A GUI (Graphical User Interface) in C++ can be created using libraries like Qt or wxWidgets, enabling developers to design interactive applications with visual components.
Here's a basic example using Qt:
#include <QApplication>
#include <QPushButton>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QPushButton button("Hello, World!");
button.resize(200, 100);
button.show();
return app.exec();
}
Understanding GUI Frameworks
What is a GUI Framework?
A GUI framework is a set of tools and libraries designed to help developers create graphical user interfaces. These frameworks provide a structure that facilitates application development by offering pre-built components, event handling systems, and layout management. Unlike GUI libraries, which may contain individual components, frameworks typically provide a holistic environment where all components work together smoothly.
Popular GUI Frameworks for C++
Several GUI frameworks are available for C++ developers, each with its unique features and advantages. Understanding these can help you choose the right tool for your project.
Qt: Qt is perhaps the most versatile and widely used framework for C++. It supports cross-platform development, meaning applications made with Qt can run on various operating systems without substantial changes. The framework offers a rich set of pre-built widgets, along with powerful features such as signals and slots for communication between components.
Getting Started: To set up Qt for the first time, you can download the Qt Creator IDE along with the appropriate Qt libraries. Once installed, you can create a new project using the IDE's straightforward interface.
wxWidgets: Another robust option is wxWidgets, a cross-platform GUI library that mirrors the native look and feel of the operating system. It provides a wide range of widgets and is a great choice for developers looking for native integration.
Installation: To install wxWidgets, follow the instructions provided on their official site, ensuring you link the library properly to your C++ projects.
SFML: If you're focused on multimedia applications, SFML (Simple and Fast Multimedia Library) might be ideal. While it's primarily a multimedia library, it also supports window creation and basic GUI operations and is particularly suited for games and graphics-intensive applications.
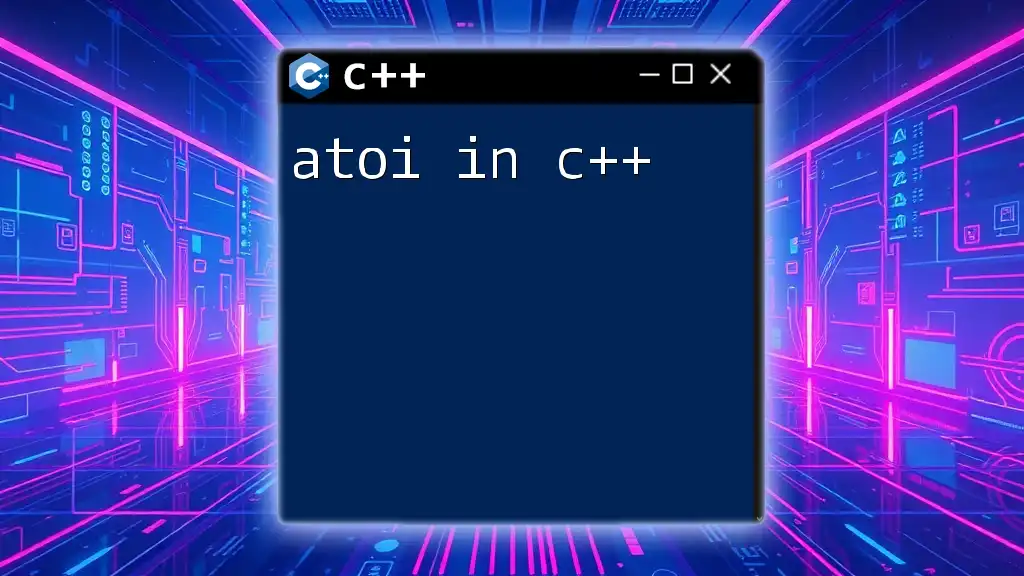
Getting Started with Your First GUI Application
Setting Up the Development Environment
When developing a GUI in C++, your choice of Integrated Development Environment (IDE) can significantly impact your productivity. Popular options include Qt Creator, Code::Blocks, and Visual Studio. Ensure you have installed any necessary libraries and dependencies before embarking on your project.
Creating a Basic Window with Qt
Let’s dive into the coding aspect by creating a basic window using Qt. This foundational step will introduce you to the essentials of building a GUI application.
