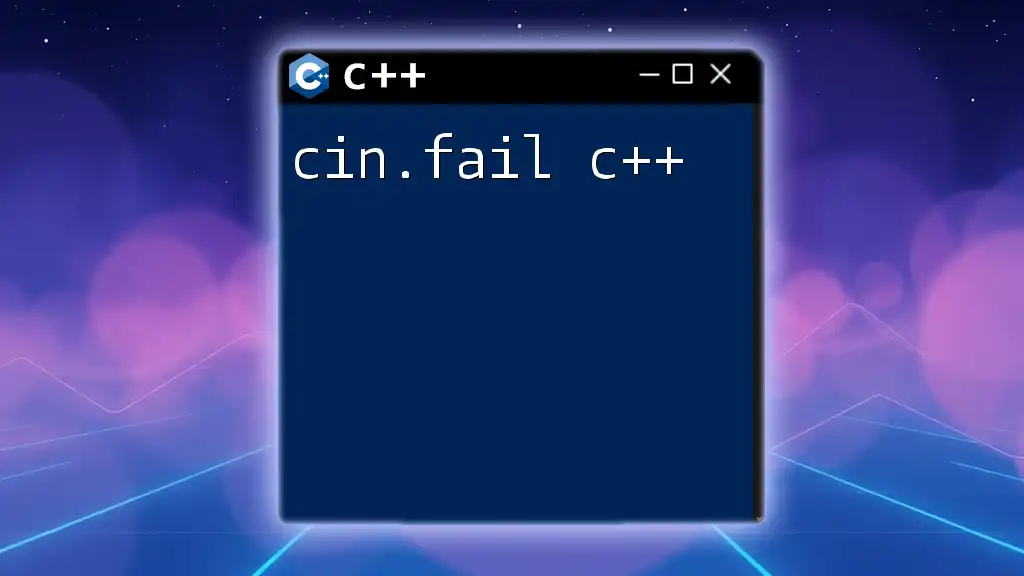
Best Practices to Avoid Failures in C++
Code Reviews and Pair Programming
Engaging in code reviews and pair programming is a powerful way to catch potential issues before code is finalized. Collaboration allows programmers to share knowledge, promote good practices, and approach coding problems from multiple perspectives, significantly enhancing code quality. Feedback from peers can often illuminate areas that an individual developer might overlook.
Writing Unit Tests
Unit tests provide a framework for systematically testing individual components of a program to ensure they function as intended. Implementing unit tests early in the development process can catch failures before they propagate into larger issues.
Example of a simple unit test:
#include <cassert>
void test_add() {
assert(add(2, 3) == 5);
}
Using assertions allows developers to automatically verify that functions return expected results, fostering continuous feedback during the development process.
User Input Validation
Validating user input is critical in any program involving external data. By checking user input before processing, developers can safeguard against invalid data that could lead to runtime errors or undefined behavior.
Example of input validation:
int number;
std::cout << "Enter a number: ";
while (!(std::cin >> number)) {
std::cout << "Invalid input. Please enter a number: ";
std::cin.clear(); // clear the error flag
std::cin.ignore(std::numeric_limits<std::streamsize>::max(), '\n'); // discard invalid input
}
This code snippet safeguards against invalid data entry, enhancing the robustness of user interactions within the program.
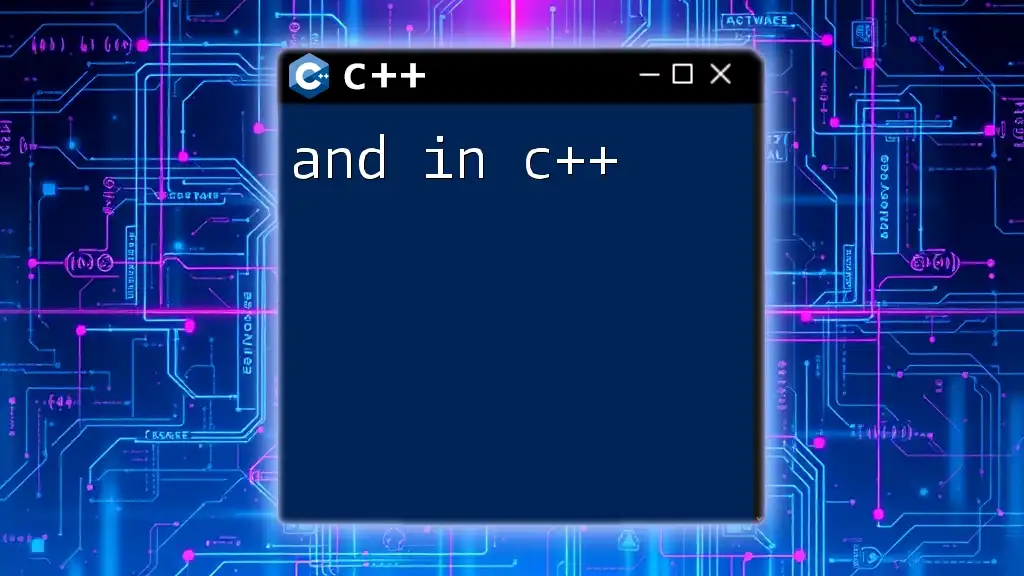
Conclusion
Understanding how to effectively navigate failures in C++ is crucial for both novice and experienced programmers alike. By recognizing various fail types, employing debugging techniques, and adopting best practices, developers can significantly improve their programming skills and productivity. Embrace failure as a vital part of the learning journey, allowing it to inform and enhance your proficiency in C++.