The `equal_range` function in C++ returns a pair of iterators indicating the range of equal elements in a sorted range that matches a specified value.
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 2, 2, 3, 4, 5};
auto range = std::equal_range(vec.begin(), vec.end(), 2);
std::cout << "Range for 2: [" << (range.first - vec.begin()) << ", " << (range.second - vec.begin()) << ")\n";
return 0;
}
What is `equal_range`?
`equal_range` is a powerful function in the C++ Standard Template Library (STL) that helps you efficiently locate a range of equivalent elements in a sorted collection. Unlike other searching algorithms, `equal_range` does not just find a single instance of a value but identifies the entire contiguous sequence of elements that are equal to the specified search value.
In cases where you need to determine the start and end of several equal elements (like finding all occurrences of a specific number in a sorted array), `equal_range` becomes particularly useful. This function works on the principle of binary search, providing an efficient way to perform your search with a time complexity of O(log n).
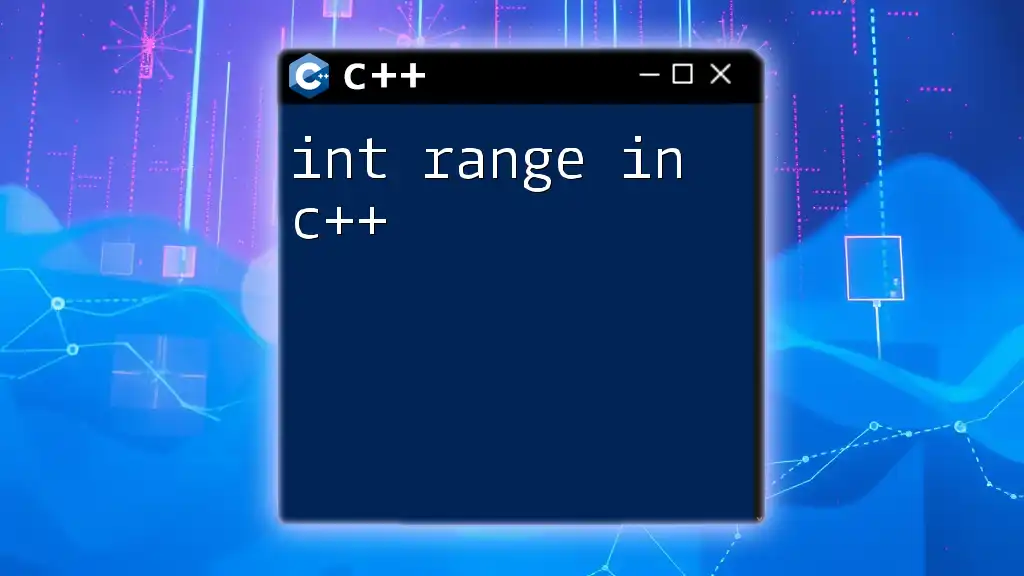
Syntax of `equal_range`
The syntax of `equal_range` is straightforward, making it easy for developers to integrate into their code:
template<class ForwardIt, class T>
std::pair<ForwardIt, ForwardIt> equal_range(ForwardIt first, ForwardIt last, const T& value);
This function takes three parameters:
- ForwardIt first: This is the beginning iterator of the range.
- ForwardIt last: This is the iterator pointing to the end of the range.
- const T& value: This is the value you want to search for in the specified range.
The return value is a pair of iterators representing the range of elements that match the given value. The first iterator points to the first occurrence, and the second iterator points one past the last occurrence.