Here's a simple example that demonstrates how to create a window in Qt:
#include <QApplication>
#include <QWidget>
int main(int argc, char *argv[]) {
QApplication app(argc, argv);
QWidget window;
window.resize(320, 240);
window.setWindowTitle("Simple GUI Example");
window.show();
return app.exec();
}
In this code:
- QApplication initializes the application.
- QWidget is the basic building block of all GUI applications in Qt, representing the window.
- The window’s size is set to 320x240 pixels with `resize()`, and `setWindowTitle()` defines its title.
After writing the code, compile and run it. You will see a simple window pop up, laying the groundwork for your GUI application.
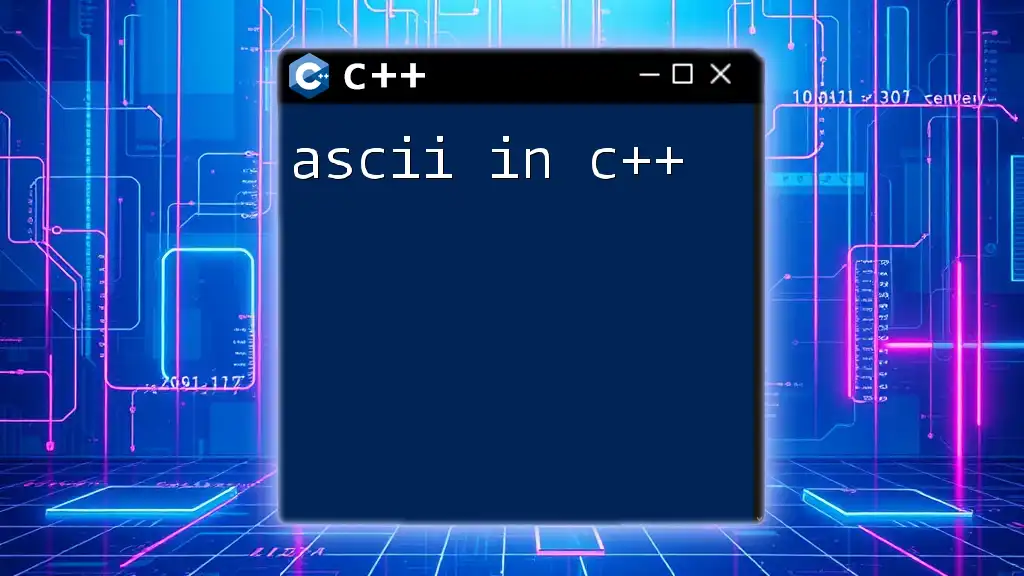
Adding Elements to Your GUI
Common GUI Components
One of the best aspects of GUI frameworks is the ease of adding components. Knowing the most commonly used components and how to integrate them will significantly enhance your application.
- Buttons: Adding buttons is straightforward. In Qt, you can create buttons that trigger actions through the signals and slots mechanism. Here’s a code snippet demonstrating a button’s creation:
QPushButton *button = new QPushButton("Click Me", &window);
QObject::connect(button, &QPushButton::clicked, []() {
qDebug() << "Button was clicked!";
});
In this code, when the button is clicked, it will output a message to the console, showcasing event handling in action.
-
Labels and Text Fields: Labels display static text, while text fields allow user input. In Qt, you can create these components easily using `QLabel` and `QLineEdit`, respectively.
-
Checkboxes and Radio Buttons: Collecting user preferences can be done efficiently with checkboxes and radio buttons. Both components can be linked to slots, which handle their state changes.
Layout Management
Proper layout management is crucial for building aesthetically pleasing and functional GUI applications. Different layout managers (such as Vertical, Horizontal, and Grid) help organize your components intelligently.