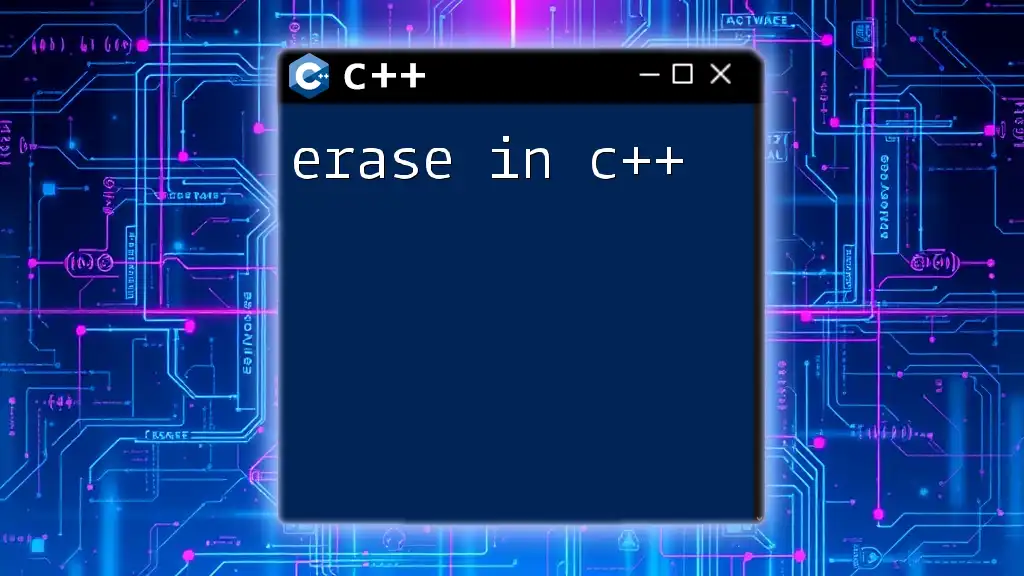
How `equal_range` Works
To understand how `equal_range` works, it’s essential to grasp the concept of binary search. The function scans the range using a divide-and-conquer approach, efficiently finding the smallest and largest elements that match the search criterion.
During the operation, `equal_range` identifies both the starting and ending positions of the desired value, thereby allowing you to work with any number of duplicates. If the value does not exist in the collection, `equal_range` will return iterators pointing to the position where the value could be inserted while preserving order.
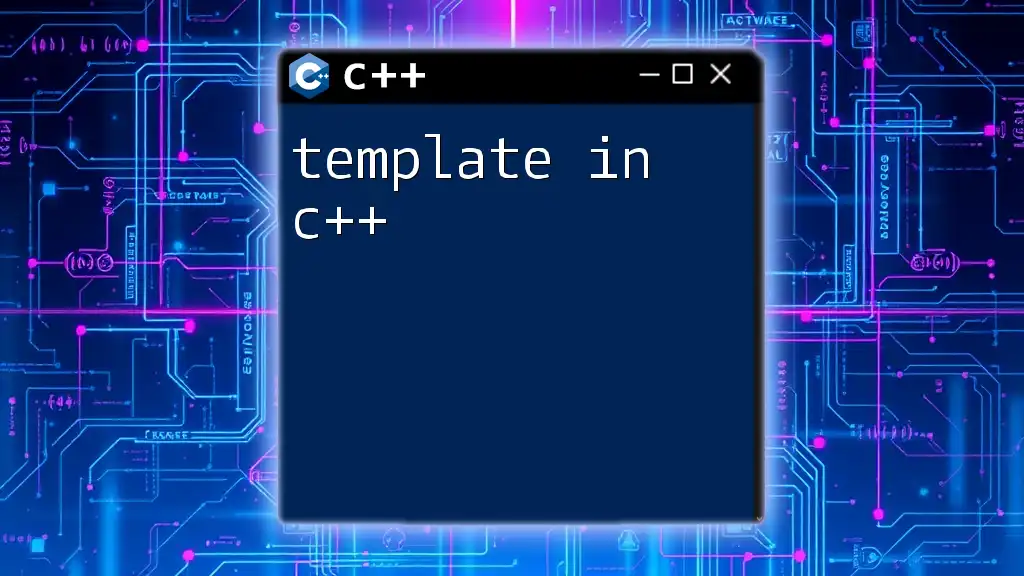
Example Usage of `equal_range`
Here’s a simple example illustrating the basic usage of `equal_range`:
#include <iostream>
#include <vector>
#include <algorithm>
int main() {
std::vector<int> vec = {1, 2, 2, 2, 3, 4};
auto range = std::equal_range(vec.begin(), vec.end(), 2);
std::cout << "The range for value 2 is from index "
<< (range.first - vec.begin()) << " to "
<< (range.second - vec.begin()) << std::endl;
return 0;
}
In this example, we create a sorted vector containing integers. We then call `equal_range` to find all occurrences of the value 2. The output will indicate the indices marking the start and end of this value in the vector. Here, the result would show indices 1 to 4, meaning there are three occurrences of 2 contained between those indices.
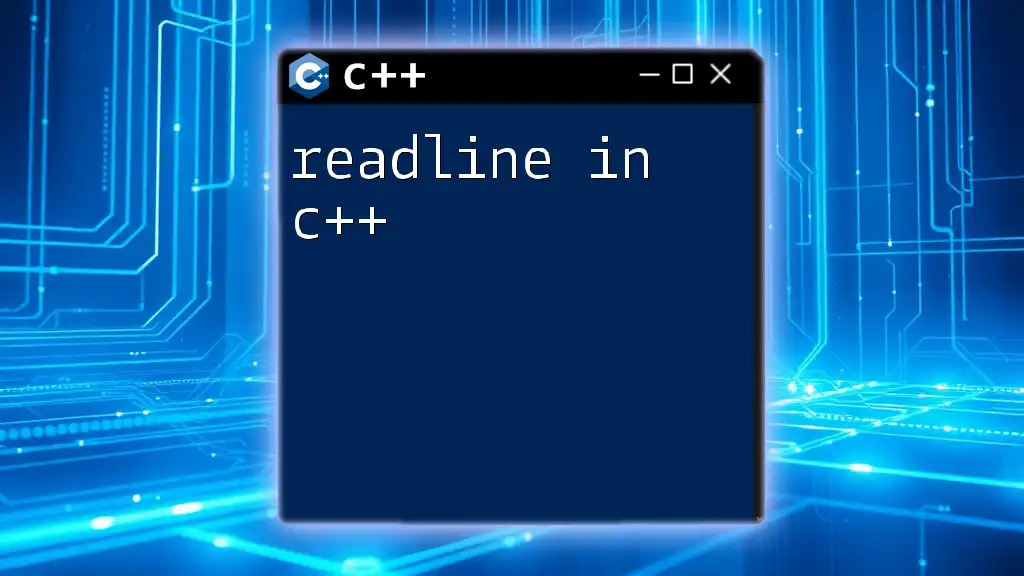
Practical Applications of `equal_range`
`equal_range` proves advantageous in several scenarios, particularly when dealing with sorted datasets. Here are a few practical applications where `equal_range` shines:
-
Searching in Sorted Arrays: If you need to find duplicates efficiently without iterating through the entire array, `equal_range` offers a quick solution.
-
Frequency Counting of Elements: By leveraging `equal_range`, you can determine how frequently an element appears in a sorted collection. Here's how you could implement this:
std::vector<int> elements = {1, 1, 2, 2, 2, 3, 4};
int element_to_count = 2;
// Using equal_range to find the frequency
auto range = std::equal_range(elements.begin(), elements.end(), element_to_count);
int count = range.second - range.first;
std::cout << element_to_count << " appears " << count << " times." << std::endl;
In the example above, `equal_range` identifies the positions of 2 in the vector and allows us to calculate its frequency in constant time after the initial search.
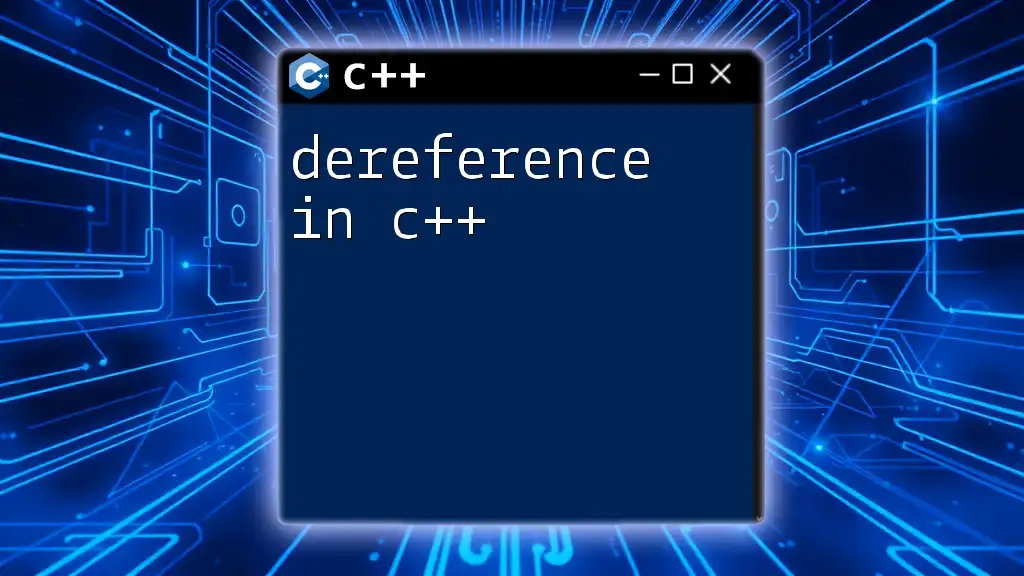
Performance Considerations
The time complexity of `equal_range` is O(log n), which is significantly more efficient than a linear search, especially for large datasets. When compared to other searching techniques like `lower_bound` and `upper_bound`, `equal_range` provides a more comprehensive solution, as it returns both limits of the range in one go.