Using a layout manager ensures your application looks good on various screen sizes and resolutions. For instance, if you want to arrange buttons vertically, you can use `QVBoxLayout`:
QVBoxLayout *layout = new QVBoxLayout;
layout->addWidget(button);
layout->addWidget(label);
window.setLayout(layout);
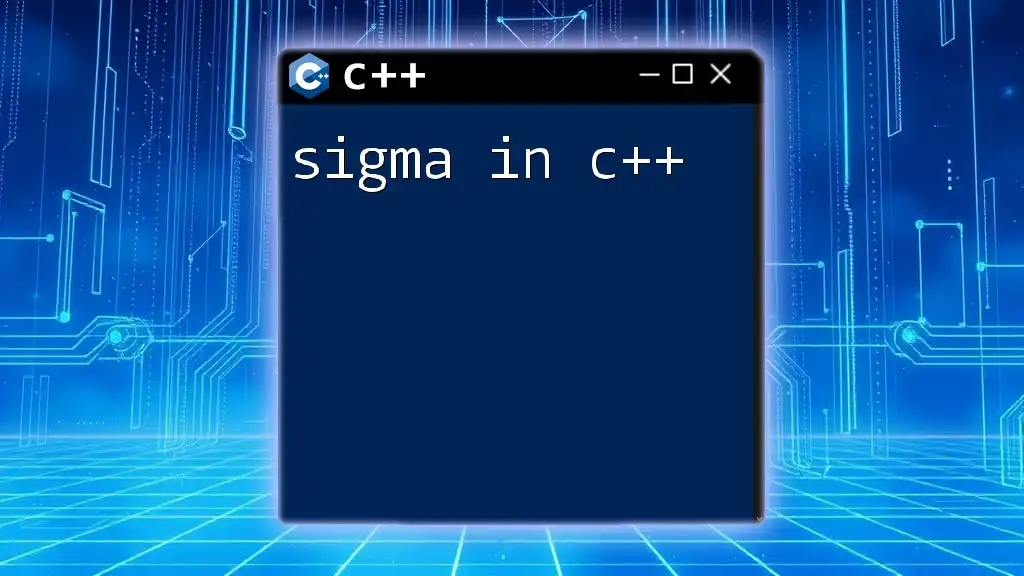
Advanced GUI Features
Event Handling in C++
Event handling is a core aspect of GUI development, allowing applications to respond dynamically to user interactions. In C++, this is primarily achieved through an event-driven architecture.
You can handle events like mouse clicks and keyboard inputs by connecting signals emitted by widgets to appropriate slots that define the expected behavior. This interaction ensures that your GUI remains responsive and user-friendly.
Custom Widgets
Sometimes, standard widgets do not meet your needs. In such cases, you can create custom widgets. Custom widgets allow you to encapsulate functionality and UI components that suit your application’s specific needs.
To create a custom widget, derive your class from `QWidget` or another relevant class and override the `paintEvent()` method to handle custom drawing.
Using Graphics in Your GUI
Incorporating graphics makes your GUI more engaging. The `QPainter` class in Qt serves as an excellent tool for drawing shapes, lines, and text.
Here’s an example of how to use `QPainter` to draw a rectangle in a custom widget:
void MyCustomWidget::paintEvent(QPaintEvent *event) {
QPainter painter(this);
painter.setBrush(Qt::blue);
painter.drawRect(10, 10, 100, 100);
}
This method will draw a blue rectangle at the specified coordinates, enhancing your UI with visual elements.