However, one should always ensure that the input data is sorted before utilizing `equal_range`. If the data is not sorted, the function may yield undefined behavior or incorrect results.
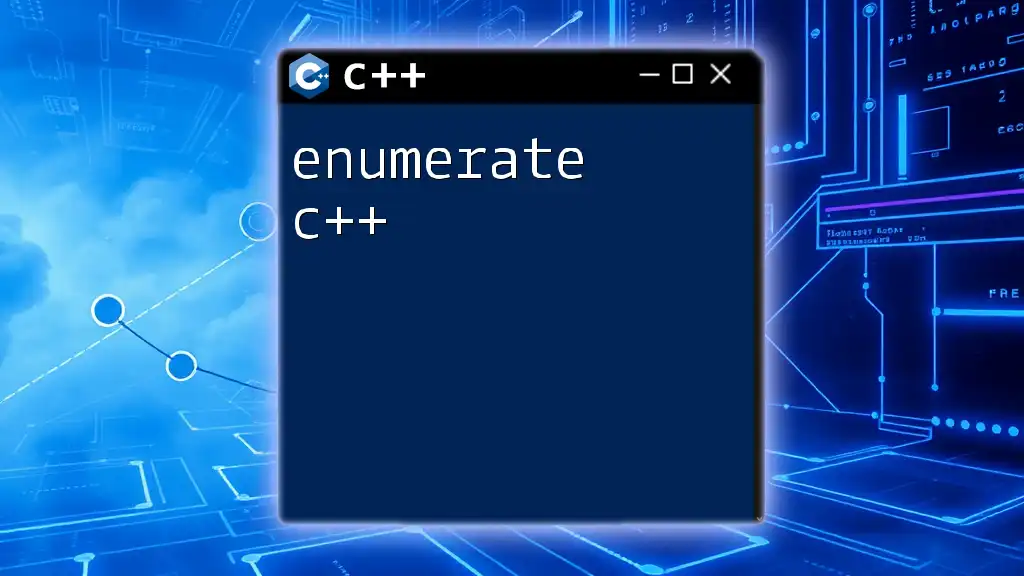
Tips for Using `equal_range`
-
Ensure Sorting: Always guarantee that the data you provide to `equal_range` is sorted. If it isn't, the results will not be reliable.
-
Consider Edge Cases: Be alert for scenarios where the specified value isn’t found. `equal_range` will return iterators pointing to the end of the range, and understanding that balance is crucial for effective coding.
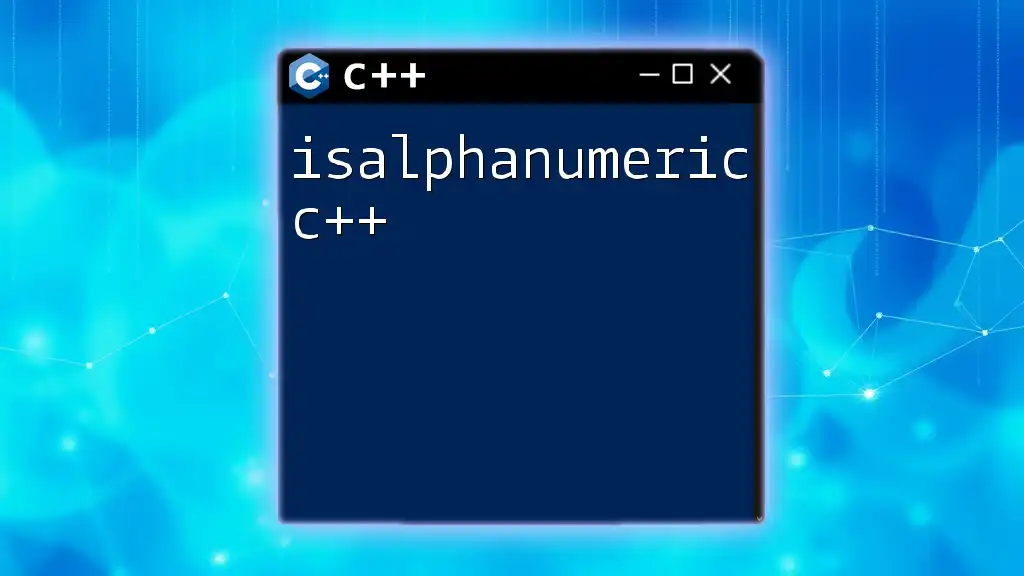
Common Mistakes When Using `equal_range`
-
Missing Headers: Ensure that you include `<algorithm>` alongside any necessary STL headers.
-
Unsorted Ranges: A common error is to use `equal_range` on an unsorted collection. This will lead to inaccurate results.
-
Misinterpreting Output: Many developers may confuse the returned iterators. Remember, the first iterator points to the first occurrence, while the second points just past the last occurrence.
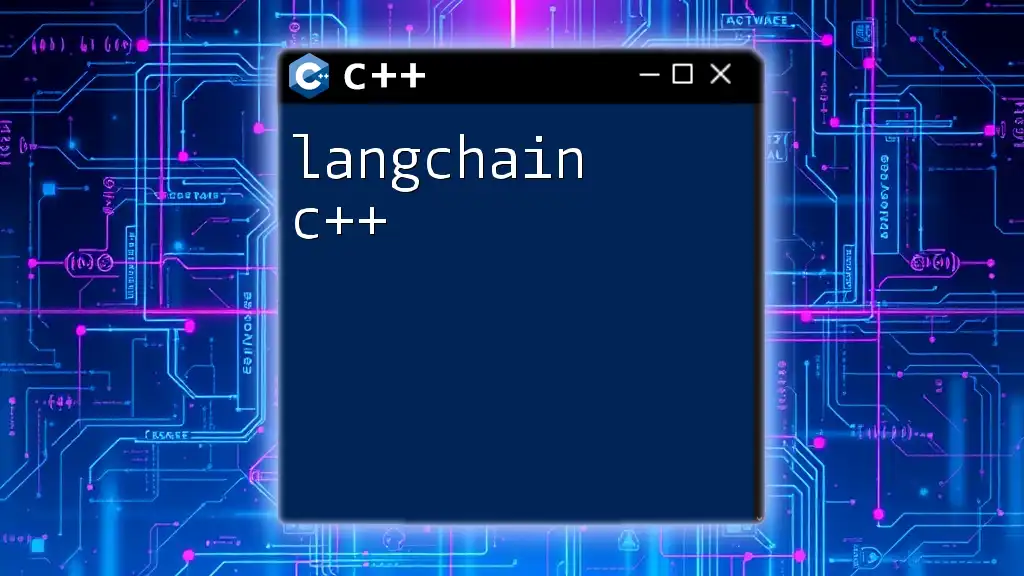
Conclusion
In conclusion, equal_range in C++ is a formidable tool embedded within the STL, allowing for efficient searching and range detection of equivalent elements in sorted sequences. Its utility in developing efficient algorithms cannot be overstated and encourages developers to consider its merits over traditional searching methods. To get the most out of your C++ programming, practicing with `equal_range` and exploring its numerous applications will prove invaluable.