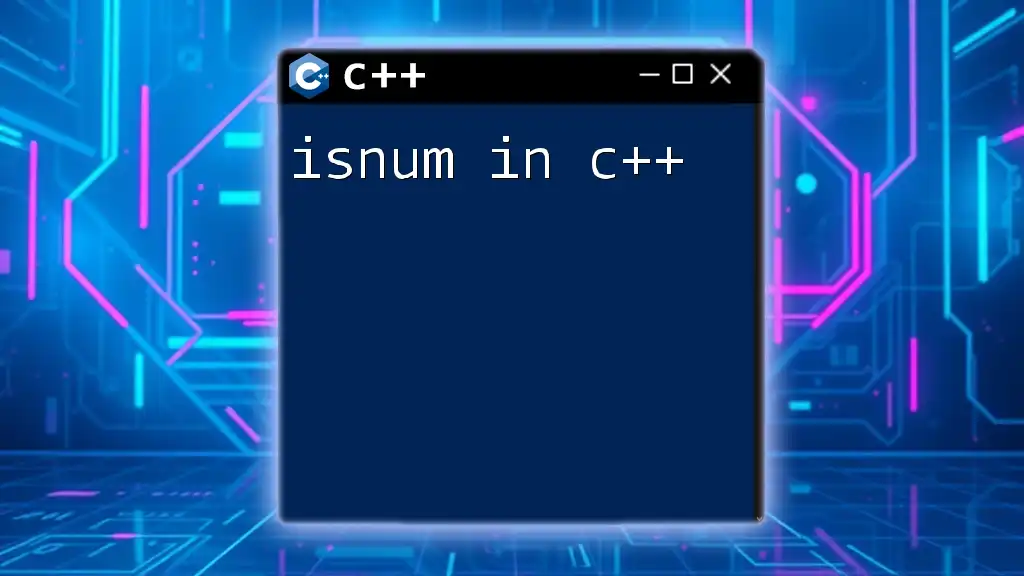
Best Practices for Designing GUIs in C++
User Experience (UX) Considerations
User-centered design should always be at the forefront of your development process. Focus on ensuring that your GUI is intuitive, accessible, and easy to navigate. Conduct user testing to collect feedback and make necessary adjustments to improve the overall experience.
Code Maintenance and Reusability
Writing clean, maintainable code is vital for the longevity of any project. Structured code increases readability and eases collaboration. By creating reusable components, you can save time and reduce redundancy in your projects.
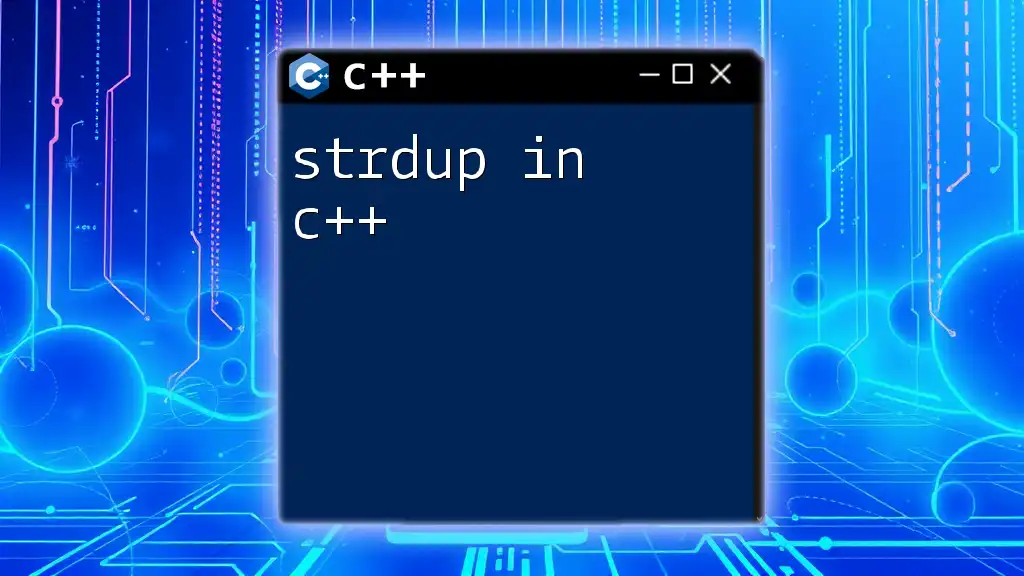
Testing and Debugging GUI Applications
Debugging Techniques
Debugging is an integral part of the development process. Common issues in GUI applications include incorrect layout, unresponsive buttons, and crashes. Utilizing debugging tools can help identify and resolve issues efficiently.
Writing Tests for GUI Applications
Automated testing enhances software reliability. Consider writing unit tests for individual GUI components. Frameworks like Google Test can help you set up integration testing for your C++ applications, ensuring each component functions as expected.
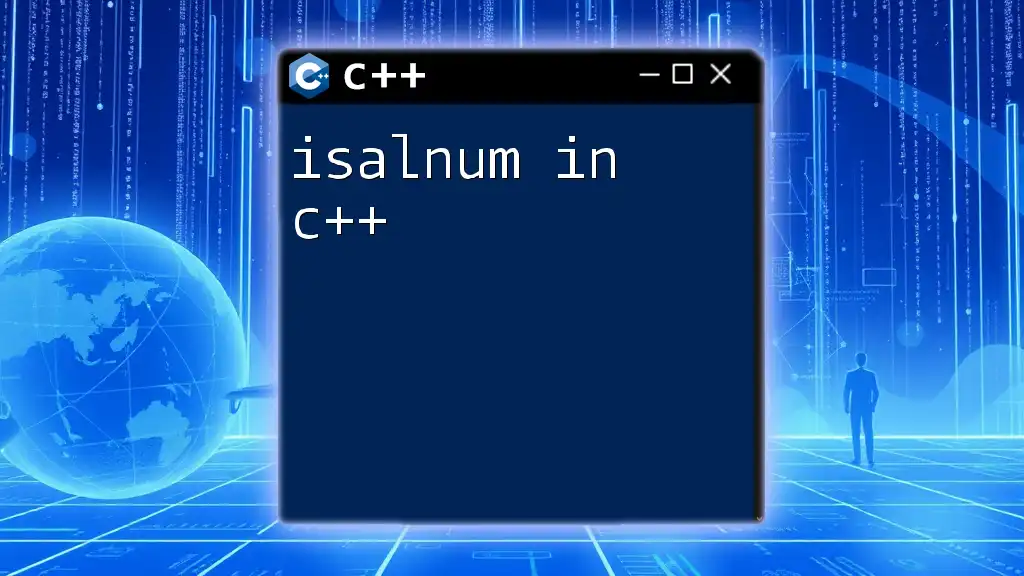
Conclusion
Learning how to create a GUI in C++ opens up new avenues for application development. As you gain proficiency with various frameworks and paradigms, you'll be well-equipped to create intuitive and engaging software. Embrace the journey of GUI design, and explore the endless possibilities it offers.
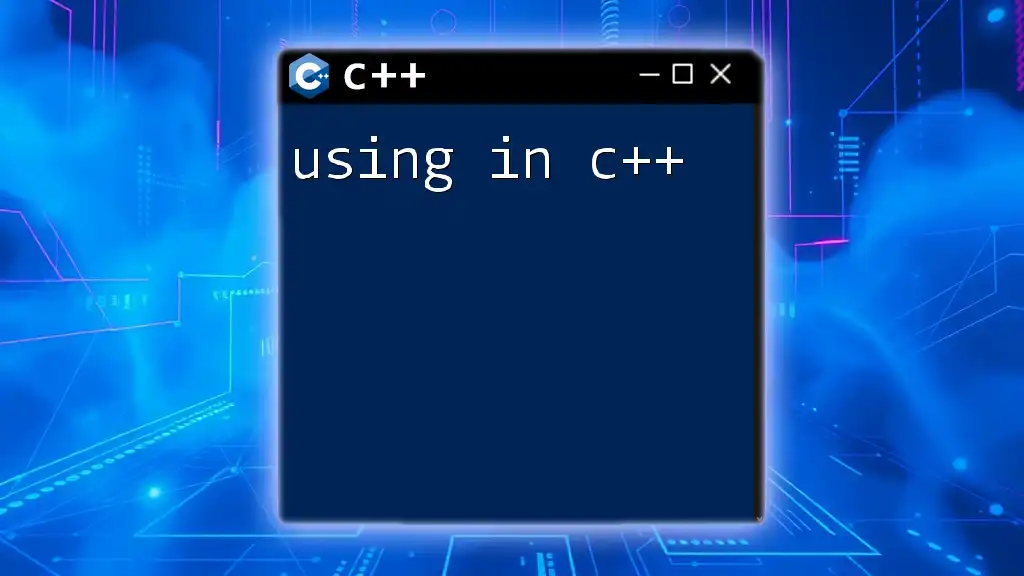
Additional Resources
Recommended Books and Documentation
Consider diving into books such as "C++ GUI Programming with Qt" and the official Qt and wxWidgets documentation for in-depth learning.
Online Communities and Forums
Joining C++ communities and forums can provide support and resources. Engage with peers who share your interest in C++ GUI development to expand your knowledge and skills.