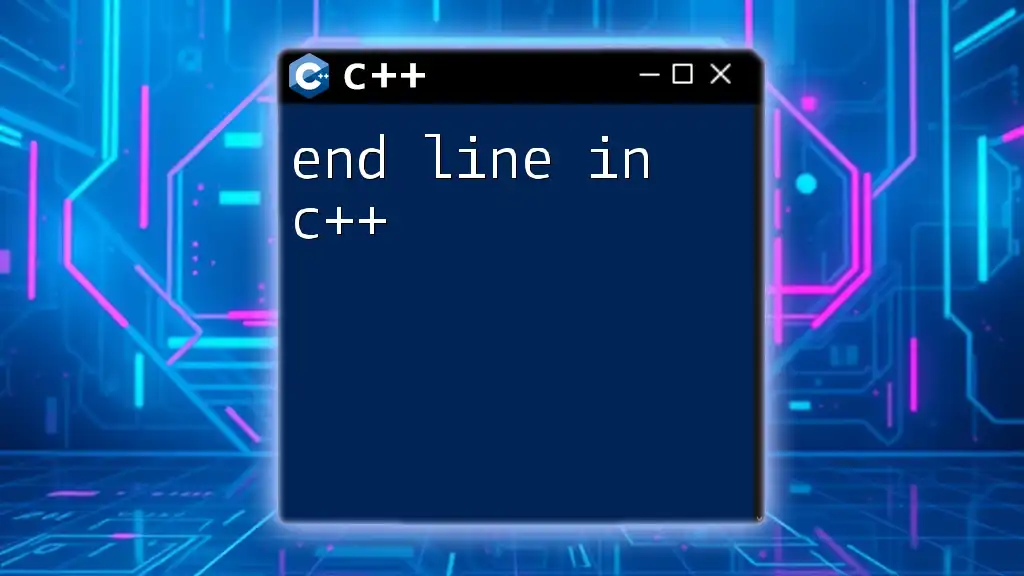
Additional Resources
For further exploration, consider looking into:
- Official C++ documentation on `equal_range`
- Tutorials on searching algorithms in C++
- Practice problems focusing on STL and searching techniques
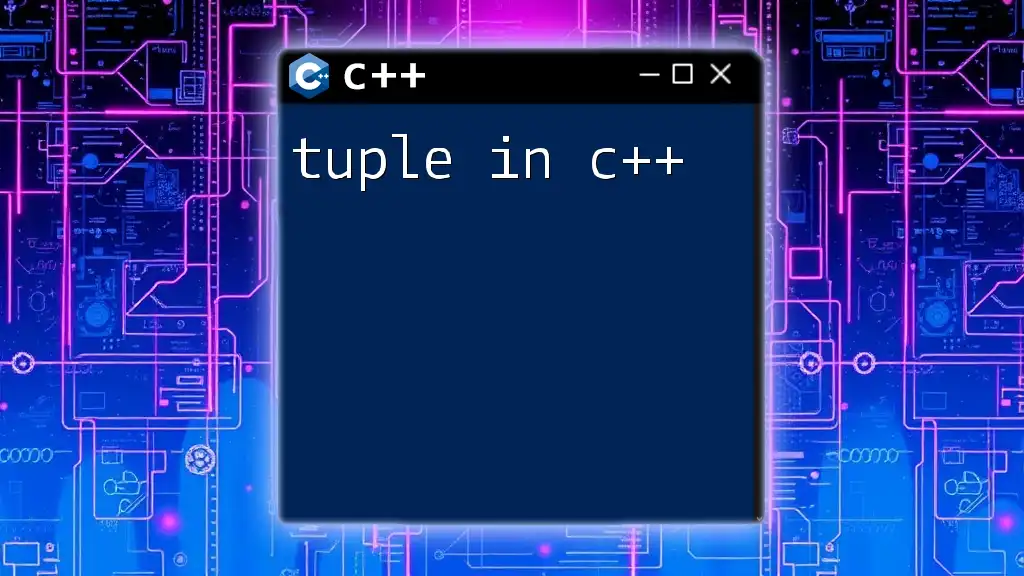
FAQs
What is the difference between `equal_range` and `find`?
`find` locates a single occurrence of a value, while `equal_range` determines a range of all equivalent values.
Can I use `equal_range` on non-sorted data?
No, `equal_range` requires sorted data; using it on unsorted data will yield undefined behavior.
How does `equal_range` handle duplicates?
`equal_range` identifies all contiguous duplicates and returns a range of iterators corresponding to these values